Last active
September 16, 2018 00:58
-
-
Save boazblake/7d07f449b79b42e46919036aea922f97 to your computer and use it in GitHub Desktop.
mithril-prezentation on OO patterns and designs
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"Title": "OO- patterns and designs", | |
"slides": [ | |
{ | |
"id": "eb4c4664-b947-11e8-96f8-529269fb1459", | |
"title": "SLIDE _11 DIP", | |
"contents": "__Dependency inversion__\n===\n---\n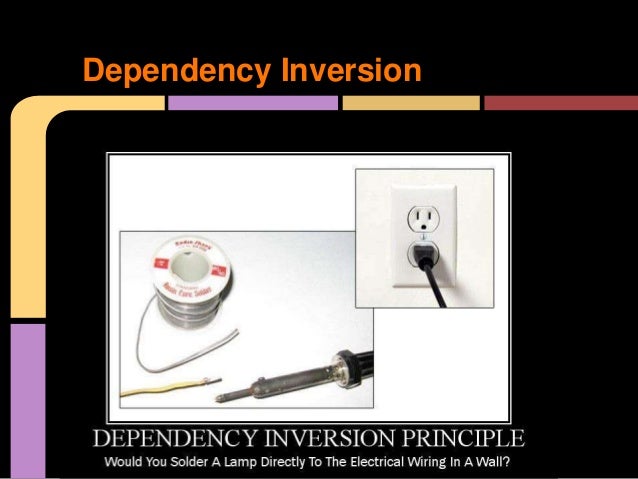\n>High level modules should not depend on low level modules rather both should depend on abstraction.\n\n>Abstraction should not depend on concrete details; rather details should depend on abstractions.”\n\nIn JS the __ concern__ of the dependancy inversion principle is to ensure decoupling between components and their depenencies.\n\n__Dependency Injection__ is __Not__ related to the principle of dependancy inversion.\n\n__DI__ a different aspect of __Inversion of Control__ in which the concern being inverted is \n__how a component obtains__ its dependencies rather than __who defines__ the interface.\n\nin DI dependencies are supplied to a component rather than the component obtaining the dependency by means of creating an instance of the dependency\n\nhttp://aspiringcraftsman.com/2012/01/22/solid-javascript-the-dependency-inversion-principle/", | |
"isSelected": false | |
}, | |
{ | |
"id": "eb4c4de4-b947-11e8-96f8-529269fb1459", | |
"title": "SLIDE _10- ISP", | |
"contents": "__Interface Segregation Principle__\n===\n-----\n\n\n#### The cohesion of __interfaces within a system__.\n\n##### ... JS does not have interfaces ...\n\n>#### Clients should not be forced to depend on __methods__ they do not use.\n\n__The operation’s signature__ :\nEvery operation declared by an object specifies the operation’s name, the objects it takes as parameters, and the operation’s return value. \n\n__The set of all signatures__ defined by an object’s operations is called the interface to the object.\n\nhttp://aspiringcraftsman.com/2012/01/08/solid-javascript-the-interface-segregation-principle/", | |
"isSelected": false | |
}, | |
{ | |
"id": "eb4c5032-b947-11e8-96f8-529269fb1459", | |
"title": "SLIDE _9 - LSP", | |
"contents": "__Liskov substitution__\n===\n---\n\n\n> ### Any extended entity should be able to replace the entity which it is an extension of without modifiyng the __behavior__ of the application.\n\n```\nif S is a subtype of T, \nthen objects of type T\nin a program may be replaced \nwith objects of type S \nwithout altering any of the desirable properties of that program```\n\n\n\n\n#### “a violation of LSP is a latent violation of OCP”.\n\n\nhttp://aspiringcraftsman.com/2011/12/31/solid-javascript-the-liskov-substitution-principle/", | |
"isSelected": false | |
}, | |
{ | |
"id": "eb4c5244-b947-11e8-96f8-529269fb1459", | |
"title": "SLIDE 8 OCP", | |
"contents": "__Open/closed__\n==\n----\n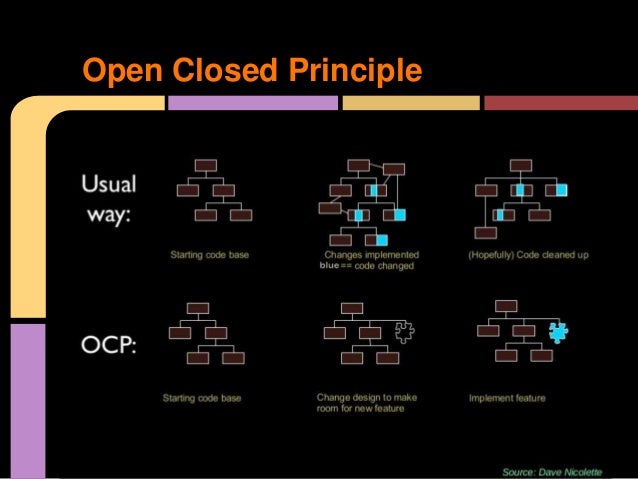\n\n>#### Software entities (classes, modules, functions, etc.) should be __open for extension but closed for modification__.\n\n#### The __foundation__ for building reusable maintainable code.\n\n\n\n #### __open for extension:__ reusing an element in different contexts\n\n#### __ closed to modification__ the changes do not require a modification in the behaviour of the element.\n\n### __Leads to__ \n* #### Loose coupling\n* #### improve readability\n* #### reducing the risk of breaking existing functionality.\n\nhttp://aspiringcraftsman.com/2011/12/19/solid-javascript-the-openclosed-principle/", | |
"isSelected": false | |
}, | |
{ | |
"id": "eb4c5d20-b947-11e8-96f8-529269fb1459", | |
"title": "SLIDE 7 SRP", | |
"contents": "__Single Responsibility__\n===\n-----\n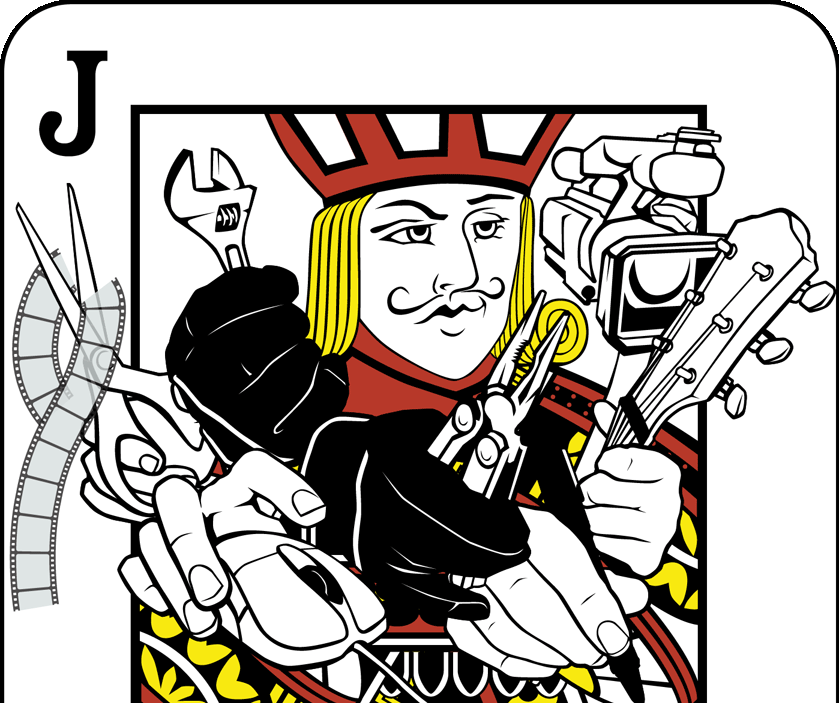\n> ### A class should have __only one reason to change__\n\n### __NOT__ the _same as only do one thing_.\n\n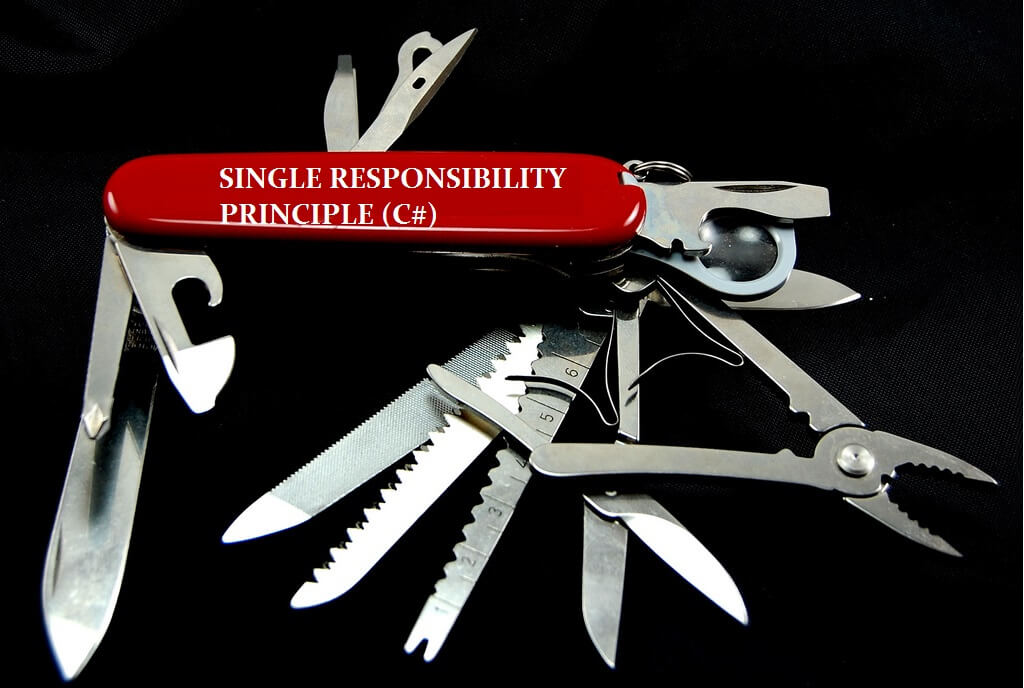\n\n----\n### An object should have a __cohesive set of behaviors__, together comprising a single responsibility that, if changed, would require the modification of the object’s definition. \n\n### __cohesion__\n* #### derives from the functional relatedness of elements in a module.\n\n* #### This leads to better/concise tests and improved code reliability\n \n### code smells:\n * #### large setups for testing,\n * #### more than one contextually different piece of code\n\nhttp://aspiringcraftsman.com/2011/12/08/solid-javascript-single-responsibility-principle/", | |
"isSelected": false | |
}, | |
{ | |
"id": "eb4c5f64-b947-11e8-96f8-529269fb1459", | |
"title": "SLIDE 6 - Princips of OOD", | |
"contents": "__Principles of Object Oriented Design__\n===\n---\n#### Principles are distillation of __patterns__ in nature.\n#### __ Principles__ constrain our code and helps keep our code simple and bug free.\n\n #### __Robert C. Martin__ promoted 11 Principles\n #### Detailing how to __design (5) and package (6)__ software.\n \n\n#### Michael Feathers coined the term __SOLID PRINCIPLES__ \n\n#### http://butunclebob.com/ArticleS.UncleBob.PrinciplesOfOod\n\n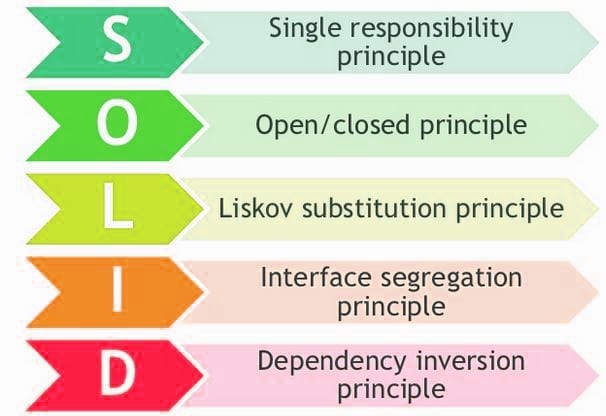", | |
"isSelected": false | |
}, | |
{ | |
"id": "eb4c6180-b947-11e8-96f8-529269fb1459", | |
"title": "SLIDE 5 - GOF", | |
"contents": "### __Patterns__ are __repetitive__ designs;\n### __simplify__ solutions to common problems.\n\n __The__ Gang of Four\n====\n-----\n#### __Design Patterns__: Elements of Reusable Object-Oriented Software \n##### by _Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides_.\n----\n<table>\n<tr>\n<th>__Creational patterns (5)__</th>\n<th>__Structural patterns (7)__</th>\n<th>__Behavioral patterns (11)__</th>\n</tr>\n<tr>\n<td>Factory method pattern</td>\n<td>Adapter pattern</td>\n<td>Chain-of-responsibility pattern</td>\n</tr>\n<tr>\n<td>Abstract factory pattern</td>\n<td>Bridge pattern</td>\n<td>Command pattern</td>\n</tr>\n<tr>\n<td>Singleton pattern</td>\n<td>Composite pattern</td>\n<td>Interpreter pattern</td>\n</tr>\n<tr>\n<td>Builder pattern</td>\n<td>Decorator pattern</td>\n<td>Iterator pattern</td>\n</tr>\n<tr>\n<td>Prototype pattern</td>\n<td>Facade pattern</td>\n<td>Mediator pattern</td>\n</tr>\n<tr>\n<td></td>\n<td>Flyweight pattern</td>\n<td>Memento pattern</td>\n</tr>\n<tr>\n<td></td>\n<td>Proxy pattern</td>\n<td>Observer pattern</td>\n</tr>\n<tr>\n<td></td>\n<td></td>\n<td>State pattern</td>\n</tr>\n<tr>\n<td></td>\n<td></td>\n<td>Strategy pattern</td>\n</tr>\n<tr>\n<td></td>\n<td></td>\n<td>Template method pattern</td>\n</tr>\n<tr>\n<td></td>\n<td></td>\n<td>Visitor pattern</td>\n</tr>\n</table>\n", | |
"isSelected": false | |
}, | |
{ | |
"id": "eb4c63a6-b947-11e8-96f8-529269fb1459", | |
"title": "SLIDE 4 - Princ of Design", | |
"contents": "# __PRINCIPLES (...OF DESIGN)__\n----------------\n\n### Occam's razor\n >\"the simpleset solution is generely the correct one\"\n\n### Hanlon's razor:\n > \"Never attribute to malice that which is adequately explained by stupidity\"\n\n### __QED__ \n#### The __less complex__ the system - the more correct it will tend to be.", | |
"isSelected": false | |
}, | |
{ | |
"id": "eb4c6a40-b947-11e8-96f8-529269fb1459", | |
"title": "SLIDE 3 bad design", | |
"contents": "# __On Bad Design__\n--------------\n### At the heart of this issue is our __lack__ of a good working _definition_ of “bad” design.\n\n**Uncle Bob**: http://butunclebob.com/ArticleS.UncleBob.PrinciplesOfOod\n\n### I would say that a system that allowed other metathings to be done in the ordinary course of programming (like __changing__ what inheritance means, or what is an instance) is a bad design.\n\n**Alan Kay** http://lists.squeakfoundation.org/pipermail/squeak-dev/1998-October/017019.html\n\n----------------------\n\n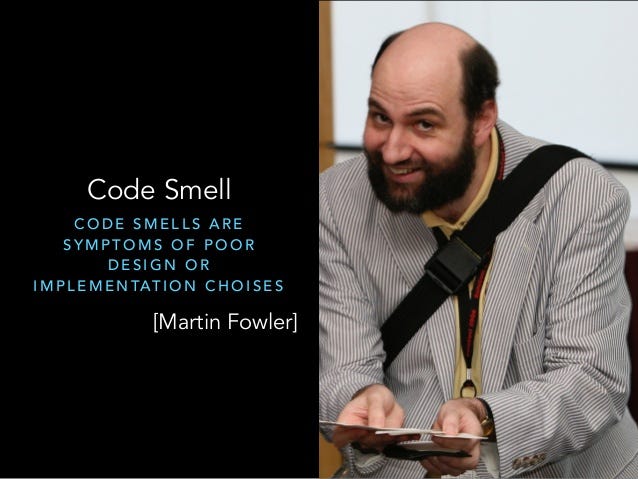\n## A piece of software that __fulfills__ its requirements and yet __exhibits__ any or all of the following three traits has a bad design.\n\n### __Rigidity__\n#### It is hard to change. (because every change affects too many other parts of the system.)\n### __Fragility__\n#### When you make a change, unexpected parts of the system break.\n### __Immobility__\n#### It is hard to reuse in another application. (because it cannot be disentangled from the current application)", | |
"isSelected": false | |
}, | |
{ | |
"id": "eb4c6c66-b947-11e8-96f8-529269fb1459", | |
"title": "SLIDE 2 good design", | |
"contents": "# __On Good Design__\n--------------\n# The __key__ in making great and growable systems is much more to design how its modules __communicate__ rather than what their internal properties and behaviors should be. \n\n**Alan Kay**\n http://lists.squeakfoundation.org/pipermail/squeak-dev/1998-October/017019.html\n\n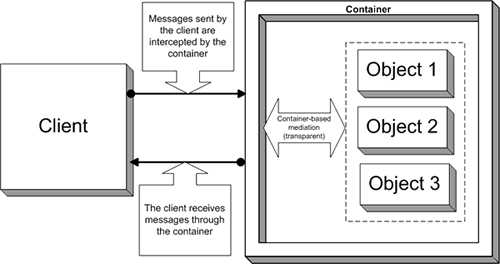", | |
"isSelected": false | |
}, | |
{ | |
"id": "eb4c6ef0-b947-11e8-96f8-529269fb1459", | |
"title": "SLIDE 01 What?", | |
"contents": "# __What goes wrong with software?__\n-----------------------------\n\n>### __Efficient__ code organization is about Dependency Management.\n***Uncle Bob\n\n### __Most__ software engineers don’t set out to create “bad designs”.\n\n### Yet __most__ software eventually degrades to the point where someone will declare the design to be unsound.\n\n### __Why__ does this happen?\n\n### __Was__ the design poor to begin with, or did the design actually degrade like a piece of rotten meat?\n\n**Uncle Bob** http://butunclebob.com/ArticleS.UncleBob.PrinciplesOfOod\n", | |
"isSelected": false | |
}, | |
{ | |
"id": "eb4c7120-b947-11e8-96f8-529269fb1459", | |
"title": "INTRO", | |
"contents": "\n\n\n # __A significant barrier to progress__ _in computer science_ \n## is the fact that many practitioners are ignorant of the history of computer science: old accomplishments (and failures!) are forgotten, and consequently the industry reinvents itself every 5-10 years. \n\n**Alan Kay** The Cuneiform Tablets of 2015\n http://www.vpri.org/pdf/tr2015004_cuneiform.pdf\n" , | |
"isSelected": false | |
} | |
] | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment