Last active
May 3, 2017 17:21
-
-
Save martindebrunne/b4878f3c598a1dd06c519acc4b879b17 to your computer and use it in GitHub Desktop.
Get a s3 signature for Ruby (used in ROR here) and how to set an image uploader using s3
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class AmazonService | |
class << self | |
def get_s3_upload_key(user_folder, project_folder) | |
bucket = < TO FILL > | |
access_key = < TO FILL > | |
secret_key = < TO FILL > | |
env = ENV["S3_ENV"] | |
key = "#{env}/#{user_folder}/#{project_folder}/" | |
expiration = 1.days.from_now.utc.strftime('%Y-%m-%dT%H:%M:%S.000Z') | |
max_filesize = 16.megabytes | |
acl = 'public-read' | |
region = 'eu-west-2' | |
date = Time.now.utc.strftime('%Y%m%d') | |
x_amz_credential = "#{access_key}/#{date}/#{region}/s3/aws4_request" | |
x_amz_date = Time.now.utc.strftime('%Y%m%dT%H%M%S000Z') | |
service = 's3' | |
policy = { | |
'expiration' => expiration, | |
'conditions' => [ | |
{'bucket' => bucket}, | |
['starts-with', '$key', key], | |
{'acl' => acl}, | |
{'success_action_redirect' => '< TO FILL >'}, | |
{'Cache-Control' => 'max-age=31536000'}, | |
{'x-amz-meta-uuid' => '14365123651274'}, | |
{'x-amz-server-side-encryption' => 'AES256'}, | |
['starts-with', '$x-amz-meta-tag', 'test'], | |
['starts-with', '$Content-Type', 'image/jpeg'], | |
["starts-with", "$key", "#{env}/user_test/project_test/"], | |
['content-length-range', 1, max_filesize], | |
{'x-amz-credential' => x_amz_credential}, | |
{'x-amz-algorithm' => 'AWS4-HMAC-SHA256'}, | |
{'x-amz-date' => x_amz_date} | |
] | |
} | |
policy = Base64.encode64(policy.to_json).gsub(/\n|\r/, '') | |
signature = AmazonS3Service.get_signature_key(secret_key, date, region, service, policy) | |
{ | |
:access_key => access_key, | |
:key => key, | |
:policy => policy, | |
:signature => signature, | |
:bucket => bucket, | |
:acl => acl, | |
:x_amz_credential => x_amz_credential, | |
:x_amz_date => x_amz_date, | |
:expiration => expiration | |
} | |
end | |
def get_signature_key(secred_key, date_stamp, region_name, service_name, policy) | |
k_date = OpenSSL::HMAC.digest('sha256', "AWS4" + secred_key, date_stamp) | |
k_region = OpenSSL::HMAC.digest('sha256', k_date, region_name) | |
k_service = OpenSSL::HMAC.digest('sha256', k_region, service_name) | |
k_signing = OpenSSL::HMAC.digest('sha256', k_service, "aws4_request") | |
OpenSSL::HMAC.hexdigest('sha256', k_signing, policy) | |
end | |
end | |
end |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<!DOCTYPE html> | |
<html> | |
<head> | |
<title>test</title> | |
</head> | |
<body> | |
<form action="< YOUR BUCKET >.s3.amazonaws.com/" method="post" enctype="multipart/form-data"> | |
Key to upload: | |
<input type="input" name="key" value="development/user_test/project_test/${filename}" /><br /> | |
<input type="hidden" name="acl" value="public-read" /> | |
<input type="hidden" name="success_action_redirect" value="< TO FILL >" /> | |
Content-Type: | |
<input type="input" name="Content-Type" value="image/jpeg" /><br /> | |
<input type="input" name="Cache-Control" value="max-age=31536000" /><br /> | |
<input type="hidden" name="x-amz-meta-uuid" value="14365123651274" /> | |
<input type="hidden" name="x-amz-server-side-encryption" value="AES256" /> | |
<input type="text" name="X-Amz-Credential" value="< TO FILL >" /> | |
<input type="text" name="X-Amz-Algorithm" value="AWS4-HMAC-SHA256" /> | |
<input type="text" name="X-Amz-Date" value="< TO FILL >" /> | |
Tags for File: | |
<input type="input" name="x-amz-meta-tag" value="< TO FILL >" /><br /> | |
<input type="hidden" name="Policy" value='< TO FILL >' /> | |
<input type="hidden" name="X-Amz-Signature" value="< TO FILL >" /> | |
File: | |
<input type="file" name="file" /> <br /> | |
<!-- The elements after this will be ignored --> | |
<input type="submit" name="submit" value="Upload to Amazon S3" /> | |
</form> | |
</body> | |
</html> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
According to this:
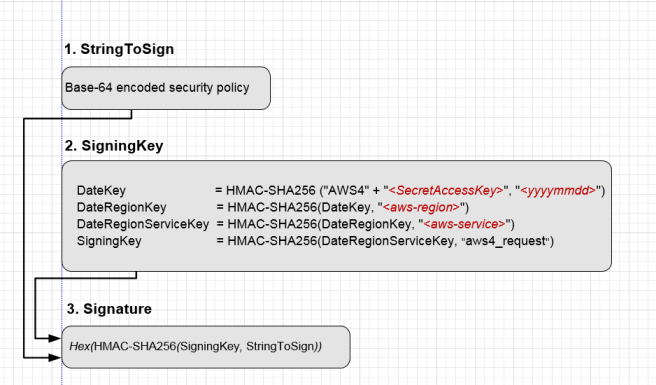
http://docs.aws.amazon.com/AmazonS3/latest/API/sigv4-post-example.html
http://docs.aws.amazon.com/AmazonS3/latest/API/sigv4-HTTPPOSTConstructPolicy.html
http://docs.aws.amazon.com/AmazonS3/latest/API/sigv4-authentication-HTTPPOST.html
http://docs.aws.amazon.com/AmazonS3/latest/API/sigv4-HTTPPOSTForms.html