Created
May 31, 2019 05:23
-
-
Save aras-p/4882120965470f29dd50aa8b836ebed4 to your computer and use it in GitHub Desktop.
DX10 HLSL style textures and samplers in Unity
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// Example of using DX10-style HLSL texture and sampler objects in | |
// Unity shaders | |
Shader "Unlit/Example" | |
{ | |
Properties | |
{ | |
_MainTex ("Texture", 2D) = "white" {} | |
} | |
SubShader | |
{ | |
Pass | |
{ | |
CGPROGRAM | |
#pragma vertex vert | |
#pragma fragment frag | |
#include "UnityCG.cginc" | |
struct appdata | |
{ | |
float4 vertex : POSITION; | |
float2 uv : TEXCOORD0; | |
}; | |
struct v2f | |
{ | |
float2 uv : TEXCOORD0; | |
float4 pos : SV_POSITION; | |
}; | |
float4 _MainTex_ST; | |
v2f vert (appdata v) | |
{ | |
v2f o; | |
o.pos = UnityObjectToClipPos(v.vertex); | |
o.uv = TRANSFORM_TEX(v.uv, _MainTex); | |
return o; | |
} | |
// traditional DX9-style HLSL: | |
//sampler2D _MainTex; | |
// the same, but with DX10-style HLSL syntax: | |
Texture2D _MainTex; | |
SamplerState sampler_MainTex; | |
// we could also use sampling settings directly specified in the | |
// shader, see https://docs.unity3d.com/Manual/SL-SamplerStates.html | |
//SamplerState my_point_clamp_sampler; | |
half4 frag (v2f i) : SV_Target | |
{ | |
// traditional DX9-style HLSL: | |
//half4 col = tex2D(_MainTex, i.uv); | |
// the same, but with DX10-style HLSL syntax: | |
half4 col = _MainTex.Sample(sampler_MainTex, i.uv); | |
// using sampler state from the shader itself | |
//half4 col = _MainTex.Sample(my_point_clamp_sampler, i.uv); | |
// SV_POSITION input to pixel shader in HLSL will contain | |
// pixel coordinates in .xy, so we can do a texture Load | |
// directly with that | |
// https://docs.microsoft.com/en-us/windows/desktop/direct3dhlsl/dx-graphics-hlsl-to-load | |
// this will of course only do something in the screen corner coordinates | |
// that are smaller than texture size in pixels, everywhere else it will result in black | |
//col = _MainTex.Load(int3(i.pos.xy, 0)); | |
return col; | |
} | |
ENDCG | |
} | |
} | |
} |
Examples like these would be amazing in improving the Unity shader docs!
In the meantime, thanks for sharing this :)
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here's screenshot of the
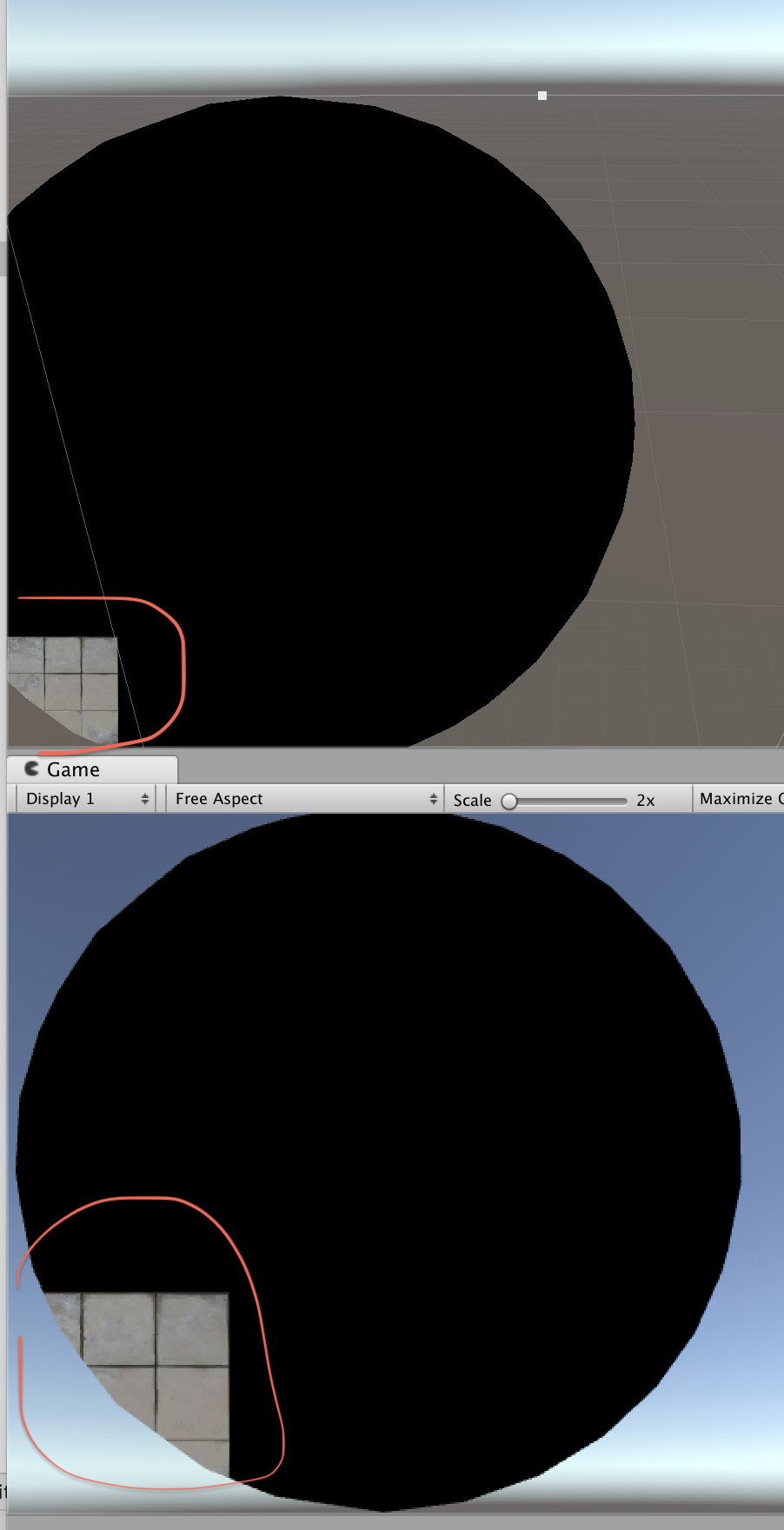
_MainTex.Load
case