Created
January 21, 2023 12:44
-
-
Save unrealwill/07069676a577f6dfbc35553696869798 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
//Add this to the browser console of https://www.funnyhowtheknightmoves.com/ | |
/** | |
* Creates line element on given position, with given length and angle. | |
*/ | |
function createLineElement(x, y, length, angle) { | |
var line = document.createElement("div"); | |
var styles = 'border: 1px solid black; ' | |
+ 'width: ' + length + 'px; ' | |
+ 'height: 0px; ' | |
+ '-moz-transform: rotate(' + angle + 'rad); ' | |
+ '-webkit-transform: rotate(' + angle + 'rad); ' | |
+ '-o-transform: rotate(' + angle + 'rad); ' | |
+ '-ms-transform: rotate(' + angle + 'rad); ' | |
+ 'position: absolute; ' | |
+ 'top: ' + y + 'px; ' | |
+ 'left: ' + x + 'px; '; | |
line.setAttribute('style', styles); | |
return line; | |
} | |
function createCircleDiv(x,y,r) | |
{ | |
var dot = document.createElement("div"); | |
var styles = "height: " + 2*r +"px;" | |
+ "width: " + 2*r +"px;" | |
+ "background-color:#00ff00;" | |
+ "border-radius:50%;" | |
+ "position: absolute;" | |
+ "top: " + (y -r) + "px;" | |
+ "left: " + (x - r) + "px;"; | |
dot.setAttribute("style",styles); | |
return dot; | |
} | |
/** | |
* Creates element which represents line from point [x1, y1] to [x2, y2]. | |
* It is html div element with minimal height and width corresponding to line length. | |
* It's position and rotation is calculated below. | |
*/ | |
function createLine(x1, y1, x2, y2) { | |
var a = x1 - x2, | |
b = y1 - y2, | |
c = Math.sqrt(a * a + b * b); | |
var sx = (x1 + x2) / 2, | |
sy = (y1 + y2) / 2; | |
var x = sx - c / 2, | |
y = sy; | |
var alpha = Math.PI - Math.atan2(-b, a); | |
return createLineElement(x, y, c, alpha); | |
} | |
// Draw some lines | |
function getBoardCase(id) | |
{ | |
let board = document.querySelector('div.m-auto'); | |
return board.querySelector('[data-squareid="'+id+'"]'); | |
} | |
function getBoardCaseCenterCoord(id) | |
{ | |
let bcase = getBoardCase(id).getBoundingClientRect(); | |
return [bcase.left+bcase.width/2,bcase.top+bcase.height/2] | |
} | |
function drawLineBetweenBoardCases(id1, id2) | |
{ | |
let xy1 = getBoardCaseCenterCoord(id1); | |
let xy2 = getBoardCaseCenterCoord(id2); | |
document.body.appendChild(createLine(xy1[0], xy1[1], xy2[0], xy2[1])); | |
} | |
let forbidden = ["d8", "d7", "d6","d5","d4", "d3", "d2", "d1", "e6", "f7", "g8", "c6", "b7", "a8", "c5", "b5", "a5", "e5", "f5", "g5", "h5", "c4", "b3", "a2", "e4", "f3", "g2", "h1"]; | |
function buildAllGraph() | |
{ | |
let letters = ["a","b","c","d","e","f","g","h"]; | |
let numbers = ["1","2","3","4","5","6","7","8"]; | |
let knightoffset = [[2,1],[1,2],[-1,-2],[-2,-1],[-1,2],[2,-1],[-2,1],[1,-2]]; | |
let ids = []; | |
for( i = 0 ; i < 8 ; i++) | |
{ | |
for( j = 0 ; j < 8 ; j++) | |
{ | |
id = letters[i]+numbers[j]; | |
if( forbidden.includes(id ) ) continue; | |
for( k = 0 ; k < knightoffset.length ; k++) | |
{ | |
nx = i+knightoffset[k][0]; | |
ny = j+knightoffset[k][1]; | |
if( nx < 0 || ny < 0 || nx > 7 || ny > 7) continue; | |
nid = letters[nx]+numbers[ny]; | |
if( forbidden.includes(nid ) ) continue; | |
ids.push([id,nid]); | |
} | |
} | |
} | |
return ids; | |
} | |
//Making the forbidden cases red | |
((sq) => { let board = document.querySelector('div.m-auto'); sq.forEach(s => board.querySelector('[data-squareid="'+s+'"]').style.backgroundColor='red'); })(forbidden) | |
var lines =buildAllGraph(); | |
//Display nodes | |
for( i = 0 ; i < lines.length ; i++) | |
{ | |
coord = getBoardCaseCenterCoord( lines[i][0] ); | |
document.body.appendChild(createCircleDiv(coord[0],coord[1],10) ); | |
} | |
//Display edges | |
for( i = 0 ; i < lines.length ; i++) | |
{ | |
drawLineBetweenBoardCases(lines[i][0],lines[i][1]); | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Additional interesting visualization found in the comments :
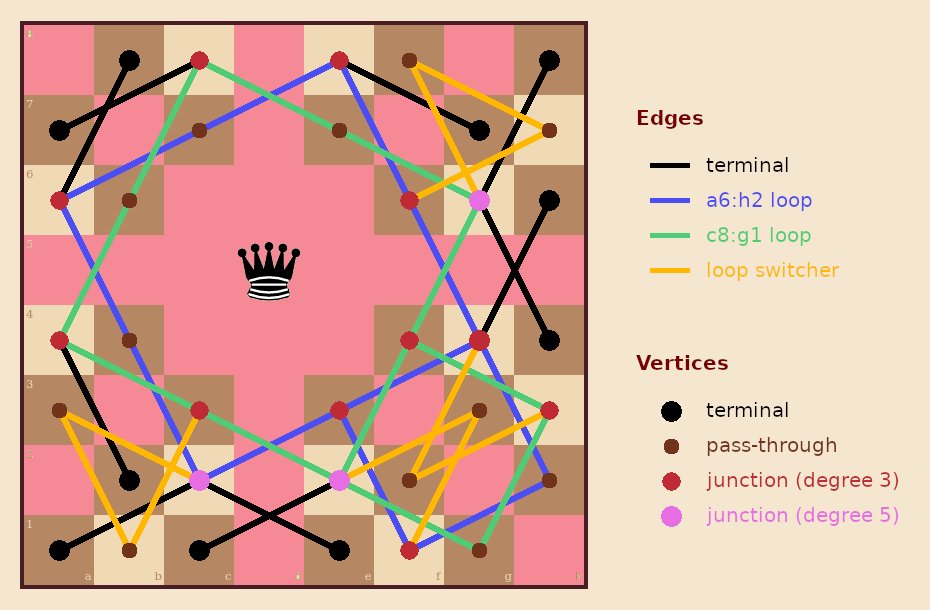
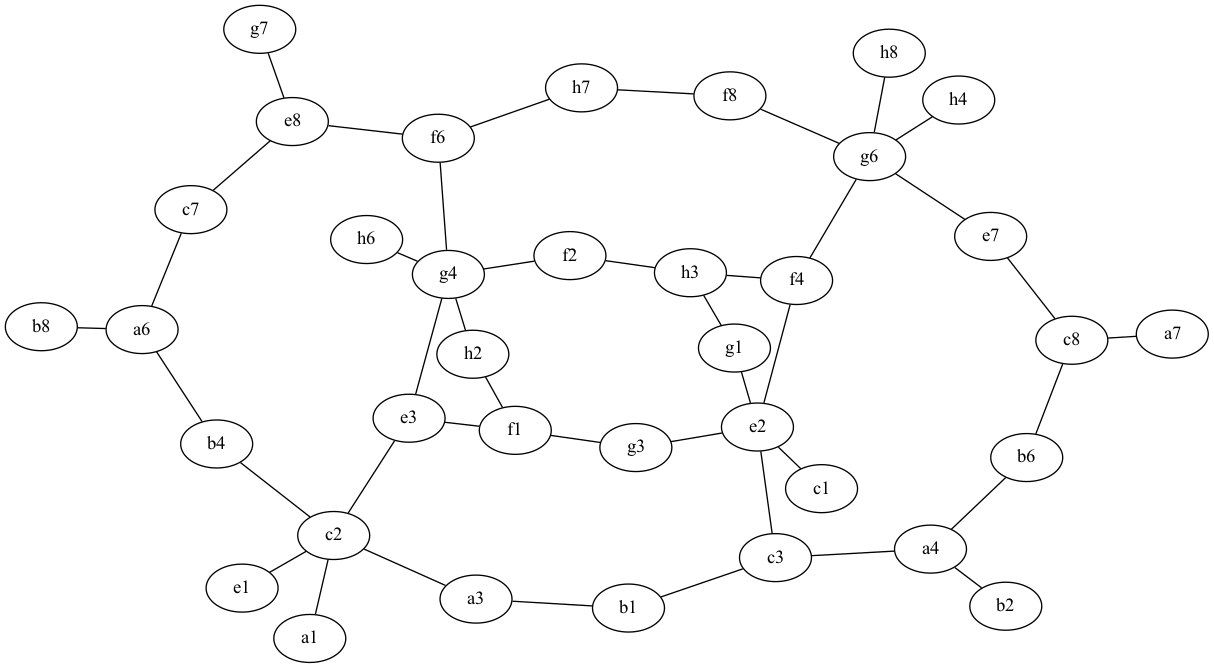
https://news.ycombinator.com/item?id=34462822 :
https://news.ycombinator.com/item?id=34464240 :