Created
December 21, 2018 14:21
-
-
Save rngtm/241c86c6a5732a43f6f5eeefef55a99f to your computer and use it in GitHub Desktop.
板を1メッシュで大量に生成するC#スクリプト
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
#if UNITY_EDITOR | |
using UnityEditor; | |
/// <summary> | |
/// エディター拡張 | |
/// </summary> | |
[CustomEditor(typeof(GenerateManyPlane))] | |
public class GenerateManyPlaneInspector : Editor | |
{ | |
public override void OnInspectorGUI() | |
{ | |
var generateManyPlane = target as GenerateManyPlane; | |
// 頂点数表示 | |
EditorGUILayout.LabelField("VertexCount", generateManyPlane.VertexCount.ToString()); | |
// メッシュ作成ボタン | |
EditorGUI.BeginDisabledGroup(!EditorApplication.isPlaying); | |
if (GUILayout.Button("Generate Mesh")) | |
{ | |
generateManyPlane.GenerateMesh(); | |
} | |
EditorGUI.EndDisabledGroup(); | |
base.OnInspectorGUI(); | |
} | |
} | |
#endif | |
/// <summary> | |
/// 板を1メッシュで大量に生成するC#スクリプト | |
/// </summary> | |
[RequireComponent(typeof(MeshFilter))] | |
[RequireComponent(typeof(MeshRenderer))] | |
public class GenerateManyPlane : MonoBehaviour | |
{ | |
[SerializeField] bool generateMeshOnUpdate = false; // 毎フレームメッシュを生成するかどうか | |
[SerializeField] int zCount = 16; // z方向のPlaneの個数 | |
[SerializeField] float zSize = 1f; | |
private Mesh mesh; | |
public int VertexCount { get { return zCount * 4; } } // 頂点数 | |
public int TriangleCount { get { return zCount * 6; } } // 頂点インデックス数 | |
void Start() | |
{ | |
mesh = new Mesh(); | |
GetComponent<MeshFilter>().mesh = mesh; | |
GenerateMesh(); | |
} | |
void Update() | |
{ | |
if (generateMeshOnUpdate) | |
{ | |
GenerateMesh(); | |
} | |
} | |
/// <summary> | |
/// メッシュ作成 | |
/// </summary> | |
public void GenerateMesh() | |
{ | |
if (zSize < 0f) { zSize = 0f; } | |
if (zCount < 1) { zCount = 1; } | |
float planeInterval = zSize / zCount; | |
// 頂点(vertices)作成・UV作成 | |
int vi = 0; | |
Vector3[] vertices = new Vector3[VertexCount]; | |
Vector2[] uv = new Vector2[vertices.Length]; | |
Vector3 vertexOffset = new Vector3(); | |
for (int zi = 0; zi < zCount; zi++) | |
{ | |
vertexOffset.z = planeInterval * zi; | |
// 反時計回りに並べる | |
vertices[vi + 0] = new Vector3(+1f, +1f, 0f) + vertexOffset; // 0 | |
vertices[vi + 1] = new Vector3(+1f, -1f, 0f) + vertexOffset; // 1 | |
vertices[vi + 2] = new Vector3(-1f, -1f, 0f) + vertexOffset; // 2 | |
vertices[vi + 3] = new Vector3(-1f, +1f, 0f) + vertexOffset; // 3 | |
uv[vi + 0] = new Vector2(1f, 1f); // 0 | |
uv[vi + 1] = new Vector2(1f, 0f); // 1 | |
uv[vi + 2] = new Vector2(0f, 0f); // 2 | |
uv[vi + 3] = new Vector2(0f, 1f); // 3 | |
vi += 4; | |
} | |
// 頂点インデックス(triangles)作成 | |
int ti = 0; | |
int[] triangles = new int[TriangleCount]; | |
int triangleOffset = 0; | |
for (int zi = 0; zi < zCount; zi++) | |
{ | |
triangles[ti++] = 0 + triangleOffset; | |
triangles[ti++] = 1 + triangleOffset; | |
triangles[ti++] = 2 + triangleOffset; | |
triangles[ti++] = 2 + triangleOffset; | |
triangles[ti++] = 3 + triangleOffset; | |
triangles[ti++] = 0 + triangleOffset; | |
triangleOffset += 4; | |
} | |
mesh.vertices = vertices; | |
mesh.uv = uv; | |
mesh.triangles = triangles; | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
1メッシュで板を大量に生成するC#スクリプトです。
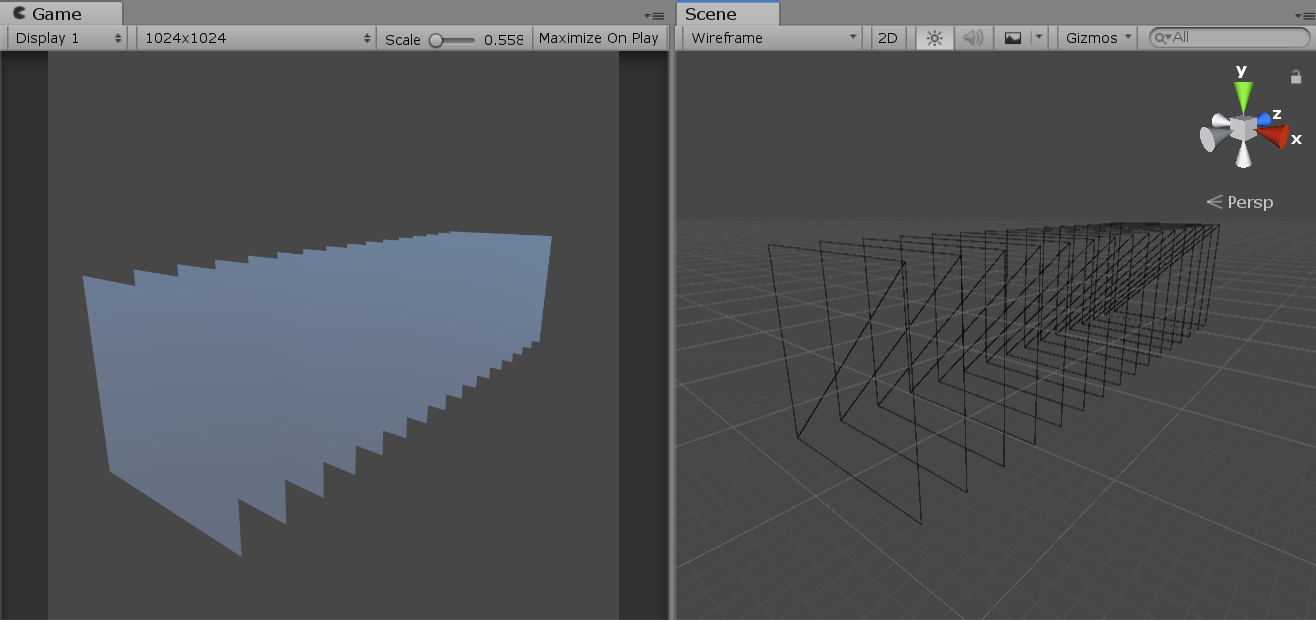