Created
February 13, 2023 12:53
-
-
Save wuuconix/957adc7a11b98fb930e45a8c3645e490 to your computer and use it in GitHub Desktop.
using AbortController to abort a fetch
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const controller = new AbortController() | |
setTimeout(() => { | |
if (res.body.locked) { // body is locked means still reading | |
console.log("Two seconds passed. It's time to abort this slow fetch") | |
controller.abort() | |
} else { | |
console.log("Wow, you have get all body. So fast!") | |
} | |
}, 2000) | |
const res = await fetch("http://oss.wuuconix.link:2023/98764160_p0.png", { signal: controller.signal }) | |
const reader = res.body.getReader() | |
let bytesRead = 0, bytesTotal = res.headers.get("content-length") | |
try { | |
while (true) { | |
const { value, done } = await reader.read() | |
if (value) { | |
bytesRead += value.length | |
console.log(`${(bytesRead / 1000).toFixed(2)}KB / ${(bytesTotal / 1000).toFixed(2)}KB ${(bytesRead / bytesTotal).toFixed(2)}%`) | |
} else if (done) { | |
reader.releaseLock() // reader releastLock which make the body.locked turn from true to false | |
break | |
} | |
} | |
} catch(e) { | |
console.log(String(e)) | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
fetch big images:
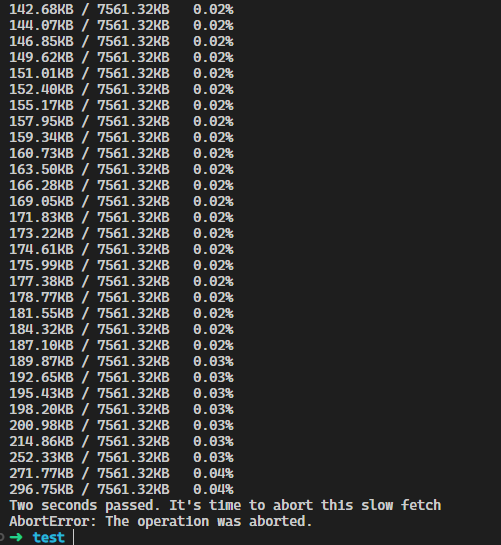
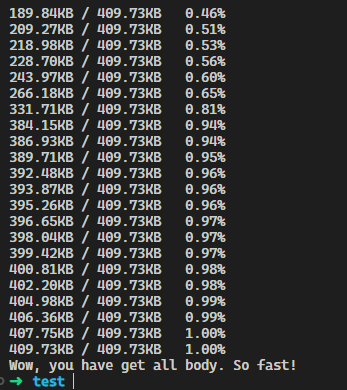
fetch small images: