|
import '../flutter_flow/flutter_flow_theme.dart'; |
|
import '../flutter_flow/flutter_flow_util.dart'; |
|
import '../flutter_flow/flutter_flow_widgets.dart'; |
|
import 'package:flutter/material.dart'; |
|
import 'package:google_fonts/google_fonts.dart'; |
|
|
|
class HomePageWidget extends StatefulWidget { |
|
HomePageWidget({Key key}) : super(key: key); |
|
|
|
@override |
|
_HomePageWidgetState createState() => _HomePageWidgetState(); |
|
} |
|
|
|
class _HomePageWidgetState extends State<HomePageWidget> { |
|
final scaffoldKey = GlobalKey<ScaffoldState>(); |
|
|
|
@override |
|
Widget build(BuildContext context) { |
|
return Scaffold( |
|
key: scaffoldKey, |
|
appBar: AppBar( |
|
backgroundColor: Color(0xFF51307B), |
|
automaticallyImplyLeading: false, |
|
title: Text( |
|
'GOD APP', |
|
style: FlutterFlowTheme.title1.override( |
|
fontFamily: 'Poppins', |
|
color: Colors.white, |
|
), |
|
), |
|
actions: [], |
|
centerTitle: true, |
|
elevation: 4, |
|
), |
|
backgroundColor: Color(0xFF372154), |
|
body: SafeArea( |
|
child: Column( |
|
mainAxisSize: MainAxisSize.max, |
|
mainAxisAlignment: MainAxisAlignment.center, |
|
children: [ |
|
Padding( |
|
padding: EdgeInsets.fromLTRB(1, 0, 0, 0), |
|
child: Row( |
|
mainAxisSize: MainAxisSize.max, |
|
mainAxisAlignment: MainAxisAlignment.spaceEvenly, |
|
children: [ |
|
Align( |
|
alignment: Alignment(0, 0), |
|
child: Padding( |
|
padding: EdgeInsets.fromLTRB(10, 10, 10, 10), |
|
child: FFButtonWidget( |
|
onPressed: () async { |
|
await launchURL( |
|
'https://www.youtube.com/watch?v=dH30vEH6YfI'); |
|
}, |
|
text: 'Tracer', |
|
options: FFButtonOptions( |
|
width: 130, |
|
height: 100, |
|
color: Color(0xFFA060EE), |
|
textStyle: FlutterFlowTheme.subtitle2.override( |
|
fontFamily: 'Poppins', |
|
color: Colors.white, |
|
), |
|
borderSide: BorderSide( |
|
color: Colors.transparent, |
|
width: 1, |
|
), |
|
borderRadius: 12, |
|
), |
|
), |
|
), |
|
), |
|
Padding( |
|
padding: EdgeInsets.fromLTRB(10, 10, 10, 10), |
|
child: FFButtonWidget( |
|
onPressed: () async { |
|
await launchURL( |
|
'https://www.youtube.com/watch?v=x0jPbnuZ3Rc&ab_channel=Dozzyable'); |
|
}, |
|
text: 'Rick Astley', |
|
options: FFButtonOptions( |
|
width: 130, |
|
height: 100, |
|
color: Color(0xFFA060EE), |
|
textStyle: FlutterFlowTheme.subtitle2.override( |
|
fontFamily: 'Poppins', |
|
color: Colors.white, |
|
), |
|
borderSide: BorderSide( |
|
color: Colors.transparent, |
|
width: 1, |
|
), |
|
borderRadius: 12, |
|
), |
|
), |
|
) |
|
], |
|
), |
|
), |
|
Padding( |
|
padding: EdgeInsets.fromLTRB(1, 0, 0, 0), |
|
child: Row( |
|
mainAxisSize: MainAxisSize.max, |
|
mainAxisAlignment: MainAxisAlignment.spaceEvenly, |
|
children: [ |
|
Align( |
|
alignment: Alignment(0, 0), |
|
child: Padding( |
|
padding: EdgeInsets.fromLTRB(10, 10, 10, 10), |
|
child: FFButtonWidget( |
|
onPressed: () async { |
|
await launchURL( |
|
'https://www.youtube.com/watch?v=x0jPbnuZ3Rc'); |
|
}, |
|
text: 'boop', |
|
options: FFButtonOptions( |
|
width: 130, |
|
height: 100, |
|
color: Color(0xFFA060EE), |
|
textStyle: FlutterFlowTheme.subtitle2.override( |
|
fontFamily: 'Poppins', |
|
color: Colors.white, |
|
), |
|
borderSide: BorderSide( |
|
color: Colors.transparent, |
|
width: 1, |
|
), |
|
borderRadius: 12, |
|
), |
|
), |
|
), |
|
), |
|
Padding( |
|
padding: EdgeInsets.fromLTRB(10, 10, 10, 10), |
|
child: FFButtonWidget( |
|
onPressed: () { |
|
print('Button pressed ...'); |
|
}, |
|
text: 'y u gae', |
|
options: FFButtonOptions( |
|
width: 130, |
|
height: 100, |
|
color: Color(0xFFA060EE), |
|
textStyle: FlutterFlowTheme.subtitle2.override( |
|
fontFamily: 'Poppins', |
|
color: Colors.white, |
|
), |
|
borderSide: BorderSide( |
|
color: Colors.transparent, |
|
width: 1, |
|
), |
|
borderRadius: 12, |
|
), |
|
), |
|
) |
|
], |
|
), |
|
) |
|
], |
|
), |
|
), |
|
); |
|
} |
|
} |
I got an errors on import , how can I fixed it ?!
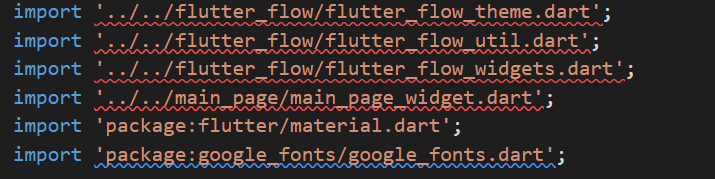