Last active
May 19, 2023 16:58
-
-
Save mlschmitt/e06b1d82ddfcf68497d7fe994f7daea3 to your computer and use it in GitHub Desktop.
Random wisdom from Merlin Mann in a handy widget - https://github.com/merlinmann/wisdom
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// Variables used by Scriptable. | |
// These must be at the very top of the file. Do not edit. | |
// icon-color: blue; icon-glyph: brain; | |
let items = await loadItems() | |
if (config.runsInWidget) { | |
let widget = await createWidget(items) | |
Script.setWidget(widget) | |
} else if (config.runsWithSiri) { | |
let firstItems = items.slice(0, 5) | |
let table = createTable(firstItems) | |
await QuickLook.present(table) | |
} else { | |
let table = createTable(items) | |
await QuickLook.present(table) | |
} | |
Script.complete() | |
async function createWidget(items) { | |
// Get random wisdom entry | |
let item = items[Math.floor(Math.random()*items.length)] | |
let gradient = new LinearGradient() | |
gradient.locations = [0, 1] | |
let gradientOptions = [ | |
[new Color("#9b59b6"), new Color("#8e44ad")], // purple | |
[new Color("#3498db"), new Color("#2980b9")], // blue | |
[new Color("#1abc9c"), new Color("#16a085")], // teal | |
[new Color("#e67e22"), new Color("#d35400")], // orange | |
[new Color("#e74c3c"), new Color("#c0392b")] // red | |
] | |
let targetGradient = gradientOptions[Math.floor(Math.random()*gradientOptions.length)] | |
gradient.colors = targetGradient | |
let widget = new ListWidget() | |
widget.backgroundColor = targetGradient[1] | |
widget.backgroundGradient = gradient | |
// Add spacer above content to center it vertically. | |
widget.addSpacer() | |
let title = cleanString(item) | |
let titleElement = widget.addText(title) | |
titleElement.font = Font.boldSystemFont(16) | |
titleElement.textColor = Color.white() | |
titleElement.minimumScaleFactor = 0.5 | |
// Add spacing below content to center it vertically. | |
widget.addSpacer() | |
return widget | |
} | |
function createTable(items) { | |
let table = new UITable() | |
for (item of items) { | |
let row = new UITableRow() | |
let title = cleanString(item) | |
let titleCell = row.addText(title) | |
titleCell.minimumScaleFactor = 0.5 | |
titleCell.widthWeight = 60 | |
row.height = 60 | |
row.cellSpacing = 10 | |
row.onSelect = (idx) => { | |
let item = items[idx] | |
let alert = new Alert() | |
alert.message = cleanString(item) | |
alert.presentAlert() | |
} | |
row.dismissOnSelect = false | |
table.addRow(row) | |
} | |
return table | |
} | |
async function loadItems() { | |
let url = "https://raw.githubusercontent.com/merlinmann/wisdom/master/wisdom.md" | |
let req = new Request(url) | |
let rawBody = await req.loadString() | |
let wisdomComponents = rawBody.split("# The Wisdom So Far")[1] | |
wisdomComponents = wisdomComponents.split("----\n\n[**")[0] | |
let bodyComponents = wisdomComponents.split("\n-") | |
bodyComponents.splice(0, 1) | |
return bodyComponents | |
} | |
function cleanString(str) { | |
let cleanedString = str.replace("Related: ", "") | |
cleanedString = cleanedString.split("\n\n")[0] | |
cleanedString = cleanedString.split("\n* *")[0] | |
cleanedString = cleanedString.split("\n##")[0] | |
cleanedString = cleanedString.trim() | |
let regex = /&#(\d+);/g | |
return cleanedString.replace(regex, (match, dec) => { | |
return String.fromCharCode(dec) | |
}) | |
} |
This is amazing! Thanks for sharing.
Thanks for this! I swapped out the gist URL to use https://github.com/merlinmann/wisdom/blob/master/wisdom.md
and it pulls everything from the new location just the same.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Preview: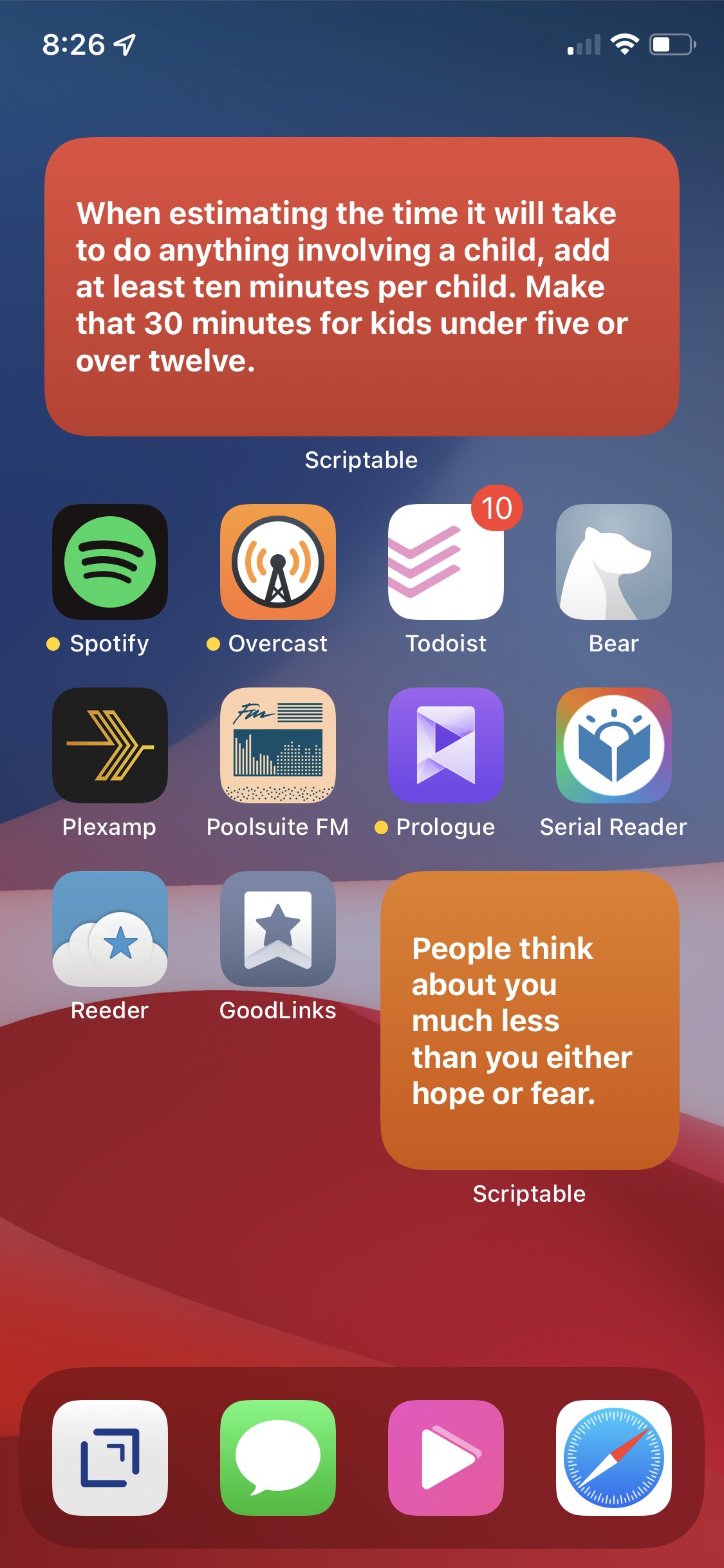