Created
October 19, 2017 02:41
-
-
Save monkeywithacupcake/394c2ade2e28bbc97528e1e791cc3068 to your computer and use it in GitHub Desktop.
Swift 4 Playground showing how to build StackView, Horizontal CollectionView, and work with unicode emojis programmatically
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
//: Playground - noun: a place where people can play | |
import UIKit | |
import PlaygroundSupport | |
extension String { | |
func getUnicodeCodePoints() -> [String] { | |
return unicodeScalars.map { "U+" + String($0.value, radix: 16, uppercase: true) } | |
} | |
} | |
class MyCell: UICollectionViewCell { | |
let myLabel: UILabel = { | |
let label = UILabel(frame: CGRect(x:0,y: 0,width: 100,height: 100)) | |
label.font = UIFont.systemFont(ofSize: 64) | |
label.textAlignment = .center | |
label.textColor = UIColor.white | |
return label | |
}() | |
override func awakeFromNib() { | |
// self.addSubview(self.myLabel) | |
} | |
override init(frame: CGRect) { | |
super.init(frame: frame) | |
addViews() | |
} | |
func addViews(){ | |
backgroundColor = UIColor.gray | |
addSubview(myLabel) | |
} | |
required init?(coder aDecoder: NSCoder) { | |
fatalError("init(coder:) has not been implemented") | |
} | |
} | |
class MyViewController : UIViewController, UICollectionViewDelegateFlowLayout, UICollectionViewDelegate, UICollectionViewDataSource { | |
var headerView: UIView! | |
var titleLabel: UILabel! | |
var selView: UIView! | |
var selLabel: UILabel! | |
var collectionView: UICollectionView? | |
let myChars = ["π€", "π¦", "π¨βπ€", "π©βπΎ", "π©βπ»", "π΅οΈ", "π©βπ", "π¨βπ", "π©βπ¬"] | |
override func viewDidLoad() { | |
super.viewDidLoad() | |
setupHeader() | |
setupCollection() | |
setupSelection() | |
setupStack() | |
} | |
// MARK: CollectionView Methods | |
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int { | |
return myChars.count | |
} | |
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize { | |
return CGSize(width: 100.0, height: 100.0) | |
} | |
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell { | |
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "PlayCell", for: indexPath) as! MyCell | |
cell.myLabel.text = myChars[indexPath.row] | |
return cell | |
} | |
func collectionView(_ collectionView: UICollectionView, didSelectItemAt indexPath: IndexPath) { | |
let selected = myChars[indexPath.row] | |
let s = String(selected).getUnicodeCodePoints() | |
let selString = "You picked \(selected) with scalars \(s)" | |
selLabel.text = selString | |
} | |
// MARK: SetUp Methods | |
func setupHeader() { | |
headerView = UIView() | |
headerView.backgroundColor = .white | |
titleLabel = UILabel() | |
titleLabel.text = "Which One?" | |
titleLabel.textAlignment = .center | |
titleLabel.font = UIFont(name: titleLabel.font.fontName, size: 20) | |
headerView.addSubview(titleLabel) | |
titleLabel.translatesAutoresizingMaskIntoConstraints = false | |
titleLabel.centerXAnchor.constraint(equalTo: headerView.centerXAnchor).isActive = true | |
titleLabel.centerYAnchor.constraint(equalTo: headerView.centerYAnchor).isActive = true | |
} | |
func setupCollection() { | |
let frame = self.view.frame | |
let layout = UICollectionViewFlowLayout() | |
layout.scrollDirection = .horizontal | |
collectionView = UICollectionView(frame: frame, collectionViewLayout: layout) | |
collectionView?.delegate = self | |
collectionView?.dataSource = self | |
self.collectionView?.backgroundColor = .white | |
self.collectionView?.register(MyCell.self, forCellWithReuseIdentifier: "PlayCell") | |
collectionView?.alwaysBounceVertical = false | |
} | |
func setupSelection() { | |
selView = UIView() | |
selView.backgroundColor = .white | |
selLabel = UILabel() | |
selLabel.text = "" | |
selLabel.numberOfLines = 0 | |
selLabel.lineBreakMode = .byWordWrapping | |
selLabel.textAlignment = .center | |
selLabel.font = UIFont(name: selLabel.font.fontName, size: 14) | |
selView.addSubview(selLabel) | |
selLabel.translatesAutoresizingMaskIntoConstraints = false | |
selLabel.centerXAnchor.constraint(equalTo: selView.centerXAnchor).isActive = true | |
selLabel.centerYAnchor.constraint(equalTo: selView.centerYAnchor).isActive = true | |
selLabel.widthAnchor.constraint(equalTo: selView.widthAnchor).isActive = true | |
} | |
func setupStack() { | |
let stackView = UIStackView(arrangedSubviews: [headerView, collectionView!, selView]) | |
stackView.axis = .vertical | |
stackView.distribution = .fillEqually | |
stackView.alignment = .fill | |
stackView.spacing = 10 | |
stackView.translatesAutoresizingMaskIntoConstraints = false | |
view.addSubview(stackView) | |
//autolayout the stack view | |
let sH = NSLayoutConstraint.constraints(withVisualFormat: "H:|-20-[stackView]-20-|", options: NSLayoutFormatOptions(rawValue: 0), metrics: nil, views: ["stackView":stackView]) | |
let sV = NSLayoutConstraint.constraints(withVisualFormat: "V:|-30-[stackView]-30-|", options: NSLayoutFormatOptions(rawValue:0), metrics: nil, views: ["stackView":stackView]) | |
view.addConstraints(sH) | |
view.addConstraints(sV) | |
} | |
} | |
PlaygroundPage.current.liveView = MyViewController() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
The playground will display the stack view with the horizontal scrolling layout. This can also be used in a real project.
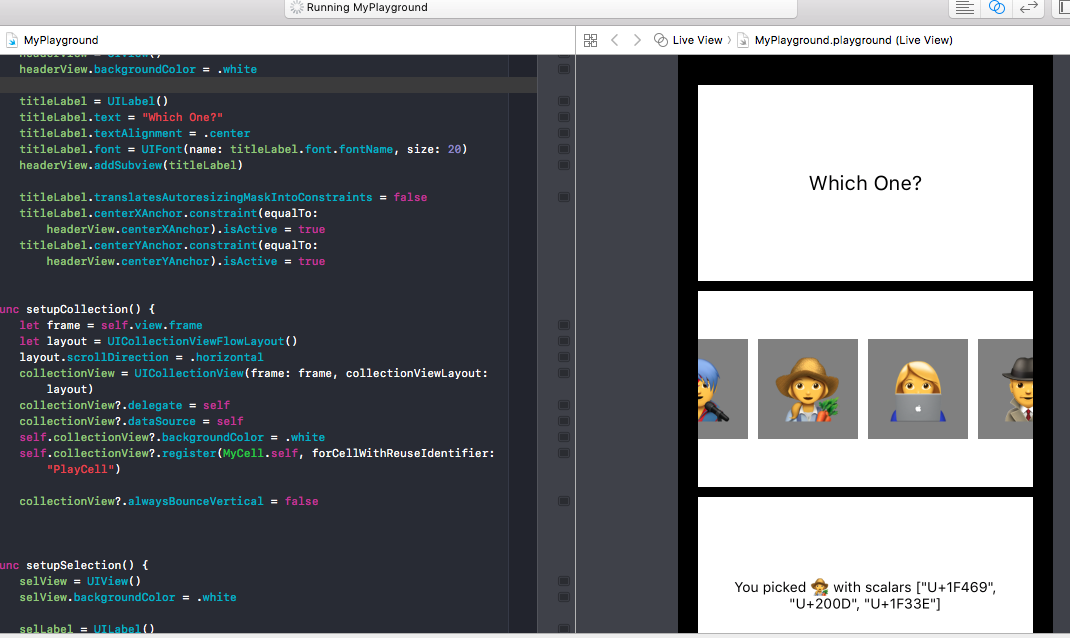