Last active
November 18, 2024 23:03
-
-
Save britweb-tim/f9d06fc5fc70fd1d8188c1ffcaa8d306 to your computer and use it in GitHub Desktop.
Add a WP CLI Command for importing woocommerce products from csv
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
// Add a WP CLI Command which allows import of CSV files from the command line. | |
if ( defined( 'WP_CLI' ) && WP_CLI ) { | |
require_once WP_PLUGIN_DIR . '/woocommerce/includes/import/class-wc-product-csv-importer.php'; | |
// Maybe require and invoke any other filters you need here as theme/plugins may not be loaded at this point. | |
class WP_CLI_WC_Import_CSV { | |
protected $_mappings = [ | |
'from'=>[], | |
'to'=>[] | |
]; | |
public function __invoke($args, $assoc_args) { | |
$filename = realpath($args[0]); | |
if(!file_exists($filename)) { | |
WP_CLI::error('File not found : '.$filename); | |
return; | |
} | |
if ( ! current_user_can( 'manage_product_terms' ) ) { | |
WP_CLI::error('Current user cannot manage categories, ensure you set the --user parameter'); | |
return; | |
} | |
$params = [ | |
'mapping'=>$this->_readMappings(realpath($assoc_args['mappings'])), | |
'update_existing'=>array_key_exists('update', $assoc_args) ? true : false, | |
'prevent_timeouts'=>false, | |
'parse'=>true, | |
]; | |
$importer = new WC_Product_CSV_Importer($filename, $params); | |
$result = $importer->import(); | |
WP_CLI::success( print_r($result, true) ); | |
} | |
protected function _readMappings($filename) { | |
$mappings = []; | |
$row = 1; | |
if(($fh = fopen($filename, 'r')) !== FALSE) {; | |
while(($data = fgetcsv($fh)) !== FALSE) { | |
if($row > 1) { | |
$mappings['from'][] = $data[0]; | |
$mappings['to'][] = $data[1]; | |
} | |
$row++; | |
} | |
} | |
fclose($fh); | |
return $mappings; | |
} | |
} | |
$instance = new WP_CLI_WC_Import_CSV(); | |
WP_CLI::add_command('wc import-csv', $instance,[ | |
'shortdesc'=>'Import woocommerce products using the standard CSV import', | |
'synopsis'=>[ | |
[ | |
'type'=>'positional', | |
'name'=>'file', | |
'optional'=>false, | |
'repeating'=>false, | |
], | |
[ | |
'type'=>'assoc', | |
'name'=>'mappings', | |
'description'=>'Mappings csv file, with "from" and "to" column headers. The "to" column matches to "Maps to product property" on the import schema. More details here at https://github.com/woocommerce/woocommerce/wiki/Product-CSV-Import-Schema', | |
'optional'=>false, | |
], | |
[ | |
'type'=>'assoc', | |
'name'=>'delimiter', | |
'description'=>'Delimeter for csv file (defaults to comma)', | |
'default'=>',', | |
'optional'=>true | |
], | |
[ | |
'type'=>'flag', | |
'name'=>'update', | |
'description'=>'Update existing products matched on ID or SKU, omit this flag to insert new products. Those which exist will be skipped.', | |
'optional'=>true | |
] | |
] | |
]); | |
} | |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Thanks for providing this!
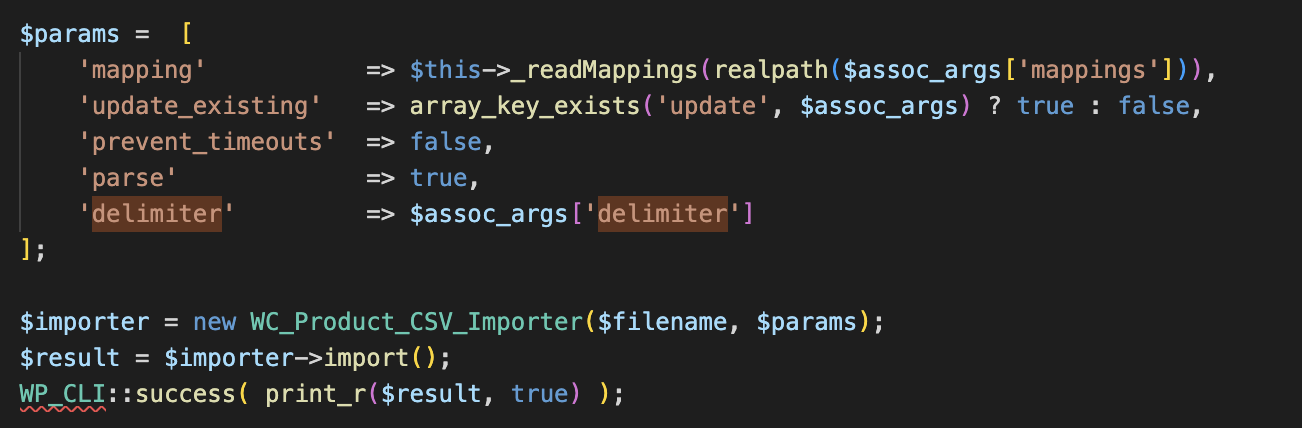
I've noticed that you forgot to pass the delimiter to the csv importer class.
This should do the trick:
Also note that you should probably use the same delimiter in the fgetcsv because otherwise fgetcsv will always use comma as a separator.