Last active
January 20, 2021 17:52
-
-
Save FedericoPonzi/1728542ae0c8057e43658a3216e385c5 to your computer and use it in GitHub Desktop.
Find thresholds for hsv for opencv (inRange opencv function) using your webcam. From: https://raw.githubusercontent.com/saurabheights/IPExperimentTools/master/AnalyzeHSV/hsvThresholder.py
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# Preview: https://raw.githubusercontent.com/FedericoPonzi/LegoLab/master/media/hsv-colour.png | |
import cv2 | |
import sys | |
import numpy as np | |
def nothing(x): | |
pass | |
useCamera=False | |
# Check if filename is passed | |
if (len(sys.argv) <= 1) : | |
print ("'Usage: python hsvThresholder.py <ImageFilePath>' to ignore camera and use a local image.") | |
useCamera = True | |
# Create a window | |
cv2.namedWindow('image') | |
# create trackbars for color change | |
cv2.createTrackbar('HMin','image',0,179,nothing) # Hue is from 0-179 for Opencv | |
cv2.createTrackbar('SMin','image',0,255,nothing) | |
cv2.createTrackbar('VMin','image',0,255,nothing) | |
cv2.createTrackbar('HMax','image',0,179,nothing) | |
cv2.createTrackbar('SMax','image',0,255,nothing) | |
cv2.createTrackbar('VMax','image',0,255,nothing) | |
# Set default value for MAX HSV trackbars. | |
cv2.setTrackbarPos('HMax', 'image', 179) | |
cv2.setTrackbarPos('SMax', 'image', 255) | |
cv2.setTrackbarPos('VMax', 'image', 255) | |
# Initialize to check if HSV min/max value changes | |
hMin = sMin = vMin = hMax = sMax = vMax = 0 | |
phMin = psMin = pvMin = phMax = psMax = pvMax = 0 | |
# Output Image to display | |
if useCamera: | |
cap = cv2.VideoCapture(0) | |
# Wait longer to prevent freeze for videos. | |
waitTime = 330 | |
else: | |
img = cv2.imread(sys.argv[1]) | |
output = img | |
waitTime = 33 | |
while(1): | |
if useCamera: | |
# Capture frame-by-frame | |
ret, img = cap.read() | |
output = img | |
# get current positions of all trackbars | |
hMin = cv2.getTrackbarPos('HMin','image') | |
sMin = cv2.getTrackbarPos('SMin','image') | |
vMin = cv2.getTrackbarPos('VMin','image') | |
hMax = cv2.getTrackbarPos('HMax','image') | |
sMax = cv2.getTrackbarPos('SMax','image') | |
vMax = cv2.getTrackbarPos('VMax','image') | |
# Set minimum and max HSV values to display | |
lower = np.array([hMin, sMin, vMin]) | |
upper = np.array([hMax, sMax, vMax]) | |
# Create HSV Image and threshold into a range. | |
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV) | |
mask = cv2.inRange(hsv, lower, upper) | |
output = cv2.bitwise_and(img,img, mask= mask) | |
# Print if there is a change in HSV value | |
if( (phMin != hMin) | (psMin != sMin) | (pvMin != vMin) | (phMax != hMax) | (psMax != sMax) | (pvMax != vMax) ): | |
print("(hMin = %d , sMin = %d, vMin = %d), (hMax = %d , sMax = %d, vMax = %d)" % (hMin , sMin , vMin, hMax, sMax , vMax)) | |
phMin = hMin | |
psMin = sMin | |
pvMin = vMin | |
phMax = hMax | |
psMax = sMax | |
pvMax = vMax | |
# Display output image | |
cv2.imshow('image',output) | |
# Wait longer to prevent freeze for videos. | |
if cv2.waitKey(waitTime) & 0xFF == ord('q'): | |
break | |
# Release resources | |
if useCamera: | |
cap.release() | |
cv2.destroyAllWindows() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Preview (this was my shirt):
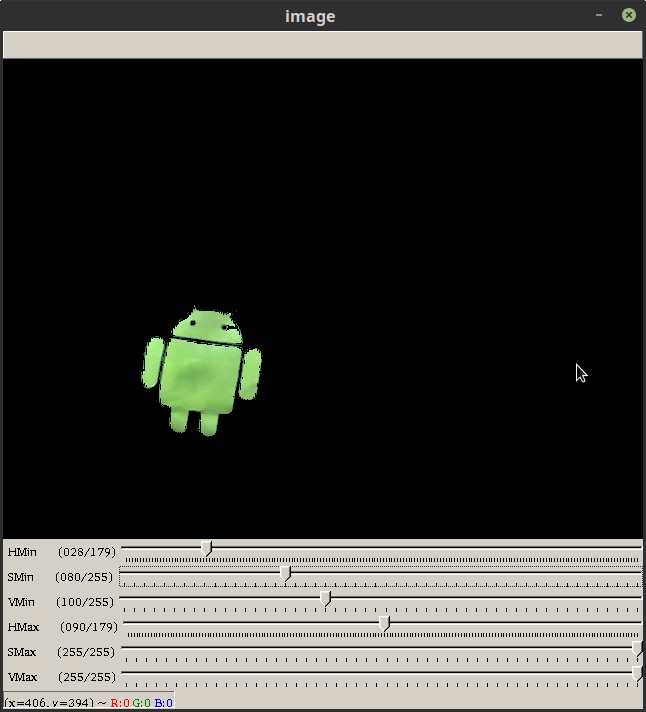