Created
April 11, 2020 19:19
-
-
Save arsho/a8ee15b23f5f7394758747ad0b76c601 to your computer and use it in GitHub Desktop.
Tensorflow Basic Linear Regression on Linear Equation
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<!doctype html> | |
<html lang="en"> | |
<head> | |
<!-- Required meta tags --> | |
<meta charset="utf-8"> | |
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> | |
<meta name="author" content="Ahmedur Rahman Shovon"> | |
<!-- Bootstrap CSS --> | |
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css" integrity="sha384-Vkoo8x4CGsO3+Hhxv8T/Q5PaXtkKtu6ug5TOeNV6gBiFeWPGFN9MuhOf23Q9Ifjh" crossorigin="anonymous"> | |
<title>Tensorflow Basic Linear Regression on Linear Equation</title> | |
<!-- Load tensorflow --> | |
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/[email protected]/dist/tf.min.js"></script> | |
</head> | |
<body> | |
<div class="container"> | |
<div class="jumbotron"> | |
<h1 class="display-4">Tensorflow Linear Regression Example</h1> | |
<hr> | |
<ul class="lead"> | |
<li>This page shows how tensorflow uses linear regression to learn from training values.</li> | |
<li>We will use basic linear equation (<code>y = mx + b</code>) for sample input and output values.</li> | |
<li>Our sample equation is <code>y = 2x + 3</code>.</li> | |
<li>We will train the model by giving sample x and y coordinates values for the above equation.</li> | |
<li>Then we will test the model for a given x coordinate value.</li> | |
</ul> | |
</div> | |
<div class="row"> | |
<div class="col-12"> | |
<div class="table-responsive"> | |
<table class="table table-bordered table-sm"> | |
<thead class="thead-dark"> | |
<tr> | |
<th>Sample Input Value</th> | |
<th>Sample Output Value</th> | |
</tr> | |
</thead> | |
<tbody id="sample_data"> | |
</tbody> | |
</table> | |
</div> | |
<!-- ./table-responsive --> | |
<div class="table-responsive"> | |
<table class="table table-bordered table-sm"> | |
<thead class="thead-dark"> | |
<tr> | |
<th>Test Input Value</th> | |
<th>Expected Output Value</th> | |
<th>Model Output Value (Number of iterations: <span id="number_of_iterations_1"></span>)</th> | |
<th>Model Output Value (Number of iterations: <span id="number_of_iterations_2"></span>)</th> | |
</tr> | |
</thead> | |
<tbody> | |
<tr> | |
<td id="test_x_value"> | |
5 | |
</td> | |
<td>Expected value: 13</td> | |
<td> | |
<p class="lead" id="result_1"></p> | |
</td> | |
<td> | |
<p class="lead" id="result_2"></p> | |
</td> | |
</tr> | |
</tbody> | |
</table> | |
</div> | |
<!-- ./table-responsive --> | |
</div> | |
</div> | |
<!-- ./row --> | |
</div> | |
<!-- ./container --> | |
<!-- Optional JavaScript --> | |
<!-- jQuery first, then Popper.js, then Bootstrap JS --> | |
<script src="https://code.jquery.com/jquery-3.4.1.slim.min.js" integrity="sha384-J6qa4849blE2+poT4WnyKhv5vZF5SrPo0iEjwBvKU7imGFAV0wwj1yYfoRSJoZ+n" crossorigin="anonymous"></script> | |
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/umd/popper.min.js" integrity="sha384-Q6E9RHvbIyZFJoft+2mJbHaEWldlvI9IOYy5n3zV9zzTtmI3UksdQRVvoxMfooAo" crossorigin="anonymous"></script> | |
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/js/bootstrap.min.js" integrity="sha384-wfSDF2E50Y2D1uUdj0O3uMBJnjuUD4Ih7YwaYd1iqfktj0Uod8GCExl3Og8ifwB6" crossorigin="anonymous"></script> | |
<script type="text/javascript"> | |
async function train_linear_model(sample_x_values, sample_y_values, epochs, test_x_value, result_element, iteration_element){ | |
const number_of_sample_values = sample_x_values.length; | |
// Linear regression model | |
const linear_model = tf.sequential(); | |
linear_model.add(tf.layers.dense({units: 1, inputShape: [1]})); | |
linear_model.compile({ | |
loss: "meanSquaredError", | |
optimizer: "sgd" | |
}); | |
// Sample input and output for y = 2x + 3 | |
const x_values = tf.tensor2d(sample_x_values, [number_of_sample_values, 1]); | |
const y_values = tf.tensor2d(sample_y_values, [number_of_sample_values, 1]); | |
// Train the model using above sample data | |
await linear_model.fit(x_values, y_values, {epochs: epochs}); | |
// Predict value for test_x_value and show in the result div | |
var result = linear_model.predict(tf.tensor2d([test_x_value], [1, 1])).dataSync(); | |
console.log(result); | |
console.log(typeof(result)); | |
$("#"+result_element).html(result[0]); | |
$("#"+iteration_element).html(epochs); | |
} | |
function set_sample_values(sample_x_values, sample_y_values){ | |
const number_of_sample_values = sample_x_values.length; | |
for(var i=0;i<number_of_sample_values;i++){ | |
$("#sample_data").append("<tr><td>"+sample_x_values[i]+"</td><td>"+sample_y_values[i]+"</td></tr>") | |
} | |
} | |
$(document).ready(function(){ | |
const sample_x_values = [-20, -5, 0, 3, 6]; | |
const sample_y_values = [-37, -7, 3, 9, 15]; | |
set_sample_values(sample_x_values, sample_y_values); | |
var test_x_value = parseInt($("#test_x_value").text()); | |
train_linear_model(sample_x_values, sample_y_values, 200, test_x_value, "result_1", "number_of_iterations_1"); | |
train_linear_model(sample_x_values, sample_y_values, 500, test_x_value, "result_2", "number_of_iterations_2"); | |
}) | |
</script> | |
</body> | |
</html> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
day_1_LinearRegressionOnLinearEquation.html: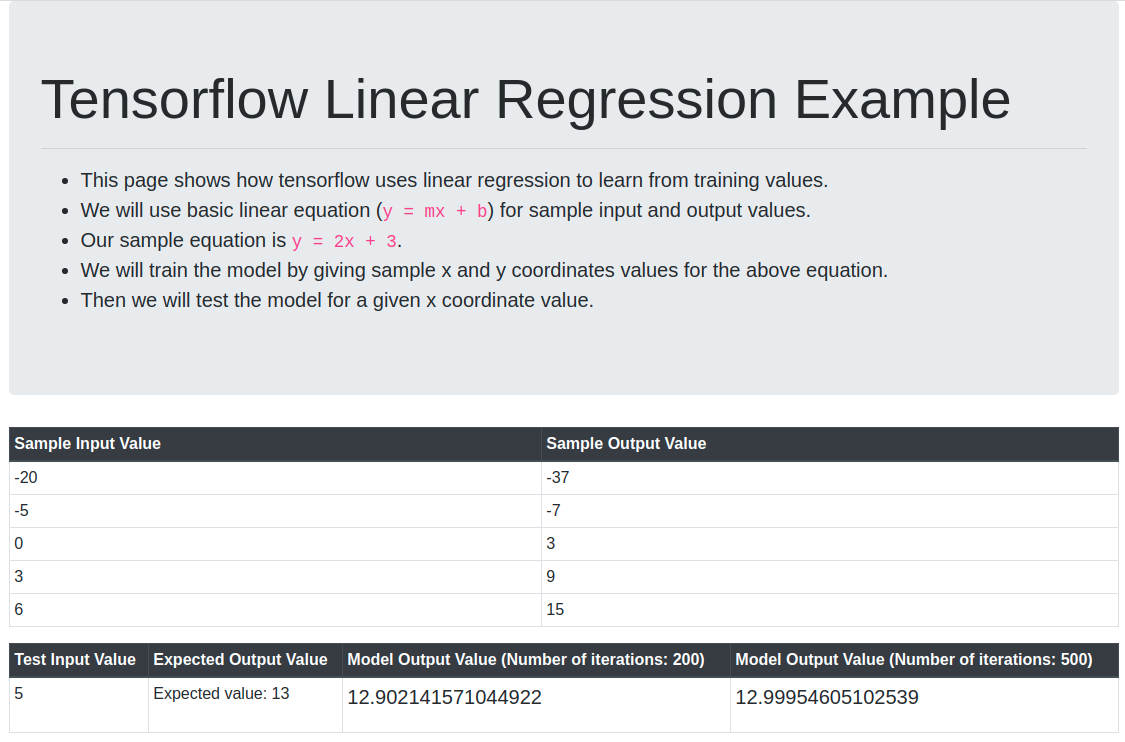