Last active
June 19, 2025 17:53
-
-
Save emmanuelnk/c34a361c79c0f3792de533170cbfcd01 to your computer and use it in GitHub Desktop.
GreaseMonkey script to show an AWS account alias in the top nav bar
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// ==UserScript== | |
// @name DisplayAWSAccountAlias | |
// @version 1 | |
// @grant none | |
// @match https://*.console.aws.amazon.com/* | |
// ==/UserScript== | |
// Replace with your account numbers and desired alias | |
// The key is the account number and the value is the alias to display | |
const accountAliases = { | |
'ACCOUNT_NUMBER': 'ALIAS', | |
'123412341234': '123412341234 - Production', | |
'123312331233': '123312331233 - Development', | |
'400050006000': '400060006000 - Audit', | |
'987698769876': '987698769876 - Sandbox', | |
'452845284528': '452845284528 - Shared' | |
} | |
function scrapeAccountNumber (node) { | |
for(const child of node.childNodes) { | |
const result = scrapeAccountNumber(child) | |
if(result) return result | |
const accountNumber = child.innerHTML?.match(/([0-9]+-[0-9]+-[0-9]+)/g) | |
if(accountNumber && accountNumber.length) return accountNumber[0].replace(/-/g, '') | |
} | |
return | |
} | |
const accountNumber = scrapeAccountNumber(document.querySelector('[data-testid="account-detail-menu"]')) | |
if(accountNumber && accountAliases[accountNumber]) { | |
const styleClasses = document.querySelector('[data-testid="more-menu__awsc-nav-regions-menu-button"]').classList | |
const topBarList = document.getElementById('awsc-navigation__more-menu--list') | |
const newTopBarListItem = topBarList.firstChild.cloneNode() | |
const newTopBarListItemTextDiv = document.createElement('div') | |
newTopBarListItemTextDiv.classList.add(styleClasses[0]) | |
newTopBarListItem.appendChild(document.createTextNode(accountAliases[accountNumber])) | |
newTopBarListItemTextDiv.appendChild(newTopBarListItem) | |
topBarList.prepend(newTopBarListItemTextDiv) | |
} |
Thanks! Works great. I have some adjustments to it since you can get the data from the metadata in the headers of the HTML now. You don't need to write out the list of your accounts manually in an array. Here is how I did it:
function extractAWSData() {
const metaTag = document.querySelector('meta[name="awsc-session-data"]');
if (metaTag) {
const content = metaTag.getAttribute('content');
const accountIdMatch = content.match(/"accountId":"(\d+)"/);
const accountAliasMatch = content.match(/"accountAlias":"([^"]+)"/); // "
if (accountIdMatch && accountAliasMatch) {
console.log('Account ID found:', accountIdMatch[1]);
console.log('Extracted account alias:', accountAliasMatch[1]);
return { accountId: accountIdMatch[1], accountAlias: accountAliasMatch[1] };
} else {
console.log('Data not complete, retrying...');
//setTimeout(initiateAWSDataExtraction, 200);
return null;
}
} else {
console.log('Meta tag not found, retrying...');
setTimeout(extractAWSData, 5000);
return null;
}
}
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
An example:
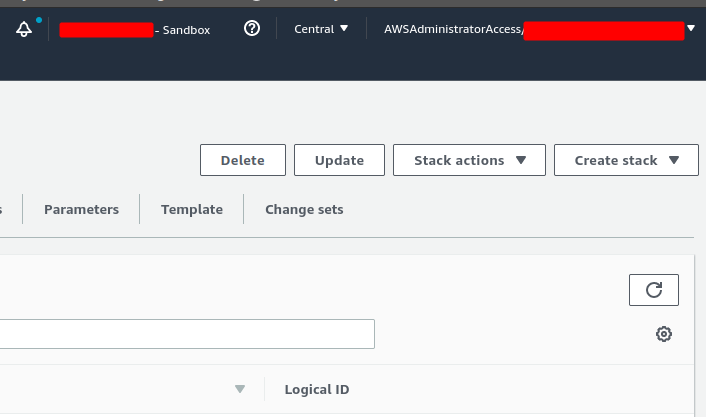