-
-
Save qmmr/d00a42c3bfb9c71b9395399dfbe3460a to your computer and use it in GitHub Desktop.
""" | |
pirple.com/python | |
Python Homework Assignment #7: Dictionaries and Sets | |
Details: | |
Return to your first homework assignments, when you described your favorite song. | |
Refactor that code so all the variables are held as dictionary keys and value. | |
Then refactor your print statements so that it's a single loop that passes through each item in the dictionary | |
and prints out it's key and then it's value. | |
Extra Credit: | |
Create a function that allows someone to guess the value of any key in the dictionary, | |
and find out if they were right or wrong. | |
This function should accept two parameters: Key and Value. | |
If the key exists in the dictionary and that value is the correct value, | |
then the function should return true. | |
In all other cases, it should return false. | |
""" | |
# Song attributes dictionary | |
song = { | |
"title": "We Are the Champions", | |
"album": "News of the World", | |
"artist": "Queen", | |
"genre": "Arena rock", | |
"release year": "1977", | |
"length": "2.59", | |
"length (seconds)": "179", | |
"songwriter": "Freddie Mercury", | |
"producers": "Queen, Mike \"Clay\" Stone" | |
} | |
# Print the info aboute song | |
for key in song: | |
print("{0:s}: \"{1:s}\"".format(key.capitalize(), song[key])) | |
def guess(key, val): | |
return key in song and song[key] == val | |
print("\n\nBonus:\n\n") | |
print("Is the title of this song \"We Are the Champions\"?: {}".format( | |
guess("title", "We Are the Champions"))) | |
print("Is the album name \"News of the World\"?: {}".format( | |
guess("album", "News of the World"))) |
I don't have any flashy input statements but works fine.
I created a dictionary with name Song and key and value pairs are of song Uptown Funk by Bruno Mars.
Song ={"Song_Name":"Uptown Funk","Artist":"Mark Ronson" ,"Featured_Artist":"Bruno Mars",
"Genre":"Funk-pop",
"DurationInSeconds":"270 Seconds","Label":"Columbia",
"Songwriters":"Mark Ronson",
"Producers":"Mark Ronson","Released":"10 November 2014",
"Accolades":"2015, British Single of the Year at the Brit Awards"
}
In the for loop the loop goes through every key in the dict and prints it in the format
for key in Song:
print("{0:s}: "{1:s}"".format(key, Song[key]))
For the extra credit one I created a function with two parameters key&value and used nested if statements to check the conditions.
def guess(key,value):
if key in Song:
if Song[key]==value:
print(True)
else:
print(False)
My was is bit easier I guess. I am not giving user the opportunity to either choose the attribute or guess the attribute but yet it is still working. you must run it for once:-
FavouriteSong = {"Song" : "Se Te Nota",
"Artist" : "Lele Ponz and Guayna",
"Genre" : "Raggae",
"Releasedon" : "October 8, 2020",
"Length" : "2:40"}
for keys in FavouriteSong:
print(keys)
def guessingGame():
while(True):
keys = input("Enter Your Attribute: \n")
if keys in FavouriteSong :
v = input("Now Guess The Respective Value: \n")
elif keys != FavouriteSong or type(keys) == "int":
print("""Attribute does not exist.\nPlease keep the 1st alphabet capital and rest small!\nPlease don't use any number.""")
break
if v == FavouriteSong[keys]:
print("CONGRATULATIONS ! You Guessed It Right !")
break
if v != FavouriteSong[keys]:
print("SORRY ! You Guessed It Wrong, Please Try Again !")
else:
print("Wrong Key")
Thank you, brother.... I am also learning Python from Pirple.com and I am new to coding. Your code really helped me a lot.
def guess(key, val):
if song[key] == val:
return True
else:
return False
this is what i did for it, pretty simple
My was is bit easier I guess. I am not giving user the opportunity to either choose the attribute or guess the attribute but yet it is still working. you must run it for once:-
FavouriteSong = {"Song" : "Se Te Nota",
"Artist" : "Lele Ponz and Guayna",
"Genre" : "Raggae",
"Releasedon" : "October 8, 2020",
"Length" : "2:40"}
for keys in FavouriteSong:
print(keys)
def guessingGame():
while(True):
keys = input("Enter Your Attribute: \n")
if keys in FavouriteSong :
v = input("Now Guess The Respective Value: \n")
elif keys != FavouriteSong or type(keys) == "int":
print("""Attribute does not exist.\nPlease keep the 1st alphabet capital and rest small!\nPlease don't use any number.""")
break
if v == FavouriteSong[keys]:
print("CONGRATULATIONS ! You Guessed It Right !")
break
if v != FavouriteSong[keys]:
print("SORRY ! You Guessed It Wrong, Please Try Again !")
else:
print("Wrong Key")
guessingGame()
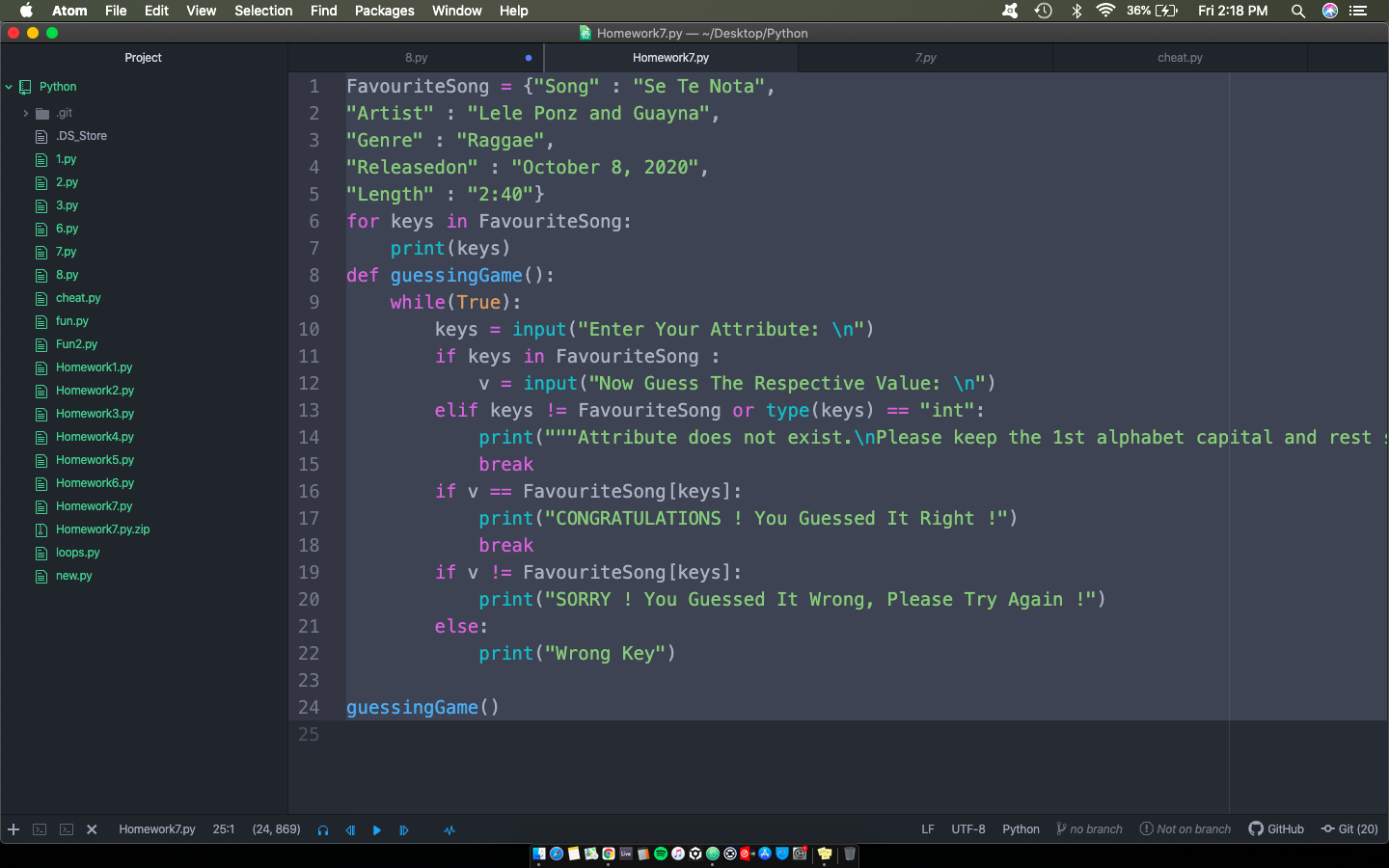