Last active
February 10, 2020 09:24
-
-
Save christian-mann/7d241f3f17d15ca79f364e72b0ae422f to your computer and use it in GitHub Desktop.
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// ==UserScript== | |
// @name Show Invasions on Group Page TTR | |
// @namespace https://github.com/christian-mann | |
// @version 1.1 | |
// @description Show invading cogs on the groups page of https://toonhq.org/groups so that it's easier to find a group for the cog you're looking for | |
// @author Christian Mann (aka Lucky Penny) | |
// @match https://toonhq.org/groups/ | |
// @grant GM.xmlHttpRequest | |
// ==/UserScript== | |
// configuration -- if you turn everything off nothing will happen | |
const SHOW_COG_NAMES = false; | |
const SHOW_COG_THUMB = false; // only applies if SHOW_COG_NAMES is true | |
const SHOW_COG_HERO = true; | |
function setIntervalImmediate(fn, time) { | |
fn(); | |
setInterval(fn, time); | |
} | |
function getCogUrl(name) { | |
var slugged = name.toLowerCase(); | |
slugged = slugged.replace(/[^\x20-\x7F]/g, ''); // apparently they give us Blood\u0003sucker for some reason | |
slugged = slugged.replace(/ |-/g, '_'); | |
slugged = slugged.replace(/&/g, 'and'); | |
if (slugged.startsWith("back")) { slugged = "backstabber"; } // ugh | |
return 'https://toonhq.org/static/2.4.4/img/cogs/' + slugged + '.png'; | |
} | |
function getCogBig(name) { | |
return '<img class="cog-big" style="position: absolute; right: 5%; top: 20%; height: 50%; opacity: 0.7" src="' + getCogUrl(name) + '" />'; | |
} | |
function getCogThumb(name) { | |
return '<img style="display: inline-block; height: 1em;" src="' + getCogUrl(name) + '" />'; | |
} | |
(function() { | |
'use strict'; | |
//console.log(GM); | |
setIntervalImmediate(function() { | |
GM.xmlHttpRequest({ | |
method: "GET", | |
url: 'https://toonhq.org/api/v1/invasion/', | |
onload: function(response) { | |
//console.log(response); | |
var existing_bigs = document.querySelectorAll('.cog-big'); | |
console.log(existing_bigs); | |
var json = JSON.parse(response.responseText); | |
//console.log(json); | |
var group_districts = document.querySelectorAll('.groups-list div.media-group div.media-body p:nth-child(3)'); | |
for (var invasion of json.invasions) { | |
//console.log(invasion.district); | |
for (var group_district of group_districts) { | |
if (group_district.innerText.startsWith(invasion.district)) { | |
if (SHOW_COG_NAMES) { | |
group_district.innerHTML = invasion.district + | |
' <span style="font-style: italic; font-color: #888">(' + | |
invasion.cog + | |
(SHOW_COG_THUMB ? (' ' + getCogThumb(invasion.cog)) : '') + | |
')</span>'; | |
} | |
if (SHOW_COG_HERO) { | |
group_district.parentElement.style.position = "relative"; | |
group_district.parentElement.insertAdjacentHTML('beforeend', getCogBig(invasion.cog)); | |
} | |
} | |
} | |
} | |
console.log(existing_bigs); | |
for (var big of existing_bigs) { | |
big.remove(); | |
} | |
} | |
}); | |
}, 5000); | |
})(); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
This is what the page looks like with every setting turned on. Personally, I prefer to just have SHOW_COG_HERO turned on and everything else off.
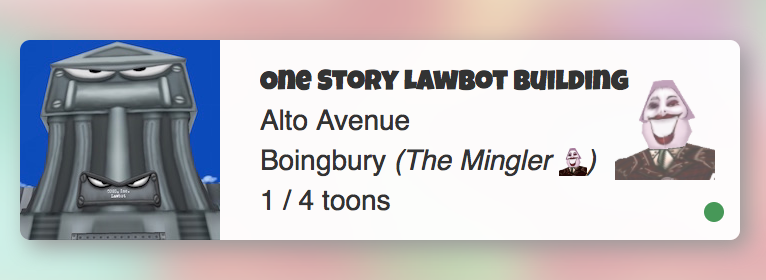