Last active
March 22, 2023 16:22
-
-
Save renatoargh/295bf22d77e38203905d3abd6ea37070 to your computer and use it in GitHub Desktop.
Naive crypto algorithm
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import { randomBytes } from 'crypto' | |
const initCircularBuffer = (buffer: Buffer) => { | |
let index = 0; | |
return (): number => { | |
if (index >= buffer.length) { | |
index = 0; | |
} | |
const byte = buffer.at(index) | |
if (!byte) { | |
throw new Error(`Invalid index ${index}. Expected number between 0 and ${buffer.length - 1}`) | |
} | |
index++; | |
return byte; | |
} | |
} | |
function naiveEncryptOrDecrypt( | |
privateKey: Buffer, | |
plainOrCypher: string | Buffer | |
): Buffer { | |
const getNextByte = initCircularBuffer(privateKey); | |
const plainOrCypherBuffer = Buffer.from(plainOrCypher); | |
const encryptedBuffer = plainOrCypherBuffer | |
.map((byte) => byte ^ getNextByte()) | |
return Buffer.from(encryptedBuffer) | |
} | |
const plaintext = process.argv[2] || 'this is an encryption test'; | |
const privateKey = process.argv[3] ? Buffer.from(process.argv[3], 'base64') : randomBytes(16) | |
const encrypted = naiveEncryptOrDecrypt(privateKey, plaintext) | |
const decrypted = naiveEncryptOrDecrypt(privateKey, encrypted) | |
console.log(JSON.stringify({ | |
plaintext, | |
privateKey: Buffer.from(privateKey).toString('base64'), | |
encrypted: encrypted.toString('base64'), | |
decrypted: decrypted.toString(), | |
match: Buffer.from(plaintext).compare(decrypted) === 0 | |
}, null, 2)) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Based on https://www.crypto101.io/
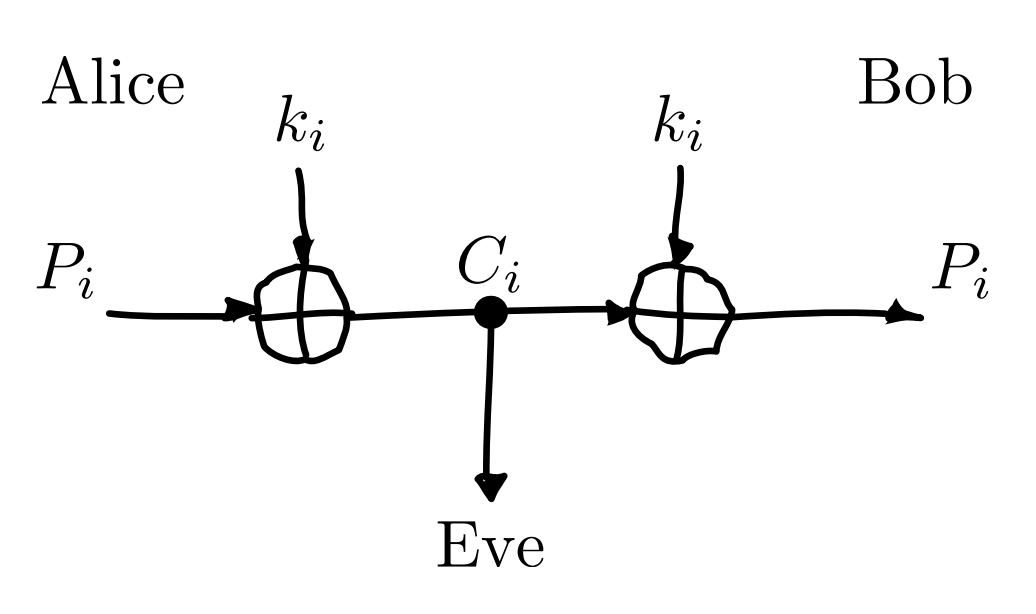