Created
October 21, 2021 00:43
-
-
Save CodySelman/f1075d8ec5530bb1c6ad26bd39d102c2 to your computer and use it in GitHub Desktop.
Editor Tool for Unity that allows for easy bulk Spritesheet slicing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System.Collections.Generic; | |
using UnityEditor; | |
using UnityEngine; | |
public class SpritesheetSlicer : ScriptableWizard | |
{ | |
public bool mipmapEnabled = false; | |
public FilterMode filterMode = FilterMode.Point; | |
public Vector2 spritePivot = Vector2.zero; | |
public TextureImporterCompression textureImporterCompression = TextureImporterCompression.Uncompressed; | |
public SpriteMeshType spriteMeshType = SpriteMeshType.Tight; | |
public uint spriteExtrude = 0; | |
public int spriteHeight = 32; | |
public int spriteWidth = 32; | |
[MenuItem("Tools/Slice Spritesheets %&s")] | |
static void CreateWizard() | |
{ | |
ScriptableWizard.DisplayWizard<SpritesheetSlicer>("Slice Spritesheets", "Slice"); | |
} | |
void OnWizardCreate() | |
{ | |
Slice(); | |
} | |
void Slice() | |
{ | |
var textures = Selection.GetFiltered<Texture2D>(SelectionMode.Assets); | |
if (textures.Length == 0) { | |
Debug.LogError("No spritesheets selected. This tool works by selecting a spritesheet in the editor."); | |
} else { | |
foreach (var texture in textures) | |
{ | |
ProcessTexture(texture); | |
} | |
} | |
} | |
void ProcessTexture(Texture2D texture) | |
{ | |
string path = AssetDatabase.GetAssetPath(texture); | |
var importer = AssetImporter.GetAtPath(path) as TextureImporter; | |
//importer.isReadable = true; | |
importer.textureType = TextureImporterType.Sprite; | |
importer.spriteImportMode = SpriteImportMode.Multiple; | |
importer.mipmapEnabled = mipmapEnabled; | |
importer.filterMode = filterMode; | |
importer.spritePivot = spritePivot; | |
importer.textureCompression = textureImporterCompression; | |
// need this class because spriteExtrude and spriteMeshType aren't exposed on TextureImporter | |
var textureSettings = new TextureImporterSettings(); | |
importer.ReadTextureSettings(textureSettings); | |
textureSettings.spriteMeshType = spriteMeshType; | |
textureSettings.spriteExtrude = spriteExtrude; | |
importer.SetTextureSettings(textureSettings); | |
int colCount = texture.width / spriteWidth; | |
int rowCount = texture.height / spriteHeight; | |
List<SpriteMetaData> metas = new List<SpriteMetaData>(); | |
for (int r = 0; r < rowCount; ++r) | |
{ | |
for (int c = 0; c < colCount; ++c) | |
{ | |
SpriteMetaData meta = new SpriteMetaData(); | |
meta.rect = new Rect(c * spriteWidth, r * spriteHeight, spriteWidth, spriteHeight); | |
meta.name = c + "-" + r; | |
metas.Add(meta); | |
} | |
} | |
importer.spritesheet = metas.ToArray(); | |
AssetDatabase.ImportAsset(path, ImportAssetOptions.ForceUpdate); | |
AssetDatabase.Refresh(); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Please note that this tool slices by CellSize. It won't work for "Slice Type: Automatic" but in my experience, that's usually not the option people use.
How to use it:
Select any number of spritesheets in the Project tab:
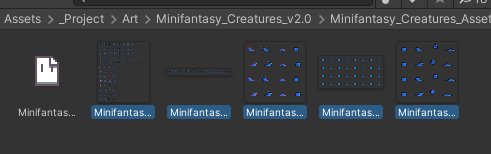
The tool creates a Menu Item under Tools/Slice Spritesheets. Click that Menu Item (or use the Ctrl + Alt + S hotkey) to open the Wizard.
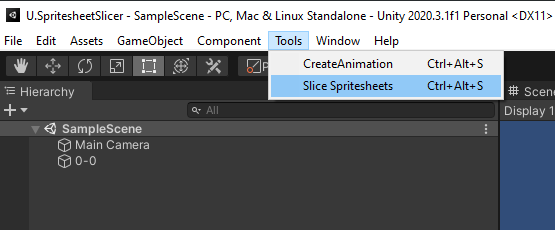
Select your Slicing options in the Wizard:
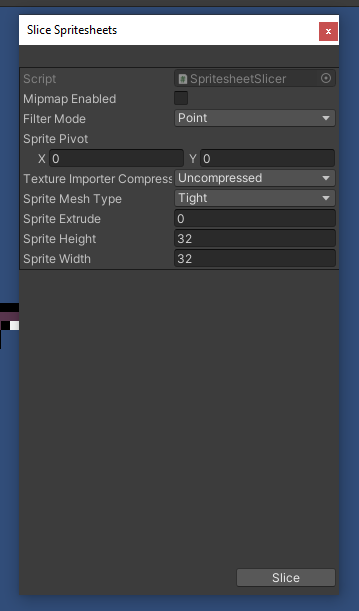
Click Slice in the above Wizard.
And that's all there is to it. This works for my use-case, but I can imagine some projects would need different options surfaced or hidden in the wizard. Let me know in the comments if you'd like to see any modifications.