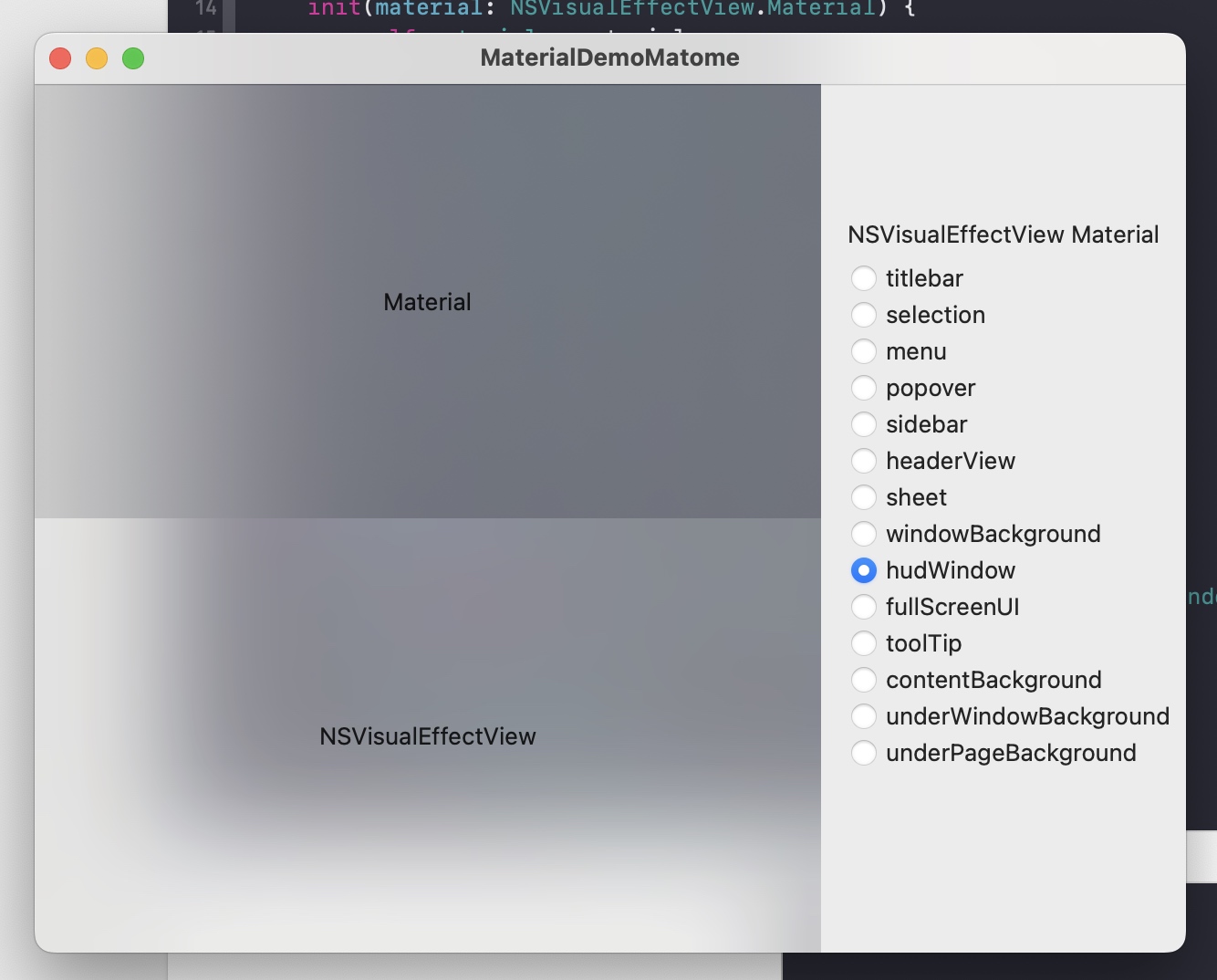
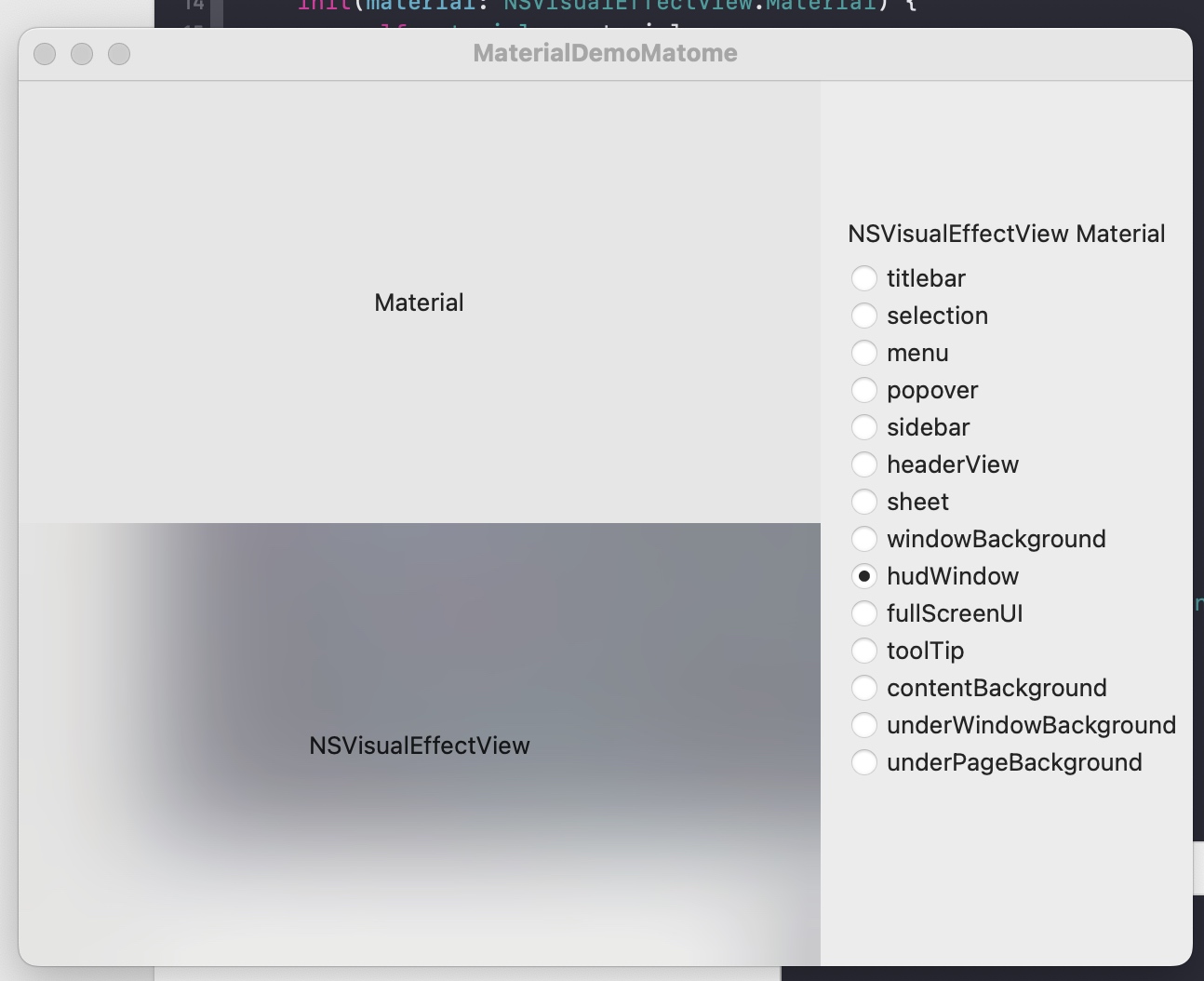
import SwiftUI
@main
struct MaterialDemoMatomeApp: App {
var body: some Scene {
WindowGroup {
ContentView()
.onReceive(NotificationCenter.default.publisher(for: NSWindow.didBecomeKeyNotification)) { notification in
if let window = notification.object as? NSWindow {
window.backgroundColor = NSColor(red: 0, green: 0, blue: 0, alpha: 0.001)
}
}
}
}
}
import SwiftUI
struct BlurView: NSViewRepresentable {
private let material: NSVisualEffectView.Material
init(material: NSVisualEffectView.Material) {
self.material = material
}
func makeNSView(context: Context) -> some NSVisualEffectView {
let view = NSVisualEffectView()
view.material = material
view.blendingMode = .behindWindow
view.state = .active
return view
}
func updateNSView(_ nsView: NSViewType, context: Context) {
nsView.material = material
}
}
struct ContentView: View {
@State private var material: NSVisualEffectView.Material = .hudWindow
var body: some View {
HStack(spacing: 0) {
VStack(spacing: 0) {
ZStack {
Color.clear
.background(.ultraThinMaterial)
Text("Material")
}
ZStack {
Color.clear
.background(BlurView(material: material))
Text("NSVisualEffectView")
}
}
ZStack {
Color(nsColor: .windowBackgroundColor)
VStack {
Text("NSVisualEffectView Material")
Picker("", selection: $material) {
ForEach(NSVisualEffectView.Material.allCasesIncludingName, id: \.self.1) { material in
Text("\(material.1)").tag(material.0)
}
}
.pickerStyle(.radioGroup)
}
}
.frame(width: 200)
.ignoresSafeArea()
}
}
}
#Preview {
ContentView()
}
// MARK: - Helpers
extension NSVisualEffectView.Material {
static var allCasesIncludingName: [(NSVisualEffectView.Material, String)] = [
(.titlebar, "titlebar"),
(.selection, "selection"),
(.menu, "menu"),
(.popover, "popover"),
(.sidebar, "sidebar"),
(.headerView, "headerView"),
(.sheet, "sheet"),
(.windowBackground, "windowBackground"),
(.hudWindow, "hudWindow"),
(.fullScreenUI, "fullScreenUI"),
(.toolTip, "toolTip"),
(.contentBackground, "contentBackground"),
(.underWindowBackground, "underWindowBackground"),
(.underPageBackground, "underPageBackground")
]
}