-
-
Save mgedmin/2762225 to your computer and use it in GitHub Desktop.
#!/usr/bin/python | |
"""Print a swatch using all 256 colors of 256-color-capable terminals.""" | |
__author__ = "Marius Gedminas <[email protected]>" | |
__url__ = "https://gist.github.com/mgedmin/2762225" | |
__version__ = '2.0' | |
def hrun(start, width, padding=0): | |
return [None] * padding + list(range(start, start + width)) + [None] * padding | |
def vrun(start, width, height, padding=0): | |
return [hrun(s, width, padding) | |
for s in range(start, start + width * height, width)] | |
layout = [ | |
vrun(0, 8, 2), # 16 standard xterm colors | |
vrun(16, 6, 6 * 6, 1), # 6x6x6 color cube | |
vrun(16 + 6 * 6 * 6, 8, 3), # 24 grey levels | |
] | |
def fg_seq(color): | |
return '\033[38;5;%dm' % color | |
def bg_seq(color): | |
return '\033[48;5;%dm' % color | |
reset_seq = '\033[0m' | |
def color_bar(seq, color, trail): | |
if color is None: | |
return '%s %s' % (reset_seq, trail) | |
else: | |
return '%s %03d%s' % (seq(color), color, trail) | |
def main(): | |
for block in layout: | |
print("") | |
for row in block: | |
fg_bar = ''.join(color_bar(fg_seq, color, '') for color in row) | |
bg_bar = ''.join(color_bar(bg_seq, color, ' ') for color in row) | |
print('%s%s %s%s' % (fg_bar, reset_seq, bg_bar, reset_seq)) | |
if __name__ == '__main__': | |
main() |
Version 2.0: support Python 3 (as well as Python 2).
wow. Thats really cool!
Really cool
This is really nicely done. I was interested in running it without Python, so I wrote a Bash translation: https://gist.github.com/ivanbrennan/8ce10a851851e5f04728d8da900ef1c5
thanks, it help me a lot!!
A newer version is at https://github.com/mgedmin/scripts/blob/master/show-all-256-colors. You can give it color numbers as command-line arguments and it'll show just those colors, plus their RGB values that match my terminal.
In my opinion if the new py program has its pic, it will be better~
I do like your pic that help me get the right color in my little program.
Yeah, without any arguments it prints the same diagram as before.
I use the RGB values to make Vim color schemes to have guifg=#rrggbb match my ctermfg=208 etc.
Output:
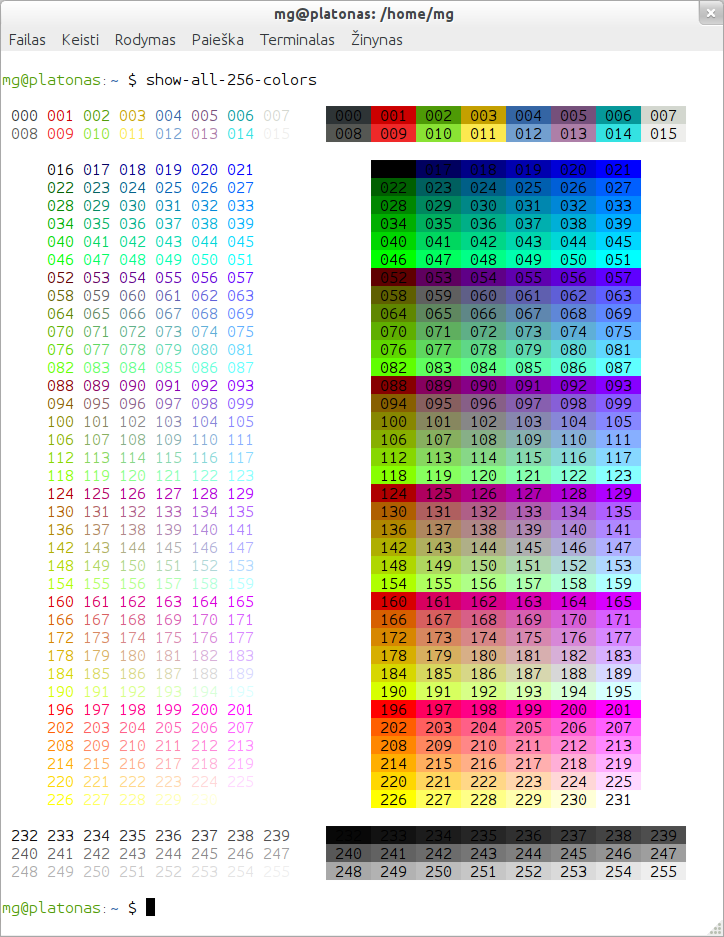