Last active
March 12, 2023 12:13
-
-
Save iKlotho/cf43bae544ae78395f736eec9647fff7 to your computer and use it in GitHub Desktop.
NVI Adres Sorgulama Python API (adres.nvi.gov.tr/VatandasIslemleri/AdresSorgu)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import requests | |
import random | |
import json | |
from bs4 import BeautifulSoup | |
captcha = "03AGdBq25SyMYChQqUZMbWMftzdsGPyK9TztL3q95FoSWY-4042gCR56xovRI3i6i2Son38GjbKVf86X4P-L7GQU_jbZtSK4PwA8zZAPWDNU7FQ-RM1fpnMcUpyxtXOk635UOr2YR_P9Jo5o_72N0OqdCUjDJk6ohOUJPLUy4aAZDHd_oEdfRpmVJpkqCqL2aPtx5BFVqvx9QvQ5wIugNyPk4V3mDVTgxV2_0nieKyLTt92fNr34AeEStL16DQrXk02_zMgKaIHbNE4yYRkJ1Ac0m6Qle3PG_L4ochPAswsdLTgmukolRF8HtZmOU3rJ7F3K4HJ7j4bNBqmf9_KQ3_U-HM2T-MVZiHygJDF4EEg_DL3GsSA8ZGUXRQuk09LKTIfUt6AaNO81ARwCGLQFWbn2-po_K_6GKqkMm5ZxFnu0pWcZeXciJL5nBZDaAYf6YypcrNcWXsw_l4" | |
class NVIScraper: | |
""" NVI address query API | |
-usage- | |
>> nvi = NVIScraper() | |
>> data = nvi.bagimsizbolum_list(1685,254329063) | |
>> data2 = nvi.neigborhood_list(1815) | |
>> print(data) | |
""" | |
# will be initalized when homepage crawled | |
token = "" | |
cookies = {} | |
def __init__(self, proxy_list=[{}]): | |
self.homepage_url = "https://adres.nvi.gov.tr/VatandasIslemleri/AdresSorgu" | |
self.ilce_url = "https://adres.nvi.gov.tr/Harita/ilceListesi" | |
self.mahalle_url = "https://adres.nvi.gov.tr/Harita/mahalleKoyBaglisiListesi" | |
self.yol_url = "https://adres.nvi.gov.tr/Harita/yolListesi" | |
self.bina_url = "https://adres.nvi.gov.tr/Harita/binaListesi" | |
self.bagimsizbolum_url = "https://adres.nvi.gov.tr/Harita/bagimsizBolumListesi" | |
self.forbina_url = "https://adres.nvi.gov.tr/Harita/graphicsForbina" | |
self.forneigh_url = ( | |
"https://adres.nvi.gov.tr/Harita/graphicsFormahalleKoyBaglisi" | |
) | |
custom_headers = { | |
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/78.0.3904.97 Safari/537.36", | |
} | |
self.proxy_list = proxy_list | |
self.sess = requests.Session() | |
self.sess.headers.update(custom_headers) | |
self.initialize_token() | |
def initialize_token(self) -> None: | |
""" | |
Initializes a token for future requests | |
""" | |
r = self.sess.get( | |
self.homepage_url, verify=False, proxies=random.choice(self.proxy_list) | |
) | |
soup = BeautifulSoup(r.content, "html.parser") | |
self.token = soup.find("input", {"name": "__RequestVerificationToken"}).get( | |
"value" | |
) | |
print("intiailized token", self.token) | |
self.cookies = self.sess.cookies.get_dict() | |
# prepare headers for post requests | |
self.sess.headers.update( | |
{ | |
"Content-Type": "application/x-www-form-urlencoded", | |
"X-Requested-With": "XMLHttpRequest", | |
"Referer": "https://adres.nvi.gov.tr/VatandasIslemleri/AdresSorgu", | |
"__RequestVerificationToken": self.token, | |
"Host": "adres.nvi.gov.tr", | |
} | |
) | |
def district_list(self, ilKimlikNo: str) -> dict: | |
""" | |
Get district list by city id | |
""" | |
r = self.sess.post( | |
self.ilce_url, | |
data="ilKimlikNo={}&adresReCaptchaResponse={}".format(ilKimlikNo, captcha), | |
verify=False, | |
proxies=random.choice(self.proxy_list), | |
) | |
return self.return_logic(r) | |
def neighborhood_list(self, ilceKimlikNo: str) -> dict: | |
""" | |
Get neigborhood list by district id | |
""" | |
r = self.sess.post( | |
self.mahalle_url, | |
data="ilceKimlikNo={}&adresReCaptchaResponse={}".format( | |
ilceKimlikNo, captcha | |
), | |
verify=False, | |
proxies=random.choice(self.proxy_list), | |
) | |
return self.return_logic(r) | |
def street_list(self, mahalleKoyBaglisiKimlikNo: str) -> dict: | |
""" | |
Get street list | |
""" | |
r = self.sess.post( | |
self.yol_url, | |
data="mahalleKoyBaglisiKimlikNo={}&adresReCaptchaResponse={}".format( | |
mahalleKoyBaglisiKimlikNo, captcha | |
), | |
verify=False, | |
proxies=random.choice(self.proxy_list), | |
) | |
return self.return_logic(r) | |
def building_list(self, mahalleKoyBaglisiKimlikNo: str, yolKimlikNo: str) -> dict: | |
""" | |
Get building list | |
""" | |
r = self.sess.post( | |
self.bina_url, | |
data="mahalleKoyBaglisiKimlikNo={}&yolKimlikNo={}&adresReCaptchaResponse={}".format( | |
mahalleKoyBaglisiKimlikNo, yolKimlikNo, captcha | |
), | |
verify=False, | |
proxies=random.choice(self.proxy_list), | |
) | |
return self.return_logic(r) | |
def bagimsizbolum_list( | |
self, mahalleKoyBaglisiKimlikNo: str, binaKimlikNo: str | |
) -> dict: | |
""" | |
Get bagimsizbolum(?) list | |
""" | |
r = self.sess.post( | |
self.bagimsizbolum_url, | |
data="mahalleKoyBaglisiKimlikNo={}&binaKimlikNo={}&adresReCaptchaResponse={}".format( | |
mahalleKoyBaglisiKimlikNo, binaKimlikNo, captcha | |
), | |
verify=False, | |
proxies=random.choice(self.proxy_list), | |
) | |
return self.return_logic(r) | |
def get_building_geometry( | |
self, mahalleKoyBaglisiKimlikNo: str, binaKimlikNo: str | |
) -> dict: | |
""" | |
Get building geo location | |
""" | |
r = self.sess.post( | |
self.forbina_url, | |
data="mahalleKoyBaglisiKimlikNo={}&binaKimlikNo={}&adresReCaptchaResponse={}".format( | |
mahalleKoyBaglisiKimlikNo, binaKimlikNo, captcha | |
), | |
verify=False, | |
proxies=random.choice(self.proxy_list), | |
) | |
return self.return_logic(r) | |
def get_neighborhood_geometry(self, mahalleKoyBaglisiKimlikNo: str) -> dict: | |
""" | |
Get neigh geo location | |
""" | |
r = self.sess.post( | |
self.forneigh_url, | |
data="mahalleKoyBaglisiKimlikNo={}&adresReCaptchaResponse={}".format( | |
mahalleKoyBaglisiKimlikNo, captcha | |
), | |
verify=False, | |
proxies=random.choice(self.proxy_list), | |
) | |
return self.return_logic(r) | |
def return_logic(self, r: requests.models.Response) -> dict: | |
""" Takes request object try to convert json and | |
check if request successfull | |
""" | |
try: | |
data = r.json() | |
if isinstance(data, dict): | |
if "success" in data.keys() and data.get("success") == False: | |
return data | |
return {"success": True, "data": data} | |
except Exception as e: | |
return {"success": False, "reason": str(e)} | |
if __name__ == "__main__": | |
nvi = NVIScraper() | |
requested_city = { | |
"id": 34, | |
"name": "İstanbul" | |
} | |
data = nvi.district_list(requested_city["id"]) | |
print("d",data) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Merhaba, ekteki hatayı alıyorum: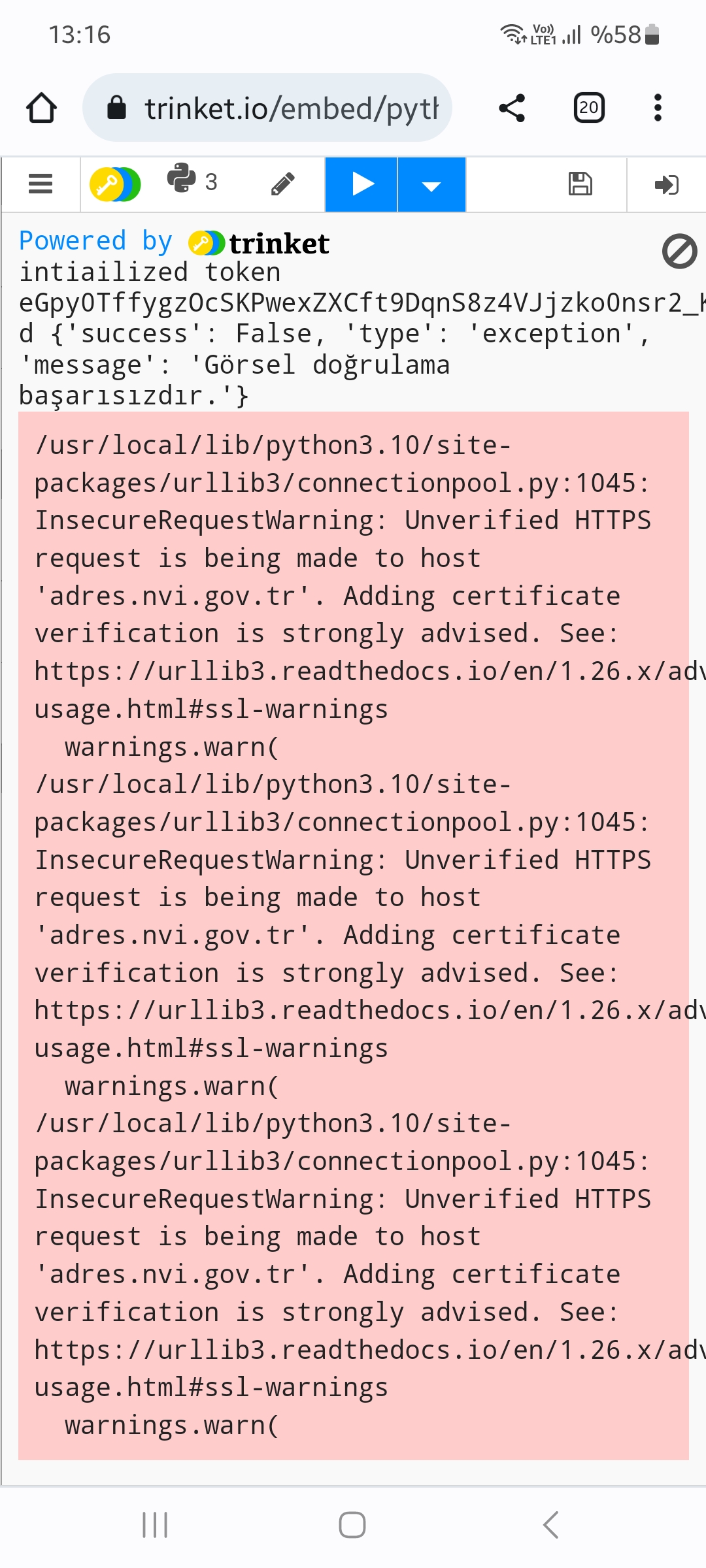