This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const { | |
compose, | |
concat, | |
curry, | |
flatten, | |
flip, | |
identity, | |
isNil, | |
isEmpty, | |
join, |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const remark = require('remark'); | |
const html = require('remark-html'); | |
const visit = require('unist-util-visit'); | |
const { log } = console; | |
const inputMD = ` | |
[A Link](#){: data-track="footer-link" .xclass onclick=alert() } with text that has *some*{: .emphclass } emphasis and something **strong**{: .strngclss} | |
[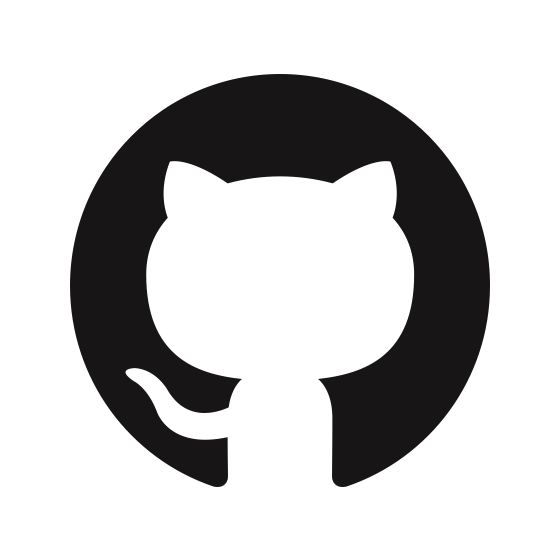{: height=100 width=100 }](/){: .rspnsv-img data-track="logo click" } |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
grep -R --exclude-dir={node_modules,build,.git} --exclude=*.{png,jpg,vim,swp,swo} 'searchString' ./ |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const md = require('markdown-it')(); | |
const markdownItAttrs = require('markdown-it-attrs'); | |
const src = ` | |
# header {: .title-class #title-id } | |
some text {: with=attrs and="attrs with space" } | |
more body copy with some *emphasis*{: .emph-class } thrown in {: .para-class} | |
- item 1 | |
- item 2 {: .item-class } | |
- item 3 |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// utilities | |
const includes = (needle) => (haystack) => (haystack.indexOf(needle) > -1); | |
const includesAtPos = (needle) => (position) => (haystack) => (haystack.indexOf(needle) === position); | |
const testFor = (re) => (str) => re.test(str); | |
const and = (...fnList) => (data) => fnList.every((fn) => fn(data)); | |
const groupBy = (fn) => (arr) => arr.reduce( | |
(out, val) => { | |
let by = fn(val); | |
(out[by] = out[by] || []).push(val); |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const izzer = (str, mod) => (num) => modulo(num, mod) === 0 | |
? str | |
: ''; | |
const izzerStr = compose( | |
join(''), | |
juxt([ | |
izzer('fizz', 3), | |
izzer('buzz', 5), | |
]) |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const toThsnds = compose( | |
multiply(1000), | |
Math.round, | |
multiply(1/1000) | |
); | |
const avgToThsnds = (arr) => compose( | |
toThsnds, | |
multiply(1/length(arr)), | |
reduce(add, 0) |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const selectBrevity= (l, r) => lte(length(l), length(r)) | |
? l | |
: r; | |
const lispy = ([fn, ...args]) => fn(...args); | |
const makeSet = (arr) => uniq(flatten(arr)); | |
const zipObjLisped = (arr) => ({ [head(arr)]: lispy(tail(arr)) }); |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const validVal = compose( | |
not, | |
anyPass([isEmpty, isNil]) | |
); | |
const validProp = curry((propName, obj) => validVal(prop(propName, obj))); | |
const propsOr = curry((fallback, propsList, obj) => compose( | |
cond, | |
append([T, always(fallback)]), |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const isntEmpty = complement(isEmpty); | |
const isntNil = complement(isNil); | |
const isntNaN = complement(isNaN); | |
const pageLens = lensProp('page'); | |
const numberValidations = [ | |
isntNaN, | |
isntNil, | |
isntEmpty, |