Created
October 21, 2022 16:00
-
-
Save jonathasborges1/6f8e0eb476a08c54f70bc80184b37537 to your computer and use it in GitHub Desktop.
Login Page with Material UI and React JS
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import { | |
Grid, | |
Paper, | |
Avatar, | |
TextField, | |
Button, | |
Typography, | |
Link, | |
Box, | |
} from "@mui/material"; | |
import EmailOutlinedIcon from '@mui/icons-material/EmailOutlined'; | |
import LockOutlinedIcon from '@mui/icons-material/LockOutlined'; | |
import RemoveRedEyeOutlinedIcon from '@mui/icons-material/RemoveRedEyeOutlined'; | |
import "./login.css"; | |
import React from "react"; | |
import myLogotipoLogin from "../../assets/logotipoLogin.png"; | |
import myLogotipoLoginBranco from "../../assets/logotipoAtemBranco.png"; | |
export default function Login() { | |
const paperStyle = { | |
display: "grid", | |
placeItems:"center", | |
padding: "1.5rem", | |
margin: "2rem", | |
width: "28rem", | |
height: "32rem", | |
border: "1rem solid white", | |
borderRadius: "1rem", | |
fontFamily: "Poppins" | |
}; | |
return ( | |
<> | |
<Avatar style={{ position: "absolute", right: "6rem", top: "3rem", width:"auto", height:"auto"}} variant="square" src={myLogotipoLoginBranco}></Avatar> | |
<Grid sx={{mt:10}}> | |
<Paper style={paperStyle}> | |
<Avatar style={{ placeItems:"center", width:"auto",height:"auto", alignSelf:"end" }} variant="square" src={myLogotipoLogin}></Avatar> | |
<Typography style={{ | |
fontFamily: "Poppins", | |
color: "#D91F05", | |
fontSize: 35, | |
fontWeight: "bolder", | |
}}>Faça Login</Typography> | |
<Box sx={{ display: 'flex', alignItems: 'flex-end', width:"100%", }}> | |
<EmailOutlinedIcon sx={{ color: 'red', mr: 1, my: 0.5, opacity: '0.5' }} /> | |
{/* <TextField id="input-with-sx" label="With sx" variant="standard" /> */} | |
<TextField sx={{ background: "white", opacity:"0.5" }} | |
label="E-mail" | |
placeholder="Enter username" | |
variant="standard" | |
fullWidth | |
required | |
/> | |
</Box> | |
<Box sx={{ display: 'flex', alignItems: 'flex-end', width:"100%" }}> | |
<LockOutlinedIcon sx={{ color: 'red', mr: 1, my: 0.5, opacity: '0.5' }} /> | |
<TextField sx={{ background: "white", opacity:"0.5" }} | |
label="Senha" | |
placeholder="Enter password" | |
type="password" | |
variant="standard" | |
fullWidth | |
required | |
/> | |
</Box> | |
<Link href="#" sx={{justifySelf:"end", alignSelf:"start", mt:-5.5 }} > | |
<RemoveRedEyeOutlinedIcon sx={{ opacity: '0.3', mr: 1, fontSize:"15px", color:"red"}} /> | |
</Link> | |
<Typography sx={{ display:"grid", justifySelf:"end", alignSelf:"start", my: -5.0, fontWeight: "800" }}> | |
<Link sx={{ textDecoration:"auto", color:"#FEC82F", fontFamily:"Raleway" }} href="#">Esqueceu a senha?</Link> | |
</Typography> | |
<Button type="submit" color="primary" variant="contained" | |
sx={{ backgroundColor:"#D91F05", color:"white", p:1.8, width: "75%", mt:0.5, mb:2 }} fullWidth> | |
<Typography>Login</Typography> | |
</Button> | |
</Paper> | |
</Grid> | |
</> | |
); | |
} | |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
results -
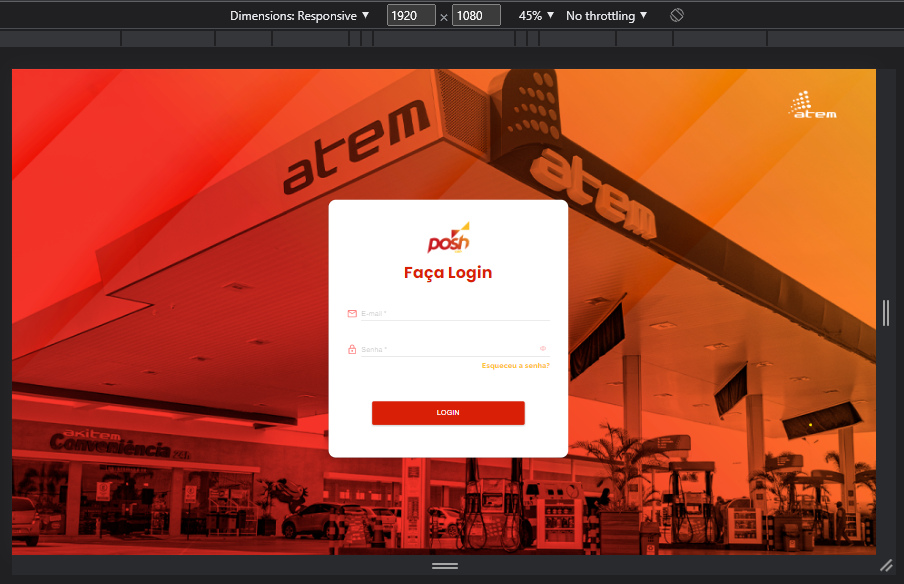