Created
May 14, 2018 12:43
-
-
Save slidenerd/80360e482c3466c137c1498426d4712b to your computer and use it in GitHub Desktop.
JS Dynamic Table Insert Rows Benchmark with different methods
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<head> | |
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.1.1/css/bootstrap.min.css" /> | |
</head> | |
<body> | |
<div id="status"></div> | |
<table id = "data" class="d-none"> | |
</table> | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.4/lodash.js"></script> | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/platform/1.3.4/platform.js"></script> | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/benchmark/2.1.4/benchmark.js"></script> | |
<script src="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.1.1/js/bootstrap.bundle.min.js"></script> | |
<script> | |
var suite = new Benchmark.Suite; | |
// add tests | |
suite | |
// .add('Get Elements By Tag Name', function() { | |
// var table = document.getElementsByTagName("table")[0] | |
// }) | |
// .add('Get Element By Id', function() { | |
// var table = document.getElementById("data") | |
// }) | |
.add('Insert with plain strings', function() { | |
//Get the table | |
var table = document.getElementById("data") | |
var contents = [] | |
//Add a 1000 rows | |
for(var i = 0; i < 1000; i++){ | |
contents.push('<tr>') | |
//Add 10 columns in each row | |
for(var j = 0; j < 10; j++){ | |
contents.push('<td>Data</td>') | |
} | |
contents.push('</tr>') | |
} | |
table.innerHTML = contents.join("") | |
}) | |
.add('Insert with createElement', function() { | |
//Get the table | |
var table = document.getElementById("data") | |
//Add a 1000 rows | |
for(var i = 0; i < 1000; i++){ | |
var newRow = document.createElement('tr') | |
//Add 10 columns in each row | |
for(var j = 0; j < 10; j++){ | |
var newCell = document.createElement('td') | |
// Append a text node to the cell | |
var newText = document.createTextNode('Data'); | |
newCell.appendChild(newText) | |
newRow.appendChild(newCell) | |
} | |
table.appendChild(newRow) | |
} | |
}) | |
.add('Insert with insertRow', function() { | |
var table = document.getElementById("data") | |
for(var i = 0; i < 1000; i++){ | |
// Insert a row in the table at row index 0 | |
var newRow = table.insertRow(i); | |
for(var j = 0; j < 10; j++){ | |
var newCell = newRow.insertCell(j) | |
// Append a text node to the cell | |
var newText = document.createTextNode('Data'); | |
newCell.appendChild(newText); | |
} | |
} | |
}) | |
// add listeners | |
.on('cycle', function(event) { | |
$(document.getElementById('status')).append('<div class="alert alert-primary" role="alert">'+String(event.target)+'</div>') | |
}) | |
.on('complete', function(data) { | |
console.log(this) | |
$(document.getElementById('status')).append('<div class="alert alert-success" role="alert">Fastest method: '+this.filter('fastest').map('name')+'</div>') | |
}) | |
// run async | |
.run({ 'async': true }); | |
$(document.getElementById('status')).append('<div class="alert alert-info" role="alert">Beginning test to find the fastest method to insert rows into dynamic</div>') | |
</script> | |
</body> | |
<!-- | |
--> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
I am aware of 3 options to insert rows dynamically into a JS table.
The fastest method not surprisingly is strings. What does your browser say?
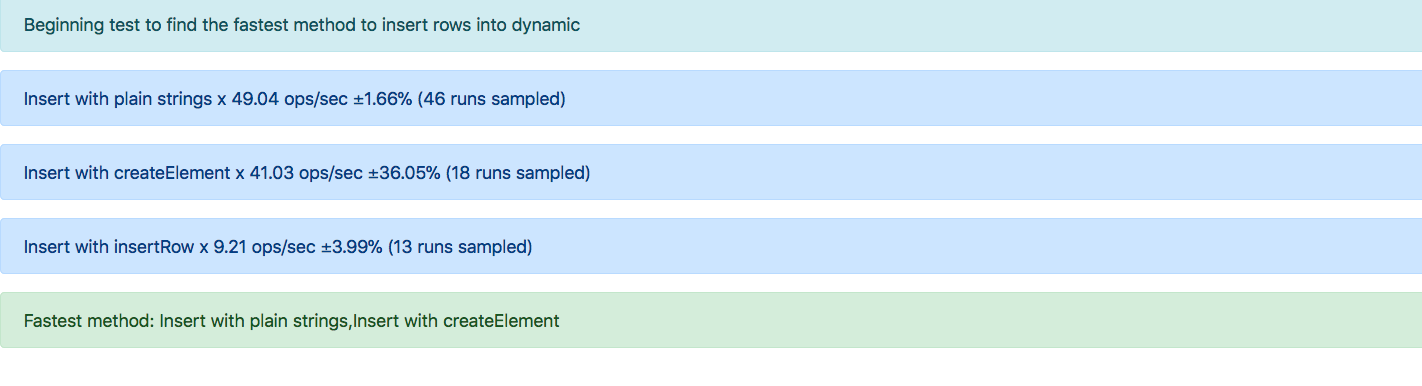