Last active
July 2, 2019 17:11
-
-
Save vanderlin/1c2a6c3cbe1612596c4bbe491e671693 to your computer and use it in GitHub Desktop.
using a shader and texture OF+iOS
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include "ofApp.h" | |
int main() { | |
// here are the most commonly used iOS window settings. | |
//------------------------------------------------------ | |
ofiOSWindowSettings settings; | |
settings.enableRetina = true; // enables retina resolution if the device supports it. | |
settings.enableDepth = false; // enables depth buffer for 3d drawing. | |
settings.enableAntiAliasing = false; // enables anti-aliasing which smooths out graphics on the screen. | |
settings.numOfAntiAliasingSamples = 0; // number of samples used for anti-aliasing. | |
settings.enableHardwareOrientation = false; // enables native view orientation. | |
settings.enableHardwareOrientationAnimation = false; // enables native orientation changes to be animated. | |
settings.glesVersion = OFXIOS_RENDERER_ES2; // type of renderer to use, ES1, ES2, ES3 | |
settings.windowControllerType = ofxiOSWindowControllerType::GL_KIT; // Window Controller Type | |
settings.colorType = ofxiOSRendererColorFormat::RGBA8888; // color format used default RGBA8888 | |
settings.depthType = ofxiOSRendererDepthFormat::DEPTH_NONE; // depth format (16/24) if depth enabled | |
settings.stencilType = ofxiOSRendererStencilFormat::STENCIL_NONE; // stencil mode | |
settings.windowMode = OF_FULLSCREEN; | |
ofCreateWindow(settings); | |
return ofRunApp(new ofApp); | |
} |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include "ofApp.h" | |
//-------------------------------------------------------------- | |
void ofApp::setup(){ | |
const std::string vertex = STRINGIFY( | |
attribute vec3 position; | |
attribute vec4 color; | |
attribute vec2 texcoord; | |
varying vec2 coord; | |
void main() { | |
// center | |
coord = position.xy * 0.5 + 0.5; | |
gl_Position = vec4(position, 1.0); | |
} | |
); | |
const std::string fragment = STRINGIFY( | |
precision highp float; | |
uniform sampler2D tex; | |
uniform vec2 u_resolution; | |
uniform vec2 u_mouse; | |
varying vec2 coord; | |
void main() { | |
vec2 uv = coord; | |
vec4 color = texture2D(tex, uv); | |
gl_FragColor = color; | |
} | |
); | |
shader.setupShaderFromSource(GL_VERTEX_SHADER, vertex); | |
shader.setupShaderFromSource(GL_FRAGMENT_SHADER, fragment); | |
shader.bindDefaults(); | |
shader.linkProgram(); | |
int w = ofGetWidth(); | |
int h = ofGetHeight(); | |
printf("allocated texture %ix%i\n", w, h); | |
// allocate tex size of screen | |
texture.allocate(w, h, GL_RGBA); | |
// make some noise and load to texture | |
ofPixels pixels; | |
pixels.allocate(w, h, OF_IMAGE_COLOR_ALPHA); | |
for(int x=0; x<w; x++) { | |
for(int y=0; y<h; y++) { | |
pixels.setColor(x, y, ofColor(ofRandom(0, 255))); | |
} | |
} | |
texture.loadData(pixels); | |
} | |
//-------------------------------------------------------------- | |
void ofApp::update(){ | |
} | |
//-------------------------------------------------------------- | |
void ofApp::draw(){ | |
int w = ofGetWidth(); | |
int h = ofGetHeight(); | |
ofPlanePrimitive plane; | |
plane.set(w, h); | |
shader.begin(); | |
shader.setUniformTexture("depthTex", texture, 0); | |
shader.setUniform2f("u_resolution", w, h); | |
shader.setUniform2f("u_mouse", ofMap(ofGetMouseX(), 0, w, 0.0, 1.0, true), ofMap(ofGetMouseY(), 0, h, 0.0, 1.0, true)); | |
ofPushMatrix(); | |
ofTranslate(w / 2, h / 2); | |
plane.draw(); | |
ofPopMatrix(); | |
shader.end(); | |
// if (ofGetMousePressed()) { | |
// ofSetColor(255); | |
// texture.draw(0, 0); | |
// } | |
} | |
//-------------------------------------------------------------- | |
void ofApp::exit(){ | |
} | |
//-------------------------------------------------------------- | |
void ofApp::touchDown(ofTouchEventArgs & touch){ | |
} | |
//-------------------------------------------------------------- | |
void ofApp::touchMoved(ofTouchEventArgs & touch){ | |
} | |
//-------------------------------------------------------------- | |
void ofApp::touchUp(ofTouchEventArgs & touch){ | |
} | |
//-------------------------------------------------------------- | |
void ofApp::touchDoubleTap(ofTouchEventArgs & touch){ | |
} | |
//-------------------------------------------------------------- | |
void ofApp::touchCancelled(ofTouchEventArgs & touch){ | |
} | |
//-------------------------------------------------------------- | |
void ofApp::lostFocus(){ | |
} | |
//-------------------------------------------------------------- | |
void ofApp::gotFocus(){ | |
} | |
//-------------------------------------------------------------- | |
void ofApp::gotMemoryWarning(){ | |
} | |
//-------------------------------------------------------------- | |
void ofApp::deviceOrientationChanged(int newOrientation){ | |
} |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#pragma once | |
#include "ofxiOS.h" | |
#define STRINGIFY(A) #A | |
class ofApp : public ofxiOSApp { | |
public: | |
void setup(); | |
void update(); | |
void draw(); | |
void exit(); | |
void touchDown(ofTouchEventArgs & touch); | |
void touchMoved(ofTouchEventArgs & touch); | |
void touchUp(ofTouchEventArgs & touch); | |
void touchDoubleTap(ofTouchEventArgs & touch); | |
void touchCancelled(ofTouchEventArgs & touch); | |
void lostFocus(); | |
void gotFocus(); | |
void gotMemoryWarning(); | |
void deviceOrientationChanged(int newOrientation); | |
ofShader shader; | |
ofTexture texture; | |
}; | |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Getting this bug?
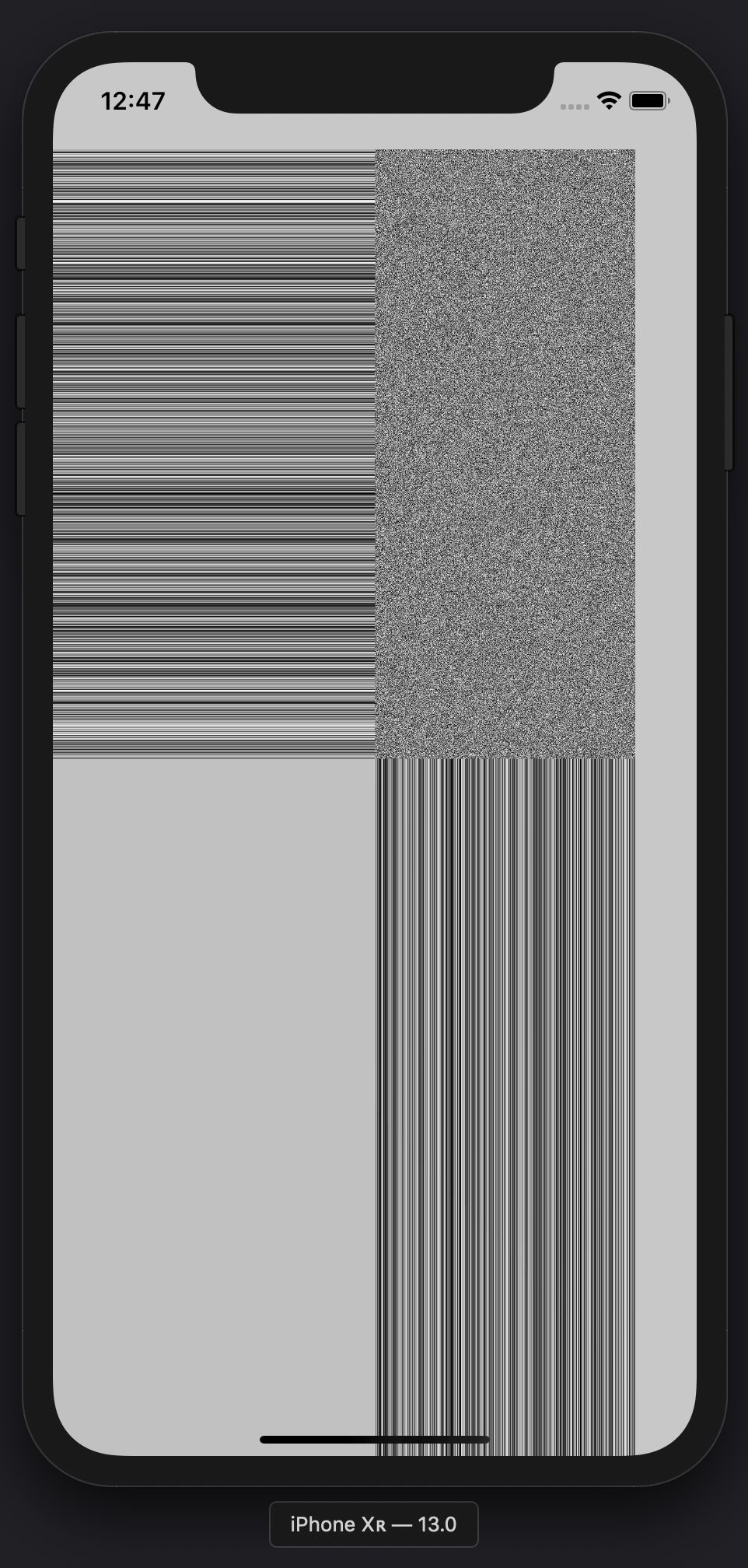