Add the create_post
function:
// AJAX for posting
function create_post() {
console.log("create post is working!") // sanity check
console.log($('#post-text').val())
};
Again, we ran a sanity check to ensure the function is called correctly, then we grab the input value of the form.
For this to work correctly we need to add an id to the form field:
class PostForm(forms.ModelForm):
class Meta:
model = Post
# exclude = ['author', 'updated', 'created', ]
fields = ['text']
widgets = {
'text': forms.TextInput(attrs={
'id': 'post-text',
'required': True,
'placeholder': 'Say something...'
}),
}
Notice how we also added a placeholder to the field and made it required along with the id. We could add some error handlers to the form template or simply let HTML5 handle it. Let’s use the latter.
Test again. Submit the form with the word “test”. You should see the following in your console:
form submitted!
create post is working!
test
So, we’ve confirmed that we’re calling the create_post() function correctly as well as grabbing the value of the form input.
Now let’s wire in some AJAX to submit the POST request.
// AJAX for posting
function create_post() {
console.log("create post is working!") // sanity check
$.ajax({
url : "create_post/", // the endpoint
type : "POST", // http method
data : { the_post : $('#post-text').val() }, // data sent with the post request
// handle a successful response
success : function(json) {
$('#post-text').val(''); // remove the value from the input
console.log(json); // log the returned json to the console
console.log("success"); // another sanity check
},
// handle a non-successful response
error : function(xhr,errmsg,err) {
$('#results').html("<div class='alert-box alert radius' data-alert>Oops! We have encountered an error: "+errmsg+
" <a href='#' class='close'>×</a></div>"); // add the error to the dom
console.log(xhr.status + ": " + xhr.responseText); // provide a bit more info about the error to the console
}
});
};
What’s happening? Well, we submit form data to the create_post/
endpoint, then wait for one of two responses - either a success or a failure.... Follow the code comments for a more detailed explanation.
Now let’s update our views to handle the POST request correctly:
def create_post(request):
if request.method == 'POST':
post_text = request.POST.get('the_post')
response_data = {}
post = Post(text=post_text, author=request.user)
post.save()
response_data['result'] = 'Create post successful!'
response_data['postpk'] = post.pk
response_data['text'] = post.text
response_data['created'] = post.created.strftime('%B %d, %Y %I:%M %p')
response_data['author'] = post.author.username
return HttpResponse(
json.dumps(response_data),
content_type="application/json"
)
else:
return HttpResponse(
json.dumps({"nothing to see": "this isn't happening"}),
content_type="application/json"
)
Here we grab the post text along with the author and update the database. Then we create a response dict, serialize it into JSON, and then send it as the response - which gets logged to the console in the success handler: console.log(json)
, as you saw in the create_post()
function in the JavaScript file above.
Test this again.
You should see the object in the console:
form submitted!
create post is working!
Object {text: "hey!", author: "michael", postpk: 15, result: "Create post successful!", created: "August 22, 2014 10:55 PM"}
success
Simply add an id of “talk” to the <ul>
:
<ul id="talk">
Then update the form so that errors will be added:
<form method="POST" id="post-form">
{% csrf_token %}
<div class="fieldWrapper" id="the_post">
{{ form.text }}
</div>
<div id="results"></div> <!-- errors go here -->
<input type="submit" value="Post" class="tiny button">
</form>
Now we can add the JSON to the DOM where that new “talk” id is:
success : function(json) {
$('#post-text').val(''); // remove the value from the input
console.log(json); // log the returned json to the console
$("#talk").prepend("<li><strong>"+json.text+"</strong> - <em> "+json.author+"</em> - <span> "+json.created+"</span></li>");
console.log("success"); // another sanity check
},
Ready to see this in action? Test it out!
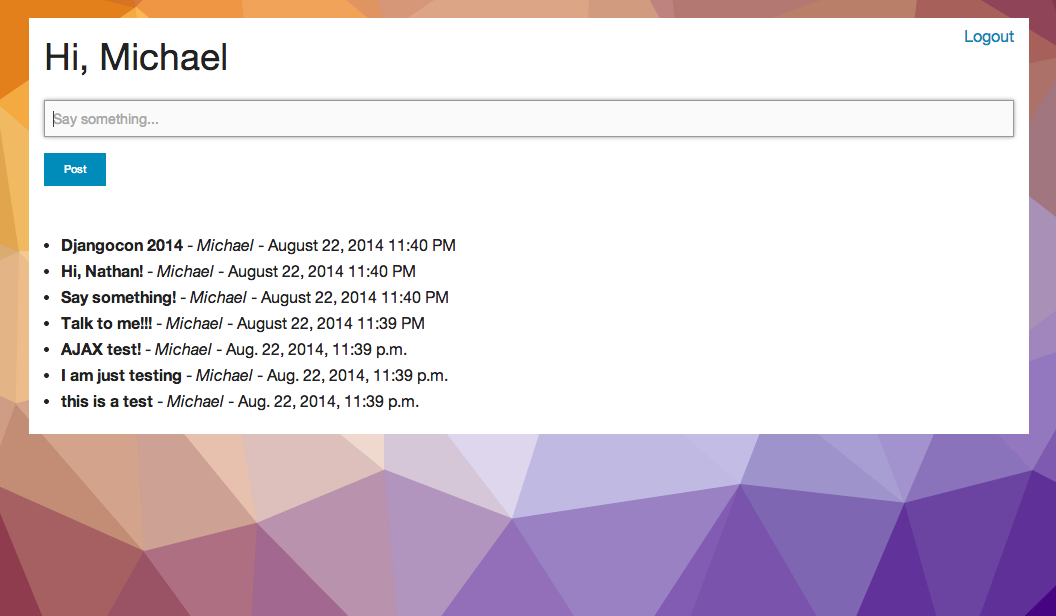