Last active
September 28, 2019 03:24
-
-
Save larsoner/3671bb6daea274bdc6812649cff574fc to your computer and use it in GitHub Desktop.
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import os | |
import mne | |
from mne.datasets import sample | |
from mne.minimum_norm import apply_inverse, read_inverse_operator | |
import numpy as np | |
sample_dir_raw = sample.data_path() | |
sample_dir = os.path.join(sample_dir_raw, 'MEG', 'sample') | |
subjects_dir = os.path.join(sample_dir_raw, 'subjects') | |
fname_evoked = os.path.join(sample_dir, 'sample_audvis-ave.fif') | |
fname_inv = os.path.join(sample_dir, 'sample_audvis-meg-vol-7-meg-inv.fif') | |
evoked = mne.read_evokeds(fname_evoked, condition=0, baseline=(None, 0)) | |
inverse_operator = read_inverse_operator(fname_inv) | |
stc = apply_inverse(evoked, inverse_operator, 1.0 / 3.0 ** 2, "dSPM") | |
stc.crop(0.09, 0.09) | |
# Use a sphere that creates a domain of the same shape (51, 51, 51) | |
pos = 5. | |
sphere_radius = 123. | |
sphere=(0., 0., 0., sphere_radius) | |
src_fs_vol_5mm = mne.setup_volume_source_space( | |
'fsaverage', subjects_dir=subjects_dir, pos=pos, sphere=sphere, | |
verbose=True) | |
assert (src_fs_vol_5mm[0]['shape'] == 51).all() | |
morph = mne.compute_source_morph( | |
inverse_operator['src'], subject_from='sample', subject_to='fsaverage', | |
zooms=pos, niter_affine=(10, 10, 1), niter_sdr=(2, 2, 1), | |
subjects_dir=subjects_dir, verbose=True) | |
stc_fsaverage = morph.apply(stc) | |
# Hack our fsaverage source space so that the vertices match | |
src_fs_vol_5mm[0]['inuse'].fill(0.) | |
src_fs_vol_5mm[0]['inuse'][stc_fsaverage.vertices] = 1 | |
# And so that the affine corresponds to that of our morph | |
src_fs_vol_5mm[0]['src_mri_t']['trans'] = morph.affine.copy() | |
src_fs_vol_5mm[0]['src_mri_t']['trans'][:3] /= 1000. | |
# All three of these are now equivalent | |
stc.plot(inverse_operator['src'], subject='sample', subjects_dir=subjects_dir) | |
stc.plot(morph, subjects_dir=subjects_dir) | |
stc_fsaverage.plot(src_fs_vol_5mm, subjects_dir=subjects_dir) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Figure 1:
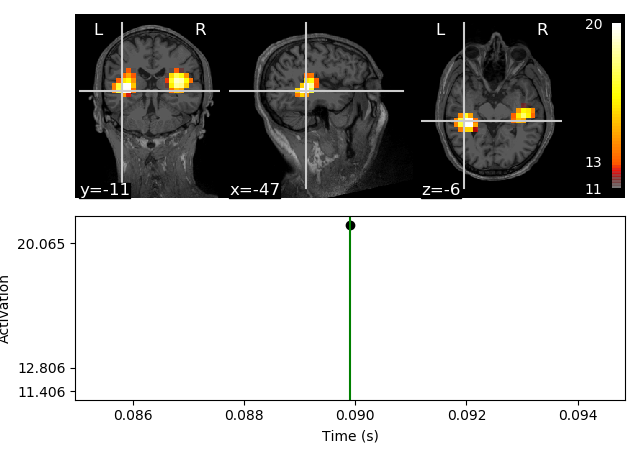
Figure 2:
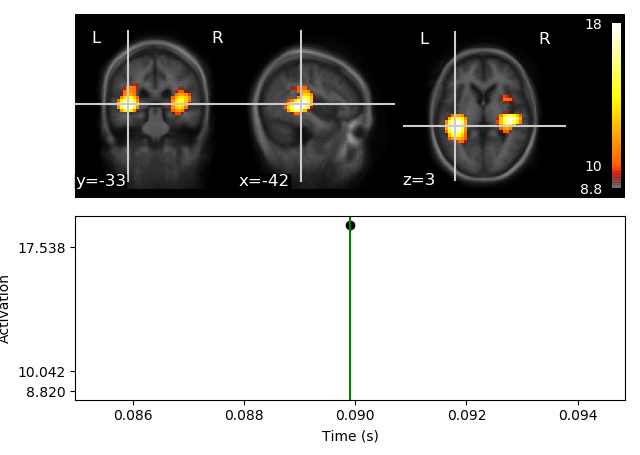
Figure 3:
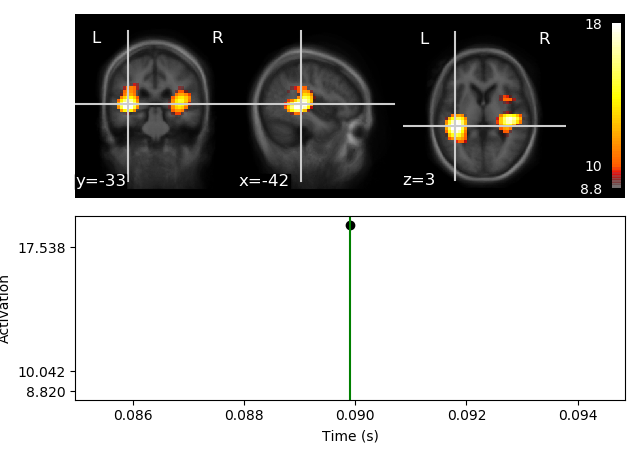