Created
April 15, 2016 20:59
-
-
Save kdzwinel/768edc1e00f7a57f8d1f4dff6bda688c to your computer and use it in GitHub Desktop.
Animate any UI component in a fancy way
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
function getFamilyTree(el) { | |
const levels = new Map(); | |
function bfs(el, level) { | |
const levelElements = levels.get(level) || []; | |
for (const child of el.children) { | |
child.style.opacity = '0'; | |
levelElements.push(child); | |
} | |
levels.set(level, levelElements); | |
for (const child of el.children) { | |
bfs(child, level + 1); | |
} | |
} | |
bfs(el, 0); | |
return levels; | |
} | |
function buildElement(el) { | |
const tree = getFamilyTree(el); | |
let lastPlayer = null; | |
for (var i = 0; i < tree.size; i++) { | |
const level = tree.get(i); | |
for (const child of level) { | |
const style = getComputedStyle(child); | |
if (child.tagName == 'DIV' && | |
style.backgroundColor === 'rgba(0, 0, 0, 0)') { | |
child.style.outline = 'solid white 25px'; | |
child.style.outlineOffset = '-25px'; | |
} | |
// if (style.display == 'inline') { | |
// child.style.display = 'inline-block'; | |
// } | |
const startFrame = { | |
opacity: 0, | |
//transform: 'translate3d(0,0,100px)', | |
color: 'white', | |
outlineColor: 'rgba(255,255,255,1)' | |
}; | |
const middleFrame = { | |
opacity: 1, | |
color: 'white', | |
outlineColor: 'rgba(255,255,255,0)', | |
offset: 0.8 | |
}; | |
const endFrame = { | |
opacity: 1, | |
//transform: 'translate3d(0,0,0)', | |
color: style.color, | |
outlineColor: 'rgba(255,255,255,0)', | |
}; | |
if (style.backgroundColor !== 'rgba(0, 0, 0, 0)') { | |
startFrame.backgroundColor = 'white'; | |
endFrame.backgroundColor = style.backgroundColor; | |
} | |
if (style.fill !== 'rgb(0, 0, 0)') { | |
startFrame.fill = 'white'; | |
endFrame.fill = style.fill; | |
} | |
var player = child.animate([startFrame, middleFrame, endFrame], { | |
duration: 300, | |
delay: i * 300, | |
easing: 'ease-in' | |
}); | |
player.addEventListener('finish', () => { | |
child.style.opacity = '1'; | |
child.style.outline = 'none'; | |
}); | |
lastPlayer = player; | |
} | |
} | |
if (lastPlayer) { | |
lastPlayer.addEventListener('finish', () => { | |
//run additional animations | |
}); | |
} | |
} | |
buildElement(document.querySelector('some-root-element')); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Variant 1 - playing with outlines:
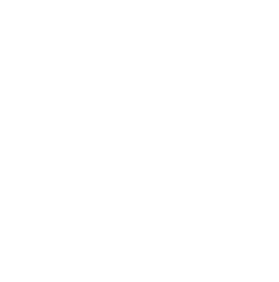
Variant 2 - playing with transforms:
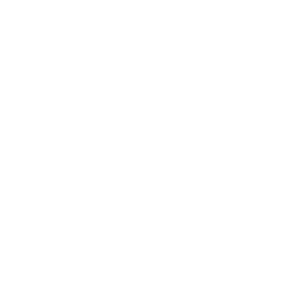
To run this one:
perspective: 200px
)