Last active
October 18, 2023 21:26
-
-
Save Anthelmed/502731b64fbc1158f86f12d08b1c74bc to your computer and use it in GitHub Desktop.
A Texture3D SDF function to be used inside Unity shader graph
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#ifndef RAYMARCHSDFINCLUDE_INCLUDED | |
#define RAYMARCHSDFINCLUDE_INCLUDED | |
#define STEPS 256 | |
#define MAX_DISTANCE 500 | |
#define MIN_DISTANCE 0.001 | |
#if !SHADERGRAPH_PREVIEW | |
#include "Packages/com.unity.render-pipelines.core/ShaderLibrary/Texture.hlsl" | |
#include "Packages/com.unity.render-pipelines.universal/ShaderLibrary/Core.hlsl" | |
#endif | |
float3 Normal(float3 position, UnityTexture3D Texture, UnitySamplerState Sampler) | |
{ | |
float2 e = float2(1.0,-1.0) * 0.5773; | |
const float eps = 0.0005; | |
return normalize(e.xyy * SAMPLE_TEXTURE3D(Texture, Sampler, position + e.xyy*eps) + | |
e.yyx * SAMPLE_TEXTURE3D(Texture, Sampler, position + e.yyx*eps) + | |
e.yxy * SAMPLE_TEXTURE3D(Texture, Sampler, position + e.yxy*eps) + | |
e.xxx * SAMPLE_TEXTURE3D(Texture, Sampler, position + e.xxx*eps)); | |
} | |
void Raymarch_float(float3 RayOrigin, float3 RayDirection, UnityTexture3D Texture, UnitySamplerState Sampler, float3 PositionOffset, out float3 OutPosition, out float OutDistance, out float3 OutNormal) | |
{ | |
float distanceOrigin = 0; | |
float distanceSurface; | |
for (int i = 0; i < STEPS; i++) | |
{ | |
float3 position = RayOrigin + distanceOrigin * RayDirection + PositionOffset; | |
float4 map = SAMPLE_TEXTURE3D(Texture, Sampler, position); | |
distanceSurface = map; | |
distanceOrigin += distanceSurface; | |
if (distanceSurface < MIN_DISTANCE || distanceOrigin > MAX_DISTANCE) break; | |
} | |
if (distanceOrigin < MAX_DISTANCE) | |
{ | |
OutPosition = RayOrigin + distanceOrigin * RayDirection + PositionOffset; | |
OutDistance = distanceOrigin; | |
OutNormal = Normal(OutPosition, Texture, Sampler); | |
} | |
else | |
{ | |
OutPosition = float3(0,0,0); | |
OutDistance = 0; | |
OutNormal = float3(0,0,0); | |
} | |
} | |
#endif |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Shader graph example:
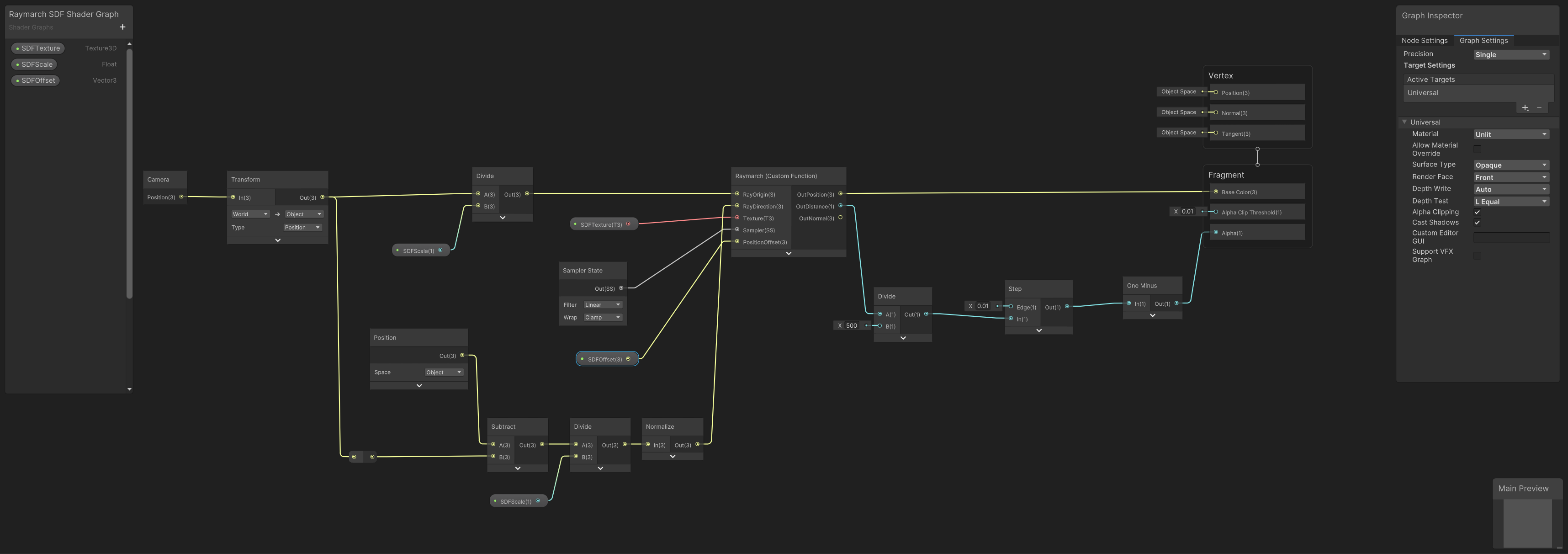