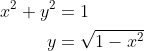
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from elasticsearch import Elasticsearch | |
import sys | |
indexes = sys.argv[1:] | |
client = Elasticsearch('host:port') | |
recovery = client.indices.recovery(",".join(indexes), human=True) | |
for index, state in recovery.items(): |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from elasticsearch import Elasticsearch | |
from elasticsearch.helpers import parallel_bulk, scan | |
import json | |
import time | |
import codecs | |
import logging | |
import sys | |
logging.basicConfig(stream=sys.stdout, level=logging.INFO) | |
logging.info("start reindex") |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package test; | |
import java.util.ArrayList; | |
import java.util.List; | |
public final class Currency { | |
public static final Currency EU = new Currency("EU"); | |
public static final Currency USD = new Currency("USD"); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package test; | |
public class Test1 { | |
public static class Node { | |
private Node parent; | |
private Node right; | |
private Node left; | |
private int value; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
int[] a = [1, 2, 3, 4, 5, 6, 7, 8, 9] | |
int[] b = [1, 2, 3, 4, 9, 8, 7, 6, 5] | |
int[] e = [9, 1, 8, 2, 7, 3, 6, 4, 5] | |
int[] f = [9, 8, 7, 6, 5, 4, 3, 2, 1] | |
int[] c = [1, 2, 3, 4, 5, 6, 7, 8, 10] | |
int[] d = [1, 2, 3, 4, 5, 6, 7, 8, 8] | |
int[] g = [1, 8, 3, 4, 5, 6, 7, 8, 8] | |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# init | |
# L - error function | |
def gbm_L(y, z): | |
return (y-z)**2 | |
# derivative of L function without coefficient `2` | |
def dbm_L_der(y, z): | |
# ignore coefficient 2 | |
return y - z |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
$ go test -bench=. | |
testing: warning: no tests to run | |
PASS | |
BenchmarkJsonReflection-8 100000 14689 ns/op | |
BenchmarkFFJson-8 200000 6345 ns/op | |
ok github.com/mitallast/goprotoserver/protocol 2.974s |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import pandas_datareader.data as web | |
import matplotlib.pyplot as plt | |
from sklearn.linear_model import LinearRegression | |
import numpy as np | |
import datetime | |
start = datetime.datetime(2010, 1, 1) | |
end = datetime.datetime(2015, 3, 8) | |
usd = web.DataReader("USD", 'google', start, end) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from scikits.audiolab import Sndfile | |
import numpy as np | |
import matplotlib.pyplot as plt | |
import scipy.signal | |
f = Sndfile('impulse.wav', 'r') | |
data = np.array(f.read_frames(f.nframes), dtype=np.float64) | |
f.close() | |
fs = f.samplerate |