Last active
March 16, 2023 07:57
-
-
Save xCyborg/2923ce9e57e65af9d417706dda4db4be to your computer and use it in GitHub Desktop.
Unity URP Custom Lit ShaderGraph - BlinnPhong (Wire in the inputs, then add Diffuse + Specular)
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#ifndef CUSTOM_LIGHTING_INCLUDED | |
#define CUSTOM_LIGHTING_INCLUDED | |
/* IN(5): SpecColor(4), Smoothness(1), WPos(3), WNormal(3), WView(3) */ | |
/* OUT(2): Diffuse(3), Specular(3) NdotL(1) for toon ramp: point fltr +clamp mode */ | |
void CalculateLights_half(half4 SpecColor, half Smoothness, half3 WPos, half3 WNormal, half3 WView, | |
out half3 Diffuse, out half3 Specular, out half NdotL) | |
{ | |
Diffuse = 0; | |
Specular = 0; | |
NdotL = 0; | |
#ifndef SHADERGRAPH_PREVIEW | |
Smoothness = exp2(10 * Smoothness + 1); // WNormal = normalize(WNormal); WView = SafeNormalize(WView); | |
Light light = GetMainLight(); // Main Pixel Light | |
half3 attenCol = light.color * light.distanceAttenuation * light.shadowAttenuation; | |
Diffuse = LightingLambert(attenCol, light.direction, WNormal); /* LAMBERT */ | |
Specular = LightingSpecular(attenCol, light.direction, WNormal, WView, SpecColor, Smoothness); /*Blinn-Phong*/ | |
NdotL = dot(light.direction, WNormal) * 0.5 + 0.5; // NdotL [-1..1] normalized [0..1] for toon ramp | |
#endif | |
} | |
#endif | |
/* //---\\ADDITIONAL LIGHTS NEARBY//---\\ | |
int pixelLightCount = GetAdditionalLightsCount(); | |
for (int i = 0; i < pixelLightCount; ++i) | |
{ | |
light = GetAdditionalLight(i, WPos); | |
attenCol = light.color * light.distanceAttenuation * light.shadowAttenuation; | |
Diffuse += LightingLambert(attenCol, light.direction, WNormal); | |
Specular += LightingSpecular(attenCol, light.direction, WNormal, WView, SpecColor, Smoothness); | |
} | |
// --- RUDIMENTARY SHADOWs Keywords: _MAIN_LIGHT_SHADOWS_CASCADE && _SHADOWS_SOFT --- \\ | |
float4 shadowCoord = TransformWorldToShadowCoord(WPos); | |
float shadowAtten = SampleShadowmap(shadowCoord, TEXTURE2D_ARGS(_MainLightShadowmapTexture, sampler_MainLightShadowmapTexture), | |
GetMainLightShadowSamplingData(), GetMainLightShadowStrength(), false); | |
*/ | |
/*///////////////////////////////////////////////////////////////////////////// | |
// ShaderLibrary Lambert and Phong lighting functions : in Lighting.hlsl // | |
// Lighting Functions + PBL // | |
/////////////////////////////////////////////////////////////////////////////// | |
half3 LightingLambert(half3 lightColor, half3 lightDir, half3 normal) | |
{ | |
half NdotL = saturate(dot(normal, lightDir)); | |
return lightColor * NdotL; | |
} | |
half3 LightingSpecular(half3 lightColor, half3 lightDir, half3 normal, half3 viewDir, half4 specular, half smoothness) | |
{ | |
float3 halfVec = SafeNormalize(float3(lightDir)+float3(viewDir)); | |
half NdotH = saturate(dot(normal, halfVec)); | |
half modifier = pow(NdotH, smoothness); | |
half3 specularReflection = specular.rgb * modifier; | |
return lightColor * specularReflection; | |
} | |
half3 LightingPhysicallyBased(BRDFData brdfData, Light light, half3 normalWS, half3 viewDirectionWS) | |
{ | |
half NdotL = saturate(dot(normalWS, light.direction)); | |
half3 radiance = lightColor * (lightAttenuation * NdotL); | |
half3 brdf = brdfData.diffuse; | |
.... | |
return brdf * radiance; | |
}*/ |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Gradual Ramps. (Diffuse + Stepped Spec) * Ramp
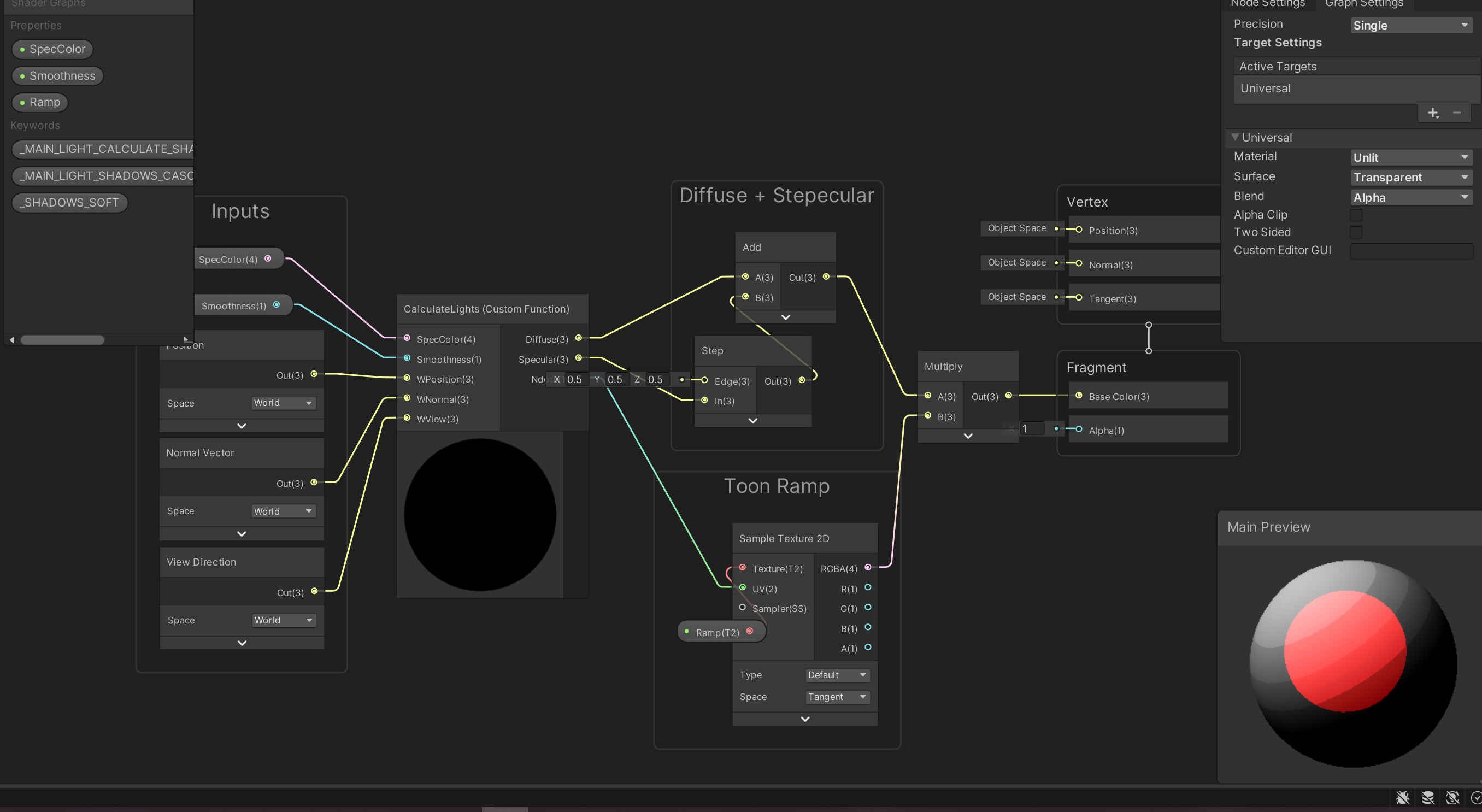