Created
August 5, 2013 18:49
-
-
Save cwebber314/6158360 to your computer and use it in GitHub Desktop.
ACCC_report.py
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#Created by knucklehead on 07-20-2013 | |
#Developed with Python 2.7.3, EXCEL 2013, and PSSE 33.4 | |
#This Module Creates an Excel Spreadsheet Comparing Results of Up to Eight(8) PSSE ACCC Solution Files | |
#Prompts for Contingency Low Bus Voltage Threshold to Report | |
#Option to Enable High Bus Voltage Reporting (GetHighV) | |
#Prompts for Contingency High Bus Voltage Threshold to Report (if GetHighV enabled) | |
#Prompts for Contingency High Branch Flow Threshold to Report | |
#Will Ask for How Many ACCC Files to Compare | |
#Prompts to Input the ACCC Files (Choose Files in the order Desired) | |
#Has Options to Report All Violations, Worst Violations, or Both on EXCEL Sheets | |
#Writes and Saves an EXCEL Report file with the name: ACCC_Report + Date... Example: ACCC_Report(070713).xlsx | |
#Writes and Saves a PSSE TEXT Report (if Enabled Below) With the Name: ACCC_PSSE_Report + Date... Example: ACCC_PSSE_Report(070713).xlsx | |
#Use it Any Way You Like ... DNL | |
#Default Options --------------------------------------------------------------- | |
BaseVLowCriteria = 0.950 #N-0 pu Low Voltage Criteria (ERCOT uses 0.950) | |
BaseVHighCriteria = 1.050 #N-0 pu Low Voltage Criteria (ERCOT uses 1.050) | |
VLowCriteria = 0.900 #Contingency pu Low Voltage Criteria (ERCOT uses 0.900) | |
VHighCriteria = 1.050 #Contingency pu High Voltage Criteria (ERCOT uses 1.050) | |
IHighCriteria = 100.0 #Contingency Percent High Flow Criteria (All RTOs use 100.0) | |
MVARatingCriteria = 'B' #Contingency Rating Criteria for Branch Flow (Enter as Text) | |
BaseVLow = 0.950 #Per Unit N-0 Low Voltage Violation Level to Report | |
BaseVHigh = 1.050 #Per Unit N-0 High Voltage Violation Level to Report | |
BaseIHigh = 95.0 #Percent N-0 High Flow Violation Level to Report | |
ExtremeBaseVLow = 0.920 #Per Unit N-0 Extreme Low Voltage Violation (for EXCEL Highlighting) | |
ExtremeBaseVHigh = 1.100 #Per Unit N-0 Extreme High Voltage (for EXCEL Highlighting) | |
ExtremeBaseIHigh = 110.0 #Percent N-0 Extreme High Flow Violation (for EXCEL Highlighting) | |
ExtremeVLow = 0.850 #Per Unit Contingency Extreme Low Voltage (for EXCEL Highlighting) | |
ExtremeVHigh = 1.150 #Per Unit Contingency Extreme High Voltage (for EXCEL Highlighting) | |
ExtremeIHigh = 125.0 #Percent Contingency Extreme High Flow (for EXCEL Highlighting) | |
DefaultGetHighV = False #Default Enable for Reporting High Voltage Violations | |
DefaultVHigh = '1.050' #Default Contingency Per Unit High Voltage Violation Level to Report (Enter as Text) | |
DefaultVLow = '0.900' #Default Contingency Per Unit Low Voltage Violation Level to Report (Enter as Text) | |
DefaultIHigh = '95.0' #Default Contingency Percent Flow Violation Level (Enter as Text) | |
DefaultGetWorst = 2 #Default Contingency Violation Reporting Option: 0=All, 1=Worst, 2=Both | |
TextReport = False #Option for PSSE Generated 'Multiple AC Contingency Run Report' | |
sleeptime=10 #Pause Time for Output Console Window | |
import wx | |
from datetime import date | |
#--- ACCC GUI ------------------------------------------------------------------ | |
def create(parent): | |
return Frame1(parent) | |
[wxID_FRAME1, wxID_FRAME1BUTTON1, wxID_FRAME1BUTTON2, wxID_FRAME1CHECKBOX2, | |
wxID_FRAME1PANEL1, wxID_FRAME1RADIOBOX1, wxID_FRAME1RADIOBOX3, | |
wxID_FRAME1STATICTEXT1, wxID_FRAME1STATICTEXT11, wxID_FRAME1STATICTEXT2, | |
wxID_FRAME1STATICTEXT3, wxID_FRAME1STATICTEXT4, wxID_FRAME1STATICTEXT5, | |
wxID_FRAME1STATICTEXT6, wxID_FRAME1STATICTEXT7, wxID_FRAME1STATICTEXT8, | |
wxID_FRAME1STATICTEXT9, wxID_FRAME1TEXTCTRL1, wxID_FRAME1TEXTCTRL10, | |
wxID_FRAME1TEXTCTRL11, wxID_FRAME1TEXTCTRL2, wxID_FRAME1TEXTCTRL3, | |
wxID_FRAME1TEXTCTRL4, wxID_FRAME1TEXTCTRL5, wxID_FRAME1TEXTCTRL6, | |
wxID_FRAME1TEXTCTRL7, wxID_FRAME1TEXTCTRL8, wxID_FRAME1TEXTCTRL9, | |
] = [wx.NewId() for _init_ctrls in range(28)] | |
class Frame1(wx.Frame): | |
def _init_coll_boxSizer9_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.textCtrl3, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddWindow(self.staticText3, 0, border=4, flag=wx.TOP | wx.BOTTOM) | |
def _init_coll_boxSizer7_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.textCtrl5, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddWindow(self.staticText5, 0, border=4, flag=wx.BOTTOM | wx.TOP) | |
def _init_coll_boxSizer8_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.textCtrl4, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddWindow(self.staticText4, 0, border=4, flag=wx.TOP | wx.BOTTOM) | |
def _init_coll_boxSizer15_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.textCtrl9, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(23, 8), border=0, flag=0) | |
parent.AddWindow(self.textCtrl10, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(23, 8), border=0, flag=0) | |
parent.AddWindow(self.textCtrl11, 0, border=0, flag=0) | |
def _init_coll_boxSizer6_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.textCtrl6, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddWindow(self.staticText6, 0, border=4, flag=wx.TOP | wx.BOTTOM) | |
def _init_coll_boxSizer13_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.radioBox1, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
def _init_coll_boxSizer4_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.textCtrl8, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddWindow(self.staticText8, 0, border=4, flag=wx.TOP | wx.BOTTOM) | |
def _init_coll_boxSizer11_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.textCtrl1, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddWindow(self.staticText1, 0, border=4, flag=wx.TOP | wx.BOTTOM) | |
def _init_coll_boxSizer12_Items(self, parent): | |
parent.AddSpacer(wx.Size(70, 8), border=0, flag=0) | |
parent.AddWindow(self.button1, 0, border=0, flag=0) | |
def _init_coll_boxSizer10_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.textCtrl2, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddWindow(self.staticText2, 0, border=4, flag=wx.TOP | wx.BOTTOM) | |
def _init_coll_boxSizer5_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.textCtrl7, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddWindow(self.staticText7, 0, border=4, flag=wx.TOP | wx.BOTTOM) | |
def _init_coll_boxSizer3_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.radioBox3, 0, border=0, flag=0) | |
def _init_coll_boxSizer14_Items(self, parent): | |
parent.AddSpacer(wx.Size(24, 8), border=0, flag=0) | |
parent.AddWindow(self.staticText9, 0, border=3, flag=wx.BOTTOM) | |
parent.AddSpacer(wx.Size(23, 8), border=0, flag=0) | |
parent.AddWindow(self.checkBox2, 0, border=1, flag=wx.BOTTOM) | |
parent.AddSpacer(wx.Size(23, 8), border=0, flag=0) | |
parent.AddWindow(self.staticText11, 0, border=3, flag=wx.BOTTOM) | |
def _init_coll_boxSizer1_Items(self, parent): | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer14, 0, border=0, flag=0) | |
parent.AddSizer(self.boxSizer15, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer13, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer12, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer11, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer10, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer9, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer8, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer7, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer6, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer5, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer4, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer3, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSizer(self.boxSizer2, 0, border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
parent.AddSpacer(wx.Size(8, 8), border=0, flag=0) | |
def _init_coll_boxSizer2_Items(self, parent): | |
parent.AddSpacer(wx.Size(70, 8), border=0, flag=0) | |
parent.AddWindow(self.button2, 0, border=0, flag=0) | |
def _init_sizers(self): | |
self.boxSizer1 = wx.BoxSizer(orient=wx.VERTICAL) | |
self.boxSizer2 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer3 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer4 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer5 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer6 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer7 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer8 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer9 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer10 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer11 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer12 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer13 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer14 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self.boxSizer15 = wx.BoxSizer(orient=wx.HORIZONTAL) | |
self._init_coll_boxSizer1_Items(self.boxSizer1) | |
self._init_coll_boxSizer2_Items(self.boxSizer2) | |
self._init_coll_boxSizer3_Items(self.boxSizer3) | |
self._init_coll_boxSizer4_Items(self.boxSizer4) | |
self._init_coll_boxSizer5_Items(self.boxSizer5) | |
self._init_coll_boxSizer6_Items(self.boxSizer6) | |
self._init_coll_boxSizer7_Items(self.boxSizer7) | |
self._init_coll_boxSizer8_Items(self.boxSizer8) | |
self._init_coll_boxSizer9_Items(self.boxSizer9) | |
self._init_coll_boxSizer10_Items(self.boxSizer10) | |
self._init_coll_boxSizer11_Items(self.boxSizer11) | |
self._init_coll_boxSizer12_Items(self.boxSizer12) | |
self._init_coll_boxSizer13_Items(self.boxSizer13) | |
self._init_coll_boxSizer14_Items(self.boxSizer14) | |
self._init_coll_boxSizer15_Items(self.boxSizer15) | |
self.panel1.SetSizer(self.boxSizer1) | |
def _init_ctrls(self, prnt): | |
wx.Frame.__init__(self, id=wxID_FRAME1, name='', parent=prnt, | |
pos=wx.Point(684, 261), size=wx.Size(321, 582), | |
style=wx.DEFAULT_FRAME_STYLE, title='ACCC Report Options') | |
self.SetClientSize(wx.Size(305, 544)) | |
self.SetToolTipString('') | |
wx.Frame.Center(self,wx.BOTH) | |
self.panel1 = wx.Panel(id=wxID_FRAME1PANEL1, name='panel1', parent=self, | |
pos=wx.Point(0, 0), size=wx.Size(305, 544), | |
style=wx.TAB_TRAVERSAL) | |
self.panel1.SetToolTipString('') | |
self.radioBox1 = wx.RadioBox(choices=['1', '2', '3', '4', '5', '6', '7', | |
'8'], id=wxID_FRAME1RADIOBOX1, label='Number of ACC Files', | |
majorDimension=1, name='radioBox1', parent=self.panel1, | |
pos=wx.Point(24, 69), size=wx.Size(256, 48), | |
style=wx.RAISED_BORDER | wx.RA_SPECIFY_ROWS) | |
self.radioBox1.SetToolTipString('') | |
self.radioBox1.SetThemeEnabled(False) | |
self.radioBox1.SetSelection(0) | |
self.radioBox1.Bind(wx.EVT_RADIOBOX, self.OnRadioBox1Radiobox, | |
id=wxID_FRAME1RADIOBOX1) | |
self.button1 = wx.Button(id=wxID_FRAME1BUTTON1, label='Get File(s)', | |
name='button1', parent=self.panel1, pos=wx.Point(70, 125), | |
size=wx.Size(76, 23), style=0) | |
self.button1.SetToolTipString('') | |
self.button1.SetFocus() | |
self.button1.Bind(wx.EVT_BUTTON, self.OnButton1Button, | |
id=wxID_FRAME1BUTTON1) | |
self.textCtrl1 = wx.TextCtrl(id=wxID_FRAME1TEXTCTRL1, name='textCtrl1', | |
parent=self.panel1, pos=wx.Point(24, 156), size=wx.Size(168, 21), | |
style=wx.SUNKEN_BORDER | wx.TE_CENTER, value='None') | |
self.textCtrl1.Enable(True) | |
self.textCtrl1.SetToolTipString('') | |
self.textCtrl1.SetEditable(False) | |
self.textCtrl1.Enable(True) | |
self.textCtrl2 = wx.TextCtrl(id=wxID_FRAME1TEXTCTRL2, name='textCtrl2', | |
parent=self.panel1, pos=wx.Point(24, 185), size=wx.Size(168, 21), | |
style=wx.SUNKEN_BORDER | wx.TE_CENTER, value='None') | |
self.textCtrl2.SetEditable(False) | |
self.textCtrl2.SetToolTipString('') | |
self.textCtrl2.Enable(False) | |
self.textCtrl3 = wx.TextCtrl(id=wxID_FRAME1TEXTCTRL3, name='textCtrl3', | |
parent=self.panel1, pos=wx.Point(24, 214), size=wx.Size(168, 21), | |
style=wx.SUNKEN_BORDER | wx.TE_CENTER, value='None') | |
self.textCtrl3.Enable(True) | |
self.textCtrl3.SetToolTipString('') | |
self.textCtrl3.SetEditable(False) | |
self.textCtrl3.Enable(False) | |
self.textCtrl4 = wx.TextCtrl(id=wxID_FRAME1TEXTCTRL4, name='textCtrl4', | |
parent=self.panel1, pos=wx.Point(24, 243), size=wx.Size(168, 21), | |
style=wx.SUNKEN_BORDER | wx.TE_CENTER, value='None') | |
self.textCtrl4.Enable(True) | |
self.textCtrl4.SetToolTipString('') | |
self.textCtrl4.SetEditable(False) | |
self.textCtrl4.Enable(False) | |
self.textCtrl5 = wx.TextCtrl(id=wxID_FRAME1TEXTCTRL5, name='textCtrl5', | |
parent=self.panel1, pos=wx.Point(24, 272), size=wx.Size(168, 21), | |
style=wx.SUNKEN_BORDER | wx.TE_CENTER, value='None') | |
self.textCtrl5.Enable(True) | |
self.textCtrl5.SetToolTipString('') | |
self.textCtrl5.SetEditable(False) | |
self.textCtrl5.Enable(False) | |
self.textCtrl6 = wx.TextCtrl(id=wxID_FRAME1TEXTCTRL6, name='textCtrl6', | |
parent=self.panel1, pos=wx.Point(24, 301), size=wx.Size(168, 21), | |
style=wx.SUNKEN_BORDER | wx.TE_CENTER, value='None') | |
self.textCtrl6.Enable(True) | |
self.textCtrl6.SetToolTipString('') | |
self.textCtrl6.SetEditable(False) | |
self.textCtrl6.Enable(False) | |
self.textCtrl7 = wx.TextCtrl(id=wxID_FRAME1TEXTCTRL7, name='textCtrl7', | |
parent=self.panel1, pos=wx.Point(24, 330), size=wx.Size(168, 21), | |
style=wx.SUNKEN_BORDER | wx.TE_CENTER, value='None') | |
self.textCtrl7.Enable(True) | |
self.textCtrl7.SetToolTipString('') | |
self.textCtrl7.SetEditable(False) | |
self.textCtrl7.Enable(False) | |
self.textCtrl8 = wx.TextCtrl(id=wxID_FRAME1TEXTCTRL8, name='textCtrl8', | |
parent=self.panel1, pos=wx.Point(24, 359), size=wx.Size(168, 21), | |
style=wx.SUNKEN_BORDER | wx.TE_CENTER, value='None') | |
self.textCtrl8.Enable(True) | |
self.textCtrl8.SetToolTipString('') | |
self.textCtrl8.SetEditable(False) | |
self.textCtrl8.Enable(False) | |
self.button2 = wx.Button(id=wxID_FRAME1BUTTON2, label='Run Report', | |
name='button2', parent=self.panel1, pos=wx.Point(70, 504), | |
size=wx.Size(76, 23), style=0) | |
self.button2.SetToolTipString('') | |
self.button2.Bind(wx.EVT_BUTTON, self.OnButton2Button, | |
id=wxID_FRAME1BUTTON2) | |
self.staticText1 = wx.StaticText(id=wxID_FRAME1STATICTEXT1, | |
label='<--- ACC File 1', name='staticText1', parent=self.panel1, | |
pos=wx.Point(200, 160), size=wx.Size(80, 13), style=0) | |
self.staticText1.SetToolTipString('') | |
self.staticText2 = wx.StaticText(id=wxID_FRAME1STATICTEXT2, | |
label='<--- ACC File 2', name='staticText2', parent=self.panel1, | |
pos=wx.Point(200, 189), size=wx.Size(80, 13), style=0) | |
self.staticText2.SetToolTipString('') | |
self.staticText3 = wx.StaticText(id=wxID_FRAME1STATICTEXT3, | |
label='<--- ACC File 3', name='staticText3', parent=self.panel1, | |
pos=wx.Point(200, 218), size=wx.Size(80, 13), style=0) | |
self.staticText3.SetToolTipString('') | |
self.staticText4 = wx.StaticText(id=wxID_FRAME1STATICTEXT4, | |
label='<--- ACC File 4', name='staticText4', parent=self.panel1, | |
pos=wx.Point(200, 247), size=wx.Size(80, 13), style=0) | |
self.staticText4.SetToolTipString('') | |
self.staticText5 = wx.StaticText(id=wxID_FRAME1STATICTEXT5, | |
label='<--- ACC File 5', name='staticText5', parent=self.panel1, | |
pos=wx.Point(200, 276), size=wx.Size(80, 13), style=0) | |
self.staticText5.SetToolTipString('') | |
self.staticText6 = wx.StaticText(id=wxID_FRAME1STATICTEXT6, | |
label='<--- ACC File 6', name='staticText6', parent=self.panel1, | |
pos=wx.Point(200, 305), size=wx.Size(80, 13), style=0) | |
self.staticText6.SetToolTipString('') | |
self.staticText7 = wx.StaticText(id=wxID_FRAME1STATICTEXT7, | |
label='<--- ACC File 7', name='staticText7', parent=self.panel1, | |
pos=wx.Point(200, 334), size=wx.Size(80, 13), style=0) | |
self.staticText7.SetToolTipString('') | |
self.staticText8 = wx.StaticText(id=wxID_FRAME1STATICTEXT8, | |
label='<--- ACC File 8', name='staticText8', parent=self.panel1, | |
pos=wx.Point(200, 363), size=wx.Size(80, 13), style=0) | |
self.staticText8.SetToolTipString('') | |
self.staticText9 = wx.StaticText(id=wxID_FRAME1STATICTEXT9, | |
label='VLow (pu)', name='staticText9', parent=self.panel1, | |
pos=wx.Point(24, 16), size=wx.Size(70, 13), | |
style=wx.ALIGN_CENTRE) | |
self.staticText9.SetToolTipString('') | |
self.staticText11 = wx.StaticText(id=wxID_FRAME1STATICTEXT11, | |
label='Loading (%)', name='staticText11', parent=self.panel1, | |
pos=wx.Point(210, 16), size=wx.Size(70, 13), | |
style=wx.ALIGN_CENTRE) | |
self.staticText11.SetToolTipString('') | |
self.textCtrl9 = wx.TextCtrl(id=wxID_FRAME1TEXTCTRL9, name='textCtrl9', | |
parent=self.panel1, pos=wx.Point(24, 32), size=wx.Size(70, 21), | |
style=wx.SUNKEN_BORDER | wx.TE_CENTER, value=DefaultVLow) | |
self.textCtrl9.SetToolTipString('') | |
self.textCtrl9.Bind(wx.EVT_TEXT, self.OnTextCtrl9Text, | |
id=wxID_FRAME1TEXTCTRL9) | |
self.textCtrl10 = wx.TextCtrl(id=wxID_FRAME1TEXTCTRL10, | |
name='textCtrl10', parent=self.panel1, pos=wx.Point(117, 32), | |
size=wx.Size(70, 21), style=wx.SUNKEN_BORDER | wx.TE_CENTER, | |
value=DefaultVHigh) | |
self.textCtrl10.SetToolTipString('') | |
self.textCtrl10.Enable(False) | |
self.textCtrl10.Bind(wx.EVT_TEXT, self.OnTextCtrl10Text, | |
id=wxID_FRAME1TEXTCTRL10) | |
self.textCtrl11 = wx.TextCtrl(id=wxID_FRAME1TEXTCTRL11, | |
name='textCtrl11', parent=self.panel1, pos=wx.Point(210, 32), | |
size=wx.Size(70, 21), style=wx.SUNKEN_BORDER | wx.TE_CENTER, | |
value=DefaultIHigh) | |
self.textCtrl11.SetToolTipString('') | |
self.textCtrl11.Bind(wx.EVT_TEXT, self.OnTextCtrl11Text, | |
id=wxID_FRAME1TEXTCTRL11) | |
self.checkBox2 = wx.CheckBox(id=wxID_FRAME1CHECKBOX2, | |
label='VHigh (pu)', name='checkBox2', parent=self.panel1, | |
pos=wx.Point(117, 16), size=wx.Size(70, 13), style=0) | |
self.checkBox2.SetValue(DefaultGetHighV) | |
self.checkBox2.SetToolTipString('') | |
self.checkBox2.Bind(wx.EVT_CHECKBOX, self.OnCheckBox2Checkbox, | |
id=wxID_FRAME1CHECKBOX2) | |
self.radioBox3 = wx.RadioBox(choices=['<--- Report All Violations', | |
'<--- Report Worst Violations', '<--- Report Both'], | |
id=wxID_FRAME1RADIOBOX3, | |
label='CONTINGENCY VIOLATION REPORTING OPTIONS:', | |
majorDimension=1, name='radioBox3', parent=self.panel1, | |
pos=wx.Point(24, 404), size=wx.Size(256, 84), | |
style=wx.RAISED_BORDER | wx.RA_SPECIFY_COLS) | |
self.radioBox3.SetSelection(DefaultGetWorst) | |
self.radioBox3.SetToolTipString('') | |
self.radioBox3.Bind(wx.EVT_RADIOBOX, self.OnRadioBox3Radiobox, | |
id=wxID_FRAME1RADIOBOX3) | |
self._init_sizers() | |
def __init__(self, parent): | |
self._init_ctrls(parent) | |
#-- GUI EVENTS ------------------------------------------------------------- | |
#-- High Voltage Enable ---------------------------------------------------- | |
def OnCheckBox2Checkbox(self, event): | |
gethighv = bool(self.checkBox2.GetValue()) | |
self.textCtrl10.Enable(gethighv) | |
self.GetHighV = gethighv | |
#-- High Voltage Selection ------------------------------------------------- | |
def OnTextCtrl10Text(self, event): | |
self.VHigh = float(self.textCtrl10.GetValue()) | |
#-- Low Voltge Selection --------------------------------------------------- | |
def OnTextCtrl9Text(self, event): | |
self.VLow=float(self.textCtrl9.GetValue()) | |
#-- High Loading Selection ------------------------------------------------- | |
def OnTextCtrl11Text(self, event): | |
self.IHigh = float(self.textCtrl11.GetValue()) | |
#-- Number of ACC Files Selection ------------------------------------------ | |
def OnRadioBox1Radiobox(self, event): | |
displaydict={0:self.textCtrl1,1:self.textCtrl2,2:self.textCtrl3,3:self.textCtrl4, | |
4:self.textCtrl5,5:self.textCtrl6,6:self.textCtrl7,7:self.textCtrl8} | |
for i in displaydict: displaydict[i].SetValue('None') | |
self.numaccfiles = self.radioBox1.GetSelection()+1 | |
for j in range(self.numaccfiles): | |
displaydict[j].Enable(1) | |
for k in range(j+1,8): | |
displaydict[k].Enable(0) | |
#-- Get ACC Files Button --------------------------------------------------- | |
def OnButton1Button(self, event): | |
displaydict={0:self.textCtrl1,1:self.textCtrl2,2:self.textCtrl3,3:self.textCtrl4, | |
4:self.textCtrl5,5:self.textCtrl6,6:self.textCtrl7,7:self.textCtrl8} | |
self.accfilelist=['','','','','','','',''] | |
wildcard = "ACCC File (*.acc)|*.acc|"\ | |
"All files (*.*)|*.*" | |
for i in range(self.numaccfiles): | |
title='Select ACCC File {0:1}'.format(i) | |
dialog = wx.FileDialog(None, title, os.getcwd(), "", wildcard, wx.OPEN|wx.CHANGE_DIR) | |
dialog.Center(wx.BOTH) | |
dialog.ShowModal() | |
fname=dialog.GetPath().encode("ascii") | |
path,fname=os.path.split(fname) | |
self.accfilelist[i]=fname | |
displaydict[i].SetValue(fname) | |
dialog.Destroy() | |
#-- Contingency Violation Options ------------------------------------------ | |
def OnRadioBox3Radiobox(self, event): | |
self.GetWorst = self.radioBox3.GetSelection() | |
#-- Done Button.. Generate Report ------------------------------------------ | |
def OnButton2Button(self, event): | |
app.ExitMainLoop() | |
#=== NON GUI FUNCTIONS ========================================================= | |
def GetReportNames(path): | |
stamp='{:%m%d%y}'.format(date.today()) | |
rptfile1=path +'\\ACCC_PSSE_Report_('+stamp+').txt' | |
rptfile2=path +'\\ACCC_PSSE_Report_(Worst)_('+stamp+').txt' | |
xlsfile=path +'\\ACCC_Report_('+stamp+').xlsx' | |
return rptfile1,rptfile2,xlsfile | |
#------------------------------------------------------------------------------- | |
def GetN0results(numaccfiles,accfilelist): | |
N0results=[[],[],[],[],[],[],[],[]] | |
for i in range(numaccfiles): | |
data = pssarrays.accc_solution(accfilelist[i], colabel='BASE CASE') | |
N0results[i]=data | |
return N0results | |
#------------------------------------------------------------------------------- | |
def GetUniqueBusList(numaccfiles,accfilelist): | |
accsummary=[[],[],[],[],[],[],[],[]] | |
buslist=() | |
for i in range(numaccfiles): | |
data = pssarrays.accc_summary(accfilelist[i]) | |
accsummary[i]= data | |
buslist=buslist+data.mvbuslabel | |
ubuslist=list(set(buslist)) | |
ubuslist.sort() | |
return ubuslist,accsummary | |
#------------------------------------------------------------------------------- | |
def GetUniqueBranchList(numaccfiles,accsummary): | |
branchlist=() | |
for i in range(numaccfiles): | |
data = accsummary[i] | |
branchlist=branchlist+data.melement | |
ubranchlist=list(set(branchlist)) | |
ubranchlist.sort() | |
return ubranchlist | |
#------------------------------------------------------------------------------- | |
def BuildMasterConlist(numaccfiles,accfilelist,accsummary): | |
conlist=[] | |
masterconlist=[] | |
desc=[[],[],[],[],[],[],[],[]] | |
for j in range(numaccfiles): | |
for outage in accsummary[j].colabel[1:]: | |
data = pssarrays.accc_solution(accfilelist[j], colabel=outage) | |
description=(outage,data.codesc) | |
desc[j].append(description) | |
masterconlist.append(description) | |
umasterconlist=list(set(masterconlist)) | |
umasterconlist.sort() | |
return desc,umasterconlist | |
#------------------------------------------------------------------------------- | |
def BuildDuplicateConList(masterconlist): | |
pmasterconlist=[] | |
for u in range(len(masterconlist)): | |
temp=[masterconlist[u][0],masterconlist[u][1]] | |
pmasterconlist.append(temp) | |
for x in range(len(pmasterconlist)-1): | |
for y in range(len(pmasterconlist)-1): | |
if pmasterconlist[y][0]== pmasterconlist[y+1][0]: | |
pmasterconlist[y][0]='@'+pmasterconlist[y+1][0] | |
return pmasterconlist | |
#------------------------------------------------------------------------------- | |
def GetN1results(numaccfiles,accfilelist,outage,desc): | |
conexist=[[],[],[],[],[],[],[],[]] | |
converged=[[],[],[],[],[],[],[],[]] | |
con=[[],[],[],[],[],[],[],[]] | |
N1results=[[],[],[],[],[],[],[],[]] | |
for i in range(numaccfiles): | |
conexist[i]=False | |
converged[i]='NA' | |
for j in desc[i]: | |
if outage[1]==j[1]: | |
con[i]=j | |
conexist[i]=True | |
for k in range(numaccfiles): | |
if conexist[k]==True: | |
data=pssarrays.accc_solution(accfilelist[k], colabel=con[k][0]) | |
N1results[k]=data | |
converged[k]=data.cnvflag | |
return N1results,conexist,converged | |
#------------------------------------------------------------------------------- | |
def GetWorstVLow(vlowviolist,vlowconlist): | |
vlowviolist.sort(key=lambda x:x[0]) #Sort the Low Bus Voltage Violation List by Bus Name | |
delviolist=[True for y in range(len(vlowviolist))] #Initialize a Delete Flag List to True (Worst Violations will be changed to False) | |
buscountlist=[1 for z in range(len(vlowviolist))] #Initialize a Violation Counter list to 1 (Will be incremented if more than 1 violation) | |
vlowconlistdict=dict(vlowconlist) #Create a dictionary of contingency violations | |
minvoltage=3.33; buscount=1; minindex=0 #Initialize MinVoltage Value, Low Voltage Violation Counter, Index of Min Bus Voltage | |
for x in range(len(vlowviolist)-1): #Loop through the Indexes of the Bus Violation List (up to next to last) | |
if vlowviolist[x][0] == vlowviolist[x+1][0]: #Determine if this loop's Bus is equal to the next loop's Bus | |
buscount+=1 #If Equal, Increment Violation Counter | |
AList=vlowviolist[x][2:] #Get this loop's Bus Voltage Values | |
BList=vlowviolist[x+1][2:] #Get the next loop's Bus Voltage Values | |
for i in range(len(AList)): #Loop through the indexes of this loop's voltage values | |
try: AList[i]=float(AList[i]) #Convert the Bus Voltage Values to 'float' (if it is 'numerical') | |
except: AList[i]=3.44 #If the Bus Voltage is not numerical, assign it a very high 'non-violation' value | |
for j in range(len(BList)): #Loop through the indexes of the next loop's voltage values | |
try: BList[j]=float(BList[j]) #Convert the Bus Voltage Values to 'float' (if it is 'numerical') | |
except: BList[j]=3.44 #If the Bus Voltage is not numerical, assign it a very high 'non-violation' value | |
a=min(AList) #Get the Min of this loop's Bus Voltage | |
b=min(BList) #Get the Min of the next loop's Bus Voltage | |
if a < minvoltage: #If the Min of this loop's Bus Voltage is < this loop's minvoltage value | |
minvoltage=a #Set the minvoltage value to this loop's Min Bus Voltage | |
minindex=x #Save the index of this loop's Min Bus Voltage | |
if b < minvoltage: #If the Min of the next loop's Bus Voltage is < this loop's minvoltage value | |
minvoltage=b #Set the minvoltage value to the next loop's Min Bus Voltage | |
minindex=x+1 #Save the index of the next loop's Min Bus Voltage | |
else: #Since this loop's Bus is NOT equal to the next loop's Bus (Means we have looped through all buses with equal names) | |
buscountlist[minindex]=buscount #Change the element in this loop's (or next loop's) Bus Counter List to the Violation Counter | |
delviolist[minindex]=False #Change the element in this loop's (or next loop's) Delete Flag List to False | |
minvoltage=3.33 #Reset the Min Voltage Value to a large 'non-violation' value | |
buscount=1 #Reset the Bus Violation Counter | |
minindex=x+1 #Reset the Bus Min Violation index to the next loop | |
buscountlist[minindex]=buscount #If last two Buses are equal, assign bus counter from equal logic. If last two Buses are NOT equal, assign bus counter from NOT equal logic. | |
delviolist[minindex]=False #If last two Buses are equal, Change Delete Flag List from equal logic. If last two Buses are NOT equal, Change Delete Flag List from NOT equal logic. | |
worstviolist=[] #Create an Empty List to hold the 'Worst' Bus/Contingency/LowVoltage Data | |
worstcountlist=[] #Create an Empty List to hold how many Contingencies Caused Low Voltage Violations | |
for x in range(len(vlowviolist)): #Loop Through the 'Total' Bus Low Voltage Violation List | |
if delviolist[x]==False: #Check if this Bus/Contingency is NOT 'Flagged' to be Deleted (Worst Contingency) | |
worstviolist.append(list(vlowviolist[x])) #If Not ... Add it to the Worst Bus Low Voltage Violation List | |
worstcountlist.append(int(buscountlist[x])) #Get how many other Contingencies Caused Low Voltage Violations for this Bus | |
for y in range(len(worstviolist)): #Loop Through 'Identified' Worst Bus Low Voltage Violation List | |
if worstviolist[y][0].startswith('*'): #Check if the worst violation had a basecase violation | |
worstviolist[y][0]=worstviolist[y][0].lstrip('*') #Remove the leading character (will add it back) | |
worstviolist[y][0]='*('+str(worstcountlist[y])+') '+worstviolist[y][0] #Add the Number of Contingencies that Caused Low Voltage Violations to the Begining of the Bus Description | |
else: #If it did not have basecase violation... | |
worstviolist[y][0]='('+str(worstcountlist[y])+') '+worstviolist[y][0] #Add the Number of Contingencies that Caused Low Voltage Violations to the Begining of the Bus Description | |
worstconlist=[] #Create an Empty List to hold the Worst Contingency Names and Descriptions | |
for z in worstviolist: #Finally...Loop through 'Identified' Worst Bus Low Voltage Violation List Again | |
conlabel=z[1] #Get the Continency Name or 'Label' | |
condesc=vlowconlistdict[conlabel] #Get the Contingency Description using the Low Voltage Contingency List Dictionary | |
condata=(conlabel,condesc) #Create a temporary list for this Contingency Label and Description | |
worstconlist.append(condata) #Add this 'Worst' Contingency to the 'worstcon' List | |
return worstviolist,worstconlist #Return Worst Results (Bus/WorstOutage/VoltageData and Contingency Names/Descriptions | |
#------------------------------------------------------------------------------- | |
def GetWorstVHigh(vhighviolist,vhighconlist): | |
vhighviolist.sort(key=lambda x:x[0]) #Sort the High Bus Voltage Violation List by Bus Name | |
delviolist=[True for y in range(len(vhighviolist))] #Initialize a Delete Flag List to True (Worst Violations will be changed to False) | |
buscountlist=[1 for z in range(len(vhighviolist))] #Initialize a Violation Counter list to 1 (Will be incremented if more than 1 violation) | |
vhighconlistdict=dict(vhighconlist) #Create a dictionary of contingency violations | |
maxvoltage=0.20; buscount=1; maxindex=0 #Initialize MaxVoltage Value, High Voltage Violation Counter, Index of Max Bus Voltage | |
for x in range(len(vhighviolist)-1): #Loop through the Indexes of the Bus Violation List (up to next to last) | |
if vhighviolist[x][0] == vhighviolist[x+1][0]: #Determine if this loop's Bus is equal to the next loop's Bus | |
buscount+=1 #If Equal, Increment Violation Counter | |
AList=vhighviolist[x][2:] #Get this loop's Bus Voltage Values | |
BList=vhighviolist[x+1][2:] #Get the next loop's Bus Voltage Values | |
for i in range(len(AList)): #Loop through the indexes of this loop's voltage values | |
try: AList[i]=float(AList[i]) #Convert the Bus Voltage Values to 'float' (if it is 'numerical') | |
except: AList[i]=0.10 #If the Bus Voltage is not numerical, assign it a very low 'non-violation' value | |
for j in range(len(BList)): #Loop through the indexes of the next loop's voltage values | |
try: BList[j]=float(BList[j]) #Convert the Bus Voltage Values to 'float' (if it is 'numerical') | |
except: BList[j]=0.10 #If the Bus Voltage is not numerical, assign it a very low 'non-violation' value | |
a=max(AList) #Get the Max of this loop's Bus Voltage | |
b=max(BList) #Get the Max of the next loop's Bus Voltage | |
if a > maxvoltage: #If the Max of this loop's Bus Voltage is > this loop's minvoltage value | |
maxvoltage=a #Set the maxvoltage value to this loop's Max Bus Voltage | |
maxindex=x #Save the index of this loop's Max Bus Voltage | |
if b > maxvoltage: #If the Max of the next loop's Bus Voltage is > this loop's maxvoltage value | |
maxvoltage=b #Set the maxvoltage value to the next loop's Max Bus Voltage | |
maxindex=x+1 #Save the index of the next loop's Max Bus Voltage | |
else: #Since this loop's Bus is NOT equal to the next loop's Bus (Means we have looped through all buses with equal names) | |
buscountlist[maxindex]=buscount #Change the element in this loop's (or next loop's) Bus Counter List to the Violation Counter | |
delviolist[maxindex]=False #Change the element in this loop's (or next loop's) Delete Flag List to False | |
maxvoltage=0.20 #Reset the Max Voltage Value to a low 'non-violation' value | |
buscount=1 #Reset the Bus Violation Counter | |
maxindex=x+1 #Reset the Bus Max Violation index to the next loop | |
buscountlist[maxindex]=buscount #If last two Buses are equal, assign bus counter from equal logic. If last two Buses are NOT equal, assign bus counter from NOT equal logic. | |
delviolist[maxindex]=False #If last two Buses are equal, Change Delete Flag List from equal logic. If last two Buses are NOT equal, Change Delete Flag List from NOT equal logic. | |
worstviolist=[] #Create an Empty List to hold the 'Worst' Bus/Contingency/High Voltage Data | |
worstcountlist=[] #Create an Empty List to hold how many Contingencies Caused High Voltge Violations | |
for x in range(len(vhighviolist)): #Loop Through the 'Total' Bus Violation List | |
if delviolist[x]==False: #Check if this Bus/Contingency is NOT 'Flagged' to be Deleted (Worst Contingency) | |
worstviolist.append(list(vhighviolist[x])) #If Not ... Add it to the Worst Bus High Voltage Violation List | |
worstcountlist.append(int(buscountlist[x])) #Get how many other Contingencies Caused High Violations for this Bus | |
for y in range(len(worstviolist)): #Loop Through 'Identified' Worst Bus High Voltage Violation List | |
if worstviolist[y][0].startswith('*'): #Check if the worst violation had a basecase violation | |
worstviolist[y][0]=worstviolist[y][0].lstrip('*') #Remove the leading character (will add it back) | |
worstviolist[y][0]='*('+str(worstcountlist[y])+') '+worstviolist[y][0] #Add the Number of Contingencies that Caused Low Voltage Violations to the Begining of the Bus Description | |
else: #If it did not have basecase violation... | |
worstviolist[y][0]='('+str(worstcountlist[y])+') '+worstviolist[y][0] #Add the Number of Contingencies that Caused Low Voltage Violations to the Begining of the Bus Description | |
worstconlist=[] #Create an Empty List to hold the Worst Contingency Names and Descriptions | |
for z in worstviolist: #Finally...Loop through 'Identified' Worst Bus High Voltage List Again | |
conlabel=z[1] #Get the Continency Name or 'Label' | |
condesc=vhighconlistdict[conlabel] #Get the Contingency Description using the Voltage Contingency List Dictionary | |
condata=(conlabel,condesc) #Create a temporary list for this Contingency Label and Description | |
worstconlist.append(condata) #Add this 'Worst' Contingency to the 'worstcon' List | |
return worstviolist,worstconlist #Return Worst Results (Bus/WorstOutage/HighVoltageData and Contingency Names/Descriptions | |
#------------------------------------------------------------------------------- | |
def GetWorstI(iviolist,iconlist): | |
iviolist.sort(key=lambda x:x[0]) #Sort the Branch Violation List by Branch Name | |
deliviolist=[True for y in range(len(iviolist))] #Initialize a Delete Flag List to True (Worst Violations will be changed to False) | |
branchcountlist=[1 for z in range(len(iviolist))] #Initialize a Violation Counter list to 1 (Will be incremented if more than 1 violation) | |
iconlistdict=dict(iconlist) #Create a dictionary of contingency violations | |
maxloading=0.15; branchcount=1; maxindex=0 #Initialize MaxLoading Value, Branch Violation Counter, Index of Max Branch flow | |
for x in range(len(iviolist)-1): #Loop through the Indexes of the Branch Violation List (up to next to last) | |
if iviolist[x][0]==iviolist[x+1][0]: #Determine if this loop's Branch is equal to the next loop's Branch | |
branchcount+=1 #If Equal, Increment Violation Counter | |
AList=iviolist[x][3:] #Get this loop's Branch Flow Values | |
BList=iviolist[x+1][3:] #Get the next loop's Branch Flow Values | |
for i in range(len(AList)): #Loop through the indexes of this loop's flow values | |
try: AList[i]=float(AList[i]) #Convert the Branch Flow Values to 'float' (if it is 'numerical') | |
except: AList[i]=0.10 #If the Branch flow is not numerical, assign it a very low 'non-violation' value | |
for j in range(len(BList)): #Loop through the indexes of the next loop's flow values | |
try: BList[j]=float(BList[j]) #Convert the Branch Flow Values to 'float' (if it is 'numerical') | |
except: BList[j]=0.10 #If the Branch flow is not numerical, assign it a very low 'non-violation' value | |
a=max(AList) #Get the Max of this loop's branch flow | |
b=max(BList) #Get the Max of the next loop's branch flow | |
if a > maxloading: #If the Max of this loop's branch flow is > this loop's maxloading value | |
maxloading=a #Set the maxloading value to this loop's Max branch flow | |
maxindex=x #Save the index of this loop's Max branch flow | |
if b > maxloading: #If the Max of the next loop's branch flow is > this loop's maxloading value | |
maxloading=b #Set the maxloading value to the next loop's Max branch flow | |
maxindex=x+1 #Save the index of the next loop's Max branch flow | |
else: #Since this loop's Branch is NOT equal to the next loop's Branch (Means we have looped through all branches with equal names) | |
branchcountlist[maxindex]=branchcount #Change the element in this loop's (or next loop's) Branch Counter List to the Violation Counter | |
deliviolist[maxindex]=False #Change the element in this loop's (or next loop's) Delete Flag List to False | |
maxloading=0.15 #Reset the Max Loading Value to a small 'non-violation' value | |
branchcount=1 #Reset the Branch Violation Counter | |
maxindex=x+1 #Reset the Branch Max Violation index to the next loop | |
branchcountlist[maxindex]=branchcount #If last two branches are equal, assign branch counter from equal logic. If last two branches are NOT equal, assign branch counter from NOT equal logic. | |
deliviolist[maxindex]=False #If last two branches are equal, Change Delete Flag List from equal logic. If last two branches are NOT equal, Change Delete Flag List from NOT equal logic. | |
worstiviolist=[] #Create an Empty List to hold the 'Worst' Branch/Contingency/Flow Data | |
worstcountlist=[] #Create an Empty List to hold how many Contingencies Caused Violations | |
for x in range(len(iviolist)): #Loop Through the 'Total' Branch Violation List | |
if deliviolist[x]==False: #Check if this Branch/Contingency is not Flagged to be Deleted (Worst Contingency) | |
worstiviolist.append(list(iviolist[x])) #If Not ... Add it to the Worst Branch Flow Violation List | |
worstcountlist.append(int(branchcountlist[x])) #Get how many other Contingencies Caused Violations for this Branch | |
for y in range(len(worstiviolist)): #Loop Through 'Identified' Worst Branch Flow Violation List | |
if worstiviolist[y][0].startswith('*'): #Check if the worst violation had a basecase violation | |
worstiviolist[y][0]=worstiviolist[y][0].lstrip('*') #Remove the leading character (will add it back) | |
worstiviolist[y][0]='*('+str(worstcountlist[y])+') '+worstiviolist[y][0] #Add the Number of Contingencies that Caused High Flow Violations to the Begining of the Bus Description | |
else: #If it did not have basecase violation... | |
worstiviolist[y][0]='('+str(worstcountlist[y])+') '+worstiviolist[y][0] #Add the Number of Contingencies that Caused High Flow Violations to the Begining of the Bus Description | |
worstconlist=[] #Create an Empty List to hold the Worst Contingency Names and Descriptions | |
for z in worstiviolist: #Finally...Loop through 'Identified' Worst Branch Flow List Again | |
conlabel=z[1] #Get the Continency Name or 'Label' | |
condesc=iconlistdict[conlabel] #Get the Contingency Description using the Flow Contingency List Dictionary | |
condata=(conlabel,condesc) #Create a temporary list for this Contingency Label and Description | |
worstconlist.append(condata) #Add this 'Worst' Contingency to the 'worstcon' List | |
return worstiviolist,worstconlist #Return Worst Results (Branch/WorstOutage/FlowData and Contingency Names/Descriptions | |
#------------------------------------------------------------------------------- | |
#----- MAIN ---------- MAIN ---------- MAIN ---------- MAIN ---------- MAIN ---- | |
#------------------------------------------------------------------------------- | |
if __name__ == '__main__': | |
import os, sys | |
#-------- SET EVIRONMENT VARIABLES to Access PSSE Modules ------------------ | |
_PSSBINPATH = r"C:\Program Files\PTI\PSSE33\PSSBIN" | |
if os.path.exists(r"C:\Program Files (x86)\PTI\PSSE33\PSSBIN"): | |
_PSSBINPATH = r"C:\Program Files (x86)\PTI\PSSE33\PSSBIN" | |
os.environ['PATH'] = _PSSBINPATH + ';' + os.environ['PATH'] | |
sys.path.insert(0,_PSSBINPATH) | |
import pssarrays, psspy | |
#Create GUI ---------------------------------------------------------------- | |
app = wx.PySimpleApp() | |
frame = create(None) | |
frame.Show() | |
#Initialize ACCC Options --------------------------------------------------- | |
frame.VLow=float(DefaultVLow) | |
frame.GetHighV=DefaultGetHighV | |
frame.VHigh=float(DefaultVHigh) | |
frame.IHigh=float(DefaultIHigh) | |
frame.numaccfiles=1 | |
frame.accfilelist=None | |
frame.GetWorst=DefaultGetWorst | |
#Go To GUI ----------------------------------------------------------------- | |
app.MainLoop() | |
#Assign ACCC Options Selections -------------------------------------------- | |
VLow=frame.VLow | |
GetHighV=frame.GetHighV | |
VHigh=frame.VHigh | |
IHigh=frame.IHigh | |
numaccfiles=frame.numaccfiles | |
accfilelist=frame.accfilelist | |
GetWorst=frame.GetWorst | |
#Close GUI ----------------------------------------------------------------- | |
frame.Show(0) | |
frame.Close() | |
app.Destroy() | |
if accfilelist==None: | |
print '\nNO SAVE FILES SELECTED!!', | |
raise Exception('NO SAVE FILES SELECTED!!') | |
#--------------------------------------------------------------------------- | |
accpath=os.getcwd() #Get the Path of Working Directory | |
rptfile1,rptfile2,xlsfile=GetReportNames(accpath) #Get the Names of Text and Excel Report Files | |
try: os.remove(xlsfile) #Delete EXCEL file with same name if it exists | |
except: pass | |
NoBus=9.999 #Temp Value if Bus is not found in Monitor List | |
NoVCon=8.888 #Temp Value if Contingency is not found in .acc File | |
NoBusNoVCon=7.777 #Temp Value Bus and Contingency is not found | |
NoVSol=6.666 #Temp Value if Contingency did not reach Convergence | |
NoBranch=999.9 #Temp Value if Branch is not found in Monitor List | |
NoICon=888.8 #Temp Value if No Flow Contingency | |
NoBranchNoICon=777.7 #Temp Value if Branch and Contingency is not found | |
NoISol=666.6 #Temp Value if Contingency did not reach Convergence | |
#=========================================================================== | |
#===== GET N-0 RESULTS ===================================================== | |
#=========================================================================== | |
print 'GETTING N-0 RESULTS .......................................' | |
N0results= GetN0results(numaccfiles,accfilelist) #Get N-0 Results (Also used for Flow) | |
#START N-0 VOLTAGE ANALYSIS ----------------------------------------------- | |
print 'COMPILING BUS LIST ........................................' | |
buslist,accsummary = GetUniqueBusList(numaccfiles,accfilelist) #Compile Unique Monitored Bus List | |
baselowviolist=[] #Initialize the N-0 Bus Low Voltage Violation List | |
basehighviolist=[] #Initialize the N-0 Bus High Voltage Violation List | |
print 'PROCESSING N-0 VOLTAGE ....................................' | |
for BUS in buslist: #Loop Through buses in the 'Total' Monitored Bus List | |
v = [0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0] #Initialize This 'BUS' Voltage Across Cases for this Loop | |
for i in range(numaccfiles): #Loop Across Cases | |
try: #Determine if this 'BUS' Exists in the Monitored List | |
busindex=accsummary[i].mvbuslabel.index(BUS) #Get this 'BUS' index from the Summary (if it exists) | |
v[i]=N0results[i].volts[busindex] #If the Bus is in the Monitored List... Get it's Voltage | |
except: v[i]=NoBus #If the Bus is not in the Monitored List... Assign it the 'NoBus' Value | |
lowviolation = False #Initialize Low Voltage Violation Flag | |
highviolation = False #Initialize High Voltage Violation Flag | |
for j in range(numaccfiles): #Loop Across Cases | |
if v[j] > 0.0 and v[j] < BaseVLow: lowviolation = True #Determine if this 'BUS' has a Low Voltage Violation, Set Flag | |
if v[j] > BaseVHigh and v[j] < NoVSol: highviolation = True #Determine if this 'BUS' has a High Voltage Violation, Set Flag | |
if lowviolation == True: #if this 'BUS' was Found in Low Voltage Violation | |
for k in range(numaccfiles): #Loop Across Cases | |
if v[k]==NoBus: v[k]='NB' #If this 'BUS' does not exist in the Monitored List for this Case, Assign a String to it's value | |
else: v[k]='{0:>5.3f}'.format(v[k]) #Otherwise... Get the 'BUS' Voltage | |
for x in range(numaccfiles,8): v[x]='- ' #If Less than Eight Cases... Set the 'BUS' voltage to a 'Null' String | |
L=[BUS,v[0],v[1],v[2],v[3],v[4],v[5],v[6],v[7]] #Create a List of Results | |
baselowviolist.append(L) #Add Results of this Loop to a Compiled N-0 Low Voltage Violation List | |
if highviolation == True: #if this 'BUS' was Found in High Voltage Violation | |
for m in range(numaccfiles): #Loop Across Cases | |
if v[m]==NoBus: v[m]='NB' #If this 'BUS' does not exist in the Monitored List for this Case, Assign a String to it's value | |
else: v[m]='{0:>5.3f}'.format(v[m]) #Otherwise... Get the 'BUS' Voltage | |
for x in range(numaccfiles,8): v[x]='- ' #If Less than Eight Cases... Set the 'BUS' voltage to a 'Null' String | |
M=[BUS,v[0],v[1],v[2],v[3],v[4],v[5],v[6],v[7]] #Create a List of Results | |
basehighviolist.append(M) #Add Results of this Loop to a Compiled N-0 High Voltage Violation List | |
baselowviolist.sort() #Sort the N-0 Bus Low Voltage Violation List | |
basehighviolist.sort() #Sort the N-0 Bus High Voltage Violation List | |
#START N-0 BRANCH FLOW ANALYSIS -------------------------------------------- | |
print 'COMPILING BRANCH LIST .....................................' | |
branchlist= GetUniqueBranchList(numaccfiles,accsummary) #Compile Unique Monitored Branch List | |
baseiviolist=[] #Initialize the N-0 Violation List | |
print 'PROCESSING N-0 FLOW .......................................' | |
for BRANCH in branchlist: #Loop Through Branches in the 'Total' Monitored Branch List | |
rating=[0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0] #Initialize the Branch Ratings across Cases | |
pct=[0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0] #Initialize the Percent Flow across Cases | |
for i in range(numaccfiles): #Loop Across Cases | |
if BRANCH in accsummary[i].melement: #Determine if this 'BRANCH' Exists in the Monitored List | |
branchindex=accsummary[i].melement.index(BRANCH) #Get this 'BRANCH' index from the Summary (if it exists) | |
mvaflow=abs(N0results[i].ampflow[branchindex]) #If this 'BRANCH' is in the Monitored List... Get it's Flow | |
rating[i] = accsummary[i].rating.a[branchindex] #If this 'BRANCH' is in the Monitored List... Get it's Rating A | |
try: pct[i]=mvaflow*100/rating[i] #Check for Division by Zero ... Calculate Percent Flow | |
except: continue #Next Loop if Rating = 0.0 | |
else: pct[i]=NoBranch #If this 'BRANCH' is not in the Monitored List... Assign it the 'NoBranch' Value | |
rate=max(rating) #Get the Max Branch Rating across Cases | |
rate='{0:>5.0f}'.format(rate) #Convert Rating to String and Format to Zero Decimal Places | |
for r in range(numaccfiles-1): #Loop Across Cases | |
if rating[r]!= 0.0 and rating[r+1]!= rating[r]: rate=rate+'~' #Determine if Ratings are Different... If So.. Add a Character to Max Rating | |
violation = False #Initialize the Violation Flag heading into the Next Loop | |
for j in range(numaccfiles): #Loop Across Cases | |
if pct[j] > BaseIHigh and pct[j] < NoISol: violation = True #Determine if this 'BRANCH' is in Violation, Set Flag | |
if violation == True: #if this 'BRANCH' was Found in Violation | |
for k in range(numaccfiles): #Loop Across Cases | |
if pct[k]==NoBranch: pct[k]='NB' #If this 'BRANCH' does not exist in the Monitored List, Assign a String to it's value | |
else: pct[k]='{0:>5.1f}'.format(pct[k]) #Otherwise... Get the 'BRANCH' Percent Flow | |
for x in range(numaccfiles,8): pct[x]='- ' #If Less than Eight Cases... Set the 'BRANCH' Percent Flow to a 'Null' String | |
L=[BRANCH,rate,pct[0],pct[1],pct[2],pct[3],pct[4],pct[5], #Create a List of Results | |
pct[6],pct[7]] | |
baseiviolist.append(L) #Add Results of this Loop to a Compiled N-0 Violation List | |
baseiviolist.sort() #Sort the N-0 Branch Violation List | |
#=========================================================================== | |
#===== GET CONTINGENCY RESULTS ============================================= | |
#=========================================================================== | |
#BUILD MASTER CONTINGENCY LISTS -------------------------------------------- | |
print 'BUILDING MASTER CONTINGENCY LIST ..........................' | |
desc,masterconlist= BuildMasterConlist(numaccfiles,accfilelist,accsummary) #Create a Contingency List for Each Case, and a Total List Across all Cases | |
print 'BUILDING DUPLICATE CONTINGENCY LIST .......................' | |
pmasterconlist=BuildDuplicateConList(masterconlist) #Create "Parallel" List to Distinguish any Duplicates | |
notconverged=[] #Holds all Contingencies that did not Converge | |
#INITIALIZE CONTINGENCY ANALYSIS ------------------------------------------ | |
vnotconverged=[] #Formated and Used to Report Non-Converged Contingencies associated with Voltage | |
inotconverged=[] #Formated and Used to Report Non-Converged Contingencies associated with Flow | |
vlowconlist=[] #Holds Low Voltage Violation Contingency List | |
vlowviolist=[] #Holds Bus Name and Low Voltage Violatons | |
vhighconlist=[] #Holds High Voltage Violation Contingency List | |
vhighviolist=[] #Holds Bus Name and High Voltage Violatons | |
iconlist=[] #Holds Branch Violation Contingency List | |
iviolist=[] #Holds Branch Name and Branch Violatons | |
vlowconlist1=[] #Holds Low Voltage Violation Contingency List for Reporting | |
vlowviolist1=[] #Holds Bus Name and Low Voltage Violatons for Reporting | |
vhighconlist1=[] #Holds High Voltage Violation Contingency List for Reporting | |
vhighviolist1=[] #Holds Bus Name and High Voltage Violatons for Reporting | |
iconlist1=[] #Holds Branch Violation Contingency List for Reporting | |
iviolist1=[] #Holds Branch Name and Branche Violatons for Reporting | |
vlowconlist2=[] #Holds Worst Low Voltage Violation Contingency List for Reporting | |
vlowviolist2=[] #Holds Worst Bus Name and Low Voltage Violatons for Reporting | |
vhighconlist2=[] #Holds Worst High Voltage Violation Contingency List for Reporting | |
vhighviolist2=[] #Holds Worst Bus Name and High Voltage Violatons for Reporting | |
iconlist2=[] #Holds Worst Branch Violation Contingency List for Reporting | |
iviolist2=[] #Holds Worst Branch Name and Branche Violatons for Reporting | |
#GET DATA FOR REPORTING PROGRESS ------------------------------------------ | |
loops=len(masterconlist) #Get how many Contingencies will be processed | |
loopcount=0.0 #Initialize a Loop Counter | |
modpath,modfname=os.path.split(sys.argv[0]) | |
modname,modext=os.path.splitext(modfname) #Get Either .py or .pyc Extension of Module | |
#START CONTINGENCY ANALYSIS ------------------------------------------------ | |
print 'PROCESSING OUTAGES ........................................' | |
for outage in masterconlist: #Loop Through the Master Contingency List | |
#PRINT PROGRESS (If in Command Console) -------------------------------- | |
if modext=='.pyc': | |
loopcount+=1 #Increment the Loop Counter | |
print( '{0:<5.1f}% OUTAGE: ---> {1:<50s}'+'\r'*5).format(loopcount*100/loops,outage[0]), #Print Progress | |
#GET CONTINGENCY RESULTS ---------------------------------------------- | |
N1results,conexist,converged= GetN1results(numaccfiles,accfilelist,outage,desc) #Get the Results for this Contingency (Also used for Flow) | |
conindex=masterconlist.index(outage) #Get the Index of this Contingency from the Master List (Used to Point to the Parallel List) | |
outagelabel=pmasterconlist[conindex][0] #Get the "Label" of this Outage from the Parallel Contingency List | |
con=(outagelabel,outage[1]) #Use the Parallel List Label and Master List Description to Identify this Outage | |
#----------------------------------------------------------------------- | |
#------------- START CONTINGENCY BUS VOLTAGE ANALYSIS ------------------ | |
#----------------------------------------------------------------------- | |
for BUS in buslist: #Loop Through the Total BUS List | |
v = [0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0] #Initialize This Contingency's BUS Voltages | |
for i in range(numaccfiles): #Loop Across Cases | |
if conexist[i]==True: #Check if this Contingency Exists in this Case | |
if BUS in accsummary[i].mvbuslabel: #Check if this Bus is in the Monitor List | |
busindex=accsummary[i].mvbuslabel.index(BUS) #Get this BUS's index from the Summary (if it exists) | |
v[i]=N1results[i].volts[busindex] #If the BUS is in the Monitored List... Get it's Voltage | |
if converged[i]==False: v[i]=NoVSol #If the Contingency did not Converge in this Case... Assign it the 'NoSol' Value | |
else: v[i]=NoBus #If the Bus is not in the Monitored List... Assign it the 'NoBus' Value | |
if conexist[i]==False: #If this Loop's Contingency does not exist for this BUS | |
if BUS in accsummary[i].mvbuslabel: v[i]=NoVCon #Check if this BUS Exists in this Case.. If so, Assign the NoContingency Value | |
else: v[i]=NoBusNoVCon #Determine if this BUS does not Exist in this Case, If not, Assign the NoBus-NoContingency Value | |
lowviolation = False #Initialize Low Voltage Violation Flag | |
for i in range(numaccfiles): #Loop Across Cases | |
if v[i] > 0.0 and v[i] < VLow: lowviolation = True #Determine if this 'BUS' has a Low Voltage Violation, Set Flag | |
if lowviolation==True: #If one of the Cases had a Low BUS Voltage violation .... Do This Next Stuff | |
baselowvio=False #Initialize a 'Basecase Violaton' Flag (Used for considering basecase violations) | |
for j in baselowviolist: #Loop through the N-0 violation list | |
if BUS==j[0]: #Check if this Bus was in the N-0 violation list | |
baselowpu=[float(x) for x in j[1:1+numaccfiles]] #Create a list of the N-0 Bus PU Voltages | |
if min(baselowpu)< BaseVLowCriteria: baselowvio=True #If the Min of the PU Volages is < N-0 Low Voltage Criteria, Set basecase violation flag | |
for k in range(numaccfiles): #Loop Across Cases | |
if v[k]==NoVSol: v[k]='NS' #If Voltage has the "NoSolution" Value... Assign it a String Value | |
elif v[k]==NoBus: v[k]='NB' #If Voltage has the "NoBus" Value... Assign it a String Value | |
elif v[k]==NoVCon: v[k]='NC' #If Voltage has the "NoContingency" Value... Assign it a String Value | |
elif v[k]==NoBusNoVCon: v[k]='NB,NC' #If Voltage has the "NoBus NoContingency" Value... Assign it a String Value | |
else: v[k]='{0:>8.3f}'.format(v[k]) #Format the BUS Voltage to Three Decimal Places | |
vlowconlist.append(con) #Add this Contingency to the Low Voltage Contingency List for Reporting | |
for x in range(numaccfiles,8): v[x]='- ' #If Less than Eight Cases... Set the 'Other BUS' voltages to a 'Null' String for Nicer Output | |
if baselowvio==True: BUS='* '+BUS #If this BUS had a N-0 Violation, #Add a Character to BUS Name to Indicate this Branch had a basecase violation | |
L=[BUS,outagelabel,v[0],v[1],v[2],v[3],v[4],v[5],v[6],v[7]] #Build a Temporary List of this Loop's Bus, Outage Label, and Low Voltage Results | |
vlowviolist.append(L) #Add the Temporary List to the Total Low Voltage violation list | |
if GetHighV==True: #If Get High BUS Voltages is Enabled ... Do This Next Stuff | |
highviolation = False #Initialize High BUS Voltage Violation Flag | |
for i in range(numaccfiles): #Loop Across Cases | |
if v[i] > VHigh and v[i] < NoVSol: highviolation = True #Determine if this 'BUS' has a High Voltage Violation, Set Flag | |
if highviolation==True: #If one of the Cases had a High BUS Voltage violation .... Do This Next Stuff | |
basehighvio=False #Initialize a 'Basecase Violaton' Flag (Used for considering basecase violations) | |
for j in basehighviolist: #Loop through the N-0 violation list | |
if BUS==j[0]: #Check if this Bus was in the N-0 violation list | |
basehighpu=[float(x) for x in j[1:1+numaccfiles]] #Create a list of the N-0 Bus PU Voltages | |
if max(basehighpu)> BaseVHighCriteria: #If the Max of the PU Volages is > N-0 High Voltage Criteria | |
basehighvio=True #Set basecase violation flag | |
for k in range(numaccfiles): #Loop Across Cases | |
if v[k]==NoVSol: v[k]='NS' #If Voltage has the "NoSolution" Value... Assign it a String Value | |
elif v[k]==NoBus: v[k]='NB' #If Voltage has the "NoBus" Value... Assign it a String Value | |
elif v[k]==NoVCon: v[k]='NC' #If Voltage has the "NoContingency" Value... Assign it a String Value | |
elif v[k]==NoBusNoVCon: v[k]='NB,NC' #If Voltage has the "NoBus NoContingency" Value... Assign it a String Value | |
else: v[k]='{0:>8.3f}'.format(v[k]) #Format the BUS Voltage to Three Decimal Places | |
vhighconlist.append(con) #Add this Contingency to the High Voltage Contingency List for Reporting | |
for x in range(numaccfiles,8): v[x]='- ' #If Less than Eight Cases... Set the 'Other BUS' voltages to a 'Null' String for Nicer Output | |
if basehighvio==True: BRANCH='* '+BRANCH #If this Branch had a N-0 Violation, #Add a Character to BRANCH Name to Indicate this Branch had a basecase violation | |
L=[BUS,outagelabel,v[0],v[1],v[2],v[3],v[4],v[5],v[6],v[7]] #Build a Temporary List of this Loop's Bus, Outage Label, and Low Voltage Results | |
vhighviolist.append(L) #Add the Temporary List to the Total High Voltage violation list | |
vlowviolist.sort() #Sort the Contingency Low Voltage Violation List | |
vhighviolist.sort() #Sort the Contingency High Voltage Violation List | |
#----------------------------------------------------------------------- | |
#-------------- START CONTINGENCY BRANCH FLOW ANALYSIS ----------------- | |
#----------------------------------------------------------------------- | |
for BRANCH in branchlist: #Loop Through the Total Branchlist | |
rating=[0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0] #Initialize the Branch Ratings across Cases | |
pct=[0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0] #Initialize the pecent Flow across Cases | |
BCRateflag=False | |
for i in range(numaccfiles): #Loop Across Case Numbers | |
if conexist[i]==True: #Check if the Contingency Exists | |
if BRANCH in accsummary[i].melement: #Determine if this 'BRANCH' Exists in the Monitored List | |
branchindex=accsummary[i].melement.index(BRANCH) #Get this BRANCH's index from the Summary (if it exists) | |
mvaflow=abs(N1results[i].ampflow[branchindex]) #If this BRANCH is in the Monitored List... Get it's Flow for this Outage | |
rateb=accsummary[i].rating.b[branchindex] #Get this BRANCH's B Rating in this case | |
ratec=accsummary[i].rating.c[branchindex] #Get this BRANCH's C Rating in this case | |
if ratec > rateb: BCRateflag=True #If Rate C > Rate B, Set flag | |
rating[i] = rateb #Set this BRANCH's Rating to Rate B | |
if MVARatingCriteria=='C': rating[i] = ratec #If BRANCH Rating Criteria is Set to 'C', Set this BRANCH's Rating to Rate C | |
try: pct[i]=mvaflow*100/rating[i] #Check for Division by Zero, Calculate Percent Flow | |
except: continue #Next Loop if Rating = 0.0 | |
if converged[i]==False: pct[i]=NoISol #If this Contingency did not converge, assign the NoSolution Value | |
else: pct[i]=NoBranch #Since this BRANCH does not Exist in the Monitored List ... Assign it the 'NoBranch' Value | |
if conexist[i]==False: #Check if This Loop's Contingency exists in this Loop's Case | |
if BRANCH in accsummary[i].melement: pct[i]=NoICon #Check if this BRANCH exists in this case... If so, Assign the No Contingency Value | |
else: pct[i]=NoBranchNoICon #Since this BRANCH does not Exist in the Monitored List ... Assign the NoBranch-NoContingency Value | |
rate=max(rating) #Get the Max BRANCH Rating across Cases | |
rate='{0:>.0f}'.format(rate) #Convert BRANCH Rating to String and Format to Zero Decimal Places | |
if BCRateflag==True: rate=chr(149)+rate #If B and C Rating is different in one of the cases, Add Character to 'rate' for reporting | |
for r in range(numaccfiles-1): #Loop Across Case Numbers | |
if rating[r]!= 0.0 and rating[r+1]!= 0.0 and rating[r+1]!= rating[r]: rate=rate+'~' #Determine if BRANCH Ratings are Different... If So.. Add a Character to Max Rating | |
violation = False #Initialize the Violation Flag for the Next Loop | |
for j in range(numaccfiles): #Loop Across Case Numbers ...n, Set Flag | |
if pct[j] >= IHigh and pct[j] < NoISol: violation = True #Determine if this BRANCH is in Violation... If So, Set Flag | |
if violation==True: #If This Branch is in Violation of IHigh ... Do this Stuff | |
skip=False #Initialize a 'Skip Contingency' Flag (Used for considering basecase violations) | |
basevio=False #Initialize a 'Basecase Violaton' Flag (Used for considering basecase violations) | |
skiplist=[False for x in range(numaccfiles)] #Iniialize a skip flag list for the cases | |
for k in baseiviolist: #Loop through the N-0 violation list | |
if BRANCH==k[0]: #Check if this Branch was in the N-0 violation list | |
basepercent=[float(x) for x in k[2:2+numaccfiles]] #Create a list of the N-0 Percent Branch Flows | |
if min(basepercent)> IHighCriteria: basevio=True #If the Max of the Percent Flows > High Flow Criteria, Set basecase violation flag | |
conpercent=[pct[x] for x in range(numaccfiles)] #Create a list of the N-1 Percent Branch Flows | |
for x in range(len(basepercent)): #Loop through the indexes of the N-0 Branch Flow list | |
if conpercent[x]<= basepercent[x]+5.0: #If cases contingency branch flow <= cases N-0 branch flow, | |
skiplist[x]=True #Set skiplist flag for this case | |
if False not in skiplist: skip=True #Check if all the skiplist flags were set True, if so set skip flag | |
if skip==False: #If the 'skip' flag was not changed to True | |
iconlist.append(con) #Add this Contingency to the List for Reporting | |
for m in range(numaccfiles): #Loop Across Cases | |
if pct[m]==NoISol: pct[m]='NS' #If the Percent Flow has a NoSolution Value... Assign it a String Value | |
elif pct[m]==NoBranch: pct[m]='NB' #If the Percent Flow has a NoBranch Value... Assign it a String Value | |
elif pct[m]==NoICon: pct[m]='NC' #If the Percent Flow has a NoContingency Value... Assign it a String Value | |
elif pct[m]==NoBranchNoICon: pct[m]='NB,NC' #If the Percent Flow has a NoBranch-NoSolution Value... Assign it a String Value | |
else: pct[m]='{0:>5.1f}'.format(pct[m]) #Else format the 'Valid' percent Flow value to three decimal places | |
for x in range(numaccfiles,8): pct[x]='- ' #If Less than Eight Cases... Set the Case's Branch' flows to a 'Null' String for Nicer Output | |
if basevio==True: BRANCH='* '+BRANCH #If this Branch had a N-0 Violation, #Add a Character to BRANCH Name to Indicate this Branch had a basecase violation | |
L=[BRANCH,outagelabel,rate,pct[0],pct[1],pct[2],pct[3], #Build a Temp List of the Violation Results of this BRANCH Loop | |
pct[4],pct[5],pct[6],pct[7]] | |
iviolist.append(L) #Add the Temp List to the Master Flow Violation List | |
iviolist.sort() #Sort the Contingency Flow Violation List by Branch ID | |
if False in converged: #Determine if this outage did not converge in any of the cases | |
notconverged.append(con) #Add this Non-Converged contingency to a Master list | |
for x in range(numaccfiles,8): converged[x]='- ' #If Less than Eight Cases... Set the 'Other Branch' flows to a 'Null' String for Nicer Output | |
for y in range(numaccfiles): #Loop Across Case Numbers | |
if converged[y]==False: converged[y]='NS' #If this outage did not converged in this case... Assign a 'No Solution' String Value | |
if converged[y]==True: converged[y]='- ' #If this outage did converge in this case... Assign a 'Null' String Value | |
K=['NON CONVERGED ->',outagelabel,converged[0],converged[1], #Build a Temporary List for the Voltage cases showing Non-Convergence | |
converged[2],converged[3],converged[4],converged[5], | |
converged[6],converged[7]] | |
M=['NON CONVERGED ->',outagelabel,' -',converged[0], #Build a Temporary List for the Flow cases showing Non-Convergence | |
converged[1],converged[2],converged[3],converged[4], | |
converged[5],converged[6],converged[7]] | |
vnotconverged.append(K) #Add the Temporary to a list for appending Voltage Field | |
inotconverged.append(M) #Add the Temporary list to a list for appending Flow Field | |
vlowconlist.append(con) #Add this Non-Converged Contingency to the Low Voltage violation contingency list (remove duplicates later) | |
vhighconlist.append(con) #Add this Non-Converged Contingency to the High Voltage violation contingency list (remove duplicates later) | |
iconlist.append(con) #Add this Non-Converged Contingency to the Flow violation contingency list (remove duplicates later) | |
#Append Non-Converged Contingencies to other Lists ------------------------- | |
vlowviolist1=vlowviolist+vnotconverged #Add the Non-Converged list to the Low Voltage Violations Field | |
vhighviolist1=vhighviolist+vnotconverged #Add the Non-Converged list to the High Voltage Violations Field | |
iviolist1=iviolist+inotconverged #Add the Non-Converged list to the Flow Violations Field | |
vlowconlist1=vlowconlist+notconverged #Add the Non-Converged Contingencies to the Low Voltage Contingency List | |
vhighconlist1=vhighconlist+notconverged #Add the Non-Converged Contingencies to the High Voltage Contingency List | |
iconlist1=iconlist+notconverged #Add the Non-Converged Contingencies to the Flow Contingency List | |
vlowconlist1=list(set(vlowconlist1)) #Remove Duplicates from the Low Voltage Contingency List | |
vhighconlist1=list(set(vhighconlist1)) #Remove Duplicates from the High Voltage Contingency List | |
iconlist1=list(set(iconlist1)) #Remove Duplicates from the Flow Contingency List | |
vlowconlist1.sort() #Sort the Low Voltage Contingency List | |
vhighconlist1.sort() #Sort the High Voltage Contingency List | |
iconlist1.sort() #Sort the Flow Contingency List | |
#If Get Worst Results Enabled ---------------------------------------------- | |
if GetWorst>0: #If GetWorst Flag is set... Determine Worst Outages | |
print '\nGETTING WORST VOLTAGE RESULTS .............................' | |
if vlowviolist!=[]: | |
vlowviolist2,vlowconlist2=GetWorstVLow(vlowviolist,vlowconlist) #Return Worst Low Voltage Violation and Contingency Lists | |
if GetHighV==True and vhighviolist!=[]: | |
vhighviolist2,vhighconlist2=GetWorstVHigh(vhighviolist,vhighconlist)#Return Worst High Voltage Violation and Contingency Lists | |
print 'GETTING WORST FLOW RESULTS ................................' | |
if iviolist!=[]: | |
iviolist2,iconlist2=GetWorstI(iviolist,iconlist) #Return Worst Flow Violation and Contingency Lists | |
vlowviolist2=vlowviolist2+vnotconverged #Add the Non-Converged list to the Worst Low Voltage Violations Field | |
vhighviolist2=vhighviolist2+vnotconverged #Add the Non-Converged list to the Worst High Voltage Violations Field | |
iviolist2=iviolist2+inotconverged #Add the Non-Converged list to the Worst Flow Violations Field | |
vlowconlist2=vlowconlist2+notconverged #Add the Non-Converged Contingencies to the Worst Low Voltage Contingency List | |
vhighconlist2=vhighconlist2+notconverged #Add the Non-Converged Contingencies to the Worst High Voltage Contingency List | |
iconlist2=iconlist2+notconverged #Add the Non-Converged Contingencies to the Worst Flow Contingency List | |
vlowconlist2=list(set(vlowconlist2)) #Remove Duplicates from the Worst Voltage Contingency List | |
vhighconlist2=list(set(vhighconlist2)) #Remove Duplicates from the Worst Voltage Contingency List | |
iconlist2=list(set(iconlist2)) #Remove Duplicates from the Worst Flow Contingency List | |
vlowconlist2.sort() #Sort the Worst Low Voltage Contingency List | |
vhighconlist2.sort() #Sort the Worst High Voltage Contingency List | |
iconlist2.sort() #Sort the Worst Flow Contingency List | |
#Write PSSE Generated Report File(s) (If Enabled) -------------------------- | |
if TextReport==True: | |
#PSSE Text Report Options ---------------------------------------------- | |
a=1 #Column Headings: 1=acc filename, 2=savcase filename | |
b=1 #Base Case Rating: 1=RateA, 2=RateB, 3=RateC | |
c=2 #Contingency Case Rating: 1=RateA, 2=RateB, 3=RateC | |
d=1 #Base Case voltage limit: 1=Normal 2=Emergency | |
e=2 #Contingency Case Voltage Limit: 1=Normal 2=Emergency | |
f=0 #Print monitored elements summary report | |
g=0 #Print missing monitored elements report | |
h=0 #print missing monitored buses report | |
i=2 #print contingency legend: 0=No 1=Complete Legend Only 2=Reduced Legend with each table, 3=Both | |
j=0 #print missing contingencies report | |
k=1 #print non-converged contingencies report | |
l=2 #print loading violations: 0=No, 1=BaseCase and WorstCase, 2=BaseCase and All, 3=BaseCase WorstCase and All | |
m=2 #print voltage violations: 0=No, 1=BaseCase and WorstCase, 2=BaseCase and All, 3=BaseCase WorstCase and All | |
n=0 #Report Interfaces in loading violation reports | |
o=1 #Skip N-0 Flow Violations in Contingency Flow Reporting | |
p=1 #Skip N-0 Voltage Violations in Contingency Voltage Reporting | |
A=0.5 #bus mismatch converged tolerance | |
B=5.0 #system mismatch converged tolerance | |
C=IHigh #percent flow rating for reporting | |
D=IHigh #worst case percent flow rating for reporting | |
E=0.0 #worst case minimum flow change from base case for reporting | |
F=0.0 #worst case minimum percent flow increase from base case for reporting | |
G=0.0 #worst case minimum voltage change from base case for reporting | |
if MVARatingCriteria=='C': c=3 | |
print 'GENERATING PSSE TEXT REPORT(S) ................' | |
import redirect | |
redirect.psse2py() | |
psspy.psseinit(buses=80000) | |
psspy.transformer_percent_units(1) | |
psspy.non_trans_percent_units(1) | |
tempout = sys.stdout #Temp placeholder for sys.stdout | |
sys.stdout = open(rptfile1,'w') #Send Print Statements to ACCC_PSSE_Report.txt File | |
filelist=accfilelist[0:numaccfiles] | |
psspy.accc_multiple_run_report_2([a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p],[A,B,C,D,E,F,G],numaccfiles,filelist) | |
if GetWorst>0: | |
l=1 | |
m=1 | |
reportout = sys.stdout | |
sys.stdout = open(rptfile2,'w') #Send Print Statements to ACCC_PSSE_Report_(Worst).txt File | |
psspy.accc_multiple_run_report_2([a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p],[A,B,C,D,E,F,G],numaccfiles,filelist) | |
sys.stdout = tempout #Direct Output Back to Screen | |
#=========================================================================== | |
#---------------------- WRITE RESULTS TO EXCEL ----------------------------- | |
#=========================================================================== | |
import excelpy | |
import win32com.client | |
Vlowviolist=list([vlowviolist1,vlowviolist2]) | |
Vlowconlist=list([vlowconlist1,vlowconlist2]) | |
Vhighviolist=list([vhighviolist1,vhighviolist2]) | |
Vhighconlist=list([vhighconlist1,vhighconlist2]) | |
Iviolist=list([iviolist1,iviolist2]) | |
Iconlist=list([iconlist1,iconlist2]) | |
looplogic=[range(1),range(1,2),range(2)] | |
worsttext=['ALL','WORST'] | |
vlowsheet=['VLow','VLow (Worst)'] | |
vhighsheet=['VHigh','VHigh (Worst)'] | |
flowsheet=['Flow','Flow (Worst)'] | |
filler=['..',''] | |
#Create Case Headings ------------------------------------------------------ | |
case=[] | |
for i in range(numaccfiles): #Loop through the number of ACCC Files | |
value='Case%s '%str(i) #Column Headers in will be Case0, Case1, etc. | |
case.append(value) #Add to case heading list | |
for j in range(i+1,8): case.append('') #If Number of Cases is <8 ... Create 'Blank' Header | |
#Create Names for Case Legend --------------------------------------------- | |
fname=[] | |
for i in range(0,8): | |
p,e=os.path.splitext(accfilelist[i]) #Get ACCC Filename (without .acc) for The Legend | |
r,f=os.path.split(p) #Get ACCC Filename (without path) for The Legend | |
fname.append(f) #Add ACCC Filename to filename list | |
print '\nCREATING EXCEL REPORT .....................................' | |
for x in looplogic[GetWorst]: | |
#======================================================================= | |
#-------- LOW VOLTAGE -------- LOW VOLTAGE -------- LOW VOLTAGE -------- | |
#======================================================================= | |
if x==0: xlswbk = excelpy.workbook(xlsfile,mode='w', sheet=vlowsheet[x],overwritesheet=True) | |
if x==1: xlswbk = excelpy.workbook(xlsfile,mode='w', sheet=vlowsheet[x],overwritesheet=True) | |
#if x==1 and GetWorst==1: xlswbk = excelpy.workbook(xlsfile,mode='w', sheet=vlowsheet[x],overwritesheet=True) | |
#if x==1 and GetWorst==2: xlswbk.worksheet_add_after(newSheet=vlowsheet[x], oldSheet=flowsheet[0], overwritesheet=True) | |
print 'WRITING {0:} LOW BUS VOLTAGE RESULTS TO EXCEL ............{1:}'.format(worsttext[x],filler[x]) | |
#----------------------------------------------------------------------- | |
#-------- WRITE CONTINGENCY LOW VOLTAGE HEADINGS ----------------------- | |
#----------------------------------------------------------------------- | |
nextrow=1 | |
#Title1 | |
title1= 'LOW BUS VOLTAGE REPORT' | |
xlswbk.set_cell((nextrow,1),title1,fontStyle="Bold",fontSize=12, fontColor="orange", sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1,nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.merge((nextrow,1,nextrow,10), sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,1),'h_center', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
nextrow+=1 | |
#Title2 | |
if x==0: title2= 'Contingency Results' | |
if x==1: title2= 'Worst Contingency Results' | |
xlswbk.set_cell((nextrow,1),title2,fontStyle="Bold",fontSize=10, fontColor="blue", sheet=vlowsheet[x]) | |
xlswbk.merge((nextrow,1,nextrow,10), sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,1),'h_center', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
nextrow+=1 | |
#Title3 | |
VLow1='{0:>5.3f}'.format(VLow) | |
title3= '< '+ VLow1 +' pu' | |
xlswbk.set_cell((nextrow,1),title3,fontStyle="Bold",numberFormat='0.000', fontSize=10, fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.merge((nextrow,1,nextrow,10), sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,1),'h_center', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
nextrow+=1 | |
#Blank Row | |
xlswbk.height((nextrow,1), height=7, sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
nextrow+=1 | |
#Column Headings | |
colheads = ['BUS', 'OUTAGE', 'Case0', 'Case1', 'Case2','Case3','Case4','Case5','Case6','Case7'] | |
xlswbk.set_range(nextrow,1,colheads,fontStyle="Bold",fontSize=8, fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,1,nextrow,10),'h_center', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1,nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin', borderColor='black', sheet=vlowsheet[x]) | |
#Case Legend | |
xlswbk.set_range(nextrow,12,['Case Legend:'],fontSize=8, fontStyle="Bold", fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.align((nextrow+1,12,18,12),'right', sheet=vlowsheet[x]) | |
xlswbk.align((nextrow+1,13,18,13),'left', sheet=vlowsheet[x]) | |
count=1 | |
if fname[0]!='': xlswbk.set_range(nextrow+count,12,['Case0: ',fname[0]],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
if fname[1]!='': xlswbk.set_range(nextrow+count,12,['Case1: ',fname[1]],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
if fname[2]!='': xlswbk.set_range(nextrow+count,12,['Case2: ',fname[2]],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
if fname[3]!='': xlswbk.set_range(nextrow+count,12,['Case3: ',fname[3]],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
if fname[4]!='': xlswbk.set_range(nextrow+count,12,['Case4: ',fname[4]],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
if fname[5]!='': xlswbk.set_range(nextrow+count,12,['Case5: ',fname[5]],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
if fname[6]!='': xlswbk.set_range(nextrow+count,12,['Case6: ',fname[6]],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
if fname[7]!='': xlswbk.set_range(nextrow+count,12,['Case7: ',fname[7]],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
count+=1 | |
xlswbk.set_range(nextrow+count,12,['NB : ','No Bus -or- Not Monitored'],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
xlswbk.set_range(nextrow+count,12,['NC : ','No Contingency'],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
xlswbk.set_range(nextrow+count,12,['NS : ','No Solution'],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
xlswbk.set_range(nextrow+count,12,['* : ','Bus Had Basecase Violation'],fontSize=8, fontColor="black", sheet=vlowsheet[x]);count+=1 | |
xlswbk.set_range(nextrow+count,12,['@ : ','Duplicate Contingency Name'],fontSize=8, fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.font((nextrow+1,12,nextrow+count,12),fontStyle="Bold", sheet=vlowsheet[x]) | |
xlswbk.autofit_columns((12,12), sheet=vlowsheet[x]) #Autofit Column12 | |
xlswbk.autofit_columns((13,13), sheet=vlowsheet[x]) #Autofit Column13 | |
nextrow+=1 | |
#--------------------------------------------------------------------------- | |
#------- WRITE CONTINGENCY LOW VOLTAGE FIELD ------------------------------- | |
#--------------------------------------------------------------------------- | |
col=1 | |
V1firstrow=nextrow | |
V1lastrow=nextrow | |
for r in range(len(Vlowviolist[x])): #Write the Contingency Low Voltage Violations Field | |
rowval = Vlowviolist[x][r] | |
xlswbk.set_range(nextrow,col,rowval,fontSize=8, fontColor="black", sheet=vlowsheet[x]) | |
V1lastrow=nextrow | |
nextrow+=1 | |
xlswbk.autofit_columns((1,2), sheet=vlowsheet[x]) #AutoFit Column2 | |
xlswbk.align((V1firstrow,1,V1lastrow,1),'right', sheet=vlowsheet[x]) #Right Justify Bus Names | |
xlswbk.align((V1firstrow,2,V1lastrow,10),'h_center', sheet=vlowsheet[x]) #Center the Field | |
xlswbk.font((V1firstrow,3,V1lastrow,10),numberFormat='0.000', sheet=vlowsheet[x]) #Format the Low Voltage Field to 3 Decimal Places | |
if Vlowviolist[x]==[]: #Check if there were no Low Voltage Violations | |
xlswbk.set_range(nextrow,col,['NONE'],fontSize=8, fontStyle="Bold", fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,col),'h_center', sheet=vlowsheet[x]) | |
nextrow+=1 | |
nextrow+=1 #Write Contingency Legend Header | |
xlswbk.set_range(nextrow,col,['Contingency Legend:'],fontSize=8, fontStyle="Bold", fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,col),'right', sheet=vlowsheet[x]) | |
nextrow+=1 | |
for r in Vlowconlist[x]: #Write Contingency Legend | |
rowval1 = [r[0],r[1][0]] | |
xlswbk.set_range(nextrow,col,rowval1,fontSize=8, fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,col),'right', sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,col+1),'left', sheet=vlowsheet[x]) | |
nextrow+=1 | |
multicon=len(r[1]) #Check if Multiple Outages per Contingency | |
for j in range(multicon-1): #Enter This Loop if More than One Outage per Contingency | |
nextoutage=['-',r[1][j+1]] | |
xlswbk.set_range(nextrow,col,nextoutage,fontSize=8, fontColor="black", sheet=vlowsheet[x]) #Put it in the nextrow | |
xlswbk.align((nextrow,col),'right', sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,col+1),'left', sheet=vlowsheet[x]) | |
nextrow+=1 | |
#--------------------------------------------------------------------------- | |
#-------- WRITE N-0 LOW VOLTAGE HEADINGS ----------------------------------- | |
#--------------------------------------------------------------------------- | |
nextrow+=3 | |
#Title4 | |
title4= 'N-0 Voltage Results' | |
xlswbk.set_cell((nextrow,1),title4, fontStyle="Bold",fontSize=10, fontColor="blue", sheet=vlowsheet[x]) | |
xlswbk.merge((nextrow,1,nextrow,10), sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,1),'h_center', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
nextrow+=1 | |
#Title5 | |
BaseVLow1='{0:>5.3f}'.format(BaseVLow) | |
title5= '< '+ BaseVLow1 +' pu' | |
xlswbk.set_cell((nextrow,1),title5,fontStyle="Bold", numberFormat='0.000', fontSize=10, fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.merge((nextrow,1,nextrow,10), sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,1),'h_center', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
nextrow+=1 | |
#Blank Row | |
xlswbk.height((nextrow,1), height=7, sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
nextrow+=1 | |
#Column Headings | |
N0row=nextrow | |
colheads = ['BUS', ' ', 'Case0', 'Case1', 'Case2','Case3','Case4','Case5','Case6','Case7'] | |
xlswbk.set_range(nextrow,1,colheads,fontStyle="Bold",fontSize=8, fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,1,nextrow,10),'h_center', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=vlowsheet[x]) | |
xlswbk.border((nextrow,1,nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin', borderColor='black', sheet=vlowsheet[x]) | |
#----------------------------------------------------------------------- | |
#------- WRITE N-0 LOW VOLTAGE FIELD ----------------------------------- | |
#----------------------------------------------------------------------- | |
nextrow+=1 | |
V0firstrow=nextrow | |
V0lastrow=nextrow | |
for r in range(len(baselowviolist)): #Write the N-0 Violations Field | |
rowval = baselowviolist[r] | |
xlswbk.set_range(nextrow,col,[rowval[0]],fontSize=8, fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.set_range(nextrow,col+1,['--'],fontSize=8, fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.set_range(nextrow,col+2,[rowval[1:]],fontSize=8, fontColor="black", sheet=vlowsheet[x]) | |
V0lastrow=nextrow | |
nextrow+=1 | |
xlswbk.align((V0firstrow,1,V0lastrow,1),'right', sheet=vlowsheet[x]) #Right Justify Bus Names | |
xlswbk.align((V0firstrow,2,V0lastrow,10),'h_center', sheet=vlowsheet[x]) #Center the Field | |
xlswbk.font((V0firstrow,2,V0lastrow,10),numberFormat='0.000', sheet=vlowsheet[x]) #Format the Voltage Field to 3 Decimal Places | |
if baselowviolist==[]: | |
xlswbk.set_range(nextrow,col,['NONE'],fontSize=8, fontStyle="Bold", fontColor="black", sheet=vlowsheet[x]) | |
xlswbk.align((nextrow,col),'h_center', sheet=vlowsheet[x]) | |
nextrow+=1 | |
xlswbk.autofit_columns((1,1), sheet=vlowsheet[x]) #AutoFit Column1 | |
xlswbk.width((1,11),3,sheet=flowsheet[x]) #Set Width of Column11 | |
#======================================================================= | |
#-------- BRANCH FLOWS -------- BRANCH FLOWS -------- BRANCH FLOWS ----- | |
#======================================================================= | |
xlswbk.worksheet_add_after(newSheet=flowsheet[x], oldSheet=vlowsheet[x], overwritesheet=True) | |
print 'WRITING {0:} BRANCH FLOW RESULTS TO EXCEL ................{1:}'.format(worsttext[x],filler[x]) | |
#----------------------------------------------------------------------- | |
#-------- WRITE CONTINGENCY FLOWS HEADINGS ----------------------------- | |
#----------------------------------------------------------------------- | |
nextrow=1 | |
#Title1 | |
title1= 'BRANCH LOADING REPORT' | |
xlswbk.set_cell((nextrow,1),title1,fontStyle="Bold",fontSize=12, fontColor="orange", sheet=flowsheet[x]) | |
xlswbk.border((nextrow,1,nextrow,11), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.merge((nextrow,1,nextrow,11), sheet=flowsheet[x]) | |
xlswbk.align((nextrow,1),'h_center', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,5), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,6), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,7), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,8), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,9), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,10), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,11), borderv='left', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,11), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
nextrow+=1 | |
#Title2 | |
if x==0: title2= 'Contingency Results' | |
if x==1: title2= 'Worst Contingency Results' | |
xlswbk.set_cell((nextrow,1),title2,fontStyle="Bold",fontSize=10, fontColor="blue", sheet=flowsheet[x]) | |
xlswbk.merge((nextrow,1,nextrow,11), sheet=flowsheet[x]) | |
xlswbk.align((nextrow,1),'h_center', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='right', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='top', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,1), borderv='bottom', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,2), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,3), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x]) | |
xlswbk.border((nextrow,4), borderv='outline', borderStyle='continuous', borderWeight='thin',borderColor='white', sheet=flowsheet[x] |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Great work! i am testing out the script, the gui opens up and i choose the acc files. The terminal window shows some progress then just hangs indefinitely. Have you experienced this issue?
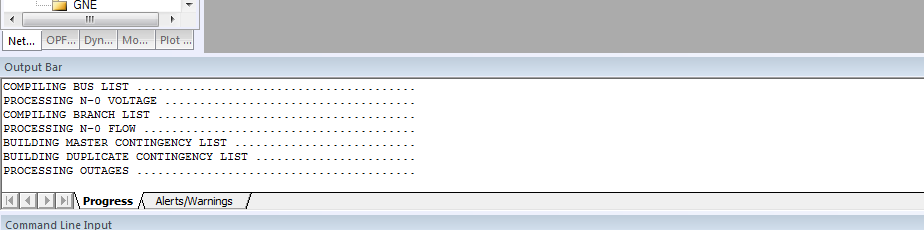