Created
January 3, 2020 15:58
-
-
Save muhleder/9df9cf63ef4da9c7b7d5f4e62ecb8010 to your computer and use it in GitHub Desktop.
Memory leak demo
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// Flutter code sample for Scaffold | |
// This example shows a [Scaffold] with an [AppBar], a [BottomAppBar] and a | |
// [FloatingActionButton]. The [body] is a [Text] placed in a [Center] in order | |
// to center the text within the [Scaffold]. The [FloatingActionButton] is | |
// centered and docked within the [BottomAppBar] using | |
// [FloatingActionButtonLocation.centerDocked]. The [FloatingActionButton] is | |
// connected to a callback that increments a counter. | |
// | |
// 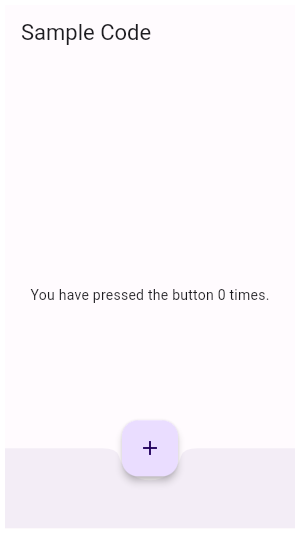 | |
import 'package:flutter/material.dart'; | |
import 'package:syncfusion_flutter_gauges/gauges.dart'; | |
void main() => runApp(MyApp()); | |
/// This Widget is the main application widget. | |
class MyApp extends StatelessWidget { | |
static const String _title = 'Flutter Code Sample'; | |
@override | |
Widget build(BuildContext context) { | |
return MaterialApp( | |
title: _title, | |
home: MyStatefulWidget(), | |
); | |
} | |
} | |
class MyStatefulWidget extends StatefulWidget { | |
MyStatefulWidget({Key key}) : super(key: key); | |
@override | |
_MyStatefulWidgetState createState() => _MyStatefulWidgetState(); | |
} | |
class _MyStatefulWidgetState extends State<MyStatefulWidget> { | |
int _count = 0; | |
Widget build(BuildContext context) { | |
return Scaffold( | |
appBar: AppBar( | |
title: Text('Sample Code'), | |
), | |
body: Center( | |
child: Column( | |
children: <Widget>[ | |
Text('You have pressed the button $_count times.'), | |
SfRadialGauge( | |
axes: <RadialAxis>[ | |
RadialAxis( | |
showLabels: false, | |
showTicks: false, | |
startAngle: 270, | |
endAngle: 270, | |
minimum: 0, | |
maximum: 100, | |
radiusFactor: 0.8, | |
axisLineStyle: AxisLineStyle(thicknessUnit: GaugeSizeUnit.factor, thickness: 0.15), | |
annotations: <GaugeAnnotation>[ | |
GaugeAnnotation( | |
angle: 180, | |
widget: Row( | |
children: <Widget>[ | |
Container( | |
child: Text('50'), | |
), | |
Container( | |
child: Text(' / 100'), | |
) | |
], | |
)), | |
], | |
pointers: <GaugePointer>[ | |
RangePointer( | |
value: 50, | |
cornerStyle: CornerStyle.bothCurve, | |
enableAnimation: true, | |
animationDuration: 1200, | |
animationType: AnimationType.ease, | |
sizeUnit: GaugeSizeUnit.factor, | |
gradient: const SweepGradient(colors: <Color>[Color(0xFF6A6EF6), Color(0xFFDB82F5)], stops: <double>[0.25, 0.75]), | |
color: const Color(0xFF00A8B5), | |
width: 0.15, | |
), | |
], | |
), | |
], | |
) | |
], | |
), | |
), | |
bottomNavigationBar: BottomAppBar( | |
shape: const CircularNotchedRectangle(), | |
child: Container( | |
height: 50.0, | |
), | |
), | |
floatingActionButton: FloatingActionButton( | |
onPressed: () => setState(() { | |
_count++; | |
}), | |
tooltip: 'Increment Counter', | |
child: Icon(Icons.add), | |
), | |
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked, | |
); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment