Created
July 13, 2017 11:46
-
-
Save miguelgrinberg/5a1b3749dbe1bb254ff7a41e59cf04c9 to your computer and use it in GitHub Desktop.
datetimepicker-example
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from flask import Flask, render_template | |
from flask_bootstrap import Bootstrap | |
from flask_wtf import Form | |
from wtforms.fields import DateField | |
app = Flask(__name__) | |
app.config['SECRET_KEY'] = 'secret' | |
Bootstrap(app) | |
class MyForm(Form): | |
date = DateField(id='datepick') | |
@app.route('/') | |
def index(): | |
form = MyForm() | |
return render_template('index.html', form=form) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{% extends "bootstrap/base.html" %} | |
{% import "bootstrap/wtf.html" as wtf %} | |
{% block title %}This is an example page{% endblock %} | |
{% block head %} | |
{{ super() }} | |
<link type="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-datetimepicker/4.17.47/css/bootstrap-datetimepicker.min.css"> | |
{% endblock %} | |
{% block content %} | |
<div class="container"> | |
<h1>Hello, Bootstrap</h1> | |
<div class="row"> | |
<div class='col-sm-6'> | |
{{ wtf.quick_form(form) }} | |
</div> | |
</div> | |
</div> | |
{% endblock %} | |
{% block scripts %} | |
{{ super() }} | |
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.18.1/moment.min.js"></script> | |
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-datetimepicker/4.17.47/js/bootstrap-datetimepicker.min.js"></script> | |
<script type="text/javascript"> | |
$(function () { | |
$('#datepick').datetimepicker(); | |
}); | |
</script> | |
{% endblock %} |
Well, simply use DateTimeLocalField
would solve all these sufferings.
Sample Here
from flask_wtf import FlaskForm
from wtforms.fields import StringField, TextAreaField, SubmitField, DateTimeLocalField
from wtforms.validators import DataRequired, Optional
class PasteForm(FlaskForm):
title = StringField('Title here')
body = TextAreaField('Just paste', validators=[DataRequired()])
expiry = DateTimeLocalField('Expiry date', validators=[Optional()])
submit = SubmitField('Submit')
And the HTML is quite simple, No JS needed except basic BootStrap
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
@miguelgrinberg I took a look inside the docs, using a time only picker, i realized that is similar as your original approach, i just add 2 more fields in the form (begin_time and end_time):
class Filters(FlaskForm):
In the script section, i just add the additional tags:
$(function () {
$('#begin_date').datetimepicker({format:'YYYY-MM-DD'});
$('#end_date').datetimepicker({format:'YYYY-MM-DD'});
$('#begin_time').datetimepicker({format: 'hh:mm A'});
$('#end_time').datetimepicker({format: 'hh:mm A'});
});
I modified your original code (thanks a lot for this), to accomplish my goals, these variables are going to be used later as filters to make some queries to the data base!
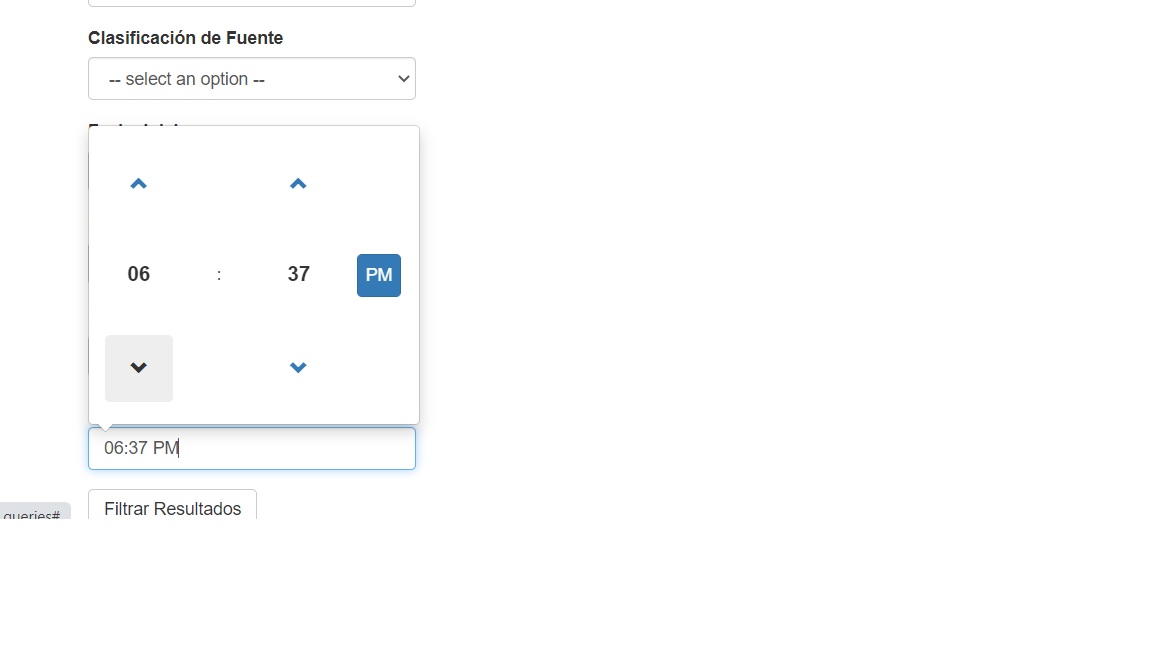
https://ibb.co/ZM4fTdf