Created
April 2, 2020 12:13
-
-
Save nielsonsantana/d4f607c933d6f2e0a9c4ef34e45f8434 to your computer and use it in GitHub Desktop.
Benchmark of a recursive function that prevent data breach
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import statistics | |
import collections | |
from timeit import default_timer as timer | |
from bench_data import DEFAULT_KEYS_TO_CLEAN | |
from bench_data import large_payload | |
from bench_data import small_payload | |
from bench_data import very_large_payload | |
def mask_suffix_asterisk(value, number=2): | |
value = str(value) | |
value_len = len(value) | |
if number <= 0: | |
return value_len * '*' | |
return value[:number] + (value_len - number) * '*' | |
def hide_sensitive_data(message, keys_to_clean=None): | |
payload = {} | |
if not keys_to_clean: | |
keys_to_clean = DEFAULT_KEYS_TO_CLEAN | |
for key, value in message.items(): | |
if isinstance(value, collections.Mapping): | |
message_updated = hide_sensitive_data(value) | |
payload.update({key: message_updated}) | |
else: | |
key_normalized = key.lower() | |
if key_normalized in keys_to_clean: | |
caracters_limit = keys_to_clean.get(key_normalized) | |
value = mask_suffix_asterisk(value, caracters_limit) | |
payload.update({key: value}) | |
return payload | |
first_time = timer() | |
logs_per_request_up_to = 11 | |
number_of_requests = 500 | |
for logs_per_request in range(1, logs_per_request_up_to): | |
snapshots = [] | |
for x in range(number_of_requests): | |
snapshot = timer() | |
for x in range(logs_per_request): | |
hide_sensitive_data(small_payload) | |
hide_sensitive_data(large_payload) | |
hide_sensitive_data(very_large_payload) | |
snapshots.append(round(timer() - snapshot, 5)) | |
print('Média', logs_per_request * 3, round(statistics.mean(snapshots), 6)) | |
print('Final time time: {}s'.format(round(timer() - first_time, 3))) |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
DEFAULT_KEYS_TO_CLEAN = { | |
"profiletoken": 3, | |
"cardtoken": 3, | |
"phone": 3, | |
"password": 0, | |
"username": 10, | |
# Random keys | |
'grateful': 3, | |
'subtract': 3, | |
'extend': 3, | |
'shoes': 3, | |
'crowded': 3, | |
'energetic': 3, | |
'land': 3, | |
'battle': 3, | |
'combative': 3, | |
'seat': 3, | |
'annoy': 3, | |
'oil': 3, | |
'queen': 3, | |
'sink': 3, | |
'sister': 3, | |
'strap': 3, | |
'fence': 3, | |
'worthless': 3, | |
'abortive': 3, | |
'glow': 3, | |
'omniscient': 3, | |
'disapprove': 3, | |
'rabbit': 3, | |
'eyes': 3, | |
'bizarre': 3, | |
'fall': 3, | |
'title': 3, | |
'anger': 3, | |
'abaft': 3, | |
'pie': 3, | |
'badge': 3, | |
'sisters': 3, | |
'board': 3, | |
'gun': 3, | |
'full': 3, | |
'shelter': 3, | |
'foregoing': 3, | |
'competition': 3, | |
'partner': 3, | |
'credit': 3, | |
'offbeat': 3, | |
'vulgar': 3, | |
'hellish': 3, | |
'flock': 3, | |
'fold': 3, | |
'habitual': 3, | |
'sound': 3, | |
'feeble': 3, | |
'suit': 3, | |
'unkempt': 2, | |
'billingZipcode': 3, | |
} | |
small_payload = { | |
"python_logger": "tem_api_buy_plan_log", | |
"message": | |
{ | |
"logger_name": "tem_api_buy_plan_log", | |
"request_id": "b6f7ca10-28e3-4c44-9124-a8f1ac0fce4b", | |
"user_id": 209, | |
"environment": "development", | |
"level": "INFO", | |
"path": "/home/work/workspace/work/general/temapi/financial/views/purchase_plan.py", | |
"@timestamp": "2020-04-01T17:55:51.153Z", | |
"message": "Input Buy Plan Payload", | |
"email": "[email protected]", | |
"payload": { | |
"stack_info": None, | |
"voucher": "", | |
"credit_card": 58, | |
"payment_type": 4, | |
"auto_renew": True, | |
"plan": "77" | |
}, | |
"tracker_id": "b6f7ca10-28e3-4c44-9124-a8f1ac0fce4b", | |
"host": "work-notebook" | |
} | |
} | |
large_payload = { | |
"python_logger": "tem_api_buy_plan_log", | |
"message": { | |
"user_id": 209, | |
"environment": "development", | |
"level": "INFO", | |
"path": "/home/work/workspace/work/general/temapi/lib/pbsc/services.py", | |
"@timestamp": "2020-04-01T17:55:51.177Z", | |
"message": "Buy Plan Payload Send To PBSC", | |
"payload": { | |
"phone": "551**********", | |
"password": "********************", | |
"nbBikes": 1, | |
"autoRenew": True, | |
"details": { | |
"phone": "55119963*****", | |
"billingCountryCode": 76, | |
"address1": "Rua São Cristovam, 112", | |
"billingCity": "São Paulo", | |
"countryCode": 76, | |
"sameBilling": True, | |
"city": "São Paulo", | |
"billingZipcode": "02111-090", | |
"billingState": 329, | |
"language": "pt", | |
"birthdate": "1989-12-30", | |
"gender": 1, | |
"billingApt": "", | |
"address2": "Vila Paradoxo", | |
"apt": "", | |
"billingAddress": "Rua São Cristovam" | |
}, | |
"email": "[email protected]", | |
"zipcode": "01222-010", | |
"redirectUrl": "http://teste.com.br", | |
"stack_info": None, | |
"planId": "1", | |
"hpp": True, | |
"lastname": "Test Full", | |
"paymentInfo": { | |
"profileToken": "1ab*****************************", | |
"gatewayprovider": "AdyenECommerce", | |
"expirationdate": "032020", | |
"cardholdername": "APPROVED", | |
"lastfourdigits": "9245", | |
"cardToken": "841*************" | |
}, | |
"firstname": "Dev" | |
}, | |
"email": "[email protected]", | |
"tracker_id": "b6f7ca10-28e3-4c44-9124-a8f1ac0fce4b", | |
"host": "work-notebook", | |
"logger_name": "tem_api_buy_plan_log", | |
"request_id": "b6f7ca10-28e3-4c44-9124-a8f1ac0fce4b" | |
} | |
} | |
very_large_payload = { | |
"python_logger": "tem_api_buy_plan_log", | |
"message": { | |
"@timestamp": "2020-04-01T17:55:54.323Z", | |
"message": "Response PBSC Payload", | |
"email": "[email protected]", | |
"payload": { | |
"stack_info": None, | |
"amount": 0, | |
"paymentDetail": { | |
"email": "[email protected]", | |
"id": 494606, | |
"canRent": True, | |
"agreementDate": "2020-04-01 17:55:53", | |
"phoneNumber": "5511996******", | |
"totpSharedSecret": "a5f10876-8115-473e-8484-790f90d55df3", | |
"card": { | |
"cardNumberFourDigits": "9245", | |
"cardName": "APPROVED", | |
"expiry": "2020-03-31" | |
}, | |
"virtualKey": "26510787818C17AC0EB5", | |
"plan": { | |
"renewsToAnotherProduct": None, | |
"endTime": "2030-04-01 17:55:53", | |
"startTime": "2020-04-01 17:55:53", | |
"canManuallyRenew": False, | |
"planId": 1, | |
"maxBikes": 1, | |
"planName": "Inscription (with credit Card)", | |
"type": "TIME_BASED", | |
"cost": 0, | |
"prepaidBalance": None, | |
"subtotal": 0, | |
"total": 0, | |
"status": "Active", | |
"autoRenew": True, | |
"canAutoRenew": True | |
}, | |
"agreementVersion": "1.0", | |
"requireKey": False, | |
"token": "d1253c34-58fc-4d3c-9f92-96e90b57545a", | |
"isUsingCreditCard": True, | |
"canReserve": True, | |
"details": { | |
"address2": "Vila Paradoxo", | |
"zipcode": "01222-010", | |
"billingAddress": "Rua São Cristovam", | |
"optInMarketingSmsLevel": 0, | |
"optInMarketingEmailsLevel": 0, | |
"phone2": "5511996******", | |
"address1": "Rua São Cristovam 256", | |
"billingCity": "São Paulo", | |
"billingStateCode": "AL", | |
"optInAccountEmails": True, | |
"optInMarketingSms": False, | |
"phone1": "5511996******", | |
"city": "São Paulo", | |
"billingZipcode": "01222-010", | |
"optInMarketingEmails": False, | |
"lastname": "Test Full", | |
"optInAccountSms": True, | |
"firstname": "Dev", | |
"billingState": 329, | |
"language": "pt", | |
"birthdate": "1989-12-30", | |
"gender": 1, | |
"complete": False | |
} | |
} | |
}, | |
"tracker_id": "b6f7ca10-28e3-4c44-9124-a8f1ac0fce4b", | |
"host": "work-notebook", | |
"logger_name": "tem_api_buy_plan_log", | |
"request_id": "b6f7ca10-28e3-4c44-9124-a8f1ac0fce4b", | |
"user_id": 209, | |
"environment": "development", | |
"level": "INFO", | |
"path": "/home/work/workspace/work/general/temapi/lib/pbsc/services.py" | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Benchmark graph of
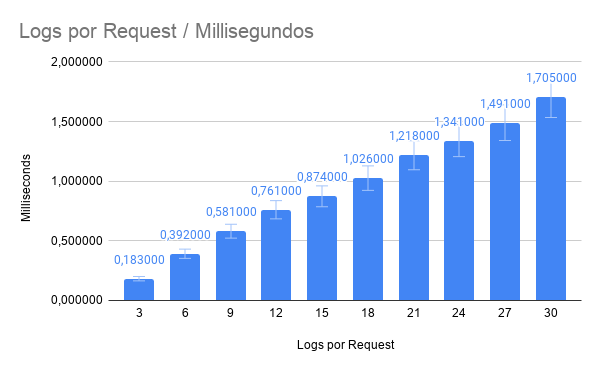
hide_sensitive_data
exection with three types of paylod(small_payload
,large_payload
,very_large_payload
).In the extreme case, 30 logs per cycle(a request) can delay in up to 1.8 milliseconds a request.
Benchmark Environment: Ubuntu 18.04, i5-8250U @ 1.60GHz.