-
-
Save binki/10ac3e91851b524546f8279733cdadad to your computer and use it in GitHub Desktop.
#!/usr/bin/env node | |
const axios = require('axios'); | |
const FormData = require('form-data'); | |
const fs = require('fs'); | |
const filePath = __dirname + '/../accept-http-post-file/cookie.jpg'; | |
fs.readFile(filePath, (err, imageData) => { | |
if (err) { | |
throw err; | |
} | |
const form = new FormData(); | |
form.append('file', imageData, { | |
filepath: filePath, | |
contentType: 'image/jpeg', | |
}); | |
axios.post('http://localhost:3000/endpoint', form, { | |
headers: form.getHeaders(), | |
}).then(response => { | |
console.log('success! ', response.status, response.statusText, response.headers, typeof response.data, Object.prototype.toString.apply(response.data)); | |
}).catch(err => { | |
console.log(err); | |
}); | |
}); |
await response.data.pipe(writer);
This line is likely the issue. The await
keyword in JavaScript will take any non-“Thenable” object and convert it to a resolved Promise
. A nodejs-style Stream
(what is returned by readable.pipe()
is not Thenable. So that await
will not wait for anything to happen.
Thus, by the time fs.readFile()
starts running, the file D:\images\${subitems[2].id}.jpg
will very likely already exist because you had to wait for the server to respond earlier. However, the file will be empty or almost empty.
It is arguable that it is pointless for your program to even write out a temporary file in the first place. You’re using fs.readFile()
to read the entire file into memory instead of using a stream based API to write it to the server. You should really do the latter! You can even wire the requests together. However, for the sake of this help here, I will suggest the minimal change which would be required to get your sample to work at all.
Try replacing the line above with this:
await new Promise((resolve, reject) => {
response.data.pipe(writer);
writer.on('finish', resolve);
writer.on('error', reject);
});
Let me know if that helps!
Thank you @binki, it worked for me. So, would it be better to use createReadStream() to read and send, and should the server use createWriteStream()?
I am confused with this example, readFile returns a Buffer if encoding is not specified and FormData only accepts Blob or USVString. Am I missing something?
readFile()
and nodejs-style Buffer
only exist in a nodejs environment. USVString
and also Blob
only exist in a browser environment. The form-data
nodejs module is meant to look similar to the DOM (browser environment) class in usage. This is because the DOM API is something that people are familiar with. But it does not support and is not intended to be used in the browser environment. It is nodejs-oriented. See its documentation for FormData.append()
for information on the types of arguments you may pass to the form-data
npmjs module’s FormData
class.
Sorry for this super late and likely now useless response ;-). But, yes, if you need to support arbitrary file sizes, you are pretty much forced to use something like createReadStream()
on the client and createWriteStream()
on the server. Doing this and adding error handling and cleanup gets into a more complicated solution which I would spend time on if I needed to do it myself, but I don’t need to at the moment ;-). Sorry, and I hope that my earlier response was enough to get you somewhere!
Could you help me?
I'm trying to send images from my local server to other local server (testing), so this is my code:
But when upload the image, the image is incomplete (I guess), see:
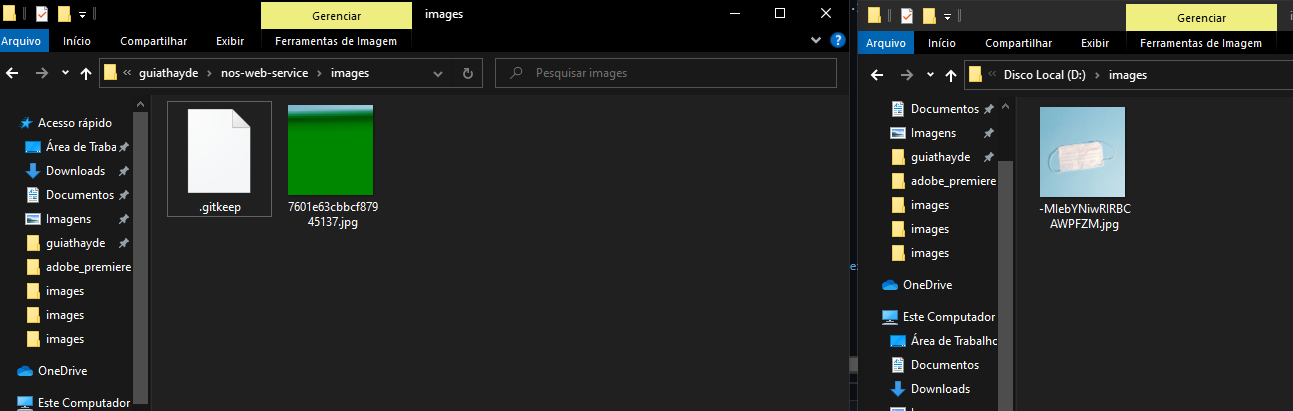