Last active
September 14, 2024 02:27
-
-
Save MeteorVE/ae5ef772218f2a6474847e12248e279a to your computer and use it in GitHub Desktop.
Painter for coloring sendou.ink build-card
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// ==UserScript== | |
// @name sendou.ink-color-changer | |
// @namespace http://tampermonkey.net/ | |
// @version 1.0 | |
// @description change sendou.ink card color | |
// @author MeteorV | |
// @match https://sendou.ink/u* | |
// @icon https://www.google.com/s2/favicons?sz=64&domain=sendou.ink | |
// @require https://openuserjs.org/src/libs/sizzle/GM_config.js | |
// @grant GM_registerMenuCommand | |
// ==/UserScript== | |
(function() { | |
'use strict'; | |
window.addEventListener('load', function() { | |
// after load | |
update() | |
}, false); | |
var color_dict = {"color:yellow": "#fbffab", "color:blue":"#abeeff", "color:green":"#dcffa8", "color:red":"#fa7070", | |
"color:grey":"#6e6f73", "color:purple":"#d9c1f5", "color:pink":"#fab4e8", "color:orange":"#fcbf79", | |
"color:navy":"#72c1ff"} | |
function update() { | |
console.log("[Info]: Start to find"); | |
var text_divs = document.getElementsByClassName("chakra-popover__body"); | |
console.log(text_divs, "[Length]:",text_divs.length) | |
if(text_divs==null){ | |
console.log("[Info]: I can't find anything."); | |
} | |
for(var i=0; i<text_divs.length; i++){ | |
if(text_divs[i].textContent.includes("color:")){ | |
let target = text_divs[i].parentNode.parentNode.parentNode.parentNode.parentNode; | |
let btn_group = text_divs[i].parentNode.parentNode.parentNode.querySelectorAll('button'); | |
for(let i=0; i<btn_group.length; i++){ | |
btn_group[i].style.color = "#000000"; | |
} | |
console.log("[Target's context]:", text_divs[i].textContent) | |
for(const [key, value] of Object.entries(color_dict)){ | |
if(text_divs[i].textContent.includes(key)){ | |
target.style.backgroundColor = color_dict[key]; | |
} | |
}// end of for | |
} | |
if(text_divs[i].textContent.includes("color:#")){ | |
let target = text_divs[i].parentNode.parentNode.parentNode.parentNode.parentNode; | |
let btn_group = text_divs[i].parentNode.parentNode.parentNode.querySelectorAll('button'); | |
for(let i=0; i<btn_group.length; i++){ | |
btn_group[i].style.color = "#000000"; | |
} | |
let idx = text_divs[i].textContent.indexOf("color:#"); | |
let color_text = text_divs[i].textContent.slice(idx+6, idx+13); | |
target.style.backgroundColor = color_text; // e.g. #fbffab | |
} | |
}// end of for | |
return | |
} | |
function color_rule(rule) { | |
console.log("[Info]: Start to find rule img"); | |
var imgs = document.getElementsByTagName("img"); | |
console.log(imgs, "[Length]:",imgs.length) | |
if(imgs==null){ | |
console.log("[Info]: I can't find anything."); | |
} | |
var rule_dict = {"TW":"Mode (TW)", "SZ":"Mode (SZ)", "TC":"Mode (TC)", "RM":"Mode (RM)", "CB":"Mode (CB)"}; | |
for(var i=0; i<imgs.length; i++){ | |
if(imgs[i].alt == rule_dict[rule]){ | |
let target = imgs[i].parentNode.parentNode.parentNode.parentNode.parentNode.parentNode.parentNode.parentNode.parentNode.parentNode; | |
let btn_group = target.querySelectorAll('button'); | |
for(let i=0; i<btn_group.length; i++){ | |
btn_group[i].style.color = "#000000"; | |
} | |
target.style.backgroundColor = color_dict["color:yellow"]; | |
} | |
}// end of for | |
return | |
} | |
function color_reset() { | |
console.log("[Info]: Start to reset"); | |
var text_divs = document.getElementsByClassName("chakra-popover__body"); | |
console.log(text_divs, "[Length]:",text_divs.length) | |
if(text_divs==null){ | |
console.log("[Info]: I can't find anything."); | |
} | |
for(var i=0; i<text_divs.length; i++){ | |
// var ldiv = text_divs[i]; | |
if(text_divs[i].textContent.includes("color:")){ | |
let target = text_divs[i].parentNode.parentNode.parentNode.parentNode.parentNode; | |
let btn_group = text_divs[i].parentNode.parentNode.parentNode.querySelectorAll('button'); | |
for(let i=0; i<btn_group.length; i++){ | |
btn_group[i].style.color = "#1bb300"; | |
} | |
target.style.backgroundColor = null; | |
} | |
if(text_divs[i].textContent.includes("hidden:true")){ | |
let target = text_divs[i].parentNode.parentNode.parentNode.parentNode.parentNode; | |
target.style.display = ""; | |
} | |
}// end of for | |
return | |
} | |
function remove() { | |
console.log("[Info]: Start to hide something."); | |
var text_divs = document.getElementsByClassName("chakra-popover__body"); | |
console.log(text_divs, "[Length]:",text_divs.length) | |
if(text_divs==null){ | |
console.log("[Info]: I can't find anything."); | |
} | |
for(var i=0; i<text_divs.length; i++){ | |
// var ldiv = text_divs[i]; | |
if(text_divs[i].textContent.includes("hidden:true")){ | |
let target = text_divs[i].parentNode.parentNode.parentNode.parentNode.parentNode; | |
target.style.display = "none"; | |
} | |
}// end of for | |
return | |
} | |
GM_registerMenuCommand("著色🎨🖌️!", update, "U"); | |
GM_registerMenuCommand("隱藏特定組合🗑️", remove, "D"); | |
GM_registerMenuCommand("高亮: 塗地💧", () => color_rule('TW')); | |
GM_registerMenuCommand("高亮: 佔地🌊", () => color_rule("SZ")); | |
GM_registerMenuCommand("高亮: 推塔🗼", () => color_rule("TC")); | |
GM_registerMenuCommand("高亮: 運魚🐟", () => color_rule("RM")); | |
GM_registerMenuCommand("高亮: 投蛤🦪", () => color_rule("CB")); | |
GM_registerMenuCommand("還原初始狀態🔄", color_reset, "R"); | |
// Emoji 參考 https://www.emojiall.com/zh-hant/categories/I | |
// 顏色挑選器 https://www.google.com/search?q=online+color+picker | |
})(); | |
// ==UserScript==
// @name 虎航登記
// @namespace http://tampermonkey.net/
// @version 2024-09-14
// @description try to take over the world!
// @author You
// @match https://10anniversary.tigerairtw.com/*
// @icon https://www.google.com/s2/favicons?sz=64&domain=tigerairtw.com
// @run-at document-end
// @require https://openuserjs.org/src/libs/sizzle/GM_config.js
// @grant GM_registerMenuCommand
// ==/UserScript==
(function() {
// 'use strict';
// focus
console.log("Script Start.");
window.addEventListener('load', function() {
exec_all();
}, false);
GM_registerMenuCommand("填寫第一份資料", exec_all, "C");
GM_registerMenuCommand("填寫第二份資料", anotherData, "D");
document.addEventListener('keydown', function(event) {
if (event.ctrlKey && event.altKey && event.key === 's') { // && event.altKey
event.preventDefault();
exec_all();
}
});
})();
function exec_all(){
expand();
setTimeout(() => {
fill('5390011675', '0972421536'); // 替換成你想要的會員編號 手機號碼
}, 1000);
}
function anotherData(){
setTimeout(() => {
fill('222222222', '0972421536');
}, 1000);
}
function expand(){
// 獲取頁面上第二個 .btn_all 元素的 a 標籤
const checkInButton = document.querySelectorAll('.btn_w .btn_all')[1].querySelector('a');
// 點擊該按鈕
checkInButton.click();
}
function fill(tid, phone){
// 獲取“巳登錄過資料”區域的元素
const registeredSection = document.querySelector('.form2 .input-field');
// 獲取“台虎會員編號” 和 “手機” 兩個輸入框
const memberIdInput = registeredSection.querySelector('input[name="id"]');
const phoneInput = registeredSection.querySelector('input[name="phone"]');
// 設置這些輸入框的值
memberIdInput.value = tid;
phoneInput.value = phone;
// 打印這些元素來驗證值是否設置成功
console.log(memberIdInput.value);
console.log(phoneInput.value);
}
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Demo
請右鍵 - 新分頁開啟,查看 https://i.imgur.com/CwHPoBz.mp4
安裝方式
安裝 TemperMonkey -> 點我到 Chrome 商店 || 點我到 Edge 商店 || 點我到 Firefox 商店
到瀏覽器右上方的拼圖圖案,找到 TemperMonkey,點選大頭針讓它 "固定"
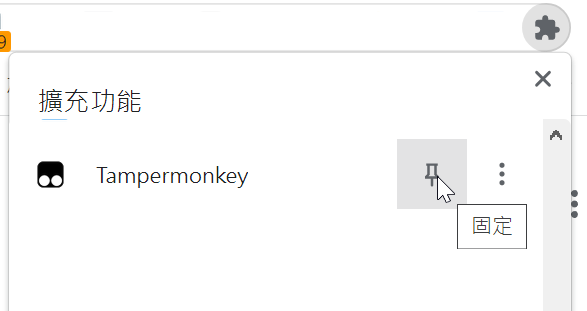
點選 "新增腳本"
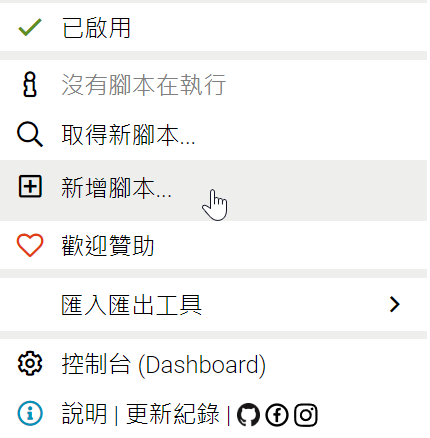
你會看到有很多的程式碼,全部刪掉 (會變成下圖這樣)
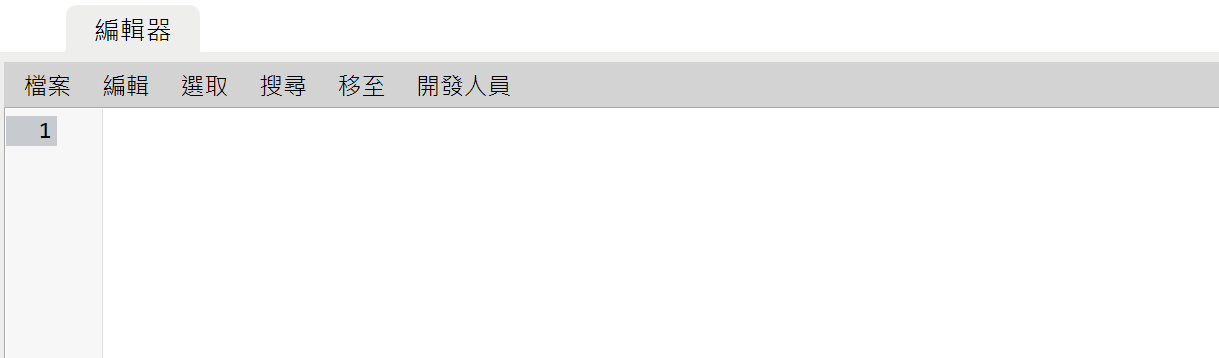
然後把上面的程式碼全部複製起來,貼到裡面去
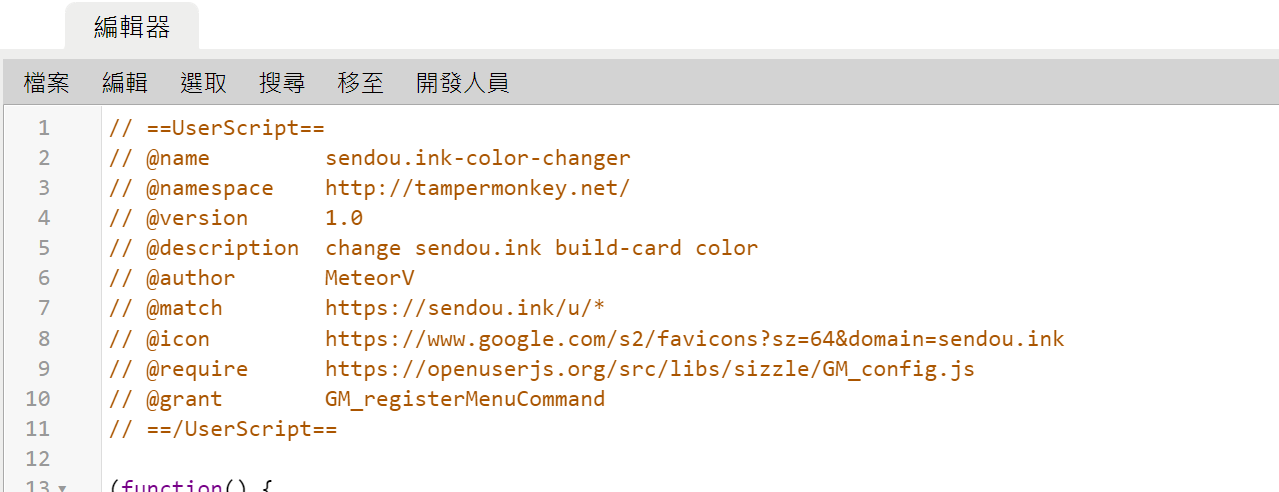
點選 "檔案" -> "儲存",或是按
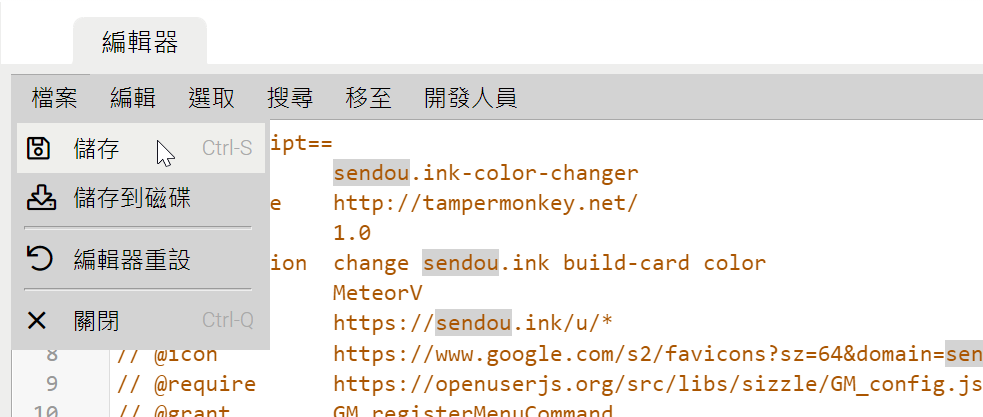
Ctrl+S
(Mac 用戶可能是Command+S
)到 https://sendou.ink/u/,你應該可以看到右上方的 TemperMonkey 插件有一個紅色的 1
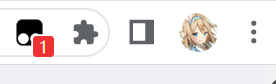
到這邊就完成安裝了,後面教學如何使用。
使用方式
到 sendou.ink 的你的個人頁面,或是你可以到這個 user 頁面 搜尋你自己。
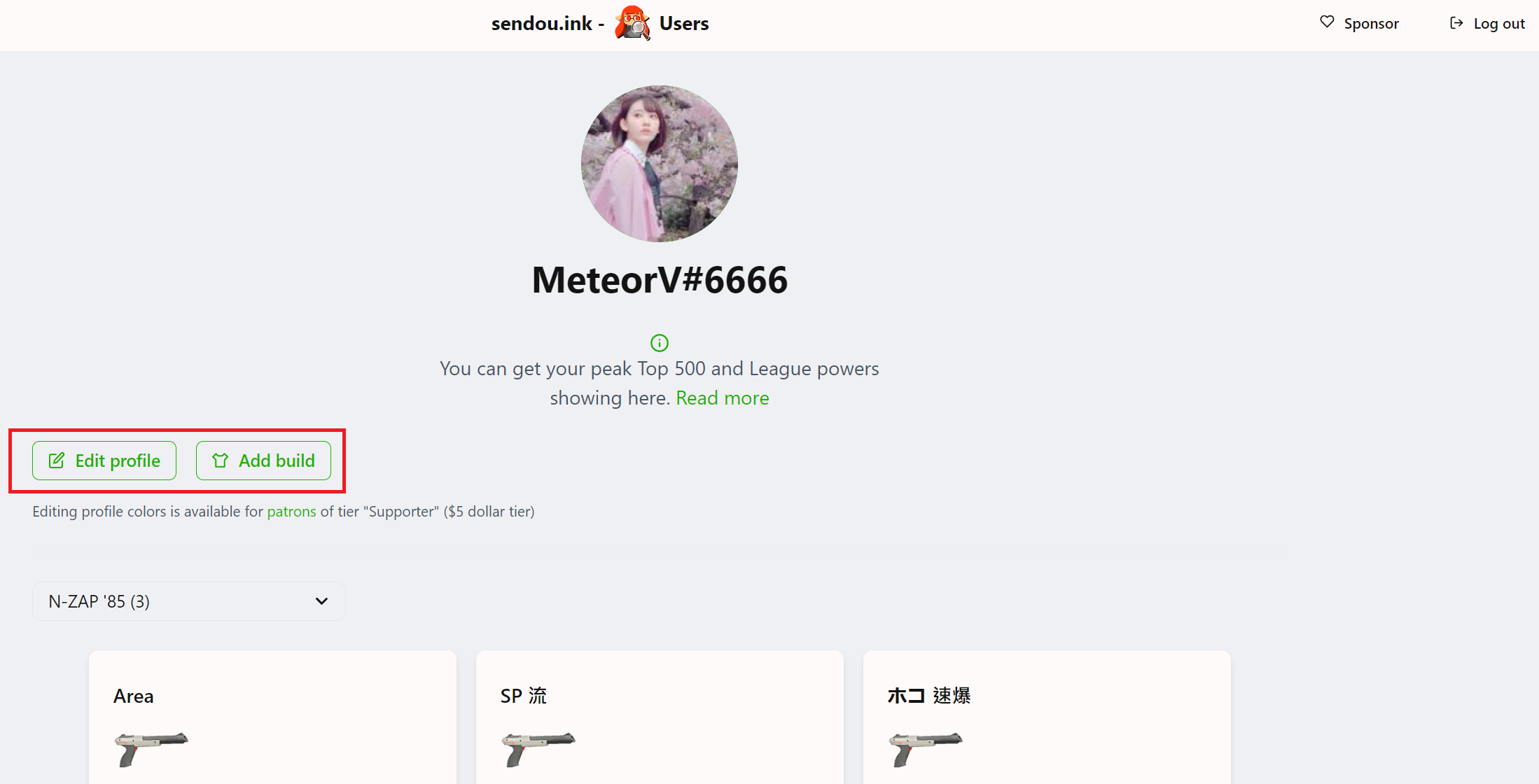
如果你有登入,你應該可以看到紅色框框的 "Add build"
(註 : 不用搜這個 ID,你應該搜不到 XD)
編輯其中一個 Build (套裝),或是想要新增一個也可以,在 Description 裡面寫
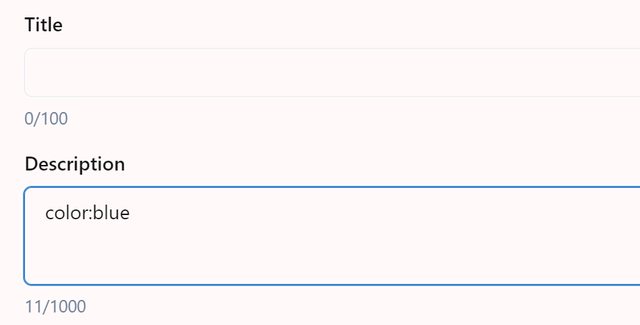
color:blue
記得儲存 !
你的插件應該要有一個紅色的 1,點下去
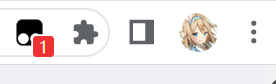
點下去之後你應該可以看到像下面這張圖片
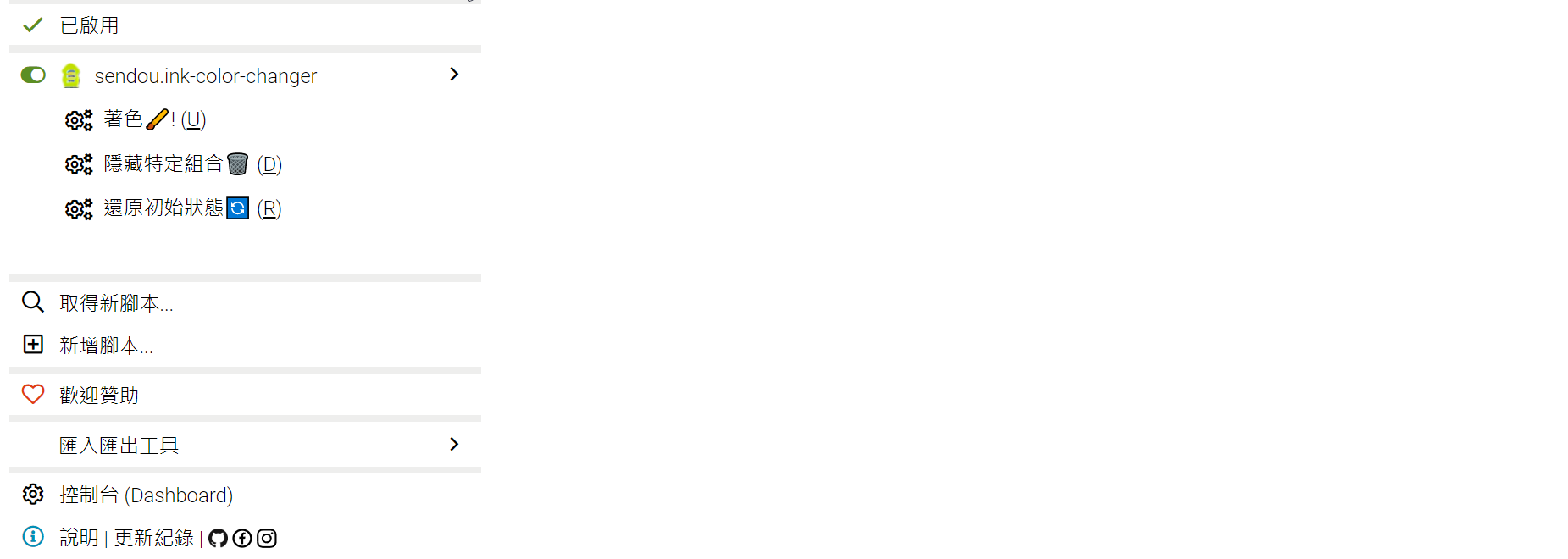
如果看的到,代表蠻順利的 ! 你點下 "著色" 後,它應該就會幫你把剛剛 Description 寫
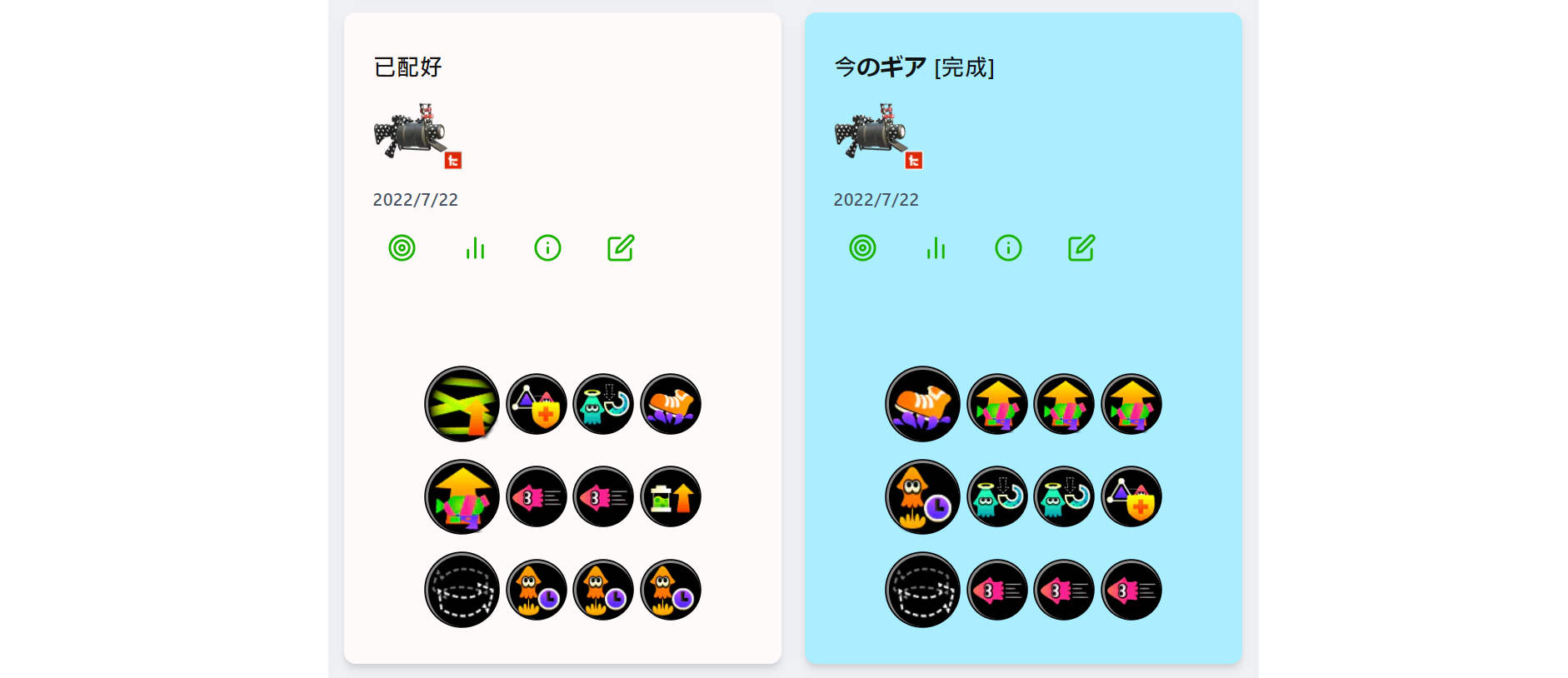
color:blue
的那張卡片著色成水藍色。效果如圖
如果您到下面這個頁面,使用 TamperMonkey 腳本點擊 "著色",您應該可以看到如圖
https://sendou.ink/u/697386710341779466
然後儲存 -> 讓我的腳本和它的腳本同時啟用
https://gist.github.com/disco0/68798129c8673d460cbb7a4bc1517cd3
後面開始介紹一些功能相關。
功能介紹
著色功能
:
的前後不可以有空格。color:#dcffa8
#
後面加六個字元。上面那個舉例可以仔細數。隱藏某些你暫時不想看到的組合
hidden:true
hidden:true
之後,可以點選 "隱藏特定組合" 來進行隱藏。高亮 (Highlight) 特定真格規則的組合
聲明