Created
May 19, 2016 23:06
-
-
Save keiono/73da21846b6f73de70122bdb545c1c14 to your computer and use it in GitHub Desktop.
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"cells": [ | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"# Introduction to _py2cytoscape_\n", | |
"#### Pythonista-friendly wrapper for cyREST\n", | |
"\n", | |
"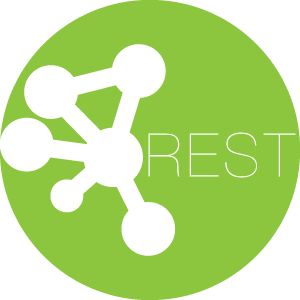\n", | |
"\n", | |
"<h1 align=\"center\">For</h1> \n", | |
"\n", | |
"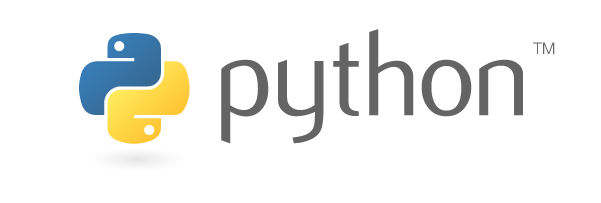\n", | |
"\n", | |
"by [Keiichiro Ono](http://keiono.github.io/) - University of California, San Diego [Trey Ideker Lab](http://healthsciences.ucsd.edu/som/medicine/research/labs/ideker/Pages/default.aspx)\n", | |
"\n", | |
"* TSRI Lecture: Applied Bioinformatics\n", | |
"* 5/12/2016 (Lecture 2)\n", | |
"\n", | |
"## What is _py2cytoscape_?\n", | |
"\n", | |
"py2cytoscape is a Python package to wrap Cytoscape REST API. You can easily access basic Cytoscape functions through simple Python API.\n", | |
"\n", | |
"\n", | |
"## System Requirments\n", | |
"* Java 8\n", | |
"* Cytoscape 3.3.0+\n", | |
"* py2cytoscape 0.5.0+" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"# Quick Overview of py2cytoscape\n", | |
"\n", | |
"**To run this script, you need to start Cytoscape before executing the following cells!** " | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 1, | |
"metadata": { | |
"collapsed": false | |
}, | |
"outputs": [ | |
{ | |
"data": { | |
"application/javascript": [ | |
"if (window['cytoscape'] === undefined) {\n", | |
" var paths = {\n", | |
" cytoscape: 'http://cytoscape.github.io/cytoscape.js/api/cytoscape.js-latest/cytoscape.min'\n", | |
" };\n", | |
"\n", | |
" require.config({\n", | |
" paths: paths\n", | |
" });\n", | |
"\n", | |
" require(['cytoscape'], function (cytoscape) {\n", | |
" console.log('Loading Cytoscape.js Module...');\n", | |
" window['cytoscape'] = cytoscape;\n", | |
"\n", | |
" var event = document.createEvent(\"HTMLEvents\");\n", | |
" event.initEvent(\"load_cytoscape\", true, false);\n", | |
" window.dispatchEvent(event);\n", | |
" });\n", | |
"}" | |
], | |
"text/plain": [ | |
"<IPython.core.display.Javascript object>" | |
] | |
}, | |
"metadata": {}, | |
"output_type": "display_data" | |
} | |
], | |
"source": [ | |
"# Loading dependencies\n", | |
"from py2cytoscape.data.cynetwork import CyNetwork\n", | |
"from py2cytoscape.data.cyrest_client import CyRestClient\n", | |
"from py2cytoscape.data.style import StyleUtil\n", | |
"import py2cytoscape.util.cytoscapejs as cyjs\n", | |
"import py2cytoscape.cytoscapejs as renderer\n", | |
"\n", | |
"import networkx as nx\n", | |
"import pandas as pd\n", | |
"import json" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"### Creating Cytoscape network from file" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 2, | |
"metadata": { | |
"collapsed": true | |
}, | |
"outputs": [], | |
"source": [ | |
"# Step 1: Create py2cytoscape client\n", | |
"cy = CyRestClient()\n", | |
"\n", | |
"# Reset (delete all existing networks)\n", | |
"cy.session.delete()\n", | |
"\n", | |
"# Step 2: Load network from somewhere\n", | |
"yeast_net = cy.network.create_from('https://raw.githubusercontent.com/idekerlab/tsri-lecture/master/lecture2/data/galFiltered.json')" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 3, | |
"metadata": { | |
"collapsed": false | |
}, | |
"outputs": [], | |
"source": [ | |
"# Step 3: Apply layout\n", | |
"cy.layout.apply(name='force-directed', network=yeast_net)\n", | |
"\n", | |
"# Step 4: Create Visual Style as code (or by hand if you prefer)\n", | |
"my_yeast_style = cy.style.create('GAL Style')\n", | |
"\n", | |
"\n", | |
"basic_settings = {\n", | |
" \n", | |
" # You can set default values as key-value pairs.\n", | |
" \n", | |
" 'NODE_FILL_COLOR': '#6AACB8',\n", | |
" 'NODE_SIZE': 55,\n", | |
" 'NODE_BORDER_WIDTH': 0,\n", | |
" 'NODE_LABEL_COLOR': '#555555',\n", | |
" \n", | |
" 'EDGE_WIDTH': 2,\n", | |
" 'EDGE_TRANSPARENCY': 100,\n", | |
" 'EDGE_STROKE_UNSELECTED_PAINT': '#333333'\n", | |
"}\n", | |
"\n", | |
"my_yeast_style.update_defaults(basic_settings)\n", | |
"\n", | |
"# Create some mappings\n", | |
"my_yeast_style.create_passthrough_mapping(column='COMMON', vp='NODE_LABEL', col_type='String')\n", | |
"\n", | |
"degrees = yeast_net.get_node_column('Degree') \n", | |
"color_gradient = StyleUtil.create_2_color_gradient(min=degrees.min(), max=degrees.max(), colors=('white', '#6AACB8'))\n", | |
"degree_to_size = StyleUtil.create_slope(min=degrees.min(), max=degrees.max(), values=(10, 100))\n", | |
"my_yeast_style.create_continuous_mapping(column='Degree', vp='NODE_FILL_COLOR', col_type='Integer', points=color_gradient)\n", | |
"my_yeast_style.create_continuous_mapping(column='Degree', vp='NODE_HEIGHT', col_type='Integer', points=degree_to_size)\n", | |
"my_yeast_style.create_continuous_mapping(column='Degree', vp='NODE_WIDTH', col_type='Integer', points=degree_to_size)\n", | |
"\n", | |
"my_yeast_style.create_continuous_mapping(column='Degree', vp='NODE_LABEL_FONT_SIZE', col_type='Integer', points=degree_to_size)\n", | |
"\n", | |
"cy.style.apply(my_yeast_style, yeast_net)" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 4, | |
"metadata": { | |
"collapsed": false | |
}, | |
"outputs": [ | |
{ | |
"data": { | |
"text/html": [ | |
"<!DOCTYPE html>\n", | |
"<html>\n", | |
"<head>\n", | |
" <meta charset=utf-8 />\n", | |
" <style type=\"text/css\">\n", | |
" body {\n", | |
" font: 14px helvetica neue, helvetica, arial, sans-serif;\n", | |
" }\n", | |
"\n", | |
" #cya6312872-861b-4b6a-82a2-b83352c19254 {\n", | |
" height: 700px;\n", | |
" width: 1098px;\n", | |
" position: absolute;\n", | |
" left: 4px;\n", | |
" top: 5px;\n", | |
" background: radial-gradient(#FFFFFF 15%, #DDDDDD 105%);\n", | |
" }\n", | |
" </style>\n", | |
"\n", | |
" <script>\n", | |
" (function() {\n", | |
" function render() {\n", | |
" $('#cya6312872-861b-4b6a-82a2-b83352c19254').cytoscape({\n", | |
" elements: {\n", | |
" nodes: [{\"position\": {\"y\": 2119.3565077825447, \"x\": 1375.117362815161}, \"selected\": false, \"data\": {\"id_original\": \"1869\", \"name\": \"YDL194W\", \"Eccentricity\": 26, \"IsSingleNode\": false, \"COMMON\": \"SNF3\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.018043, \"annotation_GO_CELLULAR_COMPONENT\": [\"plasma membrane\"], \"alias\": [\"S000002353\", \"SNF3\", \"glucose sensor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"glucose binding\", \"glucose transporter activity\", \"receptor activity\"], \"gal4RGsig\": 0.033961, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDL194W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.07623732, \"AverageShortestPathLength\": 13.11693548, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.449, \"gal1RGexp\": 0.139, \"gal80Rsig\": 0.011348, \"Stress\": 0.0, \"id\": \"1806\", \"gal4RGexp\": 0.333, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"response to glucose stimulus\", \"signal transduction\"], \"Radiality\": 0.55122461, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1806}}, {\"position\": {\"y\": 1990.1930556341072, \"x\": 1408.8522871315672}, \"selected\": false, \"data\": {\"id_original\": \"1870\", \"name\": \"YDR277C\", \"Eccentricity\": 25, \"IsSingleNode\": false, \"COMMON\": \"MTH1\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 2.186e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cellular_component\"], \"alias\": [\"BPC1\", \"DGT1\", \"HTR1\", \"MTH1\", \"S000002685\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.028044, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YDR277C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08250166, \"AverageShortestPathLength\": 12.12096774, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": 0.448, \"gal1RGexp\": 0.243, \"gal80Rsig\": 0.0005727, \"Stress\": 2722.0, \"id\": \"1805\", \"gal4RGexp\": 0.192, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glucose transport\", \"signal transduction\"], \"Radiality\": 0.58811231, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1805}}, {\"position\": {\"y\": 4368.24196386775, \"x\": 1382.2365797341063}, \"selected\": false, \"data\": {\"id_original\": \"1871\", \"name\": \"YBR043C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"YBR043C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 5.373e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"integral to plasma membrane\", \"plasma membrane\"], \"alias\": [\"AQR2\", \"QDR3\", \"S000000247\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"multidrug efflux pump activity\"], \"gal4RGsig\": 0.94178, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YBR043C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.0, \"gal1RGexp\": 0.454, \"gal80Rsig\": 0.999999, \"Stress\": 0.0, \"id\": \"1804\", \"gal4RGexp\": 0.023, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"multidrug transport\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1804}}, {\"position\": {\"y\": 1540.499210362043, \"x\": 3369.569755393286}, \"selected\": false, \"data\": {\"id_original\": \"1872\", \"name\": \"YPR145W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"ASN1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 3.174e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"ASN1\", \"S000006349\", \"asparagine synthetase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"asparagine synthase (glutamine-hydrolyzing) activity\"], \"gal4RGsig\": 1.1525e-07, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPR145W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10205761, \"AverageShortestPathLength\": 9.7983871, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.232, \"gal1RGexp\": -0.195, \"gal80Rsig\": 0.0011873, \"Stress\": 0.0, \"id\": \"1803\", \"gal4RGexp\": -0.614, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"asparagine biosynthetic process\"], \"Radiality\": 0.67413381, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1803}}, {\"position\": {\"y\": 2682.230348114576, \"x\": 2301.087852317114}, \"selected\": false, \"data\": {\"id_original\": \"1873\", \"name\": \"YER054C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"GIP2\", \"selected\": false, \"BetweennessCentrality\": 0.04427321, \"gal1RGsig\": 0.16958, \"annotation_GO_CELLULAR_COMPONENT\": [\"protein phosphatase type 1 complex\"], \"alias\": [\"GIP2\", \"S000000856\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein phosphatase regulator activity\"], \"gal4RGsig\": 0.00062032, \"TopologicalCoefficient\": 0.58333333, \"shared_name\": \"YER054C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11339735, \"AverageShortestPathLength\": 8.81854839, \"NeighborhoodConnectivity\": 4.5, \"gal80Rexp\": 0.247, \"gal1RGexp\": 0.057, \"gal80Rsig\": 0.0043603, \"Stress\": 11544.0, \"id\": \"1802\", \"gal4RGexp\": 0.206, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glycogen metabolic process\", \"protein amino acid dephosphorylation\"], \"Radiality\": 0.71042413, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1802}}, {\"position\": {\"y\": 2738.890016083326, \"x\": 2445.081230002661}, \"selected\": false, \"data\": {\"id_original\": \"1874\", \"name\": \"YBR045C\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"GIP1\", \"selected\": false, \"BetweennessCentrality\": 0.08528144, \"gal1RGsig\": 5.5911e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"prospore membrane\", \"protein phosphatase type 1 complex\"], \"alias\": [\"GIP1\", \"Glc7p regulatory subunit\", \"S000000249\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein phosphatase 1 binding\", \"protein phosphatase type 1 regulator activity\"], \"gal4RGsig\": 1.2945e-05, \"TopologicalCoefficient\": 0.44444444, \"shared_name\": \"YBR045C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10367893, \"AverageShortestPathLength\": 9.64516129, \"NeighborhoodConnectivity\": 2.33333333, \"gal80Rexp\": 0.94, \"gal1RGexp\": 0.786, \"gal80Rsig\": 0.016389, \"Stress\": 21474.0, \"id\": \"1801\", \"gal4RGexp\": 1.022, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of phosphoprotein phosphatase activity\", \"spore wall assembly (sensu the Fungi research community)\"], \"Radiality\": 0.67980884, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1801}}, {\"position\": {\"y\": 3777.1561293645764, \"x\": 1059.283508139868}, \"selected\": false, \"data\": {\"id_original\": \"1875\", \"name\": \"YBL079W\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"NUP170\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00025668, \"annotation_GO_CELLULAR_COMPONENT\": [\"nuclear pore\"], \"alias\": [\"NLE3\", \"NUP170\", \"S000000175\", \"nuclear pore complex subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural molecule activity\"], \"gal4RGsig\": 0.45723, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YBL079W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.5, \"AverageShortestPathLength\": 2.0, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.42, \"gal1RGexp\": -0.186, \"gal80Rsig\": 2.9469e-09, \"Stress\": 0.0, \"id\": \"1800\", \"gal4RGexp\": -0.032, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"NLS-bearing substrate import into nucleus\", \"chromosome segregation\", \"mRNA export from nucleus\", \"mRNA-binding (hnRNP) protein import into nucleus\", \"nuclear pore organization and biogenesis\", \"protein export from nucleus\", \"protein import into nucleus\", \"docking\", \"rRNA export from nucleus\", \"ribosomal protein import into nucleus\", \"snRNA export from nucleus\", \"snRNP protein import into nucleus\", \"tRNA export from nucleus\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1800}}, {\"position\": {\"y\": 2567.582154755201, \"x\": 1710.5166242897703}, \"selected\": false, \"data\": {\"id_original\": \"1876\", \"name\": \"YLR345W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"YLR345W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.012373, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"S000004337\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"6-phosphofructo-2-kinase activity\"], \"gal4RGsig\": 7.892e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLR345W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10968598, \"AverageShortestPathLength\": 9.11693548, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.116, \"gal1RGexp\": 0.108, \"gal80Rsig\": 0.073789, \"Stress\": 0.0, \"id\": \"1799\", \"gal4RGexp\": 0.234, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"fructose 2\", \"6-bisphosphate metabolic process\", \"regulation of glycolysis\"], \"Radiality\": 0.69937276, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1799}}, {\"position\": {\"y\": 528.2944960637947, \"x\": 1935.7426679909422}, \"selected\": false, \"data\": {\"id_original\": \"1877\", \"name\": \"YIL052C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"RPL34B\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 3.7855e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPL34B\", \"S000001314\", \"ribosomal protein L34B\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 3.4652e-07, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YIL052C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10929925, \"AverageShortestPathLength\": 9.14919355, \"NeighborhoodConnectivity\": 8.0, \"gal80Rexp\": -0.025, \"gal1RGexp\": -0.258, \"gal80Rsig\": 0.66627, \"Stress\": 0.0, \"id\": \"1798\", \"gal4RGexp\": -0.451, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"telomere maintenance\"], \"Radiality\": 0.69817802, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1798}}, {\"position\": {\"y\": 572.2568984075447, \"x\": 1888.4369123757078}, \"selected\": false, \"data\": {\"id_original\": \"1878\", \"name\": \"YER056CA\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"shared_name\": \"YER056CA\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10929925, \"AverageShortestPathLength\": 9.14919355, \"NeighborhoodConnectivity\": 8.0, \"Stress\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"Radiality\": 0.69817802, \"ClusteringCoefficient\": 0.0, \"id\": \"1797\", \"TopologicalCoefficient\": 0.0, \"Degree\": 1, \"SUID\": 1797}}, {\"position\": {\"y\": 533.0689711614509, \"x\": 2005.1980055153563}, \"selected\": false, \"data\": {\"id_original\": \"1879\", \"name\": \"YNL069C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"RPL16B\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 2.6481e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RP23\", \"RPL16B\", \"S000005013\", \"ribosomal protein L16B (L21B) (rp23) (YL15)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\", \"structural constituent of ribosome\"], \"gal4RGsig\": 5.1994e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YNL069C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10929925, \"AverageShortestPathLength\": 9.14919355, \"NeighborhoodConnectivity\": 8.0, \"gal80Rexp\": -0.124, \"gal1RGexp\": -0.346, \"gal80Rsig\": 0.026888, \"Stress\": 0.0, \"id\": \"1796\", \"gal4RGexp\": -0.284, \"Radiality\": 0.69817802, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1796}}, {\"position\": {\"y\": 569.6334242864509, \"x\": 2074.058513480444}, \"selected\": false, \"data\": {\"id_original\": \"1880\", \"name\": \"YDL075W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"RPL31A\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0041386, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPL31A\", \"RPL34\", \"S000002233\", \"ribosomal protein L31A (L34A) (YL28)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 1.5697e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDL075W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10929925, \"AverageShortestPathLength\": 9.14919355, \"NeighborhoodConnectivity\": 8.0, \"gal80Rexp\": 0.056, \"gal1RGexp\": -0.218, \"gal80Rsig\": 0.38208, \"Stress\": 0.0, \"id\": \"1795\", \"gal4RGexp\": -0.451, \"Radiality\": 0.69817802, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1795}}, {\"position\": {\"y\": 3551.9658217473884, \"x\": 1669.880000907202}, \"selected\": false, \"data\": {\"id_original\": \"1881\", \"name\": \"YFR014C\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"CMK1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.1443e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"CMK1\", \"S000001910\", \"calmodulin-dependent protein kinase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"calmodulin-dependent protein kinase I activity\"], \"gal4RGsig\": 8.1743e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YFR014C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.5, \"AverageShortestPathLength\": 2.0, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.047, \"gal1RGexp\": 0.229, \"gal80Rsig\": 0.64057, \"Stress\": 0.0, \"id\": \"1794\", \"gal4RGexp\": 0.304, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein amino acid phosphorylation\", \"signal transduction\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1794}}, {\"position\": {\"y\": 2836.8657240911384, \"x\": 1473.7385786354735}, \"selected\": false, \"data\": {\"id_original\": \"1882\", \"name\": \"YGR136W\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"YGR136W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00024958, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"LSB1\", \"S000003368\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.0042386, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGR136W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09034608, \"AverageShortestPathLength\": 11.06854839, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.204, \"gal1RGexp\": -0.167, \"gal80Rsig\": 0.0020376, \"Stress\": 0.0, \"id\": \"1793\", \"gal4RGexp\": -0.163, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.6270908, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1793}}, {\"position\": {\"y\": 1536.0337200208564, \"x\": 1809.3318403542235}, \"selected\": false, \"data\": {\"id_original\": \"1883\", \"name\": \"YDL023C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"YDL023C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 4.5602e-11, \"gal4RGsig\": 1.8858e-05, \"NumberOfDirectedEdges\": 1.0, \"shared_name\": \"YDL023C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12776919, \"AverageShortestPathLength\": 7.8266129, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.406, \"gal1RGexp\": -0.712, \"gal80Rsig\": 1.7119e-08, \"Stress\": 0.0, \"id\": \"1792\", \"gal4RGexp\": 0.497, \"Radiality\": 0.74716249, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"TopologicalCoefficient\": 0.0, \"Degree\": 1, \"SUID\": 1792}}, {\"position\": {\"y\": 4303.611978058424, \"x\": 1137.1704328835203}, \"selected\": false, \"data\": {\"id_original\": \"1884\", \"name\": \"YBR170C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"NPL4\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0078298, \"annotation_GO_CELLULAR_COMPONENT\": [\"endoplasmic reticulum\", \"nuclear envelope-endoplasmic reticulum network\"], \"alias\": [\"HRD4\", \"NPL4\", \"S000000374\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.13454, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YBR170C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.429, \"gal1RGexp\": 0.128, \"gal80Rsig\": 0.0016184, \"Stress\": 0.0, \"id\": \"1791\", \"gal4RGexp\": -0.134, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ER-associated protein catabolic process\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1791}}, {\"position\": {\"y\": 4290.826894287672, \"x\": 1307.1501234352781}, \"selected\": false, \"data\": {\"id_original\": \"1885\", \"name\": \"YGR074W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"SMD1\", \"selected\": false, \"BetweennessCentrality\": 1.0, \"gal1RGsig\": 0.11939, \"annotation_GO_CELLULAR_COMPONENT\": [\"U4/U6 x U5 tri-snRNP complex\", \"commitment complex\", \"snRNP U1\", \"snRNP U5\"], \"alias\": [\"S000003306\", \"SMD1\", \"SPP92\", \"Sm D1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA splicing factor activity\", \"transesterification mechanism\", \"mRNA binding\"], \"gal4RGsig\": 0.08221, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGR074W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.029, \"gal1RGexp\": -0.074, \"gal80Rsig\": 0.80755, \"Stress\": 2.0, \"id\": \"1790\", \"gal4RGexp\": -0.133, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nuclear mRNA splicing\", \"via spliceosome\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1790}}, {\"position\": {\"y\": 4207.79356909236, \"x\": 1237.1704328835203}, \"selected\": false, \"data\": {\"id_original\": \"1886\", \"name\": \"YGL202W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"ARO8\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 4.2677e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"ARO8\", \"S000003170\", \"aromatic amino acid aminotransferase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"aromatic-amino-acid transaminase activity\"], \"gal4RGsig\": 4.2373e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGL202W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.536, \"gal1RGexp\": -0.305, \"gal80Rsig\": 3.8938e-13, \"Stress\": 0.0, \"id\": \"1789\", \"gal4RGexp\": -0.286, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"aromatic amino acid family metabolic process\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1789}}, {\"position\": {\"y\": 3881.252198700514, \"x\": 1676.8990171730711}, \"selected\": false, \"data\": {\"id_original\": \"1887\", \"name\": \"YLR197W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"SIK1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.70174, \"annotation_GO_CELLULAR_COMPONENT\": [\"box C/D snoRNP complex\", \"nucleolus\", \"nucleus\", \"small nucleolar ribonucleoprotein complex\"], \"alias\": [\"NOP56\", \"S000004187\", \"SIK1\", \"U3 snoRNP protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 1.0814e-05, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YLR197W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.49, \"gal1RGexp\": 0.02, \"gal80Rsig\": 3.3814e-08, \"Stress\": 0.0, \"id\": \"1788\", \"gal4RGexp\": -0.521, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"35S primary transcript processing\", \"processing of 20S pre-rRNA\", \"rRNA modification\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1788}}, {\"position\": {\"y\": 3886.679730896559, \"x\": 1067.2272947609617}, \"selected\": false, \"data\": {\"id_original\": \"1888\", \"name\": \"YDL088C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"ASM4\", \"selected\": false, \"BetweennessCentrality\": 0.66666667, \"gal1RGsig\": 0.091906, \"annotation_GO_CELLULAR_COMPONENT\": [\"nuclear pore\"], \"alias\": [\"ASM4\", \"NUP59\", \"S000002246\", \"nuclear pore complex subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural molecule activity\"], \"gal4RGsig\": 0.32752, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YDL088C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.75, \"AverageShortestPathLength\": 1.33333333, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": 0.124, \"gal1RGexp\": 0.069, \"gal80Rsig\": 0.081403, \"Stress\": 4.0, \"id\": \"1787\", \"gal4RGexp\": -0.074, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"NLS-bearing substrate import into nucleus\", \"mRNA export from nucleus\", \"mRNA-binding (hnRNP) protein import into nucleus\", \"nuclear pore organization and biogenesis\", \"protein export from nucleus\", \"protein import into nucleus\", \"docking\", \"rRNA export from nucleus\", \"ribosomal protein import into nucleus\", \"snRNA export from nucleus\", \"snRNP protein import into nucleus\"], \"Radiality\": 0.88888889, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1787}}, {\"position\": {\"y\": 3937.604524139967, \"x\": 2857.597373801489}, \"selected\": false, \"data\": {\"id_original\": \"1889\", \"name\": \"YOR215C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"YOR215C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00070779, \"annotation_GO_CELLULAR_COMPONENT\": [\"mitochondrion\"], \"alias\": [\"S000005741\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.010881, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOR215C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.335, \"gal1RGexp\": 0.151, \"gal80Rsig\": 0.0001273, \"Stress\": 0.0, \"id\": \"1786\", \"gal4RGexp\": 0.137, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1786}}, {\"position\": {\"y\": 1446.598512177076, \"x\": 3338.9744184792235}, \"selected\": false, \"data\": {\"id_original\": \"1890\", \"name\": \"YPR010C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"RPA135\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0032078, \"annotation_GO_CELLULAR_COMPONENT\": [\"DNA-directed RNA polymerase I complex\"], \"alias\": [\"RNA polymerase I subunit\", \"A135\", \"RPA135\", \"RPA2\", \"RRN2\", \"S000006214\", \"SRP3\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA-directed RNA polymerase activity\"], \"gal4RGsig\": 5.8663e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPR010C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10205761, \"AverageShortestPathLength\": 9.7983871, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.359, \"gal1RGexp\": -0.129, \"gal80Rsig\": 0.0061199, \"Stress\": 0.0, \"id\": \"1785\", \"gal4RGexp\": -0.394, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"transcription from RNA polymerase I promoter\"], \"Radiality\": 0.67413381, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1785}}, {\"position\": {\"y\": 1530.281280045118, \"x\": 3239.9246137917235}, \"selected\": false, \"data\": {\"id_original\": \"1891\", \"name\": \"YMR117C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"SPC24\", \"selected\": false, \"BetweennessCentrality\": 0.01609638, \"gal1RGsig\": 0.41398, \"annotation_GO_CELLULAR_COMPONENT\": [\"Ndc80 complex\", \"condensed nuclear chromosome kinetochore\", \"condensed nuclear chromosome\", \"pericentric region\"], \"alias\": [\"S000004723\", \"SPC24\", \"spindle pole component\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of cytoskeleton\"], \"gal4RGsig\": 5.0622e-05, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YMR117C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11360513, \"AverageShortestPathLength\": 8.80241935, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.296, \"gal1RGexp\": 0.093, \"gal80Rsig\": 0.35411, \"Stress\": 1234.0, \"id\": \"1784\", \"gal4RGexp\": 0.371, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"chromosome segregation\", \"microtubule nucleation\"], \"Radiality\": 0.71102151, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1784}}, {\"position\": {\"y\": 104.31091452082592, \"x\": 1873.371513205786}, \"selected\": false, \"data\": {\"id_original\": \"1892\", \"name\": \"YML114C\", \"Eccentricity\": 24, \"IsSingleNode\": false, \"COMMON\": \"YML114C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00045247, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\", \"transcription factor TFIID complex\"], \"alias\": [\"TafII65\", \"S000004582\", \"TAF65\", \"TAF8\", \"TFIID subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 0.12134, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YML114C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08112529, \"AverageShortestPathLength\": 12.3266129, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.385, \"gal1RGexp\": 0.226, \"gal80Rsig\": 0.0058304, \"Stress\": 0.0, \"id\": \"1783\", \"gal4RGexp\": -0.145, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"transcription from RNA polymerase II promoter\"], \"Radiality\": 0.58049582, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1783}}, {\"position\": {\"y\": 1034.6408400579353, \"x\": 2732.897453147192}, \"selected\": false, \"data\": {\"id_original\": \"1893\", \"name\": \"YNL036W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"NCE103\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 4.2628e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"NCE103\", \"NCE3\", \"S000004981\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"carbonate dehydratase activity\"], \"gal4RGsig\": 1.601e-08, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YNL036W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12849741, \"AverageShortestPathLength\": 7.78225806, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": 0.686, \"gal1RGexp\": 0.998, \"gal80Rsig\": 3.3349e-07, \"Stress\": 0.0, \"id\": \"1782\", \"gal4RGexp\": 0.506, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"response to oxidative stress\"], \"Radiality\": 0.74880526, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1782}}, {\"position\": {\"y\": 1700.8447279973884, \"x\": 2581.402244406958}, \"selected\": false, \"data\": {\"id_original\": \"1894\", \"name\": \"YOR212W\", \"Eccentricity\": 14, \"IsSingleNode\": false, \"COMMON\": \"STE4\", \"selected\": false, \"BetweennessCentrality\": 0.13650914, \"gal1RGsig\": 4.187e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"heterotrimeric G-protein complex\", \"mating projection\", \"plasma membrane\"], \"alias\": [\"G protein beta subunit\", \"HMD2\", \"S000005738\", \"STE4\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"signal transducer activity\"], \"gal4RGsig\": 0.0010135, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YOR212W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14885954, \"AverageShortestPathLength\": 6.71774194, \"NeighborhoodConnectivity\": 6.5, \"gal80Rexp\": -0.158, \"gal1RGexp\": -0.189, \"gal80Rsig\": 0.023184, \"Stress\": 14000.0, \"id\": \"1781\", \"gal4RGexp\": -0.256, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"heterotrimeric G-protein complex cycle\", \"invasive growth (sensu the Saccharomyces research community)\", \"pheromone-dependent signal transduction during conjugation with cellular fusion\"], \"Radiality\": 0.78823178, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1781}}, {\"position\": {\"y\": 1949.0816054387947, \"x\": 943.4944380104735}, \"selected\": false, \"data\": {\"id_original\": \"1895\", \"name\": \"YDR070C\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"YDR070C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 4.6513e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"mitochondrion\"], \"alias\": [\"S000002477\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.0001488, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDR070C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09567901, \"AverageShortestPathLength\": 10.4516129, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.089, \"gal1RGexp\": -0.915, \"gal80Rsig\": 0.1936, \"Stress\": 0.0, \"id\": \"1780\", \"gal4RGexp\": 0.671, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.64994026, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1780}}, {\"position\": {\"y\": 884.5940566106697, \"x\": 2661.8057783425047}, \"selected\": false, \"data\": {\"id_original\": \"1896\", \"name\": \"YNL164C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"YNL164C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00073947, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"IBD2\", \"S000005108\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 3.8499e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YNL164C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11809524, \"AverageShortestPathLength\": 8.46774194, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 1.345, \"gal1RGexp\": 0.272, \"gal80Rsig\": 5.7872e-06, \"Stress\": 0.0, \"id\": \"1779\", \"gal4RGexp\": -0.949, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mitotic spindle checkpoint\"], \"Radiality\": 0.72341697, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1779}}, {\"position\": {\"y\": 2167.0576796575447, \"x\": 2348.9148786843016}, \"selected\": false, \"data\": {\"id_original\": \"1897\", \"name\": \"YGR046W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"YGR046W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0020954, \"annotation_GO_CELLULAR_COMPONENT\": [\"extrinsic to mitochondrial inner membrane\", \"mitochondrial matrix\", \"mitochondrion\"], \"alias\": [\"MMP37\", \"S000003278\", \"TAM41\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.00051497, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGR046W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10247934, \"AverageShortestPathLength\": 9.75806452, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.124, \"gal1RGexp\": 0.158, \"gal80Rsig\": 0.10073, \"Stress\": 0.0, \"id\": \"1778\", \"gal4RGexp\": 0.177, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein import into mitochondrial matrix\"], \"Radiality\": 0.67562724, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1778}}, {\"position\": {\"y\": 899.5289320989509, \"x\": 1707.166679221411}, \"selected\": false, \"data\": {\"id_original\": \"1898\", \"name\": \"YLR153C\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"ACS2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.24703, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\", \"nucleus\"], \"alias\": [\"ACS2\", \"S000004143\", \"acetyl CoA synthetase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"acetate-CoA ligase activity\"], \"gal4RGsig\": 0.78003, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLR153C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10450906, \"AverageShortestPathLength\": 9.56854839, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.172, \"gal1RGexp\": -0.042, \"gal80Rsig\": 0.011567, \"Stress\": 0.0, \"id\": \"1777\", \"gal4RGexp\": -0.014, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"acetyl-CoA biosynthetic process\", \"histone acetylation\"], \"Radiality\": 0.68264636, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1777}}, {\"position\": {\"y\": 1113.7319960637947, \"x\": 3057.0689009010985}, \"selected\": false, \"data\": {\"id_original\": \"1899\", \"name\": \"YIL070C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"MAM33\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.68369, \"annotation_GO_CELLULAR_COMPONENT\": [\"mitochondrial matrix\", \"mitochondrion\"], \"alias\": [\"MAM33\", \"S000001332\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.0033116, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YIL070C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09509202, \"AverageShortestPathLength\": 10.51612903, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.339, \"gal1RGexp\": 0.033, \"gal80Rsig\": 9.0003e-06, \"Stress\": 0.0, \"id\": \"1776\", \"gal4RGexp\": 0.146, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"aerobic respiration\"], \"Radiality\": 0.64755078, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1776}}, {\"position\": {\"y\": 1906.6965956731697, \"x\": 1981.236533957739}, \"selected\": false, \"data\": {\"id_original\": \"1900\", \"name\": \"YPR113W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"PIS1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.0456e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"endoplasmic reticulum\", \"microsome\", \"mitochondrial outer membrane\", \"mitochondrion\"], \"alias\": [\"PIS1\", \"S000006317\", \"phosphatidylinositol synthase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"CDP-diacylglycerol-inositol 3-phosphatidyltransferase activity\"], \"gal4RGsig\": 0.54593, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPR113W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14622642, \"AverageShortestPathLength\": 6.83870968, \"NeighborhoodConnectivity\": 18.0, \"gal80Rexp\": -0.89, \"gal1RGexp\": -0.495, \"gal80Rsig\": 5.6202e-18, \"Stress\": 0.0, \"id\": \"1775\", \"gal4RGexp\": 0.025, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell cycle\", \"phosphatidylinositol biosynthetic process\"], \"Radiality\": 0.78375149, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1775}}, {\"position\": {\"y\": 3777.1561293645764, \"x\": 943.4944380104735}, \"selected\": false, \"data\": {\"id_original\": \"1901\", \"name\": \"YER081W\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"SER3\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 2.5284e-10, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"3-phosphoglycerate dehydrogenase\", \"S000000883\", \"SER3\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"phosphoglycerate dehydrogenase activity\"], \"gal4RGsig\": 3.0368e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YER081W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.5, \"AverageShortestPathLength\": 2.0, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.109, \"gal1RGexp\": -0.568, \"gal80Rsig\": 0.094304, \"Stress\": 0.0, \"id\": \"1774\", \"gal4RGexp\": -0.423, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"serine family amino acid biosynthetic process\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1774}}, {\"position\": {\"y\": 1350.2727828069587, \"x\": 3000.307609397192}, \"selected\": false, \"data\": {\"id_original\": \"1902\", \"name\": \"YGR088W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"CTT1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.6823e-10, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"CTT1\", \"S000003320\", \"catalase T\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"catalase activity\"], \"gal4RGsig\": 0.00019828, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGR088W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10495133, \"AverageShortestPathLength\": 9.52822581, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": -0.394, \"gal1RGexp\": -0.91, \"gal80Rsig\": 3.0726e-06, \"Stress\": 0.0, \"id\": \"1773\", \"gal4RGexp\": 0.596, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"response to cold\", \"response to reactive oxygen species\", \"response to stress\"], \"Radiality\": 0.68413978, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1773}}, {\"position\": {\"y\": 654.2241225286384, \"x\": 1984.8901747048094}, \"selected\": false, \"data\": {\"id_original\": \"1903\", \"name\": \"YDR395W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"SXM1\", \"selected\": false, \"BetweennessCentrality\": 0.05570067, \"gal1RGsig\": 0.021972, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"KAP108\", \"S000002803\", \"SXM1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein carrier activity\"], \"gal4RGsig\": 0.5176, \"TopologicalCoefficient\": 0.25, \"shared_name\": \"YDR395W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12265084, \"AverageShortestPathLength\": 8.15322581, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": -0.214, \"gal1RGexp\": -0.119, \"gal80Rsig\": 0.40913, \"Stress\": 16272.0, \"id\": \"1772\", \"gal4RGexp\": 0.086, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mRNA export from nucleus\", \"nucleocytoplasmic transport\"], \"Radiality\": 0.73506571, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 8.0, \"Degree\": 8, \"SUID\": 1772}}, {\"position\": {\"y\": 684.6015639348884, \"x\": 1848.7909162819578}, \"selected\": false, \"data\": {\"id_original\": \"1904\", \"name\": \"YGR085C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"RPL11B\", \"selected\": false, \"BetweennessCentrality\": 0.00401593, \"gal1RGsig\": 0.0002639, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPL11B\", \"S000003317\", \"ribosomal protein L11B (L16B) (rp39B) (YL22)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 7.4028e-07, \"TopologicalCoefficient\": 0.57142857, \"shared_name\": \"YGR085C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10939568, \"AverageShortestPathLength\": 9.14112903, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.084, \"gal1RGexp\": -0.188, \"gal80Rsig\": 0.1613, \"Stress\": 2038.0, \"id\": \"1771\", \"gal4RGexp\": -0.425, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ribosomal large subunit assembly and maintenance\"], \"Radiality\": 0.6984767, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1771}}, {\"position\": {\"y\": 1646.987832550611, \"x\": 2974.038017112036}, \"selected\": false, \"data\": {\"id_original\": \"1905\", \"name\": \"YER124C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"YER124C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00018529, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud neck\"], \"alias\": [\"DSE1\", \"S000000926\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.014038, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YER124C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12724474, \"AverageShortestPathLength\": 7.85887097, \"NeighborhoodConnectivity\": 9.0, \"gal80Rexp\": -0.022, \"gal1RGexp\": 0.179, \"gal80Rsig\": 0.76846, \"Stress\": 0.0, \"id\": \"1770\", \"gal4RGexp\": 0.126, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell wall organization and biogenesis\"], \"Radiality\": 0.74596774, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1770}}, {\"position\": {\"y\": 1298.9030165716072, \"x\": 1416.6675337135985}, \"selected\": false, \"data\": {\"id_original\": \"1906\", \"name\": \"YMR005W\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"MPT1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 5.0658e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"transcription factor TFIID complex\"], \"alias\": [\"TafII48\", \"MPT1\", \"S000004607\", \"TAF4\", \"TAF48\", \"TFIID subunit\", \"TSG2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 0.00012336, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YMR005W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09121, \"AverageShortestPathLength\": 10.96370968, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.571, \"gal1RGexp\": 0.218, \"gal80Rsig\": 9.5685e-05, \"Stress\": 0.0, \"id\": \"1769\", \"gal4RGexp\": -0.419, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"transcription from RNA polymerase II promoter\"], \"Radiality\": 0.63097372, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1769}}, {\"position\": {\"y\": 1333.753053192701, \"x\": 1534.6663740456297}, \"selected\": false, \"data\": {\"id_original\": \"1907\", \"name\": \"YDL030W\", \"Eccentricity\": 22, \"IsSingleNode\": false, \"COMMON\": \"PRP9\", \"selected\": false, \"BetweennessCentrality\": 0.12179569, \"gal1RGsig\": 0.00027658, \"annotation_GO_CELLULAR_COMPONENT\": [\"snRNP U2\"], \"alias\": [\"PRP9\", \"RNA splicing factor\", \"S000002188\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\"], \"gal4RGsig\": 0.25629, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YDL030W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10032362, \"AverageShortestPathLength\": 9.96774194, \"NeighborhoodConnectivity\": 2.33333333, \"gal80Rexp\": 0.339, \"gal1RGexp\": 0.244, \"gal80Rsig\": 0.011822, \"Stress\": 55180.0, \"id\": \"1768\", \"gal4RGexp\": -0.119, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nuclear mRNA splicing\", \"via spliceosome\"], \"Radiality\": 0.66786141, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1768}}, {\"position\": {\"y\": 3514.7337050481697, \"x\": 1991.6870840370848}, \"selected\": false, \"data\": {\"id_original\": \"1908\", \"name\": \"YER079W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"YER079W\", \"selected\": false, \"BetweennessCentrality\": 0.66666667, \"gal1RGsig\": 0.00041949, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"S000000881\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 4.6903e-05, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YER079W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.046, \"gal1RGexp\": -0.174, \"gal80Rsig\": 0.40867, \"Stress\": 8.0, \"id\": \"1767\", \"gal4RGexp\": -0.267, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.875, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1767}}, {\"position\": {\"y\": 450.8151869817634, \"x\": 2073.7726896584227}, \"selected\": false, \"data\": {\"id_original\": \"1909\", \"name\": \"YDL215C\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"GDH2\", \"selected\": false, \"BetweennessCentrality\": 0.04770145, \"gal1RGsig\": 9.0717e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"mitochondrion\", \"soluble fraction\"], \"alias\": [\"GDH-B\", \"GDH2\", \"GDHB\", \"NAD-dependent glutamate dehydrogenase\", \"S000002374\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"glutamate dehydrogenase activity\"], \"gal4RGsig\": 0.21914, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YDL215C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10680448, \"AverageShortestPathLength\": 9.36290323, \"NeighborhoodConnectivity\": 2.66666667, \"gal80Rexp\": 0.0, \"gal1RGexp\": 0.485, \"gal80Rsig\": 0.999999, \"Stress\": 15318.0, \"id\": \"1766\", \"gal4RGexp\": 0.242, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nitrogen compound metabolic process\"], \"Radiality\": 0.69026284, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1766}}, {\"position\": {\"y\": 2739.0078139348884, \"x\": 2650.3809126198485}, \"selected\": false, \"data\": {\"id_original\": \"1910\", \"name\": \"YIL045W\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"PIG2\", \"selected\": false, \"BetweennessCentrality\": 0.07770667, \"gal1RGsig\": 0.056656, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"PIG2\", \"S000001307\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein phosphatase type 1 regulator activity\"], \"gal4RGsig\": 8.8839e-07, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YIL045W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09469263, \"AverageShortestPathLength\": 10.56048387, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.134, \"gal1RGexp\": -0.078, \"gal80Rsig\": 0.072301, \"Stress\": 19520.0, \"id\": \"1765\", \"gal4RGexp\": 0.478, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of glycogen biosynthetic process\"], \"Radiality\": 0.645908, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1765}}, {\"position\": {\"y\": 2031.4805007024665, \"x\": 1317.2480390846922}, \"selected\": false, \"data\": {\"id_original\": \"1911\", \"name\": \"YPR041W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"TIF5\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.11203, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic small ribosomal subunit (sensu the Eukaryota research community)\", \"multi-eIF complex\"], \"alias\": [\"eIF5\", \"S000006245\", \"SUI5\", \"TIF5\", \"Translation initiation factor eIF5\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"GTPase activator activity\", \"translation initiation factor activity\"], \"gal4RGsig\": 0.0061997, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YPR041W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09691286, \"AverageShortestPathLength\": 10.31854839, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.177, \"gal1RGexp\": -0.059, \"gal80Rsig\": 0.011738, \"Stress\": 0.0, \"id\": \"1764\", \"gal4RGexp\": -0.243, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mature ribosome assembly\", \"regulation of translational initiation\"], \"Radiality\": 0.65486858, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1764}}, {\"position\": {\"y\": 1559.7355074926277, \"x\": 2681.8426435768797}, \"selected\": false, \"data\": {\"id_original\": \"1912\", \"name\": \"YOR120W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"GCY1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 9.0303e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"GCY\", \"GCY1\", \"S000005646\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"aldo-keto reductase activity\"], \"gal4RGsig\": 1.8298e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOR120W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13100898, \"AverageShortestPathLength\": 7.63306452, \"NeighborhoodConnectivity\": 10.0, \"gal80Rexp\": 0.576, \"gal1RGexp\": 0.194, \"gal80Rsig\": 6.3927e-09, \"Stress\": 0.0, \"id\": \"1763\", \"gal4RGexp\": -0.349, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"D-xylose catabolic process\", \"arabinose catabolic process\", \"response to salt stress\"], \"Radiality\": 0.75433094, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1763}}, {\"position\": {\"y\": 3886.679730896559, \"x\": 951.4382246315672}, \"selected\": false, \"data\": {\"id_original\": \"1913\", \"name\": \"YIL074C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"SER33\", \"selected\": false, \"BetweennessCentrality\": 0.66666667, \"gal1RGsig\": 4.9389e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"3-phosphoglycerate dehydrogenase\", \"S000001336\", \"SER33\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"phosphoglycerate dehydrogenase activity\"], \"gal4RGsig\": 2.6626e-05, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YIL074C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.75, \"AverageShortestPathLength\": 1.33333333, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": 0.393, \"gal1RGexp\": -0.444, \"gal80Rsig\": 0.022682, \"Stress\": 4.0, \"id\": \"1762\", \"gal4RGexp\": -0.565, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"serine family amino acid biosynthetic process\"], \"Radiality\": 0.88888889, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1762}}, {\"position\": {\"y\": 2012.2039198919197, \"x\": 2101.116142112036}, \"selected\": false, \"data\": {\"id_original\": \"1914\", \"name\": \"YDR299W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"BFR2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.6082e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleolus\"], \"alias\": [\"BFR2\", \"S000002707\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.20033, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDR299W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12776919, \"AverageShortestPathLength\": 7.8266129, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.883, \"gal1RGexp\": 0.518, \"gal80Rsig\": 0.00010236, \"Stress\": 0.0, \"id\": \"1761\", \"gal4RGexp\": -0.287, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ER to Golgi vesicle-mediated transport\"], \"Radiality\": 0.74716249, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1761}}, {\"position\": {\"y\": 1693.485276703443, \"x\": 3030.458427268286}, \"selected\": false, \"data\": {\"id_original\": \"1915\", \"name\": \"YHR005C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"GPA1\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.64417, \"annotation_GO_CELLULAR_COMPONENT\": [\"endosome\", \"heterotrimeric G-protein complex\", \"plasma membrane\"], \"alias\": [\"CDC70\", \"DAC1\", \"G protein alpha subunit\", \"GPA1\", \"S000001047\", \"SCG1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"GTPase activity\"], \"gal4RGsig\": 5.0285e-05, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YHR005C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12737545, \"AverageShortestPathLength\": 7.85080645, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": 0.33, \"gal1RGexp\": -0.02, \"gal80Rsig\": 0.0031233, \"Stress\": 618.0, \"id\": \"1760\", \"gal4RGexp\": -0.413, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"heterotrimeric G-protein complex cycle\", \"inositol lipid-mediated signaling\", \"pheromone-dependent signal transduction during conjugation with cellular fusion\"], \"Radiality\": 0.74626643, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1760}}, {\"position\": {\"y\": 1708.4099135442634, \"x\": 3161.310233908911}, \"selected\": false, \"data\": {\"id_original\": \"1916\", \"name\": \"YLR452C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"SST2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 2.4276e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"plasma membrane\"], \"alias\": [\"GTPase activating protein (GAP)\", \"S000004444\", \"SST2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"GTPase activator activity\"], \"gal4RGsig\": 6.6966e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLR452C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11303555, \"AverageShortestPathLength\": 8.84677419, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.269, \"gal1RGexp\": -0.507, \"gal80Rsig\": 0.014595, \"Stress\": 0.0, \"id\": \"1759\", \"gal4RGexp\": -0.393, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"adaptation to pheromone during conjugation with cellular fusion\", \"signal transduction\"], \"Radiality\": 0.70937873, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1759}}, {\"position\": {\"y\": 2719.5822768255134, \"x\": 3184.732597190161}, \"selected\": false, \"data\": {\"id_original\": \"1917\", \"name\": \"YMR255W\", \"Eccentricity\": 24, \"IsSingleNode\": false, \"COMMON\": \"GFD1\", \"selected\": false, \"BetweennessCentrality\": 0.03186627, \"gal1RGsig\": 0.00022142, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nuclear pore\"], \"alias\": [\"GFD1\", \"S000004868\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.11742, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YMR255W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.0746089, \"AverageShortestPathLength\": 13.40322581, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.397, \"gal1RGexp\": 0.211, \"gal80Rsig\": 0.0029074, \"Stress\": 7856.0, \"id\": \"1758\", \"gal4RGexp\": -0.159, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mRNA export from nucleus\"], \"Radiality\": 0.54062127, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1758}}, {\"position\": {\"y\": 2741.120973114576, \"x\": 3022.4128950417235}, \"selected\": false, \"data\": {\"id_original\": \"1918\", \"name\": \"YBR274W\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"CHK1\", \"selected\": false, \"BetweennessCentrality\": 0.03966958, \"gal1RGsig\": 0.35655, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"CHK1\", \"S000000478\", \"protein kinase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein kinase activity\"], \"gal4RGsig\": 0.0049782, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YBR274W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08038898, \"AverageShortestPathLength\": 12.43951613, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": -0.052, \"gal1RGexp\": -0.045, \"gal80Rsig\": 0.55148, \"Stress\": 9810.0, \"id\": \"1757\", \"gal4RGexp\": 0.135, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"DNA damage checkpoint\", \"protein amino acid phosphorylation\"], \"Radiality\": 0.57631422, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1757}}, {\"position\": {\"y\": 1741.4512343450447, \"x\": 1962.7844312966063}, \"selected\": false, \"data\": {\"id_original\": \"1919\", \"name\": \"YHR084W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"STE12\", \"selected\": false, \"BetweennessCentrality\": 3.265e-05, \"gal1RGsig\": 0.014623, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"S000001126\", \"STE12\", \"transcription factor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcription factor activity\"], \"gal4RGsig\": 6.6062e-06, \"TopologicalCoefficient\": 0.375, \"shared_name\": \"YHR084W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14648553, \"AverageShortestPathLength\": 6.8266129, \"NeighborhoodConnectivity\": 6.75, \"gal80Rexp\": 0.057, \"gal1RGexp\": -0.109, \"gal80Rsig\": 0.65365, \"Stress\": 6.0, \"id\": \"1756\", \"gal4RGexp\": -0.541, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"conjugation with cellular fusion\", \"invasive growth (sensu the Saccharomyces research community)\", \"positive regulation of transcription from RNA polymerase II promoter by pheromones\", \"pseudohyphal growth\"], \"Radiality\": 0.78419952, \"ClusteringCoefficient\": 0.5, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1756}}, {\"position\": {\"y\": 2174.712037567701, \"x\": 2701.162040549536}, \"selected\": false, \"data\": {\"id_original\": \"1920\", \"name\": \"YBL050W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"SEC17\", \"selected\": false, \"BetweennessCentrality\": 0.0932363, \"gal1RGsig\": 0.11213, \"annotation_GO_CELLULAR_COMPONENT\": [\"peripheral to membrane of membrane fraction\"], \"alias\": [\"S000000146\", \"SEC17\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"soluble NSF attachment protein activity\"], \"gal4RGsig\": 0.48722, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YBL050W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11166141, \"AverageShortestPathLength\": 8.95564516, \"NeighborhoodConnectivity\": 5.5, \"gal80Rexp\": 0.106, \"gal1RGexp\": -0.066, \"gal80Rsig\": 0.23706, \"Stress\": 10384.0, \"id\": \"1755\", \"gal4RGexp\": -0.044, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ER to Golgi vesicle-mediated transport\", \"vacuole fusion\", \"non-autophagic\"], \"Radiality\": 0.70534648, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1755}}, {\"position\": {\"y\": 1986.1980910344978, \"x\": 1598.1079023659422}, \"selected\": false, \"data\": {\"id_original\": \"1921\", \"name\": \"YBL026W\", \"Eccentricity\": 26, \"IsSingleNode\": false, \"COMMON\": \"LSM2\", \"selected\": false, \"BetweennessCentrality\": 8.16e-06, \"gal1RGsig\": 0.48423, \"annotation_GO_CELLULAR_COMPONENT\": [\"U4/U6 x U5 tri-snRNP complex\", \"nucleolus\", \"small nucleolar ribonucleoprotein complex\", \"snRNP U6\"], \"alias\": [\"Sm class\", \"LSM2\", \"S000000122\", \"SMX5\", \"SNP3\", \"snRNA-associated protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\", \"RNA splicing factor activity\", \"transesterification mechanism\"], \"gal4RGsig\": 0.019199, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YBL026W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.0763782, \"AverageShortestPathLength\": 13.09274194, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": -0.064, \"gal1RGexp\": -0.032, \"gal80Rsig\": 0.59933, \"Stress\": 2.0, \"id\": \"1754\", \"gal4RGexp\": -0.158, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mRNA catabolic process\", \"nuclear mRNA splicing\", \"via spliceosome\"], \"Radiality\": 0.55212067, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1754}}, {\"position\": {\"y\": 1899.8337111516853, \"x\": 2075.3315809548094}, \"selected\": false, \"data\": {\"id_original\": \"1922\", \"name\": \"YJL194W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"CDC6\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.83635, \"annotation_GO_CELLULAR_COMPONENT\": [\"pre-replicative complex\"], \"alias\": [\"CDC6\", \"S000003730\", \"pre-initiation complex component\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"ATPase activity\", \"DNA clamp loader activity\", \"protein binding\"], \"gal4RGsig\": 4.5029e-05, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YJL194W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14639906, \"AverageShortestPathLength\": 6.83064516, \"NeighborhoodConnectivity\": 9.5, \"gal80Rexp\": 0.525, \"gal1RGexp\": 0.018, \"gal80Rsig\": 0.050004, \"Stress\": 1032.0, \"id\": \"1753\", \"gal4RGexp\": -0.661, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"pre-replicative complex formation\"], \"Radiality\": 0.78405018, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1753}}, {\"position\": {\"y\": 2729.6386733098884, \"x\": 2849.1170576393797}, \"selected\": false, \"data\": {\"id_original\": \"1923\", \"name\": \"YLR258W\", \"Eccentricity\": 22, \"IsSingleNode\": false, \"COMMON\": \"GSY2\", \"selected\": false, \"BetweennessCentrality\": 0.07081755, \"gal1RGsig\": 4.4171e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"GSY2\", \"S000004248\", \"glycogen synthase (UDP-glucose-starch glucosyltransferase)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"glycogen (starch) synthase activity\"], \"gal4RGsig\": 1.8329e-06, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YLR258W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08707865, \"AverageShortestPathLength\": 11.48387097, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.487, \"gal1RGexp\": -0.405, \"gal80Rsig\": 5.8675e-12, \"Stress\": 17622.0, \"id\": \"1752\", \"gal4RGexp\": 0.4, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glycogen biosynthetic process\"], \"Radiality\": 0.61170848, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1752}}, {\"position\": {\"y\": 2657.6311049505134, \"x\": 2956.122550803442}, \"selected\": false, \"data\": {\"id_original\": \"1924\", \"name\": \"YGL134W\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"PCL10\", \"selected\": false, \"BetweennessCentrality\": 0.01606373, \"gal1RGsig\": 0.0020577, \"annotation_GO_CELLULAR_COMPONENT\": [\"cyclin-dependent protein kinase holoenzyme complex\"], \"alias\": [\"PCL10\", \"S000003102\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"cyclin-dependent protein kinase regulator activity\"], \"gal4RGsig\": 3.6977e-07, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YGL134W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08023293, \"AverageShortestPathLength\": 12.46370968, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.839, \"gal1RGexp\": 0.138, \"gal80Rsig\": 3.7595e-07, \"Stress\": 3936.0, \"id\": \"1751\", \"gal4RGexp\": -0.548, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of glycogen biosynthetic process\", \"regulation of glycogen catabolic process\"], \"Radiality\": 0.57541816, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1751}}, {\"position\": {\"y\": 3063.9384779973884, \"x\": 2350.3408735573485}, \"selected\": false, \"data\": {\"id_original\": \"1925\", \"name\": \"YHR055C\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"CUP1B\", \"selected\": false, \"BetweennessCentrality\": 0.00799922, \"gal1RGsig\": 2.8813e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\"], \"alias\": [\"CUP1\", \"CUP1-2\", \"S000001097\", \"copper binding metallothionein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"copper ion binding\"], \"gal4RGsig\": 0.026753, \"TopologicalCoefficient\": 0.6, \"shared_name\": \"YHR055C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09383277, \"AverageShortestPathLength\": 10.65725806, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": 0.802, \"gal1RGexp\": -0.867, \"gal80Rsig\": 1.9696e-12, \"Stress\": 2004.0, \"id\": \"1750\", \"gal4RGexp\": -0.416, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"response to copper ion\"], \"Radiality\": 0.64232378, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1750}}, {\"position\": {\"y\": 3078.892457489576, \"x\": 2270.0906446755125}, \"selected\": false, \"data\": {\"id_original\": \"1926\", \"name\": \"YHR053C\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"CUP1A\", \"selected\": false, \"BetweennessCentrality\": 0.00799922, \"gal1RGsig\": 0.00016731, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\"], \"alias\": [\"CUP1\", \"CUP1-1\", \"S000001095\", \"copper binding metallothionein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"copper ion binding\"], \"gal4RGsig\": 0.00084638, \"TopologicalCoefficient\": 0.6, \"shared_name\": \"YHR053C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09383277, \"AverageShortestPathLength\": 10.65725806, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": 0.795, \"gal1RGexp\": -0.656, \"gal80Rsig\": 1.5187e-10, \"Stress\": 2004.0, \"id\": \"1749\", \"gal4RGexp\": -0.75, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"response to copper ion\"], \"Radiality\": 0.64232378, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1749}}, {\"position\": {\"y\": 3872.974538330641, \"x\": 2612.531226950903}, \"selected\": false, \"data\": {\"id_original\": \"1927\", \"name\": \"YPR124W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"CTR1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 8.4218e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"plasma membrane\"], \"alias\": [\"CTR1\", \"S000006328\", \"copper transport protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"copper uptake transporter activity\"], \"gal4RGsig\": 2.4189e-08, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPR124W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.462, \"gal1RGexp\": 0.76, \"gal80Rsig\": 8.3821e-06, \"Stress\": 0.0, \"id\": \"1748\", \"gal4RGexp\": 0.469, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"copper ion import\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1748}}, {\"position\": {\"y\": 2288.958680634107, \"x\": 1985.247032004614}, \"selected\": false, \"data\": {\"id_original\": \"1928\", \"name\": \"YNL135C\", \"Eccentricity\": 22, \"IsSingleNode\": false, \"COMMON\": \"FPR1\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.047943, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"mitochondrion\", \"nucleus\"], \"alias\": [\"FKB1\", \"FKBP12\", \"FPR1\", \"RBP1\", \"S000005079\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"peptidyl-prolyl cis-trans isomerase activity\"], \"gal4RGsig\": 0.068027, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YNL135C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08469945, \"AverageShortestPathLength\": 11.80645161, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.169, \"gal1RGexp\": -0.071, \"gal80Rsig\": 0.0016167, \"Stress\": 978.0, \"id\": \"1747\", \"gal4RGexp\": 0.08, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"establishment and/or maintenance of chromatin architecture\", \"homoserine metabolic process\"], \"Radiality\": 0.59976105, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1747}}, {\"position\": {\"y\": 2238.111939911451, \"x\": 1891.3052748024656}, \"selected\": false, \"data\": {\"id_original\": \"1929\", \"name\": \"YER052C\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"HOM3\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 4.7628e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"BOR1\", \"HOM3\", \"S000000854\", \"SIL4\", \"aspartate kinase (L-aspartate 4-P-transferase) (EC 2.7.2.4)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"aspartate kinase activity\"], \"gal4RGsig\": 1.9577e-09, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YER052C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.07811024, \"AverageShortestPathLength\": 12.80241935, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.579, \"gal1RGexp\": -0.47, \"gal80Rsig\": 1.3742e-05, \"Stress\": 0.0, \"id\": \"1746\", \"gal4RGexp\": -1.321, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"homoserine biosynthetic process\", \"methionine metabolic process\", \"threonine metabolic process\"], \"Radiality\": 0.56287336, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1746}}, {\"position\": {\"y\": 2189.7585463567634, \"x\": 1715.0863264382078}, \"selected\": false, \"data\": {\"id_original\": \"1930\", \"name\": \"YLR284C\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"ECI1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0052304, \"annotation_GO_CELLULAR_COMPONENT\": [\"peroxisome\"], \"alias\": [\"ECI1\", \"S000004274\", \"d2-Enoyl-CoA Isomerase\", \"d3\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"dodecenoyl-CoA delta-isomerase activity\"], \"gal4RGsig\": 0.21531, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLR284C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08217362, \"AverageShortestPathLength\": 12.16935484, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": 0.716, \"gal1RGexp\": 0.195, \"gal80Rsig\": 0.00039241, \"Stress\": 0.0, \"id\": \"1745\", \"gal4RGexp\": -0.148, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"fatty acid beta-oxidation\"], \"Radiality\": 0.58632019, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1745}}, {\"position\": {\"y\": 2251.3535780950447, \"x\": 1775.1436079323485}, \"selected\": false, \"data\": {\"id_original\": \"1931\", \"name\": \"YHR198C\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"YHR198C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.20226, \"annotation_GO_CELLULAR_COMPONENT\": [\"mitochondrion\"], \"alias\": [\"S000001241\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 1.9253e-07, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YHR198C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08217362, \"AverageShortestPathLength\": 12.16935484, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": -0.379, \"gal1RGexp\": -0.053, \"gal80Rsig\": 5.4324e-08, \"Stress\": 0.0, \"id\": \"1744\", \"gal4RGexp\": 0.401, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.58632019, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1744}}, {\"position\": {\"y\": 1870.2854628606697, \"x\": 2907.834709006567}, \"selected\": false, \"data\": {\"id_original\": \"1932\", \"name\": \"YPL240C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"HSP82\", \"selected\": false, \"BetweennessCentrality\": 0.13656267, \"gal1RGsig\": 1.8582e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"HSP82\", \"HSP90\", \"S000006161\", \"heat shock protein 90\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"ATPase activity\", \"coupled\", \"unfolded protein binding\"], \"gal4RGsig\": 0.0024157, \"TopologicalCoefficient\": 0.35897436, \"shared_name\": \"YPL240C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1324079, \"AverageShortestPathLength\": 7.55241935, \"NeighborhoodConnectivity\": 5.66666667, \"gal80Rexp\": -0.661, \"gal1RGexp\": 0.222, \"gal80Rsig\": 6.7688e-11, \"Stress\": 14116.0, \"id\": \"1743\", \"gal4RGexp\": -0.201, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"'de novo' protein folding\", \"proteasome assembly\", \"protein refolding\", \"response to osmotic stress\", \"response to stress\"], \"Radiality\": 0.7573178, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1743}}, {\"position\": {\"y\": 618.8364882512947, \"x\": 1845.5437544167235}, \"selected\": false, \"data\": {\"id_original\": \"1933\", \"name\": \"YPR102C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"RPL11A\", \"selected\": false, \"BetweennessCentrality\": 0.00401593, \"gal1RGsig\": 0.00037183, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPL11A\", \"S000006306\", \"ribosomal protein L11A (L16A) (rp39A) (YL22)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 1.7424e-06, \"TopologicalCoefficient\": 0.57142857, \"shared_name\": \"YPR102C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10939568, \"AverageShortestPathLength\": 9.14112903, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.058, \"gal1RGexp\": -0.177, \"gal80Rsig\": 0.26052, \"Stress\": 2038.0, \"id\": \"1742\", \"gal4RGexp\": -0.38, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ribosomal large subunit assembly and maintenance\"], \"Radiality\": 0.6984767, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1742}}, {\"position\": {\"y\": 629.9019179387947, \"x\": 1736.0017927468016}, \"selected\": false, \"data\": {\"id_original\": \"1934\", \"name\": \"YLR075W\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"RPL10\", \"selected\": false, \"BetweennessCentrality\": 1.632e-05, \"gal1RGsig\": 0.00017255, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"GRC5\", \"QSR1\", \"RPL10\", \"S000004065\", \"ribosomal protein L10\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 0.00042857, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YLR075W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09872611, \"AverageShortestPathLength\": 10.12903226, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.002, \"gal1RGexp\": -0.169, \"gal80Rsig\": 0.9794, \"Stress\": 2.0, \"id\": \"1741\", \"gal4RGexp\": -0.2, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ribosomal large subunit assembly and maintenance\"], \"Radiality\": 0.66188769, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1741}}, {\"position\": {\"y\": 1879.2874465032478, \"x\": 2774.140067893286}, \"selected\": false, \"data\": {\"id_original\": \"1935\", \"name\": \"YKL161C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"YKL161C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00075801, \"annotation_GO_CELLULAR_COMPONENT\": [\"cellular_component\"], \"alias\": [\"MLP1\", \"S000001644\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein kinase activity\"], \"gal4RGsig\": 0.022048, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YKL161C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11106135, \"AverageShortestPathLength\": 9.00403226, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.202, \"gal1RGexp\": -0.198, \"gal80Rsig\": 0.18731, \"Stress\": 0.0, \"id\": \"1740\", \"gal4RGexp\": -0.319, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.70355436, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1740}}, {\"position\": {\"y\": 1036.7802748723884, \"x\": 1487.8471601784422}, \"selected\": false, \"data\": {\"id_original\": \"1936\", \"name\": \"YAR007C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"RFA1\", \"selected\": false, \"BetweennessCentrality\": 0.00401593, \"gal1RGsig\": 0.0081844, \"annotation_GO_CELLULAR_COMPONENT\": [\"DNA replication factor A complex\", \"chromosome\", \"telomeric region\", \"cytoplasm\", \"nucleus\"], \"alias\": [\"heterotrimeric RPA (RF-A) single-stranded DNA binding protein 69 kDa subunit\", \"BUF2\", \"FUN3\", \"RF-A\", \"RFA1\", \"RPA1\", \"S000000065\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"damaged DNA binding\", \"single-stranded DNA binding\"], \"gal4RGsig\": 0.91522, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YAR007C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10222589, \"AverageShortestPathLength\": 9.78225806, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": -0.302, \"gal1RGexp\": 0.2, \"gal80Rsig\": 0.0060792, \"Stress\": 1030.0, \"id\": \"1739\", \"gal4RGexp\": -0.007, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"DNA recombination\", \"DNA replication\", \"synthesis of RNA primer\", \"DNA strand elongation during DNA replication\", \"DNA unwinding during replication\", \"double-strand break repair via homologous recombination\", \"nucleotide-excision repair\", \"postreplication repair\"], \"Radiality\": 0.67473118, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1739}}, {\"position\": {\"y\": 3584.6231474920173, \"x\": 943.4944380104735}, \"selected\": false, \"data\": {\"id_original\": \"1937\", \"name\": \"YIL160C\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"POT1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.071e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"peroxisomal matrix\"], \"alias\": [\"3-oxoacyl CoA thiolase\", \"FOX3\", \"POT1\", \"POX3\", \"S000001422\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"acetyl-CoA C-acyltransferase activity\"], \"gal4RGsig\": 3.103e-08, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YIL160C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.42857143, \"AverageShortestPathLength\": 2.33333333, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.92, \"gal1RGexp\": 1.044, \"gal80Rsig\": 6.0801e-07, \"Stress\": 0.0, \"id\": \"1738\", \"gal4RGexp\": 0.674, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"fatty acid beta-oxidation\"], \"Radiality\": 0.55555556, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1738}}, {\"position\": {\"y\": 3396.6752944036384, \"x\": 1172.8320844948485}, \"selected\": false, \"data\": {\"id_original\": \"1938\", \"name\": \"YDL078C\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"MDH3\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 4.0255e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"peroxisomal matrix\", \"peroxisome\"], \"alias\": [\"MDH3\", \"S000002236\", \"malate dehydrogenase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"L-malate dehydrogenase activity\"], \"gal4RGsig\": 0.038943, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDL078C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.5, \"AverageShortestPathLength\": 2.0, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.08, \"gal1RGexp\": 0.217, \"gal80Rsig\": 0.16505, \"Stress\": 0.0, \"id\": \"1737\", \"gal4RGexp\": 0.102, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"NADH regeneration\", \"fatty acid beta-oxidation\", \"glyoxylate cycle\", \"malate metabolic process\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1737}}, {\"position\": {\"y\": 3557.027284149732, \"x\": 1053.246024924536}, \"selected\": false, \"data\": {\"id_original\": \"1939\", \"name\": \"YDR142C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"PEX7\", \"selected\": false, \"BetweennessCentrality\": 0.33333333, \"gal1RGsig\": 0.016464, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\", \"peroxisomal matrix\"], \"alias\": [\"PAS7\", \"PEB1\", \"PEX7\", \"S000002549\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"peroxisome targeting sequence binding\", \"peroxisome targeting signal-2 binding\"], \"gal4RGsig\": 0.16704, \"TopologicalCoefficient\": 0.6, \"shared_name\": \"YDR142C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 3.33333333, \"gal80Rexp\": -0.069, \"gal1RGexp\": -0.101, \"gal80Rsig\": 0.401, \"Stress\": 14.0, \"id\": \"1736\", \"gal4RGexp\": 0.112, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"peroxisome organization and biogenesis\", \"protein targeting to peroxisome\"], \"Radiality\": 0.83333333, \"ClusteringCoefficient\": 0.33333333, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1736}}, {\"position\": {\"y\": 3510.8709120794197, \"x\": 1160.9581526100828}, \"selected\": false, \"data\": {\"id_original\": \"1940\", \"name\": \"YDR244W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"PEX5\", \"selected\": false, \"BetweennessCentrality\": 0.5, \"gal1RGsig\": 2.7503e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\", \"peroxisome\"], \"alias\": [\"PAS10\", \"PEX5\", \"S000002652\", \"peroxin\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"peroxisome targeting sequence binding\", \"peroxisome targeting signal-1 binding\"], \"gal4RGsig\": 0.73489, \"TopologicalCoefficient\": 0.44, \"shared_name\": \"YDR244W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.85714286, \"AverageShortestPathLength\": 1.16666667, \"NeighborhoodConnectivity\": 2.4, \"gal80Rexp\": 0.483, \"gal1RGexp\": 0.369, \"gal80Rsig\": 0.00043149, \"Stress\": 20.0, \"id\": \"1735\", \"gal4RGexp\": -0.021, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"peroxisome organization and biogenesis\", \"protein targeting to peroxisome\"], \"Radiality\": 0.94444444, \"ClusteringCoefficient\": 0.3, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 5.0, \"Degree\": 5, \"SUID\": 1735}}, {\"position\": {\"y\": 304.0595717473884, \"x\": 2033.2780988991453}, \"selected\": false, \"data\": {\"id_original\": \"1941\", \"name\": \"YLR432W\", \"Eccentricity\": 22, \"IsSingleNode\": false, \"COMMON\": \"YLR432W\", \"selected\": false, \"BetweennessCentrality\": 0.01606373, \"gal1RGsig\": 6.6826e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"IMD3\", \"S000004424\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"IMP dehydrogenase activity\"], \"gal4RGsig\": 0.36112, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YLR432W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09668616, \"AverageShortestPathLength\": 10.34274194, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": -0.301, \"gal1RGexp\": -0.197, \"gal80Rsig\": 4.8207e-05, \"Stress\": 5116.0, \"id\": \"1734\", \"gal4RGexp\": -0.054, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"GTP biosynthetic process\"], \"Radiality\": 0.65397252, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1734}}, {\"position\": {\"y\": 187.48681784113842, \"x\": 1962.123481589575}, \"selected\": false, \"data\": {\"id_original\": \"1942\", \"name\": \"YDR167W\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"TAF25\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.00021837, \"annotation_GO_CELLULAR_COMPONENT\": [\"SAGA complex\", \"SLIK (SAGA-like) complex\", \"transcription factor TFIID complex\"], \"alias\": [\"TafII25\", \"S000002574\", \"TAF10\", \"TAF23\", \"TAF25\", \"TFIID subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"general RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 0.0039024, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YDR167W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08825623, \"AverageShortestPathLength\": 11.33064516, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": 0.355, \"gal1RGexp\": 0.246, \"gal80Rsig\": 0.01484, \"Stress\": 2560.0, \"id\": \"1733\", \"gal4RGexp\": -0.219, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"G1-specific transcription in mitotic cell cycle\", \"chromatin modification\", \"establishment and/or maintenance of chromatin architecture\", \"histone acetylation\", \"protein amino acid acetylation\", \"transcription initiation from RNA polymerase II promoter\"], \"Radiality\": 0.61738351, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1733}}, {\"position\": {\"y\": 749.2350478216072, \"x\": 2669.630058127661}, \"selected\": false, \"data\": {\"id_original\": \"1943\", \"name\": \"YLR175W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"CBF5\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.47804, \"annotation_GO_CELLULAR_COMPONENT\": [\"box H/ACA snoRNP complex\", \"nucleolus\"], \"alias\": [\"CBF5\", \"S000004165\", \"pseudouridine synthase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"pseudouridylate synthase activity\"], \"gal4RGsig\": 5.549e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLR175W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11221719, \"AverageShortestPathLength\": 8.91129032, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.498, \"gal1RGexp\": 0.038, \"gal80Rsig\": 1.4113e-07, \"Stress\": 0.0, \"id\": \"1732\", \"gal4RGexp\": -0.597, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"35S primary transcript processing\", \"rRNA pseudouridine synthesis\"], \"Radiality\": 0.70698925, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1732}}, {\"position\": {\"y\": 1500.0232787176033, \"x\": 2116.9473703682615}, \"selected\": false, \"data\": {\"id_original\": \"1944\", \"name\": \"YNL117W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"MLS1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.92e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"peroxisomal matrix\"], \"alias\": [\"MLS1\", \"S000005061\", \"carbon-catabolite sensitive malate synthase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"malate synthase activity\"], \"gal4RGsig\": 9.0335e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YNL117W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1302521, \"AverageShortestPathLength\": 7.67741935, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": 0.941, \"gal1RGexp\": 0.973, \"gal80Rsig\": 1.2597e-05, \"Stress\": 0.0, \"id\": \"1731\", \"gal4RGexp\": 0.452, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glyoxylate cycle\"], \"Radiality\": 0.75268817, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1731}}, {\"position\": {\"y\": 2193.0361952825447, \"x\": 3121.664054709692}, \"selected\": false, \"data\": {\"id_original\": \"1945\", \"name\": \"YOR089C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"VPS21\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00011748, \"annotation_GO_CELLULAR_COMPONENT\": [\"late endosome\", \"mitochondrial outer membrane\", \"mitochondrion\"], \"alias\": [\"S000005615\", \"VPS12\", \"VPS21\", \"VPT12\", \"YPT21\", \"YPT51\", \"small GTP-binding protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"GTPase activity\"], \"gal4RGsig\": 0.32501, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOR089C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.0977918, \"AverageShortestPathLength\": 10.22580645, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.378, \"gal1RGexp\": 0.193, \"gal80Rsig\": 8.2229e-06, \"Stress\": 0.0, \"id\": \"1730\", \"gal4RGexp\": -0.042, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"endocytosis\", \"protein targeting to vacuole\"], \"Radiality\": 0.65830346, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1730}}, {\"position\": {\"y\": 508.8234877630134, \"x\": 2456.327140647192}, \"selected\": false, \"data\": {\"id_original\": \"1946\", \"name\": \"YPR167C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"MET16\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.17278, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"intracellular\"], \"alias\": [\"3'phosphoadenylylsulfate reductase\", \"MET16\", \"S000006371\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"phosphoadenylyl-sulfate reductase (thioredoxin) activity\"], \"gal4RGsig\": 1.3067e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPR167C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11221719, \"AverageShortestPathLength\": 8.91129032, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.708, \"gal1RGexp\": -0.066, \"gal80Rsig\": 0.00053086, \"Stress\": 0.0, \"id\": \"1729\", \"gal4RGexp\": -1.034, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"methionine metabolic process\", \"sulfate assimilation\"], \"Radiality\": 0.70698925, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1729}}, {\"position\": {\"y\": 3539.4175276800056, \"x\": 1256.3644789040281}, \"selected\": false, \"data\": {\"id_original\": \"1947\", \"name\": \"YNL214W\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"PEX17\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.166, \"annotation_GO_CELLULAR_COMPONENT\": [\"peroxisomal membrane\"], \"alias\": [\"PAS9\", \"PEX17\", \"S000005158\", \"peroxin\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein binding\"], \"gal4RGsig\": 0.64313, \"TopologicalCoefficient\": 0.9, \"shared_name\": \"YNL214W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.54545455, \"AverageShortestPathLength\": 1.83333333, \"NeighborhoodConnectivity\": 4.5, \"gal80Rexp\": 0.199, \"gal1RGexp\": 0.122, \"gal80Rsig\": 0.11918, \"Stress\": 0.0, \"id\": \"1728\", \"gal4RGexp\": 0.041, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"peroxisome organization and biogenesis\"], \"Radiality\": 0.72222222, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1728}}, {\"position\": {\"y\": 1792.8090529485603, \"x\": 1506.2204511940672}, \"selected\": false, \"data\": {\"id_original\": \"1948\", \"name\": \"YBR135W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"CKS1\", \"selected\": false, \"BetweennessCentrality\": 0.05196364, \"gal1RGsig\": 0.0084321, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"CKS1\", \"Cdc28 protein kinase subunit\", \"S000000339\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein kinase activator activity\"], \"gal4RGsig\": 0.6558, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YBR135W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13073274, \"AverageShortestPathLength\": 7.64919355, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.057, \"gal1RGexp\": 0.108, \"gal80Rsig\": 0.34065, \"Stress\": 6576.0, \"id\": \"1727\", \"gal4RGexp\": -0.018, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of progression through cell cycle\", \"transcription\"], \"Radiality\": 0.75373357, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1727}}, {\"position\": {\"y\": 4107.79356909236, \"x\": 1075.0725782692625}, \"selected\": false, \"data\": {\"id_original\": \"1949\", \"name\": \"YML007W\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"YAP1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 9.2417e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"PAR1\", \"S000004466\", \"SNQ3\", \"YAP1\", \"jun-like transcription factor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcription factor activity\"], \"gal4RGsig\": 0.42858, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YML007W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.5, \"AverageShortestPathLength\": 2.0, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.331, \"gal1RGexp\": 0.359, \"gal80Rsig\": 0.0024709, \"Stress\": 0.0, \"id\": \"1726\", \"gal4RGexp\": -0.039, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"response to arsenic\", \"response to drug\", \"response to heat\", \"response to singlet oxygen\", \"transcription\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1726}}, {\"position\": {\"y\": 3998.0106558843518, \"x\": 1072.6479795754149}, \"selected\": false, \"data\": {\"id_original\": \"1950\", \"name\": \"YER110C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"KAP123\", \"selected\": false, \"BetweennessCentrality\": 0.66666667, \"gal1RGsig\": 0.26052, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nuclear pore\", \"nucleus\"], \"alias\": [\"KAP123\", \"S000000912\", \"YRB4\", \"karyopherin beta 4\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein carrier activity\"], \"gal4RGsig\": 0.00029645, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YER110C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.75, \"AverageShortestPathLength\": 1.33333333, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": 0.43, \"gal1RGexp\": 0.05, \"gal80Rsig\": 3.6344e-07, \"Stress\": 4.0, \"id\": \"1725\", \"gal4RGexp\": -0.233, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein import into nucleus\"], \"Radiality\": 0.88888889, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1725}}, {\"position\": {\"y\": 3605.231370453443, \"x\": 1149.665733176489}, \"selected\": false, \"data\": {\"id_original\": \"1951\", \"name\": \"YGL153W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"PEX14\", \"selected\": false, \"BetweennessCentrality\": 0.16666667, \"gal1RGsig\": 0.00012953, \"annotation_GO_CELLULAR_COMPONENT\": [\"peroxisomal membrane\"], \"alias\": [\"PEX14\", \"S000003121\", \"peroxin\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein binding\"], \"gal4RGsig\": 0.077941, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YGL153W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.75, \"AverageShortestPathLength\": 1.33333333, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.627, \"gal1RGexp\": 0.242, \"gal80Rsig\": 0.0023167, \"Stress\": 10.0, \"id\": \"1724\", \"gal4RGexp\": -0.132, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein import into peroxisome matrix\", \"protein targeting to peroxisome\"], \"Radiality\": 0.88888889, \"ClusteringCoefficient\": 0.5, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1724}}, {\"position\": {\"y\": 3612.3445296331306, \"x\": 1243.1416700661375}, \"selected\": false, \"data\": {\"id_original\": \"1952\", \"name\": \"YLR191W\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"PEX13\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00076925, \"annotation_GO_CELLULAR_COMPONENT\": [\"peroxisomal membrane\"], \"alias\": [\"PAS20\", \"PEX13\", \"S000004181\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein binding\"], \"gal4RGsig\": 0.58305, \"TopologicalCoefficient\": 0.9, \"shared_name\": \"YLR191W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.54545455, \"AverageShortestPathLength\": 1.83333333, \"NeighborhoodConnectivity\": 4.5, \"gal80Rexp\": 0.222, \"gal1RGexp\": 0.178, \"gal80Rsig\": 0.097987, \"Stress\": 0.0, \"id\": \"1723\", \"gal4RGexp\": -0.056, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"peroxisome organization and biogenesis\", \"protein import into peroxisome matrix\", \"protein targeting to peroxisome\"], \"Radiality\": 0.72222222, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1723}}, {\"position\": {\"y\": 2072.473268036451, \"x\": 1496.4283369362547}, \"selected\": false, \"data\": {\"id_original\": \"1953\", \"name\": \"YOL149W\", \"Eccentricity\": 26, \"IsSingleNode\": false, \"COMMON\": \"DCP1\", \"selected\": false, \"BetweennessCentrality\": 8.16e-06, \"gal1RGsig\": 9.0847e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasmic mRNA processing body\"], \"alias\": [\"DCP1\", \"S000005509\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"enzyme activator activity\", \"m7G(5')pppN diphosphatase activity\", \"mRNA binding\"], \"gal4RGsig\": 0.0015911, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YOL149W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.0763782, \"AverageShortestPathLength\": 13.09274194, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": 0.254, \"gal1RGexp\": 0.228, \"gal80Rsig\": 0.059331, \"Stress\": 2.0, \"id\": \"1722\", \"gal4RGexp\": -0.248, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"deadenylation-dependent decapping\", \"mRNA catabolic process\"], \"Radiality\": 0.55212067, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1722}}, {\"position\": {\"y\": 1033.4023451848884, \"x\": 1848.6805036843016}, \"selected\": false, \"data\": {\"id_original\": \"1954\", \"name\": \"YMR044W\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"YMR044W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 4.4526e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"ISW1 complex\"], \"alias\": [\"IOC4\", \"S000004647\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein binding\"], \"gal4RGsig\": 0.15055, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YMR044W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10450906, \"AverageShortestPathLength\": 9.56854839, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": 0.357, \"gal1RGexp\": 0.255, \"gal80Rsig\": 0.00088242, \"Stress\": 0.0, \"id\": \"1721\", \"gal4RGexp\": -0.093, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"chromatin remodeling\"], \"Radiality\": 0.68264636, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1721}}, {\"position\": {\"y\": 2021.1225600286384, \"x\": 987.877128440161}, \"selected\": false, \"data\": {\"id_original\": \"1955\", \"name\": \"YOR362C\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"PRE10\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.31634, \"annotation_GO_CELLULAR_COMPONENT\": [\"proteasome core complex\", \"alpha-subunit complex (sensu the Eukaryota research community)\"], \"alias\": [\"PRE10\", \"S000005889\", \"proteasome component YC1 (protease yscE subunit 1)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"endopeptidase activity\"], \"gal4RGsig\": 0.2984, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOR362C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09567901, \"AverageShortestPathLength\": 10.4516129, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": 0.225, \"gal1RGexp\": 0.036, \"gal80Rsig\": 0.0013356, \"Stress\": 0.0, \"id\": \"1720\", \"gal4RGexp\": -0.043, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ubiquitin-dependent protein catabolic process\"], \"Radiality\": 0.64994026, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1720}}, {\"position\": {\"y\": 1853.1281752630134, \"x\": 1274.4085005104735}, \"selected\": false, \"data\": {\"id_original\": \"1956\", \"name\": \"YER102W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"RPS8B\", \"selected\": false, \"BetweennessCentrality\": 0.04463374, \"gal1RGsig\": 3.2712e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic small ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPS8B\", \"S000000904\", \"ribosomal protein S8B (S14B) (rp19) (YS9)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 3.6609e-06, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YER102W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11687088, \"AverageShortestPathLength\": 8.55645161, \"NeighborhoodConnectivity\": 3.5, \"gal80Rexp\": -0.135, \"gal1RGexp\": -0.249, \"gal80Rsig\": 0.017595, \"Stress\": 5590.0, \"id\": \"1719\", \"gal4RGexp\": -0.364, \"Radiality\": 0.72013142, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1719}}, {\"position\": {\"y\": 1865.015260223951, \"x\": 961.326103049536}, \"selected\": false, \"data\": {\"id_original\": \"1957\", \"name\": \"YOL059W\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"GPD2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 3.3837e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\", \"mitochondrion\"], \"alias\": [\"GPD2\", \"GPD3\", \"S000005420\", \"glycerol-3-phosphate dehydrogenase (NAD+)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"glycerol-3-phosphate dehydrogenase (NAD+) activity\"], \"gal4RGsig\": 2.3802e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOL059W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09567901, \"AverageShortestPathLength\": 10.4516129, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.591, \"gal1RGexp\": -0.499, \"gal80Rsig\": 1.5256e-09, \"Stress\": 0.0, \"id\": \"1718\", \"gal4RGexp\": -0.29, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"NADH oxidation\", \"glycerol metabolic process\"], \"Radiality\": 0.64994026, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1718}}, {\"position\": {\"y\": 2475.908814911451, \"x\": 2119.138647872229}, \"selected\": false, \"data\": {\"id_original\": \"1958\", \"name\": \"YBR190W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"YBR190W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 4.514e-05, \"gal4RGsig\": 0.00038823, \"NumberOfDirectedEdges\": 1.0, \"shared_name\": \"YBR190W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10968598, \"AverageShortestPathLength\": 9.11693548, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.07, \"gal1RGexp\": -0.209, \"gal80Rsig\": 0.66515, \"Stress\": 0.0, \"id\": \"1717\", \"gal4RGexp\": -0.3, \"Radiality\": 0.69937276, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"TopologicalCoefficient\": 0.0, \"Degree\": 1, \"SUID\": 1717}}, {\"position\": {\"y\": 3028.887574677076, \"x\": 2167.755588370581}, \"selected\": false, \"data\": {\"id_original\": \"1959\", \"name\": \"YER103W\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"SSA4\", \"selected\": false, \"BetweennessCentrality\": 0.01606373, \"gal1RGsig\": 0.00012362, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"S000000905\", \"SSA4\", \"YG107\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"ATPase activity\", \"unfolded protein binding\"], \"gal4RGsig\": 0.013281, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YER103W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09383277, \"AverageShortestPathLength\": 10.65725806, \"NeighborhoodConnectivity\": 3.5, \"gal80Rexp\": -0.826, \"gal1RGexp\": -0.405, \"gal80Rsig\": 8.3702e-13, \"Stress\": 2016.0, \"id\": \"1716\", \"gal4RGexp\": 0.176, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"SRP-dependent cotranslational protein targeting to membrane\", \"translocation\", \"protein folding\", \"response to stress\"], \"Radiality\": 0.64232378, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1716}}, {\"position\": {\"y\": 4207.79356909236, \"x\": 2309.3936498940184}, \"selected\": false, \"data\": {\"id_original\": \"1960\", \"name\": \"YPR110C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"RPC40\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0019607, \"annotation_GO_CELLULAR_COMPONENT\": [\"DNA-directed RNA polymerase I complex\", \"DNA-directed RNA polymerase III complex\"], \"alias\": [\"RNA polymerase III subunit\", \"AC40\", \"RPC40\", \"RPC5\", \"S000006314\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA-directed RNA polymerase activity\"], \"gal4RGsig\": 1.2743e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPR110C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": -0.026, \"gal1RGexp\": -0.12, \"gal80Rsig\": 0.70564, \"Stress\": 0.0, \"id\": \"1715\", \"gal4RGexp\": -0.339, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"transcription from RNA polymerase I promoter\", \"transcription from RNA polymerase III promoter\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1715}}, {\"position\": {\"y\": 4315.51573706111, \"x\": 2306.376197654028}, \"selected\": false, \"data\": {\"id_original\": \"1961\", \"name\": \"YNL113W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"RPC19\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.0276e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"DNA-directed RNA polymerase I complex\", \"DNA-directed RNA polymerase III complex\"], \"alias\": [\"RNA polymerases I (A) and III (C) subunit\", \"AC19\", \"RPC19\", \"S000005057\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA-directed RNA polymerase activity\"], \"gal4RGsig\": 4.8347e-07, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YNL113W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.789, \"gal1RGexp\": 0.304, \"gal80Rsig\": 0.0028937, \"Stress\": 0.0, \"id\": \"1714\", \"gal4RGexp\": -0.979, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"transcription from RNA polymerase I promoter\", \"transcription from RNA polymerase III promoter\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1714}}, {\"position\": {\"y\": 1119.4570632024665, \"x\": 2227.694595176001}, \"selected\": false, \"data\": {\"id_original\": \"1962\", \"name\": \"YDR354W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"TRP4\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0090277, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"S000002762\", \"TRP4\", \"anthranilate phosphoribosyl transferase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"anthranilate phosphoribosyltransferase activity\"], \"gal4RGsig\": 0.0028196, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDR354W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11605054, \"AverageShortestPathLength\": 8.61693548, \"NeighborhoodConnectivity\": 7.0, \"gal80Rexp\": -0.253, \"gal1RGexp\": -0.122, \"gal80Rsig\": 0.0012089, \"Stress\": 0.0, \"id\": \"1713\", \"gal4RGexp\": -0.202, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"tryptophan biosynthetic process\"], \"Radiality\": 0.71789128, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1713}}, {\"position\": {\"y\": 4207.79356909236, \"x\": 2206.376197654028}, \"selected\": false, \"data\": {\"id_original\": \"1963\", \"name\": \"YER090W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"TRP2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.079619, \"annotation_GO_CELLULAR_COMPONENT\": [\"anthranilate synthase complex\", \"cytoplasm\"], \"alias\": [\"S000000892\", \"TRP2\", \"anthranilate synthase component I\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"anthranilate synthase activity\"], \"gal4RGsig\": 1.9673e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YER090W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.231, \"gal1RGexp\": -0.067, \"gal80Rsig\": 0.0015768, \"Stress\": 0.0, \"id\": \"1712\", \"gal4RGexp\": -0.38, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"tryptophan biosynthetic process\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1712}}, {\"position\": {\"y\": 4315.51573706111, \"x\": 2203.358745414038}, \"selected\": false, \"data\": {\"id_original\": \"1964\", \"name\": \"YKL211C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"TRP3\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00016589, \"annotation_GO_CELLULAR_COMPONENT\": [\"anthranilate synthase complex\", \"cytoplasm\"], \"alias\": [\"indole-3-phosphate\", \"S000001694\", \"TRP3\", \"anthranilate synthase component II\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"anthranilate synthase activity\", \"indole-3-glycerol-phosphate synthase activity\"], \"gal4RGsig\": 4.2736e-07, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YKL211C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.358, \"gal1RGexp\": -0.183, \"gal80Rsig\": 0.00015264, \"Stress\": 0.0, \"id\": \"1711\", \"gal4RGexp\": -0.6, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"tryptophan biosynthetic process\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1711}}, {\"position\": {\"y\": 1652.9908080144783, \"x\": 2190.9085043251707}, \"selected\": false, \"data\": {\"id_original\": \"1965\", \"name\": \"YDR146C\", \"Eccentricity\": 14, \"IsSingleNode\": false, \"COMMON\": \"SWI5\", \"selected\": false, \"BetweennessCentrality\": 0.3090621, \"gal1RGsig\": 0.0018541, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"S000002553\", \"SWI5\", \"transcriptional activator\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcriptional activator activity\"], \"gal4RGsig\": 0.1065, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YDR146C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.16939891, \"AverageShortestPathLength\": 5.90322581, \"NeighborhoodConnectivity\": 14.5, \"gal80Rexp\": -0.027, \"gal1RGexp\": -0.19, \"gal80Rsig\": 0.76249, \"Stress\": 66656.0, \"id\": \"1710\", \"gal4RGexp\": 0.102, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"G1-specific transcription in mitotic cell cycle\"], \"Radiality\": 0.81839904, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1710}}, {\"position\": {\"y\": 1824.7173781438728, \"x\": 2273.6905134499266}, \"selected\": false, \"data\": {\"id_original\": \"1966\", \"name\": \"YER111C\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"SWI4\", \"selected\": false, \"BetweennessCentrality\": 0.0323843, \"gal1RGsig\": 0.00056134, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"ART1\", \"S000000913\", \"SWI4\", \"transcription factor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA binding\", \"transcription factor activity\"], \"gal4RGsig\": 0.33211, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YER111C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.15158924, \"AverageShortestPathLength\": 6.59677419, \"NeighborhoodConnectivity\": 11.0, \"gal80Rexp\": 0.16, \"gal1RGexp\": 0.195, \"gal80Rsig\": 0.15558, \"Stress\": 3188.0, \"id\": \"1709\", \"gal4RGexp\": -0.105, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"G1/S transition of mitotic cell cycle\", \"transcription\"], \"Radiality\": 0.79271207, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1709}}, {\"position\": {\"y\": 3908.0143752141857, \"x\": 2025.1792372048094}, \"selected\": false, \"data\": {\"id_original\": \"1967\", \"name\": \"YOR039W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"CKB2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.87354, \"annotation_GO_CELLULAR_COMPONENT\": [\"protein kinase CK2 complex\"], \"alias\": [\"beta' subunit\", \"CKB2\", \"S000005565\", \"protein kinase CK2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein kinase CK2 regulator activity\"], \"gal4RGsig\": 0.20402, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOR039W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.006, \"gal1RGexp\": -0.006, \"gal80Rsig\": 0.92034, \"Stress\": 0.0, \"id\": \"1708\", \"gal4RGexp\": -0.058, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"G1/S transition of mitotic cell cycle\", \"G2/M transition of mitotic cell cycle\", \"cell ion homeostasis\", \"establishment of cell polarity (sensu the Fungi research community)\", \"flocculation via cell wall protein-carbohydrate interaction\", \"protein amino acid phosphorylation\", \"regulation of transcription from RNA polymerase I promoter\", \"regulation of transcription from RNA polymerase III promoter\", \"response to DNA damage stimulus\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1708}}, {\"position\": {\"y\": 999.5197157903572, \"x\": 2163.048110801001}, \"selected\": false, \"data\": {\"id_original\": \"1968\", \"name\": \"YML024W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"RPS17A\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 6.9725e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic small ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RP51A\", \"RPL51A\", \"RPS17A\", \"S000004486\", \"ribosomal protein S17A (rp51A)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 1.5459e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YML024W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13724405, \"AverageShortestPathLength\": 7.28629032, \"NeighborhoodConnectivity\": 17.0, \"gal80Rexp\": -0.864, \"gal1RGexp\": -0.551, \"gal80Rsig\": 1.3115e-12, \"Stress\": 0.0, \"id\": \"1707\", \"gal4RGexp\": -0.454, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ribosomal small subunit assembly and maintenance\", \"telomere maintenance\"], \"Radiality\": 0.76717443, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1707}}, {\"position\": {\"y\": 1945.4779982610603, \"x\": 2609.082755881567}, \"selected\": false, \"data\": {\"id_original\": \"1969\", \"name\": \"YIL113W\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"YIL113W\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.002113, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"S000001375\", \"SDP1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"MAP kinase phosphatase activity\"], \"gal4RGsig\": 0.002831, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YIL113W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12487412, \"AverageShortestPathLength\": 8.00806452, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": -0.137, \"gal1RGexp\": -0.236, \"gal80Rsig\": 0.23739, \"Stress\": 746.0, \"id\": \"1706\", \"gal4RGexp\": 0.211, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"MAPKKK cascade during cell wall biogenesis\"], \"Radiality\": 0.74044205, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1706}}, {\"position\": {\"y\": 2004.7683424993415, \"x\": 2717.0452802956297}, \"selected\": false, \"data\": {\"id_original\": \"1970\", \"name\": \"YLL019C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"KNS1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.3809e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cellular_component\"], \"alias\": [\"KNS1\", \"L124\", \"S000003942\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein serine/threonine kinase activity\", \"protein-tyrosine kinase activity\"], \"gal4RGsig\": 6.085e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLL019C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11106135, \"AverageShortestPathLength\": 9.00403226, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.295, \"gal1RGexp\": 0.258, \"gal80Rsig\": 9.3514e-05, \"Stress\": 0.0, \"id\": \"1705\", \"gal4RGexp\": 0.215, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein amino acid phosphorylation\"], \"Radiality\": 0.70355436, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1705}}, {\"position\": {\"y\": 1485.2927565618415, \"x\": 2467.602714377661}, \"selected\": false, \"data\": {\"id_original\": \"1971\", \"name\": \"YDR009W\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"GAL3\", \"selected\": false, \"BetweennessCentrality\": 0.00214797, \"gal1RGsig\": 0.01257, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"GAL3\", \"S000002416\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcriptional activator activity\"], \"gal4RGsig\": 6.9834e-08, \"TopologicalCoefficient\": 0.6, \"shared_name\": \"YDR009W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14614025, \"AverageShortestPathLength\": 6.84274194, \"NeighborhoodConnectivity\": 7.0, \"gal80Rexp\": 0.162, \"gal1RGexp\": -0.111, \"gal80Rsig\": 0.16498, \"Stress\": 816.0, \"id\": \"1704\", \"gal4RGexp\": -1.004, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"galactose metabolic process\", \"positive regulation of transcription by galactose\"], \"Radiality\": 0.78360215, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1704}}, {\"position\": {\"y\": 1608.7494673772712, \"x\": 2499.776756125708}, \"selected\": false, \"data\": {\"id_original\": \"1972\", \"name\": \"YML051W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"GAL80\", \"selected\": false, \"BetweennessCentrality\": 0.00015998, \"gal1RGsig\": 0.080275, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"GAL80\", \"S000004515\", \"transcriptional regulator\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"specific transcriptional repressor activity\"], \"gal4RGsig\": 4.3863e-08, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YML051W\", \"PartnerOfMultiEdgedNodePairs\": 1.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13121693, \"AverageShortestPathLength\": 7.62096774, \"NeighborhoodConnectivity\": 5.33333333, \"gal80Rexp\": -1.167, \"gal1RGexp\": -0.07, \"gal80Rsig\": 8.1952e-17, \"Stress\": 26.0, \"id\": \"1703\", \"gal4RGexp\": -0.606, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"galactose metabolic process\", \"positive regulation of transcription by galactose\"], \"Radiality\": 0.75477897, \"ClusteringCoefficient\": 0.33333333, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1703}}, {\"position\": {\"y\": 2539.9719862981697, \"x\": 3169.713554221411}, \"selected\": false, \"data\": {\"id_original\": \"1973\", \"name\": \"YHR071W\", \"Eccentricity\": 25, \"IsSingleNode\": false, \"COMMON\": \"PCL5\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 5.0065e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"cyclin-dependent protein kinase holoenzyme complex\"], \"alias\": [\"PCL5\", \"S000001113\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"cyclin-dependent protein kinase regulator activity\"], \"gal4RGsig\": 4.2811e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YHR071W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.06921574, \"AverageShortestPathLength\": 14.44758065, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.499, \"gal1RGexp\": -0.614, \"gal80Rsig\": 6.8054e-08, \"Stress\": 0.0, \"id\": \"1702\", \"gal4RGexp\": -0.67, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell cycle\"], \"Radiality\": 0.50194146, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1702}}, {\"position\": {\"y\": 2596.840943817701, \"x\": 3066.897697287817}, \"selected\": false, \"data\": {\"id_original\": \"1974\", \"name\": \"YPL031C\", \"Eccentricity\": 24, \"IsSingleNode\": false, \"COMMON\": \"PHO85\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.012838, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"LDB15\", \"PHO85\", \"S000005952\", \"cyclin-dependent protein kinase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"cyclin-dependent protein kinase activity\"], \"gal4RGsig\": 0.0056375, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YPL031C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.07434053, \"AverageShortestPathLength\": 13.4516129, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": -0.139, \"gal1RGexp\": -0.106, \"gal80Rsig\": 0.019984, \"Stress\": 1970.0, \"id\": \"1701\", \"gal4RGexp\": 0.125, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell cycle\", \"glycogen metabolic process\", \"phosphate metabolic process\", \"protein amino acid phosphorylation\", \"telomere maintenance\"], \"Radiality\": 0.53882915, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1701}}, {\"position\": {\"y\": 600.5227065130134, \"x\": 2628.748924094458}, \"selected\": false, \"data\": {\"id_original\": \"1975\", \"name\": \"YML123C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"PHO84\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0015163, \"annotation_GO_CELLULAR_COMPONENT\": [\"integral to plasma membrane\"], \"alias\": [\"PHO84\", \"S000004592\", \"inorganic phosphate transporter\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"inorganic phosphate transporter activity\", \"manganese ion transporter activity\"], \"gal4RGsig\": 0.019412, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YML123C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.108061, \"AverageShortestPathLength\": 9.25403226, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.692, \"gal1RGexp\": 0.283, \"gal80Rsig\": 6.9606e-07, \"Stress\": 0.0, \"id\": \"1700\", \"gal4RGexp\": -0.114, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"manganese ion transport\", \"phosphate transport\"], \"Radiality\": 0.6942951, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1700}}, {\"position\": {\"y\": 4207.79356909236, \"x\": 2103.358745414038}, \"selected\": false, \"data\": {\"id_original\": \"1976\", \"name\": \"YER145C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"FTR1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 6.6247e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"plasma membrane\"], \"alias\": [\"FTR1\", \"S000000947\", \"iron permease\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"iron ion transporter activity\"], \"gal4RGsig\": 6.332e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YER145C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.868, \"gal1RGexp\": 0.647, \"gal80Rsig\": 5.9656e-07, \"Stress\": 0.0, \"id\": \"1699\", \"gal4RGexp\": -0.78, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"high affinity iron ion transport\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1699}}, {\"position\": {\"y\": 4315.51573706111, \"x\": 2100.3412931740477}, \"selected\": false, \"data\": {\"id_original\": \"1977\", \"name\": \"YMR058W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"FET3\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 3.6962e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"plasma membrane\"], \"alias\": [\"FET3\", \"S000004662\", \"multicopper oxidase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"ferroxidase activity\", \"iron ion transporter activity\"], \"gal4RGsig\": 0.00047738, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YMR058W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.64, \"gal1RGexp\": 0.449, \"gal80Rsig\": 1.6005e-08, \"Stress\": 0.0, \"id\": \"1698\", \"gal4RGexp\": -0.293, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"high affinity iron ion transport\", \"response to copper ion\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1698}}, {\"position\": {\"y\": 4207.79356909236, \"x\": 2000.3412931740477}, \"selected\": false, \"data\": {\"id_original\": \"1978\", \"name\": \"YJL190C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"RPS22A\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.0479e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic small ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPS22A\", \"RPS24\", \"S000003726\", \"ribosomal protein S22A (S24A) (rp50) (YS22)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 1.8121e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YJL190C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": -0.184, \"gal1RGexp\": -0.444, \"gal80Rsig\": 0.0020712, \"Stress\": 0.0, \"id\": \"1697\", \"gal4RGexp\": -0.443, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"telomere maintenance\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1697}}, {\"position\": {\"y\": 4315.51573706111, \"x\": 1997.3238409340574}, \"selected\": false, \"data\": {\"id_original\": \"1979\", \"name\": \"YML074C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"NPI46\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.02495, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleolus\"], \"alias\": [\"FPR3\", \"NPI46\", \"S000004539\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"peptidyl-prolyl cis-trans isomerase activity\"], \"gal4RGsig\": 5.2953e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YML074C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.389, \"gal1RGexp\": 0.118, \"gal80Rsig\": 3.3233e-05, \"Stress\": 0.0, \"id\": \"1696\", \"gal4RGexp\": -0.38, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"meiotic recombination checkpoint\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1696}}, {\"position\": {\"y\": 731.2512221380134, \"x\": 2379.830253440161}, \"selected\": false, \"data\": {\"id_original\": \"1980\", \"name\": \"YOR355W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"GDS1\", \"selected\": false, \"BetweennessCentrality\": 0.03283069, \"gal1RGsig\": 0.00016613, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"mitochondrion\", \"nucleus\"], \"alias\": [\"GDS1\", \"S000005882\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.45777, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YOR355W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12393803, \"AverageShortestPathLength\": 8.06854839, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.43, \"gal1RGexp\": -0.176, \"gal80Rsig\": 0.0062666, \"Stress\": 4248.0, \"id\": \"1695\", \"gal4RGexp\": -0.044, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"aerobic respiration\"], \"Radiality\": 0.73820191, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1695}}, {\"position\": {\"y\": 2037.171052460279, \"x\": 3003.6543501198485}, \"selected\": false, \"data\": {\"id_original\": \"1981\", \"name\": \"YFL038C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"YPT1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.056883, \"annotation_GO_CELLULAR_COMPONENT\": [\"Golgi membrane\", \"endoplasmic reticulum membrane\", \"mitochondrion\"], \"alias\": [\"GTP-binding protein\", \"S000001856\", \"YP2\", \"YPT1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"GTPase activity\"], \"gal4RGsig\": 0.44841, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YFL038C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10824967, \"AverageShortestPathLength\": 9.23790323, \"NeighborhoodConnectivity\": 6.0, \"gal80Rexp\": 0.12, \"gal1RGexp\": 0.075, \"gal80Rsig\": 0.073548, \"Stress\": 0.0, \"id\": \"1694\", \"gal4RGexp\": -0.033, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ER to Golgi vesicle-mediated transport\", \"protein complex assembly\"], \"Radiality\": 0.69489247, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1694}}, {\"position\": {\"y\": 1447.872193817701, \"x\": 2285.0920790016844}, \"selected\": false, \"data\": {\"id_original\": \"1982\", \"name\": \"YIL162W\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"SUC2\", \"selected\": false, \"BetweennessCentrality\": 0.01410579, \"gal1RGsig\": 4.1442e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"extracellular region\", \"mitochondrion\"], \"alias\": [\"S000001424\", \"SUC2\", \"invertase (sucrose hydrolyzing enzyme)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"beta-fructofuranosidase activity\"], \"gal4RGsig\": 1.7862e-09, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YIL162W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14735591, \"AverageShortestPathLength\": 6.78629032, \"NeighborhoodConnectivity\": 6.5, \"gal80Rexp\": -1.131, \"gal1RGexp\": -0.318, \"gal80Rsig\": 1.7403e-20, \"Stress\": 2426.0, \"id\": \"1693\", \"gal4RGexp\": 0.688, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"sucrose catabolic process\"], \"Radiality\": 0.78569295, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1693}}, {\"position\": {\"y\": 2751.732545380201, \"x\": 2154.9682939827635}, \"selected\": false, \"data\": {\"id_original\": \"1983\", \"name\": \"YBR050C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"REG2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 6.914e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"protein phosphatase type 1 complex\"], \"alias\": [\"Glc7p regulatory subunit\", \"REG2\", \"S000000254\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein phosphatase type 1 regulator activity\"], \"gal4RGsig\": 2.9767e-08, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YBR050C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11216644, \"AverageShortestPathLength\": 8.91532258, \"NeighborhoodConnectivity\": 6.0, \"gal80Rexp\": 0.122, \"gal1RGexp\": 0.432, \"gal80Rsig\": 0.28411, \"Stress\": 0.0, \"id\": \"1692\", \"gal4RGexp\": 0.679, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"negative regulation of transcription from RNA polymerase II promoter\"], \"Radiality\": 0.7068399, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1692}}, {\"position\": {\"y\": 2718.0195326848884, \"x\": 2060.3063047707274}, \"selected\": false, \"data\": {\"id_original\": \"1984\", \"name\": \"YMR311C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"GLC8\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0015202, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"GLC8\", \"S000004928\", \"protein phosphatase 1 (Glc7p) regulator\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"enzyme activator activity\"], \"gal4RGsig\": 0.64469, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YMR311C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11216644, \"AverageShortestPathLength\": 8.91532258, \"NeighborhoodConnectivity\": 6.0, \"gal80Rexp\": 0.349, \"gal1RGexp\": 0.214, \"gal80Rsig\": 6.5604e-06, \"Stress\": 0.0, \"id\": \"1691\", \"gal4RGexp\": 0.021, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"chromosome segregation\", \"glycogen biosynthetic process\"], \"Radiality\": 0.7068399, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1691}}, {\"position\": {\"y\": 2580.569703583326, \"x\": 2238.683914023657}, \"selected\": false, \"data\": {\"id_original\": \"1985\", \"name\": \"YOR315W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"YOR315W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.13594, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"S000005842\", \"SFG1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"specific RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 4.7159e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOR315W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11216644, \"AverageShortestPathLength\": 8.91532258, \"NeighborhoodConnectivity\": 6.0, \"gal80Rexp\": -0.063, \"gal1RGexp\": 0.066, \"gal80Rsig\": 0.50938, \"Stress\": 0.0, \"id\": \"1690\", \"gal4RGexp\": -0.462, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"positive regulation of transcription from RNA polymerase II promoter\", \"pseudohyphal growth\"], \"Radiality\": 0.7068399, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1690}}, {\"position\": {\"y\": 2778.807252411451, \"x\": 2283.217201071997}, \"selected\": false, \"data\": {\"id_original\": \"1986\", \"name\": \"YOR178C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"GAC1\", \"selected\": false, \"BetweennessCentrality\": 0.11450307, \"gal1RGsig\": 0.0010176, \"annotation_GO_CELLULAR_COMPONENT\": [\"protein phosphatase type 1 complex\"], \"alias\": [\"GAC1\", \"Glc7p regulatory subunit\", \"S000005704\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"heat shock protein binding\", \"protein phosphatase type 1 regulator activity\", \"structural molecule activity\"], \"gal4RGsig\": 1.5883e-06, \"TopologicalCoefficient\": 0.36666667, \"shared_name\": \"YOR178C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1143384, \"AverageShortestPathLength\": 8.74596774, \"NeighborhoodConnectivity\": 4.66666667, \"gal80Rexp\": -0.187, \"gal1RGexp\": -0.137, \"gal80Rsig\": 0.0036854, \"Stress\": 22434.0, \"id\": \"1689\", \"gal4RGexp\": 0.462, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glycogen metabolic process\", \"meiosis\", \"mitotic spindle checkpoint\", \"negative regulation of transcription from RNA polymerase II promoter\", \"response to heat\"], \"Radiality\": 0.71311231, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1689}}, {\"position\": {\"y\": 2635.9509291692634, \"x\": 2141.9547193825438}, \"selected\": false, \"data\": {\"id_original\": \"1987\", \"name\": \"YER133W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"GLC7\", \"selected\": false, \"BetweennessCentrality\": 0.19096905, \"gal1RGsig\": 0.20733, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud neck\", \"mRNA cleavage and polyadenylation specificity factor complex\", \"mating projection base\", \"nucleolus\", \"protein phosphatase type 1 complex\", \"spindle pole body\"], \"alias\": [\"CID1\", \"DIS2\", \"DIS2S1\", \"GLC7\", \"PP1\", \"S000000935\", \"protein phosphatase type I\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein phosphatase type 1 activity\"], \"gal4RGsig\": 0.063996, \"TopologicalCoefficient\": 0.1875, \"shared_name\": \"YER133W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12627291, \"AverageShortestPathLength\": 7.91935484, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": -0.051, \"gal1RGexp\": 0.051, \"gal80Rsig\": 0.36616, \"Stress\": 38412.0, \"id\": \"1688\", \"gal4RGexp\": -0.085, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"35S primary transcript processing\", \"cell budding\", \"cell ion homeostasis\", \"cellular morphogenesis during vegetative growth\", \"chromosome segregation\", \"glycogen metabolic process\", \"histone dephosphorylation\", \"meiosis\", \"mitotic spindle checkpoint\", \"protein amino acid dephosphorylation\", \"regulation of carbohydrate metabolic process\", \"regulation of progression through cell cycle\", \"response to heat\", \"sporulation (sensu the Fungi research community)\", \"transcription termination from Pol II promoter\", \"RNA polymerase(A) coupled\", \"transcription termination from Pol II promoter\", \"RNA polymerase(A)-independent\"], \"Radiality\": 0.7437276, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 6.0, \"Degree\": 6, \"SUID\": 1688}}, {\"position\": {\"y\": 4207.79356909236, \"x\": 1897.3238409340574}, \"selected\": false, \"data\": {\"id_original\": \"1988\", \"name\": \"YOR290C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"SNF2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0030289, \"annotation_GO_CELLULAR_COMPONENT\": [\"SWI/SNF complex\", \"chromatin remodeling complex\"], \"alias\": [\"GAM1\", \"HAF1\", \"S000005816\", \"SNF2\", \"SWI2\", \"TYE3\", \"transcriptional regulator\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA-dependent ATPase activity\", \"general RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 0.029664, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOR290C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": -0.577, \"gal1RGexp\": -0.119, \"gal80Rsig\": 3.6284e-13, \"Stress\": 0.0, \"id\": \"1687\", \"gal4RGexp\": -0.101, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"chromatin remodeling\", \"double-strand break repair\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1687}}, {\"position\": {\"y\": 4315.51573706111, \"x\": 1894.3063886940672}, \"selected\": false, \"data\": {\"id_original\": \"1989\", \"name\": \"YFR037C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"RSC8\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.5078e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"RSC complex\", \"nucleus\"], \"alias\": [\"RSC8\", \"S000001933\", \"SWH3\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.013849, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YFR037C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.35, \"gal1RGexp\": 0.297, \"gal80Rsig\": 0.0035541, \"Stress\": 0.0, \"id\": \"1686\", \"gal4RGexp\": -0.226, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"chromatin remodeling\", \"double-strand break repair via nonhomologous end joining\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1686}}, {\"position\": {\"y\": 685.9437270208259, \"x\": 2530.938163596411}, \"selected\": false, \"data\": {\"id_original\": \"1990\", \"name\": \"YFR034C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"PHO4\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.099513, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"PHO4\", \"S000001930\", \"myc-family transcription factor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcription factor activity\"], \"gal4RGsig\": 0.00069671, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YFR034C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12109375, \"AverageShortestPathLength\": 8.25806452, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": 0.348, \"gal1RGexp\": 0.121, \"gal80Rsig\": 0.020802, \"Stress\": 1048.0, \"id\": \"1685\", \"gal4RGexp\": -0.336, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cellular response to phosphate starvation\", \"phosphate metabolic process\"], \"Radiality\": 0.7311828, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1685}}, {\"position\": {\"y\": 1777.4368147893806, \"x\": 2164.1495703041746}, \"selected\": false, \"data\": {\"id_original\": \"1991\", \"name\": \"YAL040C\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"CLN3\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.66515, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"CLN3\", \"DAF1\", \"FUN10\", \"G1 cyclin\", \"S000000038\", \"WHI1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"cyclin-dependent protein kinase regulator activity\"], \"gal4RGsig\": 0.0017369, \"TopologicalCoefficient\": 0.55, \"shared_name\": \"YAL040C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.15085158, \"AverageShortestPathLength\": 6.62903226, \"NeighborhoodConnectivity\": 11.0, \"gal80Rexp\": 0.303, \"gal1RGexp\": -0.027, \"gal80Rsig\": 0.0010074, \"Stress\": 0.0, \"id\": \"1684\", \"gal4RGexp\": -0.206, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"G1/S transition of mitotic cell cycle\", \"regulation of cell size\", \"regulation of cyclin-dependent protein kinase activity\", \"vacuole fusion\", \"non-autophagic\", \"vacuole organization and biogenesis\"], \"Radiality\": 0.79151732, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1684}}, {\"position\": {\"y\": 4207.79356909236, \"x\": 943.4944380104735}, \"selected\": false, \"data\": {\"id_original\": \"1992\", \"name\": \"YPL222W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"YPL222W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 6.9654e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"mitochondrion\"], \"alias\": [\"S000006143\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.00013673, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPL222W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.514, \"gal1RGexp\": 0.344, \"gal80Rsig\": 4.6216e-05, \"Stress\": 0.0, \"id\": \"1683\", \"gal4RGexp\": 0.284, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1683}}, {\"position\": {\"y\": 4249.981992249098, \"x\": 1042.6931379616453}, \"selected\": false, \"data\": {\"id_original\": \"1993\", \"name\": \"YGR048W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"UFD1\", \"selected\": false, \"BetweennessCentrality\": 1.0, \"gal1RGsig\": 0.696, \"annotation_GO_CELLULAR_COMPONENT\": [\"endoplasmic reticulum\"], \"alias\": [\"S000003280\", \"UFD1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein binding\"], \"gal4RGsig\": 0.028001, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGR048W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.364, \"gal1RGexp\": 0.017, \"gal80Rsig\": 0.0043651, \"Stress\": 2.0, \"id\": \"1682\", \"gal4RGexp\": -0.155, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mRNA processing\", \"protein transport\", \"ubiquitin-dependent protein catabolic process\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1682}}, {\"position\": {\"y\": 3872.974538330641, \"x\": 2318.8552320778563}, \"selected\": false, \"data\": {\"id_original\": \"1994\", \"name\": \"YMR291W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"YMR291W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 7.9783e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"S000004905\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein kinase activity\"], \"gal4RGsig\": 0.012617, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YMR291W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.397, \"gal1RGexp\": -0.363, \"gal80Rsig\": 5.3037e-09, \"Stress\": 0.0, \"id\": \"1681\", \"gal4RGexp\": 0.256, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1681}}, {\"position\": {\"y\": 2263.4270644231697, \"x\": 2510.529929953833}, \"selected\": false, \"data\": {\"id_original\": \"1995\", \"name\": \"YGR009C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"SEC9\", \"selected\": false, \"BetweennessCentrality\": 0.09214253, \"gal1RGsig\": 2.9579e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"SNARE complex\", \"extrinsic to plasma membrane\"], \"alias\": [\"HSS7\", \"S000003241\", \"SEC9\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"t-SNARE activity\"], \"gal4RGsig\": 0.017416, \"TopologicalCoefficient\": 0.26666667, \"shared_name\": \"YGR009C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10359231, \"AverageShortestPathLength\": 9.65322581, \"NeighborhoodConnectivity\": 1.8, \"gal80Rexp\": 0.302, \"gal1RGexp\": 0.236, \"gal80Rsig\": 0.00044067, \"Stress\": 10322.0, \"id\": \"1680\", \"gal4RGexp\": -0.185, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Golgi to plasma membrane transport\", \"vesicle fusion\"], \"Radiality\": 0.67951016, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 5.0, \"Degree\": 5, \"SUID\": 1680}}, {\"position\": {\"y\": 2373.1360487981697, \"x\": 2465.6531599343016}, \"selected\": false, \"data\": {\"id_original\": \"1996\", \"name\": \"YMR183C\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"SSO2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 2.1741e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"endoplasmic reticulum\", \"integral to plasma membrane\"], \"alias\": [\"S000004795\", \"SSO2\", \"t-SNARE\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"t-SNARE activity\"], \"gal4RGsig\": 0.0021146, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YMR183C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09390382, \"AverageShortestPathLength\": 10.64919355, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.825, \"gal1RGexp\": -0.822, \"gal80Rsig\": 1.0306e-16, \"Stress\": 0.0, \"id\": \"1679\", \"gal4RGexp\": 0.256, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Golgi to plasma membrane transport\", \"vesicle fusion\"], \"Radiality\": 0.64262246, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1679}}, {\"position\": {\"y\": 2182.664247040357, \"x\": 2853.0920942604735}, \"selected\": false, \"data\": {\"id_original\": \"1997\", \"name\": \"YDR100W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"YDR100W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.86711, \"annotation_GO_CELLULAR_COMPONENT\": [\"COPI-coated vesicle\", \"integral to Golgi membrane\"], \"alias\": [\"S000002507\", \"TVP15\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 1.3164e-05, \"TopologicalCoefficient\": 0.66666667, \"shared_name\": \"YDR100W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10829694, \"AverageShortestPathLength\": 9.23387097, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": -0.415, \"gal1RGexp\": -0.007, \"gal80Rsig\": 5.7948e-10, \"Stress\": 0.0, \"id\": \"1678\", \"gal4RGexp\": 0.275, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.69504182, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1678}}, {\"position\": {\"y\": 2177.317811493482, \"x\": 2948.4241255104735}, \"selected\": false, \"data\": {\"id_original\": \"1998\", \"name\": \"YGL161C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"YGL161C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.053915, \"annotation_GO_CELLULAR_COMPONENT\": [\"membrane\"], \"alias\": [\"S000003129\", \"YIP5\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"Rab GTPase binding\"], \"gal4RGsig\": 0.00089167, \"TopologicalCoefficient\": 0.66666667, \"shared_name\": \"YGL161C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10829694, \"AverageShortestPathLength\": 9.23387097, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": -0.164, \"gal1RGexp\": -0.07, \"gal80Rsig\": 0.0065587, \"Stress\": 0.0, \"id\": \"1677\", \"gal4RGexp\": 0.172, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"vesicle-mediated transport\"], \"Radiality\": 0.69504182, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1677}}, {\"position\": {\"y\": 4207.79356909236, \"x\": 1794.3063886940672}, \"selected\": false, \"data\": {\"id_original\": \"1999\", \"name\": \"YPL131W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"RPL5\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.0523e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"LPI14\", \"RPL1\", \"RPL5\", \"S000006052\", \"ribosomal protein L5 (L1a)(YL3)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\", \"structural constituent of ribosome\"], \"gal4RGsig\": 0.0049489, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPL131W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": -0.246, \"gal1RGexp\": -0.228, \"gal80Rsig\": 0.00014759, \"Stress\": 0.0, \"id\": \"1676\", \"gal4RGexp\": -0.149, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ribosomal large subunit assembly and maintenance\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1676}}, {\"position\": {\"y\": 4315.51573706111, \"x\": 1791.288936454077}, \"selected\": false, \"data\": {\"id_original\": \"2000\", \"name\": \"YDL063C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"YDL063C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.055312, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"S000002221\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.0023991, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDL063C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.971, \"gal1RGexp\": 0.093, \"gal80Rsig\": 0.0013668, \"Stress\": 0.0, \"id\": \"1675\", \"gal4RGexp\": -0.755, \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1675}}, {\"position\": {\"y\": 1348.6572737737556, \"x\": 2302.7601393044188}, \"selected\": false, \"data\": {\"id_original\": \"2001\", \"name\": \"YNL167C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"SKO1\", \"selected\": false, \"BetweennessCentrality\": 0.00818695, \"gal1RGsig\": 0.024036, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\", \"nucleus\"], \"alias\": [\"ACR1\", \"S000005111\", \"SKO1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 0.079913, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YNL167C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13052632, \"AverageShortestPathLength\": 7.66129032, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.18, \"gal1RGexp\": 0.095, \"gal80Rsig\": 0.015501, \"Stress\": 1474.0, \"id\": \"1674\", \"gal4RGexp\": 0.1, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"negative regulation of transcription from RNA polymerase II promoter\"], \"Radiality\": 0.75328554, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1674}}, {\"position\": {\"y\": 3860.189454559889, \"x\": 2782.510917502661}, \"selected\": false, \"data\": {\"id_original\": \"2002\", \"name\": \"YHR115C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"YHR115C\", \"selected\": false, \"BetweennessCentrality\": 1.0, \"gal1RGsig\": 0.143, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"CHF1\", \"DMA1\", \"S000001157\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.33678, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YHR115C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.138, \"gal1RGexp\": 0.056, \"gal80Rsig\": 0.079261, \"Stress\": 2.0, \"id\": \"1673\", \"gal4RGexp\": 0.044, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"establishment of mitotic spindle orientation\", \"mitotic spindle checkpoint\", \"septin ring assembly\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1673}}, {\"position\": {\"y\": 3777.1561293645764, \"x\": 2712.531226950903}, \"selected\": false, \"data\": {\"id_original\": \"2003\", \"name\": \"YEL041W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"YEL041W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00047824, \"annotation_GO_CELLULAR_COMPONENT\": [\"cellular_component\"], \"alias\": [\"S000000767\", \"YEF1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"NAD+ kinase activity\", \"NADH kinase activity\"], \"gal4RGsig\": 3.204e-07, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YEL041W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.265, \"gal1RGexp\": 0.211, \"gal80Rsig\": 0.035715, \"Stress\": 0.0, \"id\": \"1672\", \"gal4RGexp\": 0.393, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"NADP biosynthetic process\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1672}}, {\"position\": {\"y\": 4207.79356909236, \"x\": 1691.288936454077}, \"selected\": false, \"data\": {\"id_original\": \"2004\", \"name\": \"YDL113C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"YDL113C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 9.9404e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"endosome\", \"peripheral to membrane of membrane fraction\", \"pre-autophagosomal structure\"], \"alias\": [\"ATG20\", \"CVT20\", \"S000002271\", \"SNX42\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"lipid binding\", \"phosphatidylinositol 3-phosphate binding\"], \"gal4RGsig\": 0.8523, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDL113C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.304, \"gal1RGexp\": 0.189, \"gal80Rsig\": 0.0063436, \"Stress\": 0.0, \"id\": \"1671\", \"gal4RGexp\": 0.011, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"autophagy\", \"protein targeting to vacuole\", \"retrograde transport\", \"endosome to Golgi\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1671}}, {\"position\": {\"y\": 4315.51573706111, \"x\": 1688.2714842140867}, \"selected\": false, \"data\": {\"id_original\": \"2005\", \"name\": \"YJL036W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"SNX4\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00013457, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"pre-autophagosomal structure\"], \"alias\": [\"ATG24\", \"CVT13\", \"S000003573\", \"SNX4\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"lipid binding\", \"phosphatidylinositol 3-phosphate binding\"], \"gal4RGsig\": 0.36812, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YJL036W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.376, \"gal1RGexp\": 0.195, \"gal80Rsig\": 0.00097735, \"Stress\": 0.0, \"id\": \"1670\", \"gal4RGexp\": -0.051, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein targeting to vacuole\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1670}}, {\"position\": {\"y\": 3507.958344940748, \"x\": 1566.8887365639403}, \"selected\": false, \"data\": {\"id_original\": \"2006\", \"name\": \"YBR109C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"CMD1\", \"selected\": false, \"BetweennessCentrality\": 0.83333333, \"gal1RGsig\": 0.024989, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud neck\", \"bud tip\", \"central plaque of spindle pole body\", \"cytoplasm\", \"incipient bud site\", \"mating projection tip\"], \"alias\": [\"CMD1\", \"S000000313\", \"calmodulin\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"calcium ion binding\", \"calcium-dependent protein binding\", \"protein binding\"], \"gal4RGsig\": 0.13694, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YBR109C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.8, \"AverageShortestPathLength\": 1.25, \"NeighborhoodConnectivity\": 1.33333333, \"gal80Rexp\": -0.163, \"gal1RGexp\": -0.089, \"gal80Rsig\": 0.0092082, \"Stress\": 10.0, \"id\": \"1669\", \"gal4RGexp\": 0.074, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell budding\", \"cytoskeleton organization and biogenesis\", \"endocytosis\", \"mitosis\", \"vacuole fusion\", \"non-autophagic\"], \"Radiality\": 0.91666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1669}}, {\"position\": {\"y\": 3396.6752944036384, \"x\": 1577.5005262673094}, \"selected\": false, \"data\": {\"id_original\": \"2007\", \"name\": \"YOL016C\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"CMK2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 6.846e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"CMK2\", \"S000005376\", \"calmodulin-dependent protein kinase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"calcium- and calmodulin-dependent protein kinase activity\"], \"gal4RGsig\": 0.024371, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOL016C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.5, \"AverageShortestPathLength\": 2.0, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.138, \"gal1RGexp\": -0.365, \"gal80Rsig\": 0.027128, \"Stress\": 0.0, \"id\": \"1668\", \"gal4RGexp\": -0.108, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein amino acid phosphorylation\", \"signal transduction\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1668}}, {\"position\": {\"y\": 4107.79356909236, \"x\": 959.283508139868}, \"selected\": false, \"data\": {\"id_original\": \"2008\", \"name\": \"YKL001C\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"MET14\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0020562, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"intracellular\"], \"alias\": [\"MET14\", \"S000001484\", \"adenylylsulfate kinase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"adenylylsulfate kinase activity\"], \"gal4RGsig\": 2.7503e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YKL001C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.5, \"AverageShortestPathLength\": 2.0, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.683, \"gal1RGexp\": -0.152, \"gal80Rsig\": 2.7054e-07, \"Stress\": 0.0, \"id\": \"1667\", \"gal4RGexp\": -0.474, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"methionine metabolic process\", \"sulfate assimilation\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1667}}, {\"position\": {\"y\": 3998.0106558843518, \"x\": 956.8589094460203}, \"selected\": false, \"data\": {\"id_original\": \"2009\", \"name\": \"YNL311C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"YNL311C\", \"selected\": false, \"BetweennessCentrality\": 0.66666667, \"gal1RGsig\": 0.3444, \"annotation_GO_CELLULAR_COMPONENT\": [\"ubiquitin ligase complex\"], \"alias\": [\"F-box protein\", \"S000005255\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein binding\"], \"gal4RGsig\": 0.0089606, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YNL311C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.75, \"AverageShortestPathLength\": 1.33333333, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": -0.169, \"gal1RGexp\": -0.055, \"gal80Rsig\": 0.12031, \"Stress\": 4.0, \"id\": \"1666\", \"gal4RGexp\": -0.388, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ubiquitin-dependent protein catabolic process\"], \"Radiality\": 0.88888889, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1666}}, {\"position\": {\"y\": 1456.93910360774, \"x\": 2827.247062522192}, \"selected\": false, \"data\": {\"id_original\": \"2010\", \"name\": \"YLR319C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"BUD6\", \"selected\": false, \"BetweennessCentrality\": 0.07052718, \"gal1RGsig\": 0.18355, \"annotation_GO_CELLULAR_COMPONENT\": [\"actin cap\", \"polarisome\"], \"alias\": [\"AIP3\", \"BUD6\", \"S000004311\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"cytoskeletal regulatory protein binding\"], \"gal4RGsig\": 0.0039398, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YLR319C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1409892, \"AverageShortestPathLength\": 7.09274194, \"NeighborhoodConnectivity\": 5.5, \"gal80Rexp\": 0.21, \"gal1RGexp\": 0.071, \"gal80Rsig\": 0.072655, \"Stress\": 6196.0, \"id\": \"1665\", \"gal4RGexp\": -0.201, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Rho protein signal transduction\", \"actin filament organization\", \"bipolar bud site selection\", \"bud site selection\", \"cytokinesis\", \"establishment of cell polarity (sensu the Fungi research community)\", \"response to osmotic stress\"], \"Radiality\": 0.77434289, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1665}}, {\"position\": {\"y\": 1199.5960402532478, \"x\": 1670.3000715553953}, \"selected\": false, \"data\": {\"id_original\": \"2011\", \"name\": \"YPR062W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"FCY1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.066907, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"FCY1\", \"S000006266\", \"cytosine deaminase\", \"yCD\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"cytosine deaminase activity\"], \"gal4RGsig\": 0.006637, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPR062W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11339735, \"AverageShortestPathLength\": 8.81854839, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.071, \"gal1RGexp\": -0.07, \"gal80Rsig\": 0.26496, \"Stress\": 0.0, \"id\": \"1664\", \"gal4RGexp\": -0.145, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cytidine metabolic process\", \"cytosine metabolic process\", \"pyrimidine salvage\"], \"Radiality\": 0.71042413, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1664}}, {\"position\": {\"y\": 1134.2353224798103, \"x\": 1591.306327658911}, \"selected\": false, \"data\": {\"id_original\": \"2012\", \"name\": \"YPL111W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"CAR1\", \"selected\": false, \"BetweennessCentrality\": 0.02401397, \"gal1RGsig\": 0.00072553, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\"], \"alias\": [\"CAR1\", \"LPH15\", \"S000006032\", \"arginase\", \"cargA\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"arginase activity\", \"manganese ion binding\", \"zinc ion binding\"], \"gal4RGsig\": 0.00018582, \"TopologicalCoefficient\": 0.4, \"shared_name\": \"YPL111W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11370931, \"AverageShortestPathLength\": 8.79435484, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.957, \"gal1RGexp\": 0.186, \"gal80Rsig\": 5.2368e-11, \"Stress\": 4114.0, \"id\": \"1663\", \"gal4RGexp\": -0.26, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"arginine catabolic process to ornithine\"], \"Radiality\": 0.71132019, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1663}}, {\"position\": {\"y\": 1276.9587721868415, \"x\": 1687.0644758522703}, \"selected\": false, \"data\": {\"id_original\": \"2013\", \"name\": \"YDL236W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"PHO13\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00029509, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"PHO13\", \"S000002395\", \"p-nitrophenyl phosphatase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"4-nitrophenylphosphatase activity\", \"alkaline phosphatase activity\"], \"gal4RGsig\": 5.3874e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDL236W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11339735, \"AverageShortestPathLength\": 8.81854839, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.258, \"gal1RGexp\": -0.223, \"gal80Rsig\": 0.0008095, \"Stress\": 0.0, \"id\": \"1662\", \"gal4RGexp\": -0.311, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"histone dephosphorylation\", \"protein amino acid dephosphorylation\"], \"Radiality\": 0.71042413, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1662}}, {\"position\": {\"y\": 1215.174195770826, \"x\": 1783.3122480690672}, \"selected\": false, \"data\": {\"id_original\": \"2014\", \"name\": \"YNL189W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"SRP1\", \"selected\": false, \"BetweennessCentrality\": 0.05593286, \"gal1RGsig\": 0.045195, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"KAP60\", \"S000005133\", \"SCM1\", \"SRP1\", \"karyopherin alpha\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein carrier activity\"], \"gal4RGsig\": 0.79134, \"TopologicalCoefficient\": 0.2, \"shared_name\": \"YNL189W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12783505, \"AverageShortestPathLength\": 7.82258065, \"NeighborhoodConnectivity\": 1.8, \"gal80Rexp\": -0.573, \"gal1RGexp\": 0.082, \"gal80Rsig\": 1.4096e-08, \"Stress\": 9016.0, \"id\": \"1661\", \"gal4RGexp\": 0.014, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nucleocytoplasmic transport\"], \"Radiality\": 0.74731183, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 5.0, \"Degree\": 5, \"SUID\": 1661}}, {\"position\": {\"y\": 1497.0353140874763, \"x\": 1883.6850355446531}, \"selected\": false, \"data\": {\"id_original\": \"2015\", \"name\": \"YBL069W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"AST1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.9402e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"extrinsic to membrane\"], \"alias\": [\"AST1\", \"S000000165\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 7.0045e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YBL069W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12776919, \"AverageShortestPathLength\": 7.8266129, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.026, \"gal1RGexp\": -0.272, \"gal80Rsig\": 0.87354, \"Stress\": 0.0, \"id\": \"1660\", \"gal4RGexp\": -0.487, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein targeting to membrane\"], \"Radiality\": 0.74716249, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1660}}, {\"position\": {\"y\": 2952.049439911451, \"x\": 2281.265830832739}, \"selected\": false, \"data\": {\"id_original\": \"2016\", \"name\": \"YGL073W\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"HSF1\", \"selected\": false, \"BetweennessCentrality\": 0.06332441, \"gal1RGsig\": 0.034847, \"annotation_GO_CELLULAR_COMPONENT\": [\"mitochondrion\", \"nucleus\"], \"alias\": [\"EXA3\", \"HSF1\", \"MAS3\", \"S000003041\", \"heat shock transcription factor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcription factor activity\"], \"gal4RGsig\": 0.42981, \"TopologicalCoefficient\": 0.25, \"shared_name\": \"YGL073W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10333333, \"AverageShortestPathLength\": 9.67741935, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.543, \"gal1RGexp\": 0.104, \"gal80Rsig\": 0.00062254, \"Stress\": 9976.0, \"id\": \"1659\", \"gal4RGexp\": -0.084, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of transcription from RNA polymerase II promoter\", \"response to heat\", \"response to stress\", \"spindle pole body duplication\"], \"Radiality\": 0.6786141, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 5.0, \"Degree\": 5, \"SUID\": 1659}}, {\"position\": {\"y\": 2956.222291473951, \"x\": 2398.6396711647703}, \"selected\": false, \"data\": {\"id_original\": \"2017\", \"name\": \"YBR072W\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"HSP26\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.3135e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"HSP26\", \"S000000276\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"unfolded protein binding\"], \"gal4RGsig\": 2.3868e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YBR072W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09369097, \"AverageShortestPathLength\": 10.6733871, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.447, \"gal1RGexp\": -1.232, \"gal80Rsig\": 3.3493e-11, \"Stress\": 0.0, \"id\": \"1658\", \"gal4RGexp\": 0.895, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein folding\", \"response to stress\"], \"Radiality\": 0.6417264, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1658}}, {\"position\": {\"y\": 2504.553590302076, \"x\": 1817.269462424536}, \"selected\": false, \"data\": {\"id_original\": \"2018\", \"name\": \"YLR321C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"SFH1\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 2.6832e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"RSC complex\"], \"alias\": [\"RSC\", \"S000004313\", \"SFH1\", \"chromatin remodeling complex member\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein binding\"], \"gal4RGsig\": 0.00052576, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YLR321C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12313803, \"AverageShortestPathLength\": 8.12096774, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": 0.427, \"gal1RGexp\": 0.252, \"gal80Rsig\": 0.0047054, \"Stress\": 1032.0, \"id\": \"1657\", \"gal4RGexp\": -0.352, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"chromatin remodeling\", \"double-strand break repair\"], \"Radiality\": 0.73626045, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1657}}, {\"position\": {\"y\": 646.7320570989509, \"x\": 2171.4051283180906}, \"selected\": false, \"data\": {\"id_original\": \"2019\", \"name\": \"YPR048W\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"TAH18\", \"selected\": false, \"BetweennessCentrality\": 0.07083561, \"gal1RGsig\": 0.12893, \"annotation_GO_CELLULAR_COMPONENT\": [\"cellular_component\"], \"alias\": [\"S000006252\", \"TAH18\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.54556, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YPR048W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11883086, \"AverageShortestPathLength\": 8.41532258, \"NeighborhoodConnectivity\": 2.66666667, \"gal80Rexp\": 0.289, \"gal1RGexp\": 0.113, \"gal80Rsig\": 0.063048, \"Stress\": 19552.0, \"id\": \"1656\", \"gal4RGexp\": -0.191, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.72535842, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1656}}, {\"position\": {\"y\": 821.5850234075447, \"x\": 2041.0432279885008}, \"selected\": false, \"data\": {\"id_original\": \"2020\", \"name\": \"YNL199C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"GCR2\", \"selected\": false, \"BetweennessCentrality\": 0.19692135, \"gal1RGsig\": 0.005054, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"GCR2\", \"S000005143\", \"transcription factor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcriptional activator activity\"], \"gal4RGsig\": 0.0077746, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YNL199C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12704918, \"AverageShortestPathLength\": 7.87096774, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": 0.433, \"gal1RGexp\": 0.121, \"gal80Rsig\": 5.6535e-05, \"Stress\": 89866.0, \"id\": \"1655\", \"gal4RGexp\": -0.162, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"positive regulation of glycolysis\", \"positive regulation of transcription from RNA polymerase II promoter\"], \"Radiality\": 0.74551971, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1655}}, {\"position\": {\"y\": 867.6387953802009, \"x\": 2244.1149595558836}, \"selected\": false, \"data\": {\"id_original\": \"2021\", \"name\": \"YPL075W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"GCR1\", \"selected\": false, \"BetweennessCentrality\": 0.18002254, \"gal1RGsig\": 2.4947e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"GCR1\", \"LPF10\", \"S000005996\", \"trans-acting positive regulator of the enolase and glyceraldehyde-3-phosphate dehydrogenase gene families\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA binding\", \"transcriptional activator activity\"], \"gal4RGsig\": 0.046834, \"TopologicalCoefficient\": 0.28571429, \"shared_name\": \"YPL075W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13347686, \"AverageShortestPathLength\": 7.49193548, \"NeighborhoodConnectivity\": 2.42857143, \"gal80Rexp\": -0.53, \"gal1RGexp\": -0.373, \"gal80Rsig\": 5.2661e-06, \"Stress\": 90670.0, \"id\": \"1654\", \"gal4RGexp\": -0.207, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"positive regulation of glycolysis\", \"positive regulation of transcription from RNA polymerase II promoter\"], \"Radiality\": 0.75955795, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 7.0, \"Degree\": 7, \"SUID\": 1654}}, {\"position\": {\"y\": 3396.6752944036384, \"x\": 2385.8418081581785}, \"selected\": false, \"data\": {\"id_original\": \"2022\", \"name\": \"YHR179W\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"OYE2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 2.6358e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"mitochondrion\", \"nucleus\"], \"alias\": [\"Old Yellow Enzyme\", \"isoform 2\", \"NAPDH dehydrogenase (old yellow enzyme)\", \"OYE2\", \"S000001222\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"NADPH dehydrogenase activity\"], \"gal4RGsig\": 0.098356, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YHR179W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.5, \"AverageShortestPathLength\": 2.0, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.671, \"gal1RGexp\": -0.565, \"gal80Rsig\": 3.6995e-11, \"Stress\": 0.0, \"id\": \"1653\", \"gal4RGexp\": 0.078, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1653}}, {\"position\": {\"y\": 3540.134461884107, \"x\": 2276.1289022743895}, \"selected\": false, \"data\": {\"id_original\": \"2023\", \"name\": \"YCL040W\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"GLK1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0093643, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\"], \"alias\": [\"GLK1\", \"HOR3\", \"S000000545\", \"glucokinase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"glucokinase activity\"], \"gal4RGsig\": 1.0508e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YCL040W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.5, \"AverageShortestPathLength\": 2.0, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.221, \"gal1RGexp\": -0.146, \"gal80Rsig\": 0.00020566, \"Stress\": 0.0, \"id\": \"1652\", \"gal4RGexp\": 0.542, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glucose import\", \"glucose metabolic process\", \"glycolysis\", \"mannose metabolic process\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1652}}, {\"position\": {\"y\": 3508.519471649732, \"x\": 2383.4832495033934}, \"selected\": false, \"data\": {\"id_original\": \"2024\", \"name\": \"YFL039C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"ACT1\", \"selected\": false, \"BetweennessCentrality\": 0.83333333, \"gal1RGsig\": 0.00032175, \"annotation_GO_CELLULAR_COMPONENT\": [\"H4/H2A histone acetyltransferase complex\", \"INO80 complex\", \"SWR1 complex\", \"actin cable\", \"actin cortical patch\", \"actin filament\", \"bud neck contractile ring\", \"histone acetyltransferase complex\"], \"alias\": [\"ABY1\", \"ACT1\", \"END7\", \"S000001855\", \"actin\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of cytoskeleton\"], \"gal4RGsig\": 0.000131, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YFL039C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.8, \"AverageShortestPathLength\": 1.25, \"NeighborhoodConnectivity\": 1.33333333, \"gal80Rexp\": -0.527, \"gal1RGexp\": -0.16, \"gal80Rsig\": 6.0582e-12, \"Stress\": 10.0, \"id\": \"1651\", \"gal4RGexp\": 0.192, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"actin filament reorganization during cell cycle\", \"budding cell isotropic bud growth\", \"cell wall organization and biogenesis\", \"chronological cell aging\", \"cytokinesis\", \"cytokinesis\", \"contractile ring contraction\", \"endocytosis\", \"establishment of cell polarity (sensu the Fungi research community)\", \"establishment of mitotic spindle orientation\", \"exocytosis\", \"histone acetylation\", \"mitochondrion inheritance\", \"protein secretion\", \"regulation of transcription from RNA polymerase II promoter\", \"response to osmotic stress\", \"sporulation (sensu the Fungi research community)\", \"vacuole inheritance\", \"vesicle transport along actin filament\"], \"Radiality\": 0.91666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1651}}, {\"position\": {\"y\": 3645.779611115064, \"x\": 2562.012874442358}, \"selected\": false, \"data\": {\"id_original\": \"2025\", \"name\": \"YDL130W\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"RPP1B\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.0483e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPL44'\", \"RPLA3\", \"RPP1B\", \"S000002288\", \"ribosomal protein P1B (L44') (YP1beta) (Ax)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 0.01128, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDL130W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.44444444, \"AverageShortestPathLength\": 2.25, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.24, \"gal1RGexp\": -0.3, \"gal80Rsig\": 2.1405e-05, \"Stress\": 0.0, \"id\": \"1650\", \"gal4RGexp\": -0.123, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"translational elongation\"], \"Radiality\": 0.58333333, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1650}}, {\"position\": {\"y\": 3578.074800018873, \"x\": 2474.1391285240843}, \"selected\": false, \"data\": {\"id_original\": \"2026\", \"name\": \"YDR382W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"RPP2B\", \"selected\": false, \"BetweennessCentrality\": 0.5, \"gal1RGsig\": 1.427e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPL45\", \"RPP2B\", \"S000002790\", \"YPA1\", \"ribosomal protein P2B (YP2beta) (L45)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 0.18479, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YDR382W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.314, \"gal1RGexp\": -0.218, \"gal80Rsig\": 1.4245e-07, \"Stress\": 6.0, \"id\": \"1649\", \"gal4RGexp\": -0.058, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"translational elongation\"], \"Radiality\": 0.83333333, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1649}}, {\"position\": {\"y\": 2141.1276869817634, \"x\": 2463.3972395241453}, \"selected\": false, \"data\": {\"id_original\": \"2027\", \"name\": \"YJR066W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"TOR1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.47775, \"annotation_GO_CELLULAR_COMPONENT\": [\"Golgi membrane\", \"TORC 1 complex\", \"endosome membrane\", \"extrinsic to internal side of plasma membrane\", \"plasma membrane\", \"vacuolar membrane\"], \"alias\": [\"DRR1\", \"S000003827\", \"TOR1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"phosphatidylinositol 3-kinase activity\", \"protein binding\", \"protein kinase activity\"], \"gal4RGsig\": 2.5298e-05, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YJR066W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10247934, \"AverageShortestPathLength\": 9.75806452, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.111, \"gal1RGexp\": -0.028, \"gal80Rsig\": 0.1951, \"Stress\": 0.0, \"id\": \"1648\", \"gal4RGexp\": 0.326, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"G1 phase of mitotic cell cycle\", \"cell wall organization and biogenesis (sensu the Fungi research community)\", \"meiosis\", \"mitochondrial signaling pathway\", \"regulation of cell growth\", \"regulation of progression through cell cycle\", \"signal transduction\"], \"Radiality\": 0.67562724, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1648}}, {\"position\": {\"y\": 1379.339738373365, \"x\": 2461.0213239968016}, \"selected\": false, \"data\": {\"id_original\": \"2028\", \"name\": \"?\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"\", \"selected\": false, \"BetweennessCentrality\": 0.05357439, \"NumberOfDirectedEdges\": 3.0, \"shared_name\": \"?\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.15470992, \"AverageShortestPathLength\": 6.46370968, \"NeighborhoodConnectivity\": 8.0, \"Stress\": 7092.0, \"NumberOfUndirectedEdges\": 0.0, \"Radiality\": 0.79764038, \"ClusteringCoefficient\": 0.33333333, \"id\": \"1647\", \"TopologicalCoefficient\": 0.40350877, \"Degree\": 3, \"SUID\": 1647}}, {\"position\": {\"y\": 3528.326020721998, \"x\": 1769.880000907202}, \"selected\": false, \"data\": {\"id_original\": \"2029\", \"name\": \"YKL204W\", \"Eccentricity\": 4, \"IsSingleNode\": false, \"COMMON\": \"YKL204W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 6.4944e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"mRNA cap complex\"], \"alias\": [\"EAP1\", \"S000001687\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"eukaryotic initiation factor 4E binding\"], \"gal4RGsig\": 0.9794, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YKL204W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.4, \"AverageShortestPathLength\": 2.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.16, \"gal1RGexp\": 0.253, \"gal80Rsig\": 0.091109, \"Stress\": 0.0, \"id\": \"1646\", \"gal4RGexp\": -0.002, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"telomere maintenance\"], \"Radiality\": 0.625, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1646}}, {\"position\": {\"y\": 3532.7756667180915, \"x\": 1880.1999166786863}, \"selected\": false, \"data\": {\"id_original\": \"2030\", \"name\": \"YNL154C\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"YCK2\", \"selected\": false, \"BetweennessCentrality\": 0.5, \"gal1RGsig\": 0.76333, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud neck\", \"mating projection\", \"plasma membrane\"], \"alias\": [\"S000005098\", \"YCK2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"casein kinase I activity\"], \"gal4RGsig\": 0.21924, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YNL154C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.57142857, \"AverageShortestPathLength\": 1.75, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": -0.438, \"gal1RGexp\": -0.013, \"gal80Rsig\": 2.3558e-07, \"Stress\": 6.0, \"id\": \"1645\", \"gal4RGexp\": -0.066, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell morphogenesis\", \"cytokinesis\", \"endocytosis\", \"protein amino acid phosphorylation\", \"response to glucose stimulus\"], \"Radiality\": 0.8125, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1645}}, {\"position\": {\"y\": 1779.8496108098884, \"x\": 1579.5037153542235}, \"selected\": false, \"data\": {\"id_original\": \"2031\", \"name\": \"YNL047C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"YNL047C\", \"selected\": false, \"BetweennessCentrality\": 0.02544919, \"gal1RGsig\": 0.0026155, \"annotation_GO_CELLULAR_COMPONENT\": [\"TORC 2 complex\", \"plasma membrane\"], \"alias\": [\"LIT1\", \"S000004992\", \"SLM2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"phosphoinositide binding\"], \"gal4RGsig\": 0.19124, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YNL047C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11252269, \"AverageShortestPathLength\": 8.88709677, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.029, \"gal1RGexp\": 0.14, \"gal80Rsig\": 0.7773, \"Stress\": 6152.0, \"id\": \"1644\", \"gal4RGexp\": -0.078, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"actin cable formation\", \"actin cytoskeleton organization and biogenesis\", \"establishment and/or maintenance of actin cytoskeleton polarity\", \"regulation of cell growth\"], \"Radiality\": 0.7078853, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1644}}, {\"position\": {\"y\": 3396.6752944036384, \"x\": 2176.1289022743895}, \"selected\": false, \"data\": {\"id_original\": \"2032\", \"name\": \"YNL116W\", \"Eccentricity\": 4, \"IsSingleNode\": false, \"COMMON\": \"YNL116W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00026759, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"CHF2\", \"DMA2\", \"S000005060\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.63152, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YNL116W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.4, \"AverageShortestPathLength\": 2.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.358, \"gal1RGexp\": 0.165, \"gal80Rsig\": 0.00027658, \"Stress\": 0.0, \"id\": \"1643\", \"gal4RGexp\": 0.027, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"establishment of mitotic spindle orientation\", \"mitotic spindle checkpoint\", \"septin ring assembly\"], \"Radiality\": 0.625, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1643}}, {\"position\": {\"y\": 3468.016023163404, \"x\": 2092.639959174414}, \"selected\": false, \"data\": {\"id_original\": \"2033\", \"name\": \"YHR135C\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"YCK1\", \"selected\": false, \"BetweennessCentrality\": 0.5, \"gal1RGsig\": 0.037285, \"annotation_GO_CELLULAR_COMPONENT\": [\"endoplasmic reticulum\", \"mitochondrion\", \"plasma membrane\"], \"alias\": [\"CKI2\", \"S000001177\", \"YCK1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"casein kinase I activity\"], \"gal4RGsig\": 0.23999, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YHR135C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.57142857, \"AverageShortestPathLength\": 1.75, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": 0.119, \"gal1RGexp\": 0.082, \"gal80Rsig\": 0.037227, \"Stress\": 6.0, \"id\": \"1642\", \"gal4RGexp\": -0.049, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell morphogenesis\", \"cytokinesis\", \"endocytosis\", \"protein amino acid phosphorylation\", \"response to glucose stimulus\"], \"Radiality\": 0.8125, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1642}}, {\"position\": {\"y\": 2138.758241180982, \"x\": 1829.2112959206297}, \"selected\": false, \"data\": {\"id_original\": \"2034\", \"name\": \"YML064C\", \"Eccentricity\": 22, \"IsSingleNode\": false, \"COMMON\": \"TEM1\", \"selected\": false, \"BetweennessCentrality\": 0.05441934, \"gal1RGsig\": 0.37089, \"annotation_GO_CELLULAR_COMPONENT\": [\"spindle pole body\"], \"alias\": [\"GTP-binding protein\", \"S000004529\", \"TEM1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"GTPase activity\", \"protein binding\"], \"gal4RGsig\": 0.45319, \"TopologicalCoefficient\": 0.25, \"shared_name\": \"YML064C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08949838, \"AverageShortestPathLength\": 11.1733871, \"NeighborhoodConnectivity\": 1.75, \"gal80Rexp\": -0.0, \"gal1RGexp\": -0.218, \"gal80Rsig\": 0.999999, \"Stress\": 9690.0, \"id\": \"1641\", \"gal4RGexp\": -0.069, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of exit from mitosis\"], \"Radiality\": 0.62320789, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1641}}, {\"position\": {\"y\": 1721.3383193059822, \"x\": 2369.945258933325}, \"selected\": false, \"data\": {\"id_original\": \"2035\", \"name\": \"YKL074C\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"MUD2\", \"selected\": false, \"BetweennessCentrality\": 0.03966958, \"gal1RGsig\": 0.96433, \"annotation_GO_CELLULAR_COMPONENT\": [\"commitment complex\"], \"alias\": [\"MUD2\", \"S000001557\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA splicing factor activity\", \"transesterification mechanism\", \"mRNA binding\"], \"gal4RGsig\": 0.00028457, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YKL074C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14683245, \"AverageShortestPathLength\": 6.81048387, \"NeighborhoodConnectivity\": 7.0, \"gal80Rexp\": 0.608, \"gal1RGexp\": 0.002, \"gal80Rsig\": 0.00034465, \"Stress\": 5180.0, \"id\": \"1640\", \"gal4RGexp\": -0.294, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"U2-type nuclear mRNA branch site recognition\"], \"Radiality\": 0.78479689, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1640}}, {\"position\": {\"y\": 4207.79356909236, \"x\": 1588.2714842140867}, \"selected\": false, \"data\": {\"id_original\": \"2036\", \"name\": \"YLR340W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"RPP0\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 4.9964e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPL10E\", \"RPP0\", \"S000004332\", \"ribosomal protein P0 (A0) (L10E)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 0.0063648, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLR340W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": -0.361, \"gal1RGexp\": -0.259, \"gal80Rsig\": 2.0593e-07, \"Stress\": 0.0, \"id\": \"1639\", \"gal4RGexp\": -0.148, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ribosomal large subunit assembly and maintenance\", \"translational elongation\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1639}}, {\"position\": {\"y\": 4315.51573706111, \"x\": 1585.2540319740965}, \"selected\": false, \"data\": {\"id_original\": \"2037\", \"name\": \"YDL081C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"RPP1A\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.705e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPLA1\", \"RPP1A\", \"S000002239\", \"acidic ribosomal protein P1A (YP1alpha) (A1)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 0.048133, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDL081C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": -0.278, \"gal1RGexp\": -0.429, \"gal80Rsig\": 5.9631e-06, \"Stress\": 0.0, \"id\": \"1638\", \"gal4RGexp\": -0.094, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"telomere maintenance\", \"translational elongation\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1638}}, {\"position\": {\"y\": 3180.4245619817634, \"x\": 2327.010886985083}, \"selected\": false, \"data\": {\"id_original\": \"2038\", \"name\": \"YGL166W\", \"Eccentricity\": 22, \"IsSingleNode\": false, \"COMMON\": \"CUP2\", \"selected\": false, \"BetweennessCentrality\": 0.00808084, \"gal1RGsig\": 0.33486, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"ACE1\", \"CUP2\", \"S000003134\", \"transcriptional activator\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"ligand-regulated transcription factor activity\"], \"gal4RGsig\": 0.0012181, \"TopologicalCoefficient\": 0.66666667, \"shared_name\": \"YGL166W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08593209, \"AverageShortestPathLength\": 11.63709677, \"NeighborhoodConnectivity\": 1.66666667, \"gal80Rexp\": 0.147, \"gal1RGexp\": -0.073, \"gal80Rsig\": 0.032147, \"Stress\": 2010.0, \"id\": \"1637\", \"gal4RGexp\": 0.243, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"response to copper ion\", \"transcription initiation from RNA polymerase II promoter\"], \"Radiality\": 0.60603345, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1637}}, {\"position\": {\"y\": 3296.6752944036384, \"x\": 2322.1942060768797}, \"selected\": false, \"data\": {\"id_original\": \"2039\", \"name\": \"YLL028W\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"TPO1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00017163, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud\", \"plasma membrane\", \"vacuolar membrane\"], \"alias\": [\"S000003951\", \"TPO1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"spermidine transporter activity\", \"spermine transporter activity\"], \"gal4RGsig\": 0.27319, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLL028W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.07915736, \"AverageShortestPathLength\": 12.63306452, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.24, \"gal1RGexp\": -0.189, \"gal80Rsig\": 0.0047822, \"Stress\": 0.0, \"id\": \"1636\", \"gal4RGexp\": -0.101, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"polyamine transport\"], \"Radiality\": 0.56914576, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1636}}, {\"position\": {\"y\": 2206.973573212232, \"x\": 2056.3733290016844}, \"selected\": false, \"data\": {\"id_original\": \"2040\", \"name\": \"YDR174W\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"HMO1\", \"selected\": false, \"BetweennessCentrality\": 0.06016549, \"gal1RGsig\": 5.1646e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nuclear chromatin\", \"nucleolus\", \"nucleus\"], \"alias\": [\"HMO1\", \"HSM2\", \"S000002581\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA polymerase I transcription factor activity\", \"single-stranded DNA binding\"], \"gal4RGsig\": 0.10372, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YDR174W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09243384, \"AverageShortestPathLength\": 10.81854839, \"NeighborhoodConnectivity\": 2.66666667, \"gal80Rexp\": -0.356, \"gal1RGexp\": -0.314, \"gal80Rsig\": 3.4721e-08, \"Stress\": 8006.0, \"id\": \"1635\", \"gal4RGexp\": -0.083, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"plasmid maintenance\", \"telomere maintenance\"], \"Radiality\": 0.63635006, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1635}}, {\"position\": {\"y\": 2249.8711562200447, \"x\": 2280.1111830055906}, \"selected\": false, \"data\": {\"id_original\": \"2041\", \"name\": \"YDR335W\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"MSN5\", \"selected\": false, \"BetweennessCentrality\": 0.05928044, \"gal1RGsig\": 0.8595, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"KAP142\", \"MSN5\", \"S000002743\", \"STE21\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"importin-alpha export receptor activity\", \"protein binding\"], \"gal4RGsig\": 0.071598, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YDR335W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09698866, \"AverageShortestPathLength\": 10.31048387, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": 0.272, \"gal1RGexp\": 0.028, \"gal80Rsig\": 0.2334, \"Stress\": 7266.0, \"id\": \"1634\", \"gal4RGexp\": 0.189, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein export from nucleus\"], \"Radiality\": 0.65516726, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1634}}, {\"position\": {\"y\": 3777.1561293645764, \"x\": 2418.8552320778563}, \"selected\": false, \"data\": {\"id_original\": \"2042\", \"name\": \"YLR214W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"FRE1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 4.6835e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"plasma membrane\"], \"alias\": [\"ferric reductase\", \"FRE1\", \"S000004204\", \"cupric reductase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"ferric-chelate reductase activity\"], \"gal4RGsig\": 0.0018109, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLR214W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.282, \"gal1RGexp\": 0.518, \"gal80Rsig\": 0.039051, \"Stress\": 0.0, \"id\": \"1633\", \"gal4RGexp\": -0.279, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"copper ion import\", \"iron ion transport\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1633}}, {\"position\": {\"y\": 3819.3445525213147, \"x\": 2518.053932029028}, \"selected\": false, \"data\": {\"id_original\": \"2043\", \"name\": \"YMR021C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"MAC1\", \"selected\": false, \"BetweennessCentrality\": 1.0, \"gal1RGsig\": 0.6115, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"CUA1\", \"MAC1\", \"S000004623\", \"metal-binding transcriptional activator\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"specific RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 0.59236, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YMR021C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.066, \"gal1RGexp\": 0.022, \"gal80Rsig\": 0.54119, \"Stress\": 2.0, \"id\": \"1632\", \"gal4RGexp\": -0.032, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cadmium ion homeostasis\", \"positive regulation of transcription from RNA polymerase II promoter\", \"regulation of protein catabolic process\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1632}}, {\"position\": {\"y\": 1552.6202702566047, \"x\": 2201.8361357033446}, \"selected\": false, \"data\": {\"id_original\": \"2044\", \"name\": \"YLR377C\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"FBP1\", \"selected\": false, \"BetweennessCentrality\": 0.01848502, \"gal1RGsig\": 2.1938e-10, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\"], \"alias\": [\"fructose-1\", \"6-bisphosphatase\", \"ACN8\", \"FBP1\", \"FBPase-2\", \"S000004369\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"fructose-bisphosphatase activity\"], \"gal4RGsig\": 5.8901e-11, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YLR377C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1480597, \"AverageShortestPathLength\": 6.75403226, \"NeighborhoodConnectivity\": 7.5, \"gal80Rexp\": 0.371, \"gal1RGexp\": 0.873, \"gal80Rsig\": 0.0037868, \"Stress\": 3426.0, \"id\": \"1631\", \"gal4RGexp\": 1.067, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"gluconeogenesis\"], \"Radiality\": 0.78688769, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1631}}, {\"position\": {\"y\": 1333.8123488469978, \"x\": 1904.8721388161375}, \"selected\": false, \"data\": {\"id_original\": \"2045\", \"name\": \"YER065C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"ICL1\", \"selected\": false, \"BetweennessCentrality\": 0.04069207, \"gal1RGsig\": 1.8931e-10, \"annotation_GO_CELLULAR_COMPONENT\": [\"cellular_component\"], \"alias\": [\"ICL1\", \"S000000867\", \"isocitrate lyase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"isocitrate lyase activity\"], \"gal4RGsig\": 4.8501e-09, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YER065C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13233725, \"AverageShortestPathLength\": 7.55645161, \"NeighborhoodConnectivity\": 4.5, \"gal80Rexp\": 1.147, \"gal1RGexp\": 0.65, \"gal80Rsig\": 3.4625e-08, \"Stress\": 4226.0, \"id\": \"1630\", \"gal4RGexp\": 0.591, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glyoxylate cycle\"], \"Radiality\": 0.75716846, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1630}}, {\"position\": {\"y\": 1454.802125458326, \"x\": 2040.0957716286375}, \"selected\": false, \"data\": {\"id_original\": \"2046\", \"name\": \"YJL089W\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"SIP4\", \"selected\": false, \"BetweennessCentrality\": 0.05778362, \"gal1RGsig\": 0.72688, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"S000003625\", \"SIP4\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"specific RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 0.55601, \"TopologicalCoefficient\": 0.25, \"shared_name\": \"YJL089W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14966807, \"AverageShortestPathLength\": 6.68145161, \"NeighborhoodConnectivity\": 1.75, \"gal80Rexp\": 0.442, \"gal1RGexp\": 0.037, \"gal80Rsig\": 0.033306, \"Stress\": 7016.0, \"id\": \"1629\", \"gal4RGexp\": 0.169, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"positive regulation of gluconeogenesis\", \"regulation of transcription from RNA polymerase II promoter\"], \"Radiality\": 0.78957587, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1629}}, {\"position\": {\"y\": 1841.3235487981697, \"x\": 2523.656638938208}, \"selected\": false, \"data\": {\"id_original\": \"2047\", \"name\": \"YHR030C\", \"Eccentricity\": 14, \"IsSingleNode\": false, \"COMMON\": \"SLT2\", \"selected\": false, \"BetweennessCentrality\": 0.03824806, \"gal1RGsig\": 0.0001349, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud tip\", \"cytoplasm\", \"nucleus\"], \"alias\": [\"BYC2\", \"MPK1\", \"S000001072\", \"SLK2\", \"SLT2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"MAP kinase activity\"], \"gal4RGsig\": 0.00027258, \"TopologicalCoefficient\": 0.25, \"shared_name\": \"YHR030C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14244687, \"AverageShortestPathLength\": 7.02016129, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.21, \"gal1RGexp\": -0.227, \"gal80Rsig\": 0.0033506, \"Stress\": 3506.0, \"id\": \"1628\", \"gal4RGexp\": -0.24, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell wall organization and biogenesis\", \"protein amino acid phosphorylation\", \"response to acid\", \"signal transduction\"], \"Radiality\": 0.77703106, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1628}}, {\"position\": {\"y\": 1865.5661025091072, \"x\": 2653.2540205300047}, \"selected\": false, \"data\": {\"id_original\": \"2048\", \"name\": \"YPL089C\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"RLM1\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.14513, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"RLM1\", \"S000006010\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA bending activity\", \"DNA binding\", \"transcriptional activator activity\"], \"gal4RGsig\": 0.47601, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YPL089C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12487412, \"AverageShortestPathLength\": 8.00806452, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.209, \"gal1RGexp\": -0.06, \"gal80Rsig\": 0.0026491, \"Stress\": 746.0, \"id\": \"1627\", \"gal4RGexp\": -0.037, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell wall organization and biogenesis\", \"positive regulation of transcription from RNA polymerase II promoter\", \"response to acid\", \"signal transduction\"], \"Radiality\": 0.74044205, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1627}}, {\"position\": {\"y\": 3777.1561293645764, \"x\": 2125.1792372048094}, \"selected\": false, \"data\": {\"id_original\": \"2049\", \"name\": \"YGL208W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"SIP2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.7995e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"plasma membrane\"], \"alias\": [\"S000003176\", \"SIP2\", \"SPM2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"AMP-activated protein kinase activity\"], \"gal4RGsig\": 1.1069e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGL208W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.139, \"gal1RGexp\": 0.354, \"gal80Rsig\": 0.097498, \"Stress\": 0.0, \"id\": \"1626\", \"gal4RGexp\": 0.406, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell aging\", \"cellular response to glucose starvation\", \"invasive growth (sensu the Saccharomyces research community)\", \"protein amino acid phosphorylation\", \"replicative cell aging\", \"signal transduction\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1626}}, {\"position\": {\"y\": 3819.3445525213147, \"x\": 2224.3779371559813}, \"selected\": false, \"data\": {\"id_original\": \"2050\", \"name\": \"YGL115W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"SNF4\", \"selected\": false, \"BetweennessCentrality\": 1.0, \"gal1RGsig\": 0.0058541, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\", \"plasma membrane\"], \"alias\": [\"CAT3\", \"S000003083\", \"SCI1\", \"SNF4\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein kinase activator activity\"], \"gal4RGsig\": 0.011234, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGL115W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": -0.221, \"gal1RGexp\": -0.111, \"gal80Rsig\": 0.0027535, \"Stress\": 2.0, \"id\": \"1625\", \"gal4RGexp\": 0.112, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"peroxisome organization and biogenesis\", \"regulation of transcription from RNA polymerase II promoter\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1625}}, {\"position\": {\"y\": 3112.0356459661384, \"x\": 2071.865325766821}, \"selected\": false, \"data\": {\"id_original\": \"2051\", \"name\": \"YLR310C\", \"Eccentricity\": 22, \"IsSingleNode\": false, \"COMMON\": \"CDC25\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.67137, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"plasma membrane\"], \"alias\": [\"CDC25\", \"CDC25'\", \"CTN1\", \"S000004301\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"Ras guanyl-nucleotide exchange factor activity\"], \"gal4RGsig\": 0.41028, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YLR310C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08587258, \"AverageShortestPathLength\": 11.64516129, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": -0.071, \"gal1RGexp\": 0.015, \"gal80Rsig\": 0.25519, \"Stress\": 1010.0, \"id\": \"1624\", \"gal4RGexp\": -0.037, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Ras protein signal transduction\", \"regulation of progression through cell cycle\", \"replicative cell aging\", \"traversing start control point of mitotic cell cycle\"], \"Radiality\": 0.60573477, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1624}}, {\"position\": {\"y\": 3186.5593276067634, \"x\": 1982.1682356178953}, \"selected\": false, \"data\": {\"id_original\": \"2052\", \"name\": \"YNL098C\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"RAS2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.20515, \"annotation_GO_CELLULAR_COMPONENT\": [\"plasma membrane\"], \"alias\": [\"CTN5\", \"CYR3\", \"GLC5\", \"RAS2\", \"S000005042\", \"TSL7\", \"small GTP-binding protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"GTPase activity\"], \"gal4RGsig\": 0.23242, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YNL098C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.07910686, \"AverageShortestPathLength\": 12.64112903, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.293, \"gal1RGexp\": 0.05, \"gal80Rsig\": 0.00010426, \"Stress\": 0.0, \"id\": \"1623\", \"gal4RGexp\": 0.062, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Ras protein signal transduction\", \"adenylate cyclase activation\", \"positive regulation of transcription by galactose\", \"pseudohyphal growth\", \"replicative cell aging\", \"sporulation (sensu the Fungi research community)\"], \"Radiality\": 0.56884707, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1623}}, {\"position\": {\"y\": 359.6589369817634, \"x\": 1811.7912824928953}, \"selected\": false, \"data\": {\"id_original\": \"2053\", \"name\": \"YGR019W\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"UGA1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0086582, \"annotation_GO_CELLULAR_COMPONENT\": [\"intracellular\"], \"alias\": [\"S000003251\", \"UGA1\", \"gamma-aminobutyrate (GABA) transaminase (4-aminobutyrate aminotransferase)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"4-aminobutyrate transaminase activity\"], \"gal4RGsig\": 0.0011998, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGR019W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08819346, \"AverageShortestPathLength\": 11.33870968, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.258, \"gal1RGexp\": -0.163, \"gal80Rsig\": 6.2171e-05, \"Stress\": 0.0, \"id\": \"1622\", \"gal4RGexp\": 0.234, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nitrogen utilization\"], \"Radiality\": 0.61708483, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1622}}, {\"position\": {\"y\": 275.2763686223884, \"x\": 1855.5025862038328}, \"selected\": false, \"data\": {\"id_original\": \"2054\", \"name\": \"YPR035W\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"GLN1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 2.3885e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"GLN1\", \"S000006239\", \"glutamine synthetase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"glutamate-ammonia ligase activity\"], \"gal4RGsig\": 2.9298e-09, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPR035W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08819346, \"AverageShortestPathLength\": 11.33870968, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.172, \"gal1RGexp\": -0.197, \"gal80Rsig\": 0.036009, \"Stress\": 0.0, \"id\": \"1621\", \"gal4RGexp\": -1.06, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glutamine biosynthetic process\", \"nitrogen compound metabolic process\"], \"Radiality\": 0.61708483, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1621}}, {\"position\": {\"y\": 369.2630629583259, \"x\": 1937.8956373512938}, \"selected\": false, \"data\": {\"id_original\": \"2055\", \"name\": \"YER040W\", \"Eccentricity\": 22, \"IsSingleNode\": false, \"COMMON\": \"GLN3\", \"selected\": false, \"BetweennessCentrality\": 0.01609638, \"gal1RGsig\": 0.38375, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\", \"nucleus\"], \"alias\": [\"GLN3\", \"S000000842\", \"transcriptional activator of nitrogen-regulated genes\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcription factor activity\", \"transcriptional activator activity\"], \"gal4RGsig\": 0.010321, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YER040W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09668616, \"AverageShortestPathLength\": 10.34274194, \"NeighborhoodConnectivity\": 1.66666667, \"gal80Rexp\": 0.537, \"gal1RGexp\": 0.098, \"gal80Rsig\": 0.0018683, \"Stress\": 5118.0, \"id\": \"1620\", \"gal4RGexp\": -0.513, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nitrogen compound metabolic process\", \"positive regulation of transcription\", \"positive regulation of transcription from RNA polymerase II promoter\", \"regulation of nitrogen utilization\"], \"Radiality\": 0.65397252, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1620}}, {\"position\": {\"y\": 1619.0158171697517, \"x\": 1961.7272718727781}, \"selected\": false, \"data\": {\"id_original\": \"2056\", \"name\": \"YGL008C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"PMA1\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 1.0007e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"lipid raft\", \"mitochondrion\", \"plasma membrane\"], \"alias\": [\"PMA1\", \"S000002976\", \"plasma membrane H+-ATPase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"hydrogen-exporting ATPase activity\", \"phosphorylative mechanism\"], \"gal4RGsig\": 0.00071366, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YGL008C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14639906, \"AverageShortestPathLength\": 6.83064516, \"NeighborhoodConnectivity\": 9.5, \"gal80Rexp\": -0.573, \"gal1RGexp\": -0.352, \"gal80Rsig\": 1.2622e-06, \"Stress\": 1032.0, \"id\": \"1619\", \"gal4RGexp\": -0.282, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"proton transport\", \"regulation of pH\"], \"Radiality\": 0.78405018, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1619}}, {\"position\": {\"y\": 2070.5651869817634, \"x\": 2882.2385786354735}, \"selected\": false, \"data\": {\"id_original\": \"2057\", \"name\": \"YOR036W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"PEP12\", \"selected\": false, \"BetweennessCentrality\": 0.13339563, \"gal1RGsig\": 0.092135, \"annotation_GO_CELLULAR_COMPONENT\": [\"Golgi apparatus\", \"endosome\"], \"alias\": [\"PEP12\", \"S000005562\", \"VPL6\", \"VPS6\", \"VPT13\", \"c-terminal TMD\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"t-SNARE activity\"], \"gal4RGsig\": 0.43055, \"TopologicalCoefficient\": 0.22222222, \"shared_name\": \"YOR036W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12133072, \"AverageShortestPathLength\": 8.24193548, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.491, \"gal1RGexp\": 0.071, \"gal80Rsig\": 1.6313e-06, \"Stress\": 13532.0, \"id\": \"1618\", \"gal4RGexp\": 0.037, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Golgi to vacuole transport\"], \"Radiality\": 0.73178017, \"ClusteringCoefficient\": 0.06666667, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 6.0, \"Degree\": 6, \"SUID\": 1618}}, {\"position\": {\"y\": 2129.784852509107, \"x\": 3017.1143110573485}, \"selected\": false, \"data\": {\"id_original\": \"2058\", \"name\": \"YDR323C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"PEP7\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.10243, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"external side of endosome membrane\"], \"alias\": [\"PEP7\", \"S000002731\", \"VAC1\", \"VPL21\", \"VPS19\", \"VPT19\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.999999, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YDR323C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10834426, \"AverageShortestPathLength\": 9.22983871, \"NeighborhoodConnectivity\": 3.5, \"gal80Rexp\": 0.052, \"gal1RGexp\": 0.164, \"gal80Rsig\": 0.69287, \"Stress\": 618.0, \"id\": \"1617\", \"gal4RGexp\": 0.0, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Golgi to vacuole transport\", \"vesicle docking during exocytosis\", \"vesicle fusion\"], \"Radiality\": 0.69519116, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1617}}, {\"position\": {\"y\": 2488.559998993482, \"x\": 1508.383231955786}, \"selected\": false, \"data\": {\"id_original\": \"2059\", \"name\": \"YBL005W\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"PDR3\", \"selected\": false, \"BetweennessCentrality\": 1.632e-05, \"gal1RGsig\": 0.64313, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"AMY2\", \"PDR3\", \"S000000101\", \"TPE2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA binding\", \"transcriptional activator activity\"], \"gal4RGsig\": 0.999999, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YBL005W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.0992, \"AverageShortestPathLength\": 10.08064516, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.0, \"gal1RGexp\": -0.0, \"gal80Rsig\": 0.999999, \"Stress\": 2.0, \"id\": \"1616\", \"gal4RGexp\": 0.0, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"positive regulation of transcription\", \"DNA-dependent\", \"regulation of transcription from RNA polymerase II promoter\", \"response to drug\"], \"Radiality\": 0.66367981, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1616}}, {\"position\": {\"y\": 1768.8102431341072, \"x\": 1833.892051535864}, \"selected\": false, \"data\": {\"id_original\": \"2060\", \"name\": \"YBR160W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"CDC28\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.7432, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"CDC28\", \"CDK1\", \"HSL5\", \"S000000364\", \"SRM5\", \"cyclin-dependent protein kinase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"cyclin-dependent protein kinase activity\"], \"gal4RGsig\": 0.32105, \"TopologicalCoefficient\": 0.36842105, \"shared_name\": \"YBR160W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14744352, \"AverageShortestPathLength\": 6.78225806, \"NeighborhoodConnectivity\": 7.33333333, \"gal80Rexp\": -0.405, \"gal1RGexp\": -0.016, \"gal80Rsig\": 0.026081, \"Stress\": 1030.0, \"id\": \"1615\", \"gal4RGexp\": -0.087, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"G1/S transition of mitotic cell cycle\", \"G2/M transition of mitotic cell cycle\", \"S phase of mitotic cell cycle\", \"protein amino acid phosphorylation\", \"regulation of meiosis\", \"regulation of progression through cell cycle\", \"transcription\"], \"Radiality\": 0.78584229, \"ClusteringCoefficient\": 0.33333333, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1615}}, {\"position\": {\"y\": 1707.762116913404, \"x\": 1706.4707563698485}, \"selected\": false, \"data\": {\"id_original\": \"2061\", \"name\": \"YKL101W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"HSL1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.96433, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud neck\", \"septin ring\"], \"alias\": [\"HSL1\", \"NIK1\", \"S000001584\", \"serine-threonine kinase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein kinase activity\"], \"gal4RGsig\": 1.6928e-09, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YKL101W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12856402, \"AverageShortestPathLength\": 7.77822581, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.439, \"gal1RGexp\": -0.01, \"gal80Rsig\": 0.0030233, \"Stress\": 0.0, \"id\": \"1614\", \"gal4RGexp\": 0.78, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"G2/M transition of mitotic cell cycle\", \"cell morphogenesis checkpoint\", \"protein amino acid phosphorylation\", \"regulation of progression through cell cycle\", \"septin checkpoint\"], \"Radiality\": 0.7489546, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1614}}, {\"position\": {\"y\": 2506.171021942701, \"x\": 1620.7163008034422}, \"selected\": false, \"data\": {\"id_original\": \"2062\", \"name\": \"YOL156W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"HXT11\", \"selected\": false, \"BetweennessCentrality\": 0.00401593, \"gal1RGsig\": 8.1828e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"plasma membrane\"], \"alias\": [\"HXT11\", \"LGT3\", \"S000005516\", \"glucose permease\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"fructose transporter activity\", \"galactose transporter activity\", \"glucose transporter activity\", \"mannose transporter activity\"], \"gal4RGsig\": 5.1939e-05, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YOL156W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10997783, \"AverageShortestPathLength\": 9.09274194, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.105, \"gal1RGexp\": 0.298, \"gal80Rsig\": 0.28091, \"Stress\": 1028.0, \"id\": \"1613\", \"gal4RGexp\": 0.462, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"hexose transport\"], \"Radiality\": 0.70026882, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1613}}, {\"position\": {\"y\": 2431.2316908880134, \"x\": 1613.068046408911}, \"selected\": false, \"data\": {\"id_original\": \"2063\", \"name\": \"YJL219W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"HXT9\", \"selected\": false, \"BetweennessCentrality\": 0.00401593, \"gal1RGsig\": 5.6008e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"plasma membrane\"], \"alias\": [\"HXT9\", \"S000003755\", \"hexose permease\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"fructose transporter activity\", \"galactose transporter activity\", \"glucose transporter activity\", \"mannose transporter activity\"], \"gal4RGsig\": 1.6899e-09, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YJL219W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10997783, \"AverageShortestPathLength\": 9.09274194, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": -0.162, \"gal1RGexp\": 0.298, \"gal80Rsig\": 0.097946, \"Stress\": 1028.0, \"id\": \"1612\", \"gal4RGexp\": 0.592, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"hexose transport\"], \"Radiality\": 0.70026882, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1612}}, {\"position\": {\"y\": 1760.2400984807869, \"x\": 2706.0894087135985}, \"selected\": false, \"data\": {\"id_original\": \"2064\", \"name\": \"YLL021W\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"SPA2\", \"selected\": false, \"BetweennessCentrality\": 0.01334058, \"gal1RGsig\": 0.00034013, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud neck\", \"bud tip\", \"incipient bud site\", \"mating projection tip\", \"polarisome\"], \"alias\": [\"FUS6\", \"PEA1\", \"S000003944\", \"SPA2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"cytoskeletal regulatory protein binding\"], \"gal4RGsig\": 0.23036, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YLL021W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13648872, \"AverageShortestPathLength\": 7.3266129, \"NeighborhoodConnectivity\": 6.5, \"gal80Rexp\": -0.036, \"gal1RGexp\": -0.155, \"gal80Rsig\": 0.62229, \"Stress\": 1028.0, \"id\": \"1611\", \"gal4RGexp\": 0.05, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Rho protein signal transduction\", \"actin filament organization\", \"bipolar bud site selection\", \"establishment of cell polarity (sensu the Fungi research community)\", \"pseudohyphal growth\", \"regulation of initiation of mating projection growth\", \"regulation of termination of mating projection growth\"], \"Radiality\": 0.765681, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1611}}, {\"position\": {\"y\": 1455.6732573552986, \"x\": 1228.1326826393797}, \"selected\": false, \"data\": {\"id_original\": \"2065\", \"name\": \"YOL136C\", \"Eccentricity\": 25, \"IsSingleNode\": false, \"COMMON\": \"PFK27\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.9054e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"PFK-2\", \"6-phosphofructo-2-kinase\", \"PFK27\", \"S000005496\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"6-phosphofructo-2-kinase activity\"], \"gal4RGsig\": 0.39614, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOL136C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.07951266, \"AverageShortestPathLength\": 12.5766129, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.472, \"gal1RGexp\": -0.646, \"gal80Rsig\": 0.0081753, \"Stress\": 0.0, \"id\": \"1610\", \"gal4RGexp\": -0.086, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"fructose 2\", \"6-bisphosphate metabolic process\", \"regulation of glycolysis\"], \"Radiality\": 0.57123656, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1610}}, {\"position\": {\"y\": 1512.160466675367, \"x\": 1336.562675315161}, \"selected\": false, \"data\": {\"id_original\": \"2066\", \"name\": \"YJL203W\", \"Eccentricity\": 24, \"IsSingleNode\": false, \"COMMON\": \"PRP21\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.13783, \"annotation_GO_CELLULAR_COMPONENT\": [\"snRNP U2\"], \"alias\": [\"PRP21\", \"RNA splicing factor\", \"S000003739\", \"SPP91\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\"], \"gal4RGsig\": 0.0006541, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YJL203W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08635097, \"AverageShortestPathLength\": 11.58064516, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.401, \"gal1RGexp\": 0.083, \"gal80Rsig\": 0.0046881, \"Stress\": 2604.0, \"id\": \"1609\", \"gal4RGexp\": -0.46, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nuclear mRNA splicing\", \"via spliceosome\"], \"Radiality\": 0.60812425, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1609}}, {\"position\": {\"y\": 2752.684693817701, \"x\": 1891.9839552223875}, \"selected\": false, \"data\": {\"id_original\": \"2067\", \"name\": \"YNR007C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"AUT1\", \"selected\": false, \"BetweennessCentrality\": 0.00401593, \"gal1RGsig\": 0.061024, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"APG3\", \"ATG3\", \"AUT1\", \"S000005290\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"APG8 conjugating enzyme activity\"], \"gal4RGsig\": 0.00031083, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YNR007C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10997783, \"AverageShortestPathLength\": 9.09274194, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.161, \"gal1RGexp\": 0.078, \"gal80Rsig\": 0.10191, \"Stress\": 1028.0, \"id\": \"1608\", \"gal4RGexp\": 0.196, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"C-terminal protein lipidation\", \"autophagy\", \"protein targeting to vacuole\"], \"Radiality\": 0.70026882, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1608}}, {\"position\": {\"y\": 1835.320680145826, \"x\": 1933.0056989968016}, \"selected\": false, \"data\": {\"id_original\": \"2068\", \"name\": \"YFL026W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"STE2\", \"selected\": false, \"BetweennessCentrality\": 2.449e-05, \"gal1RGsig\": 1.3644e-10, \"annotation_GO_CELLULAR_COMPONENT\": [\"integral to plasma membrane\"], \"alias\": [\"alpha-factor pheromone receptor\", \"G protein coupled receptor (GPCR)\", \"S000001868\", \"STE2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"mating-type alpha-factor pheromone receptor activity\"], \"gal4RGsig\": 4.1738e-06, \"TopologicalCoefficient\": 0.49122807, \"shared_name\": \"YFL026W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1465721, \"AverageShortestPathLength\": 6.82258065, \"NeighborhoodConnectivity\": 9.33333333, \"gal80Rexp\": -0.74, \"gal1RGexp\": -0.653, \"gal80Rsig\": 2.884e-14, \"Stress\": 6.0, \"id\": \"1607\", \"gal4RGexp\": -0.396, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell projection biogenesis\", \"response to pheromone during conjugation with cellular fusion\"], \"Radiality\": 0.78434886, \"ClusteringCoefficient\": 0.66666667, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1607}}, {\"position\": {\"y\": 1695.1738143010994, \"x\": 2269.7104719460203}, \"selected\": false, \"data\": {\"id_original\": \"2069\", \"name\": \"YJL157C\", \"Eccentricity\": 14, \"IsSingleNode\": false, \"COMMON\": \"FAR1\", \"selected\": false, \"BetweennessCentrality\": 0.15125755, \"gal1RGsig\": 0.00059763, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"mating projection tip\", \"membrane\", \"nucleus\"], \"alias\": [\"Cdc28p kinase inhibitor\", \"FAR1\", \"S000003693\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"cyclin-dependent protein kinase inhibitor activity\"], \"gal4RGsig\": 4.7408e-08, \"TopologicalCoefficient\": 0.275, \"shared_name\": \"YJL157C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.15736041, \"AverageShortestPathLength\": 6.35483871, \"NeighborhoodConnectivity\": 6.0, \"gal80Rexp\": 0.972, \"gal1RGexp\": -0.158, \"gal80Rsig\": 6.3708e-07, \"Stress\": 15458.0, \"id\": \"1606\", \"gal4RGexp\": -0.803, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell cycle arrest\", \"pheromone-dependent signal transduction during conjugation with cellular fusion\"], \"Radiality\": 0.80167264, \"ClusteringCoefficient\": 0.16666667, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1606}}, {\"position\": {\"y\": 1787.9211134954353, \"x\": 1902.1131666481688}, \"selected\": false, \"data\": {\"id_original\": \"2070\", \"name\": \"YNL145W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"MFA2\", \"selected\": false, \"BetweennessCentrality\": 2.449e-05, \"gal1RGsig\": 3.148e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"extracellular region\"], \"alias\": [\"MFA2\", \"S000005089\", \"a-factor mating pheromone precursor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"mating pheromone activity\"], \"gal4RGsig\": 0.05338, \"TopologicalCoefficient\": 0.49122807, \"shared_name\": \"YNL145W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1465721, \"AverageShortestPathLength\": 6.82258065, \"NeighborhoodConnectivity\": 9.33333333, \"gal80Rexp\": -1.237, \"gal1RGexp\": -0.764, \"gal80Rsig\": 1.1916e-10, \"Stress\": 6.0, \"id\": \"1605\", \"gal4RGexp\": -0.098, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"pheromone-dependent signal transduction during conjugation with cellular fusion\"], \"Radiality\": 0.78434886, \"ClusteringCoefficient\": 0.66666667, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1605}}, {\"position\": {\"y\": 1830.7998976751228, \"x\": 1879.201835471411}, \"selected\": false, \"data\": {\"id_original\": \"2071\", \"name\": \"YDR461W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"MFA1\", \"selected\": false, \"BetweennessCentrality\": 2.449e-05, \"gal1RGsig\": 2.4721e-10, \"annotation_GO_CELLULAR_COMPONENT\": [\"extracellular region\", \"soluble fraction\"], \"alias\": [\"MFA1\", \"S000002869\", \"a-factor mating pheromone precursor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"mating pheromone activity\"], \"gal4RGsig\": 0.011212, \"TopologicalCoefficient\": 0.49122807, \"shared_name\": \"YDR461W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1465721, \"AverageShortestPathLength\": 6.82258065, \"NeighborhoodConnectivity\": 9.33333333, \"gal80Rexp\": -0.526, \"gal1RGexp\": -0.659, \"gal80Rsig\": 5.5223e-10, \"Stress\": 6.0, \"id\": \"1604\", \"gal4RGexp\": -0.147, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"pheromone-dependent signal transduction during conjugation with cellular fusion\"], \"Radiality\": 0.78434886, \"ClusteringCoefficient\": 0.66666667, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1604}}, {\"position\": {\"y\": 1800.1686720891853, \"x\": 1748.6147383034422}, \"selected\": false, \"data\": {\"id_original\": \"2072\", \"name\": \"YGR108W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"CLB1\", \"selected\": false, \"BetweennessCentrality\": 0.05930986, \"gal1RGsig\": 7.5764e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"B-type cyclin\", \"CLB1\", \"S000003340\", \"SCB1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"cyclin-dependent protein kinase regulator activity\"], \"gal4RGsig\": 2.3869e-07, \"TopologicalCoefficient\": 0.36666667, \"shared_name\": \"YGR108W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14832536, \"AverageShortestPathLength\": 6.74193548, \"NeighborhoodConnectivity\": 7.66666667, \"gal80Rexp\": 0.101, \"gal1RGexp\": -0.25, \"gal80Rsig\": 0.1936, \"Stress\": 7562.0, \"id\": \"1603\", \"gal4RGexp\": -0.566, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"G2/M transition of mitotic cell cycle\", \"meiotic G2/MI transition\", \"mitotic spindle organization and biogenesis in nucleus\", \"regulation of cyclin-dependent protein kinase activity\"], \"Radiality\": 0.78733572, \"ClusteringCoefficient\": 0.33333333, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1603}}, {\"position\": {\"y\": 1590.5105109258552, \"x\": 2049.652747946997}, \"selected\": false, \"data\": {\"id_original\": \"2073\", \"name\": \"YKR097W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"PCK1\", \"selected\": false, \"BetweennessCentrality\": 0.04542209, \"gal1RGsig\": 7.092e-13, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\"], \"alias\": [\"JPM2\", \"PCK1\", \"PPC1\", \"S000001805\", \"phosphoenolpyruvate carboxylkinase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"phosphoenolpyruvate carboxykinase (ATP) activity\"], \"gal4RGsig\": 1.8547e-10, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YKR097W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14921781, \"AverageShortestPathLength\": 6.7016129, \"NeighborhoodConnectivity\": 11.0, \"gal80Rexp\": 0.123, \"gal1RGexp\": 1.289, \"gal80Rsig\": 0.13819, \"Stress\": 4434.0, \"id\": \"1602\", \"gal4RGexp\": 1.224, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"gluconeogenesis\"], \"Radiality\": 0.78882915, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1602}}, {\"position\": {\"y\": 1645.664186005201, \"x\": 1895.4573591530516}, \"selected\": false, \"data\": {\"id_original\": \"2074\", \"name\": \"YJL159W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"HSP150\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 6.8879e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"cell wall (sensu the Fungi research community)\"], \"alias\": [\"secretory glycoprotein\", \"CCW7\", \"HSP150\", \"ORE1\", \"PIR2\", \"S000003695\", \"heat shock protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of cell wall\"], \"gal4RGsig\": 0.041194, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YJL159W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14639906, \"AverageShortestPathLength\": 6.83064516, \"NeighborhoodConnectivity\": 9.5, \"gal80Rexp\": 0.001, \"gal1RGexp\": -0.357, \"gal80Rsig\": 0.999999, \"Stress\": 1032.0, \"id\": \"1601\", \"gal4RGexp\": 0.111, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cell wall organization and biogenesis\"], \"Radiality\": 0.78405018, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1601}}, {\"position\": {\"y\": 1684.8164382024665, \"x\": 1964.7229688942625}, \"selected\": false, \"data\": {\"id_original\": \"2075\", \"name\": \"YIL015W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"BAR1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 7.0996e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"periplasmic space (sensu the Fungi research community)\"], \"alias\": [\"BAR1\", \"S000001277\", \"SST1\", \"protease\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"aspartic-type endopeptidase activity\"], \"gal4RGsig\": 0.0040782, \"TopologicalCoefficient\": 0.63157895, \"shared_name\": \"YIL015W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14648553, \"AverageShortestPathLength\": 6.8266129, \"NeighborhoodConnectivity\": 12.0, \"gal80Rexp\": -1.117, \"gal1RGexp\": -0.622, \"gal80Rsig\": 2.9167e-11, \"Stress\": 0.0, \"id\": \"1600\", \"gal4RGexp\": -0.207, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein catabolic process\"], \"Radiality\": 0.78419952, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1600}}, {\"position\": {\"y\": 1780.4677138372322, \"x\": 2020.5910719216063}, \"selected\": false, \"data\": {\"id_original\": \"2076\", \"name\": \"YMR043W\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"MCM1\", \"selected\": false, \"BetweennessCentrality\": 0.5264582, \"gal1RGsig\": 0.0035372, \"annotation_GO_CELLULAR_COMPONENT\": [\"nuclear chromatin\", \"nucleus\"], \"alias\": [\"FUN80\", \"MCM1\", \"S000004646\", \"transcription factor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA bending activity\", \"DNA binding\", \"DNA replication origin binding\", \"RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 4.2514e-06, \"TopologicalCoefficient\": 0.1010101, \"shared_name\": \"YMR043W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.17115252, \"AverageShortestPathLength\": 5.84274194, \"NeighborhoodConnectivity\": 2.66666667, \"gal80Rexp\": 0.457, \"gal1RGexp\": -0.183, \"gal80Rsig\": 0.00024112, \"Stress\": 86646.0, \"id\": \"1599\", \"gal4RGexp\": -0.654, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"DNA replication initiation\", \"regulation of transcription from RNA polymerase II promoter\"], \"Radiality\": 0.82063919, \"ClusteringCoefficient\": 0.05882353, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 18.0, \"Degree\": 18, \"SUID\": 1599}}, {\"position\": {\"y\": 1426.556924347486, \"x\": 2557.3399275124266}, \"selected\": false, \"data\": {\"id_original\": \"2077\", \"name\": \"YKL109W\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"HAP4\", \"selected\": false, \"BetweennessCentrality\": 0.03373819, \"gal1RGsig\": 0.045375, \"annotation_GO_CELLULAR_COMPONENT\": [\"CCAAT-binding factor complex\"], \"alias\": [\"HAP4\", \"S000001592\", \"transcriptional activator protein of CYC1 (component of HAP2/HAP3 heteromer)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcriptional activator activity\"], \"gal4RGsig\": 3.1802e-06, \"TopologicalCoefficient\": 0.33928571, \"shared_name\": \"YKL109W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14735591, \"AverageShortestPathLength\": 6.78629032, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.117, \"gal1RGexp\": 0.084, \"gal80Rsig\": 0.071395, \"Stress\": 6894.0, \"id\": \"1598\", \"gal4RGexp\": 0.295, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of carbohydrate metabolic process\", \"transcription\"], \"Radiality\": 0.78569295, \"ClusteringCoefficient\": 0.33333333, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1598}}, {\"position\": {\"y\": 2838.141969208326, \"x\": 1813.399131613989}, \"selected\": false, \"data\": {\"id_original\": \"2078\", \"name\": \"YBR217W\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"APG12\", \"selected\": false, \"BetweennessCentrality\": 1.632e-05, \"gal1RGsig\": 0.143, \"annotation_GO_CELLULAR_COMPONENT\": [\"membrane fraction\"], \"alias\": [\"APG12\", \"ATG12\", \"S000000421\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein tag\"], \"gal4RGsig\": 0.011008, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YBR217W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.0992, \"AverageShortestPathLength\": 10.08064516, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.378, \"gal1RGexp\": 0.088, \"gal80Rsig\": 0.012775, \"Stress\": 2.0, \"id\": \"1597\", \"gal4RGexp\": -0.332, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"autophagy\", \"protein targeting to vacuole\"], \"Radiality\": 0.66367981, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1597}}, {\"position\": {\"y\": 2623.1389174505134, \"x\": 1896.8353040993406}, \"selected\": false, \"data\": {\"id_original\": \"2079\", \"name\": \"YHR171W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"APG7\", \"selected\": false, \"BetweennessCentrality\": 0.02401397, \"gal1RGsig\": 0.030789, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"cytosol\", \"membrane\", \"mitochondrion\"], \"alias\": [\"APG7\", \"ATG7\", \"CVT2\", \"S000001214\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"APG12 activating enzyme activity\", \"APG8 activating enzyme activity\"], \"gal4RGsig\": 0.0002257, \"TopologicalCoefficient\": 0.38095238, \"shared_name\": \"YHR171W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12338308, \"AverageShortestPathLength\": 8.10483871, \"NeighborhoodConnectivity\": 3.66666667, \"gal80Rexp\": 0.034, \"gal1RGexp\": -0.134, \"gal80Rsig\": 0.7782, \"Stress\": 4106.0, \"id\": \"1596\", \"gal4RGexp\": 0.251, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"C-terminal protein lipidation\", \"autophagy\", \"protein modification\", \"protein targeting to vacuole\"], \"Radiality\": 0.73685783, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1596}}, {\"position\": {\"y\": 2724.7709975286384, \"x\": 1817.7115095436766}, \"selected\": false, \"data\": {\"id_original\": \"2080\", \"name\": \"YPL149W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"APG5\", \"selected\": false, \"BetweennessCentrality\": 0.00401593, \"gal1RGsig\": 0.47027, \"annotation_GO_CELLULAR_COMPONENT\": [\"autophagic vacuole\", \"cytosol\", \"pre-autophagosomal structure\"], \"alias\": [\"APG5\", \"ATG5\", \"S000006070\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.043291, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YPL149W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10997783, \"AverageShortestPathLength\": 9.09274194, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.488, \"gal1RGexp\": 0.033, \"gal80Rsig\": 1.5579e-05, \"Stress\": 1028.0, \"id\": \"1595\", \"gal4RGexp\": 0.116, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"autophagy\", \"protein targeting to vacuole\"], \"Radiality\": 0.70026882, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1595}}, {\"position\": {\"y\": 1361.1866011663337, \"x\": 2633.0474470436766}, \"selected\": false, \"data\": {\"id_original\": \"2081\", \"name\": \"YKL028W\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"TFA1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 3.5042e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"mitochondrion\", \"transcription factor TFIIE complex\"], \"alias\": [\"S000001511\", \"TFA1\", \"transcription factor tfIIE large subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"general RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 0.025221, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YKL028W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1025641, \"AverageShortestPathLength\": 9.75, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.337, \"gal1RGexp\": 0.214, \"gal80Rsig\": 4.9898e-05, \"Stress\": 0.0, \"id\": \"1594\", \"gal4RGexp\": -0.146, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"transcription initiation from RNA polymerase II promoter\"], \"Radiality\": 0.67592593, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1594}}, {\"position\": {\"y\": 1240.386353973951, \"x\": 2622.0289228737547}, \"selected\": false, \"data\": {\"id_original\": \"2082\", \"name\": \"YDR311W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"TFB1\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.056092, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleotide-excision repair factor 3 complex\", \"transcription factor TFIIH complex\"], \"alias\": [\"75 kDa subunit component\", \"S000002719\", \"TFB1\", \"transcription initiation factor IIb\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"general RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 0.60829, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YDR311W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11423307, \"AverageShortestPathLength\": 8.75403226, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": 0.332, \"gal1RGexp\": 0.098, \"gal80Rsig\": 0.0087786, \"Stress\": 612.0, \"id\": \"1593\", \"gal4RGexp\": -0.043, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"negative regulation of transcription from RNA polymerase II promoter\", \"mitotic\", \"nucleotide-excision repair\", \"nucleotide-excision repair\", \"DNA duplex unwinding\", \"transcription initiation from RNA polymerase II promoter\"], \"Radiality\": 0.71281362, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1593}}, {\"position\": {\"y\": 1483.878411774244, \"x\": 2659.7689131081297}, \"selected\": false, \"data\": {\"id_original\": \"2083\", \"name\": \"YBL021C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"HAP3\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.18207, \"annotation_GO_CELLULAR_COMPONENT\": [\"CCAAT-binding factor complex\", \"nucleus\"], \"alias\": [\"HAP3\", \"S000000117\", \"transcriptional activator protein of CYC1 (component of HAP2/HAP3 heteromer)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcriptional activator activity\"], \"gal4RGsig\": 0.015004, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YBL021C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12930136, \"AverageShortestPathLength\": 7.73387097, \"NeighborhoodConnectivity\": 4.5, \"gal80Rexp\": -0.108, \"gal1RGexp\": 0.073, \"gal80Rsig\": 0.497, \"Stress\": 0.0, \"id\": \"1592\", \"gal4RGexp\": -0.256, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of carbohydrate metabolic process\", \"transcription\"], \"Radiality\": 0.75059737, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1592}}, {\"position\": {\"y\": 1321.0486464544197, \"x\": 2655.491874533911}, \"selected\": false, \"data\": {\"id_original\": \"2084\", \"name\": \"YGL237C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"HAP2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.002588, \"annotation_GO_CELLULAR_COMPONENT\": [\"CCAAT-binding factor complex\", \"nucleus\"], \"alias\": [\"HAP2\", \"S000003206\", \"transcriptional activator protein of CYC1 (component of HAP2/HAP3 heteromer)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcriptional activator activity\"], \"gal4RGsig\": 0.22211, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YGL237C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12930136, \"AverageShortestPathLength\": 7.73387097, \"NeighborhoodConnectivity\": 4.5, \"gal80Rexp\": 0.059, \"gal1RGexp\": 0.127, \"gal80Rsig\": 0.35299, \"Stress\": 0.0, \"id\": \"1591\", \"gal4RGexp\": 0.05, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of carbohydrate metabolic process\", \"transcription\"], \"Radiality\": 0.75059737, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1591}}, {\"position\": {\"y\": 1267.7917494817634, \"x\": 2996.2559736550047}, \"selected\": false, \"data\": {\"id_original\": \"2085\", \"name\": \"YEL039C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"CYC7\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 2.8783e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"mitochondrial intermembrane space\", \"mitochondrion\"], \"alias\": [\"CYC7\", \"S000000765\", \"iso-2-cytochrome c\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"electron carrier activity\"], \"gal4RGsig\": 7.3568e-07, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YEL039C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10495133, \"AverageShortestPathLength\": 9.52822581, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": -1.373, \"gal1RGexp\": -0.319, \"gal80Rsig\": 4.9668e-07, \"Stress\": 0.0, \"id\": \"1590\", \"gal4RGexp\": 0.377, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"electron transport\"], \"Radiality\": 0.68413978, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1590}}, {\"position\": {\"y\": 1407.8143172307869, \"x\": 2705.3218916237547}, \"selected\": false, \"data\": {\"id_original\": \"2086\", \"name\": \"YJR048W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"CYC1\", \"selected\": false, \"BetweennessCentrality\": 0.04079818, \"gal1RGsig\": 3.3444e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"mitochondrial intermembrane space\", \"mitochondrion\"], \"alias\": [\"CYC1\", \"S000003809\", \"iso-1-cytochrome c\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"electron carrier activity\"], \"gal4RGsig\": 0.005959, \"TopologicalCoefficient\": 0.26666667, \"shared_name\": \"YJR048W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13212573, \"AverageShortestPathLength\": 7.56854839, \"NeighborhoodConnectivity\": 4.4, \"gal80Rexp\": -0.643, \"gal1RGexp\": 0.216, \"gal80Rsig\": 1.6071e-08, \"Stress\": 9044.0, \"id\": \"1589\", \"gal4RGexp\": 0.14, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"electron transport\"], \"Radiality\": 0.75672043, \"ClusteringCoefficient\": 0.2, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 5.0, \"Degree\": 5, \"SUID\": 1589}}, {\"position\": {\"y\": 1194.9157118841072, \"x\": 2961.5372236550047}, \"selected\": false, \"data\": {\"id_original\": \"2087\", \"name\": \"YML054C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"CYB2\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 3.5964e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"mitochondrial intermembrane space\", \"mitochondrion\"], \"alias\": [\"cytochrome b2\", \"CYB2\", \"L-lactate cytochrome c oxidoreductase\", \"S000004518\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"L-lactate dehydrogenase (cytochrome) activity\"], \"gal4RGsig\": 1.3947e-08, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YML054C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10504024, \"AverageShortestPathLength\": 9.52016129, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": -0.091, \"gal1RGexp\": 0.963, \"gal80Rsig\": 0.25421, \"Stress\": 1786.0, \"id\": \"1588\", \"gal4RGexp\": 0.856, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"electron transport\"], \"Radiality\": 0.68443847, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1588}}, {\"position\": {\"y\": 1316.7989058538337, \"x\": 2878.4678877175047}, \"selected\": false, \"data\": {\"id_original\": \"2088\", \"name\": \"YLR256W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"HAP1\", \"selected\": false, \"BetweennessCentrality\": 0.03202952, \"gal1RGsig\": 0.79725, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"CYP1\", \"HAP1\", \"S000004246\", \"zinc finger transcription factor of the Zn(2)-Cys(6) binuclear cluster domain type\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"specific RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 0.063023, \"TopologicalCoefficient\": 0.25, \"shared_name\": \"YLR256W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11720227, \"AverageShortestPathLength\": 8.53225806, \"NeighborhoodConnectivity\": 2.25, \"gal80Rexp\": 0.104, \"gal1RGexp\": 0.011, \"gal80Rsig\": 0.2243, \"Stress\": 7130.0, \"id\": \"1587\", \"gal4RGexp\": -0.087, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"aerobic respiration\", \"positive regulation of transcription from RNA polymerase II promoter\"], \"Radiality\": 0.72102748, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1587}}, {\"position\": {\"y\": 3839.1890578313732, \"x\": 1942.2270887673094}, \"selected\": false, \"data\": {\"id_original\": \"2089\", \"name\": \"YOR303W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"CPA1\", \"selected\": false, \"BetweennessCentrality\": 1.0, \"gal1RGsig\": 0.002137, \"annotation_GO_CELLULAR_COMPONENT\": [\"carbamoyl-phosphate synthase complex\"], \"alias\": [\"CPA1\", \"S000005829\", \"carbamoyl phosphate synthetase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"carbamoyl-phosphate synthase (glutamine-hydrolyzing) activity\"], \"gal4RGsig\": 0.15221, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOR303W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.403, \"gal1RGexp\": -0.127, \"gal80Rsig\": 0.00015854, \"Stress\": 2.0, \"id\": \"1586\", \"gal4RGexp\": -0.089, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"arginine biosynthetic process\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1586}}, {\"position\": {\"y\": 3777.1561293645764, \"x\": 1853.0695570290281}, \"selected\": false, \"data\": {\"id_original\": \"2090\", \"name\": \"YJR109C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"CPA2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.2917e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"carbamoyl-phosphate synthase complex\"], \"alias\": [\"CPA2\", \"S000003870\", \"carbamyl phosphate synthetase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"carbamoyl-phosphate synthase (glutamine-hydrolyzing) activity\"], \"gal4RGsig\": 0.61381, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YJR109C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.745, \"gal1RGexp\": -0.323, \"gal80Rsig\": 0.030981, \"Stress\": 0.0, \"id\": \"1585\", \"gal4RGexp\": -0.0, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"arginine biosynthetic process\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1585}}, {\"position\": {\"y\": 2764.946168427076, \"x\": 1570.1175459206297}, \"selected\": false, \"data\": {\"id_original\": \"2091\", \"name\": \"YGR058W\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"YGR058W\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.44242, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"S000003290\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.10763, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YGR058W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09927942, \"AverageShortestPathLength\": 10.07258065, \"NeighborhoodConnectivity\": 1.5, \"gal80Rexp\": -0.065, \"gal1RGexp\": 0.045, \"gal80Rsig\": 0.6708, \"Stress\": 1032.0, \"id\": \"1584\", \"gal4RGexp\": -0.16, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.66397849, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1584}}, {\"position\": {\"y\": 1514.1797385259529, \"x\": 2217.3064344704344}, \"selected\": false, \"data\": {\"id_original\": \"2092\", \"name\": \"YLR229C\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"CDC42\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.066961, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud neck\", \"bud tip\", \"mating projection tip\", \"plasma membrane\", \"soluble fraction\"], \"alias\": [\"CDC42\", \"S000004219\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"GTPase activity\", \"signal transducer activity\"], \"gal4RGsig\": 0.031442, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YLR229C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13618891, \"AverageShortestPathLength\": 7.34274194, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": -0.154, \"gal1RGexp\": -0.074, \"gal80Rsig\": 0.030339, \"Stress\": 750.0, \"id\": \"1583\", \"gal4RGexp\": 0.089, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Rho protein signal transduction\", \"budding cell apical bud growth\", \"budding cell isotropic bud growth\", \"establishment of cell polarity (sensu the Fungi research community)\", \"exocytosis\", \"invasive growth (sensu the Saccharomyces research community)\", \"pheromone-dependent signal transduction during conjugation with cellular fusion\", \"pseudohyphal growth\", \"regulation of exit from mitosis\", \"regulation of initiation of mating projection growth\"], \"Radiality\": 0.76508363, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1583}}, {\"position\": {\"y\": 1410.282594208326, \"x\": 2145.742284613867}, \"selected\": false, \"data\": {\"id_original\": \"2093\", \"name\": \"YDR309C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"GIC2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.6598e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"actin cap\", \"bud tip\", \"incipient bud site\", \"mating projection tip\"], \"alias\": [\"GIC2\", \"S000002717\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"small GTPase regulator activity\"], \"gal4RGsig\": 0.032663, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDR309C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11992263, \"AverageShortestPathLength\": 8.33870968, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.798, \"gal1RGexp\": -0.427, \"gal80Rsig\": 1.2554e-13, \"Stress\": 0.0, \"id\": \"1582\", \"gal4RGexp\": -0.129, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Rho protein signal transduction\", \"axial bud site selection\", \"establishment of cell polarity (sensu the Fungi research community)\", \"regulation of exit from mitosis\"], \"Radiality\": 0.72819594, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1582}}, {\"position\": {\"y\": 2684.3837904973884, \"x\": 1680.3298872292235}, \"selected\": false, \"data\": {\"id_original\": \"2094\", \"name\": \"YOR264W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"YOR264W\", \"selected\": false, \"BetweennessCentrality\": 0.01606373, \"gal1RGsig\": 1.939e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud neck\"], \"alias\": [\"DSE3\", \"S000005790\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.016862, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YOR264W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11007545, \"AverageShortestPathLength\": 9.08467742, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.307, \"gal1RGexp\": 0.493, \"gal80Rsig\": 0.011809, \"Stress\": 2060.0, \"id\": \"1581\", \"gal4RGexp\": 0.237, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.7005675, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1581}}, {\"position\": {\"y\": 2025.9331984563728, \"x\": 2436.967521506567}, \"selected\": false, \"data\": {\"id_original\": \"2095\", \"name\": \"YLR116W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"MSL5\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.74865, \"annotation_GO_CELLULAR_COMPONENT\": [\"commitment complex\"], \"alias\": [\"BBP\", \"MSL5\", \"S000004106\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\"], \"gal4RGsig\": 0.60558, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YLR116W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11412793, \"AverageShortestPathLength\": 8.76209677, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.101, \"gal1RGexp\": 0.015, \"gal80Rsig\": 0.35769, \"Stress\": 1044.0, \"id\": \"1580\", \"gal4RGexp\": -0.03, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nuclear mRNA splicing\", \"via spliceosome\"], \"Radiality\": 0.71251493, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1580}}, {\"position\": {\"y\": 1105.3435683294197, \"x\": 1452.476737815161}, \"selected\": false, \"data\": {\"id_original\": \"2096\", \"name\": \"YNL312W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"RFA2\", \"selected\": false, \"BetweennessCentrality\": 0.00401593, \"gal1RGsig\": 0.47284, \"annotation_GO_CELLULAR_COMPONENT\": [\"DNA replication factor A complex\", \"chromosome\", \"telomeric region\"], \"alias\": [\"BUF1\", \"RFA2\", \"RPA2\", \"S000005256\", \"replication factor RF-A subunit 2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA binding\"], \"gal4RGsig\": 0.034589, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YNL312W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10222589, \"AverageShortestPathLength\": 9.78225806, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": -0.121, \"gal1RGexp\": 0.028, \"gal80Rsig\": 0.24786, \"Stress\": 1030.0, \"id\": \"1579\", \"gal4RGexp\": -0.111, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"DNA recombination\", \"DNA replication\", \"synthesis of RNA primer\", \"DNA strand elongation during DNA replication\", \"DNA unwinding during replication\", \"double-strand break repair via homologous recombination\", \"nucleotide-excision repair\", \"postreplication repair\"], \"Radiality\": 0.67473118, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1579}}, {\"position\": {\"y\": 1015.0552992864509, \"x\": 1373.8671797096922}, \"selected\": false, \"data\": {\"id_original\": \"2097\", \"name\": \"YML032C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"RAD52\", \"selected\": false, \"BetweennessCentrality\": 1.632e-05, \"gal1RGsig\": 0.00033893, \"annotation_GO_CELLULAR_COMPONENT\": [\"nuclear chromosome\", \"nucleus\"], \"alias\": [\"RAD52\", \"S000004494\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA strand annealing activity\", \"recombinase activity\"], \"gal4RGsig\": 0.033332, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YML032C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09284912, \"AverageShortestPathLength\": 10.77016129, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.363, \"gal1RGexp\": 0.199, \"gal80Rsig\": 0.0030735, \"Stress\": 2.0, \"id\": \"1578\", \"gal4RGexp\": -0.136, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"DNA recombinase assembly\", \"double-strand break repair via break-induced replication\", \"double-strand break repair via single-strand annealing\", \"double-strand break repair via synthesis-dependent strand annealing\", \"meiotic DNA recombinase assembly\", \"postreplication repair\", \"telomere maintenance via recombination\"], \"Radiality\": 0.63814217, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1578}}, {\"position\": {\"y\": 1906.432984833326, \"x\": 2377.982871848364}, \"selected\": false, \"data\": {\"id_original\": \"2098\", \"name\": \"YKL012W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"PRP40\", \"selected\": false, \"BetweennessCentrality\": 0.03199687, \"gal1RGsig\": 0.00042719, \"annotation_GO_CELLULAR_COMPONENT\": [\"snRNP U1\"], \"alias\": [\"PRP40\", \"S000001495\", \"U1 snRNP protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\"], \"gal4RGsig\": 0.00035398, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YKL012W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12863071, \"AverageShortestPathLength\": 7.77419355, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.561, \"gal1RGexp\": 0.21, \"gal80Rsig\": 8.7173e-05, \"Stress\": 4160.0, \"id\": \"1577\", \"gal4RGexp\": -0.277, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nuclear mRNA splicing\", \"via spliceosome\"], \"Radiality\": 0.74910394, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1577}}, {\"position\": {\"y\": 2043.9910292669197, \"x\": 2344.253852683325}, \"selected\": false, \"data\": {\"id_original\": \"2099\", \"name\": \"YNL236W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"SIN4\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.018347, \"annotation_GO_CELLULAR_COMPONENT\": [\"mediator complex\"], \"alias\": [\"BEL2\", \"GAL22\", \"MED16\", \"RNA polymerase II holoenzyme/mediator subunit\", \"RYE1\", \"S000005180\", \"SDI3\", \"SIN4\", \"SSF5\", \"SSN4\", \"TSF3\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA polymerase II transcription mediator activity\"], \"gal4RGsig\": 0.053936, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YNL236W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11412793, \"AverageShortestPathLength\": 8.76209677, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.141, \"gal1RGexp\": -0.146, \"gal80Rsig\": 0.39009, \"Stress\": 1044.0, \"id\": \"1576\", \"gal4RGexp\": -0.218, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"transcription from RNA polymerase II promoter\"], \"Radiality\": 0.71251493, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1576}}, {\"position\": {\"y\": 901.1400771184822, \"x\": 2535.799003440161}, \"selected\": false, \"data\": {\"id_original\": \"2100\", \"name\": \"YNL091W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"YNL091W\", \"selected\": false, \"BetweennessCentrality\": 0.0436549, \"gal1RGsig\": 1.9735e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"NST1\", \"S000005035\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.026384, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YNL091W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13383702, \"AverageShortestPathLength\": 7.47177419, \"NeighborhoodConnectivity\": 2.66666667, \"gal80Rexp\": 0.723, \"gal1RGexp\": 0.288, \"gal80Rsig\": 0.00023704, \"Stress\": 5154.0, \"id\": \"1575\", \"gal4RGexp\": 0.154, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"response to salt stress\"], \"Radiality\": 0.76030466, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1575}}, {\"position\": {\"y\": 1259.3157362981697, \"x\": 2746.851798850317}, \"selected\": false, \"data\": {\"id_original\": \"2101\", \"name\": \"YDR184C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"ATC1\", \"selected\": false, \"BetweennessCentrality\": 0.07028775, \"gal1RGsig\": 0.4641, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"ATC1\", \"LIC4\", \"S000002592\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 9.3996e-07, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YDR184C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14043035, \"AverageShortestPathLength\": 7.12096774, \"NeighborhoodConnectivity\": 3.5, \"gal80Rexp\": 0.404, \"gal1RGexp\": 0.04, \"gal80Rsig\": 0.10407, \"Stress\": 6204.0, \"id\": \"1574\", \"gal4RGexp\": -0.886, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"bipolar bud site selection\", \"response to stress\", \"telomere maintenance\"], \"Radiality\": 0.77329749, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1574}}, {\"position\": {\"y\": 1156.911012177076, \"x\": 2702.563224631567}, \"selected\": false, \"data\": {\"id_original\": \"2102\", \"name\": \"YIL143C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"SSL2\", \"selected\": false, \"BetweennessCentrality\": 0.01606373, \"gal1RGsig\": 0.019613, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleotide-excision repair factor 3 complex\", \"transcription factor TFIIH complex\"], \"alias\": [\"DNA helicase\", \"LOM3\", \"RAD25\", \"S000001405\", \"SSL2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA helicase activity\", \"general RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 0.36733, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YIL143C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12876428, \"AverageShortestPathLength\": 7.76612903, \"NeighborhoodConnectivity\": 3.5, \"gal80Rexp\": 0.264, \"gal1RGexp\": 0.124, \"gal80Rsig\": 0.045357, \"Stress\": 1220.0, \"id\": \"1573\", \"gal4RGexp\": 0.069, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"negative regulation of transcription from RNA polymerase II promoter\", \"mitotic\", \"nucleotide-excision repair\", \"DNA duplex unwinding\", \"transcription initiation from RNA polymerase II promoter\"], \"Radiality\": 0.74940263, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1573}}, {\"position\": {\"y\": 1187.3058486028572, \"x\": 2464.372489768286}, \"selected\": false, \"data\": {\"id_original\": \"2103\", \"name\": \"YKR099W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"BAS1\", \"selected\": false, \"BetweennessCentrality\": 0.10314677, \"gal1RGsig\": 6.1231e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"BAS1\", \"S000001807\", \"transcription factor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 7.6476e-06, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YKR099W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.15470992, \"AverageShortestPathLength\": 6.46370968, \"NeighborhoodConnectivity\": 3.66666667, \"gal80Rexp\": 1.101, \"gal1RGexp\": 0.466, \"gal80Rsig\": 0.00069896, \"Stress\": 12284.0, \"id\": \"1572\", \"gal4RGexp\": -0.936, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"histidine biosynthetic process\", \"purine base biosynthetic process\", \"transcription from RNA polymerase II promoter\"], \"Radiality\": 0.79764038, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1572}}, {\"position\": {\"y\": 1094.137330536451, \"x\": 2615.5359419167235}, \"selected\": false, \"data\": {\"id_original\": \"2104\", \"name\": \"YIR009W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"MSL1\", \"selected\": false, \"BetweennessCentrality\": 0.12134232, \"gal1RGsig\": 0.74397, \"annotation_GO_CELLULAR_COMPONENT\": [\"snRNP U2\"], \"alias\": [\"YU2B''\", \"MSL1\", \"S000001448\", \"U2 snRNP component\", \"YIB9\", \"YIB9w\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\"], \"gal4RGsig\": 0.19987, \"TopologicalCoefficient\": 0.2, \"shared_name\": \"YIR009W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14735591, \"AverageShortestPathLength\": 6.78629032, \"NeighborhoodConnectivity\": 2.2, \"gal80Rexp\": -0.049, \"gal1RGexp\": -0.044, \"gal80Rsig\": 0.89526, \"Stress\": 12160.0, \"id\": \"1571\", \"gal4RGexp\": -0.337, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nuclear mRNA splicing\", \"via spliceosome\"], \"Radiality\": 0.78569295, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 5.0, \"Degree\": 5, \"SUID\": 1571}}, {\"position\": {\"y\": 1679.6256728216072, \"x\": 2489.6777876198485}, \"selected\": false, \"data\": {\"id_original\": \"2105\", \"name\": \"YBR018C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"GAL7\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00078855, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"GAL7\", \"S000000222\", \"galactose-1-phosphate uridyl transferase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"UTP:galactose-1-phosphate uridylyltransferase activity\"], \"gal4RGsig\": 3.6284e-11, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YBR018C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13107822, \"AverageShortestPathLength\": 7.62903226, \"NeighborhoodConnectivity\": 7.5, \"gal80Rexp\": 3.126, \"gal1RGexp\": 0.153, \"gal80Rsig\": 3.9427e-17, \"Stress\": 0.0, \"id\": \"1570\", \"gal4RGexp\": -1.995, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"galactose catabolic process\"], \"Radiality\": 0.75448029, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1570}}, {\"position\": {\"y\": 1522.1148257299324, \"x\": 2548.2080915749266}, \"selected\": false, \"data\": {\"id_original\": \"2106\", \"name\": \"YPL248C\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"GAL4\", \"selected\": false, \"BetweennessCentrality\": 0.05907389, \"gal1RGsig\": 0.11614, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"GAL4\", \"GAL81\", \"S000006169\", \"zinc finger transcription factor of the Zn(2)-Cys(6) binuclear cluster domain type\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcription factor activity\", \"transcriptional activator activity\"], \"gal4RGsig\": 0.0001264, \"TopologicalCoefficient\": 0.19473684, \"shared_name\": \"YPL248C\", \"PartnerOfMultiEdgedNodePairs\": 1.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.15066829, \"AverageShortestPathLength\": 6.63709677, \"NeighborhoodConnectivity\": 3.9, \"gal80Rexp\": -0.211, \"gal1RGexp\": 0.1, \"gal80Rsig\": 0.088214, \"Stress\": 10716.0, \"id\": \"1569\", \"gal4RGexp\": -0.758, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"galactose metabolic process\", \"positive regulation of transcription by galactose\"], \"Radiality\": 0.79121864, \"ClusteringCoefficient\": 0.17777778, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 11.0, \"Degree\": 11, \"SUID\": 1569}}, {\"position\": {\"y\": 1618.53871298274, \"x\": 2656.065360862036}, \"selected\": false, \"data\": {\"id_original\": \"2107\", \"name\": \"YLR081W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"GAL2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00024597, \"annotation_GO_CELLULAR_COMPONENT\": [\"plasma membrane\"], \"alias\": [\"GAL2\", \"S000004071\", \"galactose permease\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"galactose transporter activity\", \"glucose transporter activity\"], \"gal4RGsig\": 1.6652e-07, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YLR081W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13107822, \"AverageShortestPathLength\": 7.62903226, \"NeighborhoodConnectivity\": 7.5, \"gal80Rexp\": 0.892, \"gal1RGexp\": 0.176, \"gal80Rsig\": 7.3429e-10, \"Stress\": 0.0, \"id\": \"1568\", \"gal4RGexp\": -0.57, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"galactose metabolic process\", \"galactose transport\"], \"Radiality\": 0.75448029, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1568}}, {\"position\": {\"y\": 1541.740679030027, \"x\": 2455.7509992897703}, \"selected\": false, \"data\": {\"id_original\": \"2108\", \"name\": \"YBR020W\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"GAL1\", \"selected\": false, \"BetweennessCentrality\": 0.00432532, \"gal1RGsig\": 7.0101e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"GAL1\", \"S000000224\", \"galactokinase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"galactokinase activity\", \"transcription regulator activity\"], \"gal4RGsig\": 2.5038e-09, \"TopologicalCoefficient\": 0.42647059, \"shared_name\": \"YBR020W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14718101, \"AverageShortestPathLength\": 6.79435484, \"NeighborhoodConnectivity\": 7.25, \"gal80Rexp\": 2.939, \"gal1RGexp\": -2.426, \"gal80Rsig\": 2.8147e-18, \"Stress\": 1638.0, \"id\": \"1567\", \"gal4RGexp\": -2.406, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"galactose catabolic process\", \"galactose transport\", \"positive regulation of transcription by galactose\"], \"Radiality\": 0.78539427, \"ClusteringCoefficient\": 0.5, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1567}}, {\"position\": {\"y\": 1510.228524689283, \"x\": 2359.2825239479735}, \"selected\": false, \"data\": {\"id_original\": \"2109\", \"name\": \"YGL035C\", \"Eccentricity\": 14, \"IsSingleNode\": false, \"COMMON\": \"MIG1\", \"selected\": false, \"BetweennessCentrality\": 0.40813644, \"gal1RGsig\": 1.102e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"C2H2 zinc finger protein that resembles the mammalian Egr and Wilms tumour proteins\", \"CAT4\", \"MIG1\", \"S000003003\", \"SSN1\", \"TDS22\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"specific RNA polymerase II transcription factor activity\"], \"gal4RGsig\": 1.7172e-06, \"TopologicalCoefficient\": 0.14354067, \"shared_name\": \"YGL035C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1707989, \"AverageShortestPathLength\": 5.85483871, \"NeighborhoodConnectivity\": 3.36363636, \"gal80Rexp\": -0.28, \"gal1RGexp\": 0.345, \"gal80Rsig\": 0.0070533, \"Stress\": 92790.0, \"id\": \"1566\", \"gal4RGexp\": 0.31, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glucose metabolic process\", \"regulation of transcription from RNA polymerase II promoter\"], \"Radiality\": 0.82019116, \"ClusteringCoefficient\": 0.05454545, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 11.0, \"Degree\": 11, \"SUID\": 1566}}, {\"position\": {\"y\": 1619.1160445257087, \"x\": 2553.752921897192}, \"selected\": false, \"data\": {\"id_original\": \"2110\", \"name\": \"YOL051W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"GAL11\", \"selected\": false, \"BetweennessCentrality\": 9.251e-05, \"gal1RGsig\": 0.001834, \"annotation_GO_CELLULAR_COMPONENT\": [\"mediator complex\"], \"alias\": [\"positive and negative transcriptional regulator of genes involved in mating-type specialization\", \"ABE1\", \"GAL11\", \"MED15\", \"RAR3\", \"RNA polymerase II holoenzyme complex component\", \"S000005411\", \"SDS4\", \"SPT13\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA polymerase II transcription mediator activity\"], \"gal4RGsig\": 0.33923, \"TopologicalCoefficient\": 0.42, \"shared_name\": \"YOL051W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13128639, \"AverageShortestPathLength\": 7.61693548, \"NeighborhoodConnectivity\": 4.2, \"gal80Rexp\": 0.111, \"gal1RGexp\": 0.171, \"gal80Rsig\": 0.30922, \"Stress\": 12.0, \"id\": \"1565\", \"gal4RGexp\": 0.055, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"telomere maintenance\", \"transcription from RNA polymerase II promoter\"], \"Radiality\": 0.75492832, \"ClusteringCoefficient\": 0.4, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 5.0, \"Degree\": 5, \"SUID\": 1565}}, {\"position\": {\"y\": 1600.964307312574, \"x\": 2437.062949973364}, \"selected\": false, \"data\": {\"id_original\": \"2111\", \"name\": \"YBR019C\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"GAL10\", \"selected\": false, \"BetweennessCentrality\": 0.0021545, \"gal1RGsig\": 0.098603, \"annotation_GO_CELLULAR_COMPONENT\": [\"soluble fraction\"], \"alias\": [\"GAL10\", \"S000000223\", \"UDP-glucose 4-epimerase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"UDP-glucose 4-epimerase activity\", \"aldose 1-epimerase activity\"], \"gal4RGsig\": 3.3164e-11, \"TopologicalCoefficient\": 0.50980392, \"shared_name\": \"YBR019C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14709371, \"AverageShortestPathLength\": 6.7983871, \"NeighborhoodConnectivity\": 8.66666667, \"gal80Rexp\": 2.856, \"gal1RGexp\": 0.061, \"gal80Rsig\": 3.9398e-18, \"Stress\": 818.0, \"id\": \"1564\", \"gal4RGexp\": -1.993, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"galactose catabolic process\"], \"Radiality\": 0.78524492, \"ClusteringCoefficient\": 0.66666667, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1564}}, {\"position\": {\"y\": 613.3799452825447, \"x\": 2383.4248121559813}, \"selected\": false, \"data\": {\"id_original\": \"2112\", \"name\": \"YJR060W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"CBF1\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.0013953, \"annotation_GO_CELLULAR_COMPONENT\": [\"kinetochore\", \"mitochondrion\", \"nucleus\"], \"alias\": [\"CBF1\", \"CEP1\", \"CPF1\", \"S000003821\", \"basic helix-loop-helix protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA binding\", \"centromeric DNA binding\"], \"gal4RGsig\": 0.0034755, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YJR060W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12633724, \"AverageShortestPathLength\": 7.91532258, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.103, \"gal1RGexp\": 0.165, \"gal80Rsig\": 0.43529, \"Stress\": 614.0, \"id\": \"1563\", \"gal4RGexp\": -0.306, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"chromatin assembly or disassembly\", \"chromosome segregation\", \"methionine biosynthetic process\"], \"Radiality\": 0.74387694, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1563}}, {\"position\": {\"y\": 1570.0508170171638, \"x\": 2955.456962424536}, \"selected\": false, \"data\": {\"id_original\": \"2113\", \"name\": \"YDR103W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"STE5\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.34875, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"mating projection tip\", \"nucleus\", \"plasma membrane\"], \"alias\": [\"HMD3\", \"NUL3\", \"S000002510\", \"STE5\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"MAP-kinase scaffold activity\"], \"gal4RGsig\": 0.42858, \"TopologicalCoefficient\": 0.59090909, \"shared_name\": \"YDR103W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12757202, \"AverageShortestPathLength\": 7.83870968, \"NeighborhoodConnectivity\": 6.5, \"gal80Rexp\": 0.023, \"gal1RGexp\": -0.068, \"gal80Rsig\": 0.92304, \"Stress\": 0.0, \"id\": \"1562\", \"gal4RGexp\": -0.121, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"invasive growth (sensu the Saccharomyces research community)\", \"pheromone-dependent signal transduction during conjugation with cellular fusion\"], \"Radiality\": 0.74671446, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1562}}, {\"position\": {\"y\": 1667.8257536931892, \"x\": 2862.2423017800047}, \"selected\": false, \"data\": {\"id_original\": \"2114\", \"name\": \"YLR362W\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"STE11\", \"selected\": false, \"BetweennessCentrality\": 0.22363878, \"gal1RGsig\": 0.023963, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"S000004354\", \"STE11\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"MAP kinase kinase kinase activity\", \"SAM domain binding\"], \"gal4RGsig\": 0.50139, \"TopologicalCoefficient\": 0.14444444, \"shared_name\": \"YLR362W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14571093, \"AverageShortestPathLength\": 6.86290323, \"NeighborhoodConnectivity\": 2.22222222, \"gal80Rexp\": 0.374, \"gal1RGexp\": 0.144, \"gal80Rsig\": 0.0010869, \"Stress\": 21270.0, \"id\": \"1561\", \"gal4RGexp\": 0.037, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"invasive growth (sensu the Saccharomyces research community)\", \"pheromone-dependent signal transduction during conjugation with cellular fusion\", \"protein amino acid phosphorylation\", \"pseudohyphal growth\"], \"Radiality\": 0.78285544, \"ClusteringCoefficient\": 0.02777778, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 9.0, \"Degree\": 9, \"SUID\": 1561}}, {\"position\": {\"y\": 1486.3341002508064, \"x\": 3152.1379926979735}, \"selected\": false, \"data\": {\"id_original\": \"2115\", \"name\": \"YDR032C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"YDR032C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.026191, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"mitochondrion\"], \"alias\": [\"PST2\", \"S000002439\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 2.0333e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDR032C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11339735, \"AverageShortestPathLength\": 8.81854839, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": -0.211, \"gal1RGexp\": 0.113, \"gal80Rsig\": 0.00071391, \"Stress\": 0.0, \"id\": \"1560\", \"gal4RGexp\": 0.331, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.71042413, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1560}}, {\"position\": {\"y\": 1575.6389212652107, \"x\": 3066.084159690161}, \"selected\": false, \"data\": {\"id_original\": \"2116\", \"name\": \"YCL032W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"STE50\", \"selected\": false, \"BetweennessCentrality\": 0.03196422, \"gal1RGsig\": 0.003766, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"S000000537\", \"STE50\", \"protein kinase regulator\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"SAM domain binding\", \"protein kinase regulator activity\"], \"gal4RGsig\": 0.71364, \"TopologicalCoefficient\": 0.29545455, \"shared_name\": \"YCL032W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12783505, \"AverageShortestPathLength\": 7.82258065, \"NeighborhoodConnectivity\": 3.75, \"gal80Rexp\": 0.284, \"gal1RGexp\": 0.126, \"gal80Rsig\": 0.015244, \"Stress\": 2454.0, \"id\": \"1559\", \"gal4RGexp\": 0.02, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"MAPKKK cascade during osmolarity sensing\", \"pheromone-dependent signal transduction during conjugation with cellular fusion\", \"response to pheromone\", \"signal transduction during filamentous growth\"], \"Radiality\": 0.74731183, \"ClusteringCoefficient\": 0.16666667, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1559}}, {\"position\": {\"y\": 1606.4635405584236, \"x\": 1066.224052268286}, \"selected\": false, \"data\": {\"id_original\": \"2117\", \"name\": \"YLR109W\", \"Eccentricity\": 27, \"IsSingleNode\": false, \"COMMON\": \"AHP1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.4814e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"cTPxIII\", \"AHP1\", \"S000004099\", \"alkyl hydroperoxide reductase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"thioredoxin peroxidase activity\"], \"gal4RGsig\": 0.00025668, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLR109W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.07101947, \"AverageShortestPathLength\": 14.08064516, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.486, \"gal1RGexp\": -0.603, \"gal80Rsig\": 2.3432e-11, \"Stress\": 0.0, \"id\": \"1558\", \"gal4RGexp\": 0.466, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of cell redox homeostasis\", \"response to metal ion\", \"response to oxidative stress\"], \"Radiality\": 0.51553166, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1558}}, {\"position\": {\"y\": 1698.7036757512947, \"x\": 1035.0127485573485}, \"selected\": false, \"data\": {\"id_original\": \"2118\", \"name\": \"YHR141C\", \"Eccentricity\": 27, \"IsSingleNode\": false, \"COMMON\": \"RPL42B\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 7.7434e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"MAK18\", \"RPL42B\", \"S000001183\", \"ribosomal protein L42B (YL27) (L41B) (YP44)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 2.5233e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YHR141C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.07101947, \"AverageShortestPathLength\": 14.08064516, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.139, \"gal1RGexp\": -0.359, \"gal80Rsig\": 0.012998, \"Stress\": 0.0, \"id\": \"1557\", \"gal4RGexp\": -0.371, \"Radiality\": 0.51553166, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1557}}, {\"position\": {\"y\": 1691.9194045110603, \"x\": 1157.763114768286}, \"selected\": false, \"data\": {\"id_original\": \"2119\", \"name\": \"YMR138W\", \"Eccentricity\": 26, \"IsSingleNode\": false, \"COMMON\": \"CIN4\", \"selected\": false, \"BetweennessCentrality\": 0.01609638, \"gal1RGsig\": 0.037256, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"CIN4\", \"GTP-binding protein\", \"GTP1\", \"S000004746\", \"UGX1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"GTP binding\"], \"gal4RGsig\": 0.39944, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YMR138W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.07642527, \"AverageShortestPathLength\": 13.08467742, \"NeighborhoodConnectivity\": 1.33333333, \"gal80Rexp\": 0.221, \"gal1RGexp\": 0.414, \"gal80Rsig\": 0.12805, \"Stress\": 5442.0, \"id\": \"1556\", \"gal4RGexp\": -0.0, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"microtubule-based process\"], \"Radiality\": 0.55241935, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1556}}, {\"position\": {\"y\": 1085.4688429876228, \"x\": 2073.830638724585}, \"selected\": false, \"data\": {\"id_original\": \"2120\", \"name\": \"YMR300C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"ADE4\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.522e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"ADE4\", \"S000004915\", \"phosphoribosylpyrophosphate amidotransferase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"amidophosphoribosyltransferase activity\"], \"gal4RGsig\": 1.4734e-08, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YMR300C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11605054, \"AverageShortestPathLength\": 8.61693548, \"NeighborhoodConnectivity\": 7.0, \"gal80Rexp\": 1.202, \"gal1RGexp\": -0.514, \"gal80Rsig\": 4.7716e-11, \"Stress\": 0.0, \"id\": \"1555\", \"gal4RGexp\": -1.296, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"'de novo' IMP biosynthetic process\", \"purine base metabolic process\", \"purine nucleotide biosynthetic process\"], \"Radiality\": 0.71789128, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1555}}, {\"position\": {\"y\": 1191.7399611516853, \"x\": 1955.8763349831297}, \"selected\": false, \"data\": {\"id_original\": \"2121\", \"name\": \"YOL058W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"ARG1\", \"selected\": false, \"BetweennessCentrality\": 0.02615237, \"gal1RGsig\": 4.9342e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\"], \"alias\": [\"ARG1\", \"ARG10\", \"S000005419\", \"arginosuccinate synthetase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"argininosuccinate synthase activity\"], \"gal4RGsig\": 4.0958e-07, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YOL058W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12704918, \"AverageShortestPathLength\": 7.87096774, \"NeighborhoodConnectivity\": 6.0, \"gal80Rexp\": -0.815, \"gal1RGexp\": -0.652, \"gal80Rsig\": 5.9432e-10, \"Stress\": 6768.0, \"id\": \"1554\", \"gal4RGexp\": -0.541, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"arginine biosynthetic process\", \"argininosuccinate metabolic process\", \"citrulline metabolic process\"], \"Radiality\": 0.74551971, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1554}}, {\"position\": {\"y\": 1157.5398573919197, \"x\": 2035.8174207985594}, \"selected\": false, \"data\": {\"id_original\": \"2122\", \"name\": \"YBR248C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"HIS7\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 2.3151e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"intracellular\"], \"alias\": [\"HIS7\", \"S000000452\", \"imidazole glycerol phosphate synthase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"imidazoleglycerol-phosphate synthase activity\"], \"gal4RGsig\": 1.6549e-07, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YBR248C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11605054, \"AverageShortestPathLength\": 8.61693548, \"NeighborhoodConnectivity\": 7.0, \"gal80Rexp\": 0.898, \"gal1RGexp\": -0.258, \"gal80Rsig\": 3.3047e-05, \"Stress\": 0.0, \"id\": \"1553\", \"gal4RGexp\": -1.252, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"histidine biosynthetic process\", \"purine nucleoside monophosphate biosynthetic process\"], \"Radiality\": 0.71789128, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1553}}, {\"position\": {\"y\": 1258.1541457219978, \"x\": 2239.723045188208}, \"selected\": false, \"data\": {\"id_original\": \"2123\", \"name\": \"YOR202W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"HIS3\", \"selected\": false, \"BetweennessCentrality\": 0.00612624, \"gal1RGsig\": 0.010979, \"annotation_GO_CELLULAR_COMPONENT\": [\"intracellular\"], \"alias\": [\"HIS10\", \"HIS3\", \"S000005728\", \"imidazoleglycerol-phosphate dehydratase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"imidazoleglycerol-phosphate dehydratase activity\"], \"gal4RGsig\": 0.00017979, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YOR202W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12849741, \"AverageShortestPathLength\": 7.78225806, \"NeighborhoodConnectivity\": 4.5, \"gal80Rexp\": 0.239, \"gal1RGexp\": -0.432, \"gal80Rsig\": 0.0054895, \"Stress\": 1810.0, \"id\": \"1552\", \"gal4RGexp\": -0.71, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"histidine biosynthetic process\"], \"Radiality\": 0.74880526, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1552}}, {\"position\": {\"y\": 1192.0836806341072, \"x\": 2266.282127219458}, \"selected\": false, \"data\": {\"id_original\": \"2124\", \"name\": \"YMR108W\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"ILV2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.27002, \"annotation_GO_CELLULAR_COMPONENT\": [\"acetolactate synthase complex\", \"mitochondrion\"], \"alias\": [\"ILV2\", \"S000004714\", \"SMR1\", \"THI1\", \"acetolactate synthase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"FAD binding\", \"acetolactate synthase activity\"], \"gal4RGsig\": 0.00094314, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YMR108W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11605054, \"AverageShortestPathLength\": 8.61693548, \"NeighborhoodConnectivity\": 7.0, \"gal80Rexp\": 0.095, \"gal1RGexp\": 0.04, \"gal80Rsig\": 0.14717, \"Stress\": 0.0, \"id\": \"1551\", \"gal4RGexp\": -0.358, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"branched chain family amino acid biosynthetic process\"], \"Radiality\": 0.71789128, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1551}}, {\"position\": {\"y\": 1170.7133803411384, \"x\": 2143.570605117102}, \"selected\": false, \"data\": {\"id_original\": \"2125\", \"name\": \"YEL009C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"GCN4\", \"selected\": false, \"BetweennessCentrality\": 0.06299561, \"gal1RGsig\": 0.33125, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"AAS3\", \"ARG9\", \"GCN4\", \"S000000735\", \"transcriptional activator of amino acid biosynthetic genes\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA binding\", \"transcription factor activity\"], \"gal4RGsig\": 0.41727, \"TopologicalCoefficient\": 0.14285714, \"shared_name\": \"YEL009C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13121693, \"AverageShortestPathLength\": 7.62096774, \"NeighborhoodConnectivity\": 1.57142857, \"gal80Rexp\": -0.031, \"gal1RGexp\": 0.035, \"gal80Rsig\": 0.55639, \"Stress\": 14390.0, \"id\": \"1550\", \"gal4RGexp\": 0.032, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"amino acid biosynthetic process\", \"regulation of transcription from RNA polymerase II promoter\", \"unfolded protein response\", \"positive regulation of target gene transcription\"], \"Radiality\": 0.75477897, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 7.0, \"Degree\": 7, \"SUID\": 1550}}, {\"position\": {\"y\": 1849.0892043157478, \"x\": 3029.1602705300047}, \"selected\": false, \"data\": {\"id_original\": \"2126\", \"name\": \"YBR155W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"CNS1\", \"selected\": false, \"BetweennessCentrality\": 0.00060402, \"gal1RGsig\": 0.10014, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"CNS1\", \"S000000359\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"Hsp70 protein binding\", \"Hsp90 protein binding\"], \"gal4RGsig\": 1.5959e-05, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YBR155W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1170916, \"AverageShortestPathLength\": 8.54032258, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.519, \"gal1RGexp\": 0.082, \"gal80Rsig\": 0.00037064, \"Stress\": 82.0, \"id\": \"1549\", \"gal4RGexp\": -0.479, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein folding\"], \"Radiality\": 0.72072879, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1549}}, {\"position\": {\"y\": 1757.5753035589119, \"x\": 2958.0823896706297}, \"selected\": false, \"data\": {\"id_original\": \"2127\", \"name\": \"YMR186W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"HSC82\", \"selected\": false, \"BetweennessCentrality\": 0.00339559, \"gal1RGsig\": 0.52469, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"mitochondrion\"], \"alias\": [\"HSC82\", \"HSP90\", \"S000004798\", \"chaperonin\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"ATPase activity\", \"coupled\", \"unfolded protein binding\"], \"gal4RGsig\": 0.4995, \"TopologicalCoefficient\": 0.5625, \"shared_name\": \"YMR186W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12737545, \"AverageShortestPathLength\": 7.85080645, \"NeighborhoodConnectivity\": 5.5, \"gal80Rexp\": -0.608, \"gal1RGexp\": -0.032, \"gal80Rsig\": 1.6833e-14, \"Stress\": 530.0, \"id\": \"1548\", \"gal4RGexp\": -0.032, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"'de novo' protein folding\", \"proteasome assembly\", \"protein folding\", \"protein refolding\", \"response to stress\", \"telomere maintenance\"], \"Radiality\": 0.74626643, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1548}}, {\"position\": {\"y\": 3585.9258437200447, \"x\": 1356.3644789040281}, \"selected\": false, \"data\": {\"id_original\": \"2128\", \"name\": \"YGL106W\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"MLC1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.3141e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud neck\", \"bud tip\", \"vesicle\"], \"alias\": [\"MLC1\", \"S000003074\", \"myosin Myo2p light chain\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"microfilament motor activity\", \"myosin II heavy chain binding\"], \"gal4RGsig\": 9.2389e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGL106W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.44444444, \"AverageShortestPathLength\": 2.25, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.059, \"gal1RGexp\": -0.262, \"gal80Rsig\": 0.29618, \"Stress\": 0.0, \"id\": \"1547\", \"gal4RGexp\": -0.327, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"budding cell apical bud growth\", \"budding cell isotropic bud growth\", \"cytokinesis\", \"cytokinesis\", \"contractile ring formation\", \"endocytosis\", \"establishment of cell polarity (sensu the Fungi research community)\", \"membrane addition at site of cytokinesis\", \"mitochondrion inheritance\", \"vacuole inheritance\", \"vesicle-mediated transport\"], \"Radiality\": 0.58333333, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1547}}, {\"position\": {\"y\": 3555.7187361760994, \"x\": 1463.0383985817625}, \"selected\": false, \"data\": {\"id_original\": \"2129\", \"name\": \"YOR326W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"MYO2\", \"selected\": false, \"BetweennessCentrality\": 0.5, \"gal1RGsig\": 5.9183e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"actin cable\", \"bud neck\", \"bud tip\", \"filamentous actin\", \"incipient bud site\", \"mating projection tip\", \"myosin V complex\", \"vacuolar membrane (sensu the Fungi research community)\", \"vesicle\"], \"alias\": [\"CDC66\", \"MYO2\", \"S000005853\", \"class V myosin\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"actin filament binding\", \"calmodulin binding\", \"microfilament motor activity\"], \"gal4RGsig\": 8.2407e-08, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YOR326W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.296, \"gal1RGexp\": 0.51, \"gal80Rsig\": 0.00011583, \"Stress\": 6.0, \"id\": \"1546\", \"gal4RGexp\": 0.432, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Golgi inheritance\", \"budding cell apical bud growth\", \"establishment of mitotic spindle orientation\", \"membrane addition at site of cytokinesis\", \"mitochondrion inheritance\", \"peroxisome inheritance\", \"unidimensional cell growth\", \"vacuole inheritance\", \"vesicle transport along actin filament\", \"vesicle-mediated transport\"], \"Radiality\": 0.83333333, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1546}}, {\"position\": {\"y\": 1909.9678359075447, \"x\": 1388.2486494362547}, \"selected\": false, \"data\": {\"id_original\": \"2130\", \"name\": \"YMR309C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"NIP1\", \"selected\": false, \"BetweennessCentrality\": 0.01979124, \"gal1RGsig\": 0.82076, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"eukaryotic translation initiation factor 3 complex\", \"multi-eIF complex\"], \"alias\": [\"NIP1\", \"S000004926\", \"translation initiation factor eIF3 subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"translation initiation factor activity\"], \"gal4RGsig\": 0.00020319, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YMR309C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10218377, \"AverageShortestPathLength\": 9.78629032, \"NeighborhoodConnectivity\": 2.33333333, \"gal80Rexp\": -0.123, \"gal1RGexp\": 0.009, \"gal80Rsig\": 0.1009, \"Stress\": 5122.0, \"id\": \"1545\", \"gal4RGexp\": -0.248, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"translational initiation\"], \"Radiality\": 0.67458184, \"ClusteringCoefficient\": 0.33333333, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1545}}, {\"position\": {\"y\": 1976.6960158391853, \"x\": 1241.1145551979735}, \"selected\": false, \"data\": {\"id_original\": \"2131\", \"name\": \"YOR361C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"PRT1\", \"selected\": false, \"BetweennessCentrality\": 0.01710209, \"gal1RGsig\": 0.60784, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"eukaryotic translation initiation factor 3 complex\", \"multi-eIF complex\"], \"alias\": [\"CDC63\", \"DNA26\", \"PRT1\", \"S000005888\", \"translation initiation factor eIF3 subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"translation initiation factor activity\"], \"gal4RGsig\": 0.11638, \"TopologicalCoefficient\": 0.46666667, \"shared_name\": \"YOR361C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.101473, \"AverageShortestPathLength\": 9.85483871, \"NeighborhoodConnectivity\": 2.66666667, \"gal80Rexp\": -0.237, \"gal1RGexp\": -0.02, \"gal80Rsig\": 0.0031451, \"Stress\": 4108.0, \"id\": \"1544\", \"gal4RGexp\": -0.085, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"translational initiation\"], \"Radiality\": 0.67204301, \"ClusteringCoefficient\": 0.33333333, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1544}}, {\"position\": {\"y\": 1627.5955519719978, \"x\": 1768.1001814186766}, \"selected\": false, \"data\": {\"id_original\": \"2132\", \"name\": \"YIL105C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"YIL105C\", \"selected\": false, \"BetweennessCentrality\": 0.03279541, \"gal1RGsig\": 6.9834e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"TORC 2 complex\", \"cytoplasm\", \"mitochondrion\", \"plasma membrane\"], \"alias\": [\"LIT2\", \"S000001367\", \"SLM1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"phosphoinositide binding\"], \"gal4RGsig\": 0.66796, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YIL105C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12531582, \"AverageShortestPathLength\": 7.97983871, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.197, \"gal1RGexp\": 0.196, \"gal80Rsig\": 0.016148, \"Stress\": 7186.0, \"id\": \"1543\", \"gal4RGexp\": 0.023, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"actin cable formation\", \"actin cytoskeleton organization and biogenesis\", \"establishment and/or maintenance of actin cytoskeleton polarity\", \"regulation of cell growth\"], \"Radiality\": 0.74148746, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1543}}, {\"position\": {\"y\": 1635.9668288274665, \"x\": 2050.565406638403}, \"selected\": false, \"data\": {\"id_original\": \"2133\", \"name\": \"YLR134W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"PDC5\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.2585e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"PDC5\", \"S000004124\", \"pyruvate decarboxylase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"pyruvate decarboxylase activity\"], \"gal4RGsig\": 0.58514, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLR134W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12393803, \"AverageShortestPathLength\": 8.06854839, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.351, \"gal1RGexp\": -0.645, \"gal80Rsig\": 1.2076e-08, \"Stress\": 0.0, \"id\": \"1542\", \"gal4RGexp\": 0.027, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glucose catabolic process to ethanol\", \"pyruvate metabolic process\"], \"Radiality\": 0.73820191, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1542}}, {\"position\": {\"y\": 1488.194505219068, \"x\": 1973.5848463356688}, \"selected\": false, \"data\": {\"id_original\": \"2134\", \"name\": \"YER179W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"DMC1\", \"selected\": false, \"BetweennessCentrality\": 0.04783067, \"gal1RGsig\": 0.00011807, \"annotation_GO_CELLULAR_COMPONENT\": [\"condensed nuclear chromosome\", \"nucleus\"], \"alias\": [\"DMC1\", \"ISC2\", \"S000000981\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"double-stranded DNA binding\", \"single-stranded DNA binding\"], \"gal4RGsig\": 0.36733, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YER179W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14139111, \"AverageShortestPathLength\": 7.07258065, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.167, \"gal1RGexp\": -0.219, \"gal80Rsig\": 0.13801, \"Stress\": 9276.0, \"id\": \"1541\", \"gal4RGexp\": 0.059, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"meiosis\", \"meiotic joint molecule formation\", \"meiotic recombination\"], \"Radiality\": 0.77508961, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1541}}, {\"position\": {\"y\": 3804.96319723567, \"x\": 1753.0695570290281}, \"selected\": false, \"data\": {\"id_original\": \"2135\", \"name\": \"YOR310C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"NOP5\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.055533, \"annotation_GO_CELLULAR_COMPONENT\": [\"box C/D snoRNP complex\", \"small nucleolar ribonucleoprotein complex\"], \"alias\": [\"NOP5\", \"NOP58\", \"S000005837\", \"U3 snoRNP protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 1.7322e-06, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YOR310C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.157, \"gal1RGexp\": -0.073, \"gal80Rsig\": 0.041898, \"Stress\": 0.0, \"id\": \"1540\", \"gal4RGexp\": -0.499, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"35S primary transcript processing\", \"processing of 20S pre-rRNA\", \"rRNA modification\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1540}}, {\"position\": {\"y\": 3777.1561293645764, \"x\": 1648.9363172829344}, \"selected\": false, \"data\": {\"id_original\": \"2136\", \"name\": \"YDL014W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"NOP1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.32352, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleolus\", \"small nucleolar ribonucleoprotein complex\"], \"alias\": [\"LOT3\", \"NOP1\", \"S000002172\", \"U3 snoRNP protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"methyltransferase activity\"], \"gal4RGsig\": 0.012945, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YDL014W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.038, \"gal1RGexp\": -0.039, \"gal80Rsig\": 0.62955, \"Stress\": 0.0, \"id\": \"1539\", \"gal4RGexp\": -0.176, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"35S primary transcript processing\", \"RNA methylation\", \"processing of 20S pre-rRNA\", \"rRNA modification\", \"ribosomal large subunit assembly and maintenance\", \"snoRNA 3'-end processing\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 1.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1539}}, {\"position\": {\"y\": 2120.610963348951, \"x\": 1973.6108473122313}, \"selected\": false, \"data\": {\"id_original\": \"2137\", \"name\": \"YPR119W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"CLB2\", \"selected\": false, \"BetweennessCentrality\": 0.29306517, \"gal1RGsig\": 3.9246e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"bud neck\", \"cytoplasm\", \"nucleus\", \"spindle\", \"spindle pole body\"], \"alias\": [\"B-type cyclin\", \"CLB2\", \"S000006323\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"cyclin-dependent protein kinase regulator activity\"], \"gal4RGsig\": 0.00062635, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YPR119W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.15422886, \"AverageShortestPathLength\": 6.48387097, \"NeighborhoodConnectivity\": 12.5, \"gal80Rexp\": -0.342, \"gal1RGexp\": -0.234, \"gal80Rsig\": 1.6424e-05, \"Stress\": 54840.0, \"id\": \"1538\", \"gal4RGexp\": -0.279, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"G2/M transition of mitotic cell cycle\", \"regulation of cyclin-dependent protein kinase activity\"], \"Radiality\": 0.79689367, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1538}}, {\"position\": {\"y\": 2519.099977020826, \"x\": 2021.3377836525633}, \"selected\": false, \"data\": {\"id_original\": \"2138\", \"name\": \"YLR117C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"SYF3\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 7.6184e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"chromatin\"], \"alias\": [\"CLF1\", \"NTC77\", \"S000004107\", \"SYF3\", \"pre-mRNA splicing factor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein binding\"], \"gal4RGsig\": 0.0048407, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YLR117C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12313803, \"AverageShortestPathLength\": 8.12096774, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": 0.712, \"gal1RGexp\": 0.326, \"gal80Rsig\": 6.8931e-06, \"Stress\": 1032.0, \"id\": \"1537\", \"gal4RGexp\": -0.234, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"DNA replication\", \"cell cycle\", \"cis assembly of pre-catalytic spliceosome\", \"nuclear mRNA splicing\", \"via spliceosome\"], \"Radiality\": 0.73626045, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1537}}, {\"position\": {\"y\": 2453.562928680982, \"x\": 1747.8123091042235}, \"selected\": false, \"data\": {\"id_original\": \"2139\", \"name\": \"YGL013C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"PDR1\", \"selected\": false, \"BetweennessCentrality\": 0.02401397, \"gal1RGsig\": 0.0001959, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"AMY1\", \"ANT1\", \"BOR2\", \"CYH3\", \"NRA2\", \"PDR1\", \"S000002981\", \"SMR2\", \"TIL1\", \"TPE1\", \"TPE3\", \"zinc finger transcription factor of the Zn(2)-Cys(6) binuclear cluster domain type\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA binding\", \"transcriptional activator activity\"], \"gal4RGsig\": 0.013422, \"TopologicalCoefficient\": 0.38095238, \"shared_name\": \"YGL013C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12338308, \"AverageShortestPathLength\": 8.10483871, \"NeighborhoodConnectivity\": 3.66666667, \"gal80Rexp\": 0.173, \"gal1RGexp\": 0.185, \"gal80Rsig\": 0.0945, \"Stress\": 4106.0, \"id\": \"1536\", \"gal4RGexp\": -0.152, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of transcription from RNA polymerase II promoter\", \"response to drug\"], \"Radiality\": 0.73685783, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1536}}, {\"position\": {\"y\": 2584.669312958326, \"x\": 1805.0128095925047}, \"selected\": false, \"data\": {\"id_original\": \"2140\", \"name\": \"YCR086W\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"YCR086W\", \"selected\": false, \"BetweennessCentrality\": 0.02399765, \"gal1RGsig\": 0.32121, \"annotation_GO_CELLULAR_COMPONENT\": [\"condensed nuclear chromosome kinetochore\", \"nuclear envelope\", \"nucleolus\"], \"alias\": [\"CSM1\", \"S000000682\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.080473, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YCR086W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12338308, \"AverageShortestPathLength\": 8.10483871, \"NeighborhoodConnectivity\": 4.5, \"gal80Rexp\": 0.381, \"gal1RGexp\": -0.081, \"gal80Rsig\": 0.17239, \"Stress\": 3084.0, \"id\": \"1535\", \"gal4RGexp\": -0.397, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"DNA replication\", \"homologous chromosome segregation\", \"meiotic chromosome segregation\", \"telomere maintenance\"], \"Radiality\": 0.73685783, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1535}}, {\"position\": {\"y\": 2458.7881484075447, \"x\": 1930.4482496559813}, \"selected\": false, \"data\": {\"id_original\": \"2141\", \"name\": \"YDR412W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"YDR412W\", \"selected\": false, \"BetweennessCentrality\": 0.30517827, \"gal1RGsig\": 0.27226, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"S000002820\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 1.6949e-05, \"TopologicalCoefficient\": 0.14285714, \"shared_name\": \"YDR412W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1401922, \"AverageShortestPathLength\": 7.13306452, \"NeighborhoodConnectivity\": 2.85714286, \"gal80Rexp\": 0.991, \"gal1RGexp\": 0.067, \"gal80Rsig\": 2.4711e-05, \"Stress\": 55770.0, \"id\": \"1534\", \"gal4RGexp\": -0.641, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"rRNA processing\"], \"Radiality\": 0.77284946, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 7.0, \"Degree\": 7, \"SUID\": 1534}}, {\"position\": {\"y\": 4207.79356909236, \"x\": 1485.2540319740965}, \"selected\": false, \"data\": {\"id_original\": \"2142\", \"name\": \"YPL201C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"YPL201C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 6.0394e-10, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\", \"nucleus\"], \"alias\": [\"S000006122\", \"YIG1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 8.9209e-07, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPL201C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.597, \"gal1RGexp\": 2.058, \"gal80Rsig\": 0.0043411, \"Stress\": 0.0, \"id\": \"1533\", \"gal4RGexp\": 1.18, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glycerol biosynthetic process\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1533}}, {\"position\": {\"y\": 4315.51573706111, \"x\": 1482.2365797341063}, \"selected\": false, \"data\": {\"id_original\": \"2143\", \"name\": \"YER062C\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"HOR2\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 3.7312e-10, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"GPP2\", \"HOR2\", \"S000000864\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"glycerol-1-phosphatase activity\"], \"gal4RGsig\": 1.2051e-08, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YER062C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.359, \"gal1RGexp\": -0.486, \"gal80Rsig\": 5.8643e-06, \"Stress\": 0.0, \"id\": \"1532\", \"gal4RGexp\": -0.942, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"carbohydrate metabolic process\", \"glycerol biosynthetic process\", \"response to osmotic stress\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1532}}, {\"position\": {\"y\": 2377.0385756536384, \"x\": 2569.568015891333}, \"selected\": false, \"data\": {\"id_original\": \"2144\", \"name\": \"YOR327C\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"SNC2\", \"selected\": false, \"BetweennessCentrality\": 0.00401593, \"gal1RGsig\": 6.9534e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"Golgi trans face\", \"endosome\", \"transport vesicle\"], \"alias\": [\"S000005854\", \"SNC2\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"v-SNARE activity\"], \"gal4RGsig\": 0.06928, \"TopologicalCoefficient\": 0.625, \"shared_name\": \"YOR327C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09397499, \"AverageShortestPathLength\": 10.64112903, \"NeighborhoodConnectivity\": 3.5, \"gal80Rexp\": 0.559, \"gal1RGexp\": 0.249, \"gal80Rsig\": 4.4793e-05, \"Stress\": 602.0, \"id\": \"1531\", \"gal4RGexp\": -0.112, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Golgi to plasma membrane transport\", \"endocytosis\", \"telomere maintenance\", \"vesicle fusion\"], \"Radiality\": 0.64292115, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1531}}, {\"position\": {\"y\": 2418.5818495794197, \"x\": 2674.062919455786}, \"selected\": false, \"data\": {\"id_original\": \"2145\", \"name\": \"YER143W\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"DDI1\", \"selected\": false, \"BetweennessCentrality\": 1.632e-05, \"gal1RGsig\": 5.6125e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"plasma membrane\"], \"alias\": [\"DDI1\", \"S000000945\", \"VSM1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"SNARE binding\"], \"gal4RGsig\": 8.6796e-06, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YER143W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08599168, \"AverageShortestPathLength\": 11.62903226, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.179, \"gal1RGexp\": 0.282, \"gal80Rsig\": 0.018361, \"Stress\": 2.0, \"id\": \"1530\", \"gal4RGexp\": 0.255, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ubiquitin-dependent protein catabolic process\", \"vesicle-mediated transport\"], \"Radiality\": 0.60633214, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1530}}, {\"position\": {\"y\": 2314.785340790357, \"x\": 2625.6516340553953}, \"selected\": false, \"data\": {\"id_original\": \"2146\", \"name\": \"YAL030W\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"SNC1\", \"selected\": false, \"BetweennessCentrality\": 0.00401593, \"gal1RGsig\": 0.18782, \"annotation_GO_CELLULAR_COMPONENT\": [\"Golgi trans face\", \"SNARE complex\", \"endosome\", \"transport vesicle\"], \"alias\": [\"S000000028\", \"SNC1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"v-SNARE activity\"], \"gal4RGsig\": 0.55374, \"TopologicalCoefficient\": 0.625, \"shared_name\": \"YAL030W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09397499, \"AverageShortestPathLength\": 10.64112903, \"NeighborhoodConnectivity\": 3.5, \"gal80Rexp\": 0.003, \"gal1RGexp\": -0.05, \"gal80Rsig\": 0.95396, \"Stress\": 602.0, \"id\": \"1529\", \"gal4RGexp\": 0.027, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"Golgi to plasma membrane transport\", \"endocytosis\", \"vesicle fusion\"], \"Radiality\": 0.64292115, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1529}}, {\"position\": {\"y\": 915.1676650091072, \"x\": 2352.4565809548094}, \"selected\": false, \"data\": {\"id_original\": \"2147\", \"name\": \"YOL086C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"ADH1\", \"selected\": false, \"BetweennessCentrality\": 0.0285648, \"gal1RGsig\": 0.0039297, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\"], \"alias\": [\"ADC1\", \"ADH1\", \"S000005446\", \"alcohol dehydrogenase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"alcohol dehydrogenase activity\"], \"gal4RGsig\": 0.00032186, \"TopologicalCoefficient\": 0.64705882, \"shared_name\": \"YOL086C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14401858, \"AverageShortestPathLength\": 6.94354839, \"NeighborhoodConnectivity\": 12.0, \"gal80Rexp\": -0.322, \"gal1RGexp\": -0.113, \"gal80Rsig\": 1.3104e-05, \"Stress\": 15414.0, \"id\": \"1528\", \"gal4RGexp\": 0.245, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"NADH oxidation\", \"ethanol biosynthetic process during fermentation\", \"fermentation\"], \"Radiality\": 0.77986858, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1528}}, {\"position\": {\"y\": 970.1616835637947, \"x\": 2328.09198744895}, \"selected\": false, \"data\": {\"id_original\": \"2148\", \"name\": \"YDR050C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"TPI1\", \"selected\": false, \"BetweennessCentrality\": 0.0285648, \"gal1RGsig\": 3.6468e-10, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"S000002457\", \"TPI1\", \"triosephosphate isomerase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"triose-phosphate isomerase activity\"], \"gal4RGsig\": 0.48903, \"TopologicalCoefficient\": 0.64705882, \"shared_name\": \"YDR050C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14401858, \"AverageShortestPathLength\": 6.94354839, \"NeighborhoodConnectivity\": 12.0, \"gal80Rexp\": -0.728, \"gal1RGexp\": -0.584, \"gal80Rsig\": 8.6735e-12, \"Stress\": 15414.0, \"id\": \"1527\", \"gal4RGexp\": 0.053, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glycolysis\"], \"Radiality\": 0.77986858, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1527}}, {\"position\": {\"y\": 785.4290175481697, \"x\": 2091.6088712990477}, \"selected\": false, \"data\": {\"id_original\": \"2149\", \"name\": \"YOL127W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"RPL25\", \"selected\": false, \"BetweennessCentrality\": 0.03121327, \"gal1RGsig\": 0.00044813, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPL25\", \"S000005487\", \"ribosomal protein L25 (rpl6L) (YL25)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\", \"structural constituent of ribosome\"], \"gal4RGsig\": 9.2415e-07, \"TopologicalCoefficient\": 0.52272727, \"shared_name\": \"YOL127W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13847013, \"AverageShortestPathLength\": 7.22177419, \"NeighborhoodConnectivity\": 12.5, \"gal80Rexp\": 0.076, \"gal1RGexp\": -0.192, \"gal80Rsig\": 0.17224, \"Stress\": 9108.0, \"id\": \"1526\", \"gal4RGexp\": -0.425, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ribosomal large subunit assembly and maintenance\"], \"Radiality\": 0.76956392, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1526}}, {\"position\": {\"y\": 951.6387343450447, \"x\": 2105.243655997534}, \"selected\": false, \"data\": {\"id_original\": \"2150\", \"name\": \"YIL069C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"RPS24B\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 7.7038e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic small ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPS24B\", \"RPS24EB\", \"S000001331\", \"ribosomal protein S24B\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 1.0186e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YIL069C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13724405, \"AverageShortestPathLength\": 7.28629032, \"NeighborhoodConnectivity\": 17.0, \"gal80Rexp\": -0.018, \"gal1RGexp\": -0.313, \"gal80Rsig\": 0.74944, \"Stress\": 0.0, \"id\": \"1525\", \"gal4RGexp\": -0.467, \"Radiality\": 0.76717443, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1525}}, {\"position\": {\"y\": 887.0916152044197, \"x\": 2122.1291979134276}, \"selected\": false, \"data\": {\"id_original\": \"2151\", \"name\": \"YER074W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"RPS24A\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.8517e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic small ribosomal subunit (sensu the Eukaryota research community)\", \"mitochondrion\"], \"alias\": [\"RPS24A\", \"RPS24EA\", \"S000000876\", \"ribosomal protein S24A\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 2.1168e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YER074W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13724405, \"AverageShortestPathLength\": 7.28629032, \"NeighborhoodConnectivity\": 17.0, \"gal80Rexp\": -0.051, \"gal1RGexp\": -0.262, \"gal80Rsig\": 0.44216, \"Stress\": 0.0, \"id\": \"1524\", \"gal4RGexp\": -0.449, \"Radiality\": 0.76717443, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1524}}, {\"position\": {\"y\": 794.0017104192634, \"x\": 2410.4215009987547}, \"selected\": false, \"data\": {\"id_original\": \"2152\", \"name\": \"YBR093C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"PHO5\", \"selected\": false, \"BetweennessCentrality\": 0.01606373, \"gal1RGsig\": 2.3291e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"cell wall (sensu the Fungi research community)\", \"periplasmic space (sensu the Fungi research community)\"], \"alias\": [\"PHO5\", \"S000000297\", \"acid phosphatase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"acid phosphatase activity\", \"nucleoside-triphosphatase activity\", \"nucleoside-triphosphate diphosphatase activity\"], \"gal4RGsig\": 0.088763, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YBR093C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13754853, \"AverageShortestPathLength\": 7.27016129, \"NeighborhoodConnectivity\": 9.5, \"gal80Rexp\": -0.543, \"gal1RGexp\": -0.704, \"gal80Rsig\": 3.6198e-13, \"Stress\": 2092.0, \"id\": \"1523\", \"gal4RGexp\": 0.075, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cellular response to phosphate starvation\", \"phosphate metabolic process\", \"regulation of cell size\"], \"Radiality\": 0.7677718, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1523}}, {\"position\": {\"y\": 775.8403334661384, \"x\": 2210.77549727561}, \"selected\": false, \"data\": {\"id_original\": \"2153\", \"name\": \"YDR171W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"HSP42\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 8.1757e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"chaperonin-containing T-complex\", \"cytoplasm\", \"cytoskeleton\"], \"alias\": [\"HSP42\", \"S000002578\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"unfolded protein binding\"], \"gal4RGsig\": 8.7039e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YDR171W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13724405, \"AverageShortestPathLength\": 7.28629032, \"NeighborhoodConnectivity\": 17.0, \"gal80Rexp\": -0.723, \"gal1RGexp\": -0.31, \"gal80Rsig\": 1.4287e-12, \"Stress\": 0.0, \"id\": \"1522\", \"gal4RGexp\": 0.3, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"cytoskeleton organization and biogenesis\", \"response to stress\"], \"Radiality\": 0.76717443, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1522}}, {\"position\": {\"y\": 1092.3588881536384, \"x\": 2304.1151197731688}, \"selected\": false, \"data\": {\"id_original\": \"2154\", \"name\": \"YCL030C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"HIS4\", \"selected\": false, \"BetweennessCentrality\": 0.09883491, \"gal1RGsig\": 3.8988e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"intracellular\"], \"alias\": [\"HIS4\", \"S000000535\", \"histidinol dehydrogenase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"histidinol dehydrogenase activity\", \"phosphoribosyl-AMP cyclohydrolase activity\", \"phosphoribosyl-ATP diphosphatase activity\"], \"gal4RGsig\": 2.3894e-07, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YCL030C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14753123, \"AverageShortestPathLength\": 6.77822581, \"NeighborhoodConnectivity\": 9.0, \"gal80Rexp\": 0.29, \"gal1RGexp\": -1.067, \"gal80Rsig\": 0.0016438, \"Stress\": 18164.0, \"id\": \"1521\", \"gal4RGexp\": -0.898, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"histidine biosynthetic process\"], \"Radiality\": 0.78599164, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1521}}, {\"position\": {\"y\": 836.3242201848884, \"x\": 2331.4165571510985}, \"selected\": false, \"data\": {\"id_original\": \"2155\", \"name\": \"YNL301C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"RPL18B\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 7.3086e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RP28B\", \"RPL18B\", \"S000005245\", \"ribosomal protein L18B (rp28B)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 0.00010721, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YNL301C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13724405, \"AverageShortestPathLength\": 7.28629032, \"NeighborhoodConnectivity\": 17.0, \"gal80Rexp\": -0.271, \"gal1RGexp\": -0.379, \"gal80Rsig\": 1.2267e-05, \"Stress\": 0.0, \"id\": \"1520\", \"gal4RGexp\": -0.245, \"Radiality\": 0.76717443, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1520}}, {\"position\": {\"y\": 829.8377089544197, \"x\": 2167.930782157202}, \"selected\": false, \"data\": {\"id_original\": \"2156\", \"name\": \"YOL120C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"RPL18A\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.7242e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RP28A\", \"RPL18A\", \"S000005480\", \"ribosomal protein L18A (rp28A)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 0.00030221, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOL120C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13724405, \"AverageShortestPathLength\": 7.28629032, \"NeighborhoodConnectivity\": 17.0, \"gal80Rexp\": -0.62, \"gal1RGexp\": -0.409, \"gal80Rsig\": 3.898e-09, \"Stress\": 0.0, \"id\": \"1519\", \"gal4RGexp\": -0.225, \"Radiality\": 0.76717443, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1519}}, {\"position\": {\"y\": 1295.2564253850837, \"x\": 2181.5840833962156}, \"selected\": false, \"data\": {\"id_original\": \"2157\", \"name\": \"YLR044C\", \"Eccentricity\": 15, \"IsSingleNode\": false, \"COMMON\": \"PDC1\", \"selected\": false, \"BetweennessCentrality\": 0.2780402, \"gal1RGsig\": 6.9512e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"PDC1\", \"S000004034\", \"pyruvate decarboxylase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"pyruvate decarboxylase activity\"], \"gal4RGsig\": 0.23784, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YLR044C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.16198563, \"AverageShortestPathLength\": 6.1733871, \"NeighborhoodConnectivity\": 10.33333333, \"gal80Rexp\": -0.184, \"gal1RGexp\": -0.62, \"gal80Rsig\": 0.0064407, \"Stress\": 82528.0, \"id\": \"1518\", \"gal4RGexp\": -0.071, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glucose catabolic process to ethanol\", \"pyruvate metabolic process\"], \"Radiality\": 0.80839307, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1518}}, {\"position\": {\"y\": 742.9172987981697, \"x\": 2125.032336074133}, \"selected\": false, \"data\": {\"id_original\": \"2158\", \"name\": \"YIL133C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"RPL16A\", \"selected\": false, \"BetweennessCentrality\": 0.03121327, \"gal1RGsig\": 4.6153e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPL13\", \"RPL16A\", \"S000001395\", \"ribosomal protein L16A (L21A) (rp22) (YL15)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\", \"structural constituent of ribosome\"], \"gal4RGsig\": 0.00011962, \"TopologicalCoefficient\": 0.52272727, \"shared_name\": \"YIL133C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.13847013, \"AverageShortestPathLength\": 7.22177419, \"NeighborhoodConnectivity\": 12.5, \"gal80Rexp\": -0.146, \"gal1RGexp\": -0.453, \"gal80Rsig\": 0.012589, \"Stress\": 9108.0, \"id\": \"1517\", \"gal4RGexp\": -0.311, \"Radiality\": 0.76956392, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1517}}, {\"position\": {\"y\": 998.2576308294197, \"x\": 2254.950530844946}, \"selected\": false, \"data\": {\"id_original\": \"2159\", \"name\": \"YHR174W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"ENO2\", \"selected\": false, \"BetweennessCentrality\": 0.0285648, \"gal1RGsig\": 7.175e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"phosphopyruvate hydratase complex\", \"soluble fraction\"], \"alias\": [\"ENO2\", \"S000001217\", \"enolase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"phosphopyruvate hydratase activity\"], \"gal4RGsig\": 0.011477, \"TopologicalCoefficient\": 0.64705882, \"shared_name\": \"YHR174W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14401858, \"AverageShortestPathLength\": 6.94354839, \"NeighborhoodConnectivity\": 12.0, \"gal80Rexp\": -0.217, \"gal1RGexp\": -0.816, \"gal80Rsig\": 0.0015357, \"Stress\": 15414.0, \"id\": \"1516\", \"gal4RGexp\": 0.241, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"gluconeogenesis\", \"glycolysis\"], \"Radiality\": 0.77986858, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1516}}, {\"position\": {\"y\": 787.4050917669197, \"x\": 2273.682777243872}, \"selected\": false, \"data\": {\"id_original\": \"2160\", \"name\": \"YGR254W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"ENO1\", \"selected\": false, \"BetweennessCentrality\": 0.0285648, \"gal1RGsig\": 6.5621e-11, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"phosphopyruvate hydratase complex\"], \"alias\": [\"ENO1\", \"HSP48\", \"S000003486\", \"enolase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"phosphopyruvate hydratase activity\"], \"gal4RGsig\": 0.0079977, \"TopologicalCoefficient\": 0.64705882, \"shared_name\": \"YGR254W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14401858, \"AverageShortestPathLength\": 6.94354839, \"NeighborhoodConnectivity\": 12.0, \"gal80Rexp\": -0.171, \"gal1RGexp\": -0.737, \"gal80Rsig\": 0.025056, \"Stress\": 15414.0, \"id\": \"1515\", \"gal4RGexp\": 0.259, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"gluconeogenesis\", \"glycolysis\"], \"Radiality\": 0.77986858, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1515}}, {\"position\": {\"y\": 746.0173354192634, \"x\": 2305.530204612036}, \"selected\": false, \"data\": {\"id_original\": \"2161\", \"name\": \"YCR012W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"PGK1\", \"selected\": false, \"BetweennessCentrality\": 0.04462853, \"gal1RGsig\": 7.3129e-10, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"mitochondrion\"], \"alias\": [\"3-phosphoglycerate kinase\", \"PGK1\", \"S000000605\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"phosphoglycerate kinase activity\"], \"gal4RGsig\": 2.4163e-05, \"TopologicalCoefficient\": 0.42592593, \"shared_name\": \"YCR012W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1443539, \"AverageShortestPathLength\": 6.92741935, \"NeighborhoodConnectivity\": 8.66666667, \"gal80Rexp\": -0.746, \"gal1RGexp\": -0.668, \"gal80Rsig\": 4.2315e-13, \"Stress\": 16638.0, \"id\": \"1514\", \"gal4RGexp\": 0.329, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"gluconeogenesis\", \"glycolysis\"], \"Radiality\": 0.78046595, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1514}}, {\"position\": {\"y\": 914.4169325872322, \"x\": 2238.283912497778}, \"selected\": false, \"data\": {\"id_original\": \"2162\", \"name\": \"YNL216W\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"RAP1\", \"selected\": false, \"BetweennessCentrality\": 0.35844736, \"gal1RGsig\": 0.024692, \"annotation_GO_CELLULAR_COMPONENT\": [\"nuclear telomere cap complex\", \"nuclear telomeric heterochromatin\", \"nucleus\"], \"alias\": [\"GRF1\", \"RAP1\", \"S000005160\", \"TBA1\", \"TUF1\", \"repressor activator protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"DNA binding\", \"transcription factor activity\"], \"gal4RGsig\": 0.93493, \"TopologicalCoefficient\": 0.09803922, \"shared_name\": \"YNL216W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.15897436, \"AverageShortestPathLength\": 6.29032258, \"NeighborhoodConnectivity\": 1.88235294, \"gal80Rexp\": 0.234, \"gal1RGexp\": 0.205, \"gal80Rsig\": 0.54155, \"Stress\": 111754.0, \"id\": \"1513\", \"gal4RGexp\": 0.015, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"chromatin silencing\", \"chromatin silencing at telomere\", \"protection from non-homologous end joining at telomere\", \"telomere maintenance via telomerase\", \"transcription from RNA polymerase III promoter\"], \"Radiality\": 0.80406213, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 17.0, \"Degree\": 17, \"SUID\": 1513}}, {\"position\": {\"y\": 863.8891005559822, \"x\": 2394.1833723366453}, \"selected\": false, \"data\": {\"id_original\": \"2163\", \"name\": \"YAL038W\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"CDC19\", \"selected\": false, \"BetweennessCentrality\": 0.04462853, \"gal1RGsig\": 1.3173e-10, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosol\"], \"alias\": [\"CDC19\", \"PYK1\", \"S000000036\", \"pyruvate kinase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"pyruvate kinase activity\"], \"gal4RGsig\": 0.10377, \"TopologicalCoefficient\": 0.42592593, \"shared_name\": \"YAL038W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.1443539, \"AverageShortestPathLength\": 6.92741935, \"NeighborhoodConnectivity\": 8.66666667, \"gal80Rexp\": -0.453, \"gal1RGexp\": -0.652, \"gal80Rsig\": 1.5489e-07, \"Stress\": 16638.0, \"id\": \"1512\", \"gal4RGexp\": 0.123, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"glycolysis\", \"pyruvate metabolic process\"], \"Radiality\": 0.78046595, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1512}}, {\"position\": {\"y\": 798.9335951848884, \"x\": 2544.6567610085203}, \"selected\": false, \"data\": {\"id_original\": \"2164\", \"name\": \"YNL307C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"MCK1\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.27844, \"annotation_GO_CELLULAR_COMPONENT\": [\"soluble fraction\"], \"alias\": [\"43.1 kDa serine/threonine/tyrosine protein kinase\", \"MCK1\", \"S000005251\", \"YPK1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"glycogen synthase kinase 3 activity\", \"protein threonine/tyrosine kinase activity\"], \"gal4RGsig\": 0.029538, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YNL307C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12633724, \"AverageShortestPathLength\": 7.91532258, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.063, \"gal1RGexp\": -0.046, \"gal80Rsig\": 0.37572, \"Stress\": 614.0, \"id\": \"1511\", \"gal4RGexp\": 0.094, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"double-strand break repair via nonhomologous end joining\", \"meiosis\", \"mitotic sister chromatid segregation\", \"protein amino acid phosphorylation\", \"response to stress\", \"sporulation (sensu the Fungi research community)\"], \"Radiality\": 0.74387694, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1511}}, {\"position\": {\"y\": 1109.5300002141853, \"x\": 1665.9339826882078}, \"selected\": false, \"data\": {\"id_original\": \"2165\", \"name\": \"YDL013W\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"HEX3\", \"selected\": false, \"BetweennessCentrality\": 0.13296195, \"gal1RGsig\": 4.0439e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cellular_component\"], \"alias\": [\"HEX3\", \"S000002171\", \"SLX5\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.22129, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YDL013W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10777923, \"AverageShortestPathLength\": 9.27822581, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.593, \"gal1RGexp\": 0.214, \"gal80Rsig\": 2.0836e-05, \"Stress\": 60158.0, \"id\": \"1510\", \"gal4RGexp\": 0.073, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein sumoylation\", \"response to DNA damage stimulus\", \"telomere maintenance\"], \"Radiality\": 0.69339904, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1510}}, {\"position\": {\"y\": 1003.4048476262947, \"x\": 1603.0958174050047}, \"selected\": false, \"data\": {\"id_original\": \"2166\", \"name\": \"YER116C\", \"Eccentricity\": 22, \"IsSingleNode\": false, \"COMMON\": \"YER116C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.56099, \"annotation_GO_CELLULAR_COMPONENT\": [\"cellular_component\"], \"alias\": [\"S000000918\", \"SLX8\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.18379, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YER116C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09733124, \"AverageShortestPathLength\": 10.27419355, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.182, \"gal1RGexp\": 0.029, \"gal80Rsig\": 0.13915, \"Stress\": 0.0, \"id\": \"1509\", \"gal4RGexp\": -0.11, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"protein sumoylation\", \"response to DNA damage stimulus\", \"telomere maintenance\"], \"Radiality\": 0.65651135, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1509}}, {\"position\": {\"y\": 1573.035138611402, \"x\": 1460.7314375221922}, \"selected\": false, \"data\": {\"id_original\": \"2167\", \"name\": \"YNR053C\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"YNR053C\", \"selected\": false, \"BetweennessCentrality\": 0.11341401, \"gal1RGsig\": 2.1301e-07, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleolus\", \"nucleoplasm\", \"nucleus\"], \"alias\": [\"NOG2\", \"S000005336\", \"part of a pre-60S complex\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"GTPase activity\"], \"gal4RGsig\": 0.0048783, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YNR053C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.09440426, \"AverageShortestPathLength\": 10.59274194, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": -0.012, \"gal1RGexp\": 0.352, \"gal80Rsig\": 0.90581, \"Stress\": 50456.0, \"id\": \"1508\", \"gal4RGexp\": -0.238, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"ribosomal large subunit export from nucleus\", \"ribosome assembly\"], \"Radiality\": 0.64471326, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1508}}, {\"position\": {\"y\": 1936.5454115911384, \"x\": 1497.4738691628172}, \"selected\": false, \"data\": {\"id_original\": \"2168\", \"name\": \"YLR264W\", \"Eccentricity\": 25, \"IsSingleNode\": false, \"COMMON\": \"RPS28B\", \"selected\": false, \"BetweennessCentrality\": 0.01199882, \"gal1RGsig\": 0.00016086, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic small ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPS28B\", \"RPS33B\", \"S000004254\", \"ribosomal protein S28B (S33B) (YS27)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 0.00020632, \"TopologicalCoefficient\": 0.375, \"shared_name\": \"YLR264W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08261159, \"AverageShortestPathLength\": 12.10483871, \"NeighborhoodConnectivity\": 3.25, \"gal80Rexp\": -0.17, \"gal1RGexp\": -0.259, \"gal80Rsig\": 0.21059, \"Stress\": 8136.0, \"id\": \"1507\", \"gal4RGexp\": -0.299, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"telomere maintenance\"], \"Radiality\": 0.58870968, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1507}}, {\"position\": {\"y\": 2005.609742645826, \"x\": 1658.1348799050047}, \"selected\": false, \"data\": {\"id_original\": \"2169\", \"name\": \"YEL015W\", \"Eccentricity\": 23, \"IsSingleNode\": false, \"COMMON\": \"YEL015W\", \"selected\": false, \"BetweennessCentrality\": 0.04554212, \"gal1RGsig\": 3.0657e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasmic mRNA processing body\"], \"alias\": [\"DCP3\", \"EDC3\", \"LSM16\", \"S000000741\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.0052925, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YEL015W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08936937, \"AverageShortestPathLength\": 11.18951613, \"NeighborhoodConnectivity\": 5.5, \"gal80Rexp\": 0.275, \"gal1RGexp\": 0.222, \"gal80Rsig\": 0.032829, \"Stress\": 9804.0, \"id\": \"1506\", \"gal4RGexp\": -0.171, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"deadenylation-independent decapping\"], \"Radiality\": 0.62261051, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1506}}, {\"position\": {\"y\": 1816.183991913404, \"x\": 1356.850272971411}, \"selected\": false, \"data\": {\"id_original\": \"2170\", \"name\": \"YNL050C\", \"Eccentricity\": 25, \"IsSingleNode\": false, \"COMMON\": \"YNL050C\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.425e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cellular_component\"], \"alias\": [\"S000004995\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.0031926, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YNL050C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08244681, \"AverageShortestPathLength\": 12.12903226, \"NeighborhoodConnectivity\": 7.0, \"gal80Rexp\": 0.594, \"gal1RGexp\": 0.301, \"gal80Rsig\": 0.00075124, \"Stress\": 0.0, \"id\": \"1505\", \"gal4RGexp\": -0.448, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.58781362, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1505}}, {\"position\": {\"y\": 1747.937623505201, \"x\": 1304.7407148659422}, \"selected\": false, \"data\": {\"id_original\": \"2171\", \"name\": \"YNR050C\", \"Eccentricity\": 25, \"IsSingleNode\": false, \"COMMON\": \"LYS9\", \"selected\": false, \"BetweennessCentrality\": 0.02399765, \"gal1RGsig\": 1.1825e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"LYS13\", \"LYS9\", \"S000005333\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"saccharopine dehydrogenase (NADP+\", \"L-glutamate-forming) activity\"], \"gal4RGsig\": 0.0089039, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YNR050C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08261159, \"AverageShortestPathLength\": 12.10483871, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": 0.647, \"gal1RGexp\": 0.223, \"gal80Rsig\": 1.1773e-07, \"Stress\": 8154.0, \"id\": \"1504\", \"gal4RGexp\": -0.131, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"lysine biosynthetic process via aminoadipic acid\"], \"Radiality\": 0.58870968, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1504}}, {\"position\": {\"y\": 1838.873902802076, \"x\": 1465.192558127661}, \"selected\": false, \"data\": {\"id_original\": \"2172\", \"name\": \"YJR022W\", \"Eccentricity\": 24, \"IsSingleNode\": false, \"COMMON\": \"LSM8\", \"selected\": false, \"BetweennessCentrality\": 0.12017603, \"gal1RGsig\": 0.6011, \"annotation_GO_CELLULAR_COMPONENT\": [\"U4/U6 x U5 tri-snRNP complex\", \"nucleus\", \"snRNP U6\"], \"alias\": [\"LSM8\", \"S000003783\", \"snRNP protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\", \"RNA splicing factor activity\", \"transesterification mechanism\"], \"gal4RGsig\": 0.070701, \"TopologicalCoefficient\": 0.19642857, \"shared_name\": \"YJR022W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08982253, \"AverageShortestPathLength\": 11.13306452, \"NeighborhoodConnectivity\": 2.57142857, \"gal80Rexp\": 0.294, \"gal1RGexp\": 0.059, \"gal80Rsig\": 0.14344, \"Stress\": 47386.0, \"id\": \"1503\", \"gal4RGexp\": -0.435, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nuclear mRNA splicing\", \"via spliceosome\"], \"Radiality\": 0.62470131, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 7.0, \"Degree\": 7, \"SUID\": 1503}}, {\"position\": {\"y\": 1978.196687225904, \"x\": 1505.591728049536}, \"selected\": false, \"data\": {\"id_original\": \"2173\", \"name\": \"YOR167C\", \"Eccentricity\": 25, \"IsSingleNode\": false, \"COMMON\": \"RPS28A\", \"selected\": false, \"BetweennessCentrality\": 0.01199882, \"gal1RGsig\": 2.7303e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic small ribosomal subunit (sensu the Eukaryota research community)\"], \"alias\": [\"RPS28A\", \"RPS33A\", \"S000005693\", \"ribosomal protein S28A (S33A) (YS27)\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"structural constituent of ribosome\"], \"gal4RGsig\": 0.00010533, \"TopologicalCoefficient\": 0.375, \"shared_name\": \"YOR167C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.08261159, \"AverageShortestPathLength\": 12.10483871, \"NeighborhoodConnectivity\": 3.25, \"gal80Rexp\": -0.243, \"gal1RGexp\": -0.374, \"gal80Rsig\": 4.8633e-05, \"Stress\": 8136.0, \"id\": \"1502\", \"gal4RGexp\": -0.239, \"Radiality\": 0.58870968, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 4.0, \"Degree\": 4, \"SUID\": 1502}}, {\"position\": {\"y\": 1903.2498488469978, \"x\": 1595.0740278542235}, \"selected\": false, \"data\": {\"id_original\": \"2174\", \"name\": \"YER112W\", \"Eccentricity\": 26, \"IsSingleNode\": false, \"COMMON\": \"LSM4\", \"selected\": false, \"BetweennessCentrality\": 8.16e-06, \"gal1RGsig\": 7.0527e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"U4/U6 x U5 tri-snRNP complex\", \"nucleolus\", \"small nucleolar ribonucleoprotein complex\", \"snRNP U6\"], \"alias\": [\"LSM4\", \"S000000914\", \"SDB23\", \"USS1\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\", \"RNA splicing factor activity\", \"transesterification mechanism\"], \"gal4RGsig\": 0.0037591, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YER112W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.0763782, \"AverageShortestPathLength\": 13.09274194, \"NeighborhoodConnectivity\": 4.0, \"gal80Rexp\": 0.151, \"gal1RGexp\": 0.193, \"gal80Rsig\": 0.13653, \"Stress\": 2.0, \"id\": \"1501\", \"gal4RGexp\": -0.181, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mRNA catabolic process\", \"nuclear mRNA splicing\", \"via spliceosome\"], \"Radiality\": 0.55212067, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1501}}, {\"position\": {\"y\": 1715.766770844068, \"x\": 1858.2866438210203}, \"selected\": false, \"data\": {\"id_original\": \"2175\", \"name\": \"YCL067C\", \"Eccentricity\": 16, \"IsSingleNode\": false, \"COMMON\": \"ALPHA2\", \"selected\": false, \"BetweennessCentrality\": 0.01614536, \"gal1RGsig\": 0.0012873, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"ALPHA2\", \"HMLALPHA2\", \"S000000572\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"transcription corepressor activity\"], \"gal4RGsig\": 0.11481, \"TopologicalCoefficient\": 0.26315789, \"shared_name\": \"YCL067C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.14691943, \"AverageShortestPathLength\": 6.80645161, \"NeighborhoodConnectivity\": 5.16666667, \"gal80Rexp\": 0.301, \"gal1RGexp\": 0.169, \"gal80Rsig\": 0.0027555, \"Stress\": 2084.0, \"id\": \"1500\", \"gal4RGexp\": -0.085, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"donor selection\", \"regulation of transcription from RNA polymerase II promoter\", \"regulation of transcription\", \"mating-type specific\"], \"Radiality\": 0.78494624, \"ClusteringCoefficient\": 0.26666667, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 6.0, \"Degree\": 6, \"SUID\": 1500}}, {\"position\": {\"y\": 1528.7146067663093, \"x\": 1618.5860517800047}, \"selected\": false, \"data\": {\"id_original\": \"2176\", \"name\": \"YBR112C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"SSN6\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.009167, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"CRT8\", \"CYC8\", \"S000000316\", \"SSN6\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"general transcriptional repressor activity\", \"transcription coactivator activity\"], \"gal4RGsig\": 0.92034, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YBR112C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11376147, \"AverageShortestPathLength\": 8.79032258, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.077, \"gal1RGexp\": 0.108, \"gal80Rsig\": 0.17771, \"Stress\": 0.0, \"id\": \"1499\", \"gal4RGexp\": -0.004, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"chromatin remodeling\", \"negative regulation of transcription\", \"nucleosome spacing\", \"telomere maintenance\"], \"Radiality\": 0.71146953, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1499}}, {\"position\": {\"y\": 1595.5289397283454, \"x\": 1727.1213301003172}, \"selected\": false, \"data\": {\"id_original\": \"2177\", \"name\": \"YCR084C\", \"Eccentricity\": 17, \"IsSingleNode\": false, \"COMMON\": \"TUP1\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 0.28551, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"AAR1\", \"AER2\", \"AMM1\", \"CRT4\", \"CYC9\", \"FLK1\", \"ROX4\", \"S000000680\", \"SFL2\", \"TUP1\", \"UMR7\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"general transcriptional repressor activity\"], \"gal4RGsig\": 0.0001832, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YCR084C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.12829798, \"AverageShortestPathLength\": 7.79435484, \"NeighborhoodConnectivity\": 3.5, \"gal80Rexp\": -0.091, \"gal1RGexp\": 0.044, \"gal80Rsig\": 0.69411, \"Stress\": 1038.0, \"id\": \"1498\", \"gal4RGexp\": 0.704, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"negative regulation of transcription\", \"nucleosome spacing\"], \"Radiality\": 0.74835723, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1498}}, {\"position\": {\"y\": 936.7500624700447, \"x\": 1824.4568403542235}, \"selected\": false, \"data\": {\"id_original\": \"2178\", \"name\": \"YIL061C\", \"Eccentricity\": 20, \"IsSingleNode\": false, \"COMMON\": \"SNP1\", \"selected\": false, \"BetweennessCentrality\": 0.15820029, \"gal1RGsig\": 0.013072, \"annotation_GO_CELLULAR_COMPONENT\": [\"commitment complex\", \"snRNP U1\"], \"alias\": [\"U1-70K\", \"U1snRNP 70K protein homolog\", \"S000001323\", \"SNP1\", \"U1 70K\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"U1 snRNA binding\", \"mRNA binding\"], \"gal4RGsig\": 0.00049035, \"TopologicalCoefficient\": 0.2, \"shared_name\": \"YIL061C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.11665099, \"AverageShortestPathLength\": 8.57258065, \"NeighborhoodConnectivity\": 1.8, \"gal80Rexp\": 0.181, \"gal1RGexp\": 0.165, \"gal80Rsig\": 0.28119, \"Stress\": 70312.0, \"id\": \"1497\", \"gal4RGexp\": -0.635, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nuclear mRNA splicing\", \"via spliceosome\"], \"Radiality\": 0.71953405, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 5.0, \"Degree\": 5, \"SUID\": 1497}}, {\"position\": {\"y\": 830.3722548528572, \"x\": 1773.632041770239}, \"selected\": false, \"data\": {\"id_original\": \"2179\", \"name\": \"YGR203W\", \"Eccentricity\": 21, \"IsSingleNode\": false, \"COMMON\": \"YGR203W\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.039575, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"S000003435\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"phosphoric monoester hydrolase activity\", \"protein tyrosine phosphatase activity\"], \"gal4RGsig\": 0.43554, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGR203W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10450906, \"AverageShortestPathLength\": 9.56854839, \"NeighborhoodConnectivity\": 5.0, \"gal80Rexp\": -0.34, \"gal1RGexp\": -0.141, \"gal80Rsig\": 0.22451, \"Stress\": 0.0, \"id\": \"1496\", \"gal4RGexp\": -0.085, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"biological_process\"], \"Radiality\": 0.68264636, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1496}}, {\"position\": {\"y\": 3598.6790480657482, \"x\": 2806.1638754189207}, \"selected\": false, \"data\": {\"id_original\": \"2180\", \"name\": \"YJL013C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"MAD3\", \"selected\": false, \"BetweennessCentrality\": 0.16666667, \"gal1RGsig\": 0.14469, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"MAD3\", \"S000003550\", \"spindle checkpoint complex subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.046575, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YJL013C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.084, \"gal1RGexp\": -0.072, \"gal80Rsig\": 0.52778, \"Stress\": 4.0, \"id\": \"1495\", \"gal4RGexp\": -0.154, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mitotic spindle checkpoint\"], \"Radiality\": 0.83333333, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1495}}, {\"position\": {\"y\": 3677.1561293645764, \"x\": 2729.1520651161863}, \"selected\": false, \"data\": {\"id_original\": \"2181\", \"name\": \"YGL229C\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"SAP4\", \"selected\": false, \"BetweennessCentrality\": 0.08333333, \"gal1RGsig\": 2.3655e-09, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"mitochondrion\"], \"alias\": [\"S000003198\", \"SAP4\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein serine/threonine phosphatase activity\"], \"gal4RGsig\": 0.020857, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YGL229C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.57142857, \"AverageShortestPathLength\": 1.75, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.208, \"gal1RGexp\": -0.521, \"gal80Rsig\": 0.28721, \"Stress\": 2.0, \"id\": \"1494\", \"gal4RGexp\": 0.171, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"G1/S transition of mitotic cell cycle\"], \"Radiality\": 0.75, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1494}}, {\"position\": {\"y\": 3590.1272902532482, \"x\": 2662.012874442358}, \"selected\": false, \"data\": {\"id_original\": \"2182\", \"name\": \"YJL030W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"MAD2\", \"selected\": false, \"BetweennessCentrality\": 0.16666667, \"gal1RGsig\": 0.21701, \"annotation_GO_CELLULAR_COMPONENT\": [\"condensed nuclear chromosome kinetochore\", \"nuclear pore\"], \"alias\": [\"MAD2\", \"S000003567\", \"spindle checkpoint complex subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 0.0092841, \"TopologicalCoefficient\": 0.75, \"shared_name\": \"YJL030W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.66666667, \"AverageShortestPathLength\": 1.5, \"NeighborhoodConnectivity\": 2.5, \"gal80Rexp\": 0.46, \"gal1RGexp\": 0.08, \"gal80Rsig\": 0.0045437, \"Stress\": 4.0, \"id\": \"1493\", \"gal4RGexp\": -0.271, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"chromosome decondensation\", \"mitotic spindle checkpoint\"], \"Radiality\": 0.83333333, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1493}}, {\"position\": {\"y\": 3508.49493551692, \"x\": 2739.1552999794676}, \"selected\": false, \"data\": {\"id_original\": \"2183\", \"name\": \"YGR014W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"MSB2\", \"selected\": false, \"BetweennessCentrality\": 0.58333333, \"gal1RGsig\": 0.093717, \"annotation_GO_CELLULAR_COMPONENT\": [\"integral to plasma membrane\", \"site of polarized growth\"], \"alias\": [\"MSB2\", \"S000003246\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"osmosensor activity\"], \"gal4RGsig\": 0.01294, \"TopologicalCoefficient\": 0.66666667, \"shared_name\": \"YGR014W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.8, \"AverageShortestPathLength\": 1.25, \"NeighborhoodConnectivity\": 1.66666667, \"gal80Rexp\": -0.171, \"gal1RGexp\": -0.066, \"gal80Rsig\": 0.031836, \"Stress\": 10.0, \"id\": \"1492\", \"gal4RGexp\": -0.131, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"establishment of cell polarity (sensu the Fungi research community)\", \"response to osmotic stress\", \"signal transduction during filamentous growth\"], \"Radiality\": 0.91666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1492}}, {\"position\": {\"y\": 3396.675294403639, \"x\": 2748.6170004189207}, \"selected\": false, \"data\": {\"id_original\": \"2184\", \"name\": \"YPL211W\", \"Eccentricity\": 3, \"IsSingleNode\": false, \"COMMON\": \"NIP7\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0019352, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytosolic large ribosomal subunit (sensu the Eukaryota research community)\", \"nucleolus\"], \"alias\": [\"NIP7\", \"S000006132\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"molecular_function\"], \"gal4RGsig\": 5.2826e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YPL211W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.5, \"AverageShortestPathLength\": 2.0, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.623, \"gal1RGexp\": -0.231, \"gal80Rsig\": 0.00015609, \"Stress\": 0.0, \"id\": \"1491\", \"gal4RGexp\": -0.504, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"rRNA processing\", \"ribosomal large subunit assembly and maintenance\", \"ribosomal large subunit biogenesis and assembly\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1491}}, {\"position\": {\"y\": 2542.3266615911384, \"x\": 3550.775077658911}, \"selected\": false, \"data\": {\"id_original\": \"2185\", \"name\": \"YGL044C\", \"Eccentricity\": 27, \"IsSingleNode\": false, \"COMMON\": \"RNA15\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.0013639, \"annotation_GO_CELLULAR_COMPONENT\": [\"mRNA cleavage factor complex\"], \"alias\": [\"RNA15\", \"S000003012\", \"cleavage and polyadenylation factor CF I component involved in pre-mRNA 3'-end processing\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\", \"protein heterodimerization activity\"], \"gal4RGsig\": 0.0040902, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGL044C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.06112891, \"AverageShortestPathLength\": 16.35887097, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.281, \"gal1RGexp\": 0.162, \"gal80Rsig\": 0.0057448, \"Stress\": 0.0, \"id\": \"1490\", \"gal4RGexp\": -0.211, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mRNA cleavage\", \"mRNA polyadenylation\"], \"Radiality\": 0.43115293, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1490}}, {\"position\": {\"y\": 2602.4323744817634, \"x\": 3445.7603071510985}, \"selected\": false, \"data\": {\"id_original\": \"2186\", \"name\": \"YOL123W\", \"Eccentricity\": 26, \"IsSingleNode\": false, \"COMMON\": \"HRP1\", \"selected\": false, \"BetweennessCentrality\": 0.00806452, \"gal1RGsig\": 9.8446e-06, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"mRNA cleavage factor complex\", \"nucleus\"], \"alias\": [\"HRP1\", \"NAB4\", \"NAB5\", \"S000005483\", \"cleavage and polyadenylation factor CF I component involved in pre-mRNA 3'-end processing\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA binding\"], \"gal4RGsig\": 0.0050114, \"TopologicalCoefficient\": 0.5, \"shared_name\": \"YOL123W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.06509186, \"AverageShortestPathLength\": 15.36290323, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.036, \"gal1RGexp\": 0.245, \"gal80Rsig\": 0.59496, \"Stress\": 1970.0, \"id\": \"1489\", \"gal4RGexp\": 0.126, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mRNA cleavage\", \"mRNA polyadenylation\"], \"Radiality\": 0.46804062, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1489}}, {\"position\": {\"y\": 3876.8354201360607, \"x\": 1533.0843580544188}, \"selected\": false, \"data\": {\"id_original\": \"2187\", \"name\": \"YAL003W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"EFB1\", \"selected\": false, \"BetweennessCentrality\": 0.16666667, \"gal1RGsig\": 0.0018696, \"annotation_GO_CELLULAR_COMPONENT\": [\"eukaryotic translation elongation factor 1 complex\", \"ribosome\"], \"alias\": [\"translation elongation factor EF-1beta\", \"EF-1 beta\", \"EFB1\", \"S000000003\", \"TEF5\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"translation elongation factor activity\"], \"gal4RGsig\": 0.00062814, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YAL003W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.75, \"AverageShortestPathLength\": 1.33333333, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.146, \"gal1RGexp\": -0.157, \"gal80Rsig\": 0.013062, \"Stress\": 2.0, \"id\": \"1488\", \"gal4RGexp\": -0.2, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"translational elongation\"], \"Radiality\": 0.83333333, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1488}}, {\"position\": {\"y\": 1920.2575087591072, \"x\": 1075.518730002661}, \"selected\": false, \"data\": {\"id_original\": \"2188\", \"name\": \"YFL017C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"GNA1\", \"selected\": false, \"BetweennessCentrality\": 0.0426128, \"gal1RGsig\": 0.0017854, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"GNA1\", \"PAT1\", \"S000001877\", \"glucosamine-phosphate N-acetyltransferase\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"glucosamine 6-phosphate N-acetyltransferase activity\"], \"gal4RGsig\": 0.0055302, \"TopologicalCoefficient\": 0.2, \"shared_name\": \"YFL017C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10575693, \"AverageShortestPathLength\": 9.45564516, \"NeighborhoodConnectivity\": 1.6, \"gal80Rexp\": 0.124, \"gal1RGexp\": 0.131, \"gal80Rsig\": 0.050323, \"Stress\": 6282.0, \"id\": \"1487\", \"gal4RGexp\": 0.122, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"UDP-N-acetylglucosamine biosynthetic process\"], \"Radiality\": 0.68682796, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 5.0, \"Degree\": 5, \"SUID\": 1487}}, {\"position\": {\"y\": 2035.9573378606697, \"x\": 1122.041923362036}, \"selected\": false, \"data\": {\"id_original\": \"2189\", \"name\": \"YDR429C\", \"Eccentricity\": 18, \"IsSingleNode\": false, \"COMMON\": \"TIF35\", \"selected\": false, \"BetweennessCentrality\": 0.02250902, \"gal1RGsig\": 0.072655, \"annotation_GO_CELLULAR_COMPONENT\": [\"eukaryotic translation initiation factor 3 complex\"], \"alias\": [\"S000002837\", \"TIF35\", \"translation initiation factor eIF3 subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"translation initiation factor activity\"], \"gal4RGsig\": 0.0011724, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YDR429C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.10188989, \"AverageShortestPathLength\": 9.81451613, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.354, \"gal1RGexp\": 0.078, \"gal80Rsig\": 1.6643e-05, \"Stress\": 4106.0, \"id\": \"1486\", \"gal4RGexp\": -0.209, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"translational initiation\"], \"Radiality\": 0.67353644, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1486}}, {\"position\": {\"y\": 1943.3013014837165, \"x\": 1134.3325727760985}, \"selected\": false, \"data\": {\"id_original\": \"2190\", \"name\": \"YMR146C\", \"Eccentricity\": 19, \"IsSingleNode\": false, \"COMMON\": \"TIF34\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.30844, \"annotation_GO_CELLULAR_COMPONENT\": [\"eukaryotic translation initiation factor 3 complex\", \"multi-eIF complex\"], \"alias\": [\"S000004754\", \"TIF34\", \"translation initiation factor eIF3 subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"translation initiation factor activity\"], \"gal4RGsig\": 0.0085173, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YMR146C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.0925028, \"AverageShortestPathLength\": 10.81048387, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.151, \"gal1RGexp\": -0.05, \"gal80Rsig\": 0.072007, \"Stress\": 0.0, \"id\": \"1485\", \"gal4RGexp\": -0.143, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"translational initiation\"], \"Radiality\": 0.63664875, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1485}}, {\"position\": {\"y\": 3777.1561293645764, \"x\": 1175.0725782692625}, \"selected\": false, \"data\": {\"id_original\": \"2191\", \"name\": \"YLR293C\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"GSP1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 1.7601e-05, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"CNR1\", \"CST17\", \"GSP1\", \"GTP-binding protein\", \"S000004284\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"GTPase activity\"], \"gal4RGsig\": 0.00067968, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YLR293C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.6, \"AverageShortestPathLength\": 1.66666667, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.128, \"gal1RGexp\": -0.242, \"gal80Rsig\": 0.012703, \"Stress\": 0.0, \"id\": \"1484\", \"gal4RGexp\": -0.247, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"nuclear organization and biogenesis\", \"nucleocytoplasmic transport\", \"rRNA processing\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1484}}, {\"position\": {\"y\": 3783.684403900709, \"x\": 1548.9363172829344}, \"selected\": false, \"data\": {\"id_original\": \"2192\", \"name\": \"YBR118W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"TEF2\", \"selected\": false, \"BetweennessCentrality\": 0.16666667, \"gal1RGsig\": 0.053125, \"annotation_GO_CELLULAR_COMPONENT\": [\"eukaryotic translation elongation factor 1 complex\", \"ribosome\"], \"alias\": [\"translational elongation factor EF-1 alpha\", \"EF-1 alpha\", \"S000000322\", \"TEF2\", \"eEF1A\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"translation elongation factor activity\"], \"gal4RGsig\": 0.15497, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YBR118W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.75, \"AverageShortestPathLength\": 1.33333333, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": 0.044, \"gal1RGexp\": -0.074, \"gal80Rsig\": 0.54556, \"Stress\": 2.0, \"id\": \"1483\", \"gal4RGexp\": -0.063, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"translational elongation\"], \"Radiality\": 0.83333333, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1483}}, {\"position\": {\"y\": 3777.1561293645764, \"x\": 1454.728950339575}, \"selected\": false, \"data\": {\"id_original\": \"2193\", \"name\": \"YPR080W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"TEF1\", \"selected\": false, \"BetweennessCentrality\": 0.16666667, \"gal1RGsig\": 0.00098725, \"annotation_GO_CELLULAR_COMPONENT\": [\"eukaryotic translation elongation factor 1 complex\", \"ribosome\"], \"alias\": [\"translational elongation factor EF-1 alpha\", \"EF-1 alpha\", \"S000006284\", \"TEF1\", \"eEF1A\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"translation elongation factor activity\"], \"gal4RGsig\": 0.89728, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YPR080W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.75, \"AverageShortestPathLength\": 1.33333333, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.278, \"gal1RGexp\": -0.138, \"gal80Rsig\": 0.00067798, \"Stress\": 2.0, \"id\": \"1482\", \"gal4RGexp\": 0.009, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"translational elongation\"], \"Radiality\": 0.83333333, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1482}}, {\"position\": {\"y\": 3871.6342711492443, \"x\": 1457.6393965065672}, \"selected\": false, \"data\": {\"id_original\": \"2194\", \"name\": \"YLR249W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"YEF3\", \"selected\": false, \"BetweennessCentrality\": 0.16666667, \"gal1RGsig\": 2.713e-08, \"annotation_GO_CELLULAR_COMPONENT\": [\"ribosome\"], \"alias\": [\"Translation elongation factor 3 (EF-3)\", \"EF-3\", \"S000004239\", \"TEF3\", \"YEF3\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"translation elongation factor activity\"], \"gal4RGsig\": 0.04747, \"TopologicalCoefficient\": 1.0, \"shared_name\": \"YLR249W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.75, \"AverageShortestPathLength\": 1.33333333, \"NeighborhoodConnectivity\": 2.0, \"gal80Rexp\": -0.769, \"gal1RGexp\": -0.39, \"gal80Rsig\": 0.035939, \"Stress\": 2.0, \"id\": \"1481\", \"gal4RGexp\": -0.394, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"translational elongation\"], \"Radiality\": 0.83333333, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 2.0, \"Degree\": 2, \"SUID\": 1481}}, {\"position\": {\"y\": 3869.573159699049, \"x\": 1354.728950339575}, \"selected\": false, \"data\": {\"id_original\": \"2195\", \"name\": \"YOR204W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"DED1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.39944, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\"], \"alias\": [\"DED1\", \"S000005730\", \"SPP81\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"RNA helicase activity\"], \"gal4RGsig\": 0.31268, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YOR204W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.6, \"AverageShortestPathLength\": 1.66666667, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.91, \"gal1RGexp\": -0.033, \"gal80Rsig\": 8.349e-16, \"Stress\": 0.0, \"id\": \"1480\", \"gal4RGexp\": -0.056, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"RNA splicing\", \"translational initiation\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1480}}, {\"position\": {\"y\": 3863.618310455885, \"x\": 1244.8050611794188}, \"selected\": false, \"data\": {\"id_original\": \"2196\", \"name\": \"YGL097W\", \"Eccentricity\": 1, \"IsSingleNode\": false, \"COMMON\": \"SRM1\", \"selected\": false, \"BetweennessCentrality\": 1.0, \"gal1RGsig\": 0.0021913, \"annotation_GO_CELLULAR_COMPONENT\": [\"nuclear chromatin\", \"nucleus\"], \"alias\": [\"MTR1\", \"PRP20\", \"S000003065\", \"SRM1\", \"pheromone response pathway suppressor\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"signal transducer activity\"], \"gal4RGsig\": 0.0022461, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGL097W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 1.0, \"AverageShortestPathLength\": 1.0, \"NeighborhoodConnectivity\": 1.0, \"gal80Rexp\": 0.008, \"gal1RGexp\": 0.16, \"gal80Rsig\": 0.93826, \"Stress\": 6.0, \"id\": \"1479\", \"gal4RGexp\": -0.23, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"rRNA export from nucleus\", \"ribosome export from nucleus\"], \"Radiality\": 1.0, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1479}}, {\"position\": {\"y\": 3972.2636961980725, \"x\": 1227.2234876930906}, \"selected\": false, \"data\": {\"id_original\": \"2197\", \"name\": \"YGR218W\", \"Eccentricity\": 2, \"IsSingleNode\": false, \"COMMON\": \"CRM1\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.61381, \"annotation_GO_CELLULAR_COMPONENT\": [\"nucleus\"], \"alias\": [\"CRM1\", \"KAP124\", \"S000003450\", \"XPO1\", \"chromosome region maintenance protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"protein carrier activity\"], \"gal4RGsig\": 0.9794, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YGR218W\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.6, \"AverageShortestPathLength\": 1.66666667, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": -0.018, \"gal1RGexp\": -0.018, \"gal80Rsig\": 0.80969, \"Stress\": 0.0, \"id\": \"1478\", \"gal4RGexp\": -0.001, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mRNA export from nucleus\", \"protein export from nucleus\", \"ribosomal large subunit export from nucleus\"], \"Radiality\": 0.66666667, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1478}}, {\"position\": {\"y\": 2678.9541029973884, \"x\": 3333.076591330786}, \"selected\": false, \"data\": {\"id_original\": \"2198\", \"name\": \"YGL122C\", \"Eccentricity\": 25, \"IsSingleNode\": false, \"COMMON\": \"NAB2\", \"selected\": false, \"BetweennessCentrality\": 0.02406295, \"gal1RGsig\": 0.00087295, \"annotation_GO_CELLULAR_COMPONENT\": [\"cytoplasm\", \"nucleus\"], \"alias\": [\"NAB2\", \"S000003090\", \"polyadenylated RNA binding protein\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"poly(A) binding\"], \"gal4RGsig\": 0.61707, \"TopologicalCoefficient\": 0.33333333, \"shared_name\": \"YGL122C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.06956522, \"AverageShortestPathLength\": 14.375, \"NeighborhoodConnectivity\": 1.66666667, \"gal80Rexp\": 0.187, \"gal1RGexp\": 0.174, \"gal80Rsig\": 0.0059966, \"Stress\": 5902.0, \"id\": \"1477\", \"gal4RGexp\": 0.02, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"mRNA polyadenylation\", \"poly(A)+ mRNA export from nucleus\"], \"Radiality\": 0.50462963, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 3.0, \"Degree\": 3, \"SUID\": 1477}}, {\"position\": {\"y\": 2725.676393036451, \"x\": 3441.2708051979735}, \"selected\": false, \"data\": {\"id_original\": \"2199\", \"name\": \"YKR026C\", \"Eccentricity\": 26, \"IsSingleNode\": false, \"COMMON\": \"GCN3\", \"selected\": false, \"BetweennessCentrality\": 0.0, \"gal1RGsig\": 0.00091177, \"annotation_GO_CELLULAR_COMPONENT\": [\"eukaryotic translation initiation factor 2B complex\", \"guanyl-nucleotide exchange factor complex\"], \"alias\": [\"AAS2\", \"GCN3\", \"S000001734\", \"eIF2B 34 kDa alpha subunit\"], \"annotation_GO_MOLECULAR_FUNCTION\": [\"enzyme regulator activity\", \"guanyl-nucleotide exchange factor activity\", \"translation initiation factor activity\"], \"gal4RGsig\": 3.5692e-06, \"TopologicalCoefficient\": 0.0, \"shared_name\": \"YKR026C\", \"PartnerOfMultiEdgedNodePairs\": 0.0, \"SelfLoops\": 0.0, \"ClosenessCentrality\": 0.06505771, \"AverageShortestPathLength\": 15.37096774, \"NeighborhoodConnectivity\": 3.0, \"gal80Rexp\": 0.292, \"gal1RGexp\": -0.154, \"gal80Rsig\": 0.011229, \"Stress\": 0.0, \"id\": \"1476\", \"gal4RGexp\": -0.501, \"annotation_GO_BIOLOGICAL_PROCESS\": [\"regulation of translational initiation\"], \"Radiality\": 0.46774194, \"ClusteringCoefficient\": 0.0, \"NumberOfUndirectedEdges\": 0.0, \"NumberOfDirectedEdges\": 1.0, \"Degree\": 1, \"SUID\": 1476}}],\n", | |
" edges: [{\"selected\": false, \"data\": {\"id_original\": \"2200\", \"name\": \"YDR277C (pp) YDL194W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2168\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1870\", \"target_original\": \"1869\", \"target\": \"1806\", \"source\": \"1805\", \"shared_name\": \"YDR277C (pp) YDL194W\", \"SUID\": 2168}}, {\"selected\": false, \"data\": {\"id_original\": \"2201\", \"name\": \"YDR277C (pp) YJR022W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2167\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"1870\", \"target_original\": \"2172\", \"target\": \"1503\", \"source\": \"1805\", \"shared_name\": \"YDR277C (pp) YJR022W\", \"SUID\": 2167}}, {\"selected\": false, \"data\": {\"id_original\": \"2202\", \"name\": \"YPR145W (pp) YMR117C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2166\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1872\", \"target_original\": \"1891\", \"target\": \"1784\", \"source\": \"1803\", \"shared_name\": \"YPR145W (pp) YMR117C\", \"SUID\": 2166}}, {\"selected\": false, \"data\": {\"id_original\": \"2203\", \"name\": \"YER054C (pp) YBR045C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2165\", \"EdgeBetweenness\": 2746.0, \"shared_interaction\": \"pp\", \"source_original\": \"1873\", \"target_original\": \"1874\", \"target\": \"1801\", \"source\": \"1802\", \"shared_name\": \"YER054C (pp) YBR045C\", \"SUID\": 2165}}, {\"selected\": false, \"data\": {\"id_original\": \"2204\", \"name\": \"YER054C (pp) YER133W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2164\", \"EdgeBetweenness\": 3174.0, \"shared_interaction\": \"pp\", \"source_original\": \"1873\", \"target_original\": \"1987\", \"target\": \"1688\", \"source\": \"1802\", \"shared_name\": \"YER054C (pp) YER133W\", \"SUID\": 2164}}, {\"selected\": false, \"data\": {\"id_original\": \"2205\", \"name\": \"YBR045C (pp) YOR178C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2163\", \"EdgeBetweenness\": 2962.0, \"shared_interaction\": \"pp\", \"source_original\": \"1874\", \"target_original\": \"1986\", \"target\": \"1689\", \"source\": \"1801\", \"shared_name\": \"YBR045C (pp) YOR178C\", \"SUID\": 2163}}, {\"selected\": false, \"data\": {\"id_original\": \"2206\", \"name\": \"YBR045C (pp) YIL045W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2162\", \"EdgeBetweenness\": 5236.0, \"shared_interaction\": \"pp\", \"source_original\": \"1874\", \"target_original\": \"1910\", \"target\": \"1765\", \"source\": \"1801\", \"shared_name\": \"YBR045C (pp) YIL045W\", \"SUID\": 2162}}, {\"selected\": false, \"data\": {\"id_original\": \"2207\", \"name\": \"YBL079W (pp) YDL088C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2161\", \"EdgeBetweenness\": 6.0, \"shared_interaction\": \"pp\", \"source_original\": \"1875\", \"target_original\": \"1888\", \"target\": \"1787\", \"source\": \"1800\", \"shared_name\": \"YBL079W (pp) YDL088C\", \"SUID\": 2161}}, {\"selected\": false, \"data\": {\"id_original\": \"2208\", \"name\": \"YLR345W (pp) YLR321C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2160\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1876\", \"target_original\": \"2018\", \"target\": \"1657\", \"source\": \"1799\", \"shared_name\": \"YLR345W (pp) YLR321C\", \"SUID\": 2160}}, {\"selected\": false, \"data\": {\"id_original\": \"2209\", \"name\": \"YGR136W (pp) YGR058W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2159\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1882\", \"target_original\": \"2091\", \"target\": \"1584\", \"source\": \"1793\", \"shared_name\": \"YGR136W (pp) YGR058W\", \"SUID\": 2159}}, {\"selected\": false, \"data\": {\"id_original\": \"2210\", \"name\": \"YDL023C (pp) YJL159W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2158\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1883\", \"target_original\": \"2074\", \"target\": \"1601\", \"source\": \"1792\", \"shared_name\": \"YDL023C (pp) YJL159W\", \"SUID\": 2158}}, {\"selected\": false, \"data\": {\"id_original\": \"2211\", \"name\": \"YBR170C (pp) YGR048W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2157\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"1884\", \"target_original\": \"1993\", \"target\": \"1682\", \"source\": \"1791\", \"shared_name\": \"YBR170C (pp) YGR048W\", \"SUID\": 2157}}, {\"selected\": false, \"data\": {\"id_original\": \"2212\", \"name\": \"YGR074W (pp) YBR043C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2156\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"1885\", \"target_original\": \"1871\", \"target\": \"1804\", \"source\": \"1790\", \"shared_name\": \"YGR074W (pp) YBR043C\", \"SUID\": 2156}}, {\"selected\": false, \"data\": {\"id_original\": \"2213\", \"name\": \"YGL202W (pp) YGR074W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2155\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"1886\", \"target_original\": \"1885\", \"target\": \"1790\", \"source\": \"1789\", \"shared_name\": \"YGL202W (pp) YGR074W\", \"SUID\": 2155}}, {\"selected\": false, \"data\": {\"id_original\": \"2214\", \"name\": \"YLR197W (pp) YOR310C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2154\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"1887\", \"target_original\": \"2135\", \"target\": \"1540\", \"source\": \"1788\", \"shared_name\": \"YLR197W (pp) YOR310C\", \"SUID\": 2154}}, {\"selected\": false, \"data\": {\"id_original\": \"2215\", \"name\": \"YLR197W (pp) YDL014W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2153\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"1887\", \"target_original\": \"2136\", \"target\": \"1539\", \"source\": \"1788\", \"shared_name\": \"YLR197W (pp) YDL014W\", \"SUID\": 2153}}, {\"selected\": false, \"data\": {\"id_original\": \"2216\", \"name\": \"YDL088C (pp) YER110C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2152\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"1888\", \"target_original\": \"1950\", \"target\": \"1725\", \"source\": \"1787\", \"shared_name\": \"YDL088C (pp) YER110C\", \"SUID\": 2152}}, {\"selected\": false, \"data\": {\"id_original\": \"2217\", \"name\": \"YMR117C (pp) YPR010C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2151\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1891\", \"target_original\": \"1890\", \"target\": \"1785\", \"source\": \"1784\", \"shared_name\": \"YMR117C (pp) YPR010C\", \"SUID\": 2151}}, {\"selected\": false, \"data\": {\"id_original\": \"2218\", \"name\": \"YMR117C (pp) YCL032W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2150\", \"EdgeBetweenness\": 1476.0, \"shared_interaction\": \"pp\", \"source_original\": \"1891\", \"target_original\": \"2116\", \"target\": \"1559\", \"source\": \"1784\", \"shared_name\": \"YMR117C (pp) YCL032W\", \"SUID\": 2150}}, {\"selected\": false, \"data\": {\"id_original\": \"2219\", \"name\": \"YML114C (pp) YDR167W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2149\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1892\", \"target_original\": \"1942\", \"target\": \"1733\", \"source\": \"1783\", \"shared_name\": \"YML114C (pp) YDR167W\", \"SUID\": 2149}}, {\"selected\": false, \"data\": {\"id_original\": \"2220\", \"name\": \"YNL036W (pp) YIR009W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2148\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1893\", \"target_original\": \"2104\", \"target\": \"1571\", \"source\": \"1782\", \"shared_name\": \"YNL036W (pp) YIR009W\", \"SUID\": 2148}}, {\"selected\": false, \"data\": {\"id_original\": \"2221\", \"name\": \"YOR212W (pp) YLR362W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2147\", \"EdgeBetweenness\": 8540.0041292, \"shared_interaction\": \"pp\", \"source_original\": \"1894\", \"target_original\": \"2114\", \"target\": \"1561\", \"source\": \"1781\", \"shared_name\": \"YOR212W (pp) YLR362W\", \"SUID\": 2147}}, {\"selected\": false, \"data\": {\"id_original\": \"2222\", \"name\": \"YDR070C (pp) YFL017C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2146\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1895\", \"target_original\": \"2188\", \"target\": \"1487\", \"source\": \"1780\", \"shared_name\": \"YDR070C (pp) YFL017C\", \"SUID\": 2146}}, {\"selected\": false, \"data\": {\"id_original\": \"2223\", \"name\": \"YGR046W (pp) YNL236W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2145\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1897\", \"target_original\": \"2099\", \"target\": \"1576\", \"source\": \"1778\", \"shared_name\": \"YGR046W (pp) YNL236W\", \"SUID\": 2145}}, {\"selected\": false, \"data\": {\"id_original\": \"2224\", \"name\": \"YIL070C (pp) YML054C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2144\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1899\", \"target_original\": \"2087\", \"target\": \"1588\", \"source\": \"1776\", \"shared_name\": \"YIL070C (pp) YML054C\", \"SUID\": 2144}}, {\"selected\": false, \"data\": {\"id_original\": \"2225\", \"name\": \"YPR113W (pd) YMR043W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2143\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"1900\", \"target_original\": \"2076\", \"target\": \"1599\", \"source\": \"1775\", \"shared_name\": \"YPR113W (pd) YMR043W\", \"SUID\": 2143}}, {\"selected\": false, \"data\": {\"id_original\": \"2226\", \"name\": \"YER081W (pp) YIL074C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2142\", \"EdgeBetweenness\": 6.0, \"shared_interaction\": \"pp\", \"source_original\": \"1901\", \"target_original\": \"1913\", \"target\": \"1762\", \"source\": \"1774\", \"shared_name\": \"YER081W (pp) YIL074C\", \"SUID\": 2142}}, {\"selected\": false, \"data\": {\"id_original\": \"2227\", \"name\": \"YGR088W (pd) YLR256W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2141\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"1902\", \"target_original\": \"2088\", \"target\": \"1587\", \"source\": \"1773\", \"shared_name\": \"YGR088W (pd) YLR256W\", \"SUID\": 2141}}, {\"selected\": false, \"data\": {\"id_original\": \"2228\", \"name\": \"YDR395W (pp) YPR102C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2140\", \"EdgeBetweenness\": 739.0, \"shared_interaction\": \"pp\", \"source_original\": \"1903\", \"target_original\": \"1933\", \"target\": \"1742\", \"source\": \"1772\", \"shared_name\": \"YDR395W (pp) YPR102C\", \"SUID\": 2140}}, {\"selected\": false, \"data\": {\"id_original\": \"2229\", \"name\": \"YDR395W (pp) YOL127W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2139\", \"EdgeBetweenness\": 1929.0, \"shared_interaction\": \"pp\", \"source_original\": \"1903\", \"target_original\": \"2149\", \"target\": \"1526\", \"source\": \"1772\", \"shared_name\": \"YDR395W (pp) YOL127W\", \"SUID\": 2139}}, {\"selected\": false, \"data\": {\"id_original\": \"2230\", \"name\": \"YDR395W (pp) YIL052C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2138\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1903\", \"target_original\": \"1877\", \"target\": \"1798\", \"source\": \"1772\", \"shared_name\": \"YDR395W (pp) YIL052C\", \"SUID\": 2138}}, {\"selected\": false, \"data\": {\"id_original\": \"2231\", \"name\": \"YDR395W (pp) YER056CA\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2137\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1903\", \"target_original\": \"1878\", \"target\": \"1797\", \"source\": \"1772\", \"shared_name\": \"YDR395W (pp) YER056CA\", \"SUID\": 2137}}, {\"selected\": false, \"data\": {\"id_original\": \"2232\", \"name\": \"YDR395W (pp) YNL069C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2136\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1903\", \"target_original\": \"1879\", \"target\": \"1796\", \"source\": \"1772\", \"shared_name\": \"YDR395W (pp) YNL069C\", \"SUID\": 2136}}, {\"selected\": false, \"data\": {\"id_original\": \"2233\", \"name\": \"YDR395W (pp) YIL133C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2135\", \"EdgeBetweenness\": 1929.0, \"shared_interaction\": \"pp\", \"source_original\": \"1903\", \"target_original\": \"2158\", \"target\": \"1517\", \"source\": \"1772\", \"shared_name\": \"YDR395W (pp) YIL133C\", \"SUID\": 2135}}, {\"selected\": false, \"data\": {\"id_original\": \"2234\", \"name\": \"YDR395W (pp) YDL075W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2134\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1903\", \"target_original\": \"1880\", \"target\": \"1795\", \"source\": \"1772\", \"shared_name\": \"YDR395W (pp) YDL075W\", \"SUID\": 2134}}, {\"selected\": false, \"data\": {\"id_original\": \"2235\", \"name\": \"YGR085C (pp) YDR395W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2133\", \"EdgeBetweenness\": 739.0, \"shared_interaction\": \"pp\", \"source_original\": \"1904\", \"target_original\": \"1903\", \"target\": \"1772\", \"source\": \"1771\", \"shared_name\": \"YGR085C (pp) YDR395W\", \"SUID\": 2133}}, {\"selected\": false, \"data\": {\"id_original\": \"2236\", \"name\": \"YGR085C (pp) YLR075W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2132\", \"EdgeBetweenness\": 249.0, \"shared_interaction\": \"pp\", \"source_original\": \"1904\", \"target_original\": \"1934\", \"target\": \"1741\", \"source\": \"1771\", \"shared_name\": \"YGR085C (pp) YLR075W\", \"SUID\": 2132}}, {\"selected\": false, \"data\": {\"id_original\": \"2237\", \"name\": \"YDL030W (pp) YMR005W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2131\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1907\", \"target_original\": \"1906\", \"target\": \"1769\", \"source\": \"1768\", \"shared_name\": \"YDL030W (pp) YMR005W\", \"SUID\": 2131}}, {\"selected\": false, \"data\": {\"id_original\": \"2238\", \"name\": \"YDL030W (pp) YDL013W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2130\", \"EdgeBetweenness\": 7802.71708292, \"shared_interaction\": \"pp\", \"source_original\": \"1907\", \"target_original\": \"2165\", \"target\": \"1510\", \"source\": \"1768\", \"shared_name\": \"YDL030W (pp) YDL013W\", \"SUID\": 2130}}, {\"selected\": false, \"data\": {\"id_original\": \"2239\", \"name\": \"YER079W (pp) YNL154C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2129\", \"EdgeBetweenness\": 12.0, \"shared_interaction\": \"pp\", \"source_original\": \"1908\", \"target_original\": \"2030\", \"target\": \"1645\", \"source\": \"1767\", \"shared_name\": \"YER079W (pp) YNL154C\", \"SUID\": 2129}}, {\"selected\": false, \"data\": {\"id_original\": \"2240\", \"name\": \"YER079W (pp) YHR135C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2128\", \"EdgeBetweenness\": 12.0, \"shared_interaction\": \"pp\", \"source_original\": \"1908\", \"target_original\": \"2033\", \"target\": \"1642\", \"source\": \"1767\", \"shared_name\": \"YER079W (pp) YHR135C\", \"SUID\": 2128}}, {\"selected\": false, \"data\": {\"id_original\": \"2241\", \"name\": \"YDL215C (pp) YLR432W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2127\", \"EdgeBetweenness\": 1476.0, \"shared_interaction\": \"pp\", \"source_original\": \"1909\", \"target_original\": \"1941\", \"target\": \"1734\", \"source\": \"1766\", \"shared_name\": \"YDL215C (pp) YLR432W\", \"SUID\": 2127}}, {\"selected\": false, \"data\": {\"id_original\": \"2242\", \"name\": \"YDL215C (pd) YER040W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2126\", \"EdgeBetweenness\": 1476.0, \"shared_interaction\": \"pd\", \"source_original\": \"1909\", \"target_original\": \"2055\", \"target\": \"1620\", \"source\": \"1766\", \"shared_name\": \"YDL215C (pd) YER040W\", \"SUID\": 2126}}, {\"selected\": false, \"data\": {\"id_original\": \"2243\", \"name\": \"YPR041W (pp) YMR309C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2125\", \"EdgeBetweenness\": 265.33333333, \"shared_interaction\": \"pp\", \"source_original\": \"1911\", \"target_original\": \"2130\", \"target\": \"1545\", \"source\": \"1764\", \"shared_name\": \"YPR041W (pp) YMR309C\", \"SUID\": 2125}}, {\"selected\": false, \"data\": {\"id_original\": \"2244\", \"name\": \"YPR041W (pp) YOR361C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2124\", \"EdgeBetweenness\": 230.66666667, \"shared_interaction\": \"pp\", \"source_original\": \"1911\", \"target_original\": \"2131\", \"target\": \"1544\", \"source\": \"1764\", \"shared_name\": \"YPR041W (pp) YOR361C\", \"SUID\": 2124}}, {\"selected\": false, \"data\": {\"id_original\": \"2245\", \"name\": \"YOR120W (pd) YPL248C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2123\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"1912\", \"target_original\": \"2106\", \"target\": \"1569\", \"source\": \"1763\", \"shared_name\": \"YOR120W (pd) YPL248C\", \"SUID\": 2123}}, {\"selected\": false, \"data\": {\"id_original\": \"2246\", \"name\": \"YIL074C (pp) YNL311C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2122\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"1913\", \"target_original\": \"2009\", \"target\": \"1666\", \"source\": \"1762\", \"shared_name\": \"YIL074C (pp) YNL311C\", \"SUID\": 2122}}, {\"selected\": false, \"data\": {\"id_original\": \"2247\", \"name\": \"YDR299W (pd) YJL194W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2121\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"1914\", \"target_original\": \"1922\", \"target\": \"1753\", \"source\": \"1761\", \"shared_name\": \"YDR299W (pd) YJL194W\", \"SUID\": 2121}}, {\"selected\": false, \"data\": {\"id_original\": \"2248\", \"name\": \"YHR005C (pp) YLR362W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2120\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"1915\", \"target_original\": \"2114\", \"target\": \"1561\", \"source\": \"1760\", \"shared_name\": \"YHR005C (pp) YLR362W\", \"SUID\": 2120}}, {\"selected\": false, \"data\": {\"id_original\": \"2249\", \"name\": \"YLR452C (pp) YHR005C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2119\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1916\", \"target_original\": \"1915\", \"target\": \"1760\", \"source\": \"1759\", \"shared_name\": \"YLR452C (pp) YHR005C\", \"SUID\": 2119}}, {\"selected\": false, \"data\": {\"id_original\": \"2250\", \"name\": \"YMR255W (pp) YGL122C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2118\", \"EdgeBetweenness\": 1960.0, \"shared_interaction\": \"pp\", \"source_original\": \"1917\", \"target_original\": \"2198\", \"target\": \"1477\", \"source\": \"1758\", \"shared_name\": \"YMR255W (pp) YGL122C\", \"SUID\": 2118}}, {\"selected\": false, \"data\": {\"id_original\": \"2251\", \"name\": \"YBR274W (pp) YMR255W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2117\", \"EdgeBetweenness\": 2440.0, \"shared_interaction\": \"pp\", \"source_original\": \"1918\", \"target_original\": \"1917\", \"target\": \"1758\", \"source\": \"1757\", \"shared_name\": \"YBR274W (pp) YMR255W\", \"SUID\": 2117}}, {\"selected\": false, \"data\": {\"id_original\": \"2252\", \"name\": \"YHR084W (pd) YFL026W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2116\", \"EdgeBetweenness\": 4.83333333, \"shared_interaction\": \"pd\", \"source_original\": \"1919\", \"target_original\": \"2068\", \"target\": \"1607\", \"source\": \"1756\", \"shared_name\": \"YHR084W (pd) YFL026W\", \"SUID\": 2116}}, {\"selected\": false, \"data\": {\"id_original\": \"2253\", \"name\": \"YHR084W (pd) YDR461W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2115\", \"EdgeBetweenness\": 4.83333333, \"shared_interaction\": \"pd\", \"source_original\": \"1919\", \"target_original\": \"2071\", \"target\": \"1604\", \"source\": \"1756\", \"shared_name\": \"YHR084W (pd) YDR461W\", \"SUID\": 2115}}, {\"selected\": false, \"data\": {\"id_original\": \"2254\", \"name\": \"YHR084W (pp) YMR043W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2114\", \"EdgeBetweenness\": 485.5, \"shared_interaction\": \"pp\", \"source_original\": \"1919\", \"target_original\": \"2076\", \"target\": \"1599\", \"source\": \"1756\", \"shared_name\": \"YHR084W (pp) YMR043W\", \"SUID\": 2114}}, {\"selected\": false, \"data\": {\"id_original\": \"2255\", \"name\": \"YBL050W (pp) YOR036W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2113\", \"EdgeBetweenness\": 6133.28291708, \"shared_interaction\": \"pp\", \"source_original\": \"1920\", \"target_original\": \"2057\", \"target\": \"1618\", \"source\": \"1755\", \"shared_name\": \"YBL050W (pp) YOR036W\", \"SUID\": 2113}}, {\"selected\": false, \"data\": {\"id_original\": \"2256\", \"name\": \"YBL026W (pp) YOR167C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2112\", \"EdgeBetweenness\": 248.5, \"shared_interaction\": \"pp\", \"source_original\": \"1921\", \"target_original\": \"2173\", \"target\": \"1502\", \"source\": \"1754\", \"shared_name\": \"YBL026W (pp) YOR167C\", \"SUID\": 2112}}, {\"selected\": false, \"data\": {\"id_original\": \"2257\", \"name\": \"YJL194W (pd) YMR043W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2111\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pd\", \"source_original\": \"1922\", \"target_original\": \"2076\", \"target\": \"1599\", \"source\": \"1753\", \"shared_name\": \"YJL194W (pd) YMR043W\", \"SUID\": 2111}}, {\"selected\": false, \"data\": {\"id_original\": \"2258\", \"name\": \"YLR258W (pp) YBR274W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2110\", \"EdgeBetweenness\": 2916.0, \"shared_interaction\": \"pp\", \"source_original\": \"1923\", \"target_original\": \"1918\", \"target\": \"1757\", \"source\": \"1752\", \"shared_name\": \"YLR258W (pp) YBR274W\", \"SUID\": 2110}}, {\"selected\": false, \"data\": {\"id_original\": \"2259\", \"name\": \"YLR258W (pp) YIL045W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2109\", \"EdgeBetweenness\": 4780.0, \"shared_interaction\": \"pp\", \"source_original\": \"1923\", \"target_original\": \"1910\", \"target\": \"1765\", \"source\": \"1752\", \"shared_name\": \"YLR258W (pp) YIL045W\", \"SUID\": 2109}}, {\"selected\": false, \"data\": {\"id_original\": \"2260\", \"name\": \"YGL134W (pp) YLR258W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2108\", \"EdgeBetweenness\": 1476.0, \"shared_interaction\": \"pp\", \"source_original\": \"1924\", \"target_original\": \"1923\", \"target\": \"1752\", \"source\": \"1751\", \"shared_name\": \"YGL134W (pp) YLR258W\", \"SUID\": 2108}}, {\"selected\": false, \"data\": {\"id_original\": \"2261\", \"name\": \"YGL134W (pp) YPL031C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2107\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"1924\", \"target_original\": \"1974\", \"target\": \"1701\", \"source\": \"1751\", \"shared_name\": \"YGL134W (pp) YPL031C\", \"SUID\": 2107}}, {\"selected\": false, \"data\": {\"id_original\": \"2262\", \"name\": \"YPR124W (pd) YMR021C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2106\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pd\", \"source_original\": \"1927\", \"target_original\": \"2043\", \"target\": \"1632\", \"source\": \"1748\", \"shared_name\": \"YPR124W (pd) YMR021C\", \"SUID\": 2106}}, {\"selected\": false, \"data\": {\"id_original\": \"2263\", \"name\": \"YNL135C (pp) YDR174W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2105\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"1928\", \"target_original\": \"2040\", \"target\": \"1635\", \"source\": \"1747\", \"shared_name\": \"YNL135C (pp) YDR174W\", \"SUID\": 2105}}, {\"selected\": false, \"data\": {\"id_original\": \"2264\", \"name\": \"YER052C (pp) YNL135C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2104\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1929\", \"target_original\": \"1928\", \"target\": \"1747\", \"source\": \"1746\", \"shared_name\": \"YER052C (pp) YNL135C\", \"SUID\": 2104}}, {\"selected\": false, \"data\": {\"id_original\": \"2265\", \"name\": \"YPL240C (pp) YOR036W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2103\", \"EdgeBetweenness\": 8233.28291708, \"shared_interaction\": \"pp\", \"source_original\": \"1932\", \"target_original\": \"2057\", \"target\": \"1618\", \"source\": \"1743\", \"shared_name\": \"YPL240C (pp) YOR036W\", \"SUID\": 2103}}, {\"selected\": false, \"data\": {\"id_original\": \"2266\", \"name\": \"YPL240C (pp) YBR155W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2102\", \"EdgeBetweenness\": 323.0, \"shared_interaction\": \"pp\", \"source_original\": \"1932\", \"target_original\": \"2126\", \"target\": \"1549\", \"source\": \"1743\", \"shared_name\": \"YPL240C (pp) YBR155W\", \"SUID\": 2102}}, {\"selected\": false, \"data\": {\"id_original\": \"2267\", \"name\": \"YLR075W (pp) YPR102C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2101\", \"EdgeBetweenness\": 249.0, \"shared_interaction\": \"pp\", \"source_original\": \"1934\", \"target_original\": \"1933\", \"target\": \"1742\", \"source\": \"1741\", \"shared_name\": \"YLR075W (pp) YPR102C\", \"SUID\": 2101}}, {\"selected\": false, \"data\": {\"id_original\": \"2268\", \"name\": \"YKL161C (pp) YPL089C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2100\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1935\", \"target_original\": \"2048\", \"target\": \"1627\", \"source\": \"1740\", \"shared_name\": \"YKL161C (pp) YPL089C\", \"SUID\": 2100}}, {\"selected\": false, \"data\": {\"id_original\": \"2269\", \"name\": \"YAR007C (pd) YPL111W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2099\", \"EdgeBetweenness\": 739.0, \"shared_interaction\": \"pd\", \"source_original\": \"1936\", \"target_original\": \"2012\", \"target\": \"1663\", \"source\": \"1739\", \"shared_name\": \"YAR007C (pd) YPL111W\", \"SUID\": 2099}}, {\"selected\": false, \"data\": {\"id_original\": \"2270\", \"name\": \"YAR007C (pp) YML032C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2098\", \"EdgeBetweenness\": 249.0, \"shared_interaction\": \"pp\", \"source_original\": \"1936\", \"target_original\": \"2097\", \"target\": \"1578\", \"source\": \"1739\", \"shared_name\": \"YAR007C (pp) YML032C\", \"SUID\": 2098}}, {\"selected\": false, \"data\": {\"id_original\": \"2271\", \"name\": \"YDR142C (pp) YIL160C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2097\", \"EdgeBetweenness\": 12.0, \"shared_interaction\": \"pp\", \"source_original\": \"1939\", \"target_original\": \"1937\", \"target\": \"1738\", \"source\": \"1736\", \"shared_name\": \"YDR142C (pp) YIL160C\", \"SUID\": 2097}}, {\"selected\": false, \"data\": {\"id_original\": \"2272\", \"name\": \"YDR142C (pp) YGL153W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2096\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"1939\", \"target_original\": \"1951\", \"target\": \"1724\", \"source\": \"1736\", \"shared_name\": \"YDR142C (pp) YGL153W\", \"SUID\": 2096}}, {\"selected\": false, \"data\": {\"id_original\": \"2273\", \"name\": \"YDR244W (pp) YDL078C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2095\", \"EdgeBetweenness\": 12.0, \"shared_interaction\": \"pp\", \"source_original\": \"1940\", \"target_original\": \"1938\", \"target\": \"1737\", \"source\": \"1735\", \"shared_name\": \"YDR244W (pp) YDL078C\", \"SUID\": 2095}}, {\"selected\": false, \"data\": {\"id_original\": \"2274\", \"name\": \"YDR244W (pp) YDR142C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2094\", \"EdgeBetweenness\": 12.0, \"shared_interaction\": \"pp\", \"source_original\": \"1940\", \"target_original\": \"1939\", \"target\": \"1736\", \"source\": \"1735\", \"shared_name\": \"YDR244W (pp) YDR142C\", \"SUID\": 2094}}, {\"selected\": false, \"data\": {\"id_original\": \"2275\", \"name\": \"YDR244W (pp) YNL214W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2093\", \"EdgeBetweenness\": 7.0, \"shared_interaction\": \"pp\", \"source_original\": \"1940\", \"target_original\": \"1947\", \"target\": \"1728\", \"source\": \"1735\", \"shared_name\": \"YDR244W (pp) YNL214W\", \"SUID\": 2093}}, {\"selected\": false, \"data\": {\"id_original\": \"2276\", \"name\": \"YDR244W (pp) YGL153W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2092\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"1940\", \"target_original\": \"1951\", \"target\": \"1724\", \"source\": \"1735\", \"shared_name\": \"YDR244W (pp) YGL153W\", \"SUID\": 2092}}, {\"selected\": false, \"data\": {\"id_original\": \"2277\", \"name\": \"YDR244W (pp) YLR191W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2091\", \"EdgeBetweenness\": 7.0, \"shared_interaction\": \"pp\", \"source_original\": \"1940\", \"target_original\": \"1952\", \"target\": \"1723\", \"source\": \"1735\", \"shared_name\": \"YDR244W (pp) YLR191W\", \"SUID\": 2091}}, {\"selected\": false, \"data\": {\"id_original\": \"2278\", \"name\": \"YDR167W (pp) YLR432W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2090\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"1942\", \"target_original\": \"1941\", \"target\": \"1734\", \"source\": \"1733\", \"shared_name\": \"YDR167W (pp) YLR432W\", \"SUID\": 2090}}, {\"selected\": false, \"data\": {\"id_original\": \"2279\", \"name\": \"YLR175W (pp) YNL307C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2089\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1943\", \"target_original\": \"2164\", \"target\": \"1511\", \"source\": \"1732\", \"shared_name\": \"YLR175W (pp) YNL307C\", \"SUID\": 2089}}, {\"selected\": false, \"data\": {\"id_original\": \"2280\", \"name\": \"YNL117W (pd) YJL089W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2088\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"1944\", \"target_original\": \"2046\", \"target\": \"1629\", \"source\": \"1731\", \"shared_name\": \"YNL117W (pd) YJL089W\", \"SUID\": 2088}}, {\"selected\": false, \"data\": {\"id_original\": \"2281\", \"name\": \"YOR089C (pp) YDR323C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2087\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1945\", \"target_original\": \"2058\", \"target\": \"1617\", \"source\": \"1730\", \"shared_name\": \"YOR089C (pp) YDR323C\", \"SUID\": 2087}}, {\"selected\": false, \"data\": {\"id_original\": \"2282\", \"name\": \"YNL214W (pp) YGL153W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2086\", \"EdgeBetweenness\": 5.0, \"shared_interaction\": \"pp\", \"source_original\": \"1947\", \"target_original\": \"1951\", \"target\": \"1724\", \"source\": \"1728\", \"shared_name\": \"YNL214W (pp) YGL153W\", \"SUID\": 2086}}, {\"selected\": false, \"data\": {\"id_original\": \"2283\", \"name\": \"YBR135W (pp) YER102W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2085\", \"EdgeBetweenness\": 3206.08455433, \"shared_interaction\": \"pp\", \"source_original\": \"1948\", \"target_original\": \"1956\", \"target\": \"1719\", \"source\": \"1727\", \"shared_name\": \"YBR135W (pp) YER102W\", \"SUID\": 2085}}, {\"selected\": false, \"data\": {\"id_original\": \"2284\", \"name\": \"YER110C (pp) YML007W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2084\", \"EdgeBetweenness\": 6.0, \"shared_interaction\": \"pp\", \"source_original\": \"1950\", \"target_original\": \"1949\", \"target\": \"1726\", \"source\": \"1725\", \"shared_name\": \"YER110C (pp) YML007W\", \"SUID\": 2084}}, {\"selected\": false, \"data\": {\"id_original\": \"2285\", \"name\": \"YLR191W (pp) YGL153W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2083\", \"EdgeBetweenness\": 5.0, \"shared_interaction\": \"pp\", \"source_original\": \"1952\", \"target_original\": \"1951\", \"target\": \"1724\", \"source\": \"1723\", \"shared_name\": \"YLR191W (pp) YGL153W\", \"SUID\": 2083}}, {\"selected\": false, \"data\": {\"id_original\": \"2286\", \"name\": \"YOL149W (pp) YOR167C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2082\", \"EdgeBetweenness\": 248.5, \"shared_interaction\": \"pp\", \"source_original\": \"1953\", \"target_original\": \"2173\", \"target\": \"1502\", \"source\": \"1722\", \"shared_name\": \"YOL149W (pp) YOR167C\", \"SUID\": 2082}}, {\"selected\": false, \"data\": {\"id_original\": \"2287\", \"name\": \"YMR044W (pp) YIL061C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2081\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1954\", \"target_original\": \"2178\", \"target\": \"1497\", \"source\": \"1721\", \"shared_name\": \"YMR044W (pp) YIL061C\", \"SUID\": 2081}}, {\"selected\": false, \"data\": {\"id_original\": \"2288\", \"name\": \"YNL113W (pp) YPR110C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2080\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"1961\", \"target_original\": \"1960\", \"target\": \"1715\", \"source\": \"1714\", \"shared_name\": \"YNL113W (pp) YPR110C\", \"SUID\": 2080}}, {\"selected\": false, \"data\": {\"id_original\": \"2289\", \"name\": \"YDR354W (pd) YEL009C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2079\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"1962\", \"target_original\": \"2125\", \"target\": \"1550\", \"source\": \"1713\", \"shared_name\": \"YDR354W (pd) YEL009C\", \"SUID\": 2079}}, {\"selected\": false, \"data\": {\"id_original\": \"2290\", \"name\": \"YKL211C (pp) YER090W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2078\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"1964\", \"target_original\": \"1963\", \"target\": \"1712\", \"source\": \"1711\", \"shared_name\": \"YKL211C (pp) YER090W\", \"SUID\": 2078}}, {\"selected\": false, \"data\": {\"id_original\": \"2291\", \"name\": \"YDR146C (pd) YGL035C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2077\", \"EdgeBetweenness\": 19180.22220002, \"shared_interaction\": \"pd\", \"source_original\": \"1965\", \"target_original\": \"2109\", \"target\": \"1566\", \"source\": \"1710\", \"shared_name\": \"YDR146C (pd) YGL035C\", \"SUID\": 2077}}, {\"selected\": false, \"data\": {\"id_original\": \"2292\", \"name\": \"YDR146C (pd) YMR043W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2076\", \"EdgeBetweenness\": 19179.59362859, \"shared_interaction\": \"pd\", \"source_original\": \"1965\", \"target_original\": \"2076\", \"target\": \"1599\", \"source\": \"1710\", \"shared_name\": \"YDR146C (pd) YMR043W\", \"SUID\": 2076}}, {\"selected\": false, \"data\": {\"id_original\": \"2293\", \"name\": \"YER111C (pd) YMR043W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2075\", \"EdgeBetweenness\": 2361.73260073, \"shared_interaction\": \"pd\", \"source_original\": \"1966\", \"target_original\": \"2076\", \"target\": \"1599\", \"source\": \"1709\", \"shared_name\": \"YER111C (pd) YMR043W\", \"SUID\": 2075}}, {\"selected\": false, \"data\": {\"id_original\": \"2294\", \"name\": \"YOR039W (pp) YOR303W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2074\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"1967\", \"target_original\": \"2089\", \"target\": \"1586\", \"source\": \"1708\", \"shared_name\": \"YOR039W (pp) YOR303W\", \"SUID\": 2074}}, {\"selected\": false, \"data\": {\"id_original\": \"2295\", \"name\": \"YML024W (pd) YNL216W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2073\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"1968\", \"target_original\": \"2162\", \"target\": \"1513\", \"source\": \"1707\", \"shared_name\": \"YML024W (pd) YNL216W\", \"SUID\": 2073}}, {\"selected\": false, \"data\": {\"id_original\": \"2296\", \"name\": \"YIL113W (pp) YHR030C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2072\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"1969\", \"target_original\": \"2047\", \"target\": \"1628\", \"source\": \"1706\", \"shared_name\": \"YIL113W (pp) YHR030C\", \"SUID\": 2072}}, {\"selected\": false, \"data\": {\"id_original\": \"2297\", \"name\": \"YLL019C (pp) YIL113W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2071\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1970\", \"target_original\": \"1969\", \"target\": \"1706\", \"source\": \"1705\", \"shared_name\": \"YLL019C (pp) YIL113W\", \"SUID\": 2071}}, {\"selected\": false, \"data\": {\"id_original\": \"2298\", \"name\": \"YDR009W (pd) YGL035C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2070\", \"EdgeBetweenness\": 615.77575758, \"shared_interaction\": \"pd\", \"source_original\": \"1971\", \"target_original\": \"2109\", \"target\": \"1566\", \"source\": \"1704\", \"shared_name\": \"YDR009W (pd) YGL035C\", \"SUID\": 2070}}, {\"selected\": false, \"data\": {\"id_original\": \"2299\", \"name\": \"YML051W (pp) YDR009W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2069\", \"EdgeBetweenness\": 143.37575758, \"shared_interaction\": \"pp\", \"source_original\": \"1972\", \"target_original\": \"1971\", \"target\": \"1704\", \"source\": \"1703\", \"shared_name\": \"YML051W (pp) YDR009W\", \"SUID\": 2069}}, {\"selected\": false, \"data\": {\"id_original\": \"2300\", \"name\": \"YML051W (pp) YBR020W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2068\", \"EdgeBetweenness\": 135.97575758, \"shared_interaction\": \"pp\", \"source_original\": \"1972\", \"target_original\": \"2108\", \"target\": \"1567\", \"source\": \"1703\", \"shared_name\": \"YML051W (pp) YBR020W\", \"SUID\": 2068}}, {\"selected\": false, \"data\": {\"id_original\": \"2301\", \"name\": \"YPL031C (pp) YHR071W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2067\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1974\", \"target_original\": \"1973\", \"target\": \"1702\", \"source\": \"1701\", \"shared_name\": \"YPL031C (pp) YHR071W\", \"SUID\": 2067}}, {\"selected\": false, \"data\": {\"id_original\": \"2302\", \"name\": \"YML123C (pd) YFR034C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2066\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"1975\", \"target_original\": \"1990\", \"target\": \"1685\", \"source\": \"1700\", \"shared_name\": \"YML123C (pd) YFR034C\", \"SUID\": 2066}}, {\"selected\": false, \"data\": {\"id_original\": \"2303\", \"name\": \"YMR058W (pp) YER145C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2065\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"1977\", \"target_original\": \"1976\", \"target\": \"1699\", \"source\": \"1698\", \"shared_name\": \"YMR058W (pp) YER145C\", \"SUID\": 2065}}, {\"selected\": false, \"data\": {\"id_original\": \"2304\", \"name\": \"YML074C (pp) YJL190C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2064\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"1979\", \"target_original\": \"1978\", \"target\": \"1697\", \"source\": \"1696\", \"shared_name\": \"YML074C (pp) YJL190C\", \"SUID\": 2064}}, {\"selected\": false, \"data\": {\"id_original\": \"2305\", \"name\": \"YOR355W (pp) YNL091W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2063\", \"EdgeBetweenness\": 2356.12454212, \"shared_interaction\": \"pp\", \"source_original\": \"1980\", \"target_original\": \"2100\", \"target\": \"1575\", \"source\": \"1695\", \"shared_name\": \"YOR355W (pp) YNL091W\", \"SUID\": 2063}}, {\"selected\": false, \"data\": {\"id_original\": \"2306\", \"name\": \"YFL038C (pp) YOR036W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2062\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1981\", \"target_original\": \"2057\", \"target\": \"1618\", \"source\": \"1694\", \"shared_name\": \"YFL038C (pp) YOR036W\", \"SUID\": 2062}}, {\"selected\": false, \"data\": {\"id_original\": \"2307\", \"name\": \"YIL162W (pd) YNL167C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2061\", \"EdgeBetweenness\": 887.73076923, \"shared_interaction\": \"pd\", \"source_original\": \"1982\", \"target_original\": \"2001\", \"target\": \"1674\", \"source\": \"1693\", \"shared_name\": \"YIL162W (pd) YNL167C\", \"SUID\": 2061}}, {\"selected\": false, \"data\": {\"id_original\": \"2308\", \"name\": \"YER133W (pp) YBR050C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2060\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1987\", \"target_original\": \"1983\", \"target\": \"1692\", \"source\": \"1688\", \"shared_name\": \"YER133W (pp) YBR050C\", \"SUID\": 2060}}, {\"selected\": false, \"data\": {\"id_original\": \"2309\", \"name\": \"YER133W (pp) YMR311C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2059\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1987\", \"target_original\": \"1984\", \"target\": \"1691\", \"source\": \"1688\", \"shared_name\": \"YER133W (pp) YMR311C\", \"SUID\": 2059}}, {\"selected\": false, \"data\": {\"id_original\": \"2310\", \"name\": \"YER133W (pp) YOR315W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2058\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1987\", \"target_original\": \"1985\", \"target\": \"1690\", \"source\": \"1688\", \"shared_name\": \"YER133W (pp) YOR315W\", \"SUID\": 2058}}, {\"selected\": false, \"data\": {\"id_original\": \"2311\", \"name\": \"YER133W (pp) YOR178C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2057\", \"EdgeBetweenness\": 7242.0, \"shared_interaction\": \"pp\", \"source_original\": \"1987\", \"target_original\": \"1986\", \"target\": \"1689\", \"source\": \"1688\", \"shared_name\": \"YER133W (pp) YOR178C\", \"SUID\": 2057}}, {\"selected\": false, \"data\": {\"id_original\": \"2312\", \"name\": \"YER133W (pp) YDR412W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2056\", \"EdgeBetweenness\": 11988.0, \"shared_interaction\": \"pp\", \"source_original\": \"1987\", \"target_original\": \"2141\", \"target\": \"1534\", \"source\": \"1688\", \"shared_name\": \"YER133W (pp) YDR412W\", \"SUID\": 2056}}, {\"selected\": false, \"data\": {\"id_original\": \"2313\", \"name\": \"YFR037C (pp) YOR290C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2055\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"1989\", \"target_original\": \"1988\", \"target\": \"1687\", \"source\": \"1686\", \"shared_name\": \"YFR037C (pp) YOR290C\", \"SUID\": 2055}}, {\"selected\": false, \"data\": {\"id_original\": \"2314\", \"name\": \"YFR034C (pd) YBR093C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2054\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pd\", \"source_original\": \"1990\", \"target_original\": \"2152\", \"target\": \"1523\", \"source\": \"1685\", \"shared_name\": \"YFR034C (pd) YBR093C\", \"SUID\": 2054}}, {\"selected\": false, \"data\": {\"id_original\": \"2315\", \"name\": \"YAL040C (pd) YMR043W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2053\", \"EdgeBetweenness\": 381.0, \"shared_interaction\": \"pd\", \"source_original\": \"1991\", \"target_original\": \"2076\", \"target\": \"1599\", \"source\": \"1684\", \"shared_name\": \"YAL040C (pd) YMR043W\", \"SUID\": 2053}}, {\"selected\": false, \"data\": {\"id_original\": \"2316\", \"name\": \"YGR048W (pp) YPL222W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2052\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"1993\", \"target_original\": \"1992\", \"target\": \"1683\", \"source\": \"1682\", \"shared_name\": \"YGR048W (pp) YPL222W\", \"SUID\": 2052}}, {\"selected\": false, \"data\": {\"id_original\": \"2317\", \"name\": \"YMR291W (pp) YGL115W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2051\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"1994\", \"target_original\": \"2050\", \"target\": \"1625\", \"source\": \"1681\", \"shared_name\": \"YMR291W (pp) YGL115W\", \"SUID\": 2051}}, {\"selected\": false, \"data\": {\"id_original\": \"2318\", \"name\": \"YGR009C (pp) YBL050W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2050\", \"EdgeBetweenness\": 5785.28291708, \"shared_interaction\": \"pp\", \"source_original\": \"1995\", \"target_original\": \"1920\", \"target\": \"1755\", \"source\": \"1680\", \"shared_name\": \"YGR009C (pp) YBL050W\", \"SUID\": 2050}}, {\"selected\": false, \"data\": {\"id_original\": \"2319\", \"name\": \"YGR009C (pp) YDR335W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2049\", \"EdgeBetweenness\": 4025.28291708, \"shared_interaction\": \"pp\", \"source_original\": \"1995\", \"target_original\": \"2041\", \"target\": \"1634\", \"source\": \"1680\", \"shared_name\": \"YGR009C (pp) YDR335W\", \"SUID\": 2049}}, {\"selected\": false, \"data\": {\"id_original\": \"2320\", \"name\": \"YGR009C (pp) YOR327C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2048\", \"EdgeBetweenness\": 739.0, \"shared_interaction\": \"pp\", \"source_original\": \"1995\", \"target_original\": \"2144\", \"target\": \"1531\", \"source\": \"1680\", \"shared_name\": \"YGR009C (pp) YOR327C\", \"SUID\": 2048}}, {\"selected\": false, \"data\": {\"id_original\": \"2321\", \"name\": \"YGR009C (pp) YAL030W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2047\", \"EdgeBetweenness\": 739.0, \"shared_interaction\": \"pp\", \"source_original\": \"1995\", \"target_original\": \"2146\", \"target\": \"1529\", \"source\": \"1680\", \"shared_name\": \"YGR009C (pp) YAL030W\", \"SUID\": 2047}}, {\"selected\": false, \"data\": {\"id_original\": \"2322\", \"name\": \"YMR183C (pp) YGR009C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2046\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"1996\", \"target_original\": \"1995\", \"target\": \"1680\", \"source\": \"1679\", \"shared_name\": \"YMR183C (pp) YGR009C\", \"SUID\": 2046}}, {\"selected\": false, \"data\": {\"id_original\": \"2323\", \"name\": \"YGL161C (pp) YDR100W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2045\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"1998\", \"target_original\": \"1997\", \"target\": \"1678\", \"source\": \"1677\", \"shared_name\": \"YGL161C (pp) YDR100W\", \"SUID\": 2045}}, {\"selected\": false, \"data\": {\"id_original\": \"2324\", \"name\": \"YDL063C (pp) YPL131W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2044\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"2000\", \"target_original\": \"1999\", \"target\": \"1676\", \"source\": \"1675\", \"shared_name\": \"YDL063C (pp) YPL131W\", \"SUID\": 2044}}, {\"selected\": false, \"data\": {\"id_original\": \"2325\", \"name\": \"YNL167C (pd) YOR202W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2043\", \"EdgeBetweenness\": 611.26923077, \"shared_interaction\": \"pd\", \"source_original\": \"2001\", \"target_original\": \"2123\", \"target\": \"1552\", \"source\": \"1674\", \"shared_name\": \"YNL167C (pd) YOR202W\", \"SUID\": 2043}}, {\"selected\": false, \"data\": {\"id_original\": \"2326\", \"name\": \"YHR115C (pp) YOR215C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2042\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"2002\", \"target_original\": \"1889\", \"target\": \"1786\", \"source\": \"1673\", \"shared_name\": \"YHR115C (pp) YOR215C\", \"SUID\": 2042}}, {\"selected\": false, \"data\": {\"id_original\": \"2327\", \"name\": \"YEL041W (pp) YHR115C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2041\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"2003\", \"target_original\": \"2002\", \"target\": \"1673\", \"source\": \"1672\", \"shared_name\": \"YEL041W (pp) YHR115C\", \"SUID\": 2041}}, {\"selected\": false, \"data\": {\"id_original\": \"2328\", \"name\": \"YJL036W (pp) YDL113C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2040\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"2005\", \"target_original\": \"2004\", \"target\": \"1671\", \"source\": \"1670\", \"shared_name\": \"YJL036W (pp) YDL113C\", \"SUID\": 2040}}, {\"selected\": false, \"data\": {\"id_original\": \"2329\", \"name\": \"YBR109C (pp) YFR014C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2039\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"2006\", \"target_original\": \"1881\", \"target\": \"1794\", \"source\": \"1669\", \"shared_name\": \"YBR109C (pp) YFR014C\", \"SUID\": 2039}}, {\"selected\": false, \"data\": {\"id_original\": \"2330\", \"name\": \"YBR109C (pp) YOR326W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2038\", \"EdgeBetweenness\": 12.0, \"shared_interaction\": \"pp\", \"source_original\": \"2006\", \"target_original\": \"2129\", \"target\": \"1546\", \"source\": \"1669\", \"shared_name\": \"YBR109C (pp) YOR326W\", \"SUID\": 2038}}, {\"selected\": false, \"data\": {\"id_original\": \"2331\", \"name\": \"YOL016C (pp) YBR109C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2037\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"2007\", \"target_original\": \"2006\", \"target\": \"1669\", \"source\": \"1668\", \"shared_name\": \"YOL016C (pp) YBR109C\", \"SUID\": 2037}}, {\"selected\": false, \"data\": {\"id_original\": \"2332\", \"name\": \"YNL311C (pp) YKL001C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2036\", \"EdgeBetweenness\": 6.0, \"shared_interaction\": \"pp\", \"source_original\": \"2009\", \"target_original\": \"2008\", \"target\": \"1667\", \"source\": \"1666\", \"shared_name\": \"YNL311C (pp) YKL001C\", \"SUID\": 2036}}, {\"selected\": false, \"data\": {\"id_original\": \"2333\", \"name\": \"YLR319C (pp) YLR362W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2035\", \"EdgeBetweenness\": 4592.87952048, \"shared_interaction\": \"pp\", \"source_original\": \"2010\", \"target_original\": \"2114\", \"target\": \"1561\", \"source\": \"1665\", \"shared_name\": \"YLR319C (pp) YLR362W\", \"SUID\": 2035}}, {\"selected\": false, \"data\": {\"id_original\": \"2334\", \"name\": \"YNL189W (pp) YPR062W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2034\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2014\", \"target_original\": \"2011\", \"target\": \"1664\", \"source\": \"1661\", \"shared_name\": \"YNL189W (pp) YPR062W\", \"SUID\": 2034}}, {\"selected\": false, \"data\": {\"id_original\": \"2335\", \"name\": \"YNL189W (pp) YER065C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2033\", \"EdgeBetweenness\": 2598.55641026, \"shared_interaction\": \"pp\", \"source_original\": \"2014\", \"target_original\": \"2045\", \"target\": \"1630\", \"source\": \"1661\", \"shared_name\": \"YNL189W (pp) YER065C\", \"SUID\": 2033}}, {\"selected\": false, \"data\": {\"id_original\": \"2336\", \"name\": \"YNL189W (pp) YPL111W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2032\", \"EdgeBetweenness\": 1960.0, \"shared_interaction\": \"pp\", \"source_original\": \"2014\", \"target_original\": \"2012\", \"target\": \"1663\", \"source\": \"1661\", \"shared_name\": \"YNL189W (pp) YPL111W\", \"SUID\": 2032}}, {\"selected\": false, \"data\": {\"id_original\": \"2337\", \"name\": \"YNL189W (pp) YDL236W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2031\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2014\", \"target_original\": \"2013\", \"target\": \"1662\", \"source\": \"1661\", \"shared_name\": \"YNL189W (pp) YDL236W\", \"SUID\": 2031}}, {\"selected\": false, \"data\": {\"id_original\": \"2338\", \"name\": \"YBL069W (pp) YGL008C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2030\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2015\", \"target_original\": \"2056\", \"target\": \"1619\", \"source\": \"1660\", \"shared_name\": \"YBL069W (pp) YGL008C\", \"SUID\": 2030}}, {\"selected\": false, \"data\": {\"id_original\": \"2339\", \"name\": \"YGL073W (pd) YER103W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2029\", \"EdgeBetweenness\": 1476.0, \"shared_interaction\": \"pd\", \"source_original\": \"2016\", \"target_original\": \"1959\", \"target\": \"1716\", \"source\": \"1659\", \"shared_name\": \"YGL073W (pd) YER103W\", \"SUID\": 2029}}, {\"selected\": false, \"data\": {\"id_original\": \"2340\", \"name\": \"YGL073W (pd) YHR055C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2028\", \"EdgeBetweenness\": 981.0, \"shared_interaction\": \"pd\", \"source_original\": \"2016\", \"target_original\": \"1925\", \"target\": \"1750\", \"source\": \"1659\", \"shared_name\": \"YGL073W (pd) YHR055C\", \"SUID\": 2028}}, {\"selected\": false, \"data\": {\"id_original\": \"2341\", \"name\": \"YGL073W (pd) YHR053C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2027\", \"EdgeBetweenness\": 981.0, \"shared_interaction\": \"pd\", \"source_original\": \"2016\", \"target_original\": \"1926\", \"target\": \"1749\", \"source\": \"1659\", \"shared_name\": \"YGL073W (pd) YHR053C\", \"SUID\": 2027}}, {\"selected\": false, \"data\": {\"id_original\": \"2342\", \"name\": \"YGL073W (pp) YOR178C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2026\", \"EdgeBetweenness\": 4320.0, \"shared_interaction\": \"pp\", \"source_original\": \"2016\", \"target_original\": \"1986\", \"target\": \"1689\", \"source\": \"1659\", \"shared_name\": \"YGL073W (pp) YOR178C\", \"SUID\": 2026}}, {\"selected\": false, \"data\": {\"id_original\": \"2343\", \"name\": \"YBR072W (pd) YGL073W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2025\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2017\", \"target_original\": \"2016\", \"target\": \"1659\", \"source\": \"1658\", \"shared_name\": \"YBR072W (pd) YGL073W\", \"SUID\": 2025}}, {\"selected\": false, \"data\": {\"id_original\": \"2344\", \"name\": \"YLR321C (pp) YDR412W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2024\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2018\", \"target_original\": \"2141\", \"target\": \"1534\", \"source\": \"1657\", \"shared_name\": \"YLR321C (pp) YDR412W\", \"SUID\": 2024}}, {\"selected\": false, \"data\": {\"id_original\": \"2345\", \"name\": \"YPR048W (pp) YDL215C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2023\", \"EdgeBetweenness\": 3388.0, \"shared_interaction\": \"pp\", \"source_original\": \"2019\", \"target_original\": \"1909\", \"target\": \"1766\", \"source\": \"1656\", \"shared_name\": \"YPR048W (pp) YDL215C\", \"SUID\": 2023}}, {\"selected\": false, \"data\": {\"id_original\": \"2346\", \"name\": \"YPR048W (pp) YOR355W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2022\", \"EdgeBetweenness\": 2162.02930403, \"shared_interaction\": \"pp\", \"source_original\": \"2019\", \"target_original\": \"1980\", \"target\": \"1695\", \"source\": \"1656\", \"shared_name\": \"YPR048W (pp) YOR355W\", \"SUID\": 2022}}, {\"selected\": false, \"data\": {\"id_original\": \"2347\", \"name\": \"YNL199C (pp) YPR048W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2021\", \"EdgeBetweenness\": 3624.18315018, \"shared_interaction\": \"pp\", \"source_original\": \"2020\", \"target_original\": \"2019\", \"target\": \"1656\", \"source\": \"1655\", \"shared_name\": \"YNL199C (pp) YPR048W\", \"SUID\": 2021}}, {\"selected\": false, \"data\": {\"id_original\": \"2348\", \"name\": \"YPL075W (pd) YOL086C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2020\", \"EdgeBetweenness\": 1846.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2021\", \"target_original\": \"2147\", \"target\": \"1528\", \"source\": \"1654\", \"shared_name\": \"YPL075W (pd) YOL086C\", \"SUID\": 2020}}, {\"selected\": false, \"data\": {\"id_original\": \"2349\", \"name\": \"YPL075W (pd) YDR050C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2019\", \"EdgeBetweenness\": 1846.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2021\", \"target_original\": \"2148\", \"target\": \"1527\", \"source\": \"1654\", \"shared_name\": \"YPL075W (pd) YDR050C\", \"SUID\": 2019}}, {\"selected\": false, \"data\": {\"id_original\": \"2350\", \"name\": \"YPL075W (pp) YNL199C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2018\", \"EdgeBetweenness\": 11094.32880453, \"shared_interaction\": \"pp\", \"source_original\": \"2021\", \"target_original\": \"2020\", \"target\": \"1655\", \"source\": \"1654\", \"shared_name\": \"YPL075W (pp) YNL199C\", \"SUID\": 2018}}, {\"selected\": false, \"data\": {\"id_original\": \"2351\", \"name\": \"YPL075W (pd) YHR174W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2017\", \"EdgeBetweenness\": 1846.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2021\", \"target_original\": \"2159\", \"target\": \"1516\", \"source\": \"1654\", \"shared_name\": \"YPL075W (pd) YHR174W\", \"SUID\": 2017}}, {\"selected\": false, \"data\": {\"id_original\": \"2352\", \"name\": \"YPL075W (pd) YGR254W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2016\", \"EdgeBetweenness\": 1846.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2021\", \"target_original\": \"2160\", \"target\": \"1515\", \"source\": \"1654\", \"shared_name\": \"YPL075W (pd) YGR254W\", \"SUID\": 2016}}, {\"selected\": false, \"data\": {\"id_original\": \"2353\", \"name\": \"YPL075W (pd) YCR012W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2015\", \"EdgeBetweenness\": 2034.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2021\", \"target_original\": \"2161\", \"target\": \"1514\", \"source\": \"1654\", \"shared_name\": \"YPL075W (pd) YCR012W\", \"SUID\": 2015}}, {\"selected\": false, \"data\": {\"id_original\": \"2354\", \"name\": \"YFL039C (pp) YHR179W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2014\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"2024\", \"target_original\": \"2022\", \"target\": \"1653\", \"source\": \"1651\", \"shared_name\": \"YFL039C (pp) YHR179W\", \"SUID\": 2014}}, {\"selected\": false, \"data\": {\"id_original\": \"2355\", \"name\": \"YFL039C (pp) YCL040W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2013\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"2024\", \"target_original\": \"2023\", \"target\": \"1652\", \"source\": \"1651\", \"shared_name\": \"YFL039C (pp) YCL040W\", \"SUID\": 2013}}, {\"selected\": false, \"data\": {\"id_original\": \"2356\", \"name\": \"YDR382W (pp) YFL039C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2012\", \"EdgeBetweenness\": 12.0, \"shared_interaction\": \"pp\", \"source_original\": \"2026\", \"target_original\": \"2024\", \"target\": \"1651\", \"source\": \"1649\", \"shared_name\": \"YDR382W (pp) YFL039C\", \"SUID\": 2012}}, {\"selected\": false, \"data\": {\"id_original\": \"2357\", \"name\": \"YDR382W (pp) YDL130W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2011\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"2026\", \"target_original\": \"2025\", \"target\": \"1650\", \"source\": \"1649\", \"shared_name\": \"YDR382W (pp) YDL130W\", \"SUID\": 2011}}, {\"selected\": false, \"data\": {\"id_original\": \"2358\", \"name\": \"YJR066W (pp) YLR116W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2010\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2027\", \"target_original\": \"2095\", \"target\": \"1580\", \"source\": \"1648\", \"shared_name\": \"YJR066W (pp) YLR116W\", \"SUID\": 2010}}, {\"selected\": false, \"data\": {\"id_original\": \"2359\", \"name\": \"? (pd) YGL035C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2009\", \"EdgeBetweenness\": 2608.54053724, \"shared_interaction\": \"pd\", \"source_original\": \"2028\", \"target_original\": \"2109\", \"target\": \"1566\", \"source\": \"1647\", \"shared_name\": \"? (pd) YGL035C\", \"SUID\": 2009}}, {\"selected\": false, \"data\": {\"id_original\": \"2360\", \"name\": \"? (pd) YKR099W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"2008\", \"EdgeBetweenness\": 3465.75265845, \"shared_interaction\": \"pd\", \"source_original\": \"2028\", \"target_original\": \"2103\", \"target\": \"1572\", \"source\": \"1647\", \"shared_name\": \"? (pd) YKR099W\", \"SUID\": 2008}}, {\"selected\": false, \"data\": {\"id_original\": \"2361\", \"name\": \"YNL154C (pp) YKL204W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2007\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"2030\", \"target_original\": \"2029\", \"target\": \"1646\", \"source\": \"1645\", \"shared_name\": \"YNL154C (pp) YKL204W\", \"SUID\": 2007}}, {\"selected\": false, \"data\": {\"id_original\": \"2362\", \"name\": \"YNL047C (pp) YIL105C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2006\", \"EdgeBetweenness\": 2031.91544567, \"shared_interaction\": \"pp\", \"source_original\": \"2031\", \"target_original\": \"2132\", \"target\": \"1543\", \"source\": \"1644\", \"shared_name\": \"YNL047C (pp) YIL105C\", \"SUID\": 2006}}, {\"selected\": false, \"data\": {\"id_original\": \"2363\", \"name\": \"YHR135C (pp) YNL116W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2005\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"2033\", \"target_original\": \"2032\", \"target\": \"1643\", \"source\": \"1642\", \"shared_name\": \"YHR135C (pp) YNL116W\", \"SUID\": 2005}}, {\"selected\": false, \"data\": {\"id_original\": \"2364\", \"name\": \"YML064C (pp) YDR174W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2004\", \"EdgeBetweenness\": 3145.71148851, \"shared_interaction\": \"pp\", \"source_original\": \"2034\", \"target_original\": \"2040\", \"target\": \"1635\", \"source\": \"1641\", \"shared_name\": \"YML064C (pp) YDR174W\", \"SUID\": 2004}}, {\"selected\": false, \"data\": {\"id_original\": \"2365\", \"name\": \"YML064C (pp) YLR284C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2003\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2034\", \"target_original\": \"1930\", \"target\": \"1745\", \"source\": \"1641\", \"shared_name\": \"YML064C (pp) YLR284C\", \"SUID\": 2003}}, {\"selected\": false, \"data\": {\"id_original\": \"2366\", \"name\": \"YML064C (pp) YHR198C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2002\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2034\", \"target_original\": \"1931\", \"target\": \"1744\", \"source\": \"1641\", \"shared_name\": \"YML064C (pp) YHR198C\", \"SUID\": 2002}}, {\"selected\": false, \"data\": {\"id_original\": \"2367\", \"name\": \"YKL074C (pp) YGL035C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2001\", \"EdgeBetweenness\": 2916.0, \"shared_interaction\": \"pp\", \"source_original\": \"2035\", \"target_original\": \"2109\", \"target\": \"1566\", \"source\": \"1640\", \"shared_name\": \"YKL074C (pp) YGL035C\", \"SUID\": 2001}}, {\"selected\": false, \"data\": {\"id_original\": \"2368\", \"name\": \"YDL081C (pp) YLR340W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"2000\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"2037\", \"target_original\": \"2036\", \"target\": \"1639\", \"source\": \"1638\", \"shared_name\": \"YDL081C (pp) YLR340W\", \"SUID\": 2000}}, {\"selected\": false, \"data\": {\"id_original\": \"2369\", \"name\": \"YGL166W (pd) YHR055C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1999\", \"EdgeBetweenness\": 495.0, \"shared_interaction\": \"pd\", \"source_original\": \"2038\", \"target_original\": \"1925\", \"target\": \"1750\", \"source\": \"1637\", \"shared_name\": \"YGL166W (pd) YHR055C\", \"SUID\": 1999}}, {\"selected\": false, \"data\": {\"id_original\": \"2370\", \"name\": \"YGL166W (pd) YHR053C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1998\", \"EdgeBetweenness\": 495.0, \"shared_interaction\": \"pd\", \"source_original\": \"2038\", \"target_original\": \"1926\", \"target\": \"1749\", \"source\": \"1637\", \"shared_name\": \"YGL166W (pd) YHR053C\", \"SUID\": 1998}}, {\"selected\": false, \"data\": {\"id_original\": \"2371\", \"name\": \"YLL028W (pp) YGL166W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1997\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2039\", \"target_original\": \"2038\", \"target\": \"1637\", \"source\": \"1636\", \"shared_name\": \"YLL028W (pp) YGL166W\", \"SUID\": 1997}}, {\"selected\": false, \"data\": {\"id_original\": \"2372\", \"name\": \"YDR335W (pp) YDR174W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1996\", \"EdgeBetweenness\": 3733.28291708, \"shared_interaction\": \"pp\", \"source_original\": \"2041\", \"target_original\": \"2040\", \"target\": \"1635\", \"source\": \"1634\", \"shared_name\": \"YDR335W (pp) YDR174W\", \"SUID\": 1996}}, {\"selected\": false, \"data\": {\"id_original\": \"2373\", \"name\": \"YMR021C (pd) YLR214W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1995\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pd\", \"source_original\": \"2043\", \"target_original\": \"2042\", \"target\": \"1633\", \"source\": \"1632\", \"shared_name\": \"YMR021C (pd) YLR214W\", \"SUID\": 1995}}, {\"selected\": false, \"data\": {\"id_original\": \"2374\", \"name\": \"YJL089W (pd) YLR377C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1994\", \"EdgeBetweenness\": 1271.90164835, \"shared_interaction\": \"pd\", \"source_original\": \"2046\", \"target_original\": \"2044\", \"target\": \"1631\", \"source\": \"1629\", \"shared_name\": \"YJL089W (pd) YLR377C\", \"SUID\": 1994}}, {\"selected\": false, \"data\": {\"id_original\": \"2375\", \"name\": \"YJL089W (pd) YKR097W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1993\", \"EdgeBetweenness\": 2924.57527473, \"shared_interaction\": \"pd\", \"source_original\": \"2046\", \"target_original\": \"2073\", \"target\": \"1602\", \"source\": \"1629\", \"shared_name\": \"YJL089W (pd) YKR097W\", \"SUID\": 1993}}, {\"selected\": false, \"data\": {\"id_original\": \"2376\", \"name\": \"YJL089W (pd) YER065C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1992\", \"EdgeBetweenness\": 2882.71025641, \"shared_interaction\": \"pd\", \"source_original\": \"2046\", \"target_original\": \"2045\", \"target\": \"1630\", \"source\": \"1629\", \"shared_name\": \"YJL089W (pd) YER065C\", \"SUID\": 1992}}, {\"selected\": false, \"data\": {\"id_original\": \"2377\", \"name\": \"YHR030C (pp) YER111C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1991\", \"EdgeBetweenness\": 2101.73260073, \"shared_interaction\": \"pp\", \"source_original\": \"2047\", \"target_original\": \"1966\", \"target\": \"1709\", \"source\": \"1628\", \"shared_name\": \"YHR030C (pp) YER111C\", \"SUID\": 1991}}, {\"selected\": false, \"data\": {\"id_original\": \"2378\", \"name\": \"YHR030C (pp) YLL021W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1990\", \"EdgeBetweenness\": 1104.11355311, \"shared_interaction\": \"pp\", \"source_original\": \"2047\", \"target_original\": \"2064\", \"target\": \"1611\", \"source\": \"1628\", \"shared_name\": \"YHR030C (pp) YLL021W\", \"SUID\": 1990}}, {\"selected\": false, \"data\": {\"id_original\": \"2379\", \"name\": \"YPL089C (pp) YHR030C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1989\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2048\", \"target_original\": \"2047\", \"target\": \"1628\", \"source\": \"1627\", \"shared_name\": \"YPL089C (pp) YHR030C\", \"SUID\": 1989}}, {\"selected\": false, \"data\": {\"id_original\": \"2380\", \"name\": \"YGL115W (pp) YGL208W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1988\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"2050\", \"target_original\": \"2049\", \"target\": \"1626\", \"source\": \"1625\", \"shared_name\": \"YGL115W (pp) YGL208W\", \"SUID\": 1988}}, {\"selected\": false, \"data\": {\"id_original\": \"2381\", \"name\": \"YLR310C (pp) YER103W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1987\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2051\", \"target_original\": \"1959\", \"target\": \"1716\", \"source\": \"1624\", \"shared_name\": \"YLR310C (pp) YER103W\", \"SUID\": 1987}}, {\"selected\": false, \"data\": {\"id_original\": \"2382\", \"name\": \"YNL098C (pp) YLR310C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1986\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2052\", \"target_original\": \"2051\", \"target\": \"1624\", \"source\": \"1623\", \"shared_name\": \"YNL098C (pp) YLR310C\", \"SUID\": 1986}}, {\"selected\": false, \"data\": {\"id_original\": \"2383\", \"name\": \"YER040W (pd) YGR019W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1985\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2055\", \"target_original\": \"2053\", \"target\": \"1622\", \"source\": \"1620\", \"shared_name\": \"YER040W (pd) YGR019W\", \"SUID\": 1985}}, {\"selected\": false, \"data\": {\"id_original\": \"2384\", \"name\": \"YER040W (pd) YPR035W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1984\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2055\", \"target_original\": \"2054\", \"target\": \"1621\", \"source\": \"1620\", \"shared_name\": \"YER040W (pd) YPR035W\", \"SUID\": 1984}}, {\"selected\": false, \"data\": {\"id_original\": \"2385\", \"name\": \"YGL008C (pd) YMR043W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1983\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pd\", \"source_original\": \"2056\", \"target_original\": \"2076\", \"target\": \"1599\", \"source\": \"1619\", \"shared_name\": \"YGL008C (pd) YMR043W\", \"SUID\": 1983}}, {\"selected\": false, \"data\": {\"id_original\": \"2386\", \"name\": \"YOR036W (pp) YGL161C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1982\", \"EdgeBetweenness\": 494.0, \"shared_interaction\": \"pp\", \"source_original\": \"2057\", \"target_original\": \"1998\", \"target\": \"1677\", \"source\": \"1618\", \"shared_name\": \"YOR036W (pp) YGL161C\", \"SUID\": 1982}}, {\"selected\": false, \"data\": {\"id_original\": \"2387\", \"name\": \"YOR036W (pp) YDR100W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1981\", \"EdgeBetweenness\": 494.0, \"shared_interaction\": \"pp\", \"source_original\": \"2057\", \"target_original\": \"1997\", \"target\": \"1678\", \"source\": \"1618\", \"shared_name\": \"YOR036W (pp) YDR100W\", \"SUID\": 1981}}, {\"selected\": false, \"data\": {\"id_original\": \"2388\", \"name\": \"YDR323C (pp) YOR036W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1980\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2058\", \"target_original\": \"2057\", \"target\": \"1618\", \"source\": \"1617\", \"shared_name\": \"YDR323C (pp) YOR036W\", \"SUID\": 1980}}, {\"selected\": false, \"data\": {\"id_original\": \"2389\", \"name\": \"YBL005W (pd) YJL219W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1979\", \"EdgeBetweenness\": 249.0, \"shared_interaction\": \"pd\", \"source_original\": \"2059\", \"target_original\": \"2063\", \"target\": \"1612\", \"source\": \"1616\", \"shared_name\": \"YBL005W (pd) YJL219W\", \"SUID\": 1979}}, {\"selected\": false, \"data\": {\"id_original\": \"2390\", \"name\": \"YBR160W (pp) YGR108W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1978\", \"EdgeBetweenness\": 48.0, \"shared_interaction\": \"pp\", \"source_original\": \"2060\", \"target_original\": \"2072\", \"target\": \"1603\", \"source\": \"1615\", \"shared_name\": \"YBR160W (pp) YGR108W\", \"SUID\": 1978}}, {\"selected\": false, \"data\": {\"id_original\": \"2391\", \"name\": \"YBR160W (pd) YMR043W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1977\", \"EdgeBetweenness\": 940.0, \"shared_interaction\": \"pd\", \"source_original\": \"2060\", \"target_original\": \"2076\", \"target\": \"1599\", \"source\": \"1615\", \"shared_name\": \"YBR160W (pd) YMR043W\", \"SUID\": 1977}}, {\"selected\": false, \"data\": {\"id_original\": \"2392\", \"name\": \"YKL101W (pp) YBR160W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1976\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2061\", \"target_original\": \"2060\", \"target\": \"1615\", \"source\": \"1614\", \"shared_name\": \"YKL101W (pp) YBR160W\", \"SUID\": 1976}}, {\"selected\": false, \"data\": {\"id_original\": \"2393\", \"name\": \"YOL156W (pd) YBL005W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1975\", \"EdgeBetweenness\": 249.0, \"shared_interaction\": \"pd\", \"source_original\": \"2062\", \"target_original\": \"2059\", \"target\": \"1616\", \"source\": \"1613\", \"shared_name\": \"YOL156W (pd) YBL005W\", \"SUID\": 1975}}, {\"selected\": false, \"data\": {\"id_original\": \"2394\", \"name\": \"YLL021W (pp) YLR362W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1974\", \"EdgeBetweenness\": 1026.26739927, \"shared_interaction\": \"pp\", \"source_original\": \"2064\", \"target_original\": \"2114\", \"target\": \"1561\", \"source\": \"1611\", \"shared_name\": \"YLL021W (pp) YLR362W\", \"SUID\": 1974}}, {\"selected\": false, \"data\": {\"id_original\": \"2395\", \"name\": \"YJL203W (pp) YOL136C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1973\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2066\", \"target_original\": \"2065\", \"target\": \"1610\", \"source\": \"1609\", \"shared_name\": \"YJL203W (pp) YOL136C\", \"SUID\": 1973}}, {\"selected\": false, \"data\": {\"id_original\": \"2396\", \"name\": \"YJL157C (pp) YOR212W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1972\", \"EdgeBetweenness\": 8680.0041292, \"shared_interaction\": \"pp\", \"source_original\": \"2069\", \"target_original\": \"1894\", \"target\": \"1781\", \"source\": \"1606\", \"shared_name\": \"YJL157C (pp) YOR212W\", \"SUID\": 1972}}, {\"selected\": false, \"data\": {\"id_original\": \"2397\", \"name\": \"YJL157C (pp) YAL040C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1971\", \"EdgeBetweenness\": 115.0, \"shared_interaction\": \"pp\", \"source_original\": \"2069\", \"target_original\": \"1991\", \"target\": \"1684\", \"source\": \"1606\", \"shared_name\": \"YJL157C (pp) YAL040C\", \"SUID\": 1971}}, {\"selected\": false, \"data\": {\"id_original\": \"2398\", \"name\": \"YNL145W (pd) YHR084W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1970\", \"EdgeBetweenness\": 4.83333333, \"shared_interaction\": \"pd\", \"source_original\": \"2070\", \"target_original\": \"1919\", \"target\": \"1756\", \"source\": \"1605\", \"shared_name\": \"YNL145W (pd) YHR084W\", \"SUID\": 1970}}, {\"selected\": false, \"data\": {\"id_original\": \"2399\", \"name\": \"YNL145W (pd) YCL067C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1969\", \"EdgeBetweenness\": 9.83333333, \"shared_interaction\": \"pd\", \"source_original\": \"2070\", \"target_original\": \"2175\", \"target\": \"1500\", \"source\": \"1605\", \"shared_name\": \"YNL145W (pd) YCL067C\", \"SUID\": 1969}}, {\"selected\": false, \"data\": {\"id_original\": \"2400\", \"name\": \"YGR108W (pp) YBR135W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1968\", \"EdgeBetweenness\": 3656.08455433, \"shared_interaction\": \"pp\", \"source_original\": \"2072\", \"target_original\": \"1948\", \"target\": \"1727\", \"source\": \"1603\", \"shared_name\": \"YGR108W (pp) YBR135W\", \"SUID\": 1968}}, {\"selected\": false, \"data\": {\"id_original\": \"2401\", \"name\": \"YMR043W (pd) YFL026W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1967\", \"EdgeBetweenness\": 484.33333333, \"shared_interaction\": \"pd\", \"source_original\": \"2076\", \"target_original\": \"2068\", \"target\": \"1607\", \"source\": \"1599\", \"shared_name\": \"YMR043W (pd) YFL026W\", \"SUID\": 1967}}, {\"selected\": false, \"data\": {\"id_original\": \"2402\", \"name\": \"YMR043W (pd) YJL157C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1966\", \"EdgeBetweenness\": 9243.86127206, \"shared_interaction\": \"pd\", \"source_original\": \"2076\", \"target_original\": \"2069\", \"target\": \"1606\", \"source\": \"1599\", \"shared_name\": \"YMR043W (pd) YJL157C\", \"SUID\": 1966}}, {\"selected\": false, \"data\": {\"id_original\": \"2403\", \"name\": \"YMR043W (pd) YNL145W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1965\", \"EdgeBetweenness\": 484.33333333, \"shared_interaction\": \"pd\", \"source_original\": \"2076\", \"target_original\": \"2070\", \"target\": \"1605\", \"source\": \"1599\", \"shared_name\": \"YMR043W (pd) YNL145W\", \"SUID\": 1965}}, {\"selected\": false, \"data\": {\"id_original\": \"2404\", \"name\": \"YMR043W (pd) YDR461W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1964\", \"EdgeBetweenness\": 484.33333333, \"shared_interaction\": \"pd\", \"source_original\": \"2076\", \"target_original\": \"2071\", \"target\": \"1604\", \"source\": \"1599\", \"shared_name\": \"YMR043W (pd) YDR461W\", \"SUID\": 1964}}, {\"selected\": false, \"data\": {\"id_original\": \"2405\", \"name\": \"YMR043W (pd) YGR108W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1963\", \"EdgeBetweenness\": 4058.08455433, \"shared_interaction\": \"pd\", \"source_original\": \"2076\", \"target_original\": \"2072\", \"target\": \"1603\", \"source\": \"1599\", \"shared_name\": \"YMR043W (pd) YGR108W\", \"SUID\": 1963}}, {\"selected\": false, \"data\": {\"id_original\": \"2406\", \"name\": \"YMR043W (pd) YKR097W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1962\", \"EdgeBetweenness\": 3136.17527473, \"shared_interaction\": \"pd\", \"source_original\": \"2076\", \"target_original\": \"2073\", \"target\": \"1602\", \"source\": \"1599\", \"shared_name\": \"YMR043W (pd) YKR097W\", \"SUID\": 1962}}, {\"selected\": false, \"data\": {\"id_original\": \"2407\", \"name\": \"YMR043W (pd) YJL159W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1961\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pd\", \"source_original\": \"2076\", \"target_original\": \"2074\", \"target\": \"1601\", \"source\": \"1599\", \"shared_name\": \"YMR043W (pd) YJL159W\", \"SUID\": 1961}}, {\"selected\": false, \"data\": {\"id_original\": \"2408\", \"name\": \"YMR043W (pd) YIL015W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1960\", \"EdgeBetweenness\": 487.0, \"shared_interaction\": \"pd\", \"source_original\": \"2076\", \"target_original\": \"2075\", \"target\": \"1600\", \"source\": \"1599\", \"shared_name\": \"YMR043W (pd) YIL015W\", \"SUID\": 1960}}, {\"selected\": false, \"data\": {\"id_original\": \"2409\", \"name\": \"YKL109W (pd) YGL035C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1959\", \"EdgeBetweenness\": 2540.66666667, \"shared_interaction\": \"pd\", \"source_original\": \"2077\", \"target_original\": \"2109\", \"target\": \"1566\", \"source\": \"1598\", \"shared_name\": \"YKL109W (pd) YGL035C\", \"SUID\": 1959}}, {\"selected\": false, \"data\": {\"id_original\": \"2410\", \"name\": \"YKL109W (pd) YJR048W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1958\", \"EdgeBetweenness\": 1185.8, \"shared_interaction\": \"pd\", \"source_original\": \"2077\", \"target_original\": \"2086\", \"target\": \"1589\", \"source\": \"1598\", \"shared_name\": \"YKL109W (pd) YJR048W\", \"SUID\": 1958}}, {\"selected\": false, \"data\": {\"id_original\": \"2411\", \"name\": \"YKL109W (pp) YBL021C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1957\", \"EdgeBetweenness\": 451.43333333, \"shared_interaction\": \"pp\", \"source_original\": \"2077\", \"target_original\": \"2083\", \"target\": \"1592\", \"source\": \"1598\", \"shared_name\": \"YKL109W (pp) YBL021C\", \"SUID\": 1957}}, {\"selected\": false, \"data\": {\"id_original\": \"2412\", \"name\": \"YKL109W (pp) YGL237C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1956\", \"EdgeBetweenness\": 451.43333333, \"shared_interaction\": \"pp\", \"source_original\": \"2077\", \"target_original\": \"2084\", \"target\": \"1591\", \"source\": \"1598\", \"shared_name\": \"YKL109W (pp) YGL237C\", \"SUID\": 1956}}, {\"selected\": false, \"data\": {\"id_original\": \"2413\", \"name\": \"YBR217W (pp) YNR007C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1955\", \"EdgeBetweenness\": 249.0, \"shared_interaction\": \"pp\", \"source_original\": \"2078\", \"target_original\": \"2067\", \"target\": \"1608\", \"source\": \"1597\", \"shared_name\": \"YBR217W (pp) YNR007C\", \"SUID\": 1955}}, {\"selected\": false, \"data\": {\"id_original\": \"2414\", \"name\": \"YHR171W (pp) YNR007C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1954\", \"EdgeBetweenness\": 739.0, \"shared_interaction\": \"pp\", \"source_original\": \"2079\", \"target_original\": \"2067\", \"target\": \"1608\", \"source\": \"1596\", \"shared_name\": \"YHR171W (pp) YNR007C\", \"SUID\": 1954}}, {\"selected\": false, \"data\": {\"id_original\": \"2415\", \"name\": \"YHR171W (pp) YDR412W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1953\", \"EdgeBetweenness\": 1960.0, \"shared_interaction\": \"pp\", \"source_original\": \"2079\", \"target_original\": \"2141\", \"target\": \"1534\", \"source\": \"1596\", \"shared_name\": \"YHR171W (pp) YDR412W\", \"SUID\": 1953}}, {\"selected\": false, \"data\": {\"id_original\": \"2416\", \"name\": \"YPL149W (pp) YBR217W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1952\", \"EdgeBetweenness\": 249.0, \"shared_interaction\": \"pp\", \"source_original\": \"2080\", \"target_original\": \"2078\", \"target\": \"1597\", \"source\": \"1595\", \"shared_name\": \"YPL149W (pp) YBR217W\", \"SUID\": 1952}}, {\"selected\": false, \"data\": {\"id_original\": \"2417\", \"name\": \"YPL149W (pp) YHR171W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1951\", \"EdgeBetweenness\": 739.0, \"shared_interaction\": \"pp\", \"source_original\": \"2080\", \"target_original\": \"2079\", \"target\": \"1596\", \"source\": \"1595\", \"shared_name\": \"YPL149W (pp) YHR171W\", \"SUID\": 1951}}, {\"selected\": false, \"data\": {\"id_original\": \"2418\", \"name\": \"YDR311W (pp) YKL028W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1950\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2082\", \"target_original\": \"2081\", \"target\": \"1594\", \"source\": \"1593\", \"shared_name\": \"YDR311W (pp) YKL028W\", \"SUID\": 1950}}, {\"selected\": false, \"data\": {\"id_original\": \"2419\", \"name\": \"YBL021C (pd) YJR048W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1949\", \"EdgeBetweenness\": 44.56666667, \"shared_interaction\": \"pd\", \"source_original\": \"2083\", \"target_original\": \"2086\", \"target\": \"1589\", \"source\": \"1592\", \"shared_name\": \"YBL021C (pd) YJR048W\", \"SUID\": 1949}}, {\"selected\": false, \"data\": {\"id_original\": \"2420\", \"name\": \"YGL237C (pd) YJR048W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1948\", \"EdgeBetweenness\": 44.56666667, \"shared_interaction\": \"pd\", \"source_original\": \"2084\", \"target_original\": \"2086\", \"target\": \"1589\", \"source\": \"1591\", \"shared_name\": \"YGL237C (pd) YJR048W\", \"SUID\": 1948}}, {\"selected\": false, \"data\": {\"id_original\": \"2421\", \"name\": \"YLR256W (pd) YEL039C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1947\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2088\", \"target_original\": \"2085\", \"target\": \"1590\", \"source\": \"1587\", \"shared_name\": \"YLR256W (pd) YEL039C\", \"SUID\": 1947}}, {\"selected\": false, \"data\": {\"id_original\": \"2422\", \"name\": \"YLR256W (pd) YJR048W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1946\", \"EdgeBetweenness\": 2440.0, \"shared_interaction\": \"pd\", \"source_original\": \"2088\", \"target_original\": \"2086\", \"target\": \"1589\", \"source\": \"1587\", \"shared_name\": \"YLR256W (pd) YJR048W\", \"SUID\": 1946}}, {\"selected\": false, \"data\": {\"id_original\": \"2423\", \"name\": \"YLR256W (pd) YML054C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1945\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pd\", \"source_original\": \"2088\", \"target_original\": \"2087\", \"target\": \"1588\", \"source\": \"1587\", \"shared_name\": \"YLR256W (pd) YML054C\", \"SUID\": 1945}}, {\"selected\": false, \"data\": {\"id_original\": \"2424\", \"name\": \"YJR109C (pp) YOR303W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1944\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"2090\", \"target_original\": \"2089\", \"target\": \"1586\", \"source\": \"1585\", \"shared_name\": \"YJR109C (pp) YOR303W\", \"SUID\": 1944}}, {\"selected\": false, \"data\": {\"id_original\": \"2425\", \"name\": \"YGR058W (pp) YOR264W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1943\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2091\", \"target_original\": \"2094\", \"target\": \"1581\", \"source\": \"1584\", \"shared_name\": \"YGR058W (pp) YOR264W\", \"SUID\": 1943}}, {\"selected\": false, \"data\": {\"id_original\": \"2426\", \"name\": \"YLR229C (pp) YJL157C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1942\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2092\", \"target_original\": \"2069\", \"target\": \"1606\", \"source\": \"1583\", \"shared_name\": \"YLR229C (pp) YJL157C\", \"SUID\": 1942}}, {\"selected\": false, \"data\": {\"id_original\": \"2427\", \"name\": \"YDR309C (pp) YLR229C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1941\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2093\", \"target_original\": \"2092\", \"target\": \"1583\", \"source\": \"1582\", \"shared_name\": \"YDR309C (pp) YLR229C\", \"SUID\": 1941}}, {\"selected\": false, \"data\": {\"id_original\": \"2428\", \"name\": \"YLR116W (pp) YKL012W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1940\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2095\", \"target_original\": \"2098\", \"target\": \"1577\", \"source\": \"1580\", \"shared_name\": \"YLR116W (pp) YKL012W\", \"SUID\": 1940}}, {\"selected\": false, \"data\": {\"id_original\": \"2429\", \"name\": \"YNL312W (pd) YPL111W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1939\", \"EdgeBetweenness\": 739.0, \"shared_interaction\": \"pd\", \"source_original\": \"2096\", \"target_original\": \"2012\", \"target\": \"1663\", \"source\": \"1579\", \"shared_name\": \"YNL312W (pd) YPL111W\", \"SUID\": 1939}}, {\"selected\": false, \"data\": {\"id_original\": \"2430\", \"name\": \"YML032C (pp) YNL312W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1938\", \"EdgeBetweenness\": 249.0, \"shared_interaction\": \"pp\", \"source_original\": \"2097\", \"target_original\": \"2096\", \"target\": \"1579\", \"source\": \"1578\", \"shared_name\": \"YML032C (pp) YNL312W\", \"SUID\": 1938}}, {\"selected\": false, \"data\": {\"id_original\": \"2431\", \"name\": \"YKL012W (pp) YKL074C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1937\", \"EdgeBetweenness\": 2440.0, \"shared_interaction\": \"pp\", \"source_original\": \"2098\", \"target_original\": \"2035\", \"target\": \"1640\", \"source\": \"1577\", \"shared_name\": \"YKL012W (pp) YKL074C\", \"SUID\": 1937}}, {\"selected\": false, \"data\": {\"id_original\": \"2432\", \"name\": \"YNL236W (pp) YKL012W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1936\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2099\", \"target_original\": \"2098\", \"target\": \"1577\", \"source\": \"1576\", \"shared_name\": \"YNL236W (pp) YKL012W\", \"SUID\": 1936}}, {\"selected\": false, \"data\": {\"id_original\": \"2433\", \"name\": \"YNL091W (pp) YNL164C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1935\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2100\", \"target_original\": \"1896\", \"target\": \"1779\", \"source\": \"1575\", \"shared_name\": \"YNL091W (pp) YNL164C\", \"SUID\": 1935}}, {\"selected\": false, \"data\": {\"id_original\": \"2434\", \"name\": \"YDR184C (pp) YLR319C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1934\", \"EdgeBetweenness\": 4543.54618715, \"shared_interaction\": \"pp\", \"source_original\": \"2101\", \"target_original\": \"2010\", \"target\": \"1665\", \"source\": \"1574\", \"shared_name\": \"YDR184C (pp) YLR319C\", \"SUID\": 1934}}, {\"selected\": false, \"data\": {\"id_original\": \"2435\", \"name\": \"YIL143C (pp) YDR311W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1933\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2102\", \"target_original\": \"2082\", \"target\": \"1593\", \"source\": \"1573\", \"shared_name\": \"YIL143C (pp) YDR311W\", \"SUID\": 1933}}, {\"selected\": false, \"data\": {\"id_original\": \"2436\", \"name\": \"YIR009W (pp) YNL091W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1932\", \"EdgeBetweenness\": 2992.12454212, \"shared_interaction\": \"pp\", \"source_original\": \"2104\", \"target_original\": \"2100\", \"target\": \"1575\", \"source\": \"1571\", \"shared_name\": \"YIR009W (pp) YNL091W\", \"SUID\": 1932}}, {\"selected\": false, \"data\": {\"id_original\": \"2437\", \"name\": \"YIR009W (pp) YDR184C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1931\", \"EdgeBetweenness\": 4563.54618715, \"shared_interaction\": \"pp\", \"source_original\": \"2104\", \"target_original\": \"2101\", \"target\": \"1574\", \"source\": \"1571\", \"shared_name\": \"YIR009W (pp) YDR184C\", \"SUID\": 1931}}, {\"selected\": false, \"data\": {\"id_original\": \"2438\", \"name\": \"YIR009W (pp) YIL143C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1930\", \"EdgeBetweenness\": 1476.0, \"shared_interaction\": \"pp\", \"source_original\": \"2104\", \"target_original\": \"2102\", \"target\": \"1573\", \"source\": \"1571\", \"shared_name\": \"YIR009W (pp) YIL143C\", \"SUID\": 1930}}, {\"selected\": false, \"data\": {\"id_original\": \"2439\", \"name\": \"YIR009W (pp) YKR099W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1929\", \"EdgeBetweenness\": 5834.22017982, \"shared_interaction\": \"pp\", \"source_original\": \"2104\", \"target_original\": \"2103\", \"target\": \"1572\", \"source\": \"1571\", \"shared_name\": \"YIR009W (pp) YKR099W\", \"SUID\": 1929}}, {\"selected\": false, \"data\": {\"id_original\": \"2440\", \"name\": \"YPL248C (pd) YBR018C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1928\", \"EdgeBetweenness\": 491.0, \"shared_interaction\": \"pd\", \"source_original\": \"2106\", \"target_original\": \"2105\", \"target\": \"1570\", \"source\": \"1569\", \"shared_name\": \"YPL248C (pd) YBR018C\", \"SUID\": 1928}}, {\"selected\": false, \"data\": {\"id_original\": \"2441\", \"name\": \"YPL248C (pd) YLR081W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1927\", \"EdgeBetweenness\": 491.0, \"shared_interaction\": \"pd\", \"source_original\": \"2106\", \"target_original\": \"2107\", \"target\": \"1568\", \"source\": \"1569\", \"shared_name\": \"YPL248C (pd) YLR081W\", \"SUID\": 1927}}, {\"selected\": false, \"data\": {\"id_original\": \"2442\", \"name\": \"YPL248C (pd) YBR020W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1926\", \"EdgeBetweenness\": 38.23333333, \"shared_interaction\": \"pd\", \"source_original\": \"2106\", \"target_original\": \"2108\", \"target\": \"1567\", \"source\": \"1569\", \"shared_name\": \"YPL248C (pd) YBR020W\", \"SUID\": 1926}}, {\"selected\": false, \"data\": {\"id_original\": \"2443\", \"name\": \"YPL248C (pp) YML051W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1925\", \"EdgeBetweenness\": 236.24848485, \"shared_interaction\": \"pp\", \"source_original\": \"2106\", \"target_original\": \"1972\", \"target\": \"1703\", \"source\": \"1569\", \"shared_name\": \"YPL248C (pp) YML051W\", \"SUID\": 1925}}, {\"selected\": false, \"data\": {\"id_original\": \"2444\", \"name\": \"YPL248C (pd) YML051W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1924\", \"EdgeBetweenness\": 236.24848485, \"shared_interaction\": \"pd\", \"source_original\": \"2106\", \"target_original\": \"1972\", \"target\": \"1703\", \"source\": \"1569\", \"shared_name\": \"YPL248C (pd) YML051W\", \"SUID\": 1924}}, {\"selected\": false, \"data\": {\"id_original\": \"2445\", \"name\": \"YPL248C (pd) YGL035C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1923\", \"EdgeBetweenness\": 2953.35151515, \"shared_interaction\": \"pd\", \"source_original\": \"2106\", \"target_original\": \"2109\", \"target\": \"1566\", \"source\": \"1569\", \"shared_name\": \"YPL248C (pd) YGL035C\", \"SUID\": 1923}}, {\"selected\": false, \"data\": {\"id_original\": \"2446\", \"name\": \"YPL248C (pd) YJR048W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1922\", \"EdgeBetweenness\": 1779.33333333, \"shared_interaction\": \"pd\", \"source_original\": \"2106\", \"target_original\": \"2086\", \"target\": \"1589\", \"source\": \"1569\", \"shared_name\": \"YPL248C (pd) YJR048W\", \"SUID\": 1922}}, {\"selected\": false, \"data\": {\"id_original\": \"2447\", \"name\": \"YPL248C (pd) ?\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1921\", \"EdgeBetweenness\": 985.21212121, \"shared_interaction\": \"pd\", \"source_original\": \"2106\", \"target_original\": \"2028\", \"target\": \"1647\", \"source\": \"1569\", \"shared_name\": \"YPL248C (pd) ?\", \"SUID\": 1921}}, {\"selected\": false, \"data\": {\"id_original\": \"2448\", \"name\": \"YPL248C (pd) YBR019C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1920\", \"EdgeBetweenness\": 40.23333333, \"shared_interaction\": \"pd\", \"source_original\": \"2106\", \"target_original\": \"2111\", \"target\": \"1564\", \"source\": \"1569\", \"shared_name\": \"YPL248C (pd) YBR019C\", \"SUID\": 1920}}, {\"selected\": false, \"data\": {\"id_original\": \"2449\", \"name\": \"YBR020W (pd) YGL035C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1919\", \"EdgeBetweenness\": 713.65151515, \"shared_interaction\": \"pd\", \"source_original\": \"2108\", \"target_original\": \"2109\", \"target\": \"1566\", \"source\": \"1567\", \"shared_name\": \"YBR020W (pd) YGL035C\", \"SUID\": 1919}}, {\"selected\": false, \"data\": {\"id_original\": \"2450\", \"name\": \"YGL035C (pd) YIL162W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1918\", \"EdgeBetweenness\": 1336.3974359, \"shared_interaction\": \"pd\", \"source_original\": \"2109\", \"target_original\": \"1982\", \"target\": \"1693\", \"source\": \"1566\", \"shared_name\": \"YGL035C (pd) YIL162W\", \"SUID\": 1918}}, {\"selected\": false, \"data\": {\"id_original\": \"2451\", \"name\": \"YGL035C (pd) YLR377C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1917\", \"EdgeBetweenness\": 1488.73498168, \"shared_interaction\": \"pd\", \"source_original\": \"2109\", \"target_original\": \"2044\", \"target\": \"1631\", \"source\": \"1566\", \"shared_name\": \"YGL035C (pd) YLR377C\", \"SUID\": 1917}}, {\"selected\": false, \"data\": {\"id_original\": \"2452\", \"name\": \"YGL035C (pd) YLR044C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1916\", \"EdgeBetweenness\": 15561.19504385, \"shared_interaction\": \"pd\", \"source_original\": \"2109\", \"target_original\": \"2157\", \"target\": \"1518\", \"source\": \"1566\", \"shared_name\": \"YGL035C (pd) YLR044C\", \"SUID\": 1916}}, {\"selected\": false, \"data\": {\"id_original\": \"2453\", \"name\": \"YOL051W (pd) YBR018C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1915\", \"EdgeBetweenness\": 5.0, \"shared_interaction\": \"pd\", \"source_original\": \"2110\", \"target_original\": \"2105\", \"target\": \"1570\", \"source\": \"1565\", \"shared_name\": \"YOL051W (pd) YBR018C\", \"SUID\": 1915}}, {\"selected\": false, \"data\": {\"id_original\": \"2454\", \"name\": \"YOL051W (pp) YPL248C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1914\", \"EdgeBetweenness\": 222.64848485, \"shared_interaction\": \"pp\", \"source_original\": \"2110\", \"target_original\": \"2106\", \"target\": \"1569\", \"source\": \"1565\", \"shared_name\": \"YOL051W (pp) YPL248C\", \"SUID\": 1914}}, {\"selected\": false, \"data\": {\"id_original\": \"2455\", \"name\": \"YOL051W (pd) YLR081W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1913\", \"EdgeBetweenness\": 5.0, \"shared_interaction\": \"pd\", \"source_original\": \"2110\", \"target_original\": \"2107\", \"target\": \"1568\", \"source\": \"1565\", \"shared_name\": \"YOL051W (pd) YLR081W\", \"SUID\": 1913}}, {\"selected\": false, \"data\": {\"id_original\": \"2456\", \"name\": \"YOL051W (pd) YBR020W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1912\", \"EdgeBetweenness\": 138.04242424, \"shared_interaction\": \"pd\", \"source_original\": \"2110\", \"target_original\": \"2108\", \"target\": \"1567\", \"source\": \"1565\", \"shared_name\": \"YOL051W (pd) YBR020W\", \"SUID\": 1912}}, {\"selected\": false, \"data\": {\"id_original\": \"2457\", \"name\": \"YBR019C (pd) YGL035C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1911\", \"EdgeBetweenness\": 583.07575758, \"shared_interaction\": \"pd\", \"source_original\": \"2111\", \"target_original\": \"2109\", \"target\": \"1566\", \"source\": \"1564\", \"shared_name\": \"YBR019C (pd) YGL035C\", \"SUID\": 1911}}, {\"selected\": false, \"data\": {\"id_original\": \"2458\", \"name\": \"YBR019C (pd) YOL051W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1910\", \"EdgeBetweenness\": 136.64242424, \"shared_interaction\": \"pd\", \"source_original\": \"2111\", \"target_original\": \"2110\", \"target\": \"1565\", \"source\": \"1564\", \"shared_name\": \"YBR019C (pd) YOL051W\", \"SUID\": 1910}}, {\"selected\": false, \"data\": {\"id_original\": \"2459\", \"name\": \"YJR060W (pd) YPR167C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1909\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2112\", \"target_original\": \"1946\", \"target\": \"1729\", \"source\": \"1563\", \"shared_name\": \"YJR060W (pd) YPR167C\", \"SUID\": 1909}}, {\"selected\": false, \"data\": {\"id_original\": \"2460\", \"name\": \"YDR103W (pp) YLR362W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1908\", \"EdgeBetweenness\": 486.0, \"shared_interaction\": \"pp\", \"source_original\": \"2113\", \"target_original\": \"2114\", \"target\": \"1561\", \"source\": \"1562\", \"shared_name\": \"YDR103W (pp) YLR362W\", \"SUID\": 1908}}, {\"selected\": false, \"data\": {\"id_original\": \"2461\", \"name\": \"YLR362W (pp) YPL240C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1907\", \"EdgeBetweenness\": 8670.28291708, \"shared_interaction\": \"pp\", \"source_original\": \"2114\", \"target_original\": \"1932\", \"target\": \"1743\", \"source\": \"1561\", \"shared_name\": \"YLR362W (pp) YPL240C\", \"SUID\": 1907}}, {\"selected\": false, \"data\": {\"id_original\": \"2462\", \"name\": \"YLR362W (pp) YMR186W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1906\", \"EdgeBetweenness\": 665.0, \"shared_interaction\": \"pp\", \"source_original\": \"2114\", \"target_original\": \"2127\", \"target\": \"1548\", \"source\": \"1561\", \"shared_name\": \"YLR362W (pp) YMR186W\", \"SUID\": 1906}}, {\"selected\": false, \"data\": {\"id_original\": \"2463\", \"name\": \"YLR362W (pp) YER124C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1905\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2114\", \"target_original\": \"1905\", \"target\": \"1770\", \"source\": \"1561\", \"shared_name\": \"YLR362W (pp) YER124C\", \"SUID\": 1905}}, {\"selected\": false, \"data\": {\"id_original\": \"2464\", \"name\": \"YCL032W (pp) YDR103W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1904\", \"EdgeBetweenness\": 10.0, \"shared_interaction\": \"pp\", \"source_original\": \"2116\", \"target_original\": \"2113\", \"target\": \"1562\", \"source\": \"1559\", \"shared_name\": \"YCL032W (pp) YDR103W\", \"SUID\": 1904}}, {\"selected\": false, \"data\": {\"id_original\": \"2465\", \"name\": \"YCL032W (pp) YLR362W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1903\", \"EdgeBetweenness\": 2430.0, \"shared_interaction\": \"pp\", \"source_original\": \"2116\", \"target_original\": \"2114\", \"target\": \"1561\", \"source\": \"1559\", \"shared_name\": \"YCL032W (pp) YLR362W\", \"SUID\": 1903}}, {\"selected\": false, \"data\": {\"id_original\": \"2466\", \"name\": \"YCL032W (pp) YDR032C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1902\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2116\", \"target_original\": \"2115\", \"target\": \"1560\", \"source\": \"1559\", \"shared_name\": \"YCL032W (pp) YDR032C\", \"SUID\": 1902}}, {\"selected\": false, \"data\": {\"id_original\": \"2467\", \"name\": \"YMR138W (pp) YLR109W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1901\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2119\", \"target_original\": \"2117\", \"target\": \"1558\", \"source\": \"1556\", \"shared_name\": \"YMR138W (pp) YLR109W\", \"SUID\": 1901}}, {\"selected\": false, \"data\": {\"id_original\": \"2468\", \"name\": \"YMR138W (pp) YHR141C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1900\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2119\", \"target_original\": \"2118\", \"target\": \"1557\", \"source\": \"1556\", \"shared_name\": \"YMR138W (pp) YHR141C\", \"SUID\": 1900}}, {\"selected\": false, \"data\": {\"id_original\": \"2469\", \"name\": \"YOL058W (pp) YNL189W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1899\", \"EdgeBetweenness\": 1797.88974359, \"shared_interaction\": \"pp\", \"source_original\": \"2121\", \"target_original\": \"2014\", \"target\": \"1661\", \"source\": \"1554\", \"shared_name\": \"YOL058W (pp) YNL189W\", \"SUID\": 1899}}, {\"selected\": false, \"data\": {\"id_original\": \"2470\", \"name\": \"YEL009C (pd) YMR300C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1898\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2125\", \"target_original\": \"2120\", \"target\": \"1555\", \"source\": \"1550\", \"shared_name\": \"YEL009C (pd) YMR300C\", \"SUID\": 1898}}, {\"selected\": false, \"data\": {\"id_original\": \"2471\", \"name\": \"YEL009C (pd) YOL058W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1897\", \"EdgeBetweenness\": 1902.08974359, \"shared_interaction\": \"pd\", \"source_original\": \"2125\", \"target_original\": \"2121\", \"target\": \"1554\", \"source\": \"1550\", \"shared_name\": \"YEL009C (pd) YOL058W\", \"SUID\": 1897}}, {\"selected\": false, \"data\": {\"id_original\": \"2472\", \"name\": \"YEL009C (pd) YBR248C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1896\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2125\", \"target_original\": \"2122\", \"target\": \"1553\", \"source\": \"1550\", \"shared_name\": \"YEL009C (pd) YBR248C\", \"SUID\": 1896}}, {\"selected\": false, \"data\": {\"id_original\": \"2473\", \"name\": \"YEL009C (pd) YCL030C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1895\", \"EdgeBetweenness\": 3692.35897436, \"shared_interaction\": \"pd\", \"source_original\": \"2125\", \"target_original\": \"2154\", \"target\": \"1521\", \"source\": \"1550\", \"shared_name\": \"YEL009C (pd) YCL030C\", \"SUID\": 1895}}, {\"selected\": false, \"data\": {\"id_original\": \"2474\", \"name\": \"YEL009C (pd) YOR202W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1894\", \"EdgeBetweenness\": 635.26923077, \"shared_interaction\": \"pd\", \"source_original\": \"2125\", \"target_original\": \"2123\", \"target\": \"1552\", \"source\": \"1550\", \"shared_name\": \"YEL009C (pd) YOR202W\", \"SUID\": 1894}}, {\"selected\": false, \"data\": {\"id_original\": \"2475\", \"name\": \"YEL009C (pd) YMR108W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1893\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2125\", \"target_original\": \"2124\", \"target\": \"1551\", \"source\": \"1550\", \"shared_name\": \"YEL009C (pd) YMR108W\", \"SUID\": 1893}}, {\"selected\": false, \"data\": {\"id_original\": \"2476\", \"name\": \"YMR186W (pp) YBR155W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1892\", \"EdgeBetweenness\": 247.0, \"shared_interaction\": \"pp\", \"source_original\": \"2127\", \"target_original\": \"2126\", \"target\": \"1549\", \"source\": \"1548\", \"shared_name\": \"YMR186W (pp) YBR155W\", \"SUID\": 1892}}, {\"selected\": false, \"data\": {\"id_original\": \"2477\", \"name\": \"YOR326W (pp) YGL106W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1891\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"2129\", \"target_original\": \"2128\", \"target\": \"1547\", \"source\": \"1546\", \"shared_name\": \"YOR326W (pp) YGL106W\", \"SUID\": 1891}}, {\"selected\": false, \"data\": {\"id_original\": \"2478\", \"name\": \"YMR309C (pp) YNL047C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1890\", \"EdgeBetweenness\": 1581.91544567, \"shared_interaction\": \"pp\", \"source_original\": \"2130\", \"target_original\": \"2031\", \"target\": \"1644\", \"source\": \"1545\", \"shared_name\": \"YMR309C (pp) YNL047C\", \"SUID\": 1890}}, {\"selected\": false, \"data\": {\"id_original\": \"2479\", \"name\": \"YOR361C (pp) YDR429C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1889\", \"EdgeBetweenness\": 1287.12973138, \"shared_interaction\": \"pp\", \"source_original\": \"2131\", \"target_original\": \"2189\", \"target\": \"1486\", \"source\": \"1544\", \"shared_name\": \"YOR361C (pp) YDR429C\", \"SUID\": 1889}}, {\"selected\": false, \"data\": {\"id_original\": \"2480\", \"name\": \"YOR361C (pp) YMR309C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1888\", \"EdgeBetweenness\": 1073.41544567, \"shared_interaction\": \"pp\", \"source_original\": \"2131\", \"target_original\": \"2130\", \"target\": \"1545\", \"source\": \"1544\", \"shared_name\": \"YOR361C (pp) YMR309C\", \"SUID\": 1888}}, {\"selected\": false, \"data\": {\"id_original\": \"2481\", \"name\": \"YER179W (pp) YIL105C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1887\", \"EdgeBetweenness\": 2481.91544567, \"shared_interaction\": \"pp\", \"source_original\": \"2134\", \"target_original\": \"2132\", \"target\": \"1543\", \"source\": \"1541\", \"shared_name\": \"YER179W (pp) YIL105C\", \"SUID\": 1887}}, {\"selected\": false, \"data\": {\"id_original\": \"2482\", \"name\": \"YER179W (pp) YLR134W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1886\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2134\", \"target_original\": \"2133\", \"target\": \"1542\", \"source\": \"1541\", \"shared_name\": \"YER179W (pp) YLR134W\", \"SUID\": 1886}}, {\"selected\": false, \"data\": {\"id_original\": \"2483\", \"name\": \"YER179W (pp) YLR044C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1885\", \"EdgeBetweenness\": 3377.91544567, \"shared_interaction\": \"pp\", \"source_original\": \"2134\", \"target_original\": \"2157\", \"target\": \"1518\", \"source\": \"1541\", \"shared_name\": \"YER179W (pp) YLR044C\", \"SUID\": 1885}}, {\"selected\": false, \"data\": {\"id_original\": \"2484\", \"name\": \"YDL014W (pp) YOR310C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1884\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"2136\", \"target_original\": \"2135\", \"target\": \"1540\", \"source\": \"1539\", \"shared_name\": \"YDL014W (pp) YOR310C\", \"SUID\": 1884}}, {\"selected\": false, \"data\": {\"id_original\": \"2485\", \"name\": \"YPR119W (pd) YMR043W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1883\", \"EdgeBetweenness\": 18360.0, \"shared_interaction\": \"pd\", \"source_original\": \"2137\", \"target_original\": \"2076\", \"target\": \"1599\", \"source\": \"1538\", \"shared_name\": \"YPR119W (pd) YMR043W\", \"SUID\": 1883}}, {\"selected\": false, \"data\": {\"id_original\": \"2486\", \"name\": \"YLR117C (pp) YBR190W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1882\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2138\", \"target_original\": \"1958\", \"target\": \"1717\", \"source\": \"1537\", \"shared_name\": \"YLR117C (pp) YBR190W\", \"SUID\": 1882}}, {\"selected\": false, \"data\": {\"id_original\": \"2487\", \"name\": \"YGL013C (pd) YOL156W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1881\", \"EdgeBetweenness\": 739.0, \"shared_interaction\": \"pd\", \"source_original\": \"2139\", \"target_original\": \"2062\", \"target\": \"1613\", \"source\": \"1536\", \"shared_name\": \"YGL013C (pd) YOL156W\", \"SUID\": 1881}}, {\"selected\": false, \"data\": {\"id_original\": \"2488\", \"name\": \"YGL013C (pd) YJL219W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1880\", \"EdgeBetweenness\": 739.0, \"shared_interaction\": \"pd\", \"source_original\": \"2139\", \"target_original\": \"2063\", \"target\": \"1612\", \"source\": \"1536\", \"shared_name\": \"YGL013C (pd) YJL219W\", \"SUID\": 1880}}, {\"selected\": false, \"data\": {\"id_original\": \"2489\", \"name\": \"YCR086W (pp) YOR264W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1879\", \"EdgeBetweenness\": 1476.0, \"shared_interaction\": \"pp\", \"source_original\": \"2140\", \"target_original\": \"2094\", \"target\": \"1581\", \"source\": \"1535\", \"shared_name\": \"YCR086W (pp) YOR264W\", \"SUID\": 1879}}, {\"selected\": false, \"data\": {\"id_original\": \"2490\", \"name\": \"YDR412W (pp) YPR119W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1878\", \"EdgeBetweenness\": 18040.0, \"shared_interaction\": \"pp\", \"source_original\": \"2141\", \"target_original\": \"2137\", \"target\": \"1538\", \"source\": \"1534\", \"shared_name\": \"YDR412W (pp) YPR119W\", \"SUID\": 1878}}, {\"selected\": false, \"data\": {\"id_original\": \"2491\", \"name\": \"YDR412W (pp) YLR117C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1877\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2141\", \"target_original\": \"2138\", \"target\": \"1537\", \"source\": \"1534\", \"shared_name\": \"YDR412W (pp) YLR117C\", \"SUID\": 1877}}, {\"selected\": false, \"data\": {\"id_original\": \"2492\", \"name\": \"YDR412W (pp) YGL013C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1876\", \"EdgeBetweenness\": 1960.0, \"shared_interaction\": \"pp\", \"source_original\": \"2141\", \"target_original\": \"2139\", \"target\": \"1536\", \"source\": \"1534\", \"shared_name\": \"YDR412W (pp) YGL013C\", \"SUID\": 1876}}, {\"selected\": false, \"data\": {\"id_original\": \"2493\", \"name\": \"YDR412W (pp) YCR086W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1875\", \"EdgeBetweenness\": 1960.0, \"shared_interaction\": \"pp\", \"source_original\": \"2141\", \"target_original\": \"2140\", \"target\": \"1535\", \"source\": \"1534\", \"shared_name\": \"YDR412W (pp) YCR086W\", \"SUID\": 1875}}, {\"selected\": false, \"data\": {\"id_original\": \"2494\", \"name\": \"YER062C (pp) YPL201C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1874\", \"EdgeBetweenness\": 2.0, \"shared_interaction\": \"pp\", \"source_original\": \"2143\", \"target_original\": \"2142\", \"target\": \"1533\", \"source\": \"1532\", \"shared_name\": \"YER062C (pp) YPL201C\", \"SUID\": 1874}}, {\"selected\": false, \"data\": {\"id_original\": \"2495\", \"name\": \"YOR327C (pp) YER143W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1873\", \"EdgeBetweenness\": 249.0, \"shared_interaction\": \"pp\", \"source_original\": \"2144\", \"target_original\": \"2145\", \"target\": \"1530\", \"source\": \"1531\", \"shared_name\": \"YOR327C (pp) YER143W\", \"SUID\": 1873}}, {\"selected\": false, \"data\": {\"id_original\": \"2496\", \"name\": \"YAL030W (pp) YER143W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1872\", \"EdgeBetweenness\": 249.0, \"shared_interaction\": \"pp\", \"source_original\": \"2146\", \"target_original\": \"2145\", \"target\": \"1530\", \"source\": \"1529\", \"shared_name\": \"YAL030W (pp) YER143W\", \"SUID\": 1872}}, {\"selected\": false, \"data\": {\"id_original\": \"2497\", \"name\": \"YCL030C (pd) YKR099W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1871\", \"EdgeBetweenness\": 3832.74371184, \"shared_interaction\": \"pd\", \"source_original\": \"2154\", \"target_original\": \"2103\", \"target\": \"1572\", \"source\": \"1521\", \"shared_name\": \"YCL030C (pd) YKR099W\", \"SUID\": 1871}}, {\"selected\": false, \"data\": {\"id_original\": \"2498\", \"name\": \"YCR012W (pd) YJR060W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1870\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pd\", \"source_original\": \"2161\", \"target_original\": \"2112\", \"target\": \"1563\", \"source\": \"1514\", \"shared_name\": \"YCR012W (pd) YJR060W\", \"SUID\": 1870}}, {\"selected\": false, \"data\": {\"id_original\": \"2499\", \"name\": \"YNL216W (pd) YOL086C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1869\", \"EdgeBetweenness\": 2148.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2147\", \"target\": \"1528\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YOL086C\", \"SUID\": 1869}}, {\"selected\": false, \"data\": {\"id_original\": \"2500\", \"name\": \"YNL216W (pd) YDR050C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1868\", \"EdgeBetweenness\": 2148.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2148\", \"target\": \"1527\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YDR050C\", \"SUID\": 1868}}, {\"selected\": false, \"data\": {\"id_original\": \"2501\", \"name\": \"YNL216W (pd) YOL127W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1867\", \"EdgeBetweenness\": 2391.0, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2149\", \"target\": \"1526\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YOL127W\", \"SUID\": 1867}}, {\"selected\": false, \"data\": {\"id_original\": \"2502\", \"name\": \"YNL216W (pd) YAL038W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1866\", \"EdgeBetweenness\": 2940.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2163\", \"target\": \"1512\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YAL038W\", \"SUID\": 1866}}, {\"selected\": false, \"data\": {\"id_original\": \"2503\", \"name\": \"YNL216W (pd) YIL069C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1865\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2150\", \"target\": \"1525\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YIL069C\", \"SUID\": 1865}}, {\"selected\": false, \"data\": {\"id_original\": \"2504\", \"name\": \"YNL216W (pd) YER074W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1864\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2151\", \"target\": \"1524\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YER074W\", \"SUID\": 1864}}, {\"selected\": false, \"data\": {\"id_original\": \"2505\", \"name\": \"YNL216W (pd) YBR093C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1863\", \"EdgeBetweenness\": 1476.0, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2152\", \"target\": \"1523\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YBR093C\", \"SUID\": 1863}}, {\"selected\": false, \"data\": {\"id_original\": \"2506\", \"name\": \"YNL216W (pp) YDR171W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1862\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2162\", \"target_original\": \"2153\", \"target\": \"1522\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pp) YDR171W\", \"SUID\": 1862}}, {\"selected\": false, \"data\": {\"id_original\": \"2507\", \"name\": \"YNL216W (pd) YCL030C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1861\", \"EdgeBetweenness\": 5079.35982906, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2154\", \"target\": \"1521\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YCL030C\", \"SUID\": 1861}}, {\"selected\": false, \"data\": {\"id_original\": \"2508\", \"name\": \"YNL216W (pd) YNL301C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1860\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2155\", \"target\": \"1520\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YNL301C\", \"SUID\": 1860}}, {\"selected\": false, \"data\": {\"id_original\": \"2509\", \"name\": \"YNL216W (pd) YOL120C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1859\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2156\", \"target\": \"1519\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YOL120C\", \"SUID\": 1859}}, {\"selected\": false, \"data\": {\"id_original\": \"2510\", \"name\": \"YNL216W (pd) YLR044C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1858\", \"EdgeBetweenness\": 15620.15017205, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2157\", \"target\": \"1518\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YLR044C\", \"SUID\": 1858}}, {\"selected\": false, \"data\": {\"id_original\": \"2511\", \"name\": \"YNL216W (pd) YIL133C\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1857\", \"EdgeBetweenness\": 2391.0, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2158\", \"target\": \"1517\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YIL133C\", \"SUID\": 1857}}, {\"selected\": false, \"data\": {\"id_original\": \"2512\", \"name\": \"YNL216W (pd) YHR174W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1856\", \"EdgeBetweenness\": 2148.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2159\", \"target\": \"1516\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YHR174W\", \"SUID\": 1856}}, {\"selected\": false, \"data\": {\"id_original\": \"2513\", \"name\": \"YNL216W (pd) YGR254W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1855\", \"EdgeBetweenness\": 2148.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2160\", \"target\": \"1515\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YGR254W\", \"SUID\": 1855}}, {\"selected\": false, \"data\": {\"id_original\": \"2514\", \"name\": \"YNL216W (pd) YCR012W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1854\", \"EdgeBetweenness\": 2940.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2162\", \"target_original\": \"2161\", \"target\": \"1514\", \"source\": \"1513\", \"shared_name\": \"YNL216W (pd) YCR012W\", \"SUID\": 1854}}, {\"selected\": false, \"data\": {\"id_original\": \"2515\", \"name\": \"YAL038W (pd) YPL075W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1853\", \"EdgeBetweenness\": 2034.76542347, \"shared_interaction\": \"pd\", \"source_original\": \"2163\", \"target_original\": \"2021\", \"target\": \"1654\", \"source\": \"1512\", \"shared_name\": \"YAL038W (pd) YPL075W\", \"SUID\": 1853}}, {\"selected\": false, \"data\": {\"id_original\": \"2516\", \"name\": \"YNL307C (pp) YAL038W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1852\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2164\", \"target_original\": \"2163\", \"target\": \"1512\", \"source\": \"1511\", \"shared_name\": \"YNL307C (pp) YAL038W\", \"SUID\": 1852}}, {\"selected\": false, \"data\": {\"id_original\": \"2517\", \"name\": \"YER116C (pp) YDL013W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1851\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2166\", \"target_original\": \"2165\", \"target\": \"1510\", \"source\": \"1509\", \"shared_name\": \"YER116C (pp) YDL013W\", \"SUID\": 1851}}, {\"selected\": false, \"data\": {\"id_original\": \"2518\", \"name\": \"YNR053C (pp) YDL030W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1850\", \"EdgeBetweenness\": 7118.71708292, \"shared_interaction\": \"pp\", \"source_original\": \"2167\", \"target_original\": \"1907\", \"target\": \"1768\", \"source\": \"1508\", \"shared_name\": \"YNR053C (pp) YDL030W\", \"SUID\": 1850}}, {\"selected\": false, \"data\": {\"id_original\": \"2519\", \"name\": \"YNR053C (pp) YJL203W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1849\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2167\", \"target_original\": \"2066\", \"target\": \"1609\", \"source\": \"1508\", \"shared_name\": \"YNR053C (pp) YJL203W\", \"SUID\": 1849}}, {\"selected\": false, \"data\": {\"id_original\": \"2520\", \"name\": \"YLR264W (pp) YBL026W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1848\", \"EdgeBetweenness\": 248.5, \"shared_interaction\": \"pp\", \"source_original\": \"2168\", \"target_original\": \"1921\", \"target\": \"1754\", \"source\": \"1507\", \"shared_name\": \"YLR264W (pp) YBL026W\", \"SUID\": 1848}}, {\"selected\": false, \"data\": {\"id_original\": \"2521\", \"name\": \"YLR264W (pp) YOL149W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1847\", \"EdgeBetweenness\": 248.5, \"shared_interaction\": \"pp\", \"source_original\": \"2168\", \"target_original\": \"1953\", \"target\": \"1722\", \"source\": \"1507\", \"shared_name\": \"YLR264W (pp) YOL149W\", \"SUID\": 1847}}, {\"selected\": false, \"data\": {\"id_original\": \"2522\", \"name\": \"YLR264W (pp) YER112W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1846\", \"EdgeBetweenness\": 248.5, \"shared_interaction\": \"pp\", \"source_original\": \"2168\", \"target_original\": \"2174\", \"target\": \"1501\", \"source\": \"1507\", \"shared_name\": \"YLR264W (pp) YER112W\", \"SUID\": 1846}}, {\"selected\": false, \"data\": {\"id_original\": \"2523\", \"name\": \"YEL015W (pp) YML064C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1845\", \"EdgeBetweenness\": 3025.31048951, \"shared_interaction\": \"pp\", \"source_original\": \"2169\", \"target_original\": \"2034\", \"target\": \"1641\", \"source\": \"1506\", \"shared_name\": \"YEL015W (pp) YML064C\", \"SUID\": 1845}}, {\"selected\": false, \"data\": {\"id_original\": \"2524\", \"name\": \"YNR050C (pp) YMR138W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1844\", \"EdgeBetweenness\": 1476.0, \"shared_interaction\": \"pp\", \"source_original\": \"2171\", \"target_original\": \"2119\", \"target\": \"1556\", \"source\": \"1504\", \"shared_name\": \"YNR050C (pp) YMR138W\", \"SUID\": 1844}}, {\"selected\": false, \"data\": {\"id_original\": \"2525\", \"name\": \"YJR022W (pp) YNR053C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1843\", \"EdgeBetweenness\": 6283.85994006, \"shared_interaction\": \"pp\", \"source_original\": \"2172\", \"target_original\": \"2167\", \"target\": \"1508\", \"source\": \"1503\", \"shared_name\": \"YJR022W (pp) YNR053C\", \"SUID\": 1843}}, {\"selected\": false, \"data\": {\"id_original\": \"2526\", \"name\": \"YJR022W (pp) YLR264W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1842\", \"EdgeBetweenness\": 1220.5, \"shared_interaction\": \"pp\", \"source_original\": \"2172\", \"target_original\": \"2168\", \"target\": \"1507\", \"source\": \"1503\", \"shared_name\": \"YJR022W (pp) YLR264W\", \"SUID\": 1842}}, {\"selected\": false, \"data\": {\"id_original\": \"2527\", \"name\": \"YJR022W (pp) YOR167C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1841\", \"EdgeBetweenness\": 1220.5, \"shared_interaction\": \"pp\", \"source_original\": \"2172\", \"target_original\": \"2173\", \"target\": \"1502\", \"source\": \"1503\", \"shared_name\": \"YJR022W (pp) YOR167C\", \"SUID\": 1841}}, {\"selected\": false, \"data\": {\"id_original\": \"2528\", \"name\": \"YJR022W (pp) YEL015W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1840\", \"EdgeBetweenness\": 3050.14565435, \"shared_interaction\": \"pp\", \"source_original\": \"2172\", \"target_original\": \"2169\", \"target\": \"1506\", \"source\": \"1503\", \"shared_name\": \"YJR022W (pp) YEL015W\", \"SUID\": 1840}}, {\"selected\": false, \"data\": {\"id_original\": \"2529\", \"name\": \"YJR022W (pp) YNL050C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1839\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2172\", \"target_original\": \"2170\", \"target\": \"1505\", \"source\": \"1503\", \"shared_name\": \"YJR022W (pp) YNL050C\", \"SUID\": 1839}}, {\"selected\": false, \"data\": {\"id_original\": \"2530\", \"name\": \"YJR022W (pp) YNR050C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1838\", \"EdgeBetweenness\": 1960.0, \"shared_interaction\": \"pp\", \"source_original\": \"2172\", \"target_original\": \"2171\", \"target\": \"1504\", \"source\": \"1503\", \"shared_name\": \"YJR022W (pp) YNR050C\", \"SUID\": 1838}}, {\"selected\": false, \"data\": {\"id_original\": \"2531\", \"name\": \"YER112W (pp) YOR167C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1837\", \"EdgeBetweenness\": 248.5, \"shared_interaction\": \"pp\", \"source_original\": \"2174\", \"target_original\": \"2173\", \"target\": \"1502\", \"source\": \"1501\", \"shared_name\": \"YER112W (pp) YOR167C\", \"SUID\": 1837}}, {\"selected\": false, \"data\": {\"id_original\": \"2532\", \"name\": \"YCL067C (pd) YFL026W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1836\", \"EdgeBetweenness\": 9.83333333, \"shared_interaction\": \"pd\", \"source_original\": \"2175\", \"target_original\": \"2068\", \"target\": \"1607\", \"source\": \"1500\", \"shared_name\": \"YCL067C (pd) YFL026W\", \"SUID\": 1836}}, {\"selected\": false, \"data\": {\"id_original\": \"2533\", \"name\": \"YCL067C (pd) YDR461W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1835\", \"EdgeBetweenness\": 9.83333333, \"shared_interaction\": \"pd\", \"source_original\": \"2175\", \"target_original\": \"2071\", \"target\": \"1604\", \"source\": \"1500\", \"shared_name\": \"YCL067C (pd) YDR461W\", \"SUID\": 1835}}, {\"selected\": false, \"data\": {\"id_original\": \"2534\", \"name\": \"YCL067C (pp) YMR043W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1834\", \"EdgeBetweenness\": 1447.5, \"shared_interaction\": \"pp\", \"source_original\": \"2175\", \"target_original\": \"2076\", \"target\": \"1599\", \"source\": \"1500\", \"shared_name\": \"YCL067C (pp) YMR043W\", \"SUID\": 1834}}, {\"selected\": false, \"data\": {\"id_original\": \"2535\", \"name\": \"YCL067C (pd) YIL015W\", \"interaction\": \"pd\", \"selected\": false, \"id\": \"1833\", \"EdgeBetweenness\": 9.0, \"shared_interaction\": \"pd\", \"source_original\": \"2175\", \"target_original\": \"2075\", \"target\": \"1600\", \"source\": \"1500\", \"shared_name\": \"YCL067C (pd) YIL015W\", \"SUID\": 1833}}, {\"selected\": false, \"data\": {\"id_original\": \"2536\", \"name\": \"YCR084C (pp) YCL067C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1832\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2177\", \"target_original\": \"2175\", \"target\": \"1500\", \"source\": \"1498\", \"shared_name\": \"YCR084C (pp) YCL067C\", \"SUID\": 1832}}, {\"selected\": false, \"data\": {\"id_original\": \"2537\", \"name\": \"YCR084C (pp) YBR112C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1831\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2177\", \"target_original\": \"2176\", \"target\": \"1499\", \"source\": \"1498\", \"shared_name\": \"YCR084C (pp) YBR112C\", \"SUID\": 1831}}, {\"selected\": false, \"data\": {\"id_original\": \"2538\", \"name\": \"YIL061C (pp) YLR153C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1830\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2178\", \"target_original\": \"1898\", \"target\": \"1777\", \"source\": \"1497\", \"shared_name\": \"YIL061C (pp) YLR153C\", \"SUID\": 1830}}, {\"selected\": false, \"data\": {\"id_original\": \"2539\", \"name\": \"YIL061C (pp) YNL199C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1829\", \"EdgeBetweenness\": 9902.71708292, \"shared_interaction\": \"pp\", \"source_original\": \"2178\", \"target_original\": \"2020\", \"target\": \"1655\", \"source\": \"1497\", \"shared_name\": \"YIL061C (pp) YNL199C\", \"SUID\": 1829}}, {\"selected\": false, \"data\": {\"id_original\": \"2540\", \"name\": \"YIL061C (pp) YDL013W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1828\", \"EdgeBetweenness\": 8486.71708292, \"shared_interaction\": \"pp\", \"source_original\": \"2178\", \"target_original\": \"2165\", \"target\": \"1510\", \"source\": \"1497\", \"shared_name\": \"YIL061C (pp) YDL013W\", \"SUID\": 1828}}, {\"selected\": false, \"data\": {\"id_original\": \"2541\", \"name\": \"YGR203W (pp) YIL061C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1827\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2179\", \"target_original\": \"2178\", \"target\": \"1497\", \"source\": \"1496\", \"shared_name\": \"YGR203W (pp) YIL061C\", \"SUID\": 1827}}, {\"selected\": false, \"data\": {\"id_original\": \"2542\", \"name\": \"YJL013C (pp) YGL229C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1826\", \"EdgeBetweenness\": 5.0, \"shared_interaction\": \"pp\", \"source_original\": \"2180\", \"target_original\": \"2181\", \"target\": \"1494\", \"source\": \"1495\", \"shared_name\": \"YJL013C (pp) YGL229C\", \"SUID\": 1826}}, {\"selected\": false, \"data\": {\"id_original\": \"2543\", \"name\": \"YJL030W (pp) YGL229C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1825\", \"EdgeBetweenness\": 5.0, \"shared_interaction\": \"pp\", \"source_original\": \"2182\", \"target_original\": \"2181\", \"target\": \"1494\", \"source\": \"1493\", \"shared_name\": \"YJL030W (pp) YGL229C\", \"SUID\": 1825}}, {\"selected\": false, \"data\": {\"id_original\": \"2544\", \"name\": \"YGR014W (pp) YJL013C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1824\", \"EdgeBetweenness\": 7.0, \"shared_interaction\": \"pp\", \"source_original\": \"2183\", \"target_original\": \"2180\", \"target\": \"1495\", \"source\": \"1492\", \"shared_name\": \"YGR014W (pp) YJL013C\", \"SUID\": 1824}}, {\"selected\": false, \"data\": {\"id_original\": \"2545\", \"name\": \"YGR014W (pp) YJL030W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1823\", \"EdgeBetweenness\": 7.0, \"shared_interaction\": \"pp\", \"source_original\": \"2183\", \"target_original\": \"2182\", \"target\": \"1493\", \"source\": \"1492\", \"shared_name\": \"YGR014W (pp) YJL030W\", \"SUID\": 1823}}, {\"selected\": false, \"data\": {\"id_original\": \"2546\", \"name\": \"YPL211W (pp) YGR014W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1822\", \"EdgeBetweenness\": 8.0, \"shared_interaction\": \"pp\", \"source_original\": \"2184\", \"target_original\": \"2183\", \"target\": \"1492\", \"source\": \"1491\", \"shared_name\": \"YPL211W (pp) YGR014W\", \"SUID\": 1822}}, {\"selected\": false, \"data\": {\"id_original\": \"2547\", \"name\": \"YOL123W (pp) YGL044C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1821\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2186\", \"target_original\": \"2185\", \"target\": \"1490\", \"source\": \"1489\", \"shared_name\": \"YOL123W (pp) YGL044C\", \"SUID\": 1821}}, {\"selected\": false, \"data\": {\"id_original\": \"2548\", \"name\": \"YFL017C (pp) YOR362C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1820\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2188\", \"target_original\": \"1955\", \"target\": \"1720\", \"source\": \"1487\", \"shared_name\": \"YFL017C (pp) YOR362C\", \"SUID\": 1820}}, {\"selected\": false, \"data\": {\"id_original\": \"2549\", \"name\": \"YFL017C (pp) YER102W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1819\", \"EdgeBetweenness\": 2758.08455433, \"shared_interaction\": \"pp\", \"source_original\": \"2188\", \"target_original\": \"1956\", \"target\": \"1719\", \"source\": \"1487\", \"shared_name\": \"YFL017C (pp) YER102W\", \"SUID\": 1819}}, {\"selected\": false, \"data\": {\"id_original\": \"2550\", \"name\": \"YFL017C (pp) YOL059W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1818\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2188\", \"target_original\": \"1957\", \"target\": \"1718\", \"source\": \"1487\", \"shared_name\": \"YFL017C (pp) YOL059W\", \"SUID\": 1818}}, {\"selected\": false, \"data\": {\"id_original\": \"2551\", \"name\": \"YDR429C (pp) YFL017C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1817\", \"EdgeBetweenness\": 1470.49481074, \"shared_interaction\": \"pp\", \"source_original\": \"2189\", \"target_original\": \"2188\", \"target\": \"1487\", \"source\": \"1486\", \"shared_name\": \"YDR429C (pp) YFL017C\", \"SUID\": 1817}}, {\"selected\": false, \"data\": {\"id_original\": \"2552\", \"name\": \"YMR146C (pp) YDR429C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1816\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2190\", \"target_original\": \"2189\", \"target\": \"1486\", \"source\": \"1485\", \"shared_name\": \"YMR146C (pp) YDR429C\", \"SUID\": 1816}}, {\"selected\": false, \"data\": {\"id_original\": \"2553\", \"name\": \"YLR293C (pp) YGL097W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1815\", \"EdgeBetweenness\": 6.0, \"shared_interaction\": \"pp\", \"source_original\": \"2191\", \"target_original\": \"2196\", \"target\": \"1479\", \"source\": \"1484\", \"shared_name\": \"YLR293C (pp) YGL097W\", \"SUID\": 1815}}, {\"selected\": false, \"data\": {\"id_original\": \"2554\", \"name\": \"YBR118W (pp) YAL003W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1814\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"2192\", \"target_original\": \"2187\", \"target\": \"1488\", \"source\": \"1483\", \"shared_name\": \"YBR118W (pp) YAL003W\", \"SUID\": 1814}}, {\"selected\": false, \"data\": {\"id_original\": \"2555\", \"name\": \"YPR080W (pp) YAL003W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1813\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"2193\", \"target_original\": \"2187\", \"target\": \"1488\", \"source\": \"1482\", \"shared_name\": \"YPR080W (pp) YAL003W\", \"SUID\": 1813}}, {\"selected\": false, \"data\": {\"id_original\": \"2556\", \"name\": \"YLR249W (pp) YBR118W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1812\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"2194\", \"target_original\": \"2192\", \"target\": \"1483\", \"source\": \"1481\", \"shared_name\": \"YLR249W (pp) YBR118W\", \"SUID\": 1812}}, {\"selected\": false, \"data\": {\"id_original\": \"2557\", \"name\": \"YLR249W (pp) YPR080W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1811\", \"EdgeBetweenness\": 4.0, \"shared_interaction\": \"pp\", \"source_original\": \"2194\", \"target_original\": \"2193\", \"target\": \"1482\", \"source\": \"1481\", \"shared_name\": \"YLR249W (pp) YPR080W\", \"SUID\": 1811}}, {\"selected\": false, \"data\": {\"id_original\": \"2558\", \"name\": \"YGL097W (pp) YOR204W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1810\", \"EdgeBetweenness\": 6.0, \"shared_interaction\": \"pp\", \"source_original\": \"2196\", \"target_original\": \"2195\", \"target\": \"1480\", \"source\": \"1479\", \"shared_name\": \"YGL097W (pp) YOR204W\", \"SUID\": 1810}}, {\"selected\": false, \"data\": {\"id_original\": \"2559\", \"name\": \"YGR218W (pp) YGL097W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1809\", \"EdgeBetweenness\": 6.0, \"shared_interaction\": \"pp\", \"source_original\": \"2197\", \"target_original\": \"2196\", \"target\": \"1479\", \"source\": \"1478\", \"shared_name\": \"YGR218W (pp) YGL097W\", \"SUID\": 1809}}, {\"selected\": false, \"data\": {\"id_original\": \"2560\", \"name\": \"YGL122C (pp) YOL123W\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1808\", \"EdgeBetweenness\": 988.0, \"shared_interaction\": \"pp\", \"source_original\": \"2198\", \"target_original\": \"2186\", \"target\": \"1489\", \"source\": \"1477\", \"shared_name\": \"YGL122C (pp) YOL123W\", \"SUID\": 1808}}, {\"selected\": false, \"data\": {\"id_original\": \"2561\", \"name\": \"YKR026C (pp) YGL122C\", \"interaction\": \"pp\", \"selected\": false, \"id\": \"1807\", \"EdgeBetweenness\": 496.0, \"shared_interaction\": \"pp\", \"source_original\": \"2199\", \"target_original\": \"2198\", \"target\": \"1477\", \"source\": \"1476\", \"shared_name\": \"YKR026C (pp) YGL122C\", \"SUID\": 1807}}]\n", | |
" },\n", | |
"\n", | |
" layout: {\n", | |
" name: 'preset'\n", | |
" },\n", | |
"\n", | |
" boxSelectionEnabled: true,\n", | |
"\n", | |
" ready: function() {\n", | |
" window.cy = this;\n", | |
" cy.fit();\n", | |
" cy.style().fromJson([{\"selector\": \"node\", \"css\": {\"text-opacity\": 1.0, \"height\": 55.0, \"text-valign\": \"center\", \"font-weight\": \"normal\", \"background-color\": \"rgb(106,172,184)\", \"border-color\": \"rgb(0,0,0)\", \"color\": \"rgb(85,85,85)\", \"width\": 55.0, \"border-opacity\": 1.0, \"content\": \"data(COMMON)\", \"font-family\": \"SansSerif\", \"shape\": \"ellipse\", \"background-opacity\": 1.0, \"text-halign\": \"center\", \"border-width\": 0.0, \"font-size\": 12}}, {\"selector\": \"node[Degree > 18]\", \"css\": {\"width\": 100.0}}, {\"selector\": \"node[Degree = 18]\", \"css\": {\"width\": 100.0}}, {\"selector\": \"node[Degree > 1][Degree < 18]\", \"css\": {\"width\": \"mapData(Degree,1,18,10.0,100.0)\"}}, {\"selector\": \"node[Degree = 1]\", \"css\": {\"width\": 10.0}}, {\"selector\": \"node[Degree < 1]\", \"css\": {\"width\": 10.0}}, {\"selector\": \"node[Degree > 18]\", \"css\": {\"font-size\": 100}}, {\"selector\": \"node[Degree = 18]\", \"css\": {\"font-size\": 100}}, {\"selector\": \"node[Degree > 1][Degree < 18]\", \"css\": {\"font-size\": \"mapData(Degree,1,18,10,100)\"}}, {\"selector\": \"node[Degree = 1]\", \"css\": {\"font-size\": 10}}, {\"selector\": \"node[Degree < 1]\", \"css\": {\"font-size\": 10}}, {\"selector\": \"node[Degree > 18]\", \"css\": {\"height\": 100.0}}, {\"selector\": \"node[Degree = 18]\", \"css\": {\"height\": 100.0}}, {\"selector\": \"node[Degree > 1][Degree < 18]\", \"css\": {\"height\": \"mapData(Degree,1,18,10.0,100.0)\"}}, {\"selector\": \"node[Degree = 1]\", \"css\": {\"height\": 10.0}}, {\"selector\": \"node[Degree < 1]\", \"css\": {\"height\": 10.0}}, {\"selector\": \"node[Degree > 18]\", \"css\": {\"background-color\": \"rgb(106,172,184)\"}}, {\"selector\": \"node[Degree = 18]\", \"css\": {\"background-color\": \"rgb(106,172,184)\"}}, {\"selector\": \"node[Degree > 1][Degree < 18]\", \"css\": {\"background-color\": \"mapData(Degree,1,18,rgb(255,255,255),rgb(106,172,184))\"}}, {\"selector\": \"node[Degree = 1]\", \"css\": {\"background-color\": \"rgb(255,255,255)\"}}, {\"selector\": \"node[Degree < 1]\", \"css\": {\"background-color\": \"rgb(255,255,255)\"}}, {\"selector\": \"node:selected\", \"css\": {\"background-color\": \"rgb(255,255,0)\"}}, {\"selector\": \"edge\", \"css\": {\"source-arrow-color\": \"rgb(0,0,0)\", \"content\": \"\", \"source-arrow-shape\": \"none\", \"line-style\": \"solid\", \"font-weight\": \"normal\", \"text-opacity\": 1.0, \"color\": \"rgb(0,0,0)\", \"width\": 2.0, \"target-arrow-shape\": \"none\", \"line-color\": \"rgb(51,51,51)\", \"target-arrow-color\": \"rgb(0,0,0)\", \"font-family\": \"SansSerif\", \"opacity\": 0.39215686274509803, \"font-size\": 10}}, {\"selector\": \"edge:selected\", \"css\": {\"line-color\": \"rgb(255,0,0)\"}}]).update();\n", | |
" }\n", | |
" });\n", | |
" }\n", | |
"\n", | |
" var before_render = function(){\n", | |
" if(window['cytoscape'] === undefined){\n", | |
" console.log(\"Waiting for Cyjs...\");\n", | |
" window.addEventListener(\"load_cytoscape\", before_render);\n", | |
" } else {\n", | |
" console.log(\"Ready to render graph!\");\n", | |
" render();\n", | |
" }\n", | |
" }\n", | |
"\n", | |
" before_render();\n", | |
"\n", | |
" })();\n", | |
" </script>\n", | |
"</head>\n", | |
"\n", | |
"<body>\n", | |
" <div id=\"cya6312872-861b-4b6a-82a2-b83352c19254\"></div>\n", | |
" <!-- When only #uuid div is placed on this page,\n", | |
" the height of output-box on ipynb will be 0px.\n", | |
" One line below will prevent that. -->\n", | |
" <div id=\"dummy\" style=\"width:1098px;height:700px\">\n", | |
"</body>\n", | |
"\n", | |
"</html>" | |
], | |
"text/plain": [ | |
"<IPython.core.display.HTML object>" | |
] | |
}, | |
"metadata": {}, | |
"output_type": "display_data" | |
} | |
], | |
"source": [ | |
"# Step 5: (Optional) Embed as interactive Cytoscape.js widget\n", | |
"yeast_net_view = yeast_net.get_first_view()\n", | |
"style_for_widget = cy.style.get(my_yeast_style.get_name(), data_format='cytoscapejs')\n", | |
"renderer.render(yeast_net_view, style=style_for_widget['style'], background='radial-gradient(#FFFFFF 15%, #DDDDDD 105%)')" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"# Long Description\n", | |
"From version 0.4.0, ___py2cytoscape___ has wrapper modules for [cyREST](http://apps.cytoscape.org/apps/cyrest) RESTful API. This means you can access Cytoscape features in more _Pythonic_ way instead of calling raw REST API via HTTP. \n", | |
"\n", | |
"\n", | |
"## Features\n", | |
"\n", | |
"### Pandas for basic data exchange\n", | |
"Since [pandas](http://pandas.pydata.org/) is a standard library for data mangling/analysis in Python, this new version uses its [DataFrame](http://pandas.pydata.org/pandas-docs/stable/generated/pandas.DataFrame.html#pandas.DataFrame) as its basic data object.\n", | |
"\n", | |
"### Embedded Cytoscaep.js Widget\n", | |
"You can use Cytoscape.js widget to embed your final result as a part of your notebook.\n", | |
"\n", | |
"### Simpler Code to access Cytoscape\n", | |
"cyREST provides language-agnostic RESTful API, but you need to use a lot of template code to access raw API. Here is an example. Both of the following do the same task, which is creating an empty network in Cytoscape. You will notice it is significantly simpler if you use _py2cytoscape_ wrapper API.\n", | |
"\n", | |
"#### Raw cyREST Workflow Example" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 5, | |
"metadata": { | |
"collapsed": false | |
}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"New network created with raw REST API. Its SUID is 2864\n" | |
] | |
} | |
], | |
"source": [ | |
"# HTTP Client for Python\n", | |
"import requests\n", | |
"\n", | |
"# Standard JSON library\n", | |
"import json\n", | |
"\n", | |
"# Basic Setup\n", | |
"PORT_NUMBER = 1234\n", | |
"BASE = 'http://localhost:' + str(PORT_NUMBER) + '/v1/'\n", | |
"\n", | |
"# Header for posting data to the server as JSON\n", | |
"HEADERS = {'Content-Type': 'application/json'}\n", | |
"\n", | |
"# Define dictionary of empty network\n", | |
"empty_network = {\n", | |
" 'data': {\n", | |
" 'name': 'This is an empty network...'\n", | |
" },\n", | |
" 'elements': {\n", | |
" 'nodes':[],\n", | |
" 'edges':[]\n", | |
" }\n", | |
"}\n", | |
"\n", | |
"res = requests.post(BASE + 'networks?collection=My%20Collection', data=json.dumps(empty_network), headers=HEADERS)\n", | |
"new_network_id = res.json()['networkSUID']\n", | |
"print('New network created with raw REST API. Its SUID is ' + str(new_network_id))" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"#### With py2cytoscape" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 6, | |
"metadata": { | |
"collapsed": false | |
}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"New network created with py2cytoscape. Its SUID is 2876\n" | |
] | |
} | |
], | |
"source": [ | |
"network = cy.network.create(name='Another empty network created by py2cy', collection='My network collection')\n", | |
"print('New network created with py2cytoscape. Its SUID is ' + str(network.get_id()))" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"## Status\n", | |
"\n", | |
"As of 6/4/2015, this is still in alpha status and feature requests are always welcome. If youi have questions or feature requests, please send them to our Google Groups:\n", | |
"\n", | |
"* https://groups.google.com/forum/#!forum/cytoscape-discuss" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"# Quick Tour of py2cytoscape Features\n", | |
"\n", | |
"----\n", | |
"\n", | |
"## Create a client object to connect to Cytoscape" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 7, | |
"metadata": { | |
"collapsed": true | |
}, | |
"outputs": [], | |
"source": [ | |
"# Create an instance of cyREST client. Default IP is 'localhost', and port number is 1234.\n", | |
"# cy = CyRestClient() - This default constructor creates connection to http://localhost:1234/v1\n", | |
"cy = CyRestClient(ip='127.0.0.1', port=1234)\n", | |
"\n", | |
"# Cleanup: Delete all existing networks and tables in current Cytoscape session\n", | |
"cy.session.delete()" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"## Creating empty networks" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 8, | |
"metadata": { | |
"collapsed": true | |
}, | |
"outputs": [], | |
"source": [ | |
"# Empty network\n", | |
"empty1 = cy.network.create()\n", | |
"\n", | |
"# With name\n", | |
"empty2 = cy.network.create(name='Created in Jupyter Notebook')\n", | |
"\n", | |
"# With name and collection name\n", | |
"empty3 = cy.network.create(name='Also created in Jupyter', collection='New network collection')" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"## Load networks from files, URLs or web services" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 9, | |
"metadata": { | |
"collapsed": false | |
}, | |
"outputs": [ | |
{ | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>CyNetwork</th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>file:////Users/kono/git/tsri-lecture/lecture2/data/sample_yeast_network.xgmml</th>\n", | |
" <td><py2cytoscape.data.cynetwork.CyNetwork object ...</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>http://chianti.ucsd.edu/cytoscape-data/galFiltered.sif</th>\n", | |
" <td><py2cytoscape.data.cynetwork.CyNetwork object ...</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" CyNetwork\n", | |
"file:////Users/kono/git/tsri-lecture/lecture2/d... <py2cytoscape.data.cynetwork.CyNetwork object ...\n", | |
"http://chianti.ucsd.edu/cytoscape-data/galFilte... <py2cytoscape.data.cynetwork.CyNetwork object ..." | |
] | |
}, | |
"execution_count": 9, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"# Load a single local file\n", | |
"net_from_local2 = cy.network.create_from('data/galFiltered.json')\n", | |
"net_from_local1 = cy.network.create_from('data/sample_yeast_network.xgmml', collection='My Collection')\n", | |
"net_from_local2 = cy.network.create_from('data/galFiltered.gml', collection='Human')\n", | |
"\n", | |
"# Load from multiple locations\n", | |
"network_locations = [\n", | |
" 'data/sample_yeast_network.xgmml', # Local file\n", | |
" 'http://chianti.ucsd.edu/cytoscape-data/galFiltered.sif', # Static file on a web server\n", | |
"]\n", | |
"\n", | |
"# This requrns Series\n", | |
"networks = cy.network.create_from(network_locations)\n", | |
"pd.DataFrame(networks, columns=['CyNetwork'])" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"## Create networks from various types of data\n", | |
"Currently, py2cytoscape accepts the following data as input:\n", | |
"\n", | |
"* Cytoscape.js\n", | |
"* NetworkX\n", | |
"* Pandas DataFrame\n", | |
"\n", | |
"\n", | |
"* igraph (TBD)\n", | |
"* Numpy adjacency matrix (binary or weighted) (TBD)\n", | |
"* GraphX (TBD)" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 10, | |
"metadata": { | |
"collapsed": false | |
}, | |
"outputs": [], | |
"source": [ | |
"# Cytoscape.js JSON\n", | |
"n1 = cy.network.create(data=cyjs.get_empty_network(), name='Created from Cytoscape.js JSON')" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 11, | |
"metadata": { | |
"collapsed": false | |
}, | |
"outputs": [ | |
{ | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>source</th>\n", | |
" <th>interaction</th>\n", | |
" <th>target</th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>0</th>\n", | |
" <td>YKR026C</td>\n", | |
" <td>pp</td>\n", | |
" <td>YGL122C</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>1</th>\n", | |
" <td>YGR218W</td>\n", | |
" <td>pp</td>\n", | |
" <td>YGL097W</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>2</th>\n", | |
" <td>YGL097W</td>\n", | |
" <td>pp</td>\n", | |
" <td>YOR204W</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>3</th>\n", | |
" <td>YLR249W</td>\n", | |
" <td>pp</td>\n", | |
" <td>YPR080W</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>4</th>\n", | |
" <td>YLR249W</td>\n", | |
" <td>pp</td>\n", | |
" <td>YBR118W</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" source interaction target\n", | |
"0 YKR026C pp YGL122C\n", | |
"1 YGR218W pp YGL097W\n", | |
"2 YGL097W pp YOR204W\n", | |
"3 YLR249W pp YPR080W\n", | |
"4 YLR249W pp YBR118W" | |
] | |
}, | |
"execution_count": 11, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"# Pandas DataFrame\n", | |
"\n", | |
"# Example 1: From a simple text table\n", | |
"df_from_sif = pd.read_csv('data/galFiltered.sif', names=['source', 'interaction', 'target'], sep=' ')\n", | |
"df_from_sif.head()" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 12, | |
"metadata": { | |
"collapsed": false | |
}, | |
"outputs": [ | |
{ | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>#ID(s) interactor A</th>\n", | |
" <th>ID(s) interactor B</th>\n", | |
" <th>Alt. ID(s) interactor A</th>\n", | |
" <th>Alt. ID(s) interactor B</th>\n", | |
" <th>Alias(es) interactor A</th>\n", | |
" <th>Alias(es) interactor B</th>\n", | |
" <th>Interaction detection method(s)</th>\n", | |
" <th>Publication 1st author(s)</th>\n", | |
" <th>Publication Identifier(s)</th>\n", | |
" <th>Taxid interactor A</th>\n", | |
" <th>...</th>\n", | |
" <th>Checksum(s) interactor A</th>\n", | |
" <th>Checksum(s) interactor B</th>\n", | |
" <th>Interaction Checksum(s)</th>\n", | |
" <th>Negative</th>\n", | |
" <th>Feature(s) interactor A</th>\n", | |
" <th>Feature(s) interactor B</th>\n", | |
" <th>Stoichiometry(s) interactor A</th>\n", | |
" <th>Stoichiometry(s) interactor B</th>\n", | |
" <th>Identification method participant A</th>\n", | |
" <th>Identification method participant B</th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>0</th>\n", | |
" <td>uniprotkb:Q8IZC7</td>\n", | |
" <td>uniprotkb:P11802</td>\n", | |
" <td>intact:EBI-5278328|uniprotkb:C9JU83|uniprotkb:...</td>\n", | |
" <td>intact:EBI-295644|uniprotkb:B2R9A0|uniprotkb:B...</td>\n", | |
" <td>psi-mi:zn101_human(display_long)|uniprotkb:ZNF...</td>\n", | |
" <td>psi-mi:cdk4_human(display_long)|uniprotkb:CDK4...</td>\n", | |
" <td>psi-mi:\"MI:0424\"(protein kinase assay)</td>\n", | |
" <td>Anders et al. (2011)</td>\n", | |
" <td>pubmed:22094256|imex:IM-16628</td>\n", | |
" <td>taxid:9606(human)|taxid:9606(Homo sapiens)</td>\n", | |
" <td>...</td>\n", | |
" <td>rogid:mZLXs/PbWbfgLzkjwZfoE2c6jy09606</td>\n", | |
" <td>rogid:pxIzF9EkX+gMz7zj/TDiytYtsOc9606</td>\n", | |
" <td>intact-crc:0486DE729E5A8158|rigid:3gdrHm0coV8S...</td>\n", | |
" <td>False</td>\n", | |
" <td>glutathione s tranferase tag:?-?</td>\n", | |
" <td>sufficient binding region:4-303|glutathione s ...</td>\n", | |
" <td>-</td>\n", | |
" <td>-</td>\n", | |
" <td>psi-mi:\"MI:0821\"(molecular weight estimation b...</td>\n", | |
" <td>psi-mi:\"MI:0821\"(molecular weight estimation b...</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>1</th>\n", | |
" <td>uniprotkb:P01106</td>\n", | |
" <td>uniprotkb:Q00534</td>\n", | |
" <td>intact:EBI-447544|intact:EBI-1058440|uniprotkb...</td>\n", | |
" <td>intact:EBI-295663|uniprotkb:A4D1G0</td>\n", | |
" <td>psi-mi:myc_human(display_long)|uniprotkb:BHLHE...</td>\n", | |
" <td>psi-mi:cdk6_human(display_long)|uniprotkb:CDK6...</td>\n", | |
" <td>psi-mi:\"MI:0424\"(protein kinase assay)</td>\n", | |
" <td>Anders et al. (2011)</td>\n", | |
" <td>pubmed:22094256|imex:IM-16628</td>\n", | |
" <td>taxid:9606(human)|taxid:9606(Homo sapiens)</td>\n", | |
" <td>...</td>\n", | |
" <td>rogid:CCk+p9FN7JV6cTmJDlin2hRp1tg9606</td>\n", | |
" <td>rogid:IlAJCZ9bvB5RaBdS1rlPnqDL9xg9606</td>\n", | |
" <td>intact-crc:20A3DCC8419B269E|rigid:bfSR2lJKa/lY...</td>\n", | |
" <td>False</td>\n", | |
" <td>glutathione s tranferase tag:?-?</td>\n", | |
" <td>his tag:n-n</td>\n", | |
" <td>-</td>\n", | |
" <td>-</td>\n", | |
" <td>psi-mi:\"MI:0821\"(molecular weight estimation b...</td>\n", | |
" <td>psi-mi:\"MI:0821\"(molecular weight estimation b...</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>2</th>\n", | |
" <td>uniprotkb:Q00534</td>\n", | |
" <td>uniprotkb:P28698</td>\n", | |
" <td>intact:EBI-295663|uniprotkb:A4D1G0</td>\n", | |
" <td>intact:EBI-5278283|uniprotkb:M0QXU0|uniprotkb:...</td>\n", | |
" <td>psi-mi:cdk6_human(display_long)|uniprotkb:CDK6...</td>\n", | |
" <td>psi-mi:mzf1_human(display_long)|uniprotkb:MZF1...</td>\n", | |
" <td>psi-mi:\"MI:0424\"(protein kinase assay)</td>\n", | |
" <td>Anders et al. (2011)</td>\n", | |
" <td>pubmed:22094256|imex:IM-16628</td>\n", | |
" <td>taxid:9606(human)|taxid:9606(Homo sapiens)</td>\n", | |
" <td>...</td>\n", | |
" <td>rogid:IlAJCZ9bvB5RaBdS1rlPnqDL9xg9606</td>\n", | |
" <td>rogid:1rq+ga/Uew705r63KlORpqBObbw9606</td>\n", | |
" <td>intact-crc:578214FA683591D0|rigid:8AADqx3NNAXF...</td>\n", | |
" <td>False</td>\n", | |
" <td>his tag:n-n</td>\n", | |
" <td>glutathione s tranferase tag:?-?</td>\n", | |
" <td>-</td>\n", | |
" <td>-</td>\n", | |
" <td>psi-mi:\"MI:0821\"(molecular weight estimation b...</td>\n", | |
" <td>psi-mi:\"MI:0821\"(molecular weight estimation b...</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"<p>3 rows × 42 columns</p>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" #ID(s) interactor A ID(s) interactor B \\\n", | |
"0 uniprotkb:Q8IZC7 uniprotkb:P11802 \n", | |
"1 uniprotkb:P01106 uniprotkb:Q00534 \n", | |
"2 uniprotkb:Q00534 uniprotkb:P28698 \n", | |
"\n", | |
" Alt. ID(s) interactor A \\\n", | |
"0 intact:EBI-5278328|uniprotkb:C9JU83|uniprotkb:... \n", | |
"1 intact:EBI-447544|intact:EBI-1058440|uniprotkb... \n", | |
"2 intact:EBI-295663|uniprotkb:A4D1G0 \n", | |
"\n", | |
" Alt. ID(s) interactor B \\\n", | |
"0 intact:EBI-295644|uniprotkb:B2R9A0|uniprotkb:B... \n", | |
"1 intact:EBI-295663|uniprotkb:A4D1G0 \n", | |
"2 intact:EBI-5278283|uniprotkb:M0QXU0|uniprotkb:... \n", | |
"\n", | |
" Alias(es) interactor A \\\n", | |
"0 psi-mi:zn101_human(display_long)|uniprotkb:ZNF... \n", | |
"1 psi-mi:myc_human(display_long)|uniprotkb:BHLHE... \n", | |
"2 psi-mi:cdk6_human(display_long)|uniprotkb:CDK6... \n", | |
"\n", | |
" Alias(es) interactor B \\\n", | |
"0 psi-mi:cdk4_human(display_long)|uniprotkb:CDK4... \n", | |
"1 psi-mi:cdk6_human(display_long)|uniprotkb:CDK6... \n", | |
"2 psi-mi:mzf1_human(display_long)|uniprotkb:MZF1... \n", | |
"\n", | |
" Interaction detection method(s) Publication 1st author(s) \\\n", | |
"0 psi-mi:\"MI:0424\"(protein kinase assay) Anders et al. (2011) \n", | |
"1 psi-mi:\"MI:0424\"(protein kinase assay) Anders et al. (2011) \n", | |
"2 psi-mi:\"MI:0424\"(protein kinase assay) Anders et al. (2011) \n", | |
"\n", | |
" Publication Identifier(s) Taxid interactor A \\\n", | |
"0 pubmed:22094256|imex:IM-16628 taxid:9606(human)|taxid:9606(Homo sapiens) \n", | |
"1 pubmed:22094256|imex:IM-16628 taxid:9606(human)|taxid:9606(Homo sapiens) \n", | |
"2 pubmed:22094256|imex:IM-16628 taxid:9606(human)|taxid:9606(Homo sapiens) \n", | |
"\n", | |
" ... \\\n", | |
"0 ... \n", | |
"1 ... \n", | |
"2 ... \n", | |
"\n", | |
" Checksum(s) interactor A \\\n", | |
"0 rogid:mZLXs/PbWbfgLzkjwZfoE2c6jy09606 \n", | |
"1 rogid:CCk+p9FN7JV6cTmJDlin2hRp1tg9606 \n", | |
"2 rogid:IlAJCZ9bvB5RaBdS1rlPnqDL9xg9606 \n", | |
"\n", | |
" Checksum(s) interactor B \\\n", | |
"0 rogid:pxIzF9EkX+gMz7zj/TDiytYtsOc9606 \n", | |
"1 rogid:IlAJCZ9bvB5RaBdS1rlPnqDL9xg9606 \n", | |
"2 rogid:1rq+ga/Uew705r63KlORpqBObbw9606 \n", | |
"\n", | |
" Interaction Checksum(s) Negative \\\n", | |
"0 intact-crc:0486DE729E5A8158|rigid:3gdrHm0coV8S... False \n", | |
"1 intact-crc:20A3DCC8419B269E|rigid:bfSR2lJKa/lY... False \n", | |
"2 intact-crc:578214FA683591D0|rigid:8AADqx3NNAXF... False \n", | |
"\n", | |
" Feature(s) interactor A \\\n", | |
"0 glutathione s tranferase tag:?-? \n", | |
"1 glutathione s tranferase tag:?-? \n", | |
"2 his tag:n-n \n", | |
"\n", | |
" Feature(s) interactor B \\\n", | |
"0 sufficient binding region:4-303|glutathione s ... \n", | |
"1 his tag:n-n \n", | |
"2 glutathione s tranferase tag:?-? \n", | |
"\n", | |
" Stoichiometry(s) interactor A Stoichiometry(s) interactor B \\\n", | |
"0 - - \n", | |
"1 - - \n", | |
"2 - - \n", | |
"\n", | |
" Identification method participant A \\\n", | |
"0 psi-mi:\"MI:0821\"(molecular weight estimation b... \n", | |
"1 psi-mi:\"MI:0821\"(molecular weight estimation b... \n", | |
"2 psi-mi:\"MI:0821\"(molecular weight estimation b... \n", | |
"\n", | |
" Identification method participant B \n", | |
"0 psi-mi:\"MI:0821\"(molecular weight estimation b... \n", | |
"1 psi-mi:\"MI:0821\"(molecular weight estimation b... \n", | |
"2 psi-mi:\"MI:0821\"(molecular weight estimation b... \n", | |
"\n", | |
"[3 rows x 42 columns]" | |
] | |
}, | |
"execution_count": 12, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"# By default, it uses 'source' for source node column, 'target' for target node column, and 'interaction' for interaction\n", | |
"yeast1 = cy.network.create_from_dataframe(df_from_sif, name='Yeast network created from pandas DataFrame')\n", | |
"\n", | |
"# Example 2: from more complicated table\n", | |
"df_from_mitab = pd.read_csv('data/intact_pubid_22094256.txt', sep='\\t')\n", | |
"df_from_mitab.head(3)" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 13, | |
"metadata": { | |
"collapsed": true | |
}, | |
"outputs": [], | |
"source": [ | |
"source = df_from_mitab.columns[0]\n", | |
"target = df_from_mitab.columns[1]\n", | |
"interaction = 'Interaction identifier(s)'\n", | |
"title='A Systematic Screen for CDK4/6 Substrates Links FOXM1 Phosphorylation to Senescence Suppression in Cancer Cells.'\n", | |
"\n", | |
"human1 = cy.network.create_from_dataframe(df_from_mitab, source_col=source, target_col=target, interaction_col=interaction, name=title)\n", | |
"\n", | |
"\n", | |
"# Import edge attributes and node attributes at the same time (TBD)" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 14, | |
"metadata": { | |
"collapsed": true | |
}, | |
"outputs": [], | |
"source": [ | |
"# NetworkX\n", | |
"nx_graph = nx.scale_free_graph(100)\n", | |
"nx.set_node_attributes(nx_graph, 'Degree', nx.degree(nx_graph))\n", | |
"nx.set_node_attributes(nx_graph, 'Betweenness_Centrality', nx.betweenness_centrality(nx_graph))\n", | |
"scale_free100 = cy.network.create_from_networkx(nx_graph, collection='Generated by NetworkX')\n", | |
"\n", | |
"# TODO: igraph\n", | |
"# TODO: Numpy adj. martix\n", | |
"# TODO: GraphX" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"## Get Network from Cytoscape\n", | |
"\n", | |
"You can get network data in the following forms:\n", | |
"\n", | |
"* Cytoscape.js\n", | |
"* NetworkX\n", | |
"* DataFrame" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 15, | |
"metadata": { | |
"collapsed": false | |
}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"{\n", | |
" \"elements\": {\n", | |
" \"edges\": [\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDL194W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9345\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8984\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR277C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8983\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9345,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YJR022W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9344\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8681\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR277C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8983\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9344,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR117C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9340\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8962\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPR145W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8981\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9340,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YBR045C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9339\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8979\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER054C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8980\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9339,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YER133W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9338\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8866\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER054C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8980\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9338,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR178C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9337\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8867\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBR045C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8979\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9337,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YIL045W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9336\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8943\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBR045C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8979\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9336,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDL088C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9335\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8965\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBL079W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8978\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9335,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YLR321C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9334\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8835\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLR345W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8977\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9334,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGR058W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9324\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8762\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGR136W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8971\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9324,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YJL159W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9323\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8779\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDL023C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8970\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9323,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGR048W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9320\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8860\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBR170C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8969\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9320,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YBR043C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9343\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8982\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGR074W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8968\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9343,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGR074W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9318\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8968\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGL202W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8967\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9318,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR310C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9317\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8718\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLR197W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8966\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9317,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDL014W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9316\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8717\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLR197W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8966\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9316,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YER110C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9315\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8903\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDL088C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8965\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9315,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPR010C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9309\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8963\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YMR117C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8962\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9309,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YCL032W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9308\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8737\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YMR117C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8962\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9308,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR167W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9307\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8911\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YML114C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8961\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9307,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YIR009W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9306\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8749\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL036W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8960\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9306,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YLR362W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9321\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8739\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YOR212W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8959\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9321,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YFL017C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9303\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8665\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR070C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8958\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9303,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YNL236W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9296\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8754\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGR046W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8956\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9296,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YML054C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9292\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8766\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YIL070C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8954\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9292,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR043W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9291\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8777\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPR113W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8953\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9291,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YIL074C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9290\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8940\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER081W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8952\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9290,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YLR256W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9289\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8765\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGR088W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8951\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9289,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPR102C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9333\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8920\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR395W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8950\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9333,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOL127W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9332\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8704\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR395W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8950\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9332,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YIL052C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9331\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8976\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR395W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8950\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9331,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YER056CA\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9330\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8975\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR395W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8950\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9330,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YNL069C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9329\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8974\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR395W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8950\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9329,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YIL133C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9328\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8695\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR395W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8950\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9328,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDL075W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9327\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8973\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR395W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8950\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9327,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR395W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9287\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8950\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGR085C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8949\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9287,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YLR075W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9286\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8919\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGR085C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8949\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9286,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR005W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9279\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8947\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDL030W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8946\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9279,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDL013W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9278\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8688\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDL030W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8946\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9278,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YNL154C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9277\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8823\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER079W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8945\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9277,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YHR135C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9276\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8820\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER079W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8945\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9276,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YLR432W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9275\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8912\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDL215C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8944\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9275,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YER040W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9274\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8798\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDL215C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8944\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9274,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR309C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9268\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8723\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPR041W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8942\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9268,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR361C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9267\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8722\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPR041W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8942\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9267,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPL248C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9266\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8747\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YOR120W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8941\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9266,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YNL311C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9260\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8844\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YIL074C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8940\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9260,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YJL194W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9259\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8931\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR299W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8939\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9259,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YLR362W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9302\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8739\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YHR005C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8938\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9302,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YHR005C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9258\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8938\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLR452C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8937\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9258,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGL122C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9271\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8655\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YMR255W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8936\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9271,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR255W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9257\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8936\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBR274W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8935\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9257,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YFL026W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9285\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8785\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YHR084W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8934\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9285,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR461W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9284\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8782\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YHR084W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8934\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9284,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR043W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9283\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8777\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YHR084W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8934\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9283,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR036W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9254\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8796\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBL050W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8933\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9254,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR167C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9346\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8680\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBL026W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8932\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9346,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR043W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9250\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8777\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YJL194W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8931\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9250,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YBR274W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9273\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8935\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLR258W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8930\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9273,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YIL045W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9272\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8943\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLR258W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8930\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9272,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YLR258W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9249\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8930\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGL134W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8929\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9249,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPL031C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9248\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8879\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGL134W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8929\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9248,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR021C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9245\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8810\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPR124W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8926\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9245,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR174W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9269\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8813\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL135C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8925\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9269,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YNL135C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9244\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8925\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER052C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8924\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9244,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR036W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9239\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8796\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPL240C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8921\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9239,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YBR155W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9238\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8727\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPL240C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8921\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9238,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPR102C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9236\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8920\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLR075W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8919\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9236,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPL089C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9234\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8805\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YKL161C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8918\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9234,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPL111W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9233\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8841\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YAR007C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8917\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9233,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YML032C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9232\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8756\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YAR007C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8917\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9232,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YIL160C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9220\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8916\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR142C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8914\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9220,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGL153W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9219\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8902\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR142C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8914\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9219,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDL078C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9217\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8915\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR244W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8913\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9217,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR142C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9216\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8914\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR244W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8913\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9216,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YNL214W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9215\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8906\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR244W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8913\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9215,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGL153W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9214\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8902\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR244W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8913\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9214,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YLR191W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9213\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8901\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR244W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8913\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9213,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YLR432W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9211\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8912\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR167W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8911\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9211,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YNL307C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9210\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8689\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLR175W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8910\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9210,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YJL089W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9209\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8807\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL117W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8909\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9209,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR323C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9208\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8795\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YOR089C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8908\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9208,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGL153W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9206\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8902\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL214W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8906\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9206,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YER102W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9205\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8897\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBR135W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8905\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9205,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YML007W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9204\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8904\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER110C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8903\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9204,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGL153W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9203\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8902\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLR191W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8901\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9203,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR167C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9200\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8680\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YOL149W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8900\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9200,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YIL061C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9199\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8675\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YMR044W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8899\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9199,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPR110C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9189\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8893\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL113W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8892\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9189,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YEL009C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9188\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8728\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR354W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8891\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9188,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YER090W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9187\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8890\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YKL211C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8889\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9187,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGL035C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9186\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8744\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR146C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8888\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9186,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR043W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9185\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8777\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR146C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8888\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9185,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR043W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9184\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8777\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER111C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8887\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9184,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR303W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9183\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8764\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YOR039W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8886\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9183,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YNL216W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9182\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8691\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YML024W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8885\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9182,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YHR030C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9194\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8806\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YIL113W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8884\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9194,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YIL113W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9181\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8884\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLL019C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8883\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9181,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGL035C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9222\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8744\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR009W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8882\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9222,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR009W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9180\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8882\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YML051W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8881\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9180,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YBR020W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9179\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8745\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YML051W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8881\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9179,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YHR071W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9177\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8880\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPL031C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8879\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9177,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YFR034C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9176\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8863\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YML123C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8878\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9176,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YER145C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9175\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8877\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YMR058W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8876\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9175,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YJL190C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9174\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8875\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YML074C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8874\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9174,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YNL091W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9173\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8753\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YOR355W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8873\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9173,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR036W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9172\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8796\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YFL038C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8872\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9172,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YNL167C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9319\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8852\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YIL162W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8871\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9319,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YBR050C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9168\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8870\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER133W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8866\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9168,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR311C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9167\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8869\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER133W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8866\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9167,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR315W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9166\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8868\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER133W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8866\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9166,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR178C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9165\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8867\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER133W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8866\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9165,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR412W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9164\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8712\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YER133W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8866\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9164,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR290C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9163\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8865\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YFR037C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8864\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9163,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YBR093C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9162\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8701\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YFR034C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8863\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9162,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YMR043W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9161\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8777\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YAL040C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8862\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9161,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPL222W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9160\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8861\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGR048W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8860\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9160,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGL115W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9159\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8803\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YMR291W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8859\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9159,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YBL050W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9314\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8933\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGR009C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8858\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9314,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR335W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9313\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8812\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGR009C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8858\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9313,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR327C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9312\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8709\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGR009C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8858\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9312,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YAL030W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9311\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8707\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGR009C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8858\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9311,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGR009C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9158\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8858\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YMR183C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8857\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9158,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR100W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9157\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8856\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGL161C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8855\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9157,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPL131W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9156\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8854\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDL063C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8853\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9156,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR202W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9155\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8730\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL167C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8852\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9155,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR215C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9310\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8964\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YHR115C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8851\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9310,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YHR115C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9154\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8851\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YEL041W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8850\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9154,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDL113C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9153\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8849\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YJL036W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8848\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9153,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YFR014C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9326\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8972\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBR109C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8847\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9326,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR326W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9325\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8724\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBR109C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8847\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9325,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YBR109C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9152\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8847\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YOL016C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8846\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9152,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YKL001C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9150\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8845\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL311C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8844\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9150,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YLR362W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9261\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8739\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLR319C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8843\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9261,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPR062W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9148\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8842\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL189W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8839\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9148,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YER065C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9147\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8808\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL189W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8839\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9147,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPL111W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9146\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8841\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL189W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8839\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9146,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDL236W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9145\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8840\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL189W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8839\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9145,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGL008C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9144\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8797\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBL069W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8838\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9144,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YER103W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9265\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8894\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGL073W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8837\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9265,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YHR055C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9264\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8928\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGL073W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8837\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9264,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YHR053C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9263\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8927\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGL073W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8837\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9263,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR178C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9262\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8867\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YGL073W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8837\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9262,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGL073W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9142\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8837\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YBR072W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8836\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9142,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR412W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9141\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8712\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YLR321C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8835\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9141,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDL215C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9301\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8944\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPR048W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8834\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9301,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOR355W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9300\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8873\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPR048W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8834\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9300,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YPR048W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9139\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8834\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YNL199C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8833\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9139,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YOL086C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9138\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8706\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPL075W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8832\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9138,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDR050C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9137\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8705\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPL075W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8832\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9137,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YNL199C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9136\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8833\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPL075W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8832\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9136,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YHR174W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9135\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8694\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPL075W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8832\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9135,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YGR254W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9134\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8693\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPL075W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8832\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9134,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YCR012W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pd\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pd\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9133\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8692\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YPL075W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8832\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9133,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YHR179W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9132\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8831\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YFL039C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8829\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9132,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YCL040W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9131\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8830\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YFL039C\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8829\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9131,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YFL039C\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9130\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8829\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR382W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8827\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9130,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YDL130W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9129\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8828\",\n", | |
" \"Source_Participant_Detection_Method_ID\": [],\n", | |
" \"source_original\": \"YDR382W\",\n", | |
" \"Source_Experimental_Role\": [],\n", | |
" \"Target_Biological_Role_ID\": [],\n", | |
" \"source\": \"8827\",\n", | |
" \"Target_Participant_Detection_Method_ID\": [],\n", | |
" \"Detection_Method_ID\": [],\n", | |
" \"SUID\": 9129,\n", | |
" \"Annotation\": []\n", | |
" }\n", | |
" },\n", | |
" {\n", | |
" \"data\": {\n", | |
" \"target_original\": \"YLR116W\",\n", | |
" \"Interaction_Type\": [],\n", | |
" \"Host_Organism_Taxonomy\": [],\n", | |
" \"interaction\": \"pp\",\n", | |
" \"Source_Biological_Role\": [],\n", | |
" \"Source_Biological_Role_ID\": [],\n", | |
" \"shared_interaction\": \"pp\",\n", | |
" \"Complex_Expansion\": [],\n", | |
" \"Detection_Method\": [],\n", | |
" \"Complex_Expansion_ID\": [],\n", | |
" \"Source_Participant_Detection_Method\": [],\n", | |
" \"Publication_DB\": [],\n", | |
" \"Source_Experimental_Role_ID\": [],\n", | |
" \"Host_Organism_Taxonomy_ID\": [],\n", | |
" \"Target_Participant_Detection_Method\": [],\n", | |
" \"Target_Experimental_Role_ID\": [],\n", | |
" \"selected\": false,\n", | |
" \"Author\": [],\n", | |
" \"Xref\": [],\n", | |
" \"Publication_ID\": [],\n", | |
" \"Xref_ID\": [],\n", | |
" \"id\": \"9128\",\n", | |
" \"Target_Biological_Role\": [],\n", | |
" \"Target_Experimental_Role\": [],\n", | |
" \"target\": \"8758\",\n", | |
" |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment