Last active
October 14, 2018 17:11
-
-
Save harawata/29762e8216ff68b089a582b6831595b9 to your computer and use it in GitHub Desktop.
JDBC app that INSERT/SELECT OffsetDateTime using PreparedStatement#setObject() and ResultSet#getObject()
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import java.sql.*; | |
import java.time.*; | |
import java.util.*; | |
public class OffsetDateTimeTest { | |
public static void main(String[] args) throws Exception { | |
try (Connection con = new JdbcConnection(args[0]).getConnection()) { | |
try (Statement stmt = con.createStatement()) { | |
try { | |
stmt.execute("drop table test"); | |
} catch (Exception e) { | |
// expected | |
} | |
stmt.execute("create table test (id integer, t " + args[1] + ")"); | |
} | |
insert(con); | |
select(con, 2); | |
} | |
} | |
private static void select(Connection con, final Integer id) throws SQLException { | |
try (PreparedStatement stmt = con.prepareStatement("select id, t from test where id = ?")) { | |
stmt.setInt(1, id); | |
try (ResultSet rs = stmt.executeQuery()) { | |
while (rs.next()) { | |
System.out.println("id: " + rs.getInt(1)); | |
System.out.println("time: " + rs.getObject(2, OffsetDateTime.class)); | |
} | |
} | |
} | |
} | |
private static void insert(Connection con) throws SQLException { | |
try (PreparedStatement stmt = con.prepareStatement("insert into test (id, t) values (?, ?)")) { | |
OffsetDateTime testValue = OffsetDateTime.of(2018, 10, 8, 11, 22, 33, 123456789, ZoneOffset.ofHoursMinutes(10, 20)); | |
stmt.setInt(1, 2); | |
stmt.setObject(2, testValue); | |
stmt.executeUpdate(); | |
} | |
} | |
} | |
public class JdbcConnection { | |
private final String db; | |
public JdbcConnection(String db) { | |
this.db = db; | |
} | |
public Connection getConnection() throws ClassNotFoundException, SQLException { | |
final ResourceBundle bundle = ResourceBundle.getBundle("dbs/" + db); | |
final String driver = bundle.getString("driver"); | |
final String url = bundle.getString("url"); | |
final String username = bundle.getString("username"); | |
final String password = bundle.getString("password"); | |
Class.forName(driver); | |
Connection con = DriverManager.getConnection(url, username, password); | |
DatabaseMetaData dbmd = con.getMetaData(); | |
System.out.println(">>> DB version : " + dbmd.getDatabaseProductName() + " " + dbmd.getDatabaseProductVersion()); | |
System.out.println(">>> Driver version : " + dbmd.getDriverVersion()); | |
return con; | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Put drivers in 'drivers' directory and JDBC configuration files in 'dbs' direcotry.
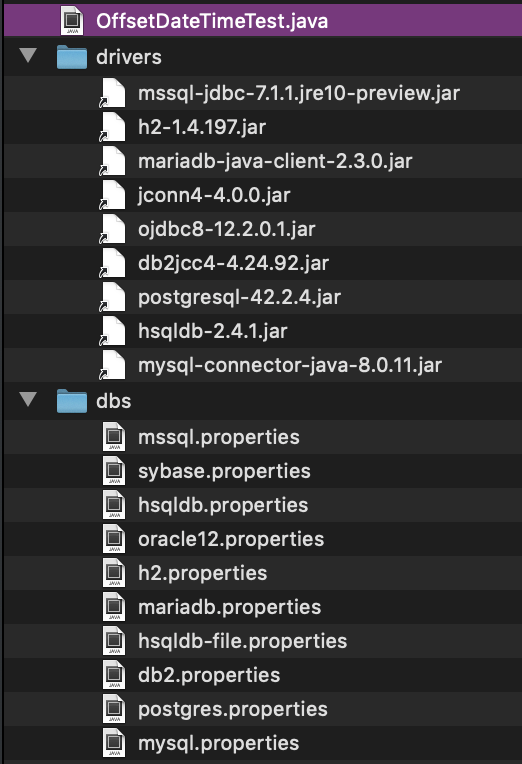
The .properties files should look as follows.With Java 11, you can run it directly as follows.
java --module-path drivers OffsetDateTimeTest.java hsqldb "timestamp with time zone"
The first argument is the file name of a DB config and the second argument is the column type.