-
-
Save udacityandroid/8c4604af1d6b6afe12d6 to your computer and use it in GitHub Desktop.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" | |
xmlns:tools="http://schemas.android.com/tools" | |
android:layout_width="match_parent" | |
android:layout_height="match_parent" | |
android:background="#B388FF" | |
android:orientation="vertical" | |
tools:context=".MainActivity"> | |
<ImageView | |
android:id="@+id/android_cookie_image_view" | |
android:layout_width="match_parent" | |
android:layout_height="0dp" | |
android:layout_weight="1" | |
android:scaleType="centerCrop" | |
android:src="@drawable/before_cookie" /> | |
<TextView | |
android:id="@+id/status_text_view" | |
android:layout_width="match_parent" | |
android:layout_height="wrap_content" | |
android:layout_marginLeft="16dp" | |
android:layout_marginRight="16dp" | |
android:layout_marginTop="16dp" | |
android:text="I'm so hungry" | |
android:textColor="@android:color/white" | |
android:textSize="34sp" /> | |
<Button | |
android:layout_width="wrap_content" | |
android:layout_height="wrap_content" | |
android:layout_margin="16dp" | |
android:text="EAT COOKIE" /> | |
</LinearLayout> |
package com.example.android.cookies; | |
import android.os.Bundle; | |
import android.support.v7.app.AppCompatActivity; | |
import android.view.View; | |
public class MainActivity extends AppCompatActivity { | |
@Override | |
protected void onCreate(Bundle savedInstanceState) { | |
super.onCreate(savedInstanceState); | |
setContentView(R.layout.activity_main); | |
} | |
/** | |
* Called when the cookie should be eaten. | |
*/ | |
public void eatCookie(View view) { | |
// TODO: Find a reference to the ImageView in the layout. Change the image. | |
// TODO: Find a reference to the TextView in the layout. Change the text. | |
} | |
} |
thanks
hello when i add the : compile 'com.android.support:appcompat-v7:22.1.0'
in
dependencies {
compile 'com.android.support:appcompat-v7:22.1.0'
implementation fileTree(dir: 'libs', include: ['*.jar'])
implementation 'com.android.support:appcompat-v7:27.1.1'
implementation 'com.android.support.constraint:constraint-layout:1.1.0'
testImplementation 'junit:junit:4.12'
androidTestImplementation 'com.android.support.test:runner:1.0.2'
androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2'
}
it shows me this error :
//AAPT2 error: check logs for details
im blocked any solution plz, thanks
This is the code I edited , while I was at it I had my fun , I added a state so whenever the button is pressed it traverse between those two state
state 1- "I m hungry"
state 2- "I m so full"
activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#B388FF"
android:orientation="vertical"
tools:context=".MainActivity">
<ImageView
android:id="@+id/android_cookie_image_view"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:scaleType="centerCrop"
android:src="@drawable/before_cookie" />
<TextView
android:id="@+id/status_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
android:layout_marginTop="16dp"
android:text="I'm so hungry"
android:textColor="@android:color/white"
android:textSize="34sp" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:text="EAT COOKIE"
android:onClick="eatCookie"/>
</LinearLayout>
MainActivity.java
package com.example.android.cookies;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;public class MainActivity extends AppCompatActivity {
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } boolean state=true; /** * Called when the cookie should be eaten. */ public void eatCookie(View view) { if(state==true) { // TODO: Find a reference to the ImageView in the layout. Change the image. ImageView androidCookieImageView = (ImageView) findViewById(R.id.android_cookie_image_view); androidCookieImageView.setImageResource(R.drawable.after_cookie); // TODO: Find a reference to the TextView in the layout. Change the text. TextView statusTextView = (TextView) findViewById(R.id.status_text_view); statusTextView.setText("I'm so full"); } else setContentView(R.layout.activity_main); state = state ^ true; }
}
what the wrong here
cannot resolve symble R in main activity
@LubnaElfarra, Try any of these solutions:
1- Build -> Clean Project
2- Tools -> Android -> Sync Project with Gradle Files
3- Restart Android Studio
I had same issue and a simple project rebuild worked for me.
So go to Build > Rebuild Project and see if it works.
@Sarcares, a button always has only capitals. Therefore, you could even write (eAtcOOkiE), and it would automatically set to all capitals to (EATCOOKIE).
my code is running but after clicking on eat cookie picture does't change
FYI for those using a newer version of Android Studio, you may not even need to include the compile command in later in the project. There should already be a few in the gradle build.
Where can I download the images?
If you have android studio 3.2.1 , you dont need to add Compile code.
you will see that the code is already placed there :
implementation 'com.android.support:appcompat-v7:28.0.0'
This Code is Correct-
No need to Paste the Code in Gradle because we are using newer version of Android.
Name of Project is "cookie".
XML -
<ImageView
android:id="@+id/android_cookie_image_view"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:scaleType="centerCrop"
android:src="@drawable/before_cookie" />
<TextView
android:id="@+id/status_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
android:layout_marginTop="16dp"
android:text="I'm so hungry"
android:textColor="@android:color/white"
android:textSize="34sp" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:text="EAT COOKIE"
android:onClick="eatCookie"/>
JAVA-
package com.example.android.cookie;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
import com.example.android.cookie.R;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
/**
* Called when the cookie should be eaten.
*/
public void eatCookie(View view) {
// TODO: Find a reference to the ImageView in the layout. Change the image.
ImageView beforeCookieImageView = (ImageView) findViewById(R.id.android_cookie_image_view);
beforeCookieImageView.setImageResource(R.drawable.after_cookie);
// TODO: Find a reference to the TextView in the layout. Change the text.
TextView statusInitialTextView = (TextView) findViewById(R.id.status_text_view);
statusInitialTextView.setText("I am Totally full");
}
}
Wow, I think I'm getting a hold on Logging. so sweet
There is no android:text parameter set in Button
implementation 'com.android.support:appcompat-v7:28.0.0'
Implementation has replaced the compile in the dependencies for gradle modular
this works for me ^_^ ( i didnt need to replace the v7 thing )
xml code here..
<ImageView
android:id="@+id/android_cookie_image_view"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:scaleType="centerCrop"
android:src="@drawable/before_cookie" />
<TextView
android:id="@+id/status_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
android:layout_marginTop="16dp"
android:text="@string/i_m_so_hungry"
android:textColor="@android:color/white"
android:textSize="34sp" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:text="@string/eat_cookie"
android:onClick="eatCookie"/>
and main activity..
package com.example.cookies;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate ( savedInstanceState );
setContentView ( R.layout.activity_main );
}
/**
* Called when the cookie should be eaten.
*/
public void eatCookie(View view) {
// TODO: Find a reference to the ImageView in the layout. Change the image.
ImageView beforeCookieImageVIew = (ImageView) findViewById ( R.id.android_cookie_image_view );
beforeCookieImageVIew.setImageResource ( R.drawable.after_cookie );
// TODO: Find a reference to the TextView in the layout. Change the text.
TextView statusTextView = (TextView) findViewById ( R.id.status_text_view );
statusTextView.setText ( "I am so full!" );
}
}
Important ( Do not forget to move the images into the drawable folder first or you will have nothing to show)
Can anyone here tell what is this error?
09-04 09:58:06.402 4775-4775/com.example.android.cookies W/ResourceType: Failure getting entry for 0x7f060056 (t=5 e=86) (error -75)
09-04 09:58:06.404 4775-4775/com.example.android.cookies D/AndroidRuntime: Shutting down VM
09-04 09:58:06.430 4775-4775/com.example.android.cookies E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.android.cookies, PID: 4775
java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.android.cookies/com.example.android.cookies.MainActivity}: android.view.InflateException: Binary XML file line #11: Binary XML file line #11: Error inflating class ImageView
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2416)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2476)
at android.app.ActivityThread.-wrap11(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1344)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5417)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616)
Caused by: android.view.InflateException: Binary XML file line #11: Binary XML file line #11: Error inflating class ImageView
at android.view.LayoutInflater.inflate(LayoutInflater.java:539)
at android.view.LayoutInflater.inflate(LayoutInflater.java:423)
at android.view.LayoutInflater.inflate(LayoutInflater.java:374)
at androidx.appcompat.app.AppCompatDelegateImpl.setContentView(AppCompatDelegateImpl.java:469)
at androidx.appcompat.app.AppCompatActivity.setContentView(AppCompatActivity.java:140)
at com.example.android.cookies.MainActivity.onCreate(MainActivity.java:16)
at android.app.Activity.performCreate(Activity.java:6237)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1107)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2369)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2476)
at android.app.ActivityThread.-wrap11(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1344)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5417)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616)
Caused by: android.view.InflateException: Binary XML file line #11: Error inflating class ImageView
at android.view.LayoutInflater.createViewFromTag(LayoutInflater.java:782)
at android.view.LayoutInflater.createViewFromTag(LayoutInflater.java:704)
at android.view.LayoutInflater.rInflate(LayoutInflater.java:835)
at android.view.LayoutInflater.rInflateChildren(LayoutInflater.java:798)
at android.view.LayoutInflater.inflate(LayoutInflater.java:515)
at android.view.LayoutInflater.inflate(LayoutInflater.java:423)
at android.view.LayoutInflater.inflate(LayoutInflater.java:374)
at androidx.appcompat.app.AppCompatDelegateImpl.setContentView(AppCompatDelegateImpl.java:469)
at androidx.appcompat.app.AppCompatActivity.setContentView(AppCompatActivity.java:140)
at com.example.android.cookies.MainActivity.onCreate(MainActivity.java:16)
at android.app.Activity.performCreate(Activity.java:6237)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1107)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2369)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2476)
at android.app.ActivityThread.-wrap11(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1344)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5417)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616)
Caused by: android.content.res.Resources$NotFoundException: Resource "com.example.android.cookies:drawable/before_cookie" (7f060056) is not a Drawable (color or path): TypedValue{t=0x1/d=0x7f060056 a=-1 r=0x7f060056}
at android.content.res.Resources.loadDrawableForCookie(Resources.java:2602)
at android.content.res.Resources.loadDrawable(Resources.java:2540)
at android.content.res.TypedArray.getDrawable(TypedArray.java:870)
at android.widget.ImageView.(ImageView.java:152)
at android.widget.ImageView.(ImageView.java:140)
at androidx.appcompat.widget.AppCompatImageView.(AppCompatImageView.java:72)
at androidx.appcompat.widget.AppCompatImageView.(AppCompatImageView.java:68)
at androidx.appcompat.app.AppCompatViewInflater.createImageView(AppCompatViewInflater.java:182)
at androidx.appcompat.app.AppCompatViewInflater.createView(AppCompatViewInflater.java:106)
at androidx.appcompat.app.AppCompatDelegateImpl.createView(AppCompatDelegateImpl.java:1266)
at androidx.appcompat.app.AppCompatDelegateImpl.onCreateView(AppCompatDelegateImpl.java:1316)
at android.view.LayoutInflater.createViewFromTag(LayoutInflater.java:746)
at android.view.LayoutInflater.createViewFromTag(LayoutInflater.java:704)
at android.view.LayoutInflater.rInflate(LayoutInflater.java:835)
at android.view.LayoutInflater.rInflateChildren(LayoutInflater.java:798)
at android.view.LayoutInflater.inflate(LayoutInflater.java:515)
at android.view.LayoutInflater.inflate(LayoutInflater.java:423)
at android.view.LayoutInflater.inflate(LayoutInflater.java:374)
at androidx.appcompat.app.AppCompatDelegateImpl.setContentView(AppCompatDelegateImpl.java:469)
at androidx.appcompat.app.AppCompatActivity.setContentView(AppCompatActivity.java:140)
at com.example.android.cookies.MainActivity.onCreate(MainActivity.java:16)
at android.app.Activity.performCreate(Activity.java:6237)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1107)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2369)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2476)
at android.app.ActivityThread.-wrap11(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1344)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5417)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616)
09-04 09:58:17.545 4775-4775/com.example.android.cookies I/Process: Sending signal. PID: 4775 SIG: 9
compile 'com.android.support:appcompat-v7:22.1.0'
should be
implementation 'androidx.appcompat:appcompat:1.1.0-rc01'
thanks @alicechen15
please anyone explain step 2
Add Gradle Dependency
Why we are adding compile 'com.android.support:appcompat-v7:22.1.0' this code to Gradle
and what is it's effect on app.
in android studio 202.3.1, for app's build.gradle file, use the following:
implementation 'androidx.appcompat:appcompat:1.1.0-rc01'
@Kgarmel Thank you!
@Kgarmel Thank you.
@xMagicXs thanks bro.
Here is My XML Code
<ImageView
android:id="@+id/android_cookie_image_view"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:scaleType="centerCrop"
android:src="@drawable/before_cookie" />
<TextView
android:id="@+id/status_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
android:layout_marginTop="16dp"
android:text="I'm so hungry"
android:textColor="@android:color/white"
android:textSize="34sp" />
=====================================================
Here is My Java Code
package com.shaalle.cookies;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
ImageView cookieImageView;
TextView statusTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
/**
* Called when the cookie should be eaten.
*/
public void eatCookie(View view) {
// TODO: Find a reference to the ImageView in the layout. Change the image.
cookieImageView = (ImageView) findViewById(R.id.android_cookie_image_view);
cookieImageView.setImageResource(R.drawable.after_cookie);
// TODO: Find a reference to the TextView in the layout. Change the text.
statusTextView = (TextView) findViewById(R.id.status_text_view);
statusTextView.setText("I'm so full");
}
/**
* Resets the cookie to it's default state.
*/
public void reset(View view) {
// TODO: Find a reference to the ImageView in the layout. Change the image.
cookieImageView = (ImageView) findViewById(R.id.android_cookie_image_view);
cookieImageView.setImageResource(R.drawable.before_cookie);
// TODO: Find a reference to the TextView in the layout. Change the text.
statusTextView = (TextView) findViewById(R.id.status_text_view);
statusTextView.setText("I'm so hungry");
}
}
Here is the app screenshots
activity_main.xml
<ImageView
android:id="@+id/android_cookie_image_view"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:scaleType="centerCrop"
android:src="@drawable/before_cookie" />
<TextView
android:id="@+id/status_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
android:layout_marginTop="16dp"
android:text="I'm so hungry"
android:textColor="@android:color/white"
android:textSize="34sp" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:layout_weight="1"
android:onClick="eatCookie"
android:text="EAT COOKIE" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:layout_weight="1"
android:onClick="goWc"
android:text="GO WC" />
</LinearLayout>
MainActivity.java
package com.example.cookies;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
/**
* Called when the cookie should be eaten.
*/
public void eatCookie(View view) {
// TODO: Find a reference to the ImageView in the layout. Change the image.
ImageView bciImageView = (ImageView) findViewById(R.id.android_cookie_image_view);
bciImageView.setImageResource(R.drawable.after_cookie);
// TODO: Find a reference to the TextView in the layout. Change the text.
TextView tiTextView = (TextView) findViewById(R.id.status_text_view);
tiTextView.setText("I'm so full");
}
public void goWc (View view){
// TODO: Find a reference to the ImageView in the layout. Change the image.
ImageView bciImageView = (ImageView) findViewById(R.id.android_cookie_image_view);
bciImageView.setImageResource(R.drawable.before_cookie);
// TODO: Find a reference to the TextView in the layout. Change the text.
TextView tiTextView = (TextView) findViewById(R.id.status_text_view);
tiTextView.setText("Ohhhh, amazing");
}
}
Screenshots:
just felt like to re-strategize by adding a RESET button to return to previous xml "I'm so Hungry" from "I'm so full
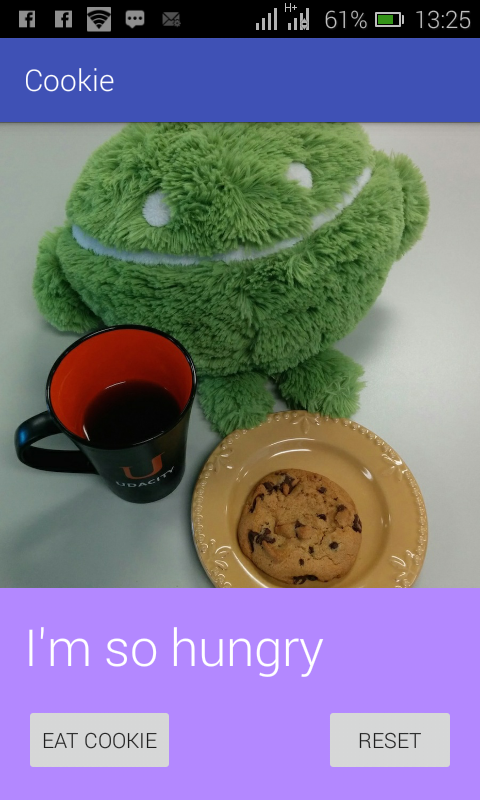
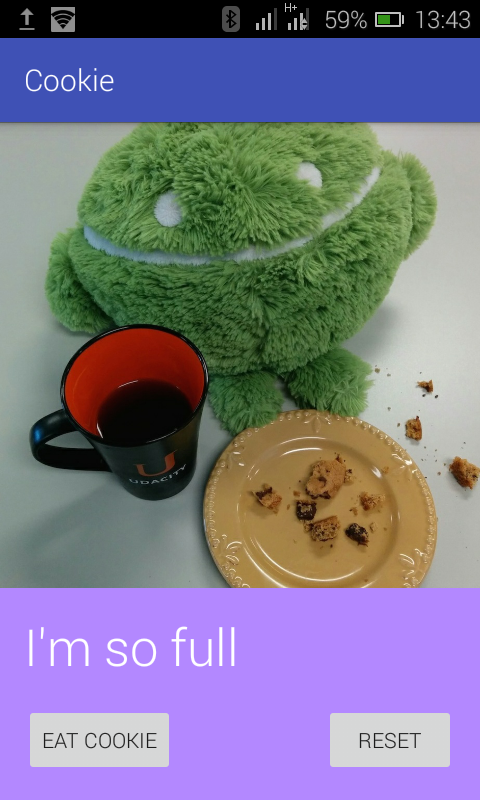
"