-
-
Save befinitiv/fd44a597a48460c100a54649c97d5984 to your computer and use it in GitHub Desktop.
#!/usr/bin/python | |
import glob | |
import socket | |
import time | |
from threading import Timer | |
import picamera | |
import RPi.GPIO as GPIO | |
SENSOR_PIN = 18 | |
W = 1296 | |
H = 972 | |
FPS = 18 | |
IP = '192.168.0.21' | |
PORT = 5000 | |
def start_recording(): | |
global camera_data | |
global camera | |
camera_data = CameraData() | |
camera.start_recording(camera_data, format='h264') | |
print('start') | |
def stop_recording(): | |
global camera_data | |
global camera | |
camera.stop_recording() | |
camera_data.stop() | |
print('stop') | |
def timer_stop(): | |
global stop_timer_running | |
stop_timer_running = False | |
stop_recording() | |
last_sensor_event = 0 | |
stop_timer_running = False | |
def sensor_change(pin): | |
global last_sensor_event | |
global stop_timer | |
global stop_timer_running | |
now = time.time() | |
if abs(last_sensor_event - now) > 0.5: | |
start_recording() | |
last_sensor_event = now | |
if stop_timer_running: | |
stop_timer.cancel() | |
stop_timer = Timer(0.5, timer_stop) | |
stop_timer.start() | |
stop_timer_running = True | |
GPIO.setmode(GPIO.BCM) | |
GPIO.setup(SENSOR_PIN, GPIO.IN) | |
GPIO.add_event_detect(SENSOR_PIN, GPIO.BOTH, sensor_change) | |
# This class writes data to file and streams it in parallel | |
class CameraData(object): | |
def __init__(self): | |
self.client_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) | |
self.client_socket.connect((IP, PORT)) | |
self.connection = self.client_socket.makefile('wb') | |
self.file = open(self.create_unique_file_name(), 'wb') | |
def create_unique_file_name(self): | |
return '%d.h264' % len(glob.glob('*')) | |
def write(self, s): | |
self.connection.write(s) | |
self.file.write(s) | |
def flush(self): | |
pass | |
def stop(self): | |
self.connection.close() | |
self.client_socket.close() | |
self.file.close() | |
# Make a file-like object out of the connection | |
try: | |
camera = picamera.PiCamera() | |
camera.resolution = (W, H) | |
camera.framerate = FPS | |
while True: | |
time.sleep(1) | |
#camera.wait_recording(60) | |
finally: | |
pass |
[Unit] | |
Description=Analog camera service | |
After=network.target | |
StartLimitIntervalSec=0 | |
[Service] | |
Type=simple | |
Restart=always | |
RestartSec=1 | |
User=pi | |
ExecStart=/home/pi/cam.py | |
WorkingDirectory=/home/pi/videos | |
[Install] | |
WantedBy=multi-user.target |
oh that's sweet ! any place where we could download the STL (or 3MF or other format) ?
I thought I did but I don't see it. We had a few versions and the last one has a cover that an allen wrench adjusts the sensor.
@finis-dev This looks spectacular! Would you be able to share the STL for this design please?
我以为我做到了,但我没有看到。我们有几个版本,最后一个版本有一个盖子,内六角扳手可以调整传感器。
![]()
@finis-dev Hello! I am a filmmaker. I saw the super8 box you modified on GitHub and I was very impressed! Your design is very exquisite, and the details are perfect. It is really a work of art!
I have a question for you. Can you share your stl file? I want to use a 3D printer to make a super8 box as a prop for a documentary. I promise not to use it for commercial purposes, just to show your creativity and technology.
If you are willing to share, I would be very grateful! If you are not convenient, I completely understand. In any case, I want to praise your design. You are a genius!
Looking forward to your reply,
I thought I did but I don't see it. We had a few versions and the last one has a cover that an allen wrench adjusts the sensor.
@finis-dev This looks spectacular! Would you be able to share the STL for this design please?
Any info on the stl? Much appreciated
yep, doesn't look like the STL file will be shared by @finis-dev :( that would have been a nice "xmas gift" :D
Here is the STL from befinitiv
https://cad.onshape.com/documents/64a99f95e94bdd2171e690a9/w/2df2681fee64d06b5b4c427b/e/396c0cd434668cf87cac40be
thanks @finis-dev
however, this doesn't look exactly the same like the version from above screenshots (abobe, the raspberry can be inserted in diagonal, and we can see holes for usb ports and hdmi, as well as inserts for screws to close the enclosure)
It's always interesting to see people continue a project on the original developer's idea, but then not share their progress (for others to then progress with) with those that also want to progress this project.
It's not like there is money to make with this anymore, or to patent it. Super-8 is very niche, and a patented marketed Digital-8 cartridge already exists. It's just kinda expensive.
hey please if anyone is working on this project still please contact me im having trouble here installing the picamera ... i am now trying picamera2 but I'm not sure what's the issue unless someone would want to share there SD card. The rest of the project is complete in housing with everything.. im just stuck with the software issue
christian@pizero:~ $ cat /etc/os-release
PRETTY_NAME="Raspbian GNU/Linux 12 (bookworm)"
NAME="Raspbian GNU/Linux"
VERSION_ID="12"
VERSION="12 (bookworm)"
VERSION_CODENAME=bookworm
ID=raspbian
ID_LIKE=debian
HOME_URL="http://www.raspbian.org/"
SUPPORT_URL="http://www.raspbian.org/RaspbianForums"
BUG_REPORT_URL="http://www.raspbian.org/RaspbianBugs"
ok its working i think im no longer getting errors and im running Bullseye. The camera is showing a small red LED when running the script now im trying to see the live feed from my computer via web browser and nothing .. i had tried changing the ip address to 192.168.1.117:5000 but that didnt change anything considering the PI is on that address already @antisaint81
actually i believe the issue is i haven't set up the
[camera.service]
(https://gist.github.com/befinitiv/fd44a597a48460c100a54649c97d5984#file-camera-service) where would i implement this..?
I thought I did but I don't see it. We had a few versions and the last one has a cover that an allen wrench adjusts the sensor.
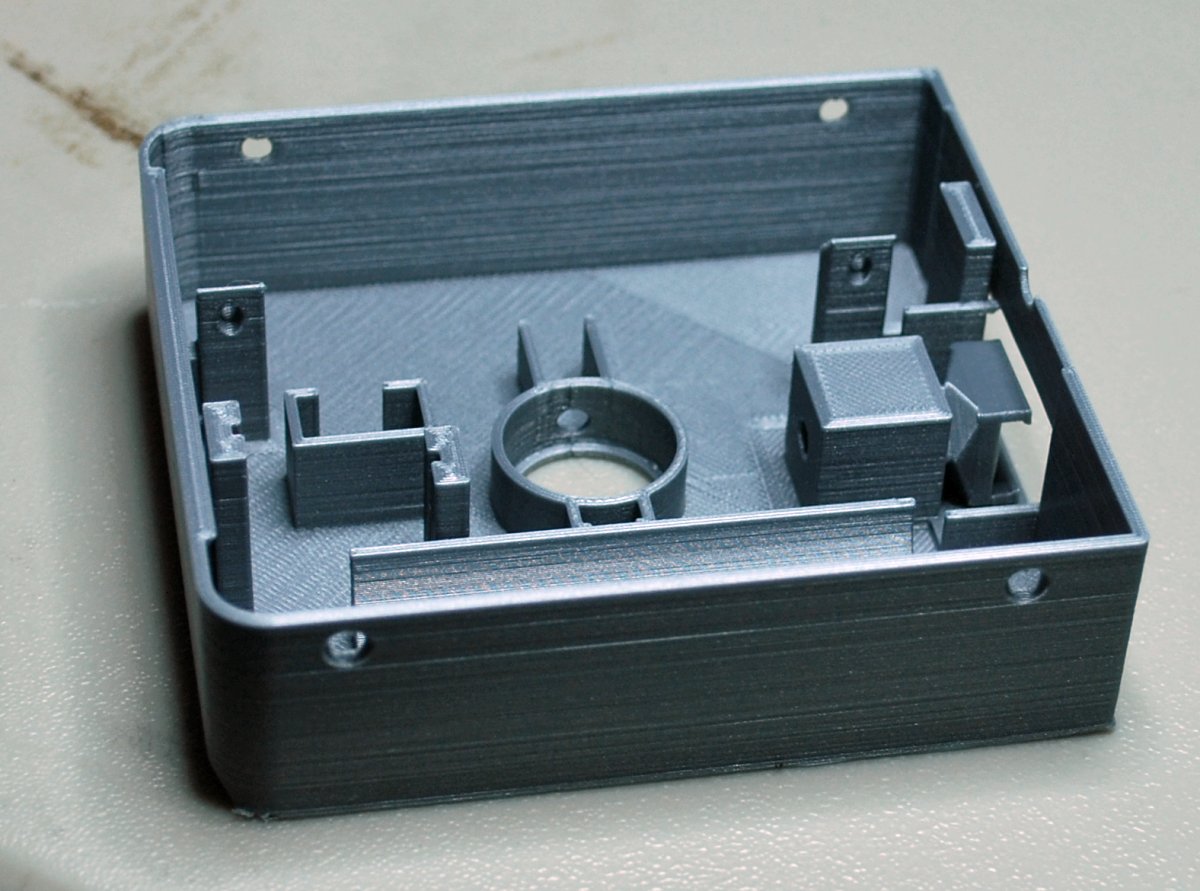
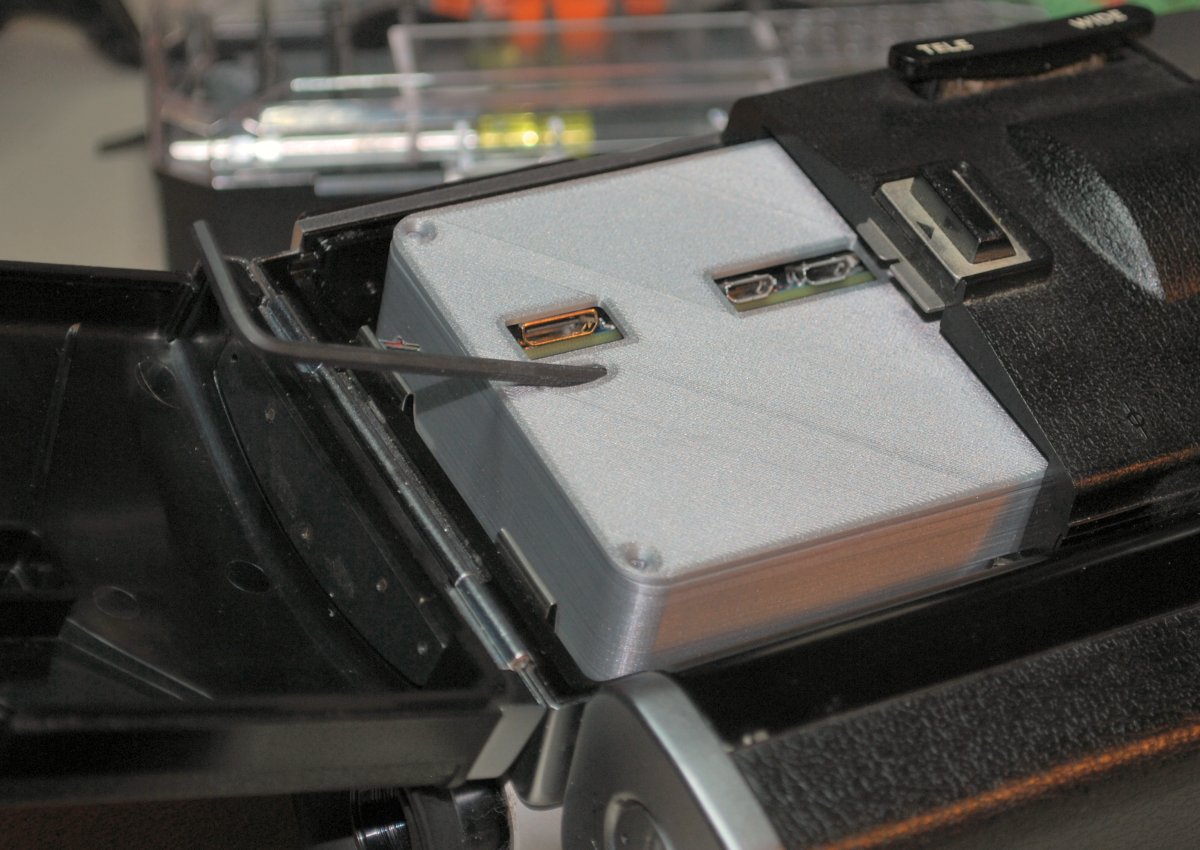