Last active
May 10, 2024 05:47
-
-
Save JCBuck/640fd3844f7a7d2508a19ccbd293eab9 to your computer and use it in GitHub Desktop.
Windows and Linux Scripts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
@title hotkeymacroF15&mode con:cols=38 lines=2&powershell.exe -ExecutionPolicy Bypass -Command "$_=((Get-Content \"%~f0\") -join \"`n\");iex $_.Substring($_.IndexOf(\"goto :\"+\"EOF\")+9)" | |
@goto :EOF | |
################################## | |
################################## | |
################################## | |
$keyToBind = 'V' # Change key to emulate F15 press | |
################################## | |
################################## | |
################################## | |
write-host "'$keyToBind' bound to F15" | |
Function KeyPress { | |
Param ( | |
#[Parameter(position=0, mandatory=$true, parametersetname='key')] | |
#[char]$Key, | |
[Parameter(position=0, mandatory=$true, parametersetname='scancode')] | |
[int]$ScanCode, | |
[Parameter(position=1, mandatory=$true, parametersetname='scancode')] | |
[bool]$isDown | |
) | |
$code = @" | |
using System; | |
using System.Collections.Generic; | |
using System.Runtime.InteropServices; | |
public static class KBEmulator { | |
public enum InputType : uint { | |
INPUT_MOUSE = 0, | |
INPUT_KEYBOARD = 1, | |
INPUT_HARDWARE = 3 | |
} | |
[Flags] | |
internal enum KEYEVENTF : uint | |
{ | |
KEYDOWN = 0x0, | |
EXTENDEDKEY = 0x0001, | |
KEYUP = 0x0002, | |
SCANCODE = 0x0008, | |
UNICODE = 0x0004 | |
} | |
[Flags] | |
internal enum MOUSEEVENTF : uint | |
{ | |
ABSOLUTE = 0x8000, | |
HWHEEL = 0x01000, | |
MOVE = 0x0001, | |
MOVE_NOCOALESCE = 0x2000, | |
LEFTDOWN = 0x0002, | |
LEFTUP = 0x0004, | |
RIGHTDOWN = 0x0008, | |
RIGHTUP = 0x0010, | |
MIDDLEDOWN = 0x0020, | |
MIDDLEUP = 0x0040, | |
VIRTUALDESK = 0x4000, | |
WHEEL = 0x0800, | |
XDOWN = 0x0080, | |
XUP = 0x0100 | |
} | |
// Master Input structure | |
[StructLayout(LayoutKind.Sequential)] | |
public struct lpInput { | |
internal InputType type; | |
internal InputUnion Data; | |
internal static int Size { get { return Marshal.SizeOf(typeof(lpInput)); } } | |
} | |
// Union structure | |
[StructLayout(LayoutKind.Explicit)] | |
internal struct InputUnion { | |
[FieldOffset(0)] | |
internal MOUSEINPUT mi; | |
[FieldOffset(0)] | |
internal KEYBDINPUT ki; | |
[FieldOffset(0)] | |
internal HARDWAREINPUT hi; | |
} | |
// Input Types | |
[StructLayout(LayoutKind.Sequential)] | |
internal struct MOUSEINPUT | |
{ | |
internal int dx; | |
internal int dy; | |
internal int mouseData; | |
internal MOUSEEVENTF dwFlags; | |
internal uint time; | |
internal UIntPtr dwExtraInfo; | |
} | |
[StructLayout(LayoutKind.Sequential)] | |
internal struct KEYBDINPUT | |
{ | |
internal short wVk; | |
internal short wScan; | |
internal KEYEVENTF dwFlags; | |
internal int time; | |
internal UIntPtr dwExtraInfo; | |
} | |
[StructLayout(LayoutKind.Sequential)] | |
internal struct HARDWAREINPUT | |
{ | |
internal int uMsg; | |
internal short wParamL; | |
internal short wParamH; | |
} | |
private class unmanaged { | |
[DllImport("user32.dll", SetLastError = true)] | |
internal static extern uint SendInput ( | |
uint cInputs, | |
[MarshalAs(UnmanagedType.LPArray)] | |
lpInput[] inputs, | |
int cbSize | |
); | |
[DllImport("user32.dll", CharSet = CharSet.Unicode, SetLastError = true)] | |
public static extern short VkKeyScan(char ch); | |
} | |
internal static short VkKeyScan(char ch) { | |
return unmanaged.VkKeyScan(ch); | |
} | |
internal static uint SendInput(uint cInputs, lpInput[] inputs, int cbSize) { | |
return unmanaged.SendInput(cInputs, inputs, cbSize); | |
} | |
public static void SendScanCodeDown(short scanCode) { | |
lpInput[] KeyInputs = new lpInput[1]; | |
lpInput KeyInput = new lpInput(); | |
// Generic Keyboard Event | |
KeyInput.type = InputType.INPUT_KEYBOARD; | |
KeyInput.Data.ki.wScan = 0; | |
KeyInput.Data.ki.time = 0; | |
KeyInput.Data.ki.dwExtraInfo = UIntPtr.Zero; | |
// Push the correct key | |
KeyInput.Data.ki.wVk = scanCode; | |
KeyInput.Data.ki.dwFlags = KEYEVENTF.KEYDOWN; | |
KeyInputs[0] = KeyInput; | |
SendInput(1, KeyInputs, lpInput.Size); | |
return; | |
} | |
public static void SendScanCodeUp(short scanCode) { | |
lpInput[] KeyInputs = new lpInput[1]; | |
lpInput KeyInput = new lpInput(); | |
// Generic Keyboard Event | |
KeyInput.type = InputType.INPUT_KEYBOARD; | |
KeyInput.Data.ki.wScan = 0; | |
KeyInput.Data.ki.time = 0; | |
KeyInput.Data.ki.dwExtraInfo = UIntPtr.Zero; | |
// Release the key | |
KeyInput.Data.ki.wVk = scanCode; | |
KeyInput.Data.ki.dwFlags = KEYEVENTF.KEYUP; | |
KeyInputs[0] = KeyInput; | |
SendInput(1, KeyInputs, lpInput.Size); | |
return; | |
} | |
} | |
"@ | |
if(([System.AppDomain]::CurrentDomain.GetAssemblies() | ?{$_ -match "KBEmulator"}) -eq $null) { | |
Add-Type -TypeDefinition $code | |
} | |
switch($PSCmdlet.ParameterSetName){ | |
#'key' { [KBEmulator]::SendKeyboard($Key) } | |
'scancode' { if($isDown) { [KBEmulator]::SendScanCodeDown($ScanCode) } else { [KBEmulator]::SendScanCodeUp($ScanCode) }} | |
} | |
} | |
Add-Type -TypeDefinition ' | |
using System; | |
using System.IO; | |
using System.Diagnostics; | |
using System.Runtime.InteropServices; | |
using System.Windows.Forms; | |
namespace PowerShell { | |
public static class KeyLogger { | |
private const int WH_KEYBOARD_LL = 13; | |
private const int WM_KEYDOWN = 0x0100; | |
private const int WM_KEYUP = 0x0101; | |
private delegate IntPtr HookProc(int nCode, IntPtr wParam, IntPtr lParam); | |
private static Action<Keys,bool> keyCallback; | |
private static IntPtr hookId = IntPtr.Zero; | |
private static HookProc hookcallback; | |
[DllImport("user32.dll")] | |
private static extern IntPtr CallNextHookEx(IntPtr hhk, int nCode, IntPtr wParam, IntPtr lParam); | |
[DllImport("user32.dll")] | |
private static extern bool UnhookWindowsHookEx(IntPtr hhk); | |
[DllImport("user32.dll")] | |
private static extern IntPtr SetWindowsHookEx(int idHook, HookProc lpfn, IntPtr hMod, uint dwThreadId); | |
[DllImport("kernel32.dll")] | |
private static extern IntPtr GetModuleHandle(string lpModuleName); | |
public static void Run(Action<Keys,bool> callback) { | |
keyCallback = callback; | |
hookcallback = HookCallback; | |
hookId = SetHook(); | |
Application.Run(); | |
UnhookWindowsHookEx(hookId); | |
} | |
private static IntPtr SetHook() { | |
IntPtr moduleHandle = GetModuleHandle(Process.GetCurrentProcess().MainModule.ModuleName); | |
return SetWindowsHookEx(WH_KEYBOARD_LL, hookcallback, moduleHandle, 0); | |
//return SetWindowsHookEx(WH_KEYBOARD_LL, HookCallback, moduleHandle, 0); | |
//must make an instance variable reference or else gc will cause null exception crash | |
} | |
private static IntPtr HookCallback(int nCode, IntPtr wParam, IntPtr lParam) { | |
if (nCode >= 0 && wParam == (IntPtr)WM_KEYDOWN) { | |
var key = (Keys)Marshal.ReadInt32(lParam); | |
keyCallback(key,true); | |
} | |
if (nCode >= 0 && wParam == (IntPtr)WM_KEYUP) { | |
var key = (Keys)Marshal.ReadInt32(lParam); | |
keyCallback(key,false); | |
} | |
return CallNextHookEx(hookId, nCode, wParam, lParam); | |
} | |
} | |
} | |
' -ReferencedAssemblies System.Windows.Forms | |
$script:pressed = $false | |
[PowerShell.KeyLogger]::Run({ | |
param($key,$keydown) | |
if ($key -eq $keyToBind.ToUpper()) { | |
if(!$script:pressed -and $keydown){ | |
$script:pressed = $true | |
Write-Host "F15 down" | |
KeyPress 0x7E $keydown | |
#KeyPress ([char]'A') $keydown | |
} elseif (!$keydown) { | |
$script:pressed = $false | |
Write-Host "F15 up" | |
KeyPress 0x7E $keydown | |
#KeyPress ([char]'A') $keydown | |
} | |
} | |
}) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
sudo yum install autoconf automake bzip2 bzip2-devel cmake freetype-devel gcc gcc-c++ git libtool make mercurial pkgconfig zlib-devel -y | |
sudo yum install gnutls-devel -y | |
mkdir ~/ffmpeg_sources | |
# nasm | |
cd ~/ffmpeg_sources | |
curl -O -L https://www.nasm.us/pub/nasm/releasebuilds/2.14.02/nasm-2.14.02.tar.bz2 | |
tar xjvf nasm-2.14.02.tar.bz2 | |
cd nasm-2.14.02 | |
./autogen.sh | |
./configure --prefix="$HOME/ffmpeg_build" --bindir="$HOME/bin" | |
make -j $(nproc) | |
make install | |
# yasm | |
cd ~/ffmpeg_sources | |
curl -O -L http://www.tortall.net/projects/yasm/releases/yasm-1.3.0.tar.gz | |
tar xzvf yasm-1.3.0.tar.gz | |
cd yasm-1.3.0 | |
./configure --prefix="$HOME/ffmpeg_build" --bindir="$HOME/bin" | |
make -j $(nproc) | |
make install | |
# libxh264 | |
cd ~/ffmpeg_sources | |
git clone --depth 1 https://code.videolan.org/videolan/x264 | |
cd x264 | |
PKG_CONFIG_PATH="$HOME/ffmpeg_build/lib/pkgconfig" ./configure --prefix="$HOME/ffmpeg_build" --bindir="$HOME/bin" --enable-static --enable-pic | |
make -j $(nproc) | |
make install | |
# ffmpeg nv codec pkg config headers | |
cd ~/ffmpeg_sources | |
git clone https://git.videolan.org/git/ffmpeg/nv-codec-headers.git | |
cd nv-codec-headers | |
git checkout n11.0.10.1 | |
sudo make install | |
# ffmpeg | |
cd ~/ffmpeg_sources | |
wget -q https://github.com/FFmpeg/FFmpeg/archive/refs/tags/n4.4.2.tar.gz | |
tar -xf n4.4.2.tar.gz | |
mv FFmpeg-n4.4.2 ffmpeg | |
cd ffmpeg | |
ccap=37 | |
PATH="$HOME/bin:$PATH" PKG_CONFIG_PATH="$HOME/ffmpeg_build/lib/pkgconfig:/usr/local/lib/pkgconfig" ./configure \ | |
--prefix='/usr/' --extra-cflags='-I/usr/local/cuda/include' \ | |
--extra-ldflags='-L/usr/local/cuda/lib64' \ | |
--nvccflags="-gencode arch=compute_${ccap},code=sm_${ccap} -O2" \ | |
--disable-doc --disable-static --disable-bsfs --disable-decoders --disable-encoders \ | |
--disable-filters --disable-demuxers --disable-devices --disable-muxers \ | |
--disable-parsers --disable-postproc --disable-protocols --enable-decoder=aac \ | |
--enable-decoder=h264 --enable-decoder=h264_cuvid --enable-decoder=hevc_cuvid \ | |
--enable-decoder=rawvideo --enable-indev=lavfi --enable-encoder=libx264 \ | |
--enable-encoder=h264_nvenc --enable-encoder=hevc_nvenc \ | |
--enable-demuxer=mov --enable-demuxer=mp4 --enable-muxer=mp4 --enable-filter=scale \ | |
--enable-filter=testsrc2 --enable-protocol=file --enable-protocol=https \ | |
--enable-gnutls --enable-shared --enable-pic --enable-gpl --enable-nonfree \ | |
--enable-cuda-nvcc --enable-libx264 --enable-nvenc --enable-cuvid --enable-nvdec | |
#sudo bash -c 'PATH="/home/ec2-user/bin:$PATH" make -j $(nproc)' | |
make -j $(nproc) | |
sudo make install | |
hash -d ffmpeg | |
exit | |
# # ffmpeg | |
# cd ~/ffmpeg_sources | |
# curl -O -L https://ffmpeg.org/releases/ffmpeg-snapshot.tar.bz2 | |
# tar xjvf ffmpeg-snapshot.tar.bz2 | |
# cd ffmpeg | |
# PATH="$HOME/bin:$PATH" PKG_CONFIG_PATH="$HOME/ffmpeg_build/lib/pkgconfig" ./configure \ | |
# --prefix="$HOME/ffmpeg_build" \ | |
# --pkg-config-flags="--static" \ | |
# --extra-cflags="-I$HOME/ffmpeg_build/include" \ | |
# --extra-ldflags="-L$HOME/ffmpeg_build/lib" \ | |
# --extra-libs=-lpthread \ | |
# --extra-libs=-lm \ | |
# --bindir="$HOME/bin" \ | |
# --enable-gpl \ | |
# --enable-libfdk-aac \ | |
# --enable-libfreetype \ | |
# --enable-libmp3lame \ | |
# --enable-libopus \ | |
# --enable-libvpx \ | |
# --enable-libx264 \ | |
# --enable-libx265 \ | |
# --enable-nonfree | |
############# don't neeed below | |
# # libx265 | |
# cd ~/ffmpeg_sources | |
# git clone https://github.com/videolan/x265 | |
# cd ~/ffmpeg_sources/x265/build/linux | |
# cmake -G "Unix Makefiles" -DCMAKE_INSTALL_PREFIX="$HOME/ffmpeg_build" -DENABLE_SHARED:bool=off ../../source | |
# make -j $(nproc) | |
# make install | |
# # libfdk_aac | |
# cd ~/ffmpeg_sources | |
# git clone --depth 1 https://github.com/mstorsjo/fdk-aac | |
# cd fdk-aac | |
# autoreconf -fiv | |
# ./configure --prefix="$HOME/ffmpeg_build" --disable-shared | |
# make -j $(nproc) | |
# make install | |
# # libmp3lame | |
# cd ~/ffmpeg_sources | |
# curl -O -L https://downloads.sourceforge.net/project/lame/lame/3.100/lame-3.100.tar.gz | |
# tar xzvf lame-3.100.tar.gz | |
# cd lame-3.100 | |
# ./configure --prefix="$HOME/ffmpeg_build" --bindir="$HOME/bin" --disable-shared --enable-nasm | |
# make -j $(nproc) | |
# make install | |
# # libopus | |
# cd ~/ffmpeg_sources | |
# curl -O -L https://archive.mozilla.org/pub/opus/opus-1.3.tar.gz | |
# tar xzvf opus-1.3.tar.gz | |
# cd opus-1.3 | |
# ./configure --prefix="$HOME/ffmpeg_build" --disable-shared | |
# make -j $(nproc) | |
# make install | |
# # libvpx | |
# cd ~/ffmpeg_sources | |
# git clone --depth 1 https://chromium.googlesource.com/webm/libvpx.git | |
# cd libvpx | |
# ./configure --prefix="$HOME/ffmpeg_build" --disable-examples --disable-unit-tests --enable-vp9-highbitdepth --as=yasm | |
# make -j $(nproc) | |
# make install | |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Demo GIF here
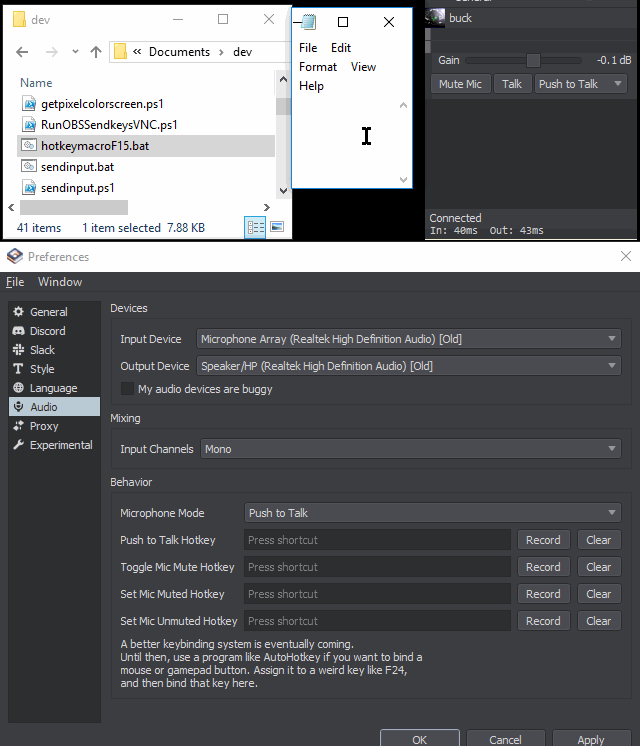
Batch file that calls itself with powershell
Useful for Ripcord discord client for push to talk since it seems to eat button presses (as of 0.4.25.0)
Allows creating macros by simulating virtual key presses with F15 from a designated Hotkey, which by default is V in the
keyToBind
variable